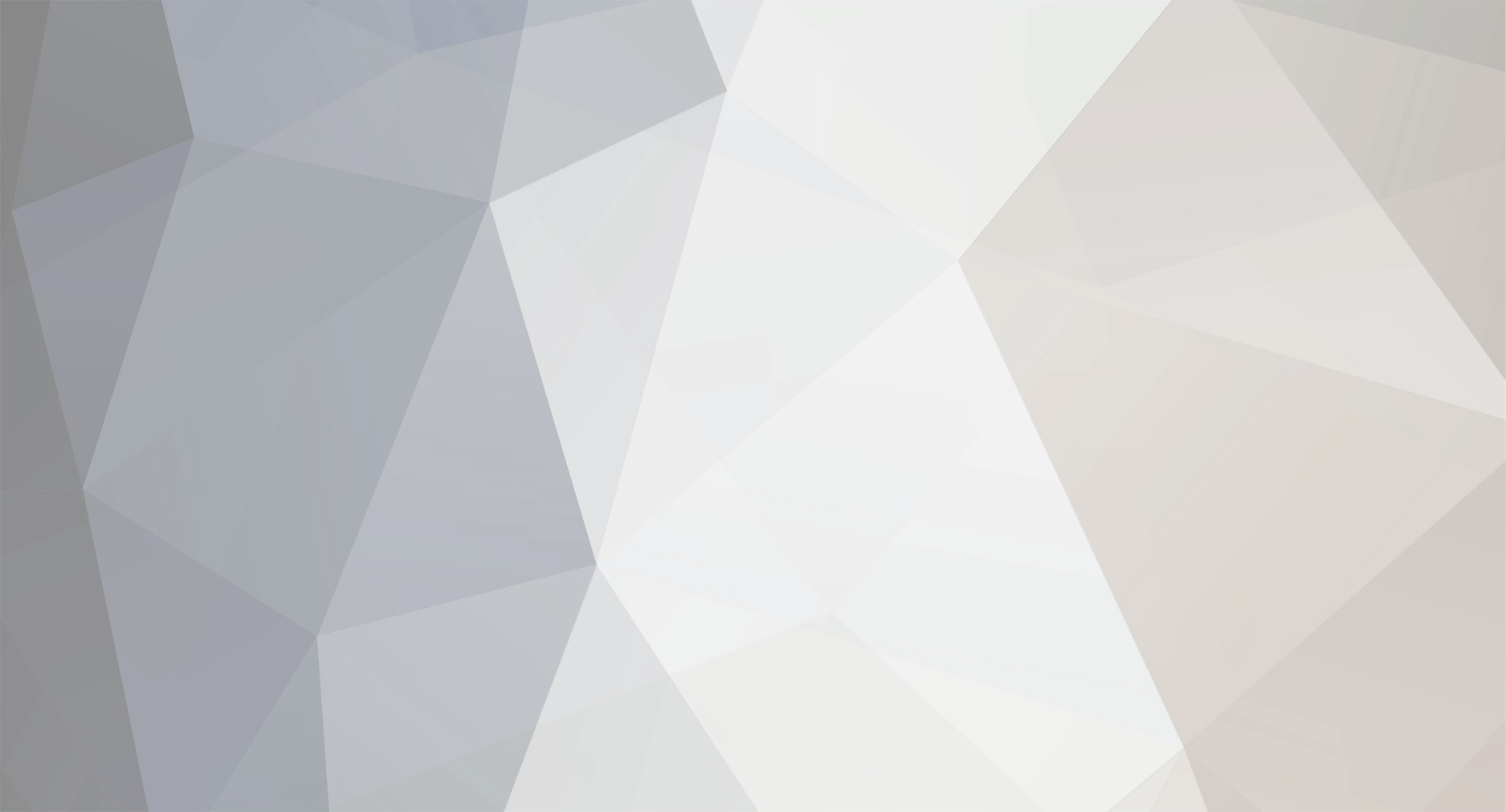
gcewing
Forge Modder-
Posts
145 -
Joined
-
Last visited
Everything posted by gcewing
-
This makes no sense. You're doing a reference comparison between an existing tile entity and a newly created instance of TileEntitySuperCalc. This will always be false. I think what you mean is: if (world.getBlockTileEntity(x+1, y, z+1) instanceof TileEntitySuperCalc) {... Also, you can make your redirecting code a lot more compact using some loops, e.g. for (int dx = -1; dx <= 1; dx++) for (int dz = -1; dz <= 1; dz++) if (world.getBlockTileEntity(x+dx, y, z+dz) instance of TileEntitySuperCalc) { player.openGui(CodeLyoko.instance, 0, world, x+dx, y, z+dz); return true; } (General programming tip: If you ever find yourself writing very similar code over and over again, there's almost always a better way of doing it!)
-
There is a hook for this, but it's not all that straightforward to use. A World object on the server side has a field called perWorldStorage that holds a MapStorage instance. This is a keyed collection of WorldSavedData instances. You can add your own object to this collection and it will be saved along with the world. Your object needs to extend WorldSavedData and provide readFromNBT and writeToNBT methods. An example would look something like this: public class MyWorldData extends WorldSavedData { final static String key = "my.unique.string"; // Fields containing your data here public static MyWorldData forWorld(World world) { // Retrieves the MyWorldData instance for the given world, creating it if necessary MapStorage storage = world.perWorldStorage; MyWorldData result = (MyWorldData)storage.loadData(MyWorldData.class, key); if (result == null) { result = new MyWorldData(); storage.setData(key, result); } return result; } @Override public void readFromNBT(NBTTagCompound nbt) { // Get your data from the nbt here } @Override public void writeToNBT(NBTTagCompound nbt) { // Put your data in the nbt here } }
-
Your TileEntity can have a field referring to another object, and you can put different subclasses there depending on metadata or other information in the TileEntity. The sub-object doesn't have to extend TileEntity, btw, it can be anything you want.
-
I don't think there's anything wrong there. Nowadays it's not necessary to decompile or recompile the server unless you want to work on the server code in particular. So you can ignore the message about the server.
-
That depends on what sort of animation you want to do. From what I've seen, a TextureFX is okay if you can get the effect you want just by changing the texture, but not if you need to change the geometry. Chests are an example of this -- they use a TileEntitySpecialRenderer to animate opening and closing the lid.
-
The same technique should work, but you will need to flush the tessellator before changing the blending and lighting modes. Something like this: tessellator.draw(); // set up blending and lighting tessellator.startDrawingQuads(); // draw the glowing bits tessellator.draw(); // restore previous blending and lighting tessellator.startDrawingQuads();
-
Is there a way to detect when a particular structure is generated and add something to it? Whenever a pyramid is generated, I want to generate a structure of my own underneath it. I've been studying the structure generation code, but it's huge, rambling and rather hard to follow. Has anyone written a description anywhere of how it all fits together?
-
[Unsolved]Custom Block Rendering Problem.
gcewing replied to SoBiohazardous's topic in Modder Support
The first thing I would do is make sure it's actually calling your rendering method. If not, there is some problem with the way you're registering your renderer or linking it up via the render ID. If your rendering code is getting called, you'll have to trace through it in detail to find out where it's going wrong. -
[Solved]How to get Adjacent Blocks to Render with Custom Model
gcewing replied to Rivendark2013's topic in Modder Support
My guess is that you had started by copying the code for a block whose isOpaqueCube() method returned true. That should be done only for blocks that occupy a whole cube -- in that case, the renderer assumes that any adjacent block face touching your block can't be seen. Any block that doesn't fill a whole cube needs to have isOpaqueCube() returning false. -
Your isIDSame() method makes no sense. You're creating a new, empty NBTTagCompound and then trying to get an integer out of it. The result of that is always going to be the default value of 0. You're going to have to explain more clearly what you want to achieve. Are you trying to recognise an item belonging to a different mod? Or one of your own items whose ID can be changed in a config file? Something else?
-
If you show us the code that didn't work, we may be able to help you more.
-
Animation is best done using a TileEntitySpecialRenderer, because those get called for every frame. (Using a normal block renderer for animation requires re-rendering the whole chunk on each change, which puts a lot of load on the client.) As for glowing, do you want your block to actually give off light and light up the surroundings, or just make it look luminescent?
-
Only if you use a TileEntitySpecialRenderer to do the rendering. You don't have to do that -- you can use an ordinary block renderer that uses information from the tile entity to decide what to draw.
-
Trying to work out a good way to stop my "portal bouncing" issue.
gcewing replied to Arphahat's topic in Modder Support
In my stargate mod, rather than triggering teleportation when the player stands in a particular place, I detect when he passes through a plane at the centre of the portal. If the player's posX, posY, posZ is on one side of the plane, and his prevPosX, prevPosY, prevPosZ is on the other side, then teleportation occurs. I do it this way because I want the player to be able to just walk straight through the portal without stopping. It seems to work very well, and I've never had any problems with bouncing. -
The tile entity gets created by the onBlockAdded() method of BlockContainer.
-
To get notified on the server side when a world is loaded, you can do this in the main class of your mod: @ForgeSubscribe void onWorldLoad(WorldEvent.Load e) { ... }
-
Infinite Terrain/Sprite Indexes... Finite Block IDs. Workaround?
gcewing replied to Seigyoku's topic in Modder Support
You need to look into tile entities. They let you attach an unlimited amount of information to a block. -
An ItemStack can have an NBTTagCompound attached to it, where you can store the information that needs to go into your tile entity when the block is placed. Getting that to actually happen is a little bit tricky. It looks like one way would be to register your own subclass of ItemBlock to go with your block, and override the placeBlockAt method. That gives you access to the ItemStack being placed, the world and the coordinates in the world, so after calling super.placeBlockAt, you can retrieve the tile entity and set it up from the NBTagCompound of the stack. You'll also need a custom item renderer for your ItemBlock subclass, so that you can render the block in the inventory correctly according to the NBTTagCompound. A normal block renderer won't work, because it doesn't get passed the ItemStack when rendering an inventory block. You might like to look at the source of Greg's Blocks, where I do something like this (although I used a slightly different and more kludgy way of doing the placement): http://www.cosc.canterbury.ac.nz/greg.ewing/minecraft/mods/GregsBlocks/ The relevant classes to study would be BlockShape, TileEntityShape, ItemBlockShape and ItemBlockShapeRenderer. You're welcome to ask me, either here or by email, if you have any questions about it. greg.ewing@canterbury.ac.nz
-
If it's for a block, then use a custom block renderer or a TileEntitySpecialRenderer. There's nothing actually restricting what you draw with those to the space of one block. Things to consider when choosing between these: * If the appearance changes infrequently, a block renderer is more efficient, because it only gets called when something in the chunk changes state. However, any change requires re-rendering the whole chunk. * A TileEntitySpecialRenderer is called for every frame, so it copes with animation better, but puts more strain on the client's frame rate.
-
Wool -> Wool Stairs help... [METADATA] (I think)
gcewing replied to DARKHAWX's topic in Modder Support
Your guess is correct. You only get 4 bits of metadata per block. Wool uses these 4 bits to encode the 16 different colours, and stairs use them to encode the orientation. You can't use them for both at the same time. You have a couple of options: * Use a different block ID for each orientation, leaving the metadata free for the colour. This is probably the simplest solution. * Attach a TileEntity subclass to your block, and store the colour and/or the orientation there. This is more complicated to set up, but it lets you store an unlimited amount of extra information. -
Greg's SG Craft This mod provides some technology based loosely on the television show Stargate SG-1. Videos Thanks to MinecraftScorpion for this spotlight video: My original preview video: Home Page Downloads and instructions can be found here: http://www.cosc.canterbury.ac.nz/greg.ewing/minecraft/mods/SGCraft/ Latest News Full change list: http://www.cosc.canterbury.ac.nz/greg.ewing/minecraft/mods/SGCraft#mozTocId153908
-
Please show us the exact error message you're getting.
-
I can tell you how I'm doing this in my upcoming stargates mod. The basic idea is that the onBlockAdded method of the component block searches the area around it looking for a particular pattern of blocks. When that pattern is found, the component blocks are flagged as having been merged into a structure. Also, one of the component blocks is picked to be the "master" block having a TileEntity holding the state of the structure as a whole. When any of the component blocks is right-clicked, the click is forwarded to the master block, which opens the GUI. Unmerging is handled by the breakBlock method of the component blocks, which clears the merge flag on all the other merged blocks of the structure. As for rendering the merged structure, I'm doing that using a TileEntitySpecialRenderer on the master block, and the normal block renderers of the component blocks don't render anything when they're merged. But you could also have the component blocks render themselves in two different ways for the merged and unmerged states, or replace the component blocks with different block types when they merge. From what was said about Railcraft above, it sounds like it's using a somewhat inefficient method to detect merging. It shouldn't be necessary to continually check for merging, only when a potential component block is placed or removed, or possibly when a neighbour of such a block changes state.
-
I haven't looked into this, so this is just a guess, but it's likely that registerServerCommand needs to know the name of the command, in which case calling it before the config file is loaded won't work. If registerServerCommand doesn't absolutely have to be called during serverStarting, you could try calling it in your preinit function after config.load().
-
Short of writing a framework to do auto-layout of GUI widgets (it would be a considerable service to the modding community if someone did that!), no, not really. My current technique is to draw a mockup of the GUI using Intaglio, put dimension lines all over it, and then transcribe the dimensions into constants in the code. You can also help yourself by writing code to do some of the layout calculations. For example, if you have a row of buttons all the same size, write a loop to lay them out.