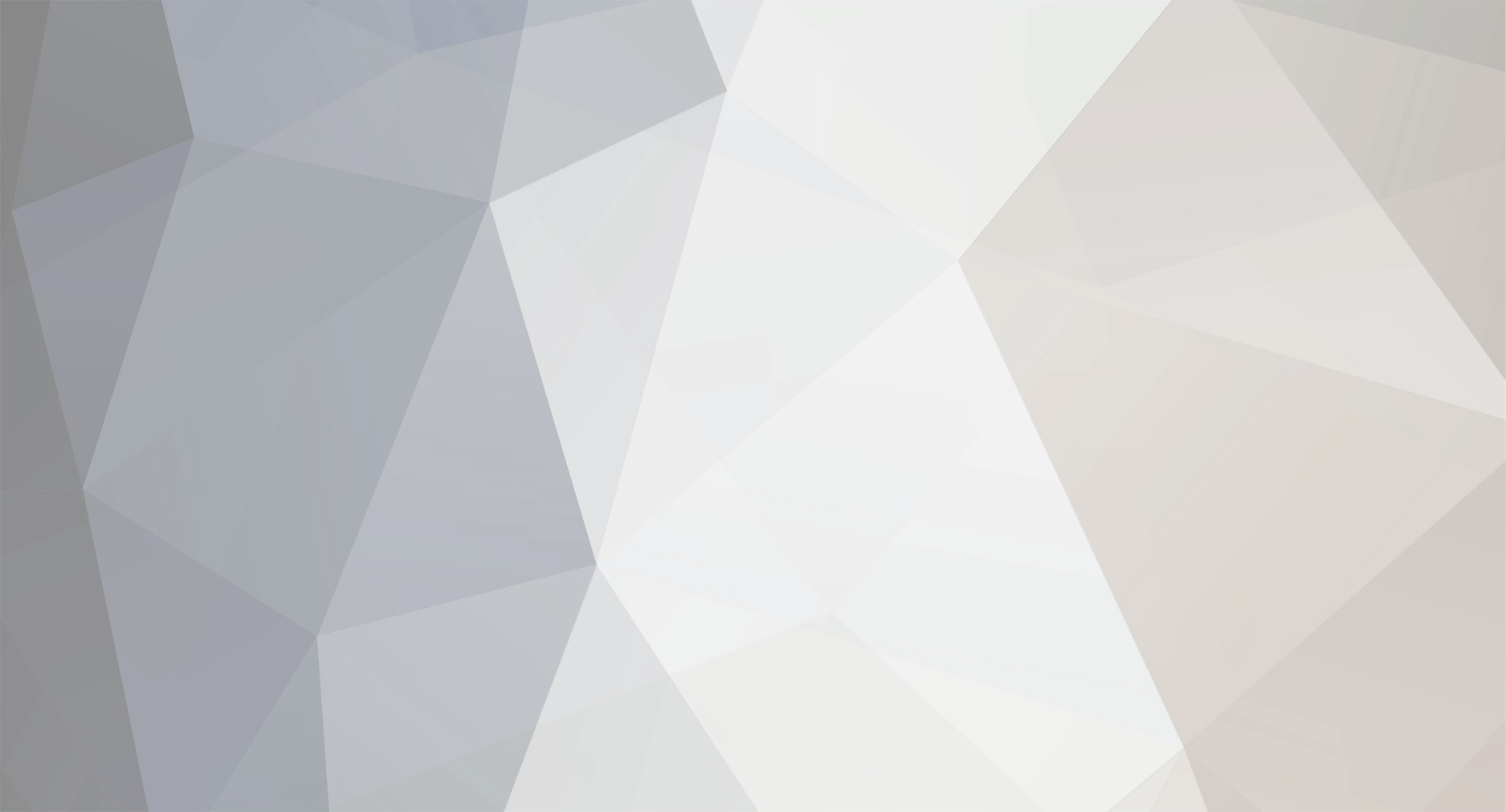
Lambda
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by Lambda
-
Hey there, So I have this capability that created a mana value, and I'm displaying that via onRenderGameOverlay . When I add to the capability, the overlay updates fine. However, when I subtract it from the player by a TE it subtracts, but does not update the overlay. However, when I subtract the values, I get 2 values that are outputted from the log, the original and the after value. ex: 15000 8100 15000 8100 So maybe its something to do with the network? However, I dont really know how to packet my information. Maybe a tutorial I can look at? Here are the classes: ManaEvents: public class ManaEvent { protected FontRenderer fontRendererObj; @SubscribeEvent public static void onRenderGameOverlay(RenderGameOverlayEvent event) { Minecraft mc = Minecraft.getMinecraft(); if(!event.isCancelable() && event.getType() == RenderGameOverlayEvent.ElementType.TEXT) { // mc.renderEngine.bindTexture(new ResourceLocation(ModUtil.MOD_ID + ":" + "textures/gui/mana.png")); CapabilityManaData storage = mc.thePlayer.getCapability(CapabilityMagic.MANA, null); String string = "MANA: " + Integer.toString(storage.getManaStored()); mc.fontRendererObj.drawString(string, 50 + 1, 50, 50); } } @SubscribeEvent public static void onAddCapabilitiesEntity(AttachCapabilitiesEvent<Entity> e) { if (canHaveAttributes(e.getObject())) { EntityLivingBase ent = (EntityLivingBase) e.getObject(); if (ent instanceof EntityPlayer) e.addCapability(CapabilityMagicProvider.KEY, new CapabilityMagicProvider(ent)); } } public static boolean canHaveAttributes(Entity entity) { if (entity instanceof EntityLivingBase) return true; return false; } } Capability Stuff: The TE thats not updating the capability: if(!this.worldObj.isRemote){ List<StandRecipeHandler> recipes = getRecipesForInput(this.slots.getStackInSlot(0)); EntityPlayer entityPlayer = worldObj.getClosestPlayer(this.getPos().getX(), this.getPos().getY(), this.getPos().getZ(), 5, false); if(!recipes.isEmpty() && entityPlayer != null){ if(entityPlayer.hasCapability(CapabilityMagic.MANA, null)) { CapabilityManaData capabilityManaData = entityPlayer.getCapability(CapabilityMagic.MANA, null); for (StandRecipeHandler recipe : recipes) { TileEntityStand[] modifierStands = this.getFittingModifiers(recipe, recipe.time); if (modifierStands != null) { //Meaning the display stands around match all the criteria if (capabilityManaData.getManaStored() >= recipe.requiredMana) { this.recipeForRenderIndex = PlentifulMiscAPI.STAND_RECIPES.indexOf(recipe); this.processTime++; boolean done = this.processTime >= recipe.time; for (TileEntityStand stand : modifierStands) { if (done) { stand.slots.decrStackSize(0, 1); } } if (this.processTime % 5 == 0 && this.worldObj instanceof WorldServer) { this.shootParticles(this.pos.getX() + 3, this.pos.getY() + 0.5, this.pos.getZ(), recipe.particleColor); this.shootParticles(this.pos.getX(), this.pos.getY() + 0.5, this.pos.getZ() + 3, recipe.particleColor); this.shootParticles(this.pos.getX() - 3, this.pos.getY() + 0.5, this.pos.getZ(), recipe.particleColor); this.shootParticles(this.pos.getX(), this.pos.getY() + 0.5, this.pos.getZ() - 3, recipe.particleColor); } if (done) { ((WorldServer) this.worldObj).spawnParticle(EnumParticleTypes.PORTAL, false, this.pos.getX() + 0.5, this.pos.getY() + 1.1, this.pos.getZ() + 0.5, 2, 0, 0, 0, 0.1D); this.slots.setStackInSlot(0, recipe.output.copy()); this.markDirty(); capabilityManaData.extractManaInternal(recipe.requiredMana, false); this.processTime = 0; this.recipeForRenderIndex = -1; } break; } else { entityPlayer.addChatMessage(new TextComponentTranslation("chat." + ModUtil.MOD_ID + ".stand.notenoughmana.warn")); } } else { entityPlayer.addChatMessage(new TextComponentTranslation("chat." + ModUtil.MOD_ID + ".stand.invalid.warn")); } } } } else{ this.processTime = 0; this.recipeForRenderIndex = -1; } if(this.lastRecipe != this.recipeForRenderIndex){ this.lastRecipe = this.recipeForRenderIndex; this.sendUpdate(); } } And if needed, some captioned pictures to explain whats happening: Thanks for reading.
-
Thanks for your response, Ah didn't catch that.. And yes, the capability WAS returning false on the player, sorry for the vague explanation, it was 3 in the morning Ended up fixed it by changing a few more things, I didnt have the CapabilityMagic set to the data, so it was not registering properly. It is working now So I thank you for that , will test out NBT write/read things shortly, so I'll reply back if there is another problem Thanks.
-
This doesnt seem to work: CapaProvider public class CapabilityMagicProvider implements ICapabilityProvider, ICapabilitySerializable<NBTTagCompound> { public static final ResourceLocation KEY = new ResourceLocation(ModUtil.MOD_ID, "mana"); private CapManaData INSTANCE = new CapManaData(); public CapabilityMagicProvider(EntityLivingBase entity) { INSTANCE.setEntity(entity); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return capability == CapabilityMagic.MANA; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (capability == CapabilityMagic.MANA) return (T) INSTANCE; return null; } @Override public NBTTagCompound serializeNBT() { return (NBTTagCompound) CapabilityMagic.MANA.writeNBT(INSTANCE, null); } @Override public void deserializeNBT(NBTTagCompound nbt) { CapabilityMagic.MANA.readNBT(INSTANCE, null, nbt); } } my CapaData: public class CapManaData implements IManaStorage { private EntityLivingBase entity; protected int mana; protected int capacity; protected int maxReceive; protected int maxExtract; public EntityLivingBase getEntity() { return entity; } public void setEntity(EntityLivingBase entity) { this.entity = entity; mana = this.getManaStored(); } @Override public int receiveMana(int maxReceive, boolean simulate) { if (!canReceive()) return 0; int energyReceived = Math.min(capacity - mana, Math.min(this.maxReceive, maxReceive)); if (!simulate) mana += energyReceived; return energyReceived; } @Override public int extractMana(int maxExtract, boolean simulate) { if (!canExtract()) return 0; int energyExtracted = Math.min(mana, Math.min(this.maxExtract, maxExtract)); if (!simulate) mana -= energyExtracted; return energyExtracted; } @Override public int getManaStored() { return mana; } @Override public int getMaxManaStored() { return capacity; } @Override public boolean canExtract() { return this.maxExtract > 0; } @Override public boolean canReceive() { return this.maxReceive > 0; } } my new events: @SubscribeEvent public static void onRenderGameOverlay(RenderGameOverlayEvent event) { Minecraft mc = Minecraft.getMinecraft(); if(!event.isCancelable() && event.getType() == RenderGameOverlayEvent.ElementType.EXPERIENCE) { int posX = event.getResolution().getScaledWidth() / 2 + 10; int posY = event.getResolution().getScaledHeight() - 48; // mc.renderEngine.bindTexture(new ResourceLocation(ModUtil.MOD_ID + ":" + "textures/gui/mana.png")); System.out.print(mc.thePlayer.hasCapability(CapabilityMagic.MANA, null)); IManaStorage storage = mc.thePlayer.getCapability(CapabilityMagic.MANA, null); // String string = "MANA: " + Integer.toString(storage.getManaStored() + 5 ); mc.fontRendererObj.drawString("yum", 50 + 1, 50, 50); } } @SubscribeEvent public void onAddCapabilitiesEntity(AttachCapabilitiesEvent<Entity> e) { if (canHaveAttributes(e.getObject())) { EntityLivingBase ent = (EntityLivingBase) e.getObject(); if (ent instanceof EntityPlayer) e.addCapability(CapabilityMagicProvider.KEY, new CapabilityMagicProvider(ent)); e.addCapability(CapabilityMagicProvider.KEY, new CapabilityMagicProvider(ent)); } } public static boolean canHaveAttributes(Entity entity) { if (entity instanceof EntityLivingBase) return true; return false; }
-
I dont think so, I dont know how to attach it to the player.
-
Okay still have a bit of an issue, it seems like the hunger bar is buggy and I cannot get the mana that my capability stores: Here is what the hunger bar looks like now: Fixed, via ElementType.EXPERIENCE. Also, doing this to get my capability results in a NullExecption: IManaStorage storage = mc.thePlayer.getCapability(CapabilityMagic.MANA, null); String string = "MANA: " + Integer.toString(storage.getManaStored()); mc.fontRendererObj.drawString(string, 50 + 1, 50, 50); Any pointers? I think it has to do with the capability isnt on the player in the first place. How would I do this? edit: Confirmed that the played does not have the capability via System.out.print(mc.thePlayer.hasCapability(CapabilityMagic.MANA, null)); how would I define one to the player?
-
I'm drawing it in my assetUtil: @SideOnly(Side.CLIENT) public static void displayNameString(FontRenderer font, int xSize, int yPositionOfMachineText, String text){ font.drawString(text, xSize/2-font.getStringWidth(text)/2, yPositionOfMachineText, StringUtil.DECIMAL_COLOR_WHITE); } @SideOnly(Side.CLIENT) public static void displayNameString(FontRenderer font, int xSize, int yPositionOfMachineText, TileEntityBase tile){ displayNameString(font, xSize, yPositionOfMachineText, tile.getDisplayedName()); }
-
Okay, this seemed NOT to work: public class ManaEvent { protected FontRenderer fontRendererObj; @SubscribeEvent public void onRenderGameOverlay(RenderGameOverlayEvent event) { Minecraft mc = Minecraft.getMinecraft(); if(!event.isCancelable()) { int posX = event.getResolution().getScaledWidth() / 2 + 10; int posY = event.getResolution().getScaledHeight() - 48; mc.renderEngine.bindTexture(new ResourceLocation(ModUtil.MOD_ID + ":" + "textures/gui/mana.png")); String string = CapabilityMagic.MANA.getStorage().toString() + " TEST"; AssetUtil.displayNameString(this.fontRendererObj, posX, posY, string); } } } registering via: MinecraftForge.EVENT_BUS.register(ManaEvent.class); in pre-init; However, will my capability display properly? And I should be able to add to it / remove / access it correct? I have it register and everything, here is the capa: public class CapabilityMagic { @CapabilityInject(IManaStorage.class) public static Capability<IManaStorage> MANA = null; public static void register() { CapabilityManager.INSTANCE.register(IManaStorage.class, new Capability.IStorage<IManaStorage>() { @Override public NBTBase writeNBT(Capability<IManaStorage> capability, IManaStorage instance, EnumFacing side) { return new NBTTagInt(instance.getManaStored()); } @Override public void readNBT(Capability<IManaStorage> capability, IManaStorage instance, EnumFacing side, NBTBase nbt) { if (!(instance instanceof ManaStorage)) throw new IllegalArgumentException("Can not deserialize to an instance that isn't the default implementation"); ((ManaStorage)instance).mana = ((NBTTagInt)nbt).getInt(); } }, new Callable<IManaStorage>() { @Override public IManaStorage call() throws Exception { return new ManaStorage(1000); } }); } } ManaStorage public class ManaStorage implements IManaStorage { protected int mana; protected int capacity; protected int maxReceive; protected int maxExtract; public ManaStorage(int capacity) { this(capacity, capacity, capacity); } public ManaStorage(int capacity, int maxTransfer) { this(capacity, maxTransfer, maxTransfer); } public ManaStorage(int capacity, int maxReceive, int maxExtract) { this.capacity = capacity; this.maxReceive = maxReceive; this.maxExtract = maxExtract; } @Override public int receiveMana(int maxReceive, boolean simulate) { if (!canReceive()) return 0; int energyReceived = Math.min(capacity - mana, Math.min(this.maxReceive, maxReceive)); if (!simulate) mana += energyReceived; return energyReceived; } @Override public int extractMana(int maxExtract, boolean simulate) { if (!canExtract()) return 0; int energyExtracted = Math.min(mana, Math.min(this.maxExtract, maxExtract)); if (!simulate) mana -= energyExtracted; return energyExtracted; } @Override public int getManaStored() { return mana; } @Override public int getMaxManaStored() { return capacity; } @Override public boolean canExtract() { return this.maxExtract > 0; } @Override public boolean canReceive() { return this.maxReceive > 0; } }
-
I have a part of a block I want to rotate when its doing x.
-
Yes, I want a part of the block being 'fullbright'
-
Okay, so how would I get a capability and display it / set it to a temp int? Aswell, how would I display it? what do I have to call, etc.
-
Hey there, So I'm in need of overlaying something over the screen when an item is held or equipped, I need it to display a % based on an int, so my questions are: - How would I make an int that stores information that can be accessed and is held to only one player (capability?) - How would I overlay that information Thanks for your time.
-
Yeah updated it, then fixed it, then removed it Still need help with animating the block.
-
Hey there, So I have a TileEntitySpecialRenderer , however, how would I animate parts of the blocks? Would I rotate them in the blockstate when a condition is true?
-
Yes it is. How would I set a default facing?
-
Hey there, So I'm looking to do something with one of my ores, to make them glow. Not like Redstone however, more or less like Thaumcraft's element ores. How would I get started with doing this? Thanks,
-
Hey there, So I'm having an issue with some items and blocks that need to be rotated so you can see their faces, however I have not found a method that has worked, some code I have tried: "display": { "thirdperson": { "rotation": [ -90, 0, 0 ], "translation": [ 0, 1, -3 ], "scale": [ 0.55, 0.55, 0.55 ] }, "firstperson": { "rotation": [ 0, -135, 25 ], "translation": [ 0, 4, 2 ], "scale": [ 1.7, 1.7, 1.7 ] } Thanks,
-
Yeah that seemed to work, however, would you know how I'd get rid of duplicates? So the size isnt something crazy. just 1,2,3,4?
-
So something like this? @Override public void updateEntity() { super.updateEntity(); System.out.print(standsAround.size()); if (!worldObj.isRemote && sendUpdateChecksWithInterval()) { for (EnumFacing facing : EnumFacing.HORIZONTALS) { for (int i = 0; i < maxSearchRange; i++) { BlockPos pos = getPos().offset(facing, i); TileEntity te = worldObj.getTileEntity(pos); if (te instanceof TileEntityStand) { this.standsAround.add(te); } if (i >= maxSearchRange) { return; } } } validateTEs(); } } public void validateTEs() { for(int i = 0; i < standsAround.size(); i++) { TileEntity te = standsAround.get(i); if(te.getPos() == null) { standsAround.remove(te); }else { return; } if(i >= standsAround.size()) { return; } } } Edit: Oh and my list being: public final List<TileEntity> standsAround = new ArrayList<TileEntity>();
-
Pretty sure you should be extending IModPlugin
-
Ah sorry: Still won't remove from the list.
-
Okay, I have a 'updateWithInverval' built in with my BaseTile so will switch to that.. Okay, I have switched to a WeakHashMap , and it seems to work okay, however, still wont remove from the list. @Override public void updateEntity() { super.updateEntity(); System.out.print(standsAround.size()); if(!worldObj.isRemote && sendUpdateWithInterval()) { for (EnumFacing facing : EnumFacing.HORIZONTALS) { for(int i = 0; i < maxSearchRange; i++) { BlockPos pos = getPos().offset(facing, i); TileEntity te = worldObj.getTileEntity(pos); if (te instanceof TileEntityStand) { if(!te.isInvalid()) { standsAround.put(te, pos); } else { standsAround.remove(te); } } if(i >= maxSearchRange) { return; } } } } }
-
Hey there, So I'm looking for a way to detect when a certain TE is broken. I need it so that when the TE is broken, remove it from a list. Here is what I current have: @Override public void updateEntity() { super.updateEntity(); System.out.print(standsAround.size()); if(!worldObj.isRemote) { for (EnumFacing facing : EnumFacing.HORIZONTALS) { for(int i = 0; i < maxSearchRange; i++) { BlockPos pos = getPos().offset(facing, i); TileEntity te = worldObj.getTileEntity(pos); if (te instanceof TileEntityStand) { standsAround.add(te); } if(i >= maxSearchRange) { return; } } } } } Thanks,
-
I see what you mean, Will take into consideration next time. With that I did some digging and found out my 'CustomEnergyStorage' was extending CoFH IEnergyStorage instead of forge's. Thanks for your help.