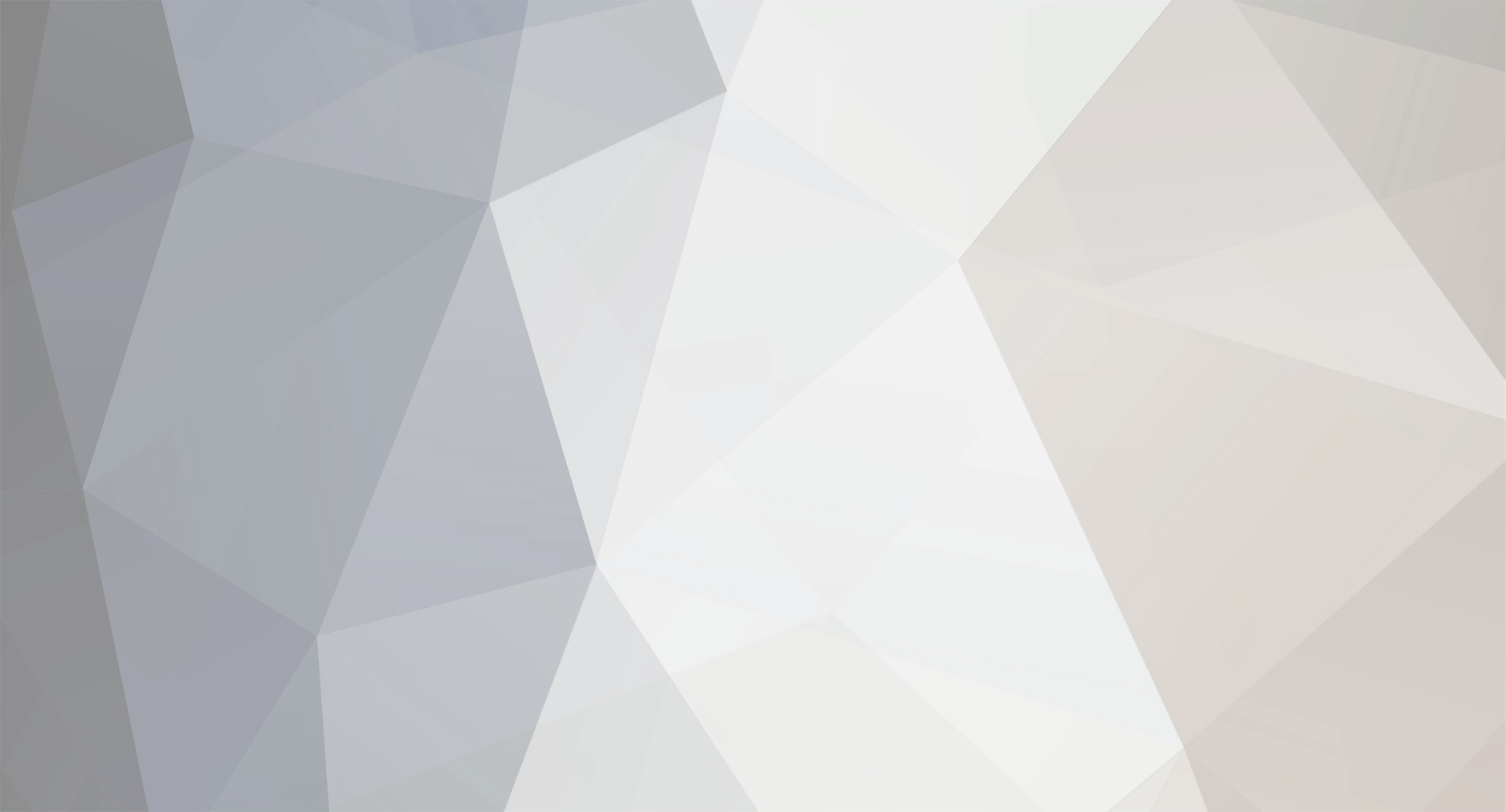
Lambda
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by Lambda
-
[1.11] Problem with NBT not saving on Capabilities.
Lambda replied to Lambda's topic in Modder Support
Hmm.. I believe I'm doing this in my event class.. (forgot to include ) Edit: Now its saving after adding a packet send to readData... Thanks! -
Hey there! So, I'm trying to create an Item that saves you when your close to death.. however, if you take a lot damage, at, say 1.5 hearts, you die instead of it give you health back. Maybe there is a way I can see how much damage a player is going to take..? Thanks,
-
Hey there! So I have a capability that adds a few integers... However, I'm having an issue with saving it.. On restart it seems to reset, even though I believe I have it properly setup... Here is the main Capability Class: public class CapabilityMagic { @CapabilityInject(CapabilityMagicData.class) public static Capability<CapabilityMagicData> MANA = null; public static void register() { CapabilityManager.INSTANCE.register(CapabilityMagicData.class, new Capability.IStorage<CapabilityMagicData>() { @Override public NBTBase writeNBT(Capability<CapabilityMagicData> capability, CapabilityMagicData instance, EnumFacing side) { return instance.writeData(); } @Override public void readNBT(Capability<CapabilityMagicData> capability, CapabilityMagicData instance, EnumFacing side, NBTBase nbt) { instance.readData(nbt); } }, new Callable<CapabilityMagicData>() { @Override public CapabilityMagicData call() throws Exception { return new CapabilityMagicData(5000, 5); } }); } } The 'Capability Data: public class CapabilityMagicData implements IManaData { private Random rand = new Random(); protected float mana; protected float corruption; protected int maxManaStored; protected boolean malignantCorruption; protected int chanceToSpread=150; //higher = more rare. protected int regen; protected boolean isRegenCooldown; protected int regenTick; private EntityLivingBase entity; private EntityPlayer entityPlayer; /** IMPLEMENTATION LIST: * CORRUPTION ADD / SUBTRACT * MANA ADD / SUBTRACT * CORRUPTED MANA */ public CapabilityMagicData(int maxManaStored, int regen) { this.maxManaStored = maxManaStored; this.regen = regen; } public EntityLivingBase getEntity() { return entity; } public EntityPlayer getPlayerEntity() { return entityPlayer; } public void setEntity(EntityLivingBase entity) { this.entity = entity; } public void setEntityPlayer(EntityPlayer entity) { this.entityPlayer = entity; } public void updateCapability() { Minecraft mc = Minecraft.getMinecraft(); regenTick++; this.setEntityPlayer(mc.thePlayer); //reference Standpoint malignantCheck(); manaCheck(); PacketHandlerHelper.sendCapabilityPacket(this.getPlayerEntity(), true); } public void malignantCheck() { if(malignantCorruption) { if(chanceToSpread > 0) { if (rand.nextInt(chanceToSpread) == 0) { this.setCorruption(this.getCorruptionStored() + rand.nextInt(5)); }else { chanceToSpread--; } } if(this.chanceToSpread <= 0) { malignantCorruption = false; } } } public void manaCheck() { if(this.getManaStored() > this.getMaxManaStored()) { this.setMana(this.getMaxManaStored()); } if(isRegenCooldown) { if(regenTick >= 160) { isRegenCooldown=false; } }else { regenTick=0; } if(this.getManaStored() < this.getMaxManaStored() && !isRegenCooldown) { this.receiveMana(regen); } if(this.getManaStored() < 0) { this.setMana(0); } if(this.getMaxManaStored() < 0) { this.setMaxMana(0); } } @Override public void receiveMana(float amount) { if(canReceiveMana(amount)) { this.setMana(this.getManaStored() + amount); } } @Override public void extractMana(float amount) { if(canExtractMana(amount)) { this.setMana(this.getManaStored() - amount); this.isRegenCooldown = true; } } @Override public void receiveCorruption() { if(this.getCorruptionStored() == 0) { if(rand.nextInt(20) == 0) { this.setCorruption(1); } }else { this.setCorruption(this.getCorruptionStored() * rand.nextFloat() + 1); if(rand.nextInt(1500) == 0) { this.malignantCorruption = true; } } } @Override public void extractCorruption(float amount) { if(this.canExtractCorruption(amount)) { this.setCorruption(this.getCorruptionStored() - amount); } } public boolean canExtractCorruption(float amount) { if((this.getCorruptionStored() - amount) >= 0) { return true; }else { return false; } } public boolean canExtractMana(float amount) { if((this.getManaStored() - amount) >= 0) { return true; }else { return false; } } public boolean canReceiveMana(float amount) { if((this.getManaStored() + amount) <= this.maxManaStored) { return true; }else { return false; } } @Override public float getManaStored() { return mana; } @Override public int getMaxManaStored() { return maxManaStored - (int)this.getCorruptionStored(); } @Override public float getCorruptionStored() { return corruption; } public void setCorruption(float corruptionToSet) { this.corruption = corruptionToSet; } public float setMana(float manaToSet) { return this.mana = manaToSet; } public int setMaxMana(int maxToSet) { return this.maxManaStored = maxToSet; } public NBTBase writeData() { NBTTagCompound tag = new NBTTagCompound(); tag.setFloat("Mana", getManaStored()); tag.setFloat("Corruption", getCorruptionStored()); return tag; } public void readData(NBTBase nbt) { NBTTagCompound tag = (NBTTagCompound) nbt; this.setMana(tag.getFloat("Mana")); this.setCorruption(tag.getInteger("Corruption")); } public float getPercentedMana() { return this.getManaStored() / this.getMaxManaStored(); } public float getCorruptedMana() { if(this.getCorruptionStored() > 0) { return this.getCorruptionStored() / this.getManaStored(); }else { return 0; } } } Lastly, the 'Capability Provider' public class CapabilityMagicProvider implements ICapabilityProvider, ICapabilitySerializable<NBTTagCompound> { public static final ResourceLocation KEY = new ResourceLocation(ModUtil.MOD_ID, "mana_atr"); private CapabilityMagicData INSTANCE = new CapabilityMagicData(5000, 5); public CapabilityMagicProvider() {} public CapabilityMagicProvider(EntityLivingBase entity) { INSTANCE.setEntity(entity); if(entity instanceof EntityPlayerMP) INSTANCE.setEntityPlayer((EntityPlayerMP)entity); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return capability == CapabilityMagic.MANA; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (capability == CapabilityMagic.MANA) return (T) INSTANCE; return null; } @Override public NBTTagCompound serializeNBT() { return (NBTTagCompound) CapabilityMagic.MANA.writeNBT(INSTANCE, null); } @Override public void deserializeNBT(NBTTagCompound nbt) { CapabilityMagic.MANA.readNBT(INSTANCE, null, nbt); } } Also, I the github if you need to check out the packets: https://github.com/LambdaXV/PlentifulMisc/tree/master/src/main/java/com/lambda/plentifulmisc/network Thanks, EDIT: as a note, I know I have a few other bools / intergers in there that are not being saved in the read/writedata, I'll add them as soon as I get the base ones working.
-
Okie, but I wanted to have multiple types of 'categories'... so I guess just use multiple integers? Thanks..
-
Hey there.. So I have this corruption system the takes over the mana bar if to many 'bad' use of magic happens. However, I'm in need of a system that taleys every time you use said item and adds a larger amount of corruption per. So I'm in need of some insight.. Should add another variable to my capability that stores a list? and add to that list every time the action is performed?
-
It works now, will change the aabb thing. Thanks.
-
Thanks.. However now if the entity isnt there it seems to crash, even though I try to put countless null checks.. here is the error: Time: 12/18/16 10:46 PM Description: Ticking block entity java.lang.NullPointerException: Ticking block entity at com.lambda.plentifulmisc.tile.magic.TileEntitySacrificeStand.updateEntity(TileEntitySacrificeStand.java:93) at com.lambda.plentifulmisc.tile.TileEntityBase.update(TileEntityBase.java:184) at net.minecraft.world.World.updateEntities(World.java:1968) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:646) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:794) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:698) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:156) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:547) at java.lang.Thread.run(Thread.java:745) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at com.lambda.plentifulmisc.tile.magic.TileEntitySacrificeStand.updateEntity(TileEntitySacrificeStand.java:93) at com.lambda.plentifulmisc.tile.TileEntityBase.update(TileEntityBase.java:184) @ if (StandRecipeHandler.sacrifice.isInstance(entityLivingBases.get(i))) { Here is the entire updated te.. if (recipe.isSacrifice) { List<EntityLivingBase> entityLivingBases = this.worldObj.getEntitiesWithinAABB(EntityLivingBase.class, new AxisAlignedBB(this.getPos().getX() - 3, this.getPos().getY() - 3, this.getPos().getY() - 3, this.getPos().getX() + 3, this.getPos().getY() + 3, this.getPos().getZ() + 3)); for (int i = 0; i < entityLivingBases.size(); i++) { if (entityLivingBases.get(i) != null) { if (modifierStands != null) { if (StandRecipeHandler.sacrifice.isInstance(entityLivingBases.get(i))) { this.recipeForRenderIndex = PlentifulMiscAPI.STAND_RECIPES.indexOf(recipe); this.processTime++; boolean done = this.processTime >= recipe.time; EntityLivingBase entityNear = entityLivingBases.get(i); //So it cannot be killed / moved during the sacrifice. entityNear.setHealth(9999f); for (TileEntityDisplayStand stand : modifierStands) { if (done) { stand.slots.decrStackSize(0, 1); } } if (this.processTime % 5 == 0 && this.worldObj instanceof WorldServer) { boolean almostDone = this.processTime + 40 >= recipe.time; if (!almostDone) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.PORTAL, false, this.pos.getX() + .5, this.pos.getY() + 1, this.pos.getZ() + .5, 10, 0, 0, 0, 0.5D); } } ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.CRIT_MAGIC, false, entityNear.posX, entityNear.posY, entityNear.posZ, 10, 0, 0, 0, 10D); if (lineMode) { this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); } if (done) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.FIREWORKS_SPARK, false, this.pos.getX() + 0.5, this.pos.getY() + 1.1, this.pos.getZ() + 0.5, 100, 0, 0, 0, 0.25D); //ensure the sacrifice dies. entityNear.setHealth(0.1f); entityNear.attackEntityFrom(DamageSource.lightningBolt, 10f); this.worldObj.spawnEntityInWorld(new EntityLightningBolt(this.worldObj, entityNear.getPosition().getX(), entityNear.getPosition().getY(), entityNear.getPosition().getZ(), false)); this.worldObj.spawnEntityInWorld(new EntityLightningBolt(this.worldObj, this.pos.getX(), this.pos.getY(), this.pos.getZ(), false)); this.slots.setStackInSlot(0, recipe.output.copy()); this.markDirty(); this.processTime = 0; this.recipeForRenderIndex = -1; } break; } else { this.processTime = 0; this.recipeForRenderIndex = -1; } } } } } Thanks..
-
Hey there, So I'm trying to create a custom fluid, however I run into this Resource Error upon lauch: 21:10:16] [Client thread/ERROR]: Exception loading model for variant plentifulmisc:block_empowered_fluid#level=6 for blockstate "plentifulmisc:block_empowered_fluid[level=6]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model plentifulmisc:block_empowered_fluid#level=6 with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:241) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:229) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:146) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:798) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:347) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1184) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 29 more [21:10:16] [Client thread/ERROR]: Exception loading blockstate for the variant plentifulmisc:block_empowered_fluid#level=6: java.lang.Exception: Could not load model definition for variant plentifulmisc:block_empowered_fluid at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:274) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:121) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:229) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:146) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:798) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:347) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.lang.RuntimeException: Encountered an exception when loading model definition of 'plentifulmisc:block_empowered_fluid' from: 'plentifulmisc:blockstates/block_empowered_fluid.json' in resourcepack: 'FMLFileResourcePack:Plentiful Misc' at net.minecraft.client.renderer.block.model.ModelBakery.loadModelBlockDefinition(ModelBakery.java:246) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:223) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:270) ~[ModelLoader.class:?] ... 28 more However, I don't know what to set as the blockstate / model files. I have the texture files + the .mcmeta files for them.. however it doesn't seem to work... Thanks,
-
So I'll just use some custom liquid and have the same properties as water... Thanks for your help.
-
Thanks, so how would I check if said entity is on fire?
-
Hey there! So I'm in need of an item, when thrown in lava, to return another item... However I really don't know how to get started on this. Any pointers / information would be great! Thanks.
-
List<EntityLivingBase> entityLivingBases = this.worldObj.getEntitiesWithinAABB(EntityLivingBase.class, new AxisAlignedBB(this.getPos().getX()-5, this.getPos().getY()-5, this.getPos().getY()-5, this.getPos().getX()+5, this.getPos().getY()+5, this.getPos().getZ()+5)); if (modifierStands != null && StandRecipeHandler.class.isInstance(entityLivingBases)) { Seems to be ruturning false on StandRecipeHandler.class.isInstance(entityLivingBases) , should I be iterating though all the list? or maybe my instance isnt working properly.
-
[SOLVED]Checking to see what entity is being hit by sunlight.
Lambda replied to Mopop's topic in Modder Support
instanceof might work. -
How would I reference the recipe.sacrifice ? I cannot do instanceof due to it not being a class, but a variable.
-
public class StandRecipeHandler{ public ItemStack input; public ItemStack output; public ItemStack modifier1; public ItemStack modifier2; public ItemStack modifier3; public ItemStack modifier4; public Class<? extends EntityLivingBase> sacrifice; public int requiredMana; public float[] particleColor; public int time; public StandRecipeHandler(ItemStack input, ItemStack output, ItemStack modifier1, ItemStack modifier2, ItemStack modifier3, ItemStack modifier4, int requiredMana, int time, float[] particleColor, Class<? extends EntityLivingBase> sacrifice){ this.input = input; this.output = output; this.modifier1 = modifier1; this.modifier2 = modifier2; this.modifier3 = modifier3; this.modifier4 = modifier4; this.sacrifice = sacrifice; this.requiredMana = requiredMana; this.particleColor = particleColor; this.time = time; } } the recipe.sacrifice is referenced towards this, which is set in the recipes. ex: PlentifulMiscAPI.addStandRecipe(new ItemStack(Items.APPLE), new ItemStack(Items.NETHER_STAR), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), 7500, 100, new float[]{1F, 91F/255F, 76F/255F}, EntityChicken.class); and this still returns nothing: List<EntityLivingBase> entityLivingBases = this.worldObj.getEntitiesWithinAABB(EntityLivingBase.class, new AxisAlignedBB(this.getPos().getX()+5, this.getPos().getY()+5, this.getPos().getY()+5, this.getPos().getX()-5, this.getPos().getY()-5, this.getPos().getZ()-5));
-
Fixed it, however, now its not detecting the entities... @Override public void updateEntity() { super.updateEntity(); if(!worldObj.isRemote) { List<StandRecipeHandler> recipes = getRecipesForInput(this.slots.getStackInSlot(0)); EntityPlayer player = this.getPlayer(); if(!recipes.isEmpty() && player != null){ for(StandRecipeHandler recipe : recipes) { TileEntityDisplayStand[] modifierStands = this.getFittingModifiers(recipe); List<EntityLivingBase> entityLivingBases = this.worldObj.getEntitiesWithinAABB(EntityLivingBase.class, new AxisAlignedBB(5, 5, 5, -5, -5, -5)); System.out.println(entityLivingBases); if (modifierStands != null && entityLivingBases.contains(recipe.sacrifice)) { this.recipeForRenderIndex = PlentifulMiscAPI.STAND_RECIPES.indexOf(recipe); this.processTime++; boolean done = this.processTime >= recipe.time; for (TileEntityDisplayStand stand : modifierStands) { if (done) { stand.slots.decrStackSize(0, 1); } } if (this.processTime % 5 == 0 && this.worldObj instanceof WorldServer) { boolean almostDone = this.processTime + 40 >= recipe.time; if (!almostDone) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.PORTAL, false, this.pos.getX() + .5, this.pos.getY() + 1.1, this.pos.getZ() + .5, 10, 0, 0, 0, 0.5D); // entityNear.attackEntityFrom(DamageSource.lightningBolt, 10f); // this.worldObj.spawnEntityInWorld(new EntityLightningBolt(this.worldObj, entityNear.getPosition().getX(), entityNear.getPosition().getY(), entityNear.getPosition().getZ(), false)); } } if (lineMode) { this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); } if (done) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.FIREWORKS_SPARK, false, this.pos.getX() + 0.5, this.pos.getY() + 1.1, this.pos.getZ() + 0.5, 100, 0, 0, 0, 0.25D); this.slots.setStackInSlot(0, recipe.output.copy()); this.markDirty(); this.processTime = 0; this.recipeForRenderIndex = -1; } break; } } } else{ this.processTime = 0; this.recipeForRenderIndex = -1; } if(this.lastRecipe != this.recipeForRenderIndex){ this.lastRecipe = this.recipeForRenderIndex; this.sendUpdate(); } } } is the function that i'm changing.
-
No the (worldObj.getEntitiesWithinAABB(EntityLivingBase.class, new AxisAlignedBB(range, range, range, -range, -range, -range)) needs a java.lang.Class<? extends T>
-
No, Everything is working fine, the full error : Wrong 1st argument type. Found: 'java.lang.Class<net.minecraft.entity.EntityLivingBase>', required: 'java.lang.Class<? extends T>
-
No crash, just an IDE error.
-
if(worldObj.getEntitiesWithinAABB(EntityLivingBase.class, new AxisAlignedBB(range, range, range, -range, -range, -range))); This, still is erroring on the parameter saying it wants java.lang.Class<? extends T> I feel like a fucking retard.. maybe because I'm running on 5 hours of sleep
-
What does it want for the first parameter? Cant really figure it out.. and for the aabb would I do: new AxisAlignedBB(range, range, range, -range, -range, -range) range being 5
-
Okay, seems to be returning the entity now... Would you know how I would find an entity nearby the TileEntity?
-
Fuck. I'm so fucking dumb. Will repost if something changes.
-
Yes, however, what would I change my EntityLivingBase to?.. because I cannot simply do that because i'm asking for a EntityLivingBase, but passing entity chicken... I want to be able for all entities to be passed in there.
-
Hey there, So I have a TE that uses 4 stands as a crafting mechanic, however, I wanted to add a 'sacrifice' portion to it.. However, I'm having issues with getting the entity from the wrapper... Which looks like: public class StandRecipeHandler{ public ItemStack input; public ItemStack output; public ItemStack modifier1; public ItemStack modifier2; public ItemStack modifier3; public ItemStack modifier4; public EntityLivingBase sacrifice; public int requiredMana; public float[] particleColor; public int time; public StandRecipeHandler(ItemStack input, ItemStack output, ItemStack modifier1, ItemStack modifier2, ItemStack modifier3, ItemStack modifier4, int requiredMana, int time, float[] particleColor, EntityLivingBase sacrifice){ this.input = input; this.output = output; this.modifier1 = modifier1; this.modifier2 = modifier2; this.modifier3 = modifier3; this.modifier4 = modifier4; this.sacrifice = sacrifice; this.requiredMana = requiredMana; this.particleColor = particleColor; this.time = time; } } I'm creating the recipes via: public final class StandRecipes{ public static final ArrayList<StandRecipeHandler> MAIN_PAGE_RECIPES = new ArrayList<StandRecipeHandler>(); public static StandRecipeHandler recipeDiamond; public static StandRecipeHandler recipeNetherStar; public static StandRecipeHandler recipeEmpoweredBloodstone; public static EntityChicken chicken; public static void init(){ ModUtil.LOGGER.info("Initializing Sacrifice Recipes"); PlentifulMiscAPI.addStandRecipe(new ItemStack(Items.GOLD_INGOT), new ItemStack(Items.DIAMOND), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), 7500, 100, new float[]{1F, 91F/255F, 76F/255F}, chicken); recipeDiamond = RecipeUtil.lastStandRecipe(); PlentifulMiscAPI.addStandRecipe(new ItemStack(Items.APPLE), new ItemStack(Items.NETHER_STAR), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), new ItemStack(Items.REDSTONE), 7500, 100, new float[]{1F, 91F/255F, 76F/255F}, chicken); recipeNetherStar = RecipeUtil.lastStandRecipe(); // PlentifulMiscAPI.addStandRecipe(new ItemStack(InitItems.item_gems, 1, 0), new ItemStack(InitItems.item_gems_empowered, 1, 0), new ItemStack(Items.GOLD_INGOT), new ItemStack(Items.GLOWSTONE_DUST), new ItemStack(Items.GOLD_INGOT), new ItemStack(Items.GLOWSTONE_DUST), 15000, 250, new float[]{1F, 0, 0}); recipeEmpoweredBloodstone = RecipeUtil.lastStandRecipe(); } } And trying to access it via: this.entityForSacrifice = recipe.sacrifice; here is the entire TE for reference: @Override public void updateEntity() { super.updateEntity(); if(!worldObj.isRemote) { List<StandRecipeHandler> recipes = getRecipesForInput(this.slots.getStackInSlot(0)); EntityPlayer player = this.getPlayer(); if(!recipes.isEmpty() && player != null){ for(StandRecipeHandler recipe : recipes) { TileEntityDisplayStand[] modifierStands = this.getFittingModifiers(recipe); if (modifierStands != null) { this.recipeForRenderIndex = PlentifulMiscAPI.STAND_RECIPES.indexOf(recipe); this.processTime++; boolean done = this.processTime >= recipe.time; this.entityForSacrifice = recipe.sacrifice; System.out.print(entityForSacrifice); for (TileEntityDisplayStand stand : modifierStands) { if (done) { stand.slots.decrStackSize(0, 1); } } if (this.processTime % 5 == 0 && this.worldObj instanceof WorldServer) { boolean almostDone = this.processTime + 40 >= recipe.time; if (!almostDone) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.PORTAL, false, this.pos.getX() + .5, this.pos.getY() + 1.1, this.pos.getZ() + .5, 10, 0, 0, 0, 0.5D); // entityNear.attackEntityFrom(DamageSource.lightningBolt, 10f); // this.worldObj.spawnEntityInWorld(new EntityLightningBolt(this.worldObj, entityNear.getPosition().getX(), entityNear.getPosition().getY(), entityNear.getPosition().getZ(), false)); } } if (lineMode) { this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() + 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); this.drawDamageLaser(this.pos.getX() - 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, this.pos.getX() + 6.5, this.pos.getY() + .75, this.pos.getZ() - 6.5, new float[]{0f / 255f, 50f / 255f, 255f / 255f}); } if (done) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.FIREWORKS_SPARK, false, this.pos.getX() + 0.5, this.pos.getY() + 1.1, this.pos.getZ() + 0.5, 100, 0, 0, 0, 0.25D); this.slots.setStackInSlot(0, recipe.output.copy()); this.markDirty(); this.processTime = 0; this.recipeForRenderIndex = -1; } break; } } } else{ this.processTime = 0; this.entityForSacrifice = null; this.recipeForRenderIndex = -1; } if(this.lastRecipe != this.recipeForRenderIndex){ this.lastRecipe = this.recipeForRenderIndex; this.sendUpdate(); } } } Well atleast the update part... With this current system, it returns null. Thanks,