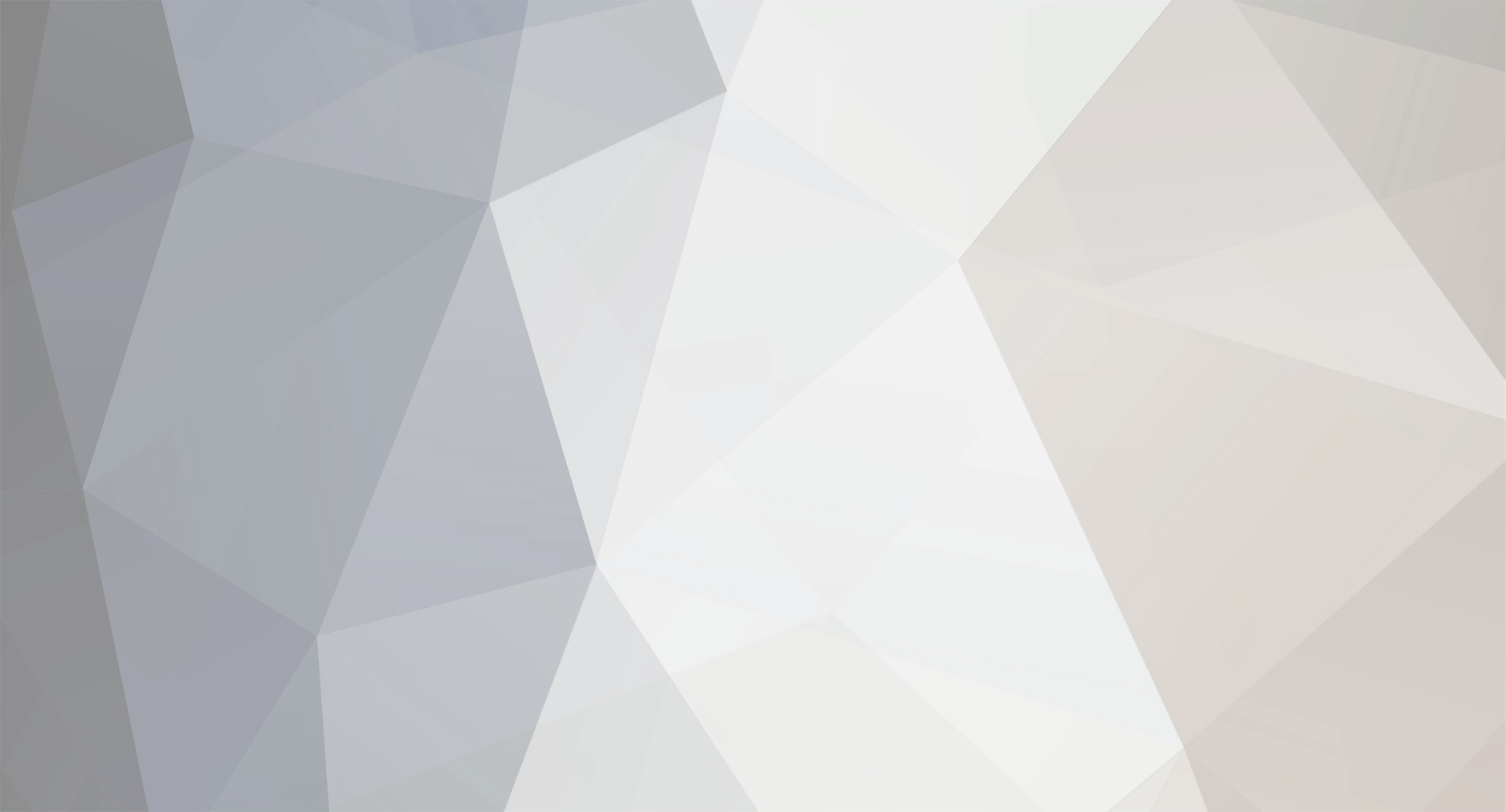
Briggybros
Members-
Posts
155 -
Joined
-
Last visited
Everything posted by Briggybros
-
Thanks, this worked perfectly
-
Hello, I'm trying to use a class that implements IExtendedEntityProperties to add a currency to the player. I initialize the class when the player entity is constructed like so: @ForgeSubscribe(priority = EventPriority.NORMAL) public void onEntityConstuct(EntityConstructing event) { if (event.entity instanceof EntityPlayerMP) { EntityPlayerMP ep = (EntityPlayerMP) event.entity; ep.registerExtendedProperties(PlayerProperties.IDENTIFIER, new PlayerProperties()); } } The PlayerProperties class looks like so: public class PlayerProperties implements IExtendedEntityProperties { public static final String IDENTIFIER = "playerproperties"; public static PlayerProperties fromPlayer(EntityPlayerMP player) { return (PlayerProperties) player.getExtendedProperties(IDENTIFIER); } int credits = 0; EntityPlayerMP player; @Override public void saveNBTData(NBTTagCompound compound) { compound.setInteger("credits", credits); } @Override public void loadNBTData(NBTTagCompound compound) { if (compound.hasKey("credits")) { this.credits = compound.getInteger("credits"); } else { this.credits = 30; } sendPacket(); } @Override public void init(Entity entity, World world) { player = (EntityPlayerMP) entity; } private void sendPacket() { PacketDispatcher.sendPacketToPlayer(new PropertiesPacket(credits).makePacket(), (Player) player); } } Where my PropertiesPacket class looks like so: public class PropertiesPacket extends BasePacket { int credits; public PropertiesPacket(int credits) { this.credits = credits; } public PropertiesPacket() { } @Override public void write(ByteArrayDataOutput out) { out.writeInt(credits); } @Override public void read(ByteArrayDataInput in) { credits = in.readInt(); } @Override public void execute(EntityPlayer player, Side side) { if (side.isClient()) { PlayerData.credits = credits; // PlayerData is just a static client side class which stores the data. } } } Where the BasePacket class looks like this: public abstract class BasePacket { public static final String CHANNEL = "MyPacket"; private static final BiMap<Integer, Class<? extends BasePacket>> idMap; static { ImmutableBiMap.Builder<Integer, Class<? extends BasePacket>> builder = ImmutableBiMap.builder(); builder.put(Integer.valueOf(0), PropertiesPacket.class); idMap = builder.build(); } public static BasePacket constructPacket(int packetId) throws ProtocolException, ReflectiveOperationException { Class<? extends BasePacket> clazz = idMap.get(Integer.valueOf(packetId)); if (clazz == null) { throw new ProtocolException("Unknown Packet Id!"); } else { return clazz.newInstance(); } } public static class ProtocolException extends Exception { public ProtocolException() { } public ProtocolException(String message, Throwable cause) { super(message, cause); } public ProtocolException(String message) { super(message); } public ProtocolException(Throwable cause) { super(cause); } } public final int getPacketId() { if (idMap.inverse().containsKey(getClass())) { return idMap.inverse().get(getClass()).intValue(); } else { throw new RuntimeException("Packet " + getClass().getSimpleName() + " is missing a mapping!"); } } public final Packet makePacket() { ByteArrayDataOutput out = ByteStreams.newDataOutput(); out.writeByte(getPacketId()); write(out); return PacketDispatcher.getPacket(CHANNEL, out.toByteArray()); } public abstract void write(ByteArrayDataOutput out); public abstract void read(ByteArrayDataInput in); public abstract void execute(EntityPlayer player, Side side); } and my packet handler is as so: public class PacketHandler implements IPacketHandler { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { try { EntityPlayer entityPlayer = (EntityPlayer) player; ByteArrayDataInput in = ByteStreams.newDataInput(packet.data); int packetId = in.readUnsignedByte(); BasePacket basePacket = BasePacket.constructPacket(packetId); basePacket.read(in); basePacket.execute(entityPlayer, entityPlayer.worldObj.isRemote ? Side.CLIENT : Side.SERVER); } catch (ProtocolException e) { if (player instanceof EntityPlayerMP) { ((EntityPlayerMP) player).playerNetServerHandler.kickPlayerFromServer("Protocol Exception!"); Logger.getLogger("DemoMod").warning("Player " + ((EntityPlayer) player).username + " caused a Protocol Exception!"); } } catch (ReflectiveOperationException e) { throw new RuntimeException("Unexpected Reflection exception during Packet construction!", e); } } } However, when this code is run, it throws a null pointer exception and the packet isn't sent. Every time I've used this method of sending packets previously it's worked a charm, but this time it doesn't. The stack trace is: 2013-09-11 21:30:37 [iNFO] [sTDERR] java.lang.NullPointerException 2013-09-11 21:30:37 [iNFO] [sTDERR] at cpw.mods.fml.common.network.PacketDispatcher.sendPacketToPlayer(PacketDispatcher.java:45) 2013-09-11 21:30:37 [iNFO] [sTDERR] at mods.SaberMod.SaberValues.sendPacket(SaberValues.java:147) 2013-09-11 21:30:37 [iNFO] [sTDERR] at mods.SaberMod.SaberValues.loadNBTData(SaberValues.java:76) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.entity.Entity.readFromNBT(Entity.java:1647) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.management.ServerConfigurationManager.readPlayerDataFromFile(ServerConfigurationManager.java:239) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.management.ServerConfigurationManager.initializeConnectionToPlayer(ServerConfigurationManager.java:113) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.integrated.IntegratedServerListenThread.networkTick(IntegratedServerListenThread.java:97) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:689) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:585) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:129) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:482) 2013-09-11 21:30:37 [iNFO] [sTDERR] at net.minecraft.server.ThreadMinecraftServer.run(ThreadMinecraftServer.java:16) which points to this line in the packet dispatcher: public static void sendPacketToPlayer(Packet packet, Player player) { if (player instanceof EntityPlayerMP) { ((EntityPlayerMP)player).playerNetServerHandler.sendPacketToPlayer(packet); //<-- this one } } This suggests the player in its own variables is wrong, the player's missing the server handler or the packet is null. I'm assuming the packet is null, but I don't see why this one shouldn't work while the others do. What is the likely problem here?
-
Checking that it's on the server side did nothing, it still cannot find the entity
-
The code is in the entities constructor, and there is no server side check, does this matter?
-
Hello, I've set up a packet to send entity data from the server to the client. However, I cannot get the instance of the entity on the client side to change its variables. Here is my packet class: public class MyEntityPacket extends MyModPacket { int entityID,flag; public MyEntityPacket(int entityID, int flag) { this.entityID = entityID; this.flag = flag; } public MyEntityPacket() {} @Override public void write(ByteArrayDataOutput out) { out.writeInt(entityID); out.writeInt(flag); } @Override public void read(ByteArrayDataInput in) { entityID = in.readInt(); flag = in.readInt(); } @Override public void execute(EntityPlayer player, Side side) { if (side.isClient()) { if (Minecraft.getMinecraft().theWorld.getEntityByID(entityID) instanceof MyEntity) { MyEntity e = (MyEntity) player.worldObj.getEntityByID(entityID); e.setFlag(flag == 1 ? true : false); } else { System.out.println("Something ****** up! - supposed my entity with id: " + entityID + " isn't"); } } } } That all works fine. My problem is that the method getEntityByID(entityID) always returns null instead of the entity. I'm using this to create the packet in the constructor of the entity (it is the final thing in the constructor so the entity should be fine). PacketDispatcher.sendPacketToAllAround(par2, par4, par6, 250, this.dimension, new MyEntityPacket(this.entityId, flag ? 1 : 0).makePacket()); // 250 is view radius Can anyone enlighten me? Do I need to use the UUID (persistent id) instead? If so, how would I get the entity from it?
-
There are no forge hooks for when options change. But you can use a client tick handler to check for changes then perform actions when the changes occur.
-
Right, I've set up my packet for this. However, I cannot get the instance of the entity on the client side to change its variables. Here is my packet class: public class MyEntityPacket extends MyModPacket { int entityID,flag; public MyEntityPacket(int entityID, int flag) { this.entityID = entityID; this.flag = flag; } public MyEntityPacket() {} @Override public void write(ByteArrayDataOutput out) { out.writeInt(entityID); out.writeInt(flag); } @Override public void read(ByteArrayDataInput in) { entityID = in.readInt(); flag = in.readInt(); } @Override public void execute(EntityPlayer player, Side side) { if (side.isClient()) { if (Minecraft.getMinecraft().theWorld.getEntityByID(entityID) instanceof MyEntity) { MyEntity e = (MyEntity) player.worldObj.getEntityByID(entityID); e.setFlag(flag == 1 ? true : false); } else { System.out.println("Something ****** up! - supposed my entity with id: " + entityID + " isn't"); } } } } I've converted my boolean to an integer for the process of sending the packet. That all works fine and dandy. My problem is that the method getEntityByID(entityID) always returns null instead of the entity. I'm using this to create the packet in the constructor of the entity (it is the final thing in the constructor so the entity should be fine). PacketDispatcher.sendPacketToAllAround(par2, par4, par6, 250, this.dimension, new MyEntityPacket(this.entityId, flag ? 1 : 0).makePacket()); // 250 is view radius Can anyone enlighten me? Do I need to use the UUID (persistent id) instead? If so, how would I get the entity from it?
-
Hello, I'm trying to make my entity render in different ways based on a simple boolean value stored in its entity class. This boolean is initialized when the entity is created like so: worldObj.spawnEntityInWorld(new MyEntity(worldObj, posX, posY, posZ, intPar5, booleanPar6)); and then the entities constructor is as so.. public MyEntity(World par1World, double par2, double par4, double par6, int par8, boolean flag) { super(par1World); this.setSize(0.5F, 0.5F); this.yOffset = this.height / 2.0F; this.setPosition(par2, par4, par6); this.rotationYaw = (float)(Math.random() * 360.0D); this.var1 = par8; this.flag = flag; System.out.println(flag); } However, when I try to access my flag variable from my renderer, it always returns the default value (false) even if true is used to construct the entity class. public boolean getFlag() { return flag; } if (par1MyEntity.getFlag()) { //do stuff } else { //do other stuff } The else section will always be run as getFlag() returns false regardless of the actual value, but as you can see above, it returns the value set by the constructor. This values is never edited from its default constructed value. Could this be because the client can't access the data, does it need to be send via packets? or should entities automatically send their data?
-
Editing how an item is rendered between 1st and 3rd person
Briggybros replied to Briggybros's topic in Modder Support
somebody? -
Editing how an item is rendered between 1st and 3rd person
Briggybros replied to Briggybros's topic in Modder Support
I haven't done anything for the 1st person because I don't know where to start, but what I've done with the 3rd person is set up an event listener listening to the special player render, both pre and post, then executing my code, like so: public class ClientListener { @ForgeSubscribe(priority = EventPriority.NORMAL) public void preItemRendered(Pre event) { ItemStack itemstack1 = event.entityPlayer.getCurrentEquippedItem(); if (itemstack1 != null) { if (itemstack1.itemID == MyMod.MyItem1.itemID || itemstack1.itemID == MyMod.MyItem2.itemID) { event.renderItem = false; } } } @ForgeSubscribe(priority = EventPriority.NORMAL) public void postItemRendered(Post event) { ItemStack itemstack1 = event.entityPlayer.getCurrentEquippedItem(); if (itemstack1 != null) { if (itemstack1.itemID == MyMod.MyItem1.itemID || itemstack1.itemID == MyMod.MyItem2.itemID) { // Can't post code because Minecraft is closed source etc. // It's just a modified item renderer } } } private ModelBiped getModelBiped(RenderPlayer renderer) { try { Field f = renderer.getClass().getDeclaredField("modelBipedMain"); // NoSuchFieldException f.setAccessible(true); return (ModelBiped) f.get(renderer); // IllegalAccessException } catch (Exception e) { try { Field f = renderer.getClass().getDeclaredField("field_77109_a"); // NoSuchFieldException f.setAccessible(true); return (ModelBiped) f.get(renderer); // IllegalAccessException } catch (Exception e1) { e.printStackTrace(); e1.printStackTrace(); return null; } } } As you can see above, I cancel the rendering of the item, then I basically replace the code. Well kinda.. it works for me -
Hello, I'm using a class which implements IExtendedEntityProperties to store additional data for the player. However, I require some of this data in client side only methods where I cannot get the instance of EntityPlayerMP as it is not accessible from the client (due to the server always hosting EntityPlayerMP (Integrated or not)). How can I aquire this data from the client side methods efficiently? Would it be wise to set up a class only on the client side to store values for the data and then access them statically? What's the best way to go about this?
-
Thanks, I thought that, but was unsure of a better way, like a handler..
-
Hello, I'm trying to listen to when a player changes the slot of their equipped item, either using the number keys 1-9 or the mouse wheel. However, I can't find any way to do this. Is there any way?
-
Hello, I'm trying to have an item that is rendered differently from others, so far I've been able to make it render as I want in 3rd person. However, it doesn't work in 1st person. I know that there are two render types for that EQUIPPED and EQUIPPED_FIRST_PERSON, what I need to know is how to change the way the item is rendered in 1st person. Any hints?
-
Listen for the slot change, I'm usure if forge has an event for this, then check if shift is being pressed (EntityPlayer.isSneaking?). Then do what you want.
-
Thanks for the reply, that'll save the casting as well
-
Modify the health and position of an offline player
Briggybros replied to Lightwave's topic in Modder Support
Examine their player.dat file, open it in java using a buffered reader and then write it back using a buffered writer with the modified exception. NOTE: I've never needed to look into the player.dat files so I don't know what you'd be looking for sorry. EDIT: Just looked into NBT and dat files, firstly the dat file is a GZIP file, with sub files, you'll have to unpack it to read and edit it. This is the actual data file stored withing the {playername}.dat <-- wouldn't let me c&p the text. you should see the variables of the palyer, this one has a few of the ones I've added too.. you can see the health section? that's what you have to edit somehow enjoy -
I know this may sound nooby, but I can't seem to be able to register an event listener without it erroring, I've done it before, but this one doesn't seem to work at all. I'm registering it on the client side as it's a rendering listener. My client proxy methods work fine, this is how I'm registering it: @Override public void init() { //KeyBindingRegistry.registerKeyBinding(new KeyBindingListener(KeyBindingListener.keyArray, KeyBindingListener.repeatings)); //TickRegistry.registerTickHandler(new ClientTickHandler(), Side.CLIENT); //MinecraftForge.EVENT_BUS.register(new OnScreenGui()); MinecraftForge.EVENT_BUS.register(new ClientListener()); // This one! It's exactly the same as the one above.. } This is the working listener: public class OnScreenGui extends Gui { private Minecraft mc; public OnScreenGui() { super(); this.mc = Minecraft.getMinecraft(); } @ForgeSubscribe(priority = EventPriority.NORMAL) public void onRender(RenderGameOverlayEvent event) { // My method for my screen } } And here's the broken one: public class ClientListener { @ForgeSubscribe(priority = EventPriority.NORMAL) public void onItemRendered(RenderPlayerEvent event) { // My method again } private Object supportingMethod1(Object args) { // Supporting method } private Object supportingMethod2(Object args) { // Supporting method } the error is as follows: 2013-09-02 23:16:01 [iNFO] [sTDERR] java.lang.InstantiationException 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.InstantiationExceptionConstructorAccessorImpl.newInstance(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at java.lang.reflect.Constructor.newInstance(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraftforge.event.EventBus.register(EventBus.java:76) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraftforge.event.EventBus.register(EventBus.java:58) 2013-09-02 23:16:01 [iNFO] [sTDERR] at mods.SaberMod.client.ClientProxy.init(ClientProxy.java:48) 2013-09-02 23:16:01 [iNFO] [sTDERR] at mods.SaberMod.SaberMod.load(SaberMod.java:97) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:540) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-09-02 23:16:01 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:193) 2013-09-02 23:16:01 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:173) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:313) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-09-02 23:16:01 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-09-02 23:16:01 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:104) 2013-09-02 23:16:01 [iNFO] [sTDERR] at cpw.mods.fml.common.Loader.initializeMods(Loader.java:697) 2013-09-02 23:16:01 [iNFO] [sTDERR] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:222) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.startGame(Minecraft.java:506) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:796) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraft.client.main.Main.main(Main.java:93) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraft.launchwrapper.Launch.launch(Launch.java:57) 2013-09-02 23:16:01 [iNFO] [sTDERR] at net.minecraft.launchwrapper.Launch.main(Launch.java:18)
-
/** * Sets the block ID and metadata at a given location. Args: X, Y, Z, new block ID, new metadata, flags. Flag 1 will * cause a block update. Flag 2 will send the change to clients (you almost always want this). Flag 4 prevents the * block from being re-rendered, if this is a client world. Flags can be added together. */
-
That's just the same as getTextureByXP It now renders, but the orb only renders as grey. I've given it the rgb values as floats, but it just doesn't work. @SideOnly(Side.CLIENT) public class RenderMyNewOrb extends Render { private static final ResourceLocation orbTexture = new ResourceLocation("textures/entity/experience_orb.png"); public RenderMyNewOrb() { this.shadowSize = 0.15F; this.shadowOpaque = 0.75F; } /** * Renders the Orb. */ public void renderTheOrb(EntityMyNewOrb par1EntityMyNewOrb, double par2, double par4, double par6, float par8, float par9) { GL11.glPushMatrix(); GL11.glTranslatef((float)par2, (float)par4, (float)par6); this.func_110777_b(par1EntityMyNewOrb); int i = par1EntityMyNewOrb.getTextureByValue(); float f2 = (float)(i % 4 * 16 + 0) / 64.0F; float f3 = (float)(i % 4 * 16 + 16) / 64.0F; float f4 = (float)(i / 4 * 16 + 0) / 64.0F; float f5 = (float)(i / 4 * 16 + 16) / 64.0F; float f6 = 1.0F; float f7 = 0.5F; float f8 = 0.25F; int j = par1EntityMyNewOrb.getBrightnessForRender(par9); int k = j % 65536; int l = j / 65536; OpenGlHelper.setLightmapTextureCoords(OpenGlHelper.lightmapTexUnit, (float)k / 1.0F, (float)l / 1.0F); float r = 0.74F, g = 0.37F, b = 0.37F; if(par1EntityMyNewOrb.isLight) { r = 0.51F; g = 0.89F; b = 0.89F; } GL11.glColor4f(r, g, b, 1.0F); float f9 = 255.0F; int i1 = (int)f9; GL11.glRotatef(180.0F - this.renderManager.playerViewY, 0.0F, 1.0F, 0.0F); GL11.glRotatef(-this.renderManager.playerViewX, 1.0F, 0.0F, 0.0F); float f11 = 0.3F; GL11.glScalef(f11, f11, f11); Tessellator tessellator = Tessellator.instance; tessellator.startDrawingQuads(); tessellator.setColorRGBA_F(r,g,b, 128); tessellator.setNormal(0.0F, 1.0F, 0.0F); tessellator.addVertexWithUV((double)(0.0F - f7), (double)(0.0F - f8), 0.0D, (double)f2, (double)f5); tessellator.addVertexWithUV((double)(f6 - f7), (double)(0.0F - f8), 0.0D, (double)f3, (double)f5); tessellator.addVertexWithUV((double)(f6 - f7), (double)(1.0F - f8), 0.0D, (double)f3, (double)f4); tessellator.addVertexWithUV((double)(0.0F - f7), (double)(1.0F - f8), 0.0D, (double)f2, (double)f4); tessellator.draw(); GL11.glDisable(GL11.GL_BLEND); GL11.glDisable(GL12.GL_RESCALE_NORMAL); GL11.glPopMatrix(); } protected ResourceLocation func_110775_a(Entity par1Entity) { return orbTexture; } public void doRender(Entity par1Entity, double par2, double par4, double par6, float par8, float par9) { this.renderTheOrb((EntityMyNewOrb)par1Entity, par2, par4, par6, par8, par9); } }