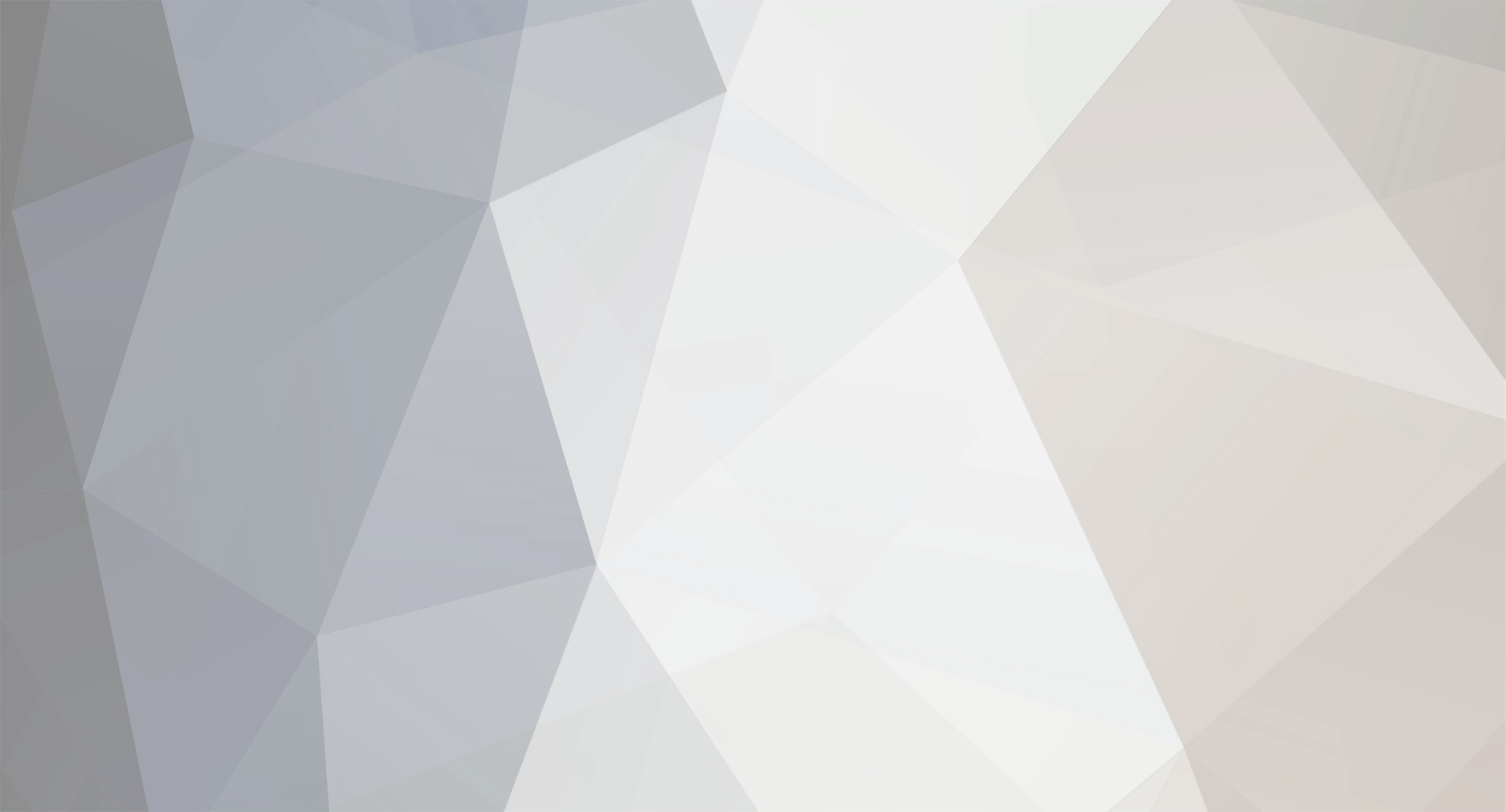
swordkorn
Members-
Posts
86 -
Joined
-
Last visited
-
Days Won
1
Everything posted by swordkorn
-
Well you are running: public class ClientProxy implements CommonProxy Instead of: public class ClientProxy extends CommonProxy
-
I don't think he's asking how to edit the base code so to speak. It would depend on which behaviours you intended to implement/"override" really. Using @SubscribeEvent and writing custom event handlers to "add" or "tweak" some existing Minecraft behaviour could give the illusion of overriding base methods, but is far simpler than trying to reflect the code.
-
Well no, I'm not anymore. I took TheGreyGhost's code advice and implemented that to no avail so I removed that block of code. Sufficed to say; when I was using that code the boolean states never switched from false defaulting to the main STONE conditional.
-
Sorry if I'm treading on toes here... but if you're using ModelLoader shouldn't it be called in the preInit? My CommonProxy setups like so: public class CommonProxy { public void preInit(FMLPreInitializationEvent e) { BlockReg.load(); ItemReg.load(); } public void init(FMLInitializationEvent e) { } public void postInit(FMLPostInitializationEvent e) { } } And my ClientProxy like so: public class ClientProxy extends CommonProxy { @Override public void preInit(FMLPreInitializationEvent e) { super.preInit(e); BlockRender.regRender(); ItemRender.regRender(); } @Override public void init(FMLInitializationEvent e) { super.init(e); } @Override public void postInit(FMLPostInitializationEvent e) { super.postInit(e); } } With the main mod section like so: @Mod.EventHandler public void preInit(FMLPreInitializationEvent e) { this.proxy.preInit(e); MinecraftForge.EVENT_BUS.register(this); FMLCommonHandler.instance().bus().register(this); path = e.getModConfigurationDirectory().getAbsolutePath() + File.separator + "NihilEnim" + File.separator; config = new Configuration(new File(path + "NihilEnim.cfg")); NihilEnimWorld.configure(config); if(config.hasChanged()) config.save(); } @Mod.EventHandler public void init(FMLInitializationEvent e) { this.proxy.init(e); } @Mod.EventHandler public void postInit(FMLPostInitializationEvent e) { this.proxy.postInit(e); } @SubscribeEvent public void onWorldLoad(WorldEvent.Load e) { if(!e.getWorld().isRemote && e.getWorld() instanceof WorldServer) { NihilEnimWorld.load(e.getWorld()); } } Another thing to bare in mind is if your items aren't set to a Creative Tab, you won't find them anywhere. They need a Registry name as well, a json file to determine textures and scale, and only then can you say for certain that you're not getting errors.
-
Both excellent suggestions and I tried them both. Sad to report it doesn't work though so I'll expand on my code for reference: @Override public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumHand hand, @Nullable ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ) { if (heldItem == null) { return false; }else{ if (!world.isRemote) { if (world.getBlockState(pos) == BlockReg.tableBlock0.getDefaultState()) { Item item = heldItem.getItem(); if (item == Item.getItemFromBlock(Blocks.COBBLESTONE)) { world.setBlockState(pos, BlockReg.tableBlock1.getDefaultState()); } if (item == Item.getItemFromBlock(Blocks.STONE)) { world.setBlockState(pos, BlockReg.tableBlock2.getDefaultState()); System.out.println("Boolean is FALSE" + itemIsAndesite + itemIsGranite + itemIsDiorite); } if (item == Item.getItemFromBlock(Blocks.STONEBRICK)) { world.setBlockState(pos, BlockReg.tableBlock3.getDefaultState()); } /*if (itemIsAndesite) { heldItem = andesite; world.setBlockState(pos, BlockReg.tableBlock4.getDefaultState()); System.out.println(heldItem); } if (itemIsDiorite) { world.setBlockState(pos, BlockReg.tableBlock5.getDefaultState()); System.out.println("Boolean is TRUE"); } if (itemIsGranite) { world.setBlockState(pos, BlockReg.tableBlock6.getDefaultState()); System.out.println("Boolean is TRUE"); }*/ } } return true; } }
-
Thanks for the detailed reply Koward but I have already tried that for several hours now. Here's a snippet of my code: Item item = heldItem.getItem(); if (item == Item.getItemFromBlock(Blocks.STONE.getDefaultState().withProperty(BlockStone.VARIANT, BlockStone.EnumType.ANDESITE))) The error message states it needs a block and this code returns a BlockState which is incompatible. You see my issue?
-
How to create an EnergyItem with CapabilityEnergy
swordkorn replied to Mattizin's topic in Modder Support
I don't understand what you're getting at here. Do you not have an Energy framework API here? In regards to your machine charging your items. You could give the item damage capabilities (this.setMaxDamage(int); ) and then programme it to "discharge" (take damage) as a visual representation of it. Your machines could be coded to look for instances of that item class type and if true, if you have internal energy stored and your machine is an energy provider, if the item's nbt is not full, internalMachineEnergy --; itemNBTEnergy ++; or something along those lines. -
[1.10.2] Check if singleplayer and get the player
swordkorn replied to Koward's topic in Modder Support
Wouldn't: if (!world.isRemote) { [...] } Work? My basic understanding is that calls only on the Client side if false. -
[1.9-1.10] Overriding universal bucket of custom liquid texutre
swordkorn replied to Eastonium's topic in Modder Support
I don't understand what you mean -
[1.10.2] Weird Rendering Glitch (Baked Model)
swordkorn replied to Technomancer's topic in Modder Support
Probably a total shot in the dark (get it? Lol!) but have you tried getting light from neighbours to keep it at the same light level? -
OK so I'm either being incredibly thick or just plain blind but I can't for the life of me figure out how to call the stone variants when checking the item in my player's hand correctly. getStateFromMeta is deprecated (and only returned the wrong one anyway so that's useless...) so I was wondering if anyone can help me out?
-
[1.9.4] Inter-mod interactions causing unusual effects
swordkorn replied to swordkorn's topic in Modder Support
Well both our mods override onBlockActivated to define a similar behaviour. However; their way of doing things adds an unwanted and unexpected drop from my blocks and for some reason, the game gives that mod the priority for handling the logic. Therefore, for my blocks at the very least, I need MY override logic to apply. Make sense? -
[1.9.4] Inter-mod interactions causing unusual effects
swordkorn replied to swordkorn's topic in Modder Support
Well that only helps in terms of checking if the mod is loaded in the instance. In terms of getting the code to ignore the methods for my mod's blocks, it doesn't really help me to determine which class to use. -
Both mine and another mod offer a similar functionality. However; when the other mod is installed alongside mine, it causes unwanted drops to occur from my blocks. Is there a way to get my mod to "ignore" the other in order to seperate the functionality?
-
It works and I have no complaints about it. How else did you expect me to map the seeds and drops to the relevant block using only one class for each? Anyway, this is all fixed now and works just as I had intended. With the added benefit that I achieved my goal of halving the file size of the mod since 1.8.9 and now.
-
If all of your plant behaviours are going to be the same as vanilla mechanics, why even override the methods at all? Save yourself excess work! But I understand it can be a pain to program custom behaviours. package com.mysticalcrops.blocks; import com.mysticalcrops.MysticalCrops; import com.mysticalcrops.items.CropItems; import net.minecraft.block.Block; import net.minecraft.block.BlockCrops; import net.minecraft.block.IGrowable; import net.minecraft.block.properties.PropertyInteger; import net.minecraft.block.state.BlockStateContainer; import net.minecraft.block.state.IBlockState; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.item.EntityItem; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.EnumBlockRenderType; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.MathHelper; import net.minecraft.world.IBlockAccess; import net.minecraft.world.World; import net.minecraftforge.common.EnumPlantType; import net.minecraftforge.common.IPlantable; import net.minecraftforge.fml.common.FMLLog; import java.util.List; import java.util.Random; /** * Created by Sword_Korn on 6/13/2016. */ public class MysticalCropBlock extends BlockCrops implements IGrowable, IPlantable { public static final PropertyInteger AGE = PropertyInteger.create("age", 0, 7); public final String regName; public MysticalCropBlock(String regName) { super(); this.regName = regName; this.setDefaultState(blockState.getBaseState().withProperty(AGE, 0)); } public boolean isSuitableForPlant(Block soil) { return soil == Blocks.FARMLAND; } protected PropertyInteger getAge() { return AGE; } public int getHarvestReadyAge() { return 7; } public boolean isHarvestReady(IBlockState state) { return state.getValue(getAge()) >= getHarvestReadyAge(); } protected Item getSeeds() { final Item seeds = CropItems.seedsMap.get(this); if(seeds == null) { FMLLog.bigWarning("No seeds detected!"); return new Item(); } return seeds; } @Override public ItemStack getItem(World world, BlockPos pos, IBlockState state) { return new ItemStack(getSeeds()); } @Override public boolean canGrow(World worl, BlockPos pos, IBlockState state, boolean isClient) { return !isHarvestReady(state); } protected Item getHarvestedItem() { final Item harvestedItem = CropItems.harvestedItemMap.get(this); if(harvestedItem == null) { FMLLog.bigWarning("No drop registered!"); return new Item(); } return harvestedItem; } @Override public IBlockState getStateFromMeta(int meta) { return getDefaultState().withProperty(getAge(), meta); } @Override public void updateTick(World world, BlockPos pos, IBlockState state, Random rnd) { this.checkAndDropBlock(world, pos, state); if(world.getLightFromNeighbors(pos.up()) >= 9) { int i = this.getMetaFromState(state); if(i < this.getHarvestReadyAge()) { float f = getGrowthChance(this, world, pos); if(rnd.nextInt((int) (25.0F / f) + 1) == 0) { world.setBlockState(pos, this.getStateFromMeta(i + 1), 2); } } } } @Override public Item getItemDropped(IBlockState state, Random rnd, int fortune) { if(!isHarvestReady(state)) { return getSeeds(); }else{ return getHarvestedItem(); } } public int getMetaFromState(IBlockState state) { return state.getValue(getAge()); } @Override public boolean canPlaceBlockAt(World world, BlockPos pos) { Block soilBlock = world.getBlockState(pos.down()).getBlock(); return this.isSuitableForPlant(soilBlock); } @Override public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumHand hand, ItemStack stack, EnumFacing side, float hitX, float hitY, float hitZ) { if(isHarvestReady(state)) { if(world.isRemote) { return true; } final ItemStack savedStack = new ItemStack(getHarvestedItem()); world.setBlockState(pos, state.withProperty(AGE, 0), 7); final EntityItem entItem = new EntityItem(world, player.posX, player.posY - 1D, player.posZ, savedStack); world.spawnEntityInWorld(entItem); entItem.onCollideWithPlayer(player); return true; } return false; } @Override public EnumPlantType getPlantType(IBlockAccess world, BlockPos pos) { return EnumPlantType.Crop; } @Override protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, AGE); } protected int getRandomInt(World world) { return MathHelper.getRandomIntegerInRange(world.rand, 1, 7); } @Override public void grow(World world, BlockPos pos, IBlockState state) { int newGrowth = getMetaFromState(state) + getRandomInt(world); int maxGrowth = getHarvestReadyAge(); if(newGrowth > maxGrowth) { newGrowth = maxGrowth; } world.setBlockState(pos, getStateFromMeta(newGrowth), 2); } @Override public void grow(World world, Random rnd, BlockPos pos, IBlockState state) { grow(world, pos, state); } @Override public boolean equals(Object obj) { return (obj instanceof MysticalCropBlock && regName.equals(((MysticalCropBlock) obj).regName)); } @Override public List<ItemStack> getDrops(IBlockAccess world, BlockPos pos, IBlockState state, int fortune) { List<ItemStack> ret = new java.util.ArrayList<ItemStack>(); Random rnd = world instanceof World ? ((World) world).rand : new Random(); int age = getMetaFromState(state); int count = quantityDropped(state, fortune, rnd); for(int i = 0; i < count; i++) { Item item = this.getItemDropped(state, rnd, fortune); if(item != null) { ret.add(new ItemStack(item, 1, this.damageDropped(state))); } } if(age >= getHarvestReadyAge()) { for(int i = 0; i < 7 + fortune; ++i) { if(rnd.nextInt(2 * getHarvestReadyAge()) <= age) { ret.add(new ItemStack(this.getSeed(), 1, 0)); } } } return ret; } } If you can decipher what's going on, try using some of that to help your growth.
-
[1.9.4] [SOLVED] NullPointer on attempted plant
swordkorn replied to swordkorn's topic in Modder Support
Never mind. I fixed it by registering both the blocks and items in the preInit of my Common Proxy. -
When I right click to plant the relevant seed it crashes on a null pointer: // Hi. I'm Minecraft, and I'm a crashaholic. Time: 6/21/16 9:15 PM Description: Unexpected error java.lang.NullPointerException: Unexpected error at net.minecraft.item.ItemSeeds.getPlant(ItemSeeds.java:52) at net.minecraft.block.Block.canSustainPlant(Block.java:1819) at net.minecraft.item.ItemSeeds.onItemUse(ItemSeeds.java:31) at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:157) at net.minecraft.client.multiplayer.PlayerControllerMP.processRightClickBlock(PlayerControllerMP.java:477) at net.minecraft.client.Minecraft.rightClickMouse(Minecraft.java:1602) at net.minecraft.client.Minecraft.processKeyBinds(Minecraft.java:2273) at net.minecraft.client.Minecraft.runTickKeyboard(Minecraft.java:2057) at net.minecraft.client.Minecraft.runTick(Minecraft.java:1845) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1119) at net.minecraft.client.Minecraft.run(Minecraft.java:404) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144) Item class: public class MysticalCropSeed extends ItemSeeds { public MysticalCropSeed(Block crops, Block soil,String regName) { super(crops, soil); this.setUnlocalizedName(regName); this.setCreativeTab(MysticalCrops.cropsTab); } } Relevant Block class methods: public class MysticalCropBlock extends BlockCrops implements IGrowable, IPlantable { protected Item getSeeds() { final Item seeds = CropItems.seedsMap.get(this); if(seeds == null) { FMLLog.bigWarning("No seeds detected!"); return new Item(); } return seeds; } @Override public ItemStack getItem(World world, BlockPos pos, IBlockState state) { return new ItemStack(getSeeds()); } protected Item getHarvestedItem() { final Item harvestedItem = CropItems.harvestedItemMap.get(this); if(harvestedItem == null) { FMLLog.bigWarning("No drop registered!"); return new Item(); } return harvestedItem; } @Override public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumHand hand, ItemStack stack, EnumFacing side, float hitX, float hitY, float hitZ) { if(isHarvestReady(state)) { if(world.isRemote) { return true; } final ItemStack savedStack = new ItemStack(getHarvestedItem()); world.setBlockState(pos, state.withProperty(AGE, 0), 7); final EntityItem entItem = new EntityItem(world, player.posX, player.posY - 1D, player.posZ, savedStack); world.spawnEntityInWorld(entItem); entItem.onCollideWithPlayer(player); return true; } return false; } } The methods to bind the seed to the block: public class CropItems { public static Item[] MCSeeds; public static Item[] MCDrops; public static HashMap<MysticalCropBlock, Item> seedsMap = new HashMap<MysticalCropBlock, Item>(); public static HashMap<MysticalCropBlock, Item> harvestedItemMap = new HashMap<MysticalCropBlock, Item>(); public static Item redstoneCropSeed; public static Item regItem(Item item, String regName) { item.setRegistryName(regName); item.setUnlocalizedName(regName); return GameRegistry.register(item); } public static Item regSeeds(String regName, Block crop) { Item item = new MysticalCropSeed(crop, Blocks.FARMLAND, regName); seedsMap.put((MysticalCropBlock) crop, item); return regItem(item, regName); } public static Item regDrops(Item item, Block crop) { return harvestedItemMap.put((MysticalCropBlock) crop, item); } public static void loadItemRegistry() { redstoneCropSeed = regSeeds("redstoneCropSeed", CropBlocks.redstoneCrop); regDrops(Items.REDSTONE, CropBlocks.redstoneCrop); MCSeeds = new Item[] {redstoneCropSeed}; MCDrops = new Item[] {Items.REDSTONE}; } }
-
Thank you Jeff! Out of the frying pan though... I fix one problem and another crops up in its place. Now when I right click to plant the relevant seed it crashes on a null pointer: // Hi. I'm Minecraft, and I'm a crashaholic. Time: 6/21/16 9:15 PM Description: Unexpected error java.lang.NullPointerException: Unexpected error at net.minecraft.item.ItemSeeds.getPlant(ItemSeeds.java:52) at net.minecraft.block.Block.canSustainPlant(Block.java:1819) at net.minecraft.item.ItemSeeds.onItemUse(ItemSeeds.java:31) at net.minecraft.item.ItemStack.onItemUse(ItemStack.java:157) at net.minecraft.client.multiplayer.PlayerControllerMP.processRightClickBlock(PlayerControllerMP.java:477) at net.minecraft.client.Minecraft.rightClickMouse(Minecraft.java:1602) at net.minecraft.client.Minecraft.processKeyBinds(Minecraft.java:2273) at net.minecraft.client.Minecraft.runTickKeyboard(Minecraft.java:2057) at net.minecraft.client.Minecraft.runTick(Minecraft.java:1845) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1119) at net.minecraft.client.Minecraft.run(Minecraft.java:404) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144) Item class: public class MysticalCropSeed extends ItemSeeds { public MysticalCropSeed(Block crops, Block soil,String regName) { super(crops, soil); this.setUnlocalizedName(regName); this.setCreativeTab(MysticalCrops.cropsTab); } } Relevant Block class methods: public class MysticalCropBlock extends BlockCrops implements IGrowable, IPlantable { protected Item getSeeds() { final Item seeds = CropItems.seedsMap.get(this); if(seeds == null) { FMLLog.bigWarning("No seeds detected!"); return new Item(); } return seeds; } @Override public ItemStack getItem(World world, BlockPos pos, IBlockState state) { return new ItemStack(getSeeds()); } protected Item getHarvestedItem() { final Item harvestedItem = CropItems.harvestedItemMap.get(this); if(harvestedItem == null) { FMLLog.bigWarning("No drop registered!"); return new Item(); } return harvestedItem; } @Override public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumHand hand, ItemStack stack, EnumFacing side, float hitX, float hitY, float hitZ) { if(isHarvestReady(state)) { if(world.isRemote) { return true; } final ItemStack savedStack = new ItemStack(getHarvestedItem()); world.setBlockState(pos, state.withProperty(AGE, 0), 7); final EntityItem entItem = new EntityItem(world, player.posX, player.posY - 1D, player.posZ, savedStack); world.spawnEntityInWorld(entItem); entItem.onCollideWithPlayer(player); return true; } return false; } } The methods to bind the seed to the block: public class CropItems { public static Item[] MCSeeds; public static Item[] MCDrops; public static HashMap<MysticalCropBlock, Item> seedsMap = new HashMap<MysticalCropBlock, Item>(); public static HashMap<MysticalCropBlock, Item> harvestedItemMap = new HashMap<MysticalCropBlock, Item>(); public static Item redstoneCropSeed; public static Item regItem(Item item, String regName) { item.setRegistryName(regName); item.setUnlocalizedName(regName); return GameRegistry.register(item); } public static Item regSeeds(String regName, Block crop) { Item item = new MysticalCropSeed(crop, Blocks.FARMLAND, regName); seedsMap.put((MysticalCropBlock) crop, item); return regItem(item, regName); } public static Item regDrops(Item item, Block crop) { return harvestedItemMap.put((MysticalCropBlock) crop, item); } public static void loadItemRegistry() { redstoneCropSeed = regSeeds("redstoneCropSeed", CropBlocks.redstoneCrop); regDrops(Items.REDSTONE, CropBlocks.redstoneCrop); MCSeeds = new Item[] {redstoneCropSeed}; MCDrops = new Item[] {Items.REDSTONE}; } } I know this is probably unrelated to the rendering but it wasn't worth starting a new thread I felt
-
[19:17:47] [Client thread/ERROR] [FML]: The following problems were captured during this phase [19:17:47] [Client thread/ERROR] [FML]: Caught exception from mysticalcrops java.lang.NullPointerException at com.mysticalcrops.items.CropItemRender.reg(CropItemRender.java:17) ~[Mystical_Crops_-_1.9.4_rewrite_main/:?] at com.mysticalcrops.items.CropItemRender.regItemRender(CropItemRender.java:13) ~[Mystical_Crops_-_1.9.4_rewrite_main/:?] at com.mysticalcrops.proxy.ClientProxy.preInit(ClientProxy.java:17) ~[Mystical_Crops_-_1.9.4_rewrite_main/:?] at com.mysticalcrops.MysticalCrops.preInit(MysticalCrops.java:37) ~[Mystical_Crops_-_1.9.4_rewrite_main/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_66] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_66] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_66] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_66] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:568) ~[forgeSrc-1.9.4-12.17.0.1960.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_66] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_66] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_66] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_66] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:228) ~[forgeSrc-1.9.4-12.17.0.1960.jar:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:206) ~[forgeSrc-1.9.4-12.17.0.1960.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_66] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_66] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_66] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_66] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) ~[guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) ~[guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) ~[guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:135) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:587) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:249) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:475) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:384) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_66] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_66] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_66] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_66] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_66] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_66] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_66] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_66] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_66] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_66] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_66] at java.lang.reflect.Method.invoke(Method.java:497) ~[?:1.8.0_66] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144) [idea_rt.jar:?] [19:17:47] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:646]: ---- Minecraft Crash Report ---- // On the bright side, I bought you a teddy bear! public class CropItemRender { public static void regItemRender() { reg(CropItems.redstoneCropSeed); } public static void reg(Item item) { String resName = item.getRegistryName().toString(); ModelResourceLocation res = new ModelResourceLocation(resName, "inventory"); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(item, 0, res); } } Any help would be appreciated
-
So I confused even the great Draco18s! I'll add that as an Achievement Unlocked! lol!!! OK so I admit the code may seem a bit broken up and in all honesty, I had missed a few steps but I've since gone back and tweaked a few things. My item class now simply looks like this: public class MysticalCropSeed extends ItemSeeds{ public MysticalCropSeed(Block crops, Block soil, String regName) { super(crops, soil); setUnlocalizedName(regName); setCreativeTab(MysticalCrops.cropsTab); } } So everything seed related is handled by the underlying seed logic. A hint I got from reading your example mod on that winter wheat or something... Block side, it now associates with the seed by checking the seedsMap as defined in CropItems: public class CropItems { public static HashMap<MysticalCropBlock, Item> seedsMap = new HashMap<MysticalCropBlock, Item>(); public static HashMap<MysticalCropBlock, Item> harvestedItemMap = new HashMap<MysticalCropBlock, Item>(); public static Item redstoneCropSeed; public static Item regItem(Item item, String regName) { item.setRegistryName(regName); item.setUnlocalizedName(regName); return GameRegistry.register(item); } public static Item regSeeds(String regName, Block crop) { Item item = new MysticalCropSeed(crop, Blocks.FARMLAND, regName); seedsMap.put((MysticalCropBlock) crop, item); return regItem(item, regName); } public static Item regDrops(Item item, Block crop) { return harvestedItemMap.put((MysticalCropBlock) crop, item); } public static void loadItemRegistry() { regSeeds("redstoneCropSeed", CropBlocks.redstoneCrop); regDrops(Items.REDSTONE, CropBlocks.redstoneCrop); MCSeeds = new Item[] {redstoneCropSeed}; MCDrops = new Item[] {Items.REDSTONE}; } The methods regSeeds and regDrops add the relevant drop item to the relevant HashMap. By doing this, the seed required to plant the block and the required drops upon breaking can be defined in the block class instead as a general constructor. I implement it like this: public class MysticalCropBlock extends BlockCrops implements IGrowable, IPlantable { protected Item getSeeds() { final Item seeds = CropItems.seedsMap.get(this); if(seeds == null) { FMLLog.bigWarning("No seeds detected!"); return new Item(); } return seeds; } @Override public ItemStack getItem(World world, BlockPos pos, IBlockState state) { return new ItemStack(getSeeds()); } protected Item getHarvestedItem() { final Item harvestedItem = CropItems.harvestedItemMap.get(this); if(harvestedItem == null) { FMLLog.bigWarning("No drop registered!"); return new Item(); } return harvestedItem; } @Override public Item getItemDropped(IBlockState state, Random rnd, int fortune) { if(!isHarvestReady(state)) { return getSeeds(); }else{ return getHarvestedItem(); } } @Override public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumHand hand, ItemStack stack, EnumFacing side, float hitX, float hitY, float hitZ) { if(isHarvestReady(state)) { if(world.isRemote) { return true; } final ItemStack savedStack = new ItemStack(getHarvestedItem()); world.setBlockState(pos, state.withProperty(AGE, 0), 7); final EntityItem entItem = new EntityItem(world, player.posX, player.posY - 1D, player.posZ, savedStack); world.spawnEntityInWorld(entItem); entItem.onCollideWithPlayer(player); return true; } return false; } So in effect, the item doesn't define the block, the block defines the item. By linking it through the getSeeds and getHarvestedItem methods, they can be bound in the item registry. Hope that makes a bit more sense. And yes; I purposely only included the methods relevant. That's why the block class may seem empty but trust me, it's all there and works great.
-
I actually found an easier way to achieve what I was trying to do, so I thought I'd post it here in case people were interested: My item registry class: public class CropItems { public static Item[] MCSeeds; public static Item[] MCDrops; public static HashMap<MysticalCropBlock, Item> seedsMap = new HashMap<MysticalCropBlock, Item>(); public static HashMap<MysticalCropBlock, Item> harvestedItemMap = new HashMap<MysticalCropBlock, Item>(); public static Item redstoneSeedItem = new MysticalCropSeed(CropBlocks.redstoneCrop, Blocks.FARMLAND, "redstoneCropSeed"); public static Item regItem(Item item, String regName) { item.setRegistryName(regName); item.setUnlocalizedName(regName); return GameRegistry.register(item); } public static void loadItemRegistry() { regItem(redstoneSeedItem, "redstoneCropSeed"); MCSeeds = new Item[] {redstoneSeedItem}; MCDrops = new Item[] {Items.REDSTONE}; } } My crop block class that binds the seeds and eventually any drops to the block in question: protected Item getSeeds() { final Item seeds = CropItems.seedsMap.get(this); if(seeds == null) { FMLLog.bigWarning("No seeds detected!"); return new Item(); } return seeds; } @Override public ItemStack getItem(World world, BlockPos pos, IBlockState state) { return new ItemStack(getSeeds()); } @Override public boolean canGrow(World worl, BlockPos pos, IBlockState state, boolean isClient) { return !isHarvestReady(state); } protected Item getHarvestedItem() { final Item harvestedItem = CropItems.harvestedItemMap.get(this); if(harvestedItem == null) { FMLLog.bigWarning("No drop registered!"); return new Item(); } return harvestedItem; } There's more to it of course, but I wanted to share this in case people were struggling like I was. Thanks everyone!