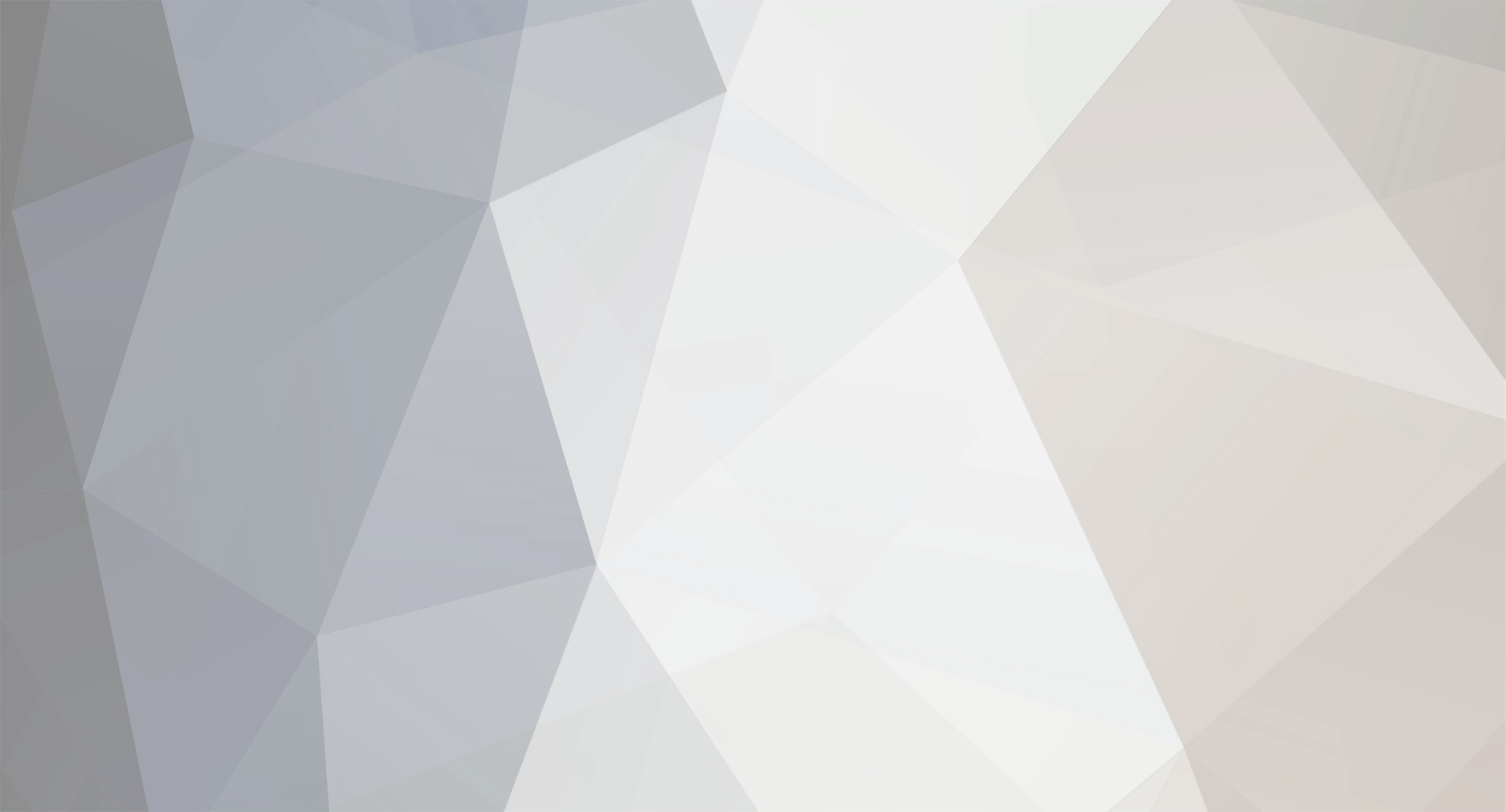
gcewing
Forge Modder-
Posts
145 -
Joined
-
Last visited
Everything posted by gcewing
-
Junction in cable network problem [UNSOLVED] [1.7.10]
gcewing replied to TheEpicTekkit's topic in Modder Support
You seem to be using a mixture of two different algorithms, a recursive one and a non-recursive one. If you're keeping a queue of backtracking locations, you should *not* also be making recursive calls. It's usually better to do this kind of thing non-recursively, because then there's no danger of running out of stack space for complicated networks. The skeleton of a non-recursive graph traversal algorithm goes something like this: set visited_notes to an an empty set set nodes_to_visit to an empty queue add initial_node to nodes_to_visit while nodes_to_visit is not empty: pop current_node from nodes_to_visit add current_node to visited_nodes process current_node for each neighbour_node in nodes connected to current_node: if neighour_node not in visited_nodes: add neighour_node to nodes_to_visit Another advantage of this algorithm is that no special cases are needed for junctions vs. non-junctions -- every node is treated the same, however many connections there are from it. My advices is this: 1) Throw away your whole existing locateNodes method. It's FAR too big and complicated as it is, and you'll only drive yourself insane trying to debug it. 2) Replace it with something that just implements the bare bones of the traversal algorithm. Essentially it will be a line-by-line translation of the above pseudocode into Java. Split off all the sub-tasks such as "process node" and "find neighbours" into separate methods, where they won't obscure the main algorithm. If you do that, you should end up with something manageable that you can debug. You might also like to do some research into algorithms for traversing graphs -- it's a well-studied subject. -
That looks like it should work. Have you checked that the pathname is correct? Also, I'm not sure if I've ever tried switching textures inside an item renderer, although I can't think of any reason it shouldn't work. Can you show us your whole renderItem method? There might be something else in there that's messing things up. OpenGL provides lots of ways to do that. :-)
-
I'm developing an add-on for Project Red, and weird things are happening with the way Java and Scala interact. Everything compiles fine, but some things misbehave at run time. 1) I have a subclass of JItemMultiPart written in Java that overrides some Item methods, such as registerIcons. But my overrides are never called -- it calls the base methods defined in class Item instead. If I replace JItemMultiPart with a re-implementation of it in pure Java, my overrides are called as expected. 2) Calling methods of a TileMultipart that are defined in TileEntity, e.g. getBlockType and markDirty, causes a Method Not Found exception at run time. These calls work if I explicitly cast the TileMultipart to TileEntity, however. It seems like the runtime is not recognising that these Scala-implemented classes inherit from java base classes. There is no Scala in my own code, only Java. I've tried compiling with two different versions of javac, javac 1.6.0_51 and javac 1.7.0-b222, with the same result. I'm on MacOSX 10.6.8. None of this was happening when I first started working on the mod a few months ago, but back then I was using my own custom builds of ForgeMultipart and Project Red for 1.7. The only thing I can think of that's changed is that I'm now compiling against the official 1.7.10 releases. Anyone have any idea what might be happening? I can work around these problems for now, but they're disconcerting and I'd like to get to the bottom of them.
-
[SOLVED] Tessellator - already tessellating error
gcewing replied to Enkey's topic in Modder Support
Also, if you want to change the texture, you need to do it while the tesselator is not tesselating, otherwise you'll mess up rendering of other blocks. You also need to restore the previous texture when you're finished, again while the tesselator is not tesselating. The correct procedure is: tes.draw(); /* bind your texture here */ tes.startDrawingQuads(); /* draw your block here */ test.draw(); /* restore the default texture */ tes.startDrawingQuads(); But note that using a custom texture for rendering blocks is no longer the recommended way of doing things. The modern way is to provide each of your block images as a separate image file, and let MC stitch them into the main texture. This eliminates the need to switch textures while rendering blocks, simplifying your rendering code and improving graphics performance. To see how it's done, take a look at the registerIcons() method of some of the vanilla blocks, and how RenderBlocks makes use of the Icon class. -
This may sound silly, but have you made sure that the same thing doesn't happen with vanilla arrows? I had a problem with very similar symptoms some time back while working on SG Craft. It turned out to be a bug in vanilla Minecraft, that was fixed in 1.5.2 according to the changelog. What version of Minecraft are you modding?
-
You might also want to make sure the blending function is set appropriately, e.g. GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); I just fixed a bug in SG Craft that was due to failing to do this.
-
[1.6.2] Problems with custom furnace [NOT SOLVED]
gcewing replied to AppliedOnce's topic in Modder Support
I can think of two possible reasons for this: 1) Items are only getting put into your tile entity on the client side, not the server side. 2) Your tile entity's onInventoryChanged() method is not getting called when it should be. -
I think the side is always reported as client side in single player. It's probably more reliable to check world.isRemote. Also, I don't think the @SideOnly annotations do anything in mod code. All methods are compiled in, so that the same jar can be used on client and server.
-
Be careful -- if you're testing in single player mode, all the client side methods are accessible, even from server-only parts of the code, which means it's possible to write code that works fine in single player, but crashes when run on a real server. Concerning the packet handler, if you use packethandler = ... in @NetworkMod, it uses the same packet handler class for both client and server, so it's possible that your packet *is* being received on the server side but you're not noticing. Also, the earlier reply concerning isRemote got it backwards -- isRemote == true on the client side and false on the server side. You might be getting confused by that.
-
Something like return plant instanceof BlockSapling;
-
Greg's French Vanilla This mod adds enhanced abilities to some vanilla game elements. It has an unusual feature: It operates entirely on the server side. Despite being a Forge mod, it allows a standard, unmodified client to connect in multiplayer. It is not necessary for Forge to be installed on the client, unless other Forge mods are being used. Features currently implemented Signs Ever get frustrated that the only way to change a sign is to break it and re-type the whole thing? Now you can edit the text of a previously placed sign by right-clicking on it with a piece of charcoal. And that's not all: if you break a sign with your hand, it keeps its text, and you can place it down somewhere else without losing the text. Screenshots A picked-up sign shows its text when you mouse over it in your inventory. Home Page For full details and download instructions, see: http://www.cosc.canterbury.ac.nz/greg.ewing/minecraft/mods/FrenchVanilla/
-
Rendering Tileentities in inventory/as block items
gcewing replied to MrCredits's topic in Modder Support
The answer to this depends on how much information your renderer needs to render the block as an item. Is the block ID and metadata enough, or do you need values from your TileEntity? Note that "rendering a tile entity as an item" is not the right way to think about this. Taken literally, that's impossible, because tile entities only exist in the world -- when in the form of an item (in hand, in inventory, or as a dropped item), there is no TileEntity instance. If your renderer only needs the block ID and metadata, you can do this using an ISimpleBlockRenderingHandler, registered using RenderingRegistry.registerBlockHandler(). Your block's getRenderType() method needs to return the ID that you used to register the handler. ISimpleBlockRenderingHandler has two entry points, one for rendering a block in the world, and the other for rendering it as an item. If you want it to have the same appearance in both cases, you'll probably want to arrange both of these to delegate to a mostly-common piece of code that does the rendering. On the other hand, if you need information that's kept in the tile entity, things become considerably more complicated. You'll need an IItemRenderer, and you'll have to find a way to attach the relevant data to the ItemStack, using the damage value and/or an attached NBTTagCompound, which may require a custom ItemBlock class. Let me know if you need more guidance about that. That's strange -- it works fine for me. Are you absolutely sure that's what's happening? -
As others have said, the best way to answer this kind of question is by experiment. Try changing each of the rotation angles a little bit, one axis at a time, and observe what happens. Once you've found the right axis, adjust the angle until you get the result you want. Keep in mind that when you perform multiple rotations, the effect of later ones will depend on the earlier ones. You may find it less confusing to start with all angles zero.
-
The root cause is this exception: Googling for "java ConcurrentModificationException" turns up this explanation: http://docs.oracle.com/javase/6/docs/api/java/util/ConcurrentModificationException.html In this case it's because you have this line inside the loop that's iterating over the drops: event.drops.add(new EntityItem(event.entityLiving.worldObj, event.entityLiving.posX, event.entityLiving.posY, event.entityLiving.posZ, new ItemStack(MetallurgyRealismModBase.FoodEggBoiled))); One way to fix this would be to add the replacement items to a temporary list while you're iterating, and then add that to the drops afterwards.
-
I have a class implementing IInventory that relies on a superclass to provide implementations for its methods. There's a message here https://github.com/BuildCraft/BuildCraft/pull/726 mentioning that this won't work unless you use reobfuscate_srg.sh instead of reobfuscate.sh. However, I am using reobfuscate_srg.sh, and it still doesn't work. I get: 2013-07-28 19:24:23 [iNFO] [sTDOUT] java.lang.AbstractMethodError 2013-07-28 19:24:23 [iNFO] [sTDOUT] at net.minecraft.inventory.Slot.func_75211_c(Slot.java:91) where that line is doing return this.inventory.getStackInSlot(this.slotIndex); It works unobfuscated, but not obfuscated. I'm using Forge 9.10.0.789. Anyone have any ideas?
-
News Version 1.2.0 Updated for Minecraft 1.7.2. Version 1.1.0 Updated for Minecraft 1.6.2. Greg's Blogging Keep a diary of your adventures while playing This mod came about because I wanted a way to make notes about interesting things I encountered during my games. I tried keeping a diary using a word processor, but having to switch to another application, find screenshots and paste them in, etc., was a nuisance and interrupted the flow of the game. I wanted a better way. That's what Greg's Blogging provides. Whenever the mood strikes, you can hit the B key, type in a few lines of text, attach a screenshot if you like, and have it all uploaded automatically to a Wordpress blogging site. Or if you prefer, emailed to your friends. User interface for making entries Resulting Wordpress blog entry Home Page For information and downloads, see Greg's Blogging Home Page
-
To anyone who isn't hearing any sounds: A Feed the Beast user reported that the sounds weren't working for him, and tracked it down to a conflict with the Integrated Soundpacks mod. Removing "integratedsoundpacks.litemod" from the mods folder reportedly fixed it.
-
Thank you! That's the piece of information I was missing.
-
Maybe I've got the wrong idea about what runtime deobfuscation means. I've been assuming it means I don't need to reobfuscate my mod -- I should be able to drop the unobfuscated classes into a standard Minecraft instance and it will run. That doesn't work for me; I get errors like 2013-04-13 12:29:34 [iNFO] [sTDERR] java.lang.RuntimeException: java.lang.reflect.InvocationTargetException 2013-04-13 12:29:34 [iNFO] [sTDERR] at gcewing.sg.BaseMod.newBlock(BaseMod.java:140) ... 2013-04-13 12:29:34 [iNFO] [sTDERR] Caused by: java.lang.NoSuchFieldError: blocksList 2013-04-13 12:29:34 [iNFO] [sTDERR] at gcewing.sg.SGRingBlock.<init>(SGRingBlock.java:41) So, have I got the wrong end of the stick? Is this meant to work or not?
-
Is anyone successfully using runtime deobfuscation with Forge 7.7.1.611? The deobfuscation_data file that this version of Forge uses appears to be missing some rather crucial field names. In particular, all the static fields defined in the Block, Material and MapColor classes are missing. Without access to these fields, it's almost impossible to define any custom block classes, so I have trouble seeing how this wouldn't affect just about every mod in existence.
-
I have a class called BaseTileEntity that defines all the methods required to implement IInventory, but doesn't claim to implement it. Then I have subclasses that do claim to implement it, and rely on inheriting the necessary methods from the base class. When I run the mod unobfuscated under MCP, this works fine. However, when I reobfuscate and run it in a real Minecraft installation, I get an AbstractMethodError from an attempt to call getSizeInventory(). It seems that the reobfuscator is getting confused somehow, and doesn't realise that the getSizeInventory() implementation in BaseTileEntity is able to satisfy IInventory. This arrangement used to work in 1.4.6-1.4.7. Has there been any change in the way reobfuscation is done that might affect this? (Searching for "AbstractMethodError" in this forum turns up a lot of references to having outdated mods in the coremodes folder. This is NOT my problem -- it's a completely clean MC installation, and my mod is the only one present.)
-
There's another thread about this here that you might find helpful: http://www.minecraftforge.net/forum/index.php/topic,5722.0.html
-
Here's some code I've successfully used to add trades to villagers. // Called during init void registerTradeHandlers() { VillagerRegistry reg = VillagerRegistry.instance(); SGTradeHandler handler = new SGTradeHandler(); reg.registerVillageTradeHandler(tokraVillagerID, handler); } // Trade handler class public class SGTradeHandler implements IVillageTradeHandler { public void manipulateTradesForVillager(EntityVillager villager, MerchantRecipeList recipes, Random random) { // Trade 1 emerald and 3 naquadah ingots for 1 stargate ring block recipes.add(new MerchantRecipe( new ItemStack(Item.emerald, 4), new ItemStack(SGCraft.naquadahIngot, 3), new ItemStack(SGCraft.sgRingBlock, 1, 0))); } }
-
This looks like my BaseContainer class from SGCraft. It's meant to be used by subclassing it and overriding the sendStateTo() and updateProgressBar() methods. For example, here's how the stargate container class communicates the stargate's fuel level from server to client: public class SGBaseContainer extends BaseContainer { ... SGBaseTE te; // the stargate's tile entity ... @Override void sendStateTo(ICrafting crafter) { crafter.sendProgressBarUpdate(this, 0, te.fuelBuffer); } @Override public void updateProgressBar(int i, int value) { switch (1) { case 0: te.fuelBuffer = value; break; } } }
-
The last four parameters to onItemUse are: int side, float px, float py, float pz side = which side of the block was clicked on px, py, pz = the point on the block that was clicked, in local coordinates (0.0 - 1.0) if I remember correctly.