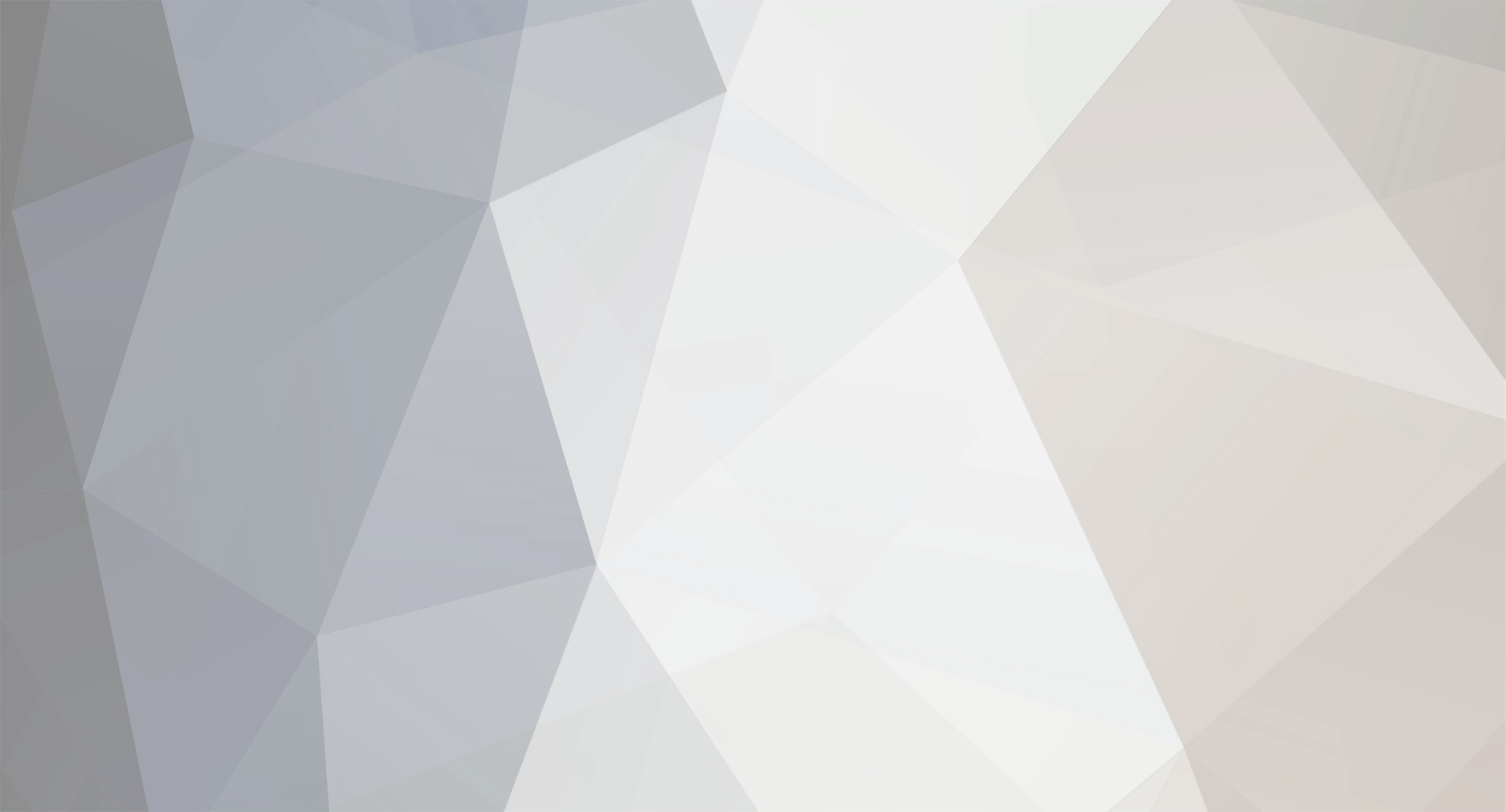
memcallen
Members-
Posts
343 -
Joined
-
Last visited
Everything posted by memcallen
-
Merging ItemStacks that are stored in an ArrayList
memcallen replied to memcallen's topic in Modder Support
So I have to redo it pretty much... -
Merging ItemStacks that are stored in an ArrayList
memcallen replied to memcallen's topic in Modder Support
same as before -
Merging ItemStacks that are stored in an ArrayList
memcallen replied to memcallen's topic in Modder Support
I changed it to: item = items.get(items.size()-1).getEntityItem(); if(CrucibleRecipeHandler.alloweds.contains(item.getItem())){ int Counter=0; for(ItemStack i : inventory){ Counter++; if(i.isItemEqual(item)) if(inventory.get(Counter)!=null) if(i.isItemEqual(inventory.get(Counter-1))){ inventory.add(new ItemStack(i.getItem(),i.stackSize+inventory.get(Counter-1).stackSize)); break; } } if(inventory.get(Counter-1)!=null) inventory.remove(Counter-1); Counter=0; inventory.add(item); items.get(items.size()-1).setDead(); item = null; } it still doesn't work, keep getting crashes on my null check... "if(inventory.get(Counter-1)!=null)" -
GameRegistry.registerBlock(yourblock,savegameID) the savegameID is for when you do "/give player yourMODID:savegameID" I'm not sure if it's your modid but that's basically what it's used for, except internally.
-
when you set your Item's unlocalized name with .setUnlocalizedName("randomtext"), in your .lang file put: Item.youritemsname.name=item's ingame name
-
this code isn't working (as in it crashes with NullPointerException): @Override public void updateTick(World world,int x,int y,int z,Random rand){ CrucibleTileEntity te = (CrucibleTileEntity)world.getTileEntity(x, y, z); List<EntityItem> items = world.getEntitiesWithinAABB(EntityItem.class, AxisAlignedBB.getBoundingBox(x+0D, y+1D, z+0D, x+1D, y+2D, z+1D)); ItemStack item=null; if(items.size()>0){ item = items.get(items.size()-1).getEntityItem(); if(CrucibleRecipeHandler.alloweds.contains(item.getItem())){ int Counter=0; for(ItemStack i : inventory){ Counter++; if(i.isItemEqual(item)) if(i.isItemEqual(inventory.get(Counter-1))){ inventory.add(new ItemStack(i.getItem(),i.stackSize+inventory.get(Counter-1).stackSize)); inventory.remove(Counter-1); break; } } Counter=0; inventory.add(item); items.get(items.size()-1).setDead(); item = null; } inventory is an ArrayList stored outside the function. Yes I realize I have to use a tileentity etc, this is just for testing purposes.
-
I'm not good at finding things, sorry.
-
What's the location of the petal Apothecary class? I can't find it.
-
(I have no idea what to call this topic) I'm creating something similar to botania's petal apothecary, and I have no idea how to store the recipes. I don't want to store an ItemStack array because then I would have to check the order, etc. How could I do this so that there is no specific order you put the items in?
-
Why are you doing setFull3D twice? Once in item class and in main class. Also does the resource location in the ItemRenderer work like that? I thought you had to do modid:texturepath.
-
I figured it out thanks.
-
Is there already a tree generator? I'm using WorldGenBigTree and I don't want to have to make my own if I don't need to.
-
I need to use IWorldGenerator right?
-
Is there a tree generation event? I want to be able to replace all trees in my dimension with my custom tree, or is there a way to disable tree generation? (I can make my own tree generator if I need to)
-
yeah I was planning on adding special drops, like a precious mineral (diamond etc.) block would drop a shard of the same kind.
-
MrCaracal, could I detect the harvest level of the block and if it isn't 0 then drop the blocks with the method I'm using now?
-
Yes but the thing is, is that I would need a TON of code for what i'm making. It's basically a tool that can break certain blocks depending on the NBT, kind of like a super Tinkerer's Construct tool. I have the part that breaks the blocks at different speeds but if I break a block that you can break with your fist and get an item, it drops two items. Here's the onBlockDestroyed function: public boolean onBlockDestroyed(ItemStack itemstack,World world, Block block,int x,int y,int z,EntityLivingBase elb){ List<ItemStack> drops = block.getDrops(world, x, y, z, world.getBlockMetadata(x,y,z), 0); for(ItemStack is:drops){ if(!world.isRemote) world.spawnEntityInWorld(new EntityItem(world,x,y,z,is)); } return true; } so it's an "artificial" tool if you want to call it that. It doesn't actually extend ItemTool.
-
I created an Item, it's essentially a customizable tool. I can't make it detect when you break a block though. Also this code doesn't work: public float getDigSpeed(ItemStack itemstack, Block block, int metadata){ NBTTagCompound nbt = itemstack.getTagCompound(); try{ if(nbt!=null){ System.out.println("has NBT"); speed = nbt.getInteger("Engine"); tool = nbt.getInteger("Tool"); }else System.out.println("Doesn't have NBT"); }catch(Exception e){e.printStackTrace();} if(block.getMaterial()==Material.rock){ if(tool==1){ return speed*10; } } if(block.getMaterial()==Material.wood){ if(tool==2){ return speed*6; } } if(block.getMaterial()==Material.ground){ return speed*3; } return super.getDigSpeed(itemstack, block, metadata)+1F; } EDIT: scratch that, I made it work, but it drops two items when I do break the block. how could I fix this?- also it's only when I break a block that you can break with your fist.
-
how could I make it so that it isn't grayed out or moving up and down? Also, thanks GL11.glscaled works
-
also how could I scale the Item down?
-
I tried using RenderManager, but the item just moves up and down really quickly. Also it's grayed out, I tried disabling GL11.gl_lighting but it doesn't work.
-
I've made a crafting table, but I want to render the ItemStack on top of the block. I made a TESR but I can't figure out how to render the item.
-
TileEntity inventory without using IInventory?
memcallen replied to memcallen's topic in Modder Support
ok cool I got it to work. I changed my code to: package com.example.gammacraft.TileEntity; import net.minecraft.entity.item.EntityItem; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.NetworkManager; import net.minecraft.network.Packet; import net.minecraft.network.play.server.S35PacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; import net.minecraft.world.World; public class MultiToolModifierTileEntity extends TileEntity { public ItemStack[] inv; public MultiToolModifierTileEntity() { inv=new ItemStack[10]; } public Packet getDescriptionPacket() { NBTTagCompound nbtTag = new NBTTagCompound(); this.writeToNBT(nbtTag); return new S35PacketUpdateTileEntity(this.xCoord, this.yCoord, this.zCoord, 1, nbtTag); } public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); } public void writeToNBT(NBTTagCompound nbt){ super.writeToNBT(nbt); for(int i =0;i!=inv.length;i++){ NBTTagCompound NBT = new NBTTagCompound(); if(inv[i]!=null){ inv[i].writeToNBT(NBT); nbt.setTag("ItemStack"+i,NBT); } System.out.println("saving:"+i); } this.markDirty(); } public void readFromNBT(NBTTagCompound nbt){ super.readFromNBT(nbt); for(int i =0;i!=inv.length;i++){ NBTTagCompound itemstack = nbt.getCompoundTag("ItemStack"+i); try{ inv[i]=ItemStack.loadItemStackFromNBT(itemstack); }catch(Exception e){ e.printStackTrace(); } System.out.println("reading:"+i); } } } -
TileEntity inventory without using IInventory?
memcallen replied to memcallen's topic in Modder Support
Where am I setting the itemstack twice? I have no idea what markdirty does The rest will be added when I polish up the code, right now I just want something that works