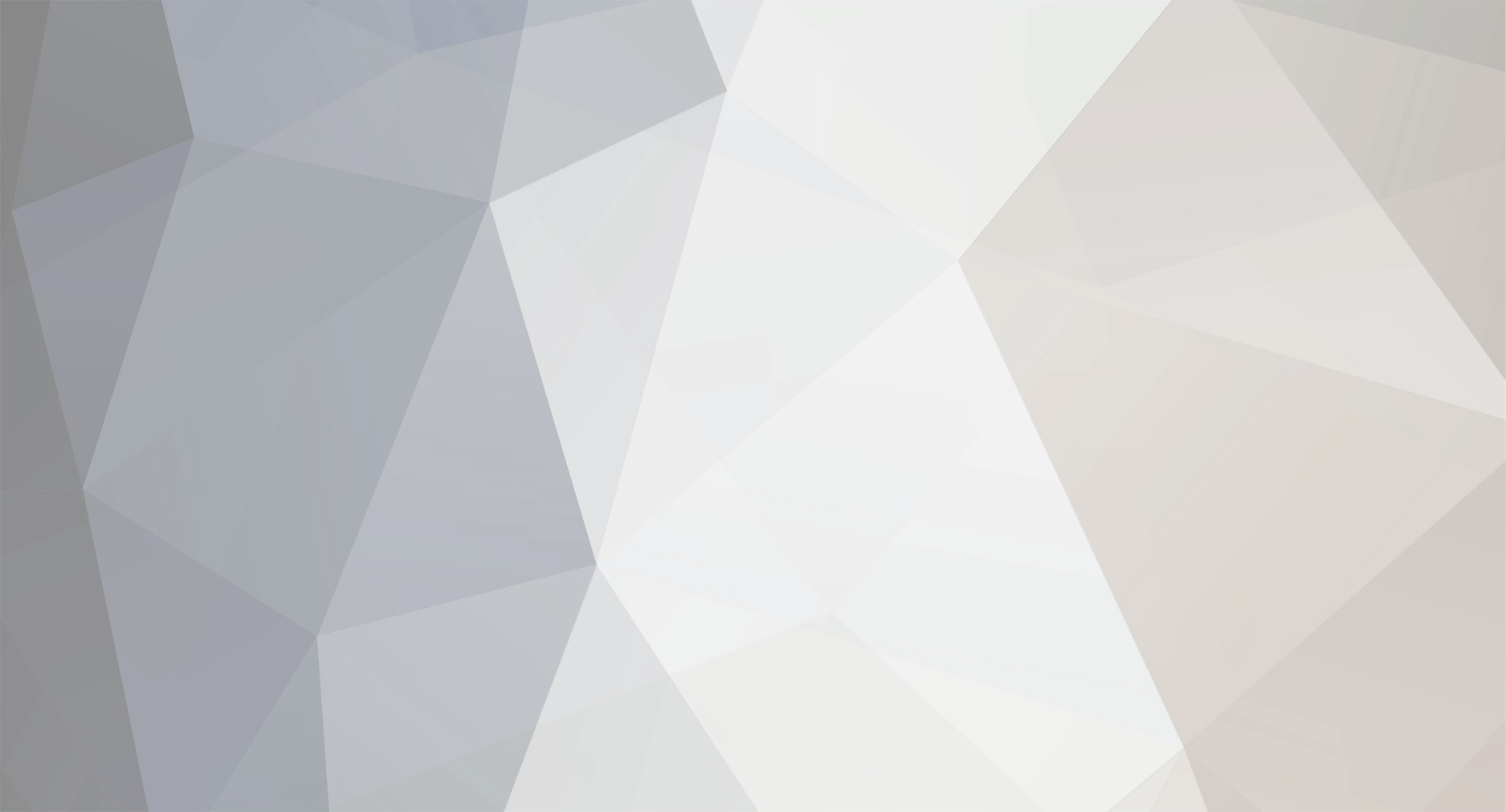
MFMods
Members-
Posts
370 -
Joined
-
Days Won
13
Everything posted by MFMods
-
ok. you have "your" storage. where you keep data. let's say this is it: public class OurMap { private HashMap<String, Integer> internal; // getter, setter, etc. } now a Saved data class. before 1.18, it had save and load methods. as of 1.18 load is done in constructor. i don't like it but it is how it is. again, you do not tell the game to save anything. save will happen when it happens. public class OurWorldSavedData extends SavedData { private final OurMap map = new OurMap(100); public OurWorldSavedData() { } public OurWorldSavedData(CompoundTag root) // load method is now this { if (root.contains(Constants.MODID)) { ListTag list = root.getList(Constants.MODID, 10); CompoundTag current; for (Tag nbt : list) { current = (CompoundTag) nbt; this.map.init(current.getString("key"), current.getInt("val")); } } } @Override public CompoundTag save(CompoundTag root) { ListTag list = new ListTag(); foreach (String k, int v) in map do something like this( { CompoundTag c = new CompoundTag(); c.putString("key", k); c.putInt("val", v); list.add(c); } ); root.put(Constants.MODID, list); return root; } public OurMap getMap() { return this.map; } } last part may sound cringy but there are many ways to do this and i can't list them all (and this isn't how i prefer to do it). maybe you'll have the class just expose the internal structure and have the rest of the mod write to it. or you'll provide getters and setters in SavedData. or keep them separate. doesn't matter much. important part - hooking it to work: this is my FoodManager class. don't worry about the logic. also implementation isn't elegant (i have separate map and saved data) but focus on the last part - i attach a single instance of saved data to overworld: public class FoodDataManager { private static OurMap foodMap = null; private static OurWorldSavedData data = null; private static final Logger LOGGER = LogManager.getLogger(); public static int increase(Player player, ItemStack item) { if (!(player.level instanceof ServerLevel)) { return -1; } VerifyInitialized(player.level); int result = foodMap.increase(player, item.getItem()); data.setDirty(); return result; } private static void VerifyInitialized(Level currentWorld) { if (foodMap == null) { data = currentWorld.getServer().overworld().getDataStorage().computeIfAbsent(OurWorldSavedData::new, OurWorldSavedData::new, Constants.MODID); foodMap = data.getMap(); } } }
-
https://forge.gemwire.uk/wiki/Saved_Data if it makes sense to split data for each dimensions then attach to dimensions separately. if it makes no sense, attach everything to overworld.
-
if you keep things simple, there likely won't be issues. i wouldn't attach this to a chunk because it doesn't logically belong to a chunk. for example a flag saying whether players have visited a chunk, whether your retrogen is finished on a chunk, whether your special slimes should spawn in a chunk? that i would store with a chunk. in short, things i would access in "entity enters section" event. your list of special block positions belongs to the world - either use world saved data (use this for simple and private data) or world capabilities (more convoluted but nice inter-mod interaction). you keep the data in memory (for example a list of locations (BlockPos) or some map), you tell the game how to write it into a given nbt and that's that. when you change the data, you mark is as dirty but the game won't write it to hard drive then. only later (usually when you hit esc to get the menu), will the data be written to storage. again, you just maintain you list/map and not worry about storage.
-
ctrl+click on setHealth
-
Method to change the "dirt" of a tree using BaseTreeFeatureConfig?
MFMods replied to PortsNotAlt's topic in Modder Support
here's help that works only on 1.19 and above. it does not work on old versions. use debugger. saplings don't care about soil other than when the player is placing them. so - it's not that your tree won't grow on given soil, it's that it won't grow at all. use debugger. -
[1.19.4, SOLVED] Get if block can be passed through
MFMods replied to MrDiamondDog's topic in Modder Support
if you when to check whether blocks are completely "empty" (in terms of collision), that's that. maybe you want to allow for blocks that are mostly empty (various rails or ladders). getCollisionShape, as Ash said, gives you blocks shape. multiply xSize * ySize * zSize and you get the volume. if you compare that to (for example) 13% of the full block, rails and pressure plates will pass but slabs will not. -
What are the Steps to create a custom Event?
MFMods replied to Jonas Handtke's topic in Modder Support
well forge modifies the game code and inserts a bunch of calls to its events (and then optionally blocks stuff depending on event result). since the main the thing about forge is that you don't need to change the game code yourself (and very much should not), we can't really help you here. modders can react to swimming anyway by reacting to tick event and doing some checks (what entity is ticking, what phase it is, what's the value of isSwimming() ), so... is there really any need for that event? -
player.inventory.add(Items.CARROT)
-
chunks canhold data, yes. respond to ChunkDataEvent.Load event and ChunkDataEvent.Save event. events have getData method which returns a nbt tag. or you can save your structure (map or list of small records) into world save data. https://forge.gemwire.uk/wiki/Saved_Data
-
[1.19.4] How do we make a specific entity not despawn at all?
MFMods replied to Feroov's topic in Modder Support
a method checkDespawn will fire. override it and see yourself. this one is useful if you want to do some checks and decide whether to keep the entity or remove it. for simple cases maybe just override isPersistenceRequired and finito.- 1 reply
-
- 1
-
-
search again for mcjty's tutorials. even if the gui tutorial is for older version, nothing has changed recently.
-
when you change the build.gradle, a small hard-to-notice floating window shows up in intellij idea - above editor, close to the right side. try increasing the version in build.gradle if that's where you keep it. then click the button with two arrows forming a circle and grab an apple. in about 5 minutes, your access transformer lines will be applied (assuming you uncommented the AT line in build.gradle).
-
How to make zombies run away from player for a certain amount of time
MFMods replied to PadFoot2008's topic in Modder Support
do not use mixins for this. maybe you'll need access transformers for some fields (where the zombie is trying to walk to) but you do not need mizins. respond to "entity joins world". add your ai goal to zombies list of goals. -
you do realize we have no idea what you wrote and where? also, even if you did it right, maybe a code from one of the goals is changing y-rotation after your code. read through all the goals that are active. delete all goals unless they meet two requirements: 1) you want the goal to execute, and 2) you 100% understand everything it does.
-
Visual problems with OptiFine (1.18.2)
MFMods replied to hereticon_'s topic in Support & Bug Reports
there is a simple answer to your optifine problem: do not use optifine. trust me on this, because it is highly unlikely your computer is older than mine. i need performance mods more than you do. rubidium plus canary. remove optifine, put those two and you're good. for a good measure, add a few minor performance mods too, clumps and maybe something that culls leaves (depending on the way you play). -
if the idea requires mathematical knowledge that you do not have (working with angles) and programming skills you do not have (skipping math and finding a way to reuse existing projectile logic), why are you still working on this mod? surely you can come up with a mod idea without impassable barriers.
-
hmm... so make a stack, okay, set the tag, okay (i'll just assume you tried that), then disregard the stack you made, make a new one without tag and put in into drops... might work...
-
nope.
-
[1.18.2] Check if block at given position is grass/foliage/water
MFMods replied to a topic in Modder Support
what do you need? if you need to know the type of block (which the title implies "Check if block at given position is grass/foliage/water"). try the isReplaceable method or compare the block material with something. if you need the color... those green stuff are usually gray (in png files) and those textures (in models) are assigned tint indexes. then, the developer tells the game how to recolor these parts of the model. the problem for you is - there is more than one way to choose a tint color. -
A single JSON for more than one texture gui, fixed, model, [...]
MFMods replied to DouglasRafael's topic in Modder Support
well trident has a separate texture for inventory. i can't say more without making a test mod, nor can others. if you give us a github link, maybe someone will be able to say more. general hint: if you need to adjust the item variants (1st person left, 1st person right hand, on ground, inventory, 3rd person...) get a mod called "itemtransformhelper" and do it in-game, then paste into your json. mostly useful for blocks and occasional weapon. -
that gui window closing is a developer's decision, not a necessity. so yes, it is possible. however, to change that, you either have to ask the author to make a change or you can change the mod yourself (if license permits and it almost certainly does).
-
1.16.5 Crafting any item with Gold Ingot crashes the server.
MFMods replied to YungDorito7's topic in Support & Bug Reports
we don't help with 1.16 anymore, but this is a problem unrelated to the version so i'll tell you what to do... did you look at the log you pasted? doesn't matter that you're not a programmer. just look for mod identifiers. usually in namespaces (something-dot-something-dot-mod_id). in this case the crash is in first few lines and mentions a mod family. remove them or update them or contact author. -
Various and general questions regarding best practices
MFMods replied to MiniBN's topic in Modder Support
2) just separate "forge" stuff (registering things, etc.) from "mod" stuff (what should happen for players to see) into separate classes and you will be fine. 3) no, not really. especially if you want to port to other mod loaders. except maybe architectury but i never used that. 4) don't worry about it, at least for controllable things like chickens. let player decide if he wants 4 or 400 chickens around. you should worry about ambient entities (things flying/swimming around) and not have hundreds of those especially if you can't prevent them from appearing all the time (like squids) or if you can't kill them (like some glowbugs/fireflies...). 1) start with 1.18. porting to 1.19 is pretty easy, but you probably wont want to maintain 1.19 in parallel, likely 1.18 and 1.20. you do not need to decide on this now because you don't know when you'll have a stable mod and free time.- 1 reply
-
- 1
-
-
are you using a vanilla Tier or your own? if you made a new tier for it (passed in constructor), you can just specify the tool speed there (see Tiers enum, third parameter in Tier constructor); not the same as attack speed which is in item constructor.