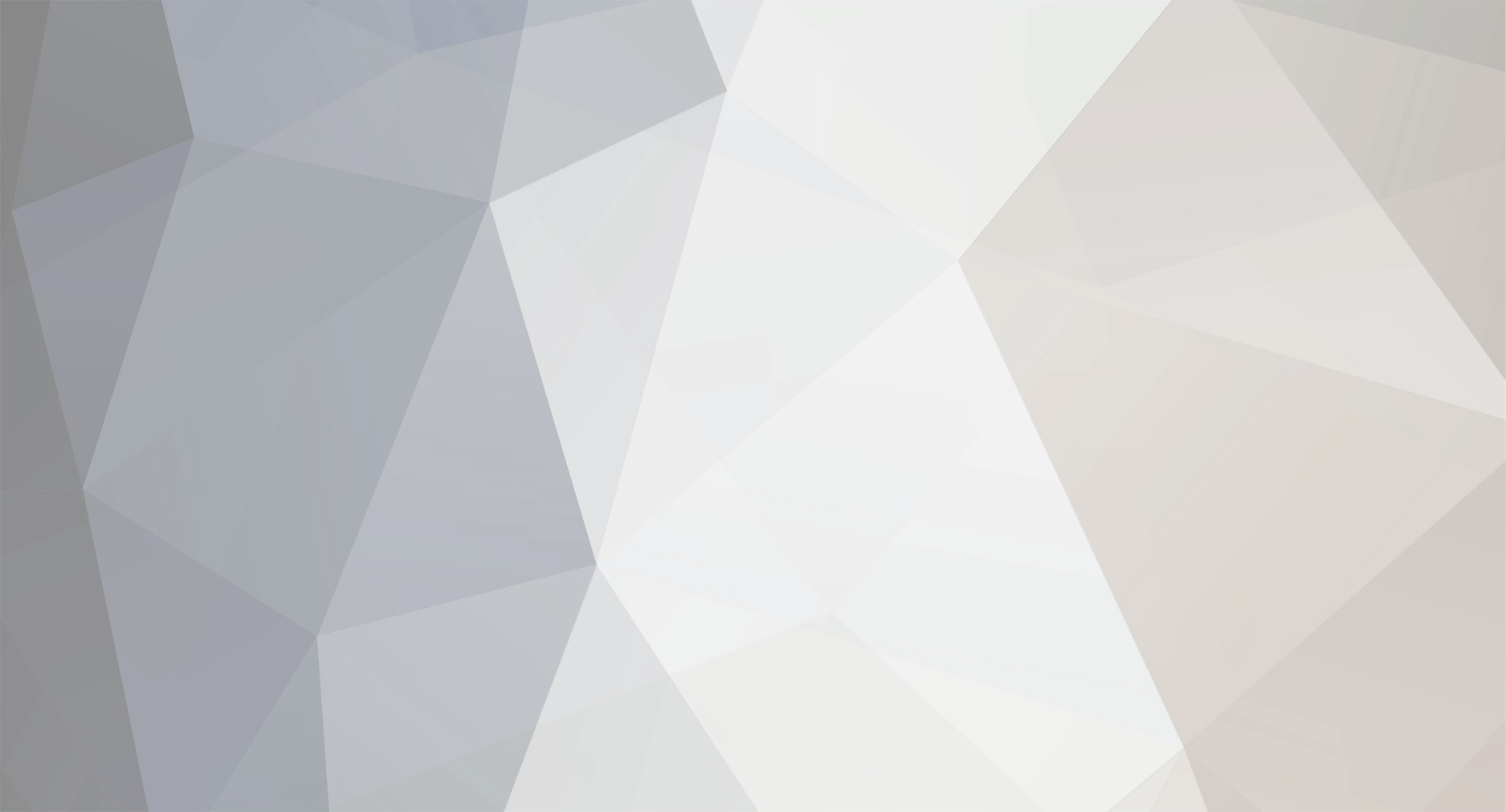
lukas2005
Forge Modder-
Posts
289 -
Joined
-
Days Won
2
Everything posted by lukas2005
-
Okay thank you I will try this when I'll turn my PC on because I am writing from phone now
-
Create a normal I storage implementation but then in your provider leave deserializeNBT And serializeNBT empty
-
GIMP,Photoshop,PAINT.NET and almost any photo editing program that can make translucent background
-
Ok now i have those classes now the only thing i am wondering about is to where but markDirty calls. The goal is to make a hashmap of all cameras in all dimensions in world that is synced to all clients and server and is saved to world file MyWorldSavedData: package io.github.lukas2005.spymod; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.world.World; import net.minecraft.world.WorldSavedData; import net.minecraft.world.storage.MapStorage; public class MyWorldSavedData extends WorldSavedData { private static final String DATA_NAME = Reference.MOD_ID + "_MyWorldSavedData"; public MyWorldSavedData() { super(DATA_NAME); } @Override public void readFromNBT(NBTTagCompound nbt) { Camera.REGISTRY.clear(); NBTTagList list = (NBTTagList) nbt.getTag("CameraRegistry"); for (int i=0;i < list.tagCount();i++) { NBTTagCompound camnbt = (NBTTagCompound) list.get(0); Camera.registerCamera(new Camera(camnbt.getUniqueId("UUID")).readFromNBT(camnbt)); } } @Override public NBTTagCompound writeToNBT(NBTTagCompound nbt) { NBTTagList list = new NBTTagList(); for (Camera cam : Camera.REGISTRY.values()) { NBTTagCompound camnbt = new NBTTagCompound(); camnbt.setUniqueId("UUID", cam.getUUID()); cam.writeToNBT(camnbt); list.appendTag(camnbt); } nbt.setTag("CameraRegistry", list); Camera.REGISTRY.clear(); return nbt; } public static MyWorldSavedData get(World world) { MapStorage storage = world.getMapStorage(); MyWorldSavedData instance = (MyWorldSavedData) storage.getOrLoadData(MyWorldSavedData.class, DATA_NAME); if (instance == null) { instance = new MyWorldSavedData(); storage.setData(DATA_NAME, instance); } return instance; } } Camera: package io.github.lukas2005.spymod; import java.util.HashMap; import java.util.UUID; import io.github.lukas2005.spymod.Network.CameraRegistryChangeMessage; import io.github.lukas2005.spymod.Network.CameraRegistryChangeMessage.Type; import io.github.lukas2005.spymod.Network.NetworkManager; import io.netty.buffer.ByteBuf; import net.minecraft.nbt.NBTTagCompound; import net.minecraftforge.fml.common.network.ByteBufUtils; public class Camera { public static HashMap<UUID, Camera> REGISTRY = new HashMap<UUID, Camera>(); private final UUID uuid; private String name; private UUID attachment; public Camera() { this.uuid = UUID.randomUUID(); } public Camera(UUID uuid) { this.uuid = uuid; } public Camera(UUID uuid, Object...data) { this.uuid = uuid; this.name = (String) data[0]; this.attachment = (UUID) data[1]; } public static Camera getCamera(UUID uuid) { return REGISTRY.get(uuid); } public static void registerCamera(Camera cam) { REGISTRY.put(cam.getUUID(), cam); NetworkManager.INSTANCE.sendToServer(new CameraRegistryChangeMessage(Type.ADD_CAMERA, cam.uuid)); } public static void registerCameraNoUpdate(Camera cam) { REGISTRY.put(cam.getUUID(), cam); } public static void unRegisterCamera(Camera cam) { REGISTRY.remove(cam.getUUID()); NetworkManager.INSTANCE.sendToServer(new CameraRegistryChangeMessage(Type.REMOVE_CAMERA, cam.uuid)); } public static void unRegisterCameraNoUpdate(Camera cam) { REGISTRY.remove(cam.getUUID()); } public static void unRegisterCamera(UUID cam) { REGISTRY.remove(cam); NetworkManager.INSTANCE.sendToServer(new CameraRegistryChangeMessage(Type.REMOVE_CAMERA, cam)); } public static void unRegisterCameraNoUpdate(UUID cam) { REGISTRY.remove(cam); } public String getName() { return name; } public Camera setName(String name) { this.name = name; NetworkManager.INSTANCE.sendToServer(new CameraRegistryChangeMessage(Type.UPDATE_CAMERA, getUUID(), name, null)); return this; } public Camera setNameNoUpdate(String name) { this.name = name; return this; } public UUID getAttachment() { return attachment; } public Camera setAttachment(UUID attachment) { this.attachment = attachment; NetworkManager.INSTANCE.sendToServer(new CameraRegistryChangeMessage(Type.UPDATE_CAMERA, getUUID(), null, attachment)); return this; } public Camera setAttachmentNoUpdate(UUID attachment) { this.attachment = attachment; return this; } public UUID getUUID() { return uuid; } public Camera writeToNBT(NBTTagCompound nbt) { nbt.setString("Name", getName()); nbt.setUniqueId("Attach", getAttachment()); return this; } public Camera readFromNBT(NBTTagCompound nbt) { setName(nbt.getString("Name")); setAttachment(nbt.getUniqueId("Attach")); return this; } public void writeToByteBuf(ByteBuf buf) { ByteBufUtils.writeUTF8String(buf, getName()); // New camera name ByteBufUtils.writeUTF8String(buf, ((UUID)getAttachment()).toString()); } public Camera readFromByteBuf(ByteBuf buf) { setName(ByteBufUtils.readUTF8String(buf)); // New camera name setAttachment(UUID.fromString(ByteBufUtils.readUTF8String(buf))); //New camera block pos attachment return this; } } CameraRegistryChangeMessage: package io.github.lukas2005.spymod.Network; import java.util.UUID; import io.github.lukas2005.spymod.Camera; import io.netty.buffer.ByteBuf; import net.minecraftforge.fml.common.network.ByteBufUtils; import net.minecraftforge.fml.common.network.simpleimpl.IMessage; import net.minecraftforge.fml.common.network.simpleimpl.IMessageHandler; import net.minecraftforge.fml.common.network.simpleimpl.MessageContext; public class CameraRegistryChangeMessage implements IMessage { int type = 0; Type etype; Object[] data; public CameraRegistryChangeMessage() {} public CameraRegistryChangeMessage(Type type, Object...data) { switch(type) { case ADD_CAMERA: this.type = 0; break; case REMOVE_CAMERA: this.type = 1; break; case UPDATE_CAMERA: this.type = 2; break; } this.etype = type; this.data = data; } @Override public void fromBytes(ByteBuf buf) { switch(buf.readInt()) { case 0: this.etype = Type.ADD_CAMERA; data = new Object[1]; data[0] = UUID.fromString(ByteBufUtils.readUTF8String(buf)); break; case 1: this.etype = Type.REMOVE_CAMERA; data = new Object[1]; data[0] = UUID.fromString(ByteBufUtils.readUTF8String(buf)); // UUID of camera to remove break; case 2: this.etype = Type.UPDATE_CAMERA; data = new Object[3]; data[0] = UUID.fromString(ByteBufUtils.readUTF8String(buf)); Camera cam = new Camera((UUID) data[0]).readFromByteBuf(buf); data[1] = cam.getName(); data[2] = cam.getAttachment(); break; } } @Override public void toBytes(ByteBuf buf) { buf.writeInt(type); switch(etype) { case ADD_CAMERA: ByteBufUtils.writeUTF8String(buf, ((UUID)data[0]).toString()); break; case REMOVE_CAMERA: ByteBufUtils.writeUTF8String(buf, ((UUID)data[0]).toString()); break; case UPDATE_CAMERA: ByteBufUtils.writeUTF8String(buf, ((UUID)data[0]).toString()); //UUID of camera to update new Camera((UUID)data[0], (String)data[1], data[2]).writeToByteBuf(buf); break; } } public static class Handler implements IMessageHandler<CameraRegistryChangeMessage, IMessage> { @Override public IMessage onMessage(final CameraRegistryChangeMessage message, MessageContext ctx) { UUID uuid = (UUID)message.data[0]; switch(message.etype) { case ADD_CAMERA: if (!Camera.REGISTRY.containsKey(uuid)) { Camera.registerCameraNoUpdate(new Camera(uuid)); } break; case REMOVE_CAMERA: if (Camera.REGISTRY.containsKey(uuid)) { Camera.unRegisterCameraNoUpdate(uuid); } break; case UPDATE_CAMERA: if (Camera.REGISTRY.containsKey(uuid)) { String name = (String) message.data[1]; UUID attachment = (UUID) message.data[2]; Camera cam = Camera.REGISTRY.get(uuid); if (name != null) cam.setNameNoUpdate(name); if (attachment != null) cam.setAttachmentNoUpdate(attachment); } break; } NetworkManager.INSTANCE.sendToAll(message); return null; } } public static enum Type { ADD_CAMERA, REMOVE_CAMERA, UPDATE_CAMERA } }
-
[Solved][1.11.2][Forge] IBakedModel help
lukas2005 replied to Zeher_Monkey's topic in Modder Support
Nevermind -
Hello i want to save some data to world and i have the WorldSavedData implementation but i am not sure what i shloud fill in those functions and how to add data docs are not really too clear about that all they say is "The existing data can be obtained using MapStorage#getOrLoadData, and new data can be attached using MapStorage#setData". Wich does not really tell me much i have this code: package io.github.lukas2005.spymod; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.world.World; import net.minecraft.world.WorldSavedData; import net.minecraft.world.storage.MapStorage; public class MyWorldSavedData extends WorldSavedData { private static final String DATA_NAME = Reference.MOD_ID + "_MyWorldSavedData"; public MyWorldSavedData() { super(DATA_NAME); } @Override public void readFromNBT(NBTTagCompound nbt) { } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { return null; } public static MyWorldSavedData get(World world) { MapStorage storage = world.getMapStorage(); MyWorldSavedData instance = (MyWorldSavedData) storage.getOrLoadData(MyWorldSavedData.class, DATA_NAME); if (instance == null) { instance = new MyWorldSavedData(); storage.setData(DATA_NAME, instance); } return instance; } }
-
refresh
-
1. Please use MineccraftForge.EVENT_BUS.register instead of FMLCommonHandler.bus() they are the same ting but one is deprecated 2. Where are you running this mod on server or client?
-
1. Yes MinecraftServer.getCommandManager().executeCommand(<Player Instance>, "/tp 0 0 0"); 2. Yes server.getPlayerList().getPlayerList().get(0).getName() (double getPlayerList is not an error) gets name of first player on server im not sure if its diffrent in 1.8 but this works in 1.11.2
-
Hello i have an item SpyMonitor wich opens up a gui and i want to be able to link monitor wit camera using that screen and i need a single slot for it how do make it???
-
[1.12] Fluid properties don't seem to be working
lukas2005 replied to DarkMorford's topic in Modder Support
i think that it would be cool if forge had just some better way of doing this for example MaterialLiquid(MapColor color, boolean pushEntities) so that you don't need to replicate the code because mc expected to only have water and lava -
[1.12] Yet another struggle with a backpack implementation
lukas2005 replied to RaijinKnight's topic in Modder Support
i think that for this you dont make a custom capability but just use the arelady made IItemHandler also provide crah report to get help with it tutor for containers (shloud work for 1.12) -
[1.12] Fluid properties don't seem to be working
lukas2005 replied to DarkMorford's topic in Modder Support
maybe try (new MaterialLiquid(MapColor.YELLOW)).setNoPushMobility(); and if it does not work then i think that it just checks if material is MaterialLiquid.WATER -
[1.11.2][Solved] Item NBT data does not update on gui button click
lukas2005 replied to lukas2005's topic in Modder Support
refresh -
[1.11.2][Solved] Item NBT data does not update on gui button click
lukas2005 replied to lukas2005's topic in Modder Support
how do i implement a container ? i want to make a single slot wich is gonna be used just to link a camera item with monitor item -
[1.11.2][Solved] Item NBT data does not update on gui button click
lukas2005 replied to lukas2005's topic in Modder Support
thanks it worked but one more thing how do i make a slot in GuiScreen?? -
[1.11.2][Solved] Item NBT data does not update on gui button click
lukas2005 replied to lukas2005's topic in Modder Support
i thought that item nbt sync automatically -
Mob or Entity that Explodes on Collide with other entity and blocks?
lukas2005 replied to Faffreux's topic in Modder Support
please use spoilers (that eye on the tool bar of the editor) for logs and code tags (that <> mark on the toolbar) for code also while mcreator is not the best solution good thing that you are writing some of the code manually -
Hello i am trying to change items nbt data when a button is clicked in it's gui but when i click the button then the nbt data of item does not change (tested with /entitydata) Code: @Override protected void actionPerformed(GuiButton button) throws IOException { switch(button.id) { case(0): //Assign camera with monitor button try { ItemStack stack = Minecraft.getMinecraft().player.getHeldItemMainhand(); NBTTagCompound stacknbt = stack.getTagCompound(); NBTTagList cams = (NBTTagList) stacknbt.getTag("Cameras"); NBTTagCompound nbt = new NBTTagCompound(); nbt.setUniqueId("UUID", UUID.randomUUID()); //Placeholder cams.appendTag(nbt); stacknbt.setTag("Cameras", cams); stack.setTagCompound(stacknbt); System.out.println("lol"); } catch (Exception e) { e.printStackTrace(); } } super.actionPerformed(button); } log:
-
maybe try to make a clean installation of forge (and BTW never download forge from other websites than files.minecraftforge.net also are you on an server on singleplayer? also maybe try using recommended version rather than Latest