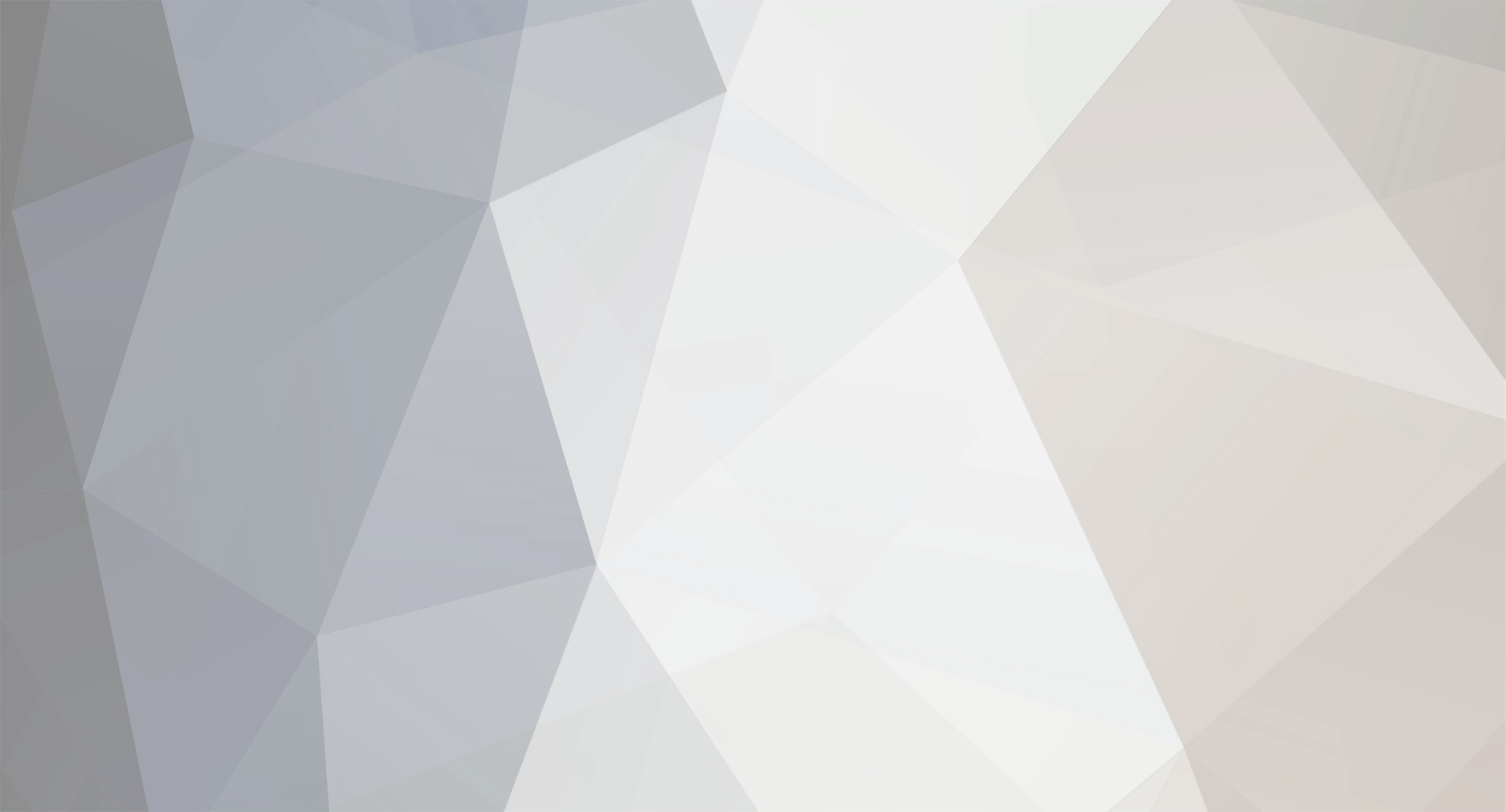
Lambda
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by Lambda
-
Oh I thought I had a spoiler with that My Bad. Here is the ItemEnergy: public abstract class ItemEnergy extends ItemBase{ private final int maxPower; private final int transfer; public ItemEnergy(int maxPower, int transfer, String name){ super(name); this.maxPower = maxPower; this.transfer = transfer; this.setHasSubtypes(true); this.setMaxStackSize(1); } @Override public boolean getShareTag(){ return true; } @Override public void addInformation(ItemStack stack, EntityPlayer player, List list, boolean bool){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ NumberFormat format = NumberFormat.getInstance(); list.add(format.format(storage.getEnergyStored())+"/"+format.format(storage.getMaxEnergyStored())+" Crystal Flux"); } } } @Override @SideOnly(Side.CLIENT) public boolean hasEffect(ItemStack stack){ return false; } @Override @SideOnly(Side.CLIENT) public void getSubItems(Item item, CreativeTabs tabs, NonNullList list){ ItemStack stackFull = new ItemStack(this); if(stackFull.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stackFull.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ this.setEnergy(stackFull, storage.getMaxEnergyStored()); list.add(stackFull); } } ItemStack stackEmpty = new ItemStack(this); this.setEnergy(stackEmpty, 0); list.add(stackEmpty); } @Override public boolean showDurabilityBar(ItemStack itemStack){ return true; } @Override public double getDurabilityForDisplay(ItemStack stack){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ double maxAmount = storage.getMaxEnergyStored(); double energyDif = maxAmount-storage.getEnergyStored(); return energyDif/maxAmount; } } return super.getDurabilityForDisplay(stack); } @Override public int getRGBDurabilityForDisplay(ItemStack stack){ EntityPlayer player = PlentifulMisc.proxy.getCurrentPlayer(); if(player != null && player.worldObj != null){ float[] color = AssetUtil.getRGB(player.worldObj.getTotalWorldTime()%256); return MathHelper.rgb(color[0]/255F, color[1]/255F, color[2]/255F); } return super.getRGBDurabilityForDisplay(stack); } public void setEnergy(ItemStack stack, int energy){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage instanceof CustomEnergyStorage){ ((CustomEnergyStorage)storage).setEnergyStored(energy); } } } public int receiveEnergyInternal(ItemStack stack, int maxReceive, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage instanceof CustomEnergyStorage){ ((CustomEnergyStorage)storage).receiveEnergyInternal(maxReceive, simulate); } } return 0; } public int extractEnergyInternal(ItemStack stack, int maxExtract, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage instanceof CustomEnergyStorage){ ((CustomEnergyStorage)storage).extractEnergyInternal(maxExtract, simulate); } } return 0; } public int receiveEnergy(ItemStack stack, int maxReceive, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.receiveEnergy(maxReceive, simulate); } } return 0; } public int extractEnergy(ItemStack stack, int maxExtract, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.extractEnergy(maxExtract, simulate); } } return 0; } public int getEnergyStored(ItemStack stack){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.getEnergyStored(); } } return 0; } public int getMaxEnergyStored(ItemStack stack){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.getMaxEnergyStored(); } } return 0; } @Override public ICapabilityProvider initCapabilities(ItemStack stack, NBTTagCompound nbt){ return new EnergyCapabilityProvider(stack, this); } private static class EnergyCapabilityProvider implements ICapabilityProvider{ private final CustomEnergyStorage storage; public EnergyCapabilityProvider(final ItemStack stack, ItemEnergy item){ this.storage = new CustomEnergyStorage(item.maxPower, item.transfer, item.transfer){ @Override public int getEnergyStored(){ if(stack.hasTagCompound()){ return stack.getTagCompound().getInteger("Energy"); } else{ return 0; } } @Override public void setEnergyStored(int energy){ if(!stack.hasTagCompound()){ stack.setTagCompound(new NBTTagCompound()); } stack.getTagCompound().setInteger("Energy", energy); } }; } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing){ return this.getCapability(capability, facing) != null; } @Nullable @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing){ if(capability == CapabilityEnergy.ENERGY){ return (T)this.storage; } return null; } } } And the battery as an example, all energy Items are crashing: public class ItemBattery extends ItemEnergy{ public ItemBattery(String name, int capacity, int transfer){ super(capacity, transfer, name); this.setMaxStackSize(1); } @Override public EnumRarity getRarity(ItemStack stack){ return EnumRarity.RARE; } @Override public boolean hasEffect(ItemStack stack){ return ItemUtil.isEnabled(stack); } @Override public void onUpdate(ItemStack stack, World world, Entity entity, int itemSlot, boolean isSelected){ if(!world.isRemote && entity instanceof EntityPlayer && ItemUtil.isEnabled(stack)){ EntityPlayer player = (EntityPlayer)entity; for(int i = 0; i < player.inventory.getSizeInventory(); i++){ ItemStack slot = player.inventory.getStackInSlot(i); if(StackUtil.isValid(slot)){ int extractable = this.extractEnergy(stack, Integer.MAX_VALUE, true); int received = 0; if(slot.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage cap = slot.getCapability(CapabilityEnergy.ENERGY, null); if(cap != null){ received = cap.receiveEnergy(extractable, false); } } if(received > 0){ this.extractEnergy(stack, received, false); } } } } } @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer player, EnumHand hand){ if(!worldIn.isRemote && player.isSneaking()){ ItemUtil.changeEnabled(player, hand); return new ActionResult<ItemStack>(EnumActionResult.SUCCESS, player.getHeldItem(hand)); } return super.onItemRightClick(worldIn, player, hand); } @Override public void addInformation(ItemStack stack, EntityPlayer player, List list, boolean bool){ super.addInformation(stack, player, list, bool); list.add(StringUtil.localize("tooltip."+ModUtil.MOD_ID+".battery."+(ItemUtil.isEnabled(stack) ? "discharge" : "noDischarge"))); list.add(StringUtil.localize("tooltip."+ModUtil.MOD_ID+".battery.changeMode")); } }
-
Usually, I don't like doing this, find it kinda scummy :^| *cough* Well, maybe not all that useless, Some Update on the situation: Anytime I open the Creative Tab it crashes with the same error, but during Pre-Init I get: [19:40:01] [Client thread/INFO]: Starting JEI... [19:40:01] [Client thread/ERROR]: Creative tab crashed while getting items. Some items from this tab will be missing from the item list. com.lambda.plentifulmisc.creative.CreativeTab@31092e1f java.lang.ClassCastException: com.lambda.plentifulmisc.items.base.ItemEnergy$EnergyCapabilityProvider$1 cannot be cast to net.minecraftforge.energy.IEnergyStorage at com.lambda.plentifulmisc.items.base.ItemEnergy.getSubItems(ItemEnergy.java:72) ~[itemEnergy.class:?] at net.minecraft.creativetab.CreativeTabs.displayAllRelevantItems(CreativeTabs.java:301) ~[CreativeTabs.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.create(ItemStackListFactory.java:33) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.VanillaPlugin.registerIngredients(VanillaPlugin.java:92) [VanillaPlugin.class:?] at mezz.jei.JeiStarter.registerIngredients(JeiStarter.java:110) [JeiStarter.class:?] at mezz.jei.JeiStarter.start(JeiStarter.java:36) [JeiStarter.class:?] at mezz.jei.ProxyCommonClient.loadComplete(ProxyCommonClient.java:111) [ProxyCommonClient.class:?] at mezz.jei.JustEnoughItems.loadComplete(JustEnoughItems.java:53) [JustEnoughItems.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:602) [FMLModContainer.class:?] at sun.reflect.GeneratedMethodAccessor7.invoke(Unknown Source) ~[?:?] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:243) [LoadController.class:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:221) [LoadController.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:145) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:804) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:331) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] [19:40:01] [Client thread/WARN]: Found an empty subItem of net.minecraft.item.ItemAir@16a3d9a7 [19:40:01] [Client thread/WARN]: Caught a crash while getting sub-items of com.lambda.plentifulmisc.items.ItemBattery@4111c29d java.lang.ClassCastException: com.lambda.plentifulmisc.items.base.ItemEnergy$EnergyCapabilityProvider$1 cannot be cast to net.minecraftforge.energy.IEnergyStorage at com.lambda.plentifulmisc.items.base.ItemEnergy.getSubItems(ItemEnergy.java:72) ~[itemEnergy.class:?] at mezz.jei.util.StackHelper.getSubtypes(StackHelper.java:242) [stackHelper.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.addItemAndSubItems(ItemStackListFactory.java:66) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.create(ItemStackListFactory.java:55) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.VanillaPlugin.registerIngredients(VanillaPlugin.java:92) [VanillaPlugin.class:?] at mezz.jei.JeiStarter.registerIngredients(JeiStarter.java:110) [JeiStarter.class:?] at mezz.jei.JeiStarter.start(JeiStarter.java:36) [JeiStarter.class:?] at mezz.jei.ProxyCommonClient.loadComplete(ProxyCommonClient.java:111) [ProxyCommonClient.class:?] at mezz.jei.JustEnoughItems.loadComplete(JustEnoughItems.java:53) [JustEnoughItems.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:602) [FMLModContainer.class:?] at sun.reflect.GeneratedMethodAccessor7.invoke(Unknown Source) ~[?:?] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:243) [LoadController.class:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:221) [LoadController.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:145) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:804) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:331) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] [19:40:01] [Client thread/WARN]: Caught a crash while getting sub-items of com.lambda.plentifulmisc.items.ItemDrill@80cb5f6 java.lang.ClassCastException: com.lambda.plentifulmisc.items.base.ItemEnergy$EnergyCapabilityProvider$1 cannot be cast to net.minecraftforge.energy.IEnergyStorage at com.lambda.plentifulmisc.items.base.ItemEnergy.getMaxEnergyStored(ItemEnergy.java:173) ~[itemEnergy.class:?] at com.lambda.plentifulmisc.items.ItemDrill.addDrillStack(ItemDrill.java:323) ~[itemDrill.class:?] at com.lambda.plentifulmisc.items.ItemDrill.getSubItems(ItemDrill.java:317) ~[itemDrill.class:?] at mezz.jei.util.StackHelper.getSubtypes(StackHelper.java:242) [stackHelper.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.addItemAndSubItems(ItemStackListFactory.java:66) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.create(ItemStackListFactory.java:55) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.VanillaPlugin.registerIngredients(VanillaPlugin.java:92) [VanillaPlugin.class:?] at mezz.jei.JeiStarter.registerIngredients(JeiStarter.java:110) [JeiStarter.class:?] at mezz.jei.JeiStarter.start(JeiStarter.java:36) [JeiStarter.class:?] at mezz.jei.ProxyCommonClient.loadComplete(ProxyCommonClient.java:111) [ProxyCommonClient.class:?] at mezz.jei.JustEnoughItems.loadComplete(JustEnoughItems.java:53) [JustEnoughItems.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:602) [FMLModContainer.class:?] at sun.reflect.GeneratedMethodAccessor7.invoke(Unknown Source) ~[?:?] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:243) [LoadController.class:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:221) [LoadController.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:145) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:804) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:331) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] [19:40:01] [Client thread/WARN]: Caught a crash while getting sub-items of com.lambda.plentifulmisc.items.ItemBattery@2a7eb66e java.lang.ClassCastException: com.lambda.plentifulmisc.items.base.ItemEnergy$EnergyCapabilityProvider$1 cannot be cast to net.minecraftforge.energy.IEnergyStorage at com.lambda.plentifulmisc.items.base.ItemEnergy.getSubItems(ItemEnergy.java:72) ~[itemEnergy.class:?] at mezz.jei.util.StackHelper.getSubtypes(StackHelper.java:242) [stackHelper.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.addItemAndSubItems(ItemStackListFactory.java:66) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.ingredients.ItemStackListFactory.create(ItemStackListFactory.java:55) [itemStackListFactory.class:?] at mezz.jei.plugins.vanilla.VanillaPlugin.registerIngredients(VanillaPlugin.java:92) [VanillaPlugin.class:?] at mezz.jei.JeiStarter.registerIngredients(JeiStarter.java:110) [JeiStarter.class:?] at mezz.jei.JeiStarter.start(JeiStarter.java:36) [JeiStarter.class:?] at mezz.jei.ProxyCommonClient.loadComplete(ProxyCommonClient.java:111) [ProxyCommonClient.class:?] at mezz.jei.JustEnoughItems.loadComplete(JustEnoughItems.java:53) [JustEnoughItems.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:602) [FMLModContainer.class:?] at sun.reflect.GeneratedMethodAccessor7.invoke(Unknown Source) ~[?:?] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:243) [LoadController.class:?] at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:221) [LoadController.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) [guava-17.0.jar:?] at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) [guava-17.0.jar:?] at com.google.common.eventbus.EventBus.post(EventBus.java:275) [guava-17.0.jar:?] at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:145) [LoadController.class:?] at net.minecraftforge.fml.common.Loader.initializeMods(Loader.java:804) [Loader.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:331) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] I still can't find a reason, I'm almost certain these can be cast, especially since the IDE isn't giving me an error
-
Hey there, So I'm having an issue with one of my items, in which I'm casting IEnergyStorage to Capability.Energy. However, I'm getting a crash saying that I cannot cast it to each other. This worked in 1.10 however. java.lang.ClassCastException: com.lambda.plentifulmisc.items.base.ItemEnergy$EnergyCapabilityProvider$1 cannot be cast to net.minecraftforge.energy.IEnergyStorage at com.lambda.plentifulmisc.items.base.ItemEnergy.getMaxEnergyStored(ItemEnergy.java:175) at com.lambda.plentifulmisc.items.ItemDrill.addDrillStack(ItemDrill.java:324) at com.lambda.plentifulmisc.items.ItemDrill.getSubItems(ItemDrill.java:318) at net.minecraft.creativetab.CreativeTabs.displayAllRelevantItems(CreativeTabs.java:301) at net.minecraft.client.gui.inventory.GuiContainerCreative.setCurrentCreativeTab(GuiContainerCreative.java:493) at net.minecraft.client.gui.inventory.GuiContainerCreative.mouseReleased(GuiContainerCreative.java:464) at net.minecraft.client.gui.GuiScreen.handleMouseInput(GuiScreen.java:621) at net.minecraft.client.gui.inventory.GuiContainerCreative.handleMouseInput(GuiContainerCreative.java:585) at net.minecraft.client.gui.GuiScreen.handleInput(GuiScreen.java:576) at net.minecraft.client.Minecraft.runTick(Minecraft.java:1790) at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1117) at net.minecraft.client.Minecraft.run(Minecraft.java:405) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) Here is the item: @Override @SideOnly(Side.CLIENT) public void getSubItems(Item item, CreativeTabs tabs, NonNullList list){ ItemStack stackFull = new ItemStack(this); if(stackFull.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stackFull.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ this.setEnergy(stackFull, storage.getMaxEnergyStored()); list.add(stackFull); } } ItemStack stackEmpty = new ItemStack(this); this.setEnergy(stackEmpty, 0); list.add(stackEmpty); } @Override public boolean showDurabilityBar(ItemStack itemStack){ return true; } @Override public double getDurabilityForDisplay(ItemStack stack){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ double maxAmount = storage.getMaxEnergyStored(); double energyDif = maxAmount-storage.getEnergyStored(); return energyDif/maxAmount; } } return super.getDurabilityForDisplay(stack); } @Override public int getRGBDurabilityForDisplay(ItemStack stack){ EntityPlayer player = PlentifulMisc.proxy.getCurrentPlayer(); if(player != null && player.worldObj != null){ float[] color = AssetUtil.getRGB(player.worldObj.getTotalWorldTime()%256); return MathHelper.rgb(color[0]/255F, color[1]/255F, color[2]/255F); } return super.getRGBDurabilityForDisplay(stack); } public void setEnergy(ItemStack stack, int energy){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage instanceof CustomEnergyStorage){ ((CustomEnergyStorage)storage).setEnergyStored(energy); } } } public int receiveEnergyInternal(ItemStack stack, int maxReceive, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage instanceof CustomEnergyStorage){ ((CustomEnergyStorage)storage).receiveEnergyInternal(maxReceive, simulate); } } return 0; } public int extractEnergyInternal(ItemStack stack, int maxExtract, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage instanceof CustomEnergyStorage){ ((CustomEnergyStorage)storage).extractEnergyInternal(maxExtract, simulate); } } return 0; } public int receiveEnergy(ItemStack stack, int maxReceive, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.receiveEnergy(maxReceive, simulate); } } return 0; } public int extractEnergy(ItemStack stack, int maxExtract, boolean simulate){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.extractEnergy(maxExtract, simulate); } } return 0; } public int getEnergyStored(ItemStack stack){ if(stack.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stack.getCapability(CapabilityEnergy.ENERGY, null); if(storage != null){ return storage.getEnergyStored(); } } return 0; } All these lines do this: ItemStack stackFull = new ItemStack(this); if(stackFull.hasCapability(CapabilityEnergy.ENERGY, null)){ IEnergyStorage storage = stackFull.getCapability(CapabilityEnergy.ENERGY, null); Thanks.
-
Hey there! So I'm running into a bit of an issue getting all connected TEs, and sending energy to them. With the current system I had, it would send it to the closest one, making the rest of the TEs on the line, not receive power. I have a working prototype, however, it seems to update the list every update tick, which makes since. However, having this happens makes the power transfer incredibly slow and causes about 4-5 frame drops. I know there is a more efficient way of doing so, just looking for some pointers. Please keep in mind, that this is all a prototype, I honestly dont know much about coding this game, so some stuff might looked fucked up and wrong. Please keep an open mind Here is the TE code: public class TileEntityPipeEnergy extends TileEntityBase implements ITickable, IEnergyReceiver, IEnergyProvider { public EnergyStorage storage = new EnergyStorage(16000); public static int SENDPERTICK = 16000; public final ConcurrentHashMap<EnumFacing, TileEntity> cablesAround = new ConcurrentHashMap<EnumFacing, TileEntity>(); public ArrayList<TileEntity> connectionList = new ArrayList(); public TileEntityPipeEnergy() { super("energypipe"); } @Override public void updateEntity() { super.updateEntity(); System.out.print(connectionList.size()); int energyStored = getEnergyStored(EnumFacing.DOWN); for (EnumFacing facing : EnumFacing.values()) { BlockPos pos = getPos().offset(facing); TileEntity te = worldObj.getTileEntity(pos); if (acceptableTE(te)) { EnumFacing opposite = facing.getOpposite(); int rfToGive = SENDPERTICK <= energyStored ? SENDPERTICK : energyStored; int received; if (te instanceof IEnergyConnection) { IEnergyConnection connection = (IEnergyConnection) te; if (connection.canConnectEnergy(opposite)) { received = receiveEnergy(te, opposite, rfToGive); cablesAround.put(facing, te); } else { received = 0; cablesAround.remove(facing, te); } } else { received = receiveEnergy(te, opposite, rfToGive); } energyStored -= storage.extractEnergy(received, false); if (energyStored <= 0) { break; } } } for (Map.Entry<EnumFacing, TileEntity> receiver : cablesAround.entrySet()) { connectionList.add(receiver.getValue()); } } public int receiveEnergy(TileEntity tileEntity, EnumFacing from, int maxReceive) { for(int i = 0; i < connectionList.size(); i++) { if (tileEntity instanceof IEnergyReceiver) { return ((IEnergyReceiver) tileEntity).receiveEnergy(from, maxReceive / connectionList.size(), false); } else if (tileEntity != null && tileEntity.hasCapability(CapabilityEnergy.ENERGY, from)) { net.minecraftforge.energy.IEnergyStorage capability = tileEntity.getCapability(CapabilityEnergy.ENERGY, from); if (capability.canReceive()) { return capability.receiveEnergy(maxReceive / connectionList.size(), false); } } } return 0; } public static boolean acceptableTE(TileEntity te) { return te instanceof IEnergyHandler || (te != null && te.hasCapability(CapabilityEnergy.ENERGY, null)); } @Override public void writeSyncableNBT(NBTTagCompound compound, NBTType type) { super.writeSyncableNBT(compound, type); this.storage.writeToNBT(compound); } @Override public void readSyncableNBT(NBTTagCompound compound, NBTType type) { super.readSyncableNBT(compound, type); storage.readFromNBT(compound); } @Override public int getEnergyStored(EnumFacing from) { return storage.getEnergyStored(); } @Override public int getMaxEnergyStored(EnumFacing from) { return storage.getMaxEnergyStored(); } public int getEnergyStored() { return storage.getEnergyStored(); } public int getMaxEnergyStored() { return storage.getMaxEnergyStored(); } @Override public boolean canConnectEnergy(EnumFacing from) { return true; } @Override public int extractEnergy(EnumFacing from, int maxExtract, boolean simulate) { return storage.extractEnergy(maxExtract, simulate); } @Override public int receiveEnergy(EnumFacing from, int maxReceive, boolean simulate) { return storage.receiveEnergy(maxReceive, simulate); } }
-
Okay, This is the only block that does this however, private void register(){ ItemUtil.registerBlock(this, this.getItemBlock(), this.getBaseName(), this.shouldAddCreative()); this.registerRendering(); } This is in my BaseBlockContainer class, inwhich it handles registration, register rendering : protected void registerRendering(){ PlentifulMisc.proxy.addRenderRegister(new ItemStack(this), this.getRegistryName(), "inventory"); } lastly, the client proxy; private static final Map<ItemStack, ModelResourceLocation> MODEL_LOCATIONS_FOR_REGISTERING = new HashMap<ItemStack, ModelResourceLocation>(); @Override public void preInit(FMLPreInitializationEvent event) { ModUtil.LOGGER.info("PreInitializing ClientProxy..."); for(Map.Entry<ItemStack, ModelResourceLocation> entry : MODEL_LOCATIONS_FOR_REGISTERING.entrySet()){ ModelLoader.setCustomModelResourceLocation(entry.getKey().getItem(), entry.getKey().getItemDamage(), entry.getValue()); } } @Override public void init(FMLInitializationEvent event) { } @Override public void postInit(FMLPostInitializationEvent event) { } @Override public void addRenderRegister(ItemStack stack, ResourceLocation location, String variant){ MODEL_LOCATIONS_FOR_REGISTERING.put(stack, new ModelResourceLocation(location, variant)); } }
-
Hey there, So I'm having an interesting error with trying to load a model that uses connections ( like redstone ), however, it says it cannot find a '.json.json' However, I have no reference where I would set it to look for a '.json.json' Here is the log: [20:10:10] [Client thread/ERROR]: Exception loading blockstate for the variant plentifulmisc:block_pipe_energy.json#down=true,east=true,north=false,south=true,up=false,west=false: java.lang.Exception: Could not load model definition for variant plentifulmisc:block_pipe_energy.json at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:274) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:121) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:229) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:146) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:798) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:347) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: java.lang.RuntimeException: Encountered an exception when loading model definition of model plentifulmisc:blockstates/block_pipe_energy.json.json at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:228) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:270) ~[ModelLoader.class:?] ... 28 more Caused by: java.io.FileNotFoundException: plentifulmisc:blockstates/block_pipe_energy.json.json at net.minecraft.client.resources.FallbackResourceManager.getAllResources(FallbackResourceManager.java:104) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getAllResources(SimpleReloadableResourceManager.java:79) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadMultipartMBD(ModelBakery.java:221) ~[ModelBakery.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.getModelBlockDefinition(ModelBakery.java:208) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.getModelBlockDefinition(ModelLoader.java:270) ~[ModelLoader.class:?] ... 28 more [20:10:10] [Client thread/ERROR]: Exception loading model for variant plentifulmisc:block_pipe_energy.json#down=false,east=false,north=true,south=false,up=true,west=false for blockstate "plentifulmisc:block_pipe_energy.json[down=false,east=false,north=true,south=false,up=true,west=false]" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model plentifulmisc:block_pipe_energy.json#down=false,east=false,north=true,south=false,up=true,west=false with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:241) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:153) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:229) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:146) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:798) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:347) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:560) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:385) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at com.intellij.rt.execution.application.AppMain.main(AppMain.java:147) [idea_rt.jar:?] Caused by: net.minecraft.client.renderer.block.model.ModelBlockDefinition$MissingVariantException at net.minecraft.client.renderer.block.model.ModelBlockDefinition.getVariant(ModelBlockDefinition.java:78) ~[ModelBlockDefinition.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1184) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 29 more Then I see its saying missing variant, so I'll give you the .json for the pipe: { "multipart": [ {"apply": {"model": "plentifulmisc:block_pipe_energy" }}, {"when": { "down": "true"}, "apply": { "model": "plentifulmisc:block_pipe_energy_connected", "x": 180 }}, { "when": { "up": "true" }, "apply": { "model": "plentifulmisc:block_pipe_energy_connected" } }, { "when": { "south": "true" }, "apply": { "model": "plentifulmisc:block_pipe_energy_connected", "x": 270 } }, { "when": { "north": "true" }, "apply": { "model": "plentifulmisc:block_pipe_energy_connected", "x": 90 } }, { "when": { "west": "true" }, "apply": { "model": "plentifulmisc:block_pipe_energy_connected", "x": 90, "y": 270 } }, { "when": { "east": "true" }, "apply": { "model": "plentifulmisc:block_pipe_energy_connected", "x": 90, "y": 90 } } ] } Also, how I'm setting the properties: public static final PropertyBool[] PROPERTIES= new PropertyBool[]{ PropertyBool.create("down"), PropertyBool.create("up"), PropertyBool.create("north"), PropertyBool.create("south"), PropertyBool.create("west"), PropertyBool.create("east") };
-
Using the Debugger, i found that it is finding the TE, however not transferring energy for some reason.
-
... So something like this? @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if(capability == CapabilityEnergy.ENERGY) { return true; } return super.hasCapability(capability, facing); } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (capability == CapabilityEnergy.ENERGY) { return (T) energyStorage; } return super.getCapability(capability, facing); } at the bottom of the class? I'm handling the energy with CoFH API.
-
Yeah I do it with inventories with some help from others, however wasn't really given a reference on how they work. Thanks!
-
I'm not particularly good with them Where should I read up on them? And how would I apply them to this?
-
[1.11] NBT does not save upon rebooting the game.
Lambda replied to Lambda's topic in Modder Support
So how would I read from the world? -
Hey there, So I have a prototype cable here, to transfer energy across a line, which detecting TEs along the way, if one is found it sends energy to them. However, it does not work, as in, the energy IS not transfer if one is found. Here is the class: public class TileEntityPipeEnergy extends TileEntityBase implements ITickable, ICustomEnergyReceiver, ISharingEnergyProvider { public EnergyStorage energyStorage = new EnergyStorage(6000, 6000); public TileEntityPipeEnergy() { super("energypipe"); } @Override public void updateEntity() { super.updateEntity(); List<EnumFacing> possibleSides = getPossibleSides(); if (!possibleSides.isEmpty()) { int forEach = energyStorage.getEnergyStored() / possibleSides.size(); for (EnumFacing side : possibleSides) { BlockPos neighbor = pos.offset(side); TileEntity tile = worldObj.getTileEntity(neighbor); if (tile != null) { if (tile instanceof ICustomEnergyReceiver) { transferEnergyToTile((ICustomEnergyReceiver) tile, side, forEach); } else if (tile instanceof ICustomEnergyReceiver) { transferEnergyToTile((ICustomEnergyReceiver) tile, side, forEach); } } } } } private void transferEnergyToTile(ICustomEnergyReceiver receiver, EnumFacing side, int energyAmount) { if (receiver.canConnectEnergy(side.getOpposite())) { receiver.receiveEnergy(side.getOpposite(), extractEnergy(side, energyAmount, false), false); } } public List<EnumFacing> getPossibleSides() { List<EnumFacing> sides = new ArrayList<EnumFacing>(); for (EnumFacing side : EnumFacing.VALUES) { BlockPos neighbor = pos.offset(side); TileEntity tile = worldObj.getTileEntity(neighbor); if (tile != null) { if (tile instanceof IEnergyReceiver) { sides.add(side); } } } if (sides.size() != 0) { int forEach = energyStorage.getEnergyStored() / sides.size(); for (EnumFacing side : EnumFacing.VALUES) { if (sides.contains(side)) { BlockPos neighbor = pos.offset(side); TileEntity tile = worldObj.getTileEntity(neighbor); if (tile != null && tile instanceof ICustomEnergyReceiver) { ICustomEnergyReceiver receiver = (ICustomEnergyReceiver) tile; if (receiver.getEnergyStored(side.getOpposite()) + forEach > receiver.getMaxEnergyStored(side.getOpposite())) { sides.remove(side); } if (!receiver.canConnectEnergy(side.getOpposite())) { sides.remove(side); } } } } } return sides; } public int getEnergyToSplitShare() { return this.getEnergyStored(); } public boolean doesShareEnergy() { return true; } public EnumFacing[] getEnergyShareSides() { return EnumFacing.values(); } @Override public void writeSyncableNBT(NBTTagCompound compound, NBTType type){ super.writeSyncableNBT(compound, type); this.energyStorage.writeToNBT(compound); } @Override public void readSyncableNBT(NBTTagCompound compound, NBTType type){ super.readSyncableNBT(compound, type); this.energyStorage.readFromNBT(compound); } @Override public int getEnergyStored(EnumFacing from) { return energyStorage.getEnergyStored(); } @Override public int getMaxEnergyStored(EnumFacing from) { return energyStorage.getMaxEnergyStored(); } public int getEnergyStored() { return energyStorage.getEnergyStored(); } public int getMaxEnergyStored() { return energyStorage.getMaxEnergyStored(); } @Override public boolean canConnectEnergy(EnumFacing from) { return worldObj.isBlockIndirectlyGettingPowered(pos) >= 1; } @Override public int extractEnergy(EnumFacing from, int maxExtract, boolean simulate) { return energyStorage.extractEnergy(maxExtract, simulate); } @Override public int receiveEnergy(EnumFacing from, int maxReceive, boolean simulate) { return energyStorage.receiveEnergy(maxReceive, simulate); } } Thanks for your time.
-
Well, now its just the block model json, you can easily create on using Mr.Crayfish's Model Creator: https://mrcrayfish.com/tools?id=mc
-
When I was porting my mod to github, I ran into some issues when creating another Idea project, Everything is fine, other than there are no run configurations. Thanks in advanced.
-
Also, get this error after validation: Exception in thread "main" java.lang.ClassNotFoundException: GradleStart at java.net.URLClassLoader.findClass(URLClassLoader.java:381) at java.lang.ClassLoader.loadClass(ClassLoader.java:424) at sun.misc.Launcher$AppClassLoader.loadClass(Launcher.java:331) at java.lang.ClassLoader.loadClass(ClassLoader.java:357) at java.lang.Class.forName0(Native Method) at java.lang.Class.forName(Class.java:264) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:123)
-
Hey there, I recently did a push into my GitHub, however when I tried lauching again I get this error: java.lang.NoClassDefFoundError: org/apache/tools/ant/util/ReaderInputStream
-
Hey there, So I'm having an issue saving connections between tile entities, they save when disconnect + reconnect, however, when I close the game completely the connections seems to get reset. Anything I could do? Here is how I'm reading/writing the NBT: @Override public void readSyncableNBT(NBTTagCompound compound, NBTType type){ super.readSyncableNBT(compound, type); if(type == NBTType.SYNC){ PlentifulMiscAPI.connectionHandler.removeRelayFromNetwork(this.pos, this.worldObj); NBTTagList list = compound.getTagList("Connections", 10); if(!list.hasNoTags()){ for(int i = 0; i < list.tagCount(); i++){ ConnectionPair pair = new ConnectionPair(); pair.readFromNBT(list.getCompoundTagAt(i)); PlentifulMiscAPI.connectionHandler.addConnection(pair.getPositions()[0], pair.getPositions()[1], this.type, this.worldObj, pair.doesSuppressRender()); } } } } @Override public void writeSyncableNBT(NBTTagCompound compound, NBTType type){ super.writeSyncableNBT(compound, type); if(type == NBTType.SYNC){ NBTTagList list = new NBTTagList(); ConcurrentSet<IConnectionPair> connections = PlentifulMiscAPI.connectionHandler.getConnectionsFor(this.pos, this.worldObj); if(connections != null && !connections.isEmpty()){ for(IConnectionPair pair : connections){ NBTTagCompound tag = new NBTTagCompound(); pair.writeToNBT(tag); list.appendTag(tag); } } compound.setTag("Connections", list); } }
-
How can I make a gun mod with a magazine?
Lambda replied to Skullmassarce7's topic in Modder Support
As long as you know some basic java, and know how to create items.. Then it should be pretty self-explanatory, just look the bow for reference. -
Oh, didnt know that world.getTileEntity could take an argument. And yeah bit of a typo on the for loop, i < 10.
-
Hello, So I'm in need to find any tile entities around the main tile, however, I'm having trouble trying to get instancesof the other TE: for(int i=0; i > 10; i++) { for(sbPos.offset(EnumFacing.EAST, i)) { } } sb is the te itself, however, im having trouble trying to get the positions if the other TEs do exist.
-
okay, so know when trying to validate the slot for input, I'm having issues setting the itemStack to the list that getResult() will take: @Override public boolean isItemValidForSlot(int i, ItemStack stack){ return (i==SLOT_INPUT_1) && StackUtil.isValid(CrusherRecipes.instance().getResult(stack)); //Cannot return just an ItemStack, must be a list } Trying to set the parameter to List<ItemStack> doesn't seem to work correctly.
-
Yes, it is.
-
So something like this? public List<ItemStack> getResult(ItemStack input) { for(CrusherRecipeHandler recipe : crusherList) { if(ItemUtil.areItemsEqual(recipe.input, input, true)) { ArrayList list = new ArrayList(); list.add(recipe.output); list.add(recipe.outputChance); return list; } } return null }
-
Hello, So, I'm needing to return multiple itemstacks for when 2 items are equal, however, I'm having trouble doing this.. Here is what I have currently: public ItemStack getResult(ItemStack input) { for(CrusherRecipeHandler recipe : crusherList) { if(ItemUtil.areItemsEqual(recipe.input, input, true)) { return ; } } } I need to return recipe.output and recipe.outputChance Both of which are itemstacks. Using ItemStack[] doesnt seem to work, as it returns mutiple of ONE itemstack. Thanks.
-
Okay thanks for your help! Really amazing community around here!