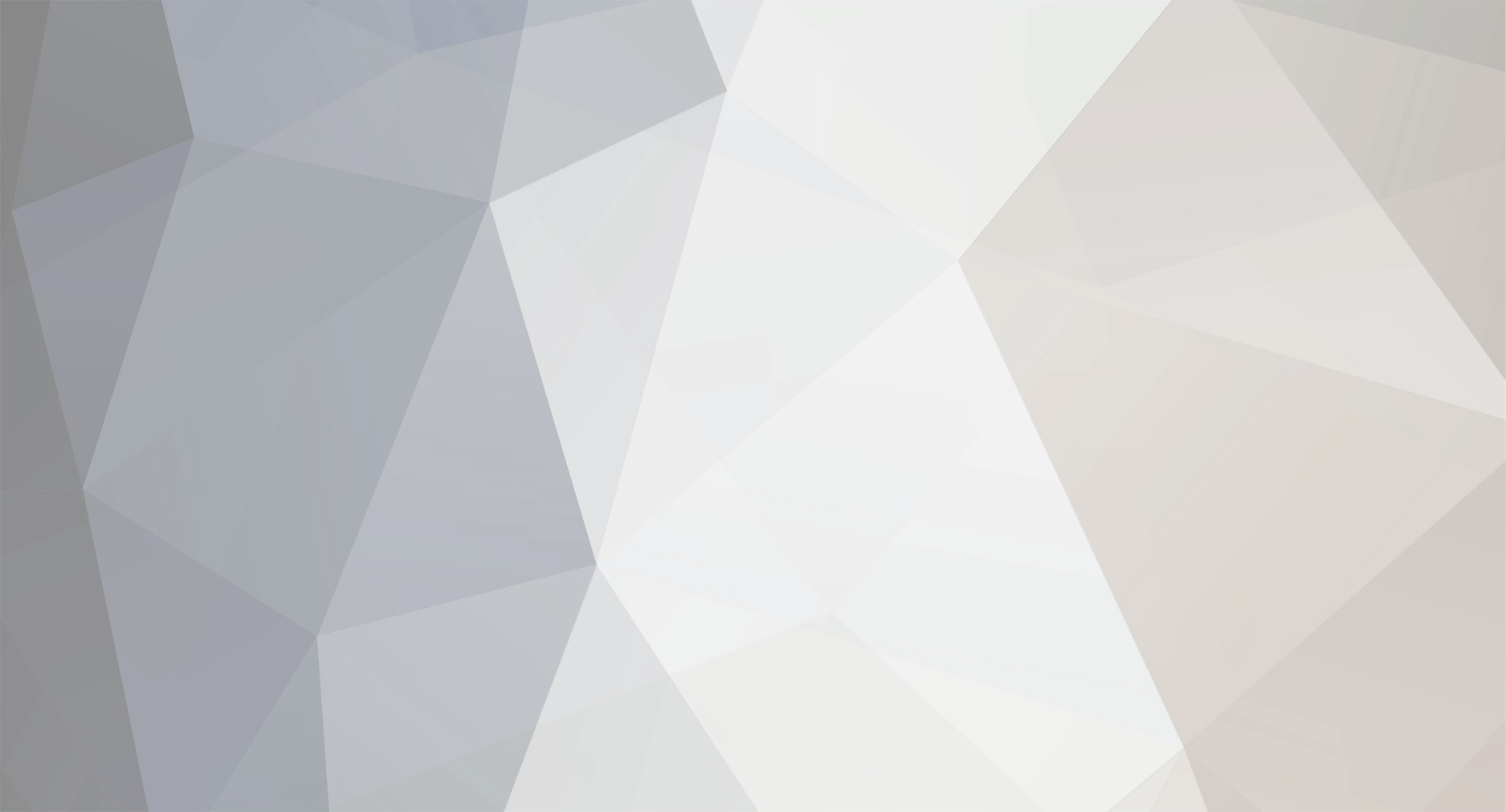
Differentiation
Members-
Posts
606 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Differentiation
-
Hello all! I have a question: Does anyone know the list of all the FML Forge Events? Please leave them below. Thanks!
-
Cool you're making a Hypixel Mod
-
Thanks!
-
Hey! So I wanted to make this command called "boom" and its purpose is to launch all players to the air. However, it doesn't seem to work My code: package me.mycustommod.command; import net.minecraft.command.CommandBase; import net.minecraft.command.ICommandSender; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.server.MinecraftServer; import net.minecraft.util.text.TextComponentString; public class CommandBoom extends CommandBase { @Override public String getCommandName() { return "boom"; } @Override public String getCommandUsage(ICommandSender sender) { return "/boom"; } @Override public void execute(MinecraftServer server, ICommandSender sender, String[] args) { if (sender instanceof EntityPlayer) { ((EntityPlayer) sender).addChatMessage(new TextComponentString(TextFormatting.GREEN + "Command successfully executed")); ((EntityPlayer) sender).addVelocity(0, 10, 0); } } @Override public int getRequiredPermissionLevel() { return 0; } @Override public boolean checkPermission(MinecraftServer server, ICommandSender sender) { return sender instanceof EntityPlayer; } } *Yes, I did register the command. So whenever I run the command, it only sends the message "Command successfully executed" to the player, but doesn't launch the players to the air. Please help. Thanks!
-
There is something wrong with the setup of the server then.
-
Well, are you using any mods? If so, which?
-
FML Log?
-
advanced Anyone tried modding on Visual Studio Code?
Differentiation replied to NovaViper's topic in Modder Support
Eclipse Java®. Stay safe, stay friendly™. -
[1.10.2] Extending reach distance
Differentiation replied to Differentiation's topic in Modder Support
Thanks guys! I just tweaked @jabelar 's tutorial to match my 1.10.2 version of code, and managed to make it happen! -
Hey, so I tried a way to extend a player's reach distance for block destruction, but the problem is that whenever I break a block that is farther than 5 blocks in radius from the player, the block is glitched and I get stuck in it. I have tried sending packets to the server but that didn't seem to work out. Please help. My classes: 1. ItemChlorophyteSword.java package me.mycustommod.items; import me.mycustommod.MyCustomMod; import me.mycustommod.Reference; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.item.ItemStack; import net.minecraft.item.ItemSword; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; public class ItemChlorophyteSword extends ItemSword { public ItemChlorophyteSword(ToolMaterial material, String unlocalizedName) { super(material); this.setUnlocalizedName(unlocalizedName); this.setRegistryName(new ResourceLocation(Reference.MODID, unlocalizedName)); } @Override public boolean hitEntity(ItemStack stack, EntityLivingBase target, EntityLivingBase attacker) { if (attacker.isSneaking()) { if (target.hurtTime == target.maxHurtTime && target.deathTime <= 0) { target.addVelocity(0, 0.5, 0); } } return super.hitEntity(stack, target, attacker); } @Override public void onUpdate(ItemStack stack, World world, Entity entity, int itemSlot, boolean isSelected) { if (entity instanceof EntityLivingBase) { if (isSelected) { MyCustomMod.proxy.setExtraReach(((EntityLivingBase) entity), 10F); } else { MyCustomMod.proxy.setExtraReach(((EntityLivingBase) entity), 0F); } } super.onUpdate(stack, world, entity, itemSlot, isSelected); } } 2. DinocraftPlayerController.java package me.mycustommod.handlers; import net.minecraft.client.Minecraft; import net.minecraft.client.multiplayer.PlayerControllerMP; import net.minecraft.client.network.NetHandlerPlayClient; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; @SideOnly(Side.CLIENT) public class DinocraftPlayerController extends PlayerControllerMP implements IExtendedPlayerController { private float distance = 0F; public DinocraftPlayerController(Minecraft mc, NetHandlerPlayClient netHandler) { super(mc, netHandler); } @Override public float getBlockReachDistance() { return super.getBlockReachDistance() + distance; } @Override public void setReachDistanceExtension(float f) { distance = f; } @Override public float getReachDistanceExtension() { return distance; } } 3. IExtendedPlayerController.java package me.mycustommod.handlers; public interface IExtendedPlayerController { /** Sets the extra reach the player should have */ public void setReachDistanceExtension(float f); /** Gets the current reach extension */ public float getReachDistanceExtension(); } 4. ClientProxy package me.mycustommod.proxy; import me.mycustommod.entity.EntityPoisonBall; import me.mycustommod.entity.EntityRayBullet; import me.mycustommod.entity.EntityVineBall; import me.mycustommod.handlers.DinocraftPlayerController; import me.mycustommod.handlers.IExtendedPlayerController; import me.mycustommod.init.DinocraftArmour; import me.mycustommod.init.DinocraftBlocks; import me.mycustommod.init.DinocraftItems; import me.mycustommod.init.DinocraftTools; import net.minecraft.client.Minecraft; import net.minecraft.client.entity.EntityPlayerSP; import net.minecraft.client.network.NetHandlerPlayClient; import net.minecraft.client.renderer.entity.RenderSnowball; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; import net.minecraftforge.fml.client.FMLClientHandler; import net.minecraftforge.fml.client.registry.RenderingRegistry; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; public class ClientProxy extends CommonProxy { @Override public void preInit(FMLPreInitializationEvent event) { initRenderers(); } private void initRenderers() { RenderingRegistry.registerEntityRenderingHandler(EntityVineBall.class, renderManager -> new RenderSnowball(renderManager, DinocraftItems.VINE_BALL, Minecraft.getMinecraft().getRenderItem())); RenderingRegistry.registerEntityRenderingHandler(EntityPoisonBall.class, renderManager -> new RenderSnowball(renderManager, DinocraftItems.POISON_BALL, Minecraft.getMinecraft().getRenderItem())); RenderingRegistry.registerEntityRenderingHandler(EntityRayBullet.class, renderManager -> new RenderSnowball(renderManager, DinocraftItems.RAY_BULLET, Minecraft.getMinecraft().getRenderItem())); } @Override public void registerRenders() { DinocraftItems.registerRenders(); DinocraftBlocks.registerRenders(); DinocraftTools.registerRenders(); DinocraftArmour.registerRenders(); } @Override public EntityPlayer getPlayer() { return FMLClientHandler.instance().getClient().thePlayer; } @Override public World getClientWorld() { return FMLClientHandler.instance().getClient().theWorld; } @Override public void setExtraReach(EntityLivingBase entity, float reach) { Minecraft mc = Minecraft.getMinecraft(); EntityPlayerSP player = mc.thePlayer; if(entity == player) { if(!(mc.playerController instanceof IExtendedPlayerController)) { NetHandlerPlayClient net = player.connection; DinocraftPlayerController controller = new DinocraftPlayerController(mc, net); boolean isFlying = player.capabilities.isFlying; boolean allowFlying = player.capabilities.allowFlying; controller.setGameType(mc.playerController.getCurrentGameType()); player.capabilities.isFlying = isFlying; player.capabilities.allowFlying = allowFlying; mc.playerController = controller; } ((IExtendedPlayerController) mc.playerController).setReachDistanceExtension(Math.max(0, reach)); } } } 5. CommonProxy package me.mycustommod.proxy; import me.mycustommod.worldgen.OreGen; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.world.World; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.registry.GameRegistry; public class CommonProxy implements IProxy { @Override public void preInit(FMLPreInitializationEvent event) {} @Override public void init(FMLInitializationEvent event) {} @Override public void postInit(FMLPostInitializationEvent event) {} public void init() { GameRegistry.registerWorldGenerator(new OreGen(), 0); } public void registerRenders() { } public EntityPlayer getPlayer() { return null; } public World getClientWorld() { return null; } @Override public void setExtraReach(EntityLivingBase entity, float reach) { if (entity instanceof EntityPlayerMP) ((EntityPlayerMP) entity).interactionManager.setBlockReachDistance(Math.max(11, reach)); } } 6. IProxy package me.mycustommod.proxy; import net.minecraft.entity.EntityLivingBase; import net.minecraft.world.World; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; public interface IProxy { void preInit(FMLPreInitializationEvent event); void init(FMLInitializationEvent event); void postInit(FMLPostInitializationEvent event); void setExtraReach(EntityLivingBase entity, float reach); } Thank you!
-
1.9 Forge Version crashes when loading world
Differentiation replied to ScarletTheReapur's topic in Support & Bug Reports
Please post the FML Log. -
./logs/fml-client-latest.log
-
You need to have Mods that are compatible with the version you're loading and compatible with each other. And next time, please include the FML Log. Hope this helped!
-
You need to go to the resources of you mod by clicking on your mod folder --> src --> main --> [yourmodname] --> textures --> and then fill in for all the item/block/entity textures that are missing. Hope this helped!
-
The Lucky Block Mod keeps crashing!
Differentiation replied to Bigc1109's topic in Support & Bug Reports
Use a newer version of Minecraft (1.10, 1.11, 1.12, or 1.13 BETA.) -
Hey, so I wanted to play a different sound whenever a specific item is picked up, but all that plays is the original Minecraft sound. Please help. My code: @SubscribeEvent(priority = EventPriority.HIGHEST) public void onItemPickup(ItemPickupEvent e) { if (e.pickedUp.getEntityItem().getItem().equals(DinocraftItems.CHLOROPHYTE_INGOT)) { e.player.playSound(SoundEvents.ENTITY_ENDERDRAGON_GROWL, 1.0F, 1.0F); } } Thanks!
-
I just can't seem to make it show up Please help. My code: @SubscribeEvent(priority = EventPriority.HIGHEST) //Function: Displays "blood" when an entity is hit public void onHit(LivingHurtEvent e) { if (e.getEntity() instanceof EntityMob) { EntityMob mob = (EntityMob) e.getEntity(); mob.worldObj.spawnParticle(EnumParticleTypes.BLOCK_CRACK, mob.posX, mob.posY, mob.posZ, Math.random() * 0.2 - 0.1, Math.random() * 0.25, Math.random() * 0.2 - 0.1, 137); } } Thanks!
-
1.12 Make block face the direction i'm facing
Differentiation replied to Artsicle's topic in Modder Support
Cool! -
1.12 Make block face the direction i'm facing
Differentiation replied to Artsicle's topic in Modder Support
Like wood logs, and such. -
[1.10.2] Spawning Particles in Front of the Player?
Differentiation posted a topic in Modder Support
Hey all, so I have been experimenting a bit with particles, and I thought to myself: how could I make them spawn in front of the player? I couldn't really figure it out Does anyone have an idea? -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
I said that I was a bit all over the place with this, that's why -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Ahhh you're a life saver! I guess I just wasn't aware. You know, there are just so many classes and I sometimes get a bit confused. -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Aha! I should add proxy#preInit() in my Main Class! Right?? Right? -
[1.10.2] How to set a projectile texture?
Differentiation replied to Differentiation's topic in Modder Support
Ohhhh! I see what you mean. I should add proxy#preInit(), but where?