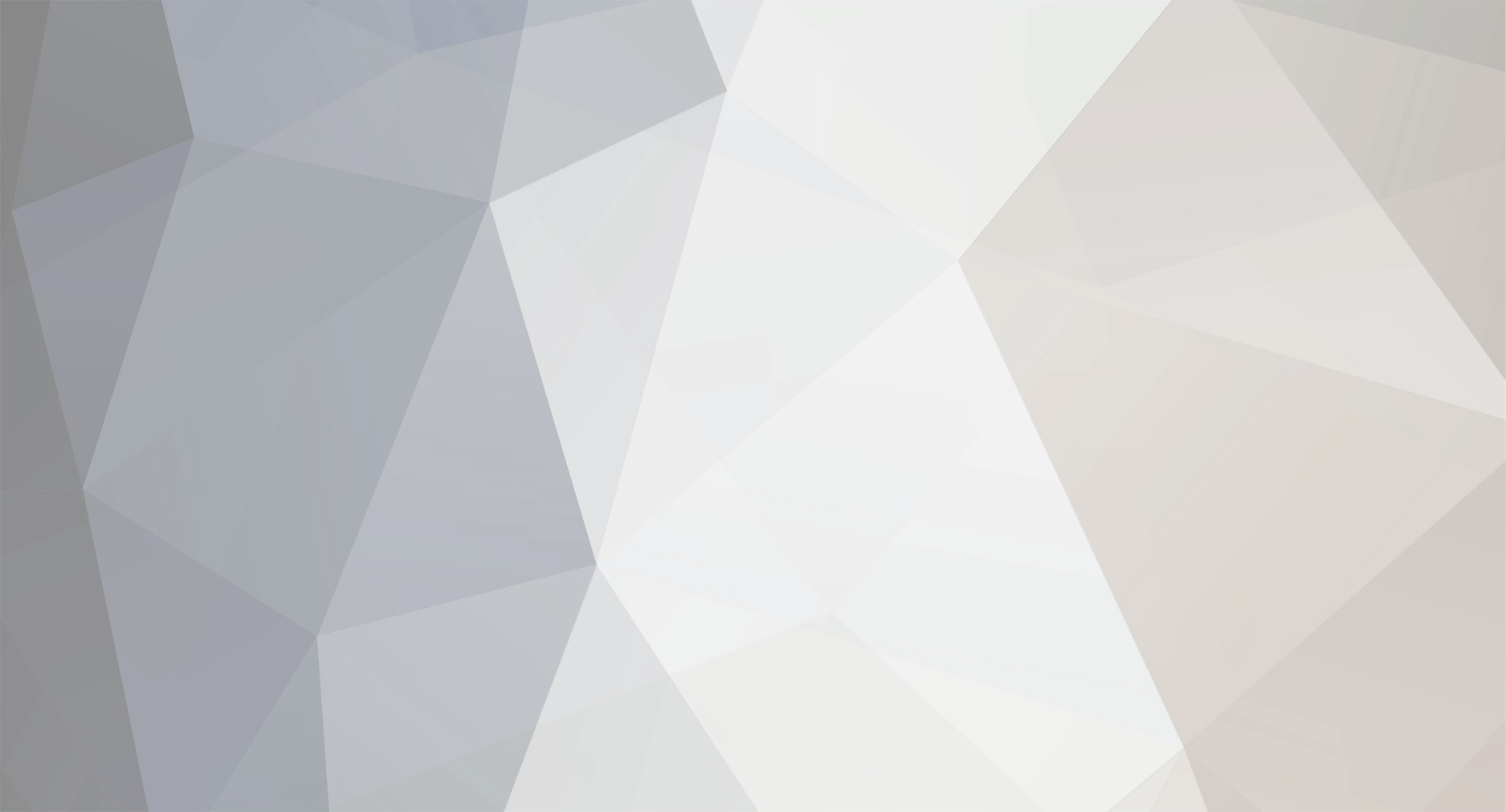
Differentiation
Members-
Posts
606 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Differentiation
-
You're good. Now its solved. Note: you don't have to make an instance of EntityPlayer, you can just use Entity.
-
I'm trying to find that Forge thread for reaching across logical sides to insert here. Do you happen to know the link?
-
I don't really understand what you're trying to do here... You have to understand that Minecraft::getMinecraft is a method only on the client thread. And the Minecraft class is only a class on the client thread. You cannot reference these on the running server thread. This will undoubtedly crash a dedicated server.
-
This is definitely not solved
-
Well, if you're gonna use that then at least include a check for World#isRemote. Otherwise you'd crash with - I believe - ClassDefNotFoundException on a dedicated server. Also, what do you mean move in the air?? The client thread does run on that event. Lastly, just use EntityPlayer#getHeldItemMainhand method to check for the item they are holding instead of what you're doing.
-
You're reaching across logical sides. Use PlayerTickEvent#player. Don't use Minecraft::getMinecraft unless you have to (adding client effects, etc.).
-
[SOLVED] [1.12.2] Runnable Scheduled Tasks
Differentiation replied to Differentiation's topic in Modder Support
Then... can I just do this? public abstract class AbstractPacket<REQ extends IMessage> implements IMessage, IMessageHandler<REQ, REQ> { @Override public REQ onMessage(REQ message, MessageContext context) { if (context.side == Side.SERVER) { EntityPlayerMP player = context.getServerHandler().player; player.getServer().addScheduledTask(new Runnable() { @Override public void run() { handleServerSide(message, player); } }); } else { EntityPlayer player = Dinocraft.PROXY.getPlayer(); Minecraft.getMinecraft().addScheduledTask(new Runnable() { @Override public void run() { handleClientSide(message, player); } }); } return null; } public abstract void handleClientSide(REQ message, EntityPlayer player); public abstract void handleServerSide(REQ message, EntityPlayer player); } -
[SOLVED] [1.12.2] Runnable Scheduled Tasks
Differentiation replied to Differentiation's topic in Modder Support
Thanks! Solved. -
[SOLVED] [1.12.2] Runnable Scheduled Tasks
Differentiation replied to Differentiation's topic in Modder Support
So if I have something as simple as EntityPlayer::capabilities#allowFlight = true; in client handler I'd still need to schedule? -
I know it's good to schedule the messages received by the client and server in order to avoid crashes and bugs. However, should I make the messages scheduled or only a certain few? If so, how do I know which ones to add as a scheduled task? Any help is appreciated. Thanks
-
public class EntityRayBullet extends EntityThrowable { private static final DataParameter<Float> GRAVITY = EntityDataManager.createKey(EntityRayBullet.class, DataSerializers.FLOAT); public ResourceLocation getTexture() { return DinocraftEntities.RAY_BULLET_TEXTURE; } public EntityRayBullet(World world) { super(world); } public EntityRayBullet(World world, EntityLivingBase shooter) { super(world, shooter); } public EntityRayBullet(EntityLivingBase shooter, float gravity) { super(shooter.world, shooter); this.dataManager.set(GRAVITY, gravity); } @Override protected void entityInit() { super.entityInit(); this.dataManager.register(GRAVITY, 0.0F); } private void kill() { if (this.thrower != null && this.thrower instanceof EntityLiving) { DinocraftEntity.getEntity(this.thrower).setShootingTick(0); } this.setDead(); } @SideOnly(Side.CLIENT) @Override public void handleStatusUpdate(byte id) { if (id == 3) { this.world.playSound(this.posX, this.posY, this.posZ, DinocraftSoundEvents.CRACK, SoundCategory.NEUTRAL, 1.0F, rand.nextFloat() + 1.0F, false); for (int i = 0; i < 16; ++i) { this.world.spawnParticle(EnumParticleTypes.ITEM_CRACK, this.posX, this.posY, this.posZ, Math.random() * 0.2 - 0.1, Math.random() * 0.25, Math.random() * 0.2 - 0.1, Item.getIdFromItem(DinocraftItems.RAY_BULLET)); } } } @Override protected void onImpact(RayTraceResult result) { switch (result.typeOfHit) { case BLOCK: { Block block = this.world.getBlockState(result.getBlockPos()).getBlock(); if (block instanceof BlockBush || block instanceof BlockReed || block instanceof BlockVine) { return; } else { if (!this.world.isRemote) { this.kill(); } this.world.spawnParticle(EnumParticleTypes.ITEM_CRACK, result.getBlockPos().getX(), result.getBlockPos().getY(), result.getBlockPos().getZ(), Math.random() * 0.2 - 0.1, Math.random() * 0.25, Math.random() * 0.2 - 0.1, Item.getIdFromItem(DinocraftItems.RAY_BULLET)); } break; } case ENTITY: { if (!this.world.isRemote && result.entityHit != null && result.entityHit instanceof EntityLivingBase && result.entityHit != this.getThrower()) { EntityLivingBase thrower = this.getThrower(); result.entityHit.attackEntityFrom(thrower != null ? thrower instanceof EntityPlayer ? DamageSource.causeThrownDamage(this, thrower) : DamageSource.causeMobDamage(thrower) : DamageSource.GENERIC, result.entityHit.isNonBoss() ? this.rand.nextFloat() + 4.5F : this.rand.nextFloat() + 0.5F); this.world.playSound(null, result.entityHit.getPosition(), DinocraftSoundEvents.HIT, SoundCategory.NEUTRAL, 1.0F, rand.nextFloat() + 1.0F); this.kill(); } else { return; } this.world.spawnParticle(EnumParticleTypes.ITEM_CRACK, result.entityHit.posX, result.entityHit.posY, result.entityHit.posZ, Math.random() * 0.2 - 0.1, Math.random() * 0.25, Math.random() * 0.2 - 0.1, Item.getIdFromItem(DinocraftItems.RAY_BULLET)); break; } default: break; } if (!this.world.isRemote) { this.world.setEntityState(this, (byte) 3); this.kill(); } } @Override public void onUpdate() { if (this.ticksExisted > 200) { this.setDead(); } if (this.isInWater()) { this.motionY -= 0.005D; this.motionX *= 0.95D; this.motionZ *= 0.95D; } super.onUpdate(); } @Override protected float getGravityVelocity() { return this.dataManager.get(GRAVITY); } }
-
Okay, that's solved. However, I don't understand why the bullets fired don't hit the entity I'm shooting at if I'm like 0 or 1 block away from it... This also happened before I got it to fire every tick. I tried setting the position of the bullet a little bit behind the player when it spawns in an attempt to avoid this, but then if the player has a block behind him, the bullet just ends up hitting the block. Is there any way to resolve this?
-
Use this guy's tutorial. It's very good if you follow it in the exact order, step-by-step.