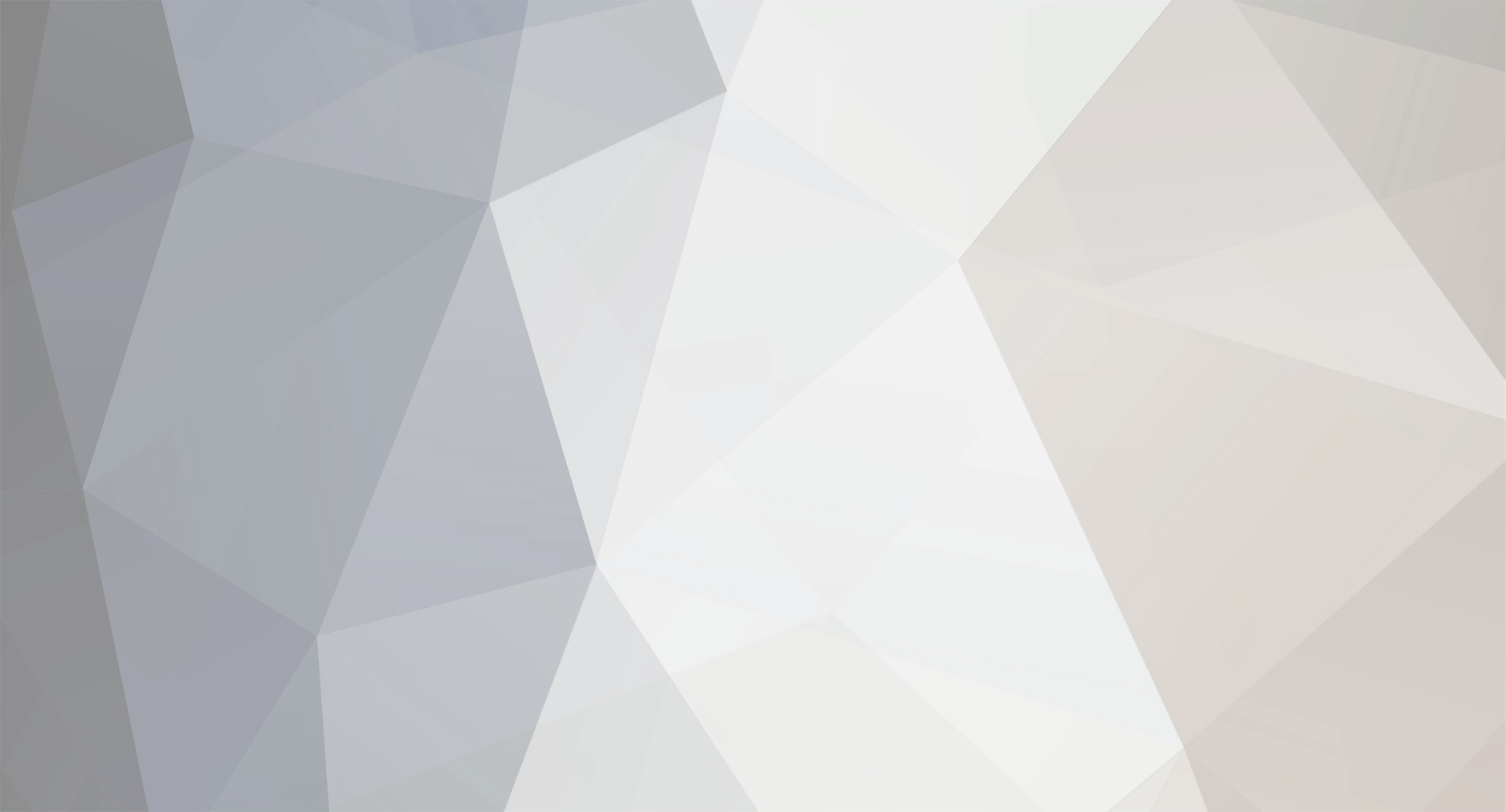
memcallen
Members-
Posts
343 -
Joined
-
Last visited
Everything posted by memcallen
-
I've made this laser block, it creates a laser beam when powered by redstone. The beam is supposed to be 100 blocks long, when I power it the beam doesn't even show up. heres the onNeighborBlockChange function: public void onNeighborBlockChange(World world, int x, int y, int z, Block block){ System.out.println("X:"+x+" Y:"+y+" Z:"+z); if(world.isBlockIndirectlyGettingPowered(x, y, z)){ System.out.println(true); int meta = world.getBlockMetadata(x, y, z); ForgeDirection dir = ForgeDirection.getOrientation(meta); BlockLaserTileEntity te = (BlockLaserTileEntity)world.getTileEntity(x, y, z); for(int i = 0; i==100;i++){ int tx = (dir.offsetX*i)+x,ty = (dir.offsetY*i)+y,tz = (dir.offsetZ*i)+z; System.out.println("X:"+tx+" Y:"+ty+" Z:"+tz); world.setBlock(tx, ty, tz, gammacraft.LaserBeam); world.scheduleBlockUpdate(tx, ty, tz, (Block)this, 10); } } } it prints the x/y/z and when there is a redstone signal, it prints true. It doesn't print tx/ty/tz because it would spam the console with 100 lines but it doesn't. I have no idea why this isn't working. -sidenote this is just a prototype, I will add extra bits to it like it stops when it hits a block.
-
I should've used that a long time ago, thanks.
-
I quite literally have no idea how...
-
So I'm guessing no one knows how it works?
-
I have a block that changes colour depending on metadata but for whatever reason it resets the metadata every frame. heres the code: package com.example.gammacraft.PlasmaTech; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.util.IIcon; public class LaserBeam extends Block { public LaserBeam() { super(Material.fire); this.setBlockName("LaserBeam"); this.setBlockUnbreakable(); } //colours are in "rainbow" configuration @SideOnly(Side.CLIENT) public static IIcon Red; @SideOnly(Side.CLIENT) public static IIcon Orange; @SideOnly(Side.CLIENT) public static IIcon Yellow; @SideOnly(Side.CLIENT) public static IIcon Green; @SideOnly(Side.CLIENT) public static IIcon Blue; @SideOnly(Side.CLIENT) public static IIcon Purple; @SideOnly(Side.CLIENT) public static IIcon White; @SideOnly(Side.CLIENT) public static IIcon Void; public void registerBlockIcons(IIconRegister i){ this.Red = i.registerIcon("gammacraft:RedConstructionBlock"); //red this.Orange = i.registerIcon("gammacraft:Orange"); //orange this.Yellow = i.registerIcon("gammacraft:Yellow"); //yellow this.Green = i.registerIcon("gammacraft:Green"); //green this.Blue = i.registerIcon("gammacraft:BlueConstructionBlock"); //blue this.Purple = i.registerIcon("gammacraft:Purple"); //purple this.White = i.registerIcon("gammacraft:White"); //white this.Void = i.registerIcon("gammacraft:Void"); //"void" } public IIcon getIcon(int side, int meta){ /*switch(meta){ case 0:return Red; case 1:return Orange; case 2:return Yellow; case 3:return Green; case 4:return Blue; case 5:return Purple; case 6:return White; case 7:return Void; default:return White; }*/ System.out.println(meta); if(meta==0)return Red; if(meta==1)return Orange; if(meta==2)return Yellow; if(meta==3)return Green; if(meta==4)return Blue; if(meta==5)return Purple; if(meta==6)return White; if(meta==7)return Void; return Void; } } I made an item that sets the metadata as a debug item, if you need the code here it is: package com.example.gammacraft.items; import com.example.gammacraft.RandomFunctions; import com.example.gammacraft.gammacraft; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; public class MetaDataSetter extends Item { public MetaDataSetter() { this.setCreativeTab(gammacraft.PlamsaTech); this.setUnlocalizedName("MetaDataSetter"); this.setMaxStackSize(1); } @Override public boolean onItemUse(ItemStack itemstack,EntityPlayer player,World world,int x,int y,int z,int side,float hx,float hy,float hz){ if(itemstack.stackTagCompound!=null){ System.out.println(itemstack.stackTagCompound.getInteger("Meta")); world.setBlockMetadataWithNotify(x, y, z, itemstack.stackTagCompound.getInteger("Meta"), 0); } return player.isSneaking(); } public ItemStack onItemRightClick(ItemStack itemstack,World world,EntityPlayer player){ if(itemstack.stackTagCompound==null){ itemstack.setTagCompound(new NBTTagCompound()); } if(player.isSneaking()){ NBTTagCompound nbt = itemstack.stackTagCompound; int Meta = nbt.getInteger("Meta"); if(Meta==15){ Meta = 0; }else{ Meta++; } if(!world.isRemote) RandomFunctions.tell(player, "Meta:"+Meta); nbt.setInteger("Meta", Meta); itemstack.setTagCompound(nbt); } return itemstack; } } EDIT: I figured it out, turns out it doesn't like having a material of fire.
-
bump
-
I want to know how thaumcraft's warding wand (or whatever it's called now) works. Mainly the "force field" part of it. I want to implement something like a force field in my mod but I don't want to make something like MFFS.
-
what is the easiest way to render a circle?
memcallen replied to memcallen's topic in Modder Support
I think I'm just gonna use a texture, it looks easier to do. -
Does that mean that world generators are also server side?
-
what is the easiest way to render a circle?
memcallen replied to memcallen's topic in Modder Support
I was planning on using the tessellator but I wasn't sure if there was some open GL wizardry that could draw a circle instead of me making a texture for it. Or some other renderer that can handle circles. -
I'm making something like ars magica's nexi and I was wondering what the easiest way to render a circle is.
-
I was wondering if dimensions were server-side. Obviously they couldn't contain any blocks not on the client, but is the world gen server side? I want to know because I've seen some servers with a farm world that has a dimension ID of -28 etc (they're usually using the overworld generation though).
-
I made it spawn a half block above the normal one and now it works properly.
-
The block has no collision box. Does it matter?
-
I made a machine that "throws" an Item into the world. It spawns but it goes flying off towards west even though I set the velocity to 0,0,0. I can't figure out why is does this. heres the code that spawns the item: EntityItem item = new EntityItem(world,x,y,z,drop); item.setVelocity(0,0,0); item.velocityChanged=true; world.spawnEntityInWorld(item);
-
How does extra utilitie's impossible object render?
memcallen replied to memcallen's topic in Modder Support
the mod isn't opensource (I'm talking about rwtema's mod if you where wondering). I checked, theres just a readme file. I was wondering if he used a tessellator or some kind of crazy model. and what the modelling software was if it's a model(I didn't ask this in the first topic, I was just wondering). -
I'm wondering how extra utilitie's impossible object renders, I know it uses a TESR/ISBR and that's about it. I was thinking it used a turbo model thingy from techne but they don't render properly in minecraft. Any help?
-
The proper forge files, I got the universal and the Installer. I have no idea where to go from there. (I made my mod a couple months ago)
-
Yeah I tried that, but I couldn't figure out what I was doing wrong. All I know is that I couldn't get the proper files. Also I changed the line to "player.motionY += 1D;" and it works.
-
1.I don't know how to update 2.again, I don't know how to update
-
gradlew setupDecompWorkspace eclipse: says "FAILURE: Build failed with an exception" then it says that I need java 7 and it's fixed with forgegradle 1.2
-
I have a different version installed, so it didn't work. I tried installing java 7 but it still doesn't work.
-
I have to install java 7...this sucks EDIT: I installed java 7, it still doesn't work