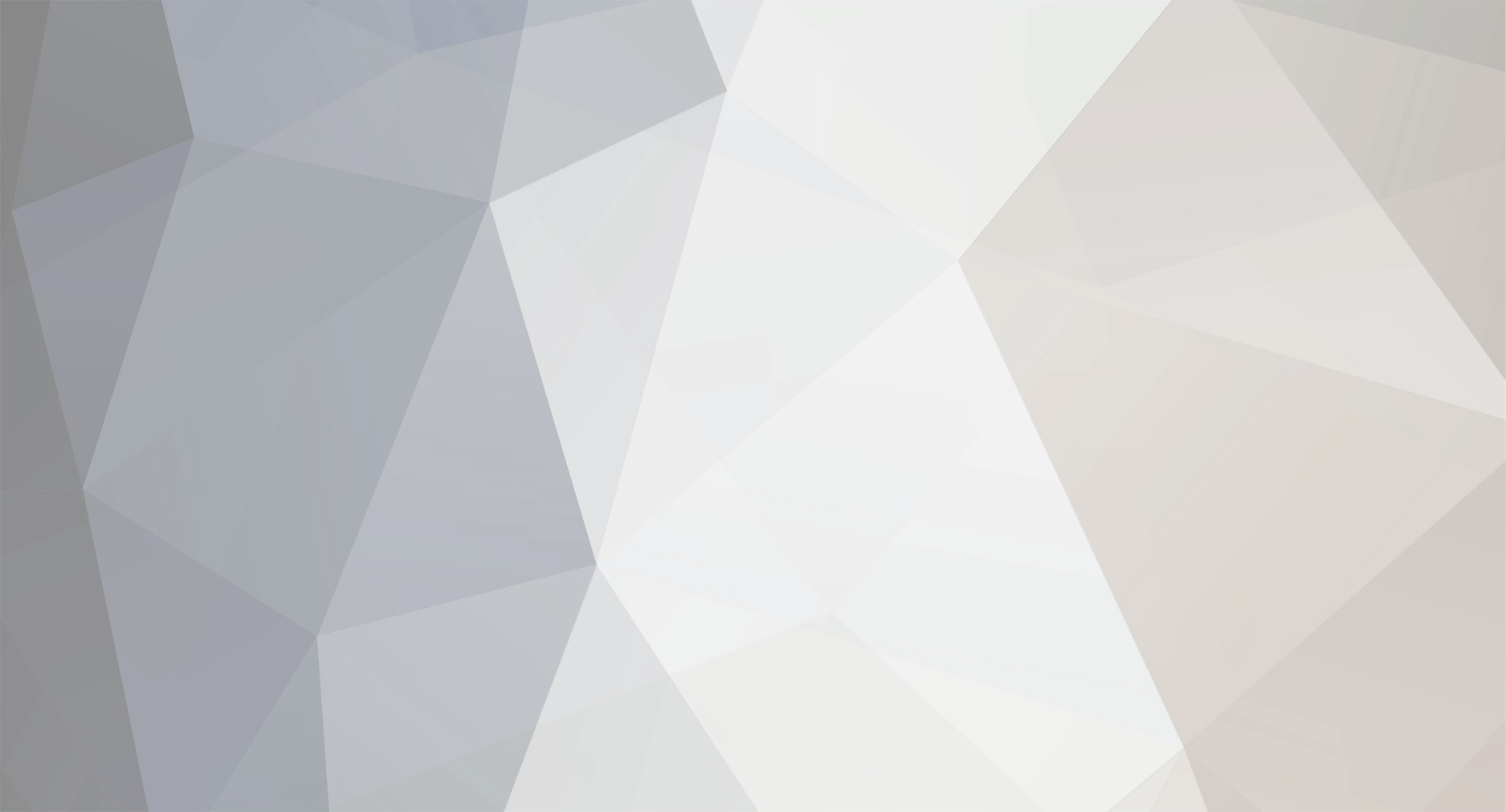
EverythingGames
Forge Modder-
Posts
388 -
Joined
-
Last visited
Everything posted by EverythingGames
-
I have a B3D model made in blender. It has no texture, it just has colors applied to the material / mesh, however, in-game it does not take effect. Here is my .JSON code and my model loading code. JSON { "forge_marker": 1, "variants": { "inventory": { "model": "armed:m4.b3d", "transform": "forge:default-tool" } } } Loading ModelLoaderRegistry.registerLoader(B3DLoader.instance); B3DLoader.instance.addDomain(MainReference.ID); ModelLoader.setCustomModelResourceLocation(MainInitialization.ITEMS.get("m4"), 0, new ModelResourceLocation(MainReference.ID + ":" + "m4", "inventory")); Does the B3DLoader not support this or do I have to do something in the .JSON to tell it to use the model's material colors? If anyone knows how this works and could tell me, I'd be appreciative.
-
[1.8] Model scaling on the ground/in item frames
EverythingGames replied to TheNuclearDuck's topic in Modder Support
Still pretty involved either way. Your method would be preferred IMO. Still, though, its hardly any waste of memory, especially if you check the instance of the item being tossed / picked up - if tossed / picked up item == your item. Anyway, the main reason why I would use Ernio's solution is because you would have full, direct access to the EntityItem itself and GL (rather than having to scale up an existing model and forming a larger one out of it). Anyways, hope the rendering goes well for you. -
[1.8] Model scaling on the ground/in item frames
EverythingGames replied to TheNuclearDuck's topic in Modder Support
I have a fix - may not be the best one, but its the only thing that I can think of - it may be involved. Each entity item is spawned for an itemstack - this means the itemstack's model is reflected by the entity item when the player throws / drag-drops an item into the world. With that logic in mind, you could return your regular sized model when the player is holding the item, and return your larger sized model when it is an entity item. Here is how you would do this: 1.) On the ItemTossEvent, set a simple NBT Boolean to the thrown itemstack (this tells you your item is an entity item) 2.) On the ItemPickupEvent, set the Boolean from above to false (this tells you your item is no longer an entity item) 3.) Implement ISmartItemModel. In the handlePerspective method, return the bigger model if the Boolean is true, and the regular sized model if the Boolean is false (entity item = true: large model | entity item = false : regular model) 4.) Register the ISmartItemModel type in the ModelBakeEvent and map it to your item's regular model. (ModelResourceLocation) This will work if you know what your doing - its simple really. I actually did exactly this to change my model altogether. Anyway, hope that gives you an idea. EDIT: Realized you also wanted to control the model when in an Item Frame - I believe this can be done in IPerspectiveAwareModel (TransformType.NONE). If that somehow isn't the case, you can always use the RenderEntityItemFrameEvent to set another itemstack Boolean and then check it in ISmartItemModel. (BTW sorry if some of the event names aren't correct, they should be very similar). -
[SOLVED] [1.8] GUI Rendering GL Alpha Problem
EverythingGames replied to EverythingGames's topic in Modder Support
Here you are: Replacing Vanilla GUI Inventory @SubscribeEvent public void onEvent(GuiOpenEvent event) { if(event.gui instanceof GuiInventory) { event.setCanceled(true); Minecraft.getMinecraft().displayGuiScreen(new GuiInventoryTextured(Minecraft.getMinecraft().thePlayer)); } } My Custom GUI Inventory @SideOnly(Side.CLIENT) public class GuiInventoryTextured extends InventoryEffectRenderer { private float oldMouseX; private float oldMouseY; public GuiInventoryTextured(EntityPlayer entityplayer) { super(entityplayer.inventoryContainer); this.allowUserInput = true; } @Override public void updateScreen() { if (this.mc.playerController.isInCreativeMode()) { this.mc.displayGuiScreen(new GuiContainerCreative(this.mc.thePlayer)); } this.updateActivePotionEffects(); } @Override public void initGui() { this.buttonList.clear(); if (this.mc.playerController.isInCreativeMode()) { this.mc.displayGuiScreen(new GuiContainerCreative(this.mc.thePlayer)); } else { super.initGui(); } } @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { GlStateManager.pushMatrix(); GlStateManager.enableAlpha(); GlStateManager.enableBlend(); this.fontRendererObj.drawString(I18n.format("container.crafting", new Object[0]), 86, 16, 4210752); GlStateManager.disableBlend(); GlStateManager.disableAlpha(); GlStateManager.popMatrix(); } @Override public void drawScreen(int mouseX, int mouseY, float partialTicks) { super.drawScreen(mouseX, mouseY, partialTicks); this.oldMouseX = (float)mouseX; this.oldMouseY = (float)mouseY; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.pushMatrix(); GlStateManager.enableAlpha(); GlStateManager.enableBlend(); this.mc.getTextureManager().bindTexture(new ResourceLocation(MainReference.ID + ":" + "textures/gui/inventory.png")); int k = this.guiLeft; int l = this.guiTop; this.drawTexturedModalRect(k, l, 0, 0, this.xSize, this.ySize); drawEntityOnScreen(k + 51, l + 75, 30, (float)(k + 51) - this.oldMouseX, (float)(l + 75 - 50) - this.oldMouseY, this.mc.thePlayer); GlStateManager.disableBlend(); GlStateManager.disableAlpha(); GlStateManager.popMatrix(); } public static void drawEntityOnScreen(int p_147046_0_, int p_147046_1_, int p_147046_2_, float p_147046_3_, float p_147046_4_, EntityLivingBase p_147046_5_) { GlStateManager.enableColorMaterial(); GlStateManager.pushMatrix(); GlStateManager.translate((float)p_147046_0_, (float)p_147046_1_, 50.0F); GlStateManager.scale((float)(-p_147046_2_), (float)p_147046_2_, (float)p_147046_2_); GlStateManager.rotate(180.0F, 0.0F, 0.0F, 1.0F); float f2 = p_147046_5_.renderYawOffset; float f3 = p_147046_5_.rotationYaw; float f4 = p_147046_5_.rotationPitch; float f5 = p_147046_5_.prevRotationYawHead; float f6 = p_147046_5_.rotationYawHead; GlStateManager.rotate(135.0F, 0.0F, 1.0F, 0.0F); RenderHelper.enableStandardItemLighting(); GlStateManager.rotate(-135.0F, 0.0F, 1.0F, 0.0F); GlStateManager.rotate(-((float)Math.atan((double)(p_147046_4_ / 40.0F))) * 20.0F, 1.0F, 0.0F, 0.0F); p_147046_5_.renderYawOffset = (float)Math.atan((double)(p_147046_3_ / 40.0F)) * 20.0F; p_147046_5_.rotationYaw = (float)Math.atan((double)(p_147046_3_ / 40.0F)) * 40.0F; p_147046_5_.rotationPitch = -((float)Math.atan((double)(p_147046_4_ / 40.0F))) * 20.0F; p_147046_5_.rotationYawHead = p_147046_5_.rotationYaw; p_147046_5_.prevRotationYawHead = p_147046_5_.rotationYaw; GlStateManager.translate(0.0F, 0.0F, 0.0F); RenderManager rendermanager = Minecraft.getMinecraft().getRenderManager(); rendermanager.setPlayerViewY(180.0F); rendermanager.setRenderShadow(false); rendermanager.renderEntityWithPosYaw(p_147046_5_, 0.0D, 0.0D, 0.0D, 0.0F, 1.0F); rendermanager.setRenderShadow(true); p_147046_5_.renderYawOffset = f2; p_147046_5_.rotationYaw = f3; p_147046_5_.rotationPitch = f4; p_147046_5_.prevRotationYawHead = f5; p_147046_5_.rotationYawHead = f6; GlStateManager.popMatrix(); RenderHelper.disableStandardItemLighting(); GlStateManager.disableRescaleNormal(); GlStateManager.setActiveTexture(OpenGlHelper.lightmapTexUnit); GlStateManager.disableTexture2D(); GlStateManager.setActiveTexture(OpenGlHelper.defaultTexUnit); } protected void actionPerformed(GuiButton button) throws IOException { if (button.id == 0) { this.mc.displayGuiScreen(new GuiAchievements(this, this.mc.thePlayer.getStatFileWriter())); } if (button.id == 1) { this.mc.displayGuiScreen(new GuiStats(this, this.mc.thePlayer.getStatFileWriter())); } } } Only Modified Method In My Custom GUI Inventory (GUI code is copied from GuiInventory and this method is modified only) @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.pushMatrix(); GlStateManager.enableAlpha(); GlStateManager.enableBlend(); this.mc.getTextureManager().bindTexture(new ResourceLocation(MainReference.ID + ":" + "textures/gui/inventory.png")); int k = this.guiLeft; int l = this.guiTop; this.drawTexturedModalRect(k, l, 0, 0, this.xSize, this.ySize); drawEntityOnScreen(k + 51, l + 75, 30, (float)(k + 51) - this.oldMouseX, (float)(l + 75 - 50) - this.oldMouseY, this.mc.thePlayer); GlStateManager.disableBlend(); GlStateManager.disableAlpha(); GlStateManager.popMatrix(); } I don't know where the slots for the armor are rendered - I'm pretty sure it is causing the issue since the alpha changes when I hover over the slots (also, same for the crafting grid). -
[1.8] How Do I Use IModel - What Is It
EverythingGames replied to EverythingGames's topic in Modder Support
So how would I use it? How do I register this to my item? -
I've heard that you can use independent transform values with two different models using IModel. Is that true? Can someone explain to me what an IModel is, what it does, and why it differs from IBakedModel? Also, can you use IBakedModel with an IModel? How do you register the IModel? Much appreciated, thanks.
-
Brightness in ISimpleBlockRenderingHandler [1.7.10]
EverythingGames replied to MFMods's topic in Modder Support
Use GL11 or GlStateManager where you need it if you can. -
Then, compare block coordinates (blockpos) to player coordinates to figure the distance in X, Y, and Z.
-
[1.8] [SOLVED] Additional Data from JSONs?
EverythingGames replied to Sgmjscorp's topic in Modder Support
Why don't you create your own .JSON file reader - search for the file the user creates with the model information, parse it to whatever you need, then apply to the item model. Really, I don't see why you would do this with this .JSON system, pretty flexible with rotations / translations / scale. -
I have a custom GuiScreen that I open on the GuiOpenEvent. It replaces the GuiIventory Gui. The problem I have is that the new GUI that I render's texture is 100% alpha, with different levels of alpha. It all works by making GL calls to enable / disable the alpha and blend, but I have a weird problem for when the player hovers over the 2x2 crafting grid or the armor slots - the alpha level darkens for the slots. What is causing this? Why is the alpha changing when I hover over only the armor slots and crafting grid? Where are the armor slot icons (the icons displaying the different types of armors) rendered? If anyone can help me out I'd appreciate it.
-
You know what TGG said earlier about copying the code from the .JSON to generate the quads yourself? First, make a regular block model in .JSON and register it normally. Then, wrap it in the IBakedModel type to change the color or do whatever you need. Do this in the ModelBakeEvent.
-
Search for tutorials on how to texture models in Blender. Exporting as an .OBJ requires you to have a .MTL file as well, containing all of your materials / meshes. For a triangular model, it's pretty simple in blender, basically you go into UV editing mode, export your UV template, create your textures, create a new mesh, import your texture that you made, and map them to your model. After that, export it and you should be good. I am no expert in Blender or Sketchup by any means - go watch a tutorial. (BTW you can also use pre-made textures and map them to your model, if you have them)
-
[1.7.10] How to properly extend ItemBow?
EverythingGames replied to kauan99's topic in Modder Support
I imagine it would be something like this (a little code example). public class ExampleBow extends ItemBow { public static final String[] ICONNAMES = new String[] {"modid:pulling_0", "modid:pulling_1", "modid:pulling_2"}; /** We call super to initialize the values in the parent constructor - if you don't you may modify these values **/ public ExampleBow() { super(); } /** We call the super method to execute it instead - if you want to spawn an arrow with more damage, ignore the super call and modify **/ @Override public void onPlayerStoppedUsing(ItemStack stack, World worldIn, EntityPlayer playerIn, int timeLeft) { super.onPlayerStoppedUsing(stack, worldIn, playerIn, timeLeft); } } -
[1.7.10] How to properly extend ItemBow?
EverythingGames replied to kauan99's topic in Modder Support
You could just extend ItemBow and override what you need to make changes to. Extending it would only allow you to get away with not writing all of the methods because its already done for you. You could also create a class extending item, override the methods onItemRightClick, getMaxItemUseDuration, onItemStoppedUsing, getEnumAction, etc. It shouldn't be hard at all, all the information is in the bow class itself ready to use. Also, one thing to note is that the bow's damage depends on the entity arrow being spawned. It is increased depending on the bow's use time (this is seen in the EntityArrow class). -
To make a successful block, follow my instructions. Registry 1.) Register your block with it's unlocalized name. 2.) Register your block on the ItemModelMesher using the unlocalized name. Files 1.) You must create the model you registered for above for the block seen in the world. It's name should match the unlocalized name. 2.) Also create the model for the block's item seen in your inventory. It should also match the unlocalized name and be placed in the assets/modid/models/item package. 3.) Create your blockstate file for your block. 4.) Double check all code and texture paths both inside Java and .JSON.
-
[1.8] Change world weather based on events
EverythingGames replied to JimiIT92's topic in Modder Support
Are you calling this on the server? -
Trust me, PlanetMinecraft is one of the largest Minecraft resource distributors out there. Websites that you don't need to fool with are sites like 9Minecrafft or MinecraftDL - their names are "cringe-worthy." Anyway, PlanetMinecraft is totally safe - which leads me to this question - where have you been getting your mods, skins, resource packs, etc. (hopefully Curse)? Discussions about correct sites for Minecraft related resources can go on and on, but I assure you PlanetMinecraft is safe, TGG wouldn't post there if it wasn't. If you however choose not to use the tool for whatever reason, I wish you the best of luck transforming your items! Trust me, its time consuming and repetitive (for a staff like your making it shouldn't be a problem at all). Good luck!
-
Yes, it prints the values you need to the console when your done transforming your items. Read the page for more info and try it yourself. No, PlanetMinecraft doesn't have any viruses...why would it? You have visited this page before haven't you?
-
I recommend MrCrayfish's model creator to create your models in .JSON (can be complex models), while also using TheGreyGhosts transform tool to position the models in-game. Here are the links: ModelCreator http://www.mrcrayfish.com/tools.php?id=mc Transform Tool http://www.planetminecraft.com/mod/item-transform-helper---interactively-rotate-scale-translate/
-
[1.8] Alternative for ISimpleBlockRenderingHandler
EverythingGames replied to Bedrock_Miner's topic in Modder Support
Sorry, how exactly can I register a model? I've only registered the model files so far (and used the normal blockstates file). You should register your block .JSON and blockstate like normal. After that, create an instance of your block's ModelResourceLocation and use the ModelBakeEvent to extract the IBakedModel from it. Then, again use the ModelBakeEvent to replace the ModelResourceLocation with your custom class implementing IBakedModel. Here is an example below. public void onEvent(ModelBakeEvent event) { final IBakedModel bakedmodel = event.modelManager.getModel(YOURMODELRESOURCELOCATION); final YourIBakedModelTypeClass youribakedmodeltypeclass = new YourIBakedModelTypeClass(bakedmodel); event.modelRegistry.putObject(YOURMODELRESOURCELOCATION, youribakedmodeltypeclass ); } -
You can't create models to render for items in Techne anymore since IItemRenderer was removed. Use the new .JSON modeling system to create 3D models.
-
[SOLVED] [1.8] Adding Variant Names To Item For Models
EverythingGames replied to EverythingGames's topic in Modder Support
Just solved it a few minutes ago. The class registries are custom wrote, but the solution should be straight forward for others. * Called by the Client Proxy * public static void initializeBlockAndItemRenders() { ManagerRegistry.registerBlockRender(MainInitialization.BLOCKS); ManagerRegistry.registerItemRender(MainInitialization.ITEMS); ManagerRegistry.registerItemRender("ak74ugui", 1, MainInitialization.ITEMS.get("ak74u")); ModelBakery.addVariantName(MainInitialization.ITEMS.get("ak74u"), MainReference.ID + ":" + "ak74u", MainReference.ID + ":" + "ak74ugui"); } -
Hi. I have an item model wrapper class setup implementing IPerspectiveAwareModel. This wrapper class returns the default item model for first person, third person, head, and none. For the transform type GUI, It returns a built-in/generated model (regular texture model). The only problem is that I can't seem to add the GUI model to the item with meta and its modelresourcelocation. Any idea why? Here is the code I tried, thanks. @SubscribeEvent public void onEvent(ModelBakeEvent event) { final ModelResourceLocation modelresourcelocationone = new ModelResourceLocation(MainReference.ID + ":" + "ak74u", "inventory"); final ModelResourceLocation modelresourcelocationtwo = new ModelResourceLocation(MainReference.ID + ":" + "ak74ugui", "inventory"); final IBakedModel bakedmodelone = event.modelManager.getModel(modelresourcelocationone); final IBakedModel bakedmodeltwo = event.modelManager.getModel(modelresourcelocationtwo); final ModelRealistic modelarms = new ModelRealistic(bakedmodelone, bakedmodeltwo, new VertexFormat()); event.modelBakery.addVariantName(MainInitialization.ITEMS.get("ak74u"), new String[] { MainReference.ID + ":" + "ak74ugui" }); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(MainInitialization.ITEMS.get("ak74u"), 1, modelresourcelocationtwo); event.modelRegistry.putObject(modelresourcelocationone, modelarms);
-
[1.8] [SOLVED] B3D item model has no texture
EverythingGames replied to Wehavecookies56's topic in Modder Support
Why didn't RainWarrioir continue the OBJLoader...B3D is horrible.