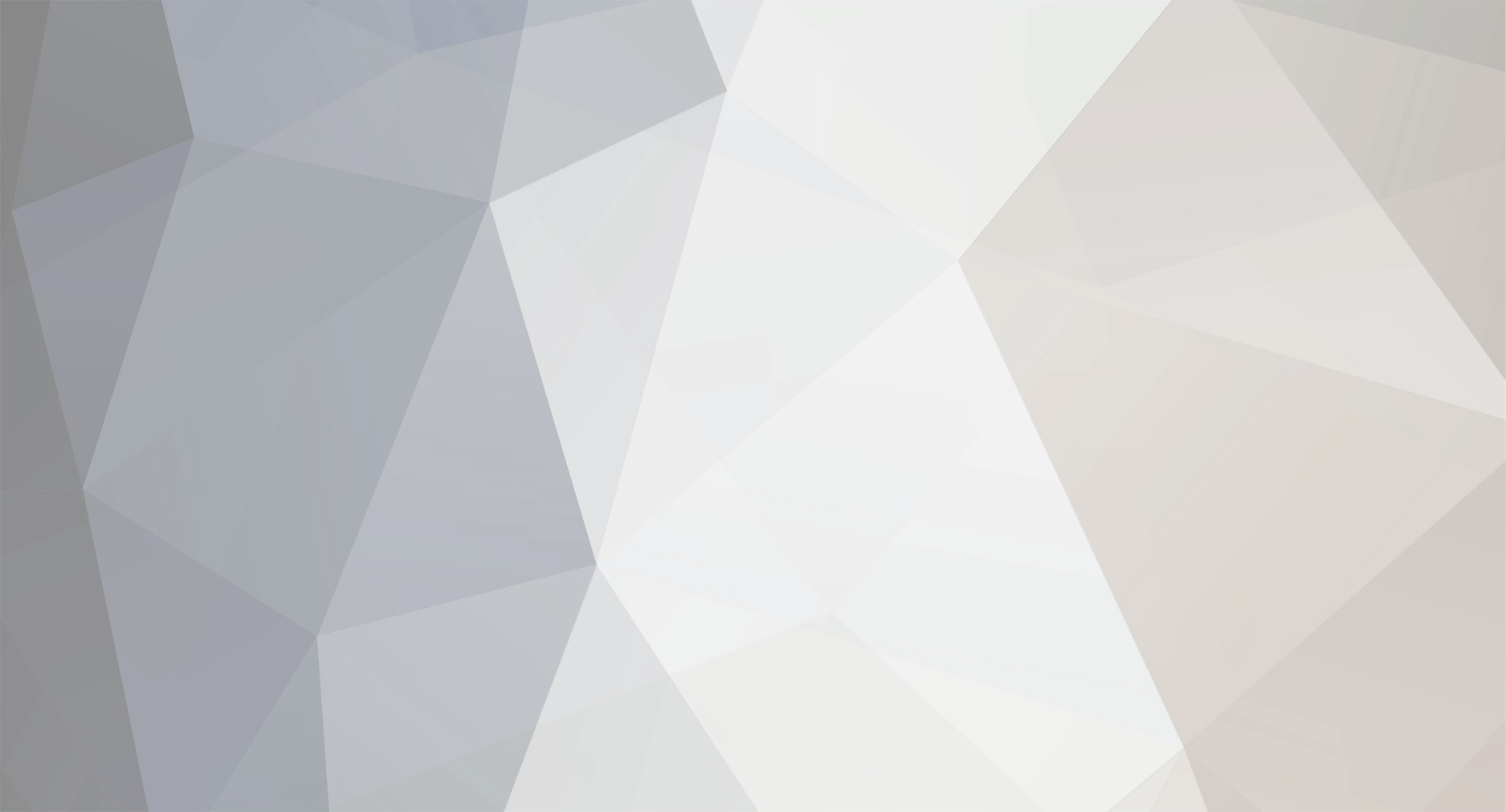
Briggybros
Members-
Posts
155 -
Joined
-
Last visited
Everything posted by Briggybros
-
Thank you!
-
Hello, all I am trying to do is give different names to different damage values of an object. for (int x = 0; x < SaberMod.starMapNames.length; x++) { ItemStack starMap = new ItemStack(SaberMod.starMap, 1, x); LanguageRegistry.addName(starMap, "Star Map: " + SaberMod.starMapNames[x]); } As you can see above, my code parses through every name for my item, then finds the item with its value depending on the name being parsed, then it adds the name to that damage value. However, When I test all of the items of the type have the last name in the String[] object as their name, not the individual name. This is happening because it's not recognizing the data value and applying it to the entire item, what can I do to solve this?
-
Thank you this is just what I needed!
-
I've got even more confused about this now. Why can't the packet handler play the sound if it's clientside? I have the trigger, all of the settings yet still no sound.
-
I've split my packet methods into the common and client proxy, thinking that if it were serverside then it would only run the CommonProxy and clientside, it would be overridden by the ClientProxy. So my PacketHandler now looks like this; package uk.co.toomuchminecraft.sabermod; import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.IOException; import net.minecraft.client.Minecraft; import net.minecraft.src.Entity; import net.minecraft.src.EntityPlayerMP; import net.minecraft.src.INetworkManager; import net.minecraft.src.InventoryPlayer; import net.minecraft.src.ItemStack; import net.minecraft.src.Packet250CustomPayload; import net.minecraft.src.World; import net.minecraft.src.WorldClient; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Side; import cpw.mods.fml.common.asm.SideOnly; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.PacketDispatcher; import cpw.mods.fml.common.network.Player; public class PacketHandler implements IPacketHandler { @SideOnly(Side.CLIENT) Minecraft mc; @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { if (packet.channel.equals("SaberExtend")) { handleExtendPacket(packet, player); } if (packet.channel.equals("SaberSound")) { handleSoundPacket(packet, player); } } private void handleExtendPacket(Packet250CustomPayload packet, Player player) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int itemID; int itemDamage; int slot; int action; int saberID; try { itemID = inputStream.readInt(); itemDamage = inputStream.readInt(); slot = inputStream.readInt(); action = inputStream.readInt(); saberID = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side == Side.SERVER) { SaberMod.proxy.extendSaber(saberID, action, player); PacketDispatcher.sendPacketToAllPlayers(packet); SaberMod.proxy.closeInventoryChange(player); } else if (side == Side.CLIENT) { SaberMod.proxy.extendSaber(saberID, action, player); SaberMod.proxy.closeInventoryChange(player); } } private void handleSoundPacket(Packet250CustomPayload packet, Player player) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int sound; try { sound = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side == Side.SERVER) { PacketDispatcher.sendPacketToAllPlayers(packet); SaberMod.proxy.playSound(sound, player); } else if (side == Side.CLIENT) { SaberMod.proxy.playSound(sound, player); } } } The CommonProxy methods are; public void playSound(int sound, Player p) { EntityClientPlayerMP player = (EntityClientPlayerMP)p; if(sound == 0) { System.out.println("SaberMod: " + player.username + " playing lightsaber retract sound"); } if(sound == 1) { System.out.println("SaberMod: " + player.username + " playing lightsaber extend sound"); } if(sound == 2) { System.out.println("SaberMod: " + player.username + " playing lightsaber swing sound"); } } public void closeInventoryChange(Player p) { EntityPlayerMP player = (EntityPlayerMP)p; player.inventory.inventoryChanged = false; } public void extendSaber(int saberID, int action, Player p) { EntityPlayerMP player = (EntityPlayerMP)p; if(saberID == 0) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 1) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 2) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 3) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 4) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 5) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 6) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 7) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } } and my ClientProxy methods are; @Override public void playSound(int sound, Player p) { EntityPlayerMP player = (EntityPlayerMP)p; if(sound == 0) { player.worldObj.playSoundAtEntity((Entity)player, "sabermod.saberoff", 1F, 1F); } if(sound == 1) { player.worldObj.playSoundAtEntity((Entity)player, "sabermod.saberon", 1F, 1F); } if(sound == 2) { player.worldObj.playSoundAtEntity((Entity)player, "sabermod.saberswing", 1F, 1F); } } @Override public void closeInventoryChange(Player p) { EntityPlayerMP player = (EntityPlayerMP)p; player.inventory.inventoryChanged = false; } @Override public void extendSaber(int saberID, int action, Player p) { EntityPlayerMP player = (EntityPlayerMP)p; // ClientProxy(303) if(saberID == 0) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 1) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 2) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 3) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 4) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 5) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 6) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 7) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } } However, when I run the game the when calling one of these methods the client throws this error; 2012-11-23 18:26:10 [iNFO] [sTDERR] net.minecraft.src.ReportedException: Exception in world tick 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1892) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:858) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.run(Minecraft.java:783) 2012-11-23 18:26:10 [iNFO] [sTDERR] at java.lang.Thread.run(Unknown Source) 2012-11-23 18:26:10 [iNFO] [sTDERR] Caused by: java.lang.ClassCastException: net.minecraft.src.EntityClientPlayerMP cannot be cast to net.minecraft.src.EntityPlayerMP 2012-11-23 18:26:10 [iNFO] [sTDERR] at uk.co.toomuchminecraft.sabermod.client.ClientProxy.extendSaber(ClientProxy.java:303) 2012-11-23 18:26:10 [iNFO] [sTDERR] at uk.co.toomuchminecraft.sabermod.PacketHandler.handleExtendPacket(PacketHandler.java:76) 2012-11-23 18:26:10 [iNFO] [sTDERR] at uk.co.toomuchminecraft.sabermod.PacketHandler.onPacketData(PacketHandler.java:34) 2012-11-23 18:26:10 [iNFO] [sTDERR] at cpw.mods.fml.common.network.NetworkRegistry.handlePacket(NetworkRegistry.java:249) 2012-11-23 18:26:10 [iNFO] [sTDERR] at cpw.mods.fml.common.network.NetworkRegistry.handleCustomPacket(NetworkRegistry.java:239) 2012-11-23 18:26:10 [iNFO] [sTDERR] at cpw.mods.fml.common.network.FMLNetworkHandler.handlePacket250Packet(FMLNetworkHandler.java:78) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.src.NetClientHandler.handleCustomPayload(NetClientHandler.java:1344) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.src.Packet250CustomPayload.processPacket(Packet250CustomPayload.java:70) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.src.MemoryConnection.processReadPackets(MemoryConnection.java:79) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.src.NetClientHandler.processReadPackets(NetClientHandler.java:104) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.src.WorldClient.tick(WorldClient.java:72) 2012-11-23 18:26:10 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1876) 2012-11-23 18:26:10 [iNFO] [sTDERR] ... 3 more Again I have marked the line in the code. I'm really stumped with this, can someone help?
-
How would I create a client side only packet handler?
-
I was debating that, but surely, typecasting the player to EntityClientPlayerMP wouldn't return a player it would return CONSOLE as if it's being handled on the client it was sent from the server. EDIT: Edited the PacketHandler; package uk.co.toomuchminecraft.sabermod; import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.IOException; import net.minecraft.client.Minecraft; import net.minecraft.src.Entity; import net.minecraft.src.EntityClientPlayerMP; import net.minecraft.src.EntityList; import net.minecraft.src.EntityPlayer; import net.minecraft.src.EntityPlayerMP; import net.minecraft.src.EntityPlayerSP; import net.minecraft.src.INetworkManager; import net.minecraft.src.InventoryPlayer; import net.minecraft.src.ItemStack; import net.minecraft.src.Packet250CustomPayload; import net.minecraft.src.World; import net.minecraft.src.WorldClient; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Side; import cpw.mods.fml.common.asm.SideOnly; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.PacketDispatcher; import cpw.mods.fml.common.network.Player; public class PacketHandler implements IPacketHandler { @SideOnly(Side.CLIENT) Minecraft mc; @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { if (packet.channel.equals("SaberExtend")) { handleExtendPacket(packet, player); } if (packet.channel.equals("SaberSound")) { handleSoundPacket(packet, player); } } private void handleExtendPacket(Packet250CustomPayload packet, Player player) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int itemID; int itemDamage; int slot; int action; int saberID; try { itemID = inputStream.readInt(); itemDamage = inputStream.readInt(); slot = inputStream.readInt(); action = inputStream.readInt(); saberID = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side == Side.SERVER) { EntityPlayerMP executingPlayer = (EntityPlayerMP)player; extendSaber(saberID, action, executingPlayer); PacketDispatcher.sendPacketToAllPlayers(packet); closeInventoryChange(executingPlayer); } else if (side == Side.CLIENT) { EntityClientPlayerMP executingPlayer = (EntityClientPlayerMP)player; extendSaber(saberID, action, executingPlayer); closeInventoryChange(executingPlayer); } } private void handleSoundPacket(Packet250CustomPayload packet, Player player) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int x; int y; int z; int sound; try { x = inputStream.readInt(); y = inputStream.readInt(); z = inputStream.readInt(); sound = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side == Side.SERVER) { PacketDispatcher.sendPacketToAllPlayers(packet); playSound(x, y, z, sound, (EntityPlayerMP)player); } else if (side == Side.CLIENT) { this.playSound(x, y, z , sound, (EntityClientPlayerMP)player); } } private void closeInventoryChange(EntityClientPlayerMP player) { player.inventory.inventoryChanged = false; } private void closeInventoryChange(EntityPlayerMP player) { player.inventory.inventoryChanged = false; } private void playSound(int x, int y, int z, int sound, EntityClientPlayerMP player) { if(sound == 0) { player.worldObj.playSoundEffect(x, y, z, "sabermod.saberoff", 1F, 1F); } if(sound == 1) { player.worldObj.playSoundEffect(x, y, z, "sabermod.saberon", 1F, 1F); } if(sound == 2) { player.worldObj.playSoundEffect(x, y, z, "sabermod.saberswing", 1F, 1F); } } private void playSound(int x, int y, int z, int sound, EntityPlayerMP player) { if(sound == 0) { System.out.println("SaberMod: " + player.username + " playing lightsaber retract sound"); } if(sound == 1) { System.out.println("SaberMod: " + player.username + " playing lightsaber extend sound"); } if(sound == 2) { System.out.println("SaberMod: " + player.username + " playing lightsaber swing sound"); } } private void extendSaber(int saberID, int action, EntityPlayerMP player) { if(saberID == 0) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 1) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 2) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 3) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 4) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 5) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 6) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == 7) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } if(saberID == { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " retracting lightsaber"); } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; System.out.println("SaberMod: " + player.username + " extending lightsaber"); } } } private void extendSaber(int saberID, int action, EntityClientPlayerMP player) { if(saberID == 0) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberPurpleOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 1) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberRedOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 2) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberYellowOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 3) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGreenOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 4) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberBlackOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 5) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberIndigoOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 6) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberDarkBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == 7) { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberGoldOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } if(saberID == { if(action == 0) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOff.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } if(action == 1) { InventoryPlayer inventoryplayer = player.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberMod.saberLightBlueOn.shiftedIndex, 1, inventoryplayer.getCurrentItem().getItemDamage())); player.inventory.inventoryChanged = true; } } } } EDIT: The system outputs the system messages for the extend sabers methods twice - not a problem, just can get cluttered. Also, the system output for the sound playing is working without error, however the sound itself isn't actually playing. I register the sounds with this clientside and play them as you can see in the PacketHandler. package uk.co.toomuchminecraft.sabermod.client; import uk.co.toomuchminecraft.sabermod.SaberMod; import net.minecraft.client.Minecraft; import net.minecraft.src.World; import net.minecraftforge.client.event.sound.SoundLoadEvent; import net.minecraftforge.event.ForgeSubscribe; public class SaberSounds { @ForgeSubscribe public void onSound(SoundLoadEvent event) { try { event.manager.soundPoolSounds.addSound("sabermod/saberoff.ogg", SaberMod.class.getResource("/SaberMod/sound/saberoff.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberoff.ogg", SaberMod.class.getResource("/SaberMod/sound/saberoff.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing0.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing0.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing1.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing1.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing2.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing2.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing3.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing3.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing4.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing4.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing5.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing5.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing6.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing6.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing7.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing7.ogg")); } catch (Exception e) { System.err.println("Failed to register one or more sounds."); } } }
-
Hello, I am currently having problems sending a sound request through a packet system. I am constructing the packet as so; private void sendSoundPacket(int x, int y, int z, int sound) { ByteArrayOutputStream bos = new ByteArrayOutputStream(16); DataOutputStream outputStream = new DataOutputStream(bos); try { outputStream.writeInt(x); outputStream.writeInt(y); outputStream.writeInt(z); outputStream.writeInt(sound); outputStream.writeInt(mc.thePlayer.entityId); } catch (Exception ex) { ex.printStackTrace(); } Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = "SaberSound"; packet.data = bos.toByteArray(); packet.length = bos.size(); PacketDispatcher.sendPacketToServer(packet); } Then handling it with; private void handleSoundPacket(Packet250CustomPayload packet, Player player) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int x; int y; int z; int sound; int playerID; try { x = inputStream.readInt(); y = inputStream.readInt(); z = inputStream.readInt(); sound = inputStream.readInt(); playerID = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side == Side.SERVER) { sendSoundPacket(x, y, z, sound, (EntityPlayerMP)player); } else if (side == Side.CLIENT) { this.playSound(x, y, z , sound, ((EntityClientPlayerMP)mc.theWorld.getEntityByID(playerID)).worldObj); //PacketHandler.java:120 } } The playSound method is; private void playSound(int x, int y, int z, int sound, World world) { if(sound == 0) { world.playSoundEffect(x, y, z, "sabermod.saberoff", 1F, 1F); } if(sound == 1) { world.playSoundEffect(x, y, z, "sabermod.saberon", 1F, 1F); } if(sound == 2) { world.playSoundEffect(x, y, z, "sabermod.saberswing", 1F, 1F); } } and the server side sendSoundPacket is; private void sendSoundPacket(int x, int y, int z, int sound, EntityPlayerMP player) { int playerID = player.entityId; ByteArrayOutputStream bos = new ByteArrayOutputStream(20); DataOutputStream outputStream = new DataOutputStream(bos); try { outputStream.writeInt(x); outputStream.writeInt(y); outputStream.writeInt(z); outputStream.writeInt(sound); outputStream.writeInt(playerID); } catch (Exception ex) { ex.printStackTrace(); } Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = "SaberSound"; packet.data = bos.toByteArray(); packet.length = bos.size(); PacketDispatcher.sendPacketToAllPlayers(packet); } The sounds are registered with; @ForgeSubscribe public void onSound(SoundLoadEvent event) { try { event.manager.soundPoolSounds.addSound("sabermod/saberoff.ogg", SaberMod.class.getResource("/SaberMod/sound/saberoff.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberoff.ogg", SaberMod.class.getResource("/SaberMod/sound/saberoff.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing0.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing0.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing1.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing1.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing2.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing2.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing3.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing3.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing4.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing4.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing5.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing5.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing6.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing6.ogg")); event.manager.soundPoolSounds.addSound("sabermod/saberswing7.ogg", SaberMod.class.getResource("/SaberMod/sound/saberswing7.ogg")); } catch (Exception e) { System.err.println("Failed to register one or more sounds."); } } The error I get is; 2012-11-21 00:13:00 [iNFO] [sTDERR] net.minecraft.src.ReportedException: Exception in world tick 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1892) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:858) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.run(Minecraft.java:783) 2012-11-21 00:13:00 [iNFO] [sTDERR] at java.lang.Thread.run(Unknown Source) 2012-11-21 00:13:00 [iNFO] [sTDERR] Caused by: java.lang.NullPointerException 2012-11-21 00:13:00 [iNFO] [sTDERR] at uk.co.toomuchminecraft.sabermod.PacketHandler.handleSoundPacket(PacketHandler.java:120) 2012-11-21 00:13:00 [iNFO] [sTDERR] at uk.co.toomuchminecraft.sabermod.PacketHandler.onPacketData(PacketHandler.java:43) 2012-11-21 00:13:00 [iNFO] [sTDERR] at cpw.mods.fml.common.network.NetworkRegistry.handlePacket(NetworkRegistry.java:249) 2012-11-21 00:13:00 [iNFO] [sTDERR] at cpw.mods.fml.common.network.NetworkRegistry.handleCustomPacket(NetworkRegistry.java:239) 2012-11-21 00:13:00 [iNFO] [sTDERR] at cpw.mods.fml.common.network.FMLNetworkHandler.handlePacket250Packet(FMLNetworkHandler.java:78) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.src.NetClientHandler.handleCustomPayload(NetClientHandler.java:1344) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.src.Packet250CustomPayload.processPacket(Packet250CustomPayload.java:70) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.src.MemoryConnection.processReadPackets(MemoryConnection.java:79) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.src.NetClientHandler.processReadPackets(NetClientHandler.java:104) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.src.WorldClient.tick(WorldClient.java:72) 2012-11-21 00:13:00 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.runTick(Minecraft.java:1876) 2012-11-21 00:13:00 [iNFO] [sTDERR] ... 3 more I have marked the error location from the log in the src. What is going wrong with this? I think it's possibly the worlds, but I can't seem to be able to fix it whatever it is.
-
Solved
-
nobody?
-
Hello, I'm trying to extend a 'saber' on a KeyBinding I have the KeyBinding as so only on the ClientSide; package info.mineverse.willow.sabers.client; import info.mineverse.willow.sabers.SaberBase; import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import java.io.IOException; import java.io.OutputStream; import java.util.EnumSet; import org.lwjgl.input.Keyboard; import net.minecraft.client.Minecraft; import net.minecraft.src.EntityClientPlayerMP; import net.minecraft.src.EntityPlayer; import net.minecraft.src.EntityPlayerMP; import net.minecraft.src.InventoryPlayer; import net.minecraft.src.Item; import net.minecraft.src.ItemStack; import net.minecraft.src.KeyBinding; import net.minecraft.src.ModLoader; import net.minecraft.src.Packet250CustomPayload; import net.minecraft.src.Packet5PlayerInventory; import cpw.mods.fml.client.registry.KeyBindingRegistry.KeyHandler; import cpw.mods.fml.common.Side; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.TickType; import cpw.mods.fml.common.network.PacketDispatcher; import cpw.mods.fml.common.network.Player; public class SaberExtendKeyHandler extends KeyHandler { private Minecraft mc; static KeyBinding saberExtend = new KeyBinding("Extend Lightsaber", Keyboard.KEY_F); public SaberExtendKeyHandler() { //the first value is an array of KeyBindings, the second is whether or not the call //keyDown should repeat as long as the key is down super(new KeyBinding[]{saberExtend}, new boolean[]{false}); mc = ModLoader.getMinecraftInstance(); } @Override public String getLabel() { return "ExtendLightsaber"; } private void sendPacket(ItemStack item, int slot) { int itemID = item.itemID; int itemDamage = item.getItemDamage(); ByteArrayOutputStream bos = new ByteArrayOutputStream(12); DataOutputStream outputStream = new DataOutputStream(bos); try { outputStream.writeInt(itemID); outputStream.writeInt(itemDamage); outputStream.writeInt(slot); } catch (Exception ex) { ex.printStackTrace(); } Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = "SaberExtend"; packet.data = bos.toByteArray(); packet.length = bos.size(); PacketDispatcher.sendPacketToServer(packet); } @Override public void keyDown(EnumSet<TickType> types, KeyBinding kb, boolean tickEnd, boolean isRepeat) { if(kb.equals(saberExtend)) { ItemStack itemstack = mc.thePlayer.inventory.getCurrentItem(); if(itemstack != null) { if(itemstack.itemID == SaberBase.saberPurpleOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberPurpleOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.theWorld.playSoundEffect(mc.thePlayer.posX, mc.thePlayer.posY, mc.thePlayer.posZ, "willow.sabers.saberoff", 1F, mc.theWorld.rand.nextFloat() * 0.1F + 0.9F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); sendPacket(itemstack, mc.thePlayer.inventory.currentItem); } if(itemstack.itemID == SaberBase.saberPurpleOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberPurpleOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.theWorld.playSoundEffect(mc.thePlayer.posX, mc.thePlayer.posY, mc.thePlayer.posZ, "willow.sabers.saberoff", 1F, mc.theWorld.rand.nextFloat() * 0.1F + 0.9F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberRedOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberRedOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberRedOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberRedOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberYellowOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberYellowOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberYellowOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberYellowOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberGreenOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberGreenOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberGreenOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberGreenOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberBlackOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberBlackOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberBlackOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberBlackOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberIndigoOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberIndigoOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberIndigoOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberIndigoOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberDarkBlueOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberDarkBlueOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberDarkBlueOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberDarkBlueOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberGoldOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberGoldOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberGoldOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberGoldOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberLightBlueOn.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberLightBlueOff.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberoff", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberoff"); System.out.println("DEBUG: extending lightsaber"); } if(itemstack.itemID == SaberBase.saberLightBlueOff.shiftedIndex) { InventoryPlayer inventoryplayer = mc.thePlayer.inventory; inventoryplayer.setInventorySlotContents(inventoryplayer.currentItem, new ItemStack(SaberBase.saberLightBlueOn.shiftedIndex, 1, itemstack.getItemDamage())); mc.sndManager.playSoundFX("mod.sound.saberon", 1.0F, 1.0F); System.out.println("DEBUG: playing sound: mod.sound.saberon"); System.out.println("DEBUG: extending lightsaber"); } } } } @Override public void keyUp(EnumSet<TickType> types, KeyBinding kb, boolean tickEnd) { } @Override public EnumSet<TickType> ticks() { return EnumSet.of(TickType.CLIENT); } } I also have the packet handler as so; package info.mineverse.willow.sabers; import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.IOException; import net.minecraft.src.Entity; import net.minecraft.src.EntityClientPlayerMP; import net.minecraft.src.EntityList; import net.minecraft.src.EntityPlayer; import net.minecraft.src.EntityPlayerMP; import net.minecraft.src.EntityPlayerSP; import net.minecraft.src.INetworkManager; import net.minecraft.src.ItemStack; import net.minecraft.src.Packet250CustomPayload; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Side; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.PacketDispatcher; import cpw.mods.fml.common.network.Player; public class PacketHandler implements IPacketHandler { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { if (packet.channel.equals("SaberExtend")) { handlePacket(packet, player); } } private void handlePacket(Packet250CustomPayload packet, Player player) { DataInputStream inputStream = new DataInputStream(new ByteArrayInputStream(packet.data)); int itemID; int itemDamage; int slot; try { itemID = inputStream.readInt(); itemDamage = inputStream.readInt(); slot = inputStream.readInt(); } catch (IOException e) { e.printStackTrace(); return; } Side side = FMLCommonHandler.instance().getEffectiveSide(); if (side == Side.SERVER) { EntityPlayerMP executingPlayer = (EntityPlayerMP)player; executingPlayer.inventory.inventoryChanged = true; sendPacket(executingPlayer, itemID, itemDamage, slot); closeInventoryChange(executingPlayer); } else if (side == Side.CLIENT) { EntityClientPlayerMP executingPlayer = (EntityClientPlayerMP)player; executingPlayer.inventory.inventoryChanged = true; closeInventoryChange(executingPlayer); } } private void closeInventoryChange(EntityClientPlayerMP player) { player.inventory.inventoryChanged = false; } private void closeInventoryChange(EntityPlayerMP player) { player.inventory.inventoryChanged = false; } private void sendPacket(EntityPlayerMP entityPlayer, int itemID, int itemDamage, int slot) { int playerID = entityPlayer.entityId; ByteArrayOutputStream bos = new ByteArrayOutputStream(16); DataOutputStream outputStream = new DataOutputStream(bos); try { outputStream.writeInt(playerID); outputStream.writeInt(itemID); outputStream.writeInt(itemDamage); outputStream.writeInt(slot); } catch (Exception ex) { ex.printStackTrace(); } Packet250CustomPayload packet = new Packet250CustomPayload(); packet.channel = "SaberExtend"; packet.data = bos.toByteArray(); packet.length = bos.size(); PacketDispatcher.sendPacketToPlayer(packet, (Player)entityPlayer); } } When I press the KeyBinding the item dissappears and comes back quickly and doesn't change. I can't see what's wrong.
-
Hello, I'm trying to make a horse which is controllable by default movements when the character has mounted it. The EntityCientPlayerMP has the movementInput whereas EntityPlayerMP doesn't and that is the player. How I make this work, my code is; package net.minecraft.src; import cpw.mods.fml.common.Side; import cpw.mods.fml.common.asm.SideOnly; import net.minecraft.client.Minecraft; @SideOnly(Side.CLIENT) public class EntityPlayerSP extends EntityPlayer { public MovementInput movementInput; protected Minecraft mc; /** * Used to tell if the player pressed forward twice. If this is at 0 and it's pressed (And they are allowed to * sprint, aka enough food on the ground etc) it sets this to 7. If it's pressed and it's greater than 0 enable * sprinting. */ protected int sprintToggleTimer = 0; /** Ticks left before sprinting is disabled. */ public int sprintingTicksLeft = 0; public float renderArmYaw; public float renderArmPitch; public float prevRenderArmYaw; public float prevRenderArmPitch; private MouseFilter field_71162_ch = new MouseFilter(); private MouseFilter field_71160_ci = new MouseFilter(); private MouseFilter field_71161_cj = new MouseFilter(); public EntityPlayerSP(Minecraft par1Minecraft, World par2World, Session par3Session, int par4) { super(par2World); this.mc = par1Minecraft; this.dimension = par4; if (par3Session != null && par3Session.username != null && par3Session.username.length() > 0) { this.skinUrl = "http://skins.minecraft.net/MinecraftSkins/" + StringUtils.stripControlCodes(par3Session.username) + ".png"; } this.username = par3Session.username; } /** * Tries to moves the entity by the passed in displacement. Args: x, y, z */ public void moveEntity(double par1, double par3, double par5) { super.moveEntity(par1, par3, par5); } public void updateEntityActionState() { super.updateEntityActionState(); this.moveStrafing = this.movementInput.moveStrafe; this.moveForward = this.movementInput.moveForward; this.isJumping = this.movementInput.jump; this.prevRenderArmYaw = this.renderArmYaw; this.prevRenderArmPitch = this.renderArmPitch; this.renderArmPitch = (float)((double)this.renderArmPitch + (double)(this.rotationPitch - this.renderArmPitch) * 0.5D); this.renderArmYaw = (float)((double)this.renderArmYaw + (double)(this.rotationYaw - this.renderArmYaw) * 0.5D); } /** * Returns whether the entity is in a local (client) world */ protected boolean isClientWorld() { return true; } /** * Called frequently so the entity can update its state every tick as required. For example, zombies and skeletons * use this to react to sunlight and start to burn. */ public void onLivingUpdate() { if (this.sprintingTicksLeft > 0) { --this.sprintingTicksLeft; if (this.sprintingTicksLeft == 0) { this.setSprinting(false); } } if (this.sprintToggleTimer > 0) { --this.sprintToggleTimer; } if (this.mc.playerController.func_78747_a()) { this.posX = this.posZ = 0.5D; this.posX = 0.0D; this.posZ = 0.0D; this.rotationYaw = (float)this.ticksExisted / 12.0F; this.rotationPitch = 10.0F; this.posY = 68.5D; } else { if (!this.mc.statFileWriter.hasAchievementUnlocked(AchievementList.openInventory)) { this.mc.guiAchievement.queueAchievementInformation(AchievementList.openInventory); } this.prevTimeInPortal = this.timeInPortal; if (this.inPortal) { if (this.mc.currentScreen != null) { this.mc.displayGuiScreen((GuiScreen)null); } if (this.timeInPortal == 0.0F) { this.mc.sndManager.playSoundFX("portal.trigger", 1.0F, this.rand.nextFloat() * 0.4F + 0.8F); } this.timeInPortal += 0.0125F; if (this.timeInPortal >= 1.0F) { this.timeInPortal = 1.0F; } this.inPortal = false; } else if (this.isPotionActive(Potion.confusion) && this.getActivePotionEffect(Potion.confusion).getDuration() > 60) { this.timeInPortal += 0.006666667F; if (this.timeInPortal > 1.0F) { this.timeInPortal = 1.0F; } } else { if (this.timeInPortal > 0.0F) { this.timeInPortal -= 0.05F; } if (this.timeInPortal < 0.0F) { this.timeInPortal = 0.0F; } } if (this.timeUntilPortal > 0) { --this.timeUntilPortal; } boolean var1 = this.movementInput.jump; float var2 = 0.8F; boolean var3 = this.movementInput.moveForward >= var2; this.movementInput.updatePlayerMoveState(); if (this.isUsingItem()) { this.movementInput.moveStrafe *= 0.2F; this.movementInput.moveForward *= 0.2F; this.sprintToggleTimer = 0; } if (this.movementInput.sneak && this.ySize < 0.2F) { this.ySize = 0.2F; } this.pushOutOfBlocks(this.posX - (double)this.width * 0.35D, this.boundingBox.minY + 0.5D, this.posZ + (double)this.width * 0.35D); this.pushOutOfBlocks(this.posX - (double)this.width * 0.35D, this.boundingBox.minY + 0.5D, this.posZ - (double)this.width * 0.35D); this.pushOutOfBlocks(this.posX + (double)this.width * 0.35D, this.boundingBox.minY + 0.5D, this.posZ - (double)this.width * 0.35D); this.pushOutOfBlocks(this.posX + (double)this.width * 0.35D, this.boundingBox.minY + 0.5D, this.posZ + (double)this.width * 0.35D); boolean var4 = (float)this.getFoodStats().getFoodLevel() > 6.0F || this.capabilities.allowFlying; if (this.onGround && !var3 && this.movementInput.moveForward >= var2 && !this.isSprinting() && var4 && !this.isUsingItem() && !this.isPotionActive(Potion.blindness)) { if (this.sprintToggleTimer == 0) { this.sprintToggleTimer = 7; } else { this.setSprinting(true); this.sprintToggleTimer = 0; } } if (this.isSneaking()) { this.sprintToggleTimer = 0; } if (this.isSprinting() && (this.movementInput.moveForward < var2 || this.isCollidedHorizontally || !var4)) { this.setSprinting(false); } if (this.capabilities.allowFlying && !var1 && this.movementInput.jump) { if (this.flyToggleTimer == 0) { this.flyToggleTimer = 7; } else { this.capabilities.isFlying = !this.capabilities.isFlying; this.sendPlayerAbilities(); this.flyToggleTimer = 0; } } if (this.capabilities.isFlying) { if (this.movementInput.sneak) { this.motionY -= 0.15D; } if (this.movementInput.jump) { this.motionY += 0.15D; } } super.onLivingUpdate(); if (this.onGround && this.capabilities.isFlying) { this.capabilities.isFlying = false; this.sendPlayerAbilities(); } } } /** * Gets the player's field of view multiplier. (ex. when flying) */ public float getFOVMultiplier() { float var1 = 1.0F; if (this.capabilities.isFlying) { var1 *= 1.1F; } var1 *= (this.landMovementFactor * this.getSpeedModifier() / this.speedOnGround + 1.0F) / 2.0F; if (this.isUsingItem() && this.getItemInUse().itemID == Item.bow.shiftedIndex) { int var2 = this.getItemInUseDuration(); float var3 = (float)var2 / 20.0F; if (var3 > 1.0F) { var3 = 1.0F; } else { var3 *= var3; } var1 *= 1.0F - var3 * 0.15F; } return var1; } /** * (abstract) Protected helper method to write subclass entity data to NBT. */ public void writeEntityToNBT(NBTTagCompound par1NBTTagCompound) { super.writeEntityToNBT(par1NBTTagCompound); par1NBTTagCompound.setInteger("Score", this.score); } public void updateCloak() { this.playerCloakUrl = "http://skins.minecraft.net/MinecraftCloaks/" + StringUtils.stripControlCodes(this.username) + ".png"; this.cloakUrl = this.playerCloakUrl; } /** * (abstract) Protected helper method to read subclass entity data from NBT. */ public void readEntityFromNBT(NBTTagCompound par1NBTTagCompound) { super.readEntityFromNBT(par1NBTTagCompound); this.score = par1NBTTagCompound.getInteger("Score"); } /** * sets current screen to null (used on escape buttons of GUIs) */ public void closeScreen() { super.closeScreen(); this.mc.displayGuiScreen((GuiScreen)null); } /** * Displays the GUI for editing a sign. Args: tileEntitySign */ public void displayGUIEditSign(TileEntitySign par1TileEntitySign) { this.mc.displayGuiScreen(new GuiEditSign(par1TileEntitySign)); } /** * Displays the GUI for interacting with a book. */ public void displayGUIBook(ItemStack par1ItemStack) { Item var2 = par1ItemStack.getItem(); if (var2 == Item.writtenBook) { this.mc.displayGuiScreen(new GuiScreenBook(this, par1ItemStack, false)); } else if (var2 == Item.writableBook) { this.mc.displayGuiScreen(new GuiScreenBook(this, par1ItemStack, true)); } } /** * Displays the GUI for interacting with a chest inventory. Args: chestInventory */ public void displayGUIChest(IInventory par1IInventory) { this.mc.displayGuiScreen(new GuiChest(this.inventory, par1IInventory)); } /** * Displays the crafting GUI for a workbench. */ public void displayGUIWorkbench(int par1, int par2, int par3) { this.mc.displayGuiScreen(new GuiCrafting(this.inventory, this.worldObj, par1, par2, par3)); } public void displayGUIEnchantment(int par1, int par2, int par3) { this.mc.displayGuiScreen(new GuiEnchantment(this.inventory, this.worldObj, par1, par2, par3)); } /** * Displays the furnace GUI for the passed in furnace entity. Args: tileEntityFurnace */ public void displayGUIFurnace(TileEntityFurnace par1TileEntityFurnace) { this.mc.displayGuiScreen(new GuiFurnace(this.inventory, par1TileEntityFurnace)); } /** * Displays the GUI for interacting with a brewing stand. */ public void displayGUIBrewingStand(TileEntityBrewingStand par1TileEntityBrewingStand) { this.mc.displayGuiScreen(new GuiBrewingStand(this.inventory, par1TileEntityBrewingStand)); } /** * Displays the dipsenser GUI for the passed in dispenser entity. Args: TileEntityDispenser */ public void displayGUIDispenser(TileEntityDispenser par1TileEntityDispenser) { this.mc.displayGuiScreen(new GuiDispenser(this.inventory, par1TileEntityDispenser)); } public void displayGUIMerchant(IMerchant par1IMerchant) { this.mc.displayGuiScreen(new GuiMerchant(this.inventory, par1IMerchant, this.worldObj)); } /** * Called when the player performs a critical hit on the Entity. Args: entity that was hit critically */ public void onCriticalHit(Entity par1Entity) { this.mc.effectRenderer.addEffect(new EntityCrit2FX(this.mc.theWorld, par1Entity)); } public void onEnchantmentCritical(Entity par1Entity) { EntityCrit2FX var2 = new EntityCrit2FX(this.mc.theWorld, par1Entity, "magicCrit"); this.mc.effectRenderer.addEffect(var2); } /** * Called whenever an item is picked up from walking over it. Args: pickedUpEntity, stackSize */ public void onItemPickup(Entity par1Entity, int par2) { this.mc.effectRenderer.addEffect(new EntityPickupFX(this.mc.theWorld, par1Entity, this, -0.5F)); } /** * Returns if this entity is sneaking. */ public boolean isSneaking() { return this.movementInput.sneak && !this.sleeping; } /** * Updates health locally. */ public void setHealth(int par1) { int var2 = this.getHealth() - par1; if (var2 <= 0) { this.setEntityHealth(par1); if (var2 < 0) { this.hurtResistantTime = this.maxHurtResistantTime / 2; } } else { this.lastDamage = var2; this.setEntityHealth(this.getHealth()); this.hurtResistantTime = this.maxHurtResistantTime; this.damageEntity(DamageSource.generic, var2); this.hurtTime = this.maxHurtTime = 10; } } /** * Add a chat message to the player */ public void addChatMessage(String par1Str) { this.mc.ingameGUI.getChatGUI().func_73757_a(par1Str, new Object[0]); } /** * Adds a value to a statistic field. */ public void addStat(StatBase par1StatBase, int par2) { if (par1StatBase != null) { if (par1StatBase.isAchievement()) { Achievement var3 = (Achievement)par1StatBase; if (var3.parentAchievement == null || this.mc.statFileWriter.hasAchievementUnlocked(var3.parentAchievement)) { if (!this.mc.statFileWriter.hasAchievementUnlocked(var3)) { this.mc.guiAchievement.queueTakenAchievement(var3); } this.mc.statFileWriter.readStat(par1StatBase, par2); } } else { this.mc.statFileWriter.readStat(par1StatBase, par2); } } } private boolean isBlockTranslucent(int par1, int par2, int par3) { return this.worldObj.isBlockNormalCube(par1, par2, par3); } /** * Adds velocity to push the entity out of blocks at the specified x, y, z position Args: x, y, z */ protected boolean pushOutOfBlocks(double par1, double par3, double par5) { int var7 = MathHelper.floor_double(par1); int var8 = MathHelper.floor_double(par3); int var9 = MathHelper.floor_double(par5); double var10 = par1 - (double)var7; double var12 = par5 - (double)var9; if (this.isBlockTranslucent(var7, var8, var9) || this.isBlockTranslucent(var7, var8 + 1, var9)) { boolean var14 = !this.isBlockTranslucent(var7 - 1, var8, var9) && !this.isBlockTranslucent(var7 - 1, var8 + 1, var9); boolean var15 = !this.isBlockTranslucent(var7 + 1, var8, var9) && !this.isBlockTranslucent(var7 + 1, var8 + 1, var9); boolean var16 = !this.isBlockTranslucent(var7, var8, var9 - 1) && !this.isBlockTranslucent(var7, var8 + 1, var9 - 1); boolean var17 = !this.isBlockTranslucent(var7, var8, var9 + 1) && !this.isBlockTranslucent(var7, var8 + 1, var9 + 1); byte var18 = -1; double var19 = 9999.0D; if (var14 && var10 < var19) { var19 = var10; var18 = 0; } if (var15 && 1.0D - var10 < var19) { var19 = 1.0D - var10; var18 = 1; } if (var16 && var12 < var19) { var19 = var12; var18 = 4; } if (var17 && 1.0D - var12 < var19) { var19 = 1.0D - var12; var18 = 5; } float var21 = 0.1F; if (var18 == 0) { this.motionX = (double)(-var21); } if (var18 == 1) { this.motionX = (double)var21; } if (var18 == 4) { this.motionZ = (double)(-var21); } if (var18 == 5) { this.motionZ = (double)var21; } } return false; } /** * Set sprinting switch for Entity. */ public void setSprinting(boolean par1) { super.setSprinting(par1); this.sprintingTicksLeft = par1 ? 600 : 0; } /** * Sets the current XP, total XP, and level number. */ public void setXPStats(float par1, int par2, int par3) { this.experience = par1; this.experienceTotal = par2; this.experienceLevel = par3; } public void sendChatToPlayer(String par1Str) { this.mc.ingameGUI.getChatGUI().printChatMessage(par1Str); } /** * Returns true if the command sender is allowed to use the given command. */ public boolean canCommandSenderUseCommand(String par1Str) { return this.worldObj.getWorldInfo().areCommandsAllowed(); } }
-
Where is this code? In what class etc?
-
Not a problem
-
Could you post both the code for the ores and the entire code for the generation?
-
I've created multiple mods under the same workspace which use eachother. However, when updating to the latest version of Forge, whenever I run it it will throw this error: cpw.mods.fml.common.LoaderException: java.lang.IllegalArgumentException: Can not set static info.mineverse.willow.sabers.SaberBase field info.mineverse.willow.sabers.SaberBase.instance to info.mineverse.willow.hats.Hats at cpw.mods.fml.common.LoadController.transition(LoadController.java:102) at cpw.mods.fml.common.Loader.loadMods(Loader.java:459) at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:144) at net.minecraft.client.Minecraft.startGame(Minecraft.java:405) at net.minecraft.client.Minecraft.run(Minecraft.java:737) at java.lang.Thread.run(Unknown Source) Caused by: java.lang.IllegalArgumentException: Can not set static info.mineverse.willow.sabers.SaberBase field info.mineverse.willow.sabers.SaberBase.instance to info.mineverse.willow.hats.Hats at sun.reflect.UnsafeFieldAccessorImpl.throwSetIllegalArgumentException(Unknown Source) at sun.reflect.UnsafeFieldAccessorImpl.throwSetIllegalArgumentException(Unknown Source) at sun.reflect.UnsafeStaticObjectFieldAccessorImpl.set(Unknown Source) at java.lang.reflect.Field.set(Unknown Source) at cpw.mods.fml.common.FMLModContainer.parseSimpleFieldAnnotation(FMLModContainer.java:329) at cpw.mods.fml.common.FMLModContainer.processFieldAnnotations(FMLModContainer.java:255) at cpw.mods.fml.common.FMLModContainer.constructMod(FMLModContainer.java:349) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:124) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) at java.lang.reflect.Method.invoke(Unknown Source) at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:69) at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:317) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:300) at com.google.common.eventbus.EventBus.post(EventBus.java:268) at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:81) at cpw.mods.fml.common.Loader.loadMods(Loader.java:458) ... 4 more --- END ERROR REPORT a1bfc336 ---------- The Mods in the error change at what seems like random to any of the mods, even ones which aren't linked what so ever. What could the cause of this be?
-
Hello, I've set up a key binding that needs a packet sent to update the inventory. How would I use the keybinding method to create the packet and send it with the EntityPlayer without having an instance of EntityPlayer within the method?
-
Now I have this method; public void grantHorse(EntityPlayer par1EntityPlayer, World par2World) { EntityHorse entityHorse = new EntityHorse(par2World); entityHorse.setLocationAndAngles(par1EntityPlayer.posX, par1EntityPlayer.posY, par1EntityPlayer.posZ, par2World.rand.nextFloat() * 360.0F, 0.0F); par2World.spawnEntityInWorld(entityHorse); entityHorse.setOwner(par1EntityPlayer.username); BattleFoxCox.addHorseToList(entityHorse); } public void moveHorse(EntityPlayer par1EntityPlayer, EntityHorse par2EntityHorse, World par3World) { par2EntityHorse.setLocationAndAngles(par1EntityPlayer.posX, par1EntityPlayer.posY, par1EntityPlayer.posZ, par3World.rand.nextFloat() * 360.0F, 0.0F); } /** * Called whenever this item is equipped and the right mouse button is pressed. Args: itemStack, world, entityPlayer */ public ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { if (!par2World.isRemote) { if(BattleFoxCox.horses != null) { for(EntityHorse horse: BattleFoxCox.horses) { if(horse.getOwnerName().equalsIgnoreCase(par3EntityPlayer.username)) { this.moveHorse(par3EntityPlayer, horse, par2World); break; } } } else { this.grantHorse(par3EntityPlayer, par2World); } } return par1ItemStack; } How would I get it so that it triggers the moveHorse if the for each loop is not broken?
-
I have done that, and that's what produced the error EDIT: Found a way to do it, I'll reply after testing EDIT: Now have the method; public ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { if (!par2World.isRemote) { for(int x = 0; x < BattleFoxCox.horses.size(); x++) { EntityHorse horse = BattleFoxCox.horses.get(x); if(horse.getOwnerName().equalsIgnoreCase(par3EntityPlayer.username)) { horse.setLocationAndAngles(par3EntityPlayer.posX, par3EntityPlayer.posY, par3EntityPlayer.posZ, par2World.rand.nextFloat() * 360.0F, 0.0F); break; } if(x == BattleFoxCox.horses.size()) { EntityHorse entityHorse = new EntityHorse(par2World); entityHorse.setLocationAndAngles(par3EntityPlayer.posX, par3EntityPlayer.posY, par3EntityPlayer.posZ, par2World.rand.nextFloat() * 360.0F, 0.0F); par2World.spawnEntityInWorld(entityHorse); entityHorse.setOwner(par3EntityPlayer.username); BattleFoxCox.horses.add(entityHorse); } } } return par1ItemStack; } not tested, I'll review it tomorrow and then test, too late now.
-
My entity does save the owner's name. In that case how would I get the horse which has that player as its owner? The data which the horse currently saves is the owners username and the owners EntityPlayer instance. How could I get the instance of the Horse which has the owner as the user of the tool without editing base classes?
-
Hello, I'm trying to make a mod which you own an entity, but you can only own one entity. I have created a list object which uses the Players EntityID as the index and then the instance of EntityHorse as the listed object. I have this code to decide whether to teleport the horse or spawn one; public ItemStack onItemRightClick(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { if (!par2World.isRemote) { int playerID = par3EntityPlayer.entityId; if(BattleFoxCox.horses.get(playerID) != null) // line 39 { EntityHorse entityHorse = new EntityHorse(par2World); entityHorse.setLocationAndAngles(par3EntityPlayer.posX, par3EntityPlayer.posY, par3EntityPlayer.posZ, par2World.rand.nextFloat() * 360.0F, 0.0F); par2World.spawnEntityInWorld(entityHorse); entityHorse.setOwner(par3EntityPlayer.username); BattleFoxCox.horses.set(par3EntityPlayer.entityId, entityHorse); } else { EntityHorse entityHorse = BattleFoxCox.horses.get(playerID); entityHorse.setLocationAndAngles(par3EntityPlayer.posX, par3EntityPlayer.posY, par3EntityPlayer.posZ, par2World.rand.nextFloat() * 360.0F, 0.0F); } } return par1ItemStack; } I know it's probably horribly wrong but I've never done anything with entities before. When I try to use the item this error is thrown. 2012-09-26 18:27:36 [sEVERE] [ForgeModLoader] A critical server error occured handling a packet, kicking net.minecraft.src.NetServerHandler@11038df java.lang.NullPointerException at uk.co.toomuchminecraft.battlefoxcox.ItemHorseShoe.onItemRightClick(ItemHorseShoe.java:39) at net.minecraft.src.ItemStack.useItemRightClick(ItemStack.java:139) at net.minecraft.src.ItemInWorldManager.tryUseItem(ItemInWorldManager.java:306) at net.minecraft.src.NetServerHandler.handlePlace(NetServerHandler.java:478) at net.minecraft.src.Packet15Place.processPacket(Packet15Place.java:78) at net.minecraft.src.MemoryConnection.processReadPackets(MemoryConnection.java:75) at net.minecraft.src.NetServerHandler.networkTick(NetServerHandler.java:72) at net.minecraft.src.NetworkListenThread.networkTick(NetworkListenThread.java:55) at net.minecraft.src.IntegratedServerListenThread.networkTick(IntegratedServerListenThread.java:111) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:630) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:547) at net.minecraft.src.IntegratedServer.tick(IntegratedServer.java:105) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:453) at net.minecraft.src.ThreadServerApplication.run(ThreadServerApplication.java:17)
-
he he, thanks!
-
Hello, I am trying to code a horse. However, when the horse is ridden this happens; and I end up in the horse, how can I change where the player sits on the entity? Code:
-
Hello, when trying to spawn my entity into the world with; ModLoader.addSpawn(EntityHorse.class, 4, 1, 3, EnumCreatureType.creature); in my load method I get this error; 2012-09-21 16:20:52 [iNFO] [sTDERR] java.lang.NoSuchMethodException: uk.co.toomuchminecraft.battlefoxcox.EntityHorse.<init>(net.minecraft.src.World) 2012-09-21 16:20:52 [iNFO] [sTDERR] at java.lang.Class.getConstructor0(Unknown Source) 2012-09-21 16:20:52 [iNFO] [sTDERR] at java.lang.Class.getConstructor(Unknown Source) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.EntityList.createEntityFromNBT(EntityList.java:87) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.AnvilChunkLoader.readChunkFromNBT(AnvilChunkLoader.java:353) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.AnvilChunkLoader.checkedReadChunkFromNBT(AnvilChunkLoader.java:90) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.AnvilChunkLoader.loadChunk(AnvilChunkLoader.java:70) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.ChunkProviderServer.safeLoadChunk(ChunkProviderServer.java:147) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.ChunkProviderServer.loadChunk(ChunkProviderServer.java:96) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.initialWorldChunkLoad(MinecraftServer.java:269) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.IntegratedServer.loadAllDimensions(IntegratedServer.java:65) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.IntegratedServer.startServer(IntegratedServer.java:81) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:415) 2012-09-21 16:20:52 [iNFO] [sTDERR] at net.minecraft.src.ThreadServerApplication.run(ThreadServerApplication.java:17) I believe it's because the World is not getting passed through the constructor of EntityHorse, why would this be? here is EntityHorse.class; package uk.co.toomuchminecraft.battlefoxcox; import net.minecraft.src.AchievementList; import net.minecraft.src.EntityAIFollowParent; import net.minecraft.src.EntityAILookIdle; import net.minecraft.src.EntityAIMate; import net.minecraft.src.EntityAIPanic; import net.minecraft.src.EntityAISwimming; import net.minecraft.src.EntityAITempt; import net.minecraft.src.EntityAIWander; import net.minecraft.src.EntityAIWatchClosest; import net.minecraft.src.EntityAnimal; import net.minecraft.src.EntityPlayer; import net.minecraft.src.Item; import net.minecraft.src.ItemStack; import net.minecraft.src.NBTTagCompound; import net.minecraft.src.World; public class EntityHorse extends EntityAnimal { public String horseName = ""; public String ownerName = ""; public EntityHorse(World par1World, String par2) { super(par1World); ownerName = par2; if(ownerName.endsWith("s")) { horseName = ownerName + "' horse"; } else { horseName = ownerName + "'s horse"; } this.texture = "/uk/co/toomuchminecraft/battlefoxcox/textures/entities/horse.png"; this.setSize(0.9F, 0.9F); this.getNavigator().setAvoidsWater(true); float var2 = 0.25F; this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(1, new EntityAIPanic(this, 0.38F)); this.tasks.addTask(2, new EntityAIMate(this, var2)); this.tasks.addTask(3, new EntityAITempt(this, 0.25F, Item.wheat.shiftedIndex, false)); this.tasks.addTask(4, new EntityAIFollowParent(this, 0.28F)); this.tasks.addTask(5, new EntityAIWander(this, var2)); this.tasks.addTask(6, new EntityAIWatchClosest(this, EntityPlayer.class, 6.0F)); } /** * Returns true if the newer Entity AI code should be run */ public boolean isAIEnabled() { return true; } public int getMaxHealth() { return 20; } protected void entityInit() { super.entityInit(); this.dataWatcher.addObject(16, Byte.valueOf((byte)0)); } /** * (abstract) Protected helper method to write subclass entity data to NBT. */ public void writeEntityToNBT(NBTTagCompound par1NBTTagCompound) { super.writeEntityToNBT(par1NBTTagCompound); par1NBTTagCompound.setString("OwnerName", this.ownerName); par1NBTTagCompound.setString("HorseName", this.horseName); } /** * (abstract) Protected helper method to read subclass entity data from NBT. */ public void readEntityFromNBT(NBTTagCompound par1NBTTagCompound) { super.readEntityFromNBT(par1NBTTagCompound); this.ownerName = (par1NBTTagCompound.getString("OwnerName")); this.horseName = (par1NBTTagCompound.getString("HorseName")); } /** * Returns the sound this mob makes while it's alive. */ protected String getLivingSound() { return null; } /** * Returns the sound this mob makes when it is hurt. */ protected String getHurtSound() { return null; } /** * Returns the sound this mob makes on death. */ protected String getDeathSound() { return null; } /** * Called when a player interacts with a mob. e.g. gets milk from a cow, gets into the saddle on a horse. */ public boolean interact(EntityPlayer par1EntityPlayer) { ItemStack itemstack = par1EntityPlayer.inventory.getCurrentItem(); if (super.interact(par1EntityPlayer)) { return true; } else if (!this.worldObj.isRemote && par1EntityPlayer.getEntityName().equalsIgnoreCase(ownerName)) { par1EntityPlayer.mountEntity(this); return true; } else { this.setBeenAttacked(); return true; } } /** * Returns the item ID for the item the mob drops on death. */ protected int getDropItemId() { return Item.leather.shiftedIndex; } /** * Drop 0-2 items of this living's type */ protected void dropFewItems(boolean par1, int par2) { int var3 = this.rand.nextInt(3) + 1 + this.rand.nextInt(1 + par2); for (int var4 = 0; var4 < var3; ++var4) { if (this.isBurning()) { this.dropItem(Item.porkCooked.shiftedIndex, 1); } else { this.dropItem(Item.porkRaw.shiftedIndex, 1); } } } /** * Returns true if the horse is saddled. */ public boolean getSaddled() { return true; } /** * This function is used when two same-species animals in 'love mode' breed to generate the new baby animal. */ public EntityAnimal spawnBabyAnimal(EntityAnimal par1EntityAnimal) { return new EntityHorse(this.worldObj, this.ownerName); } }
-
No, the construction of the packet is within a class that only runs on ClientSide