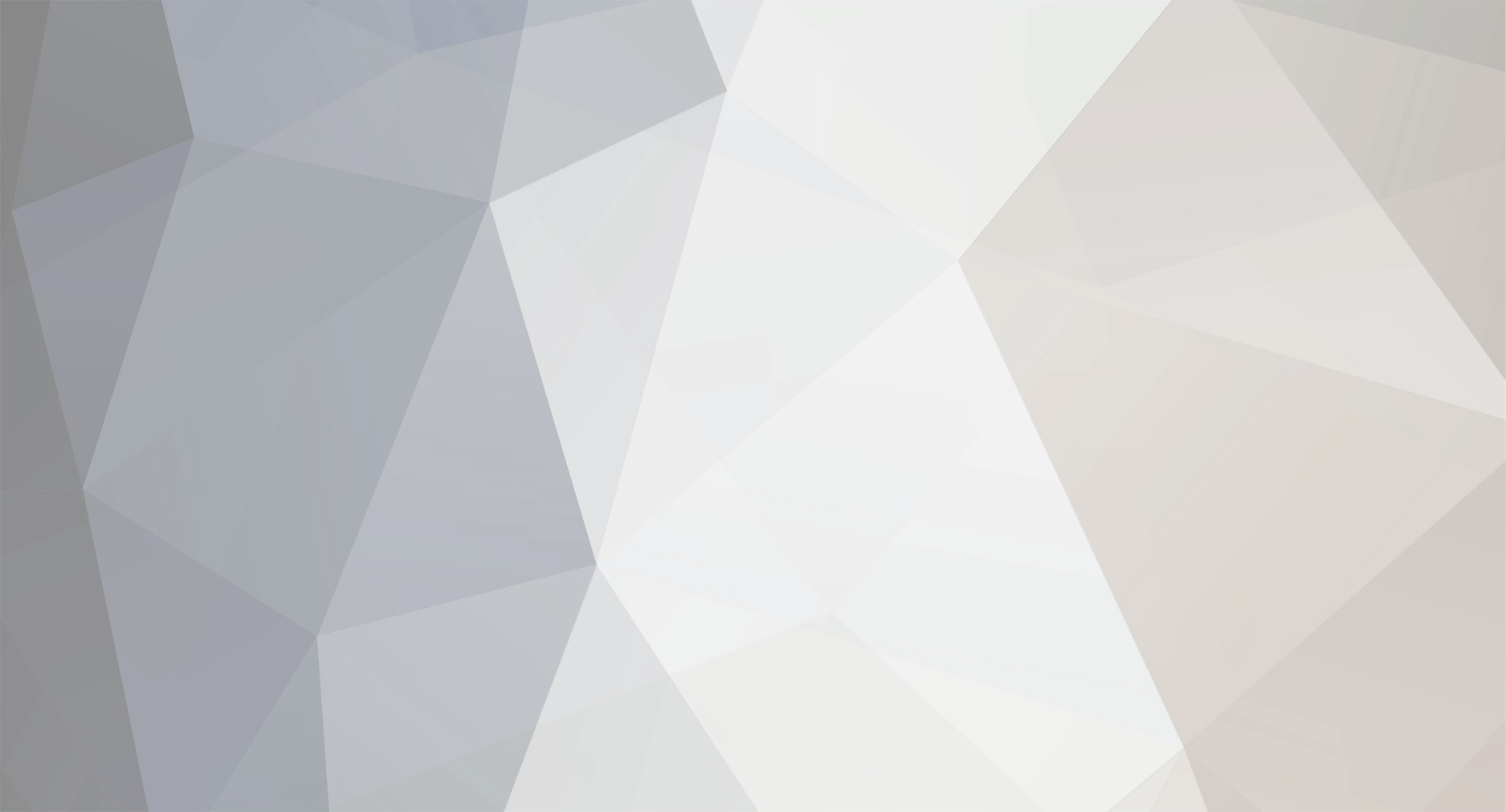
Mew
Members-
Posts
567 -
Joined
-
Last visited
Everything posted by Mew
-
As the title states, how would I do this? I thought that running it server side only via a packet would fix it, but it didn't. So I am at a loss... The packet code I am using is here: package rpg.network.packet; import net.minecraft.entity.player.EntityPlayer; import com.google.common.io.ByteArrayDataInput; import com.google.common.io.ByteArrayDataOutput; import cpw.mods.fml.relauncher.Side; public class PacketSendChat extends MinePGPacket { String message; public PacketSendChat() { } public PacketSendChat(String message) { this.message = message; } @Override protected void execute(EntityPlayer player, Side side) { if (side.isServer() && !side.isClient()) { player.sendChatToPlayer(message); } } @Override protected void writeData(ByteArrayDataOutput out) { out.writeUTF(message); } @Override protected void readData(ByteArrayDataInput in) { this.message = in.readUTF(); } }
-
I think that using the biomeList (the list of all the biomes that are available, including modded ones), you could check to see what ID's are used, and then (somehow as there is no method that I can find that returns the name of the biome) get the name of the biome. Then, using that getName() method or whatever you are using to get it, print out the ID of the biome, and the name of the corresponding ID. After obtaining that information, you could then read through and find the biomes you needed.... And if you are just wanting to spawn a mob in one biome only, its like this: EntityRegistry.addSpawn(EntityToSpawn.class, weightedProb, min, max, typeOfCreature, BiomeGenBase.nameOfBiome); or, for a modded biome: EntityRegistry.addSpawn(EntityToSpawn.class, weightedProb, min, max, typeOfCreature, WhereYourBiomeVariablesAreKept.nameOfBiome);
-
No, I don't think that is quite right. Questology uses it. Theirs is called QuestologyWorldData I think on their github repo.
-
Thats fairly easy. Just call the EntityRegistry.addSpawn(); twice, once with the mob as EnumCreatureType.CREATURE, and the other as EnumCreatureType.MONSTER. That will spawn it as a monster (night) and creature (day). Couldn't this be achieved by checking if the player is in the dimension needed? (or do you need the dimension ID still?) If this is possible, then you could spawn it in a dimension specific biome. (I assume that the dimension biomes do not spawn in the overworld, and only in the dimension?) I believe that cannot be achieved with vanilla code...
-
Ahh.... I see some of your problems... I think. I am not quite getting some of these problems So If you could try explaining them in a bit more detailed way?? Then I might be able to read it the way you want it to be read.
-
Ahh. Do you know how to generate ores in the world? Overworld/Nether/End? If you do its EXACTLY the same concept, you just have to generate the structure instead. I'll explain a bit better later.
-
What do you mean, "I don't know how to do it in already existing ones."? That doesn't quite make sense to me
-
Go to youtube. Type in "theinstitutions" and go to his playlist for structure generation. I know they are for an older version of Minecraft, and they may be for ModLoader, but that doesn't matter. The WorldGen** file (** being the name you give it) is the same across the amount of time and API used. The only thing that changes is instead of world.setBlockWithNotify();, its world.setBlock(); Pretty easy really. Another difference is the that instead of generating the structure in the world from your main mod file, you make it generate using the normal ore generation stuff. As for the randomly shaped structures, I believe you would have to code a new structure for every "Random" structure you want, that uses a random. something like this: (Note that this method will be explained in the afore mentioned tutorial) public void generate(Random random) { // I have added the random for the 'random' structure check Random rand = random; int randomNumber = rand.nextInt(3); // this generates a random number between 0 and 3 switch(randomNumber) { case 0: world.setBlock(x, y, z, Block.stone.blockID); // x, y, z variables being defined in the tutorial break; case 1: world.setBlock(x, y, z, Block.wood.blockID); break; case 2: world.setBlock(x, y, z, Block.blockDiamond.blockID); break; case 3: world.setBlock(x, y, z, Block.blockGold.blockID); break; } } That would be the sorta thing needed for making a "randomly shaped" generated structure. All you have to do is change the shape that the blocks that get spawned make the structure have the different shapes. I hope that helps... If not I wasted 5 minutes of my life writing this
-
Not needed. What you do, is that when you call the EntityRegistry.addSpawn(); method, you sawn as a EnumCreatureType.CREATURE (I think thats what it is, I know it's EnumCreatureType though) for spawning during the day, and EnumCreatureType.MONSTER (once again, same issue as before) for during the night. Then there is EnumCreatureType.WATER. If that isn't self explanatory then I don't know what is But yeah, the EntityRegistry.addSpawn(); is where you can register if it spawns or not and such. As for the spawning only one in a chunk, there is a method in the Entity class that sets that I think. And for the adjacent chunks.... Well, have fun with that
-
How to make a number increment by 1 at the same speed the player heals at
Mew replied to Mew's topic in Modder Support
Thats not quite what I meant... I DON't want the players food level, I was just wanting the mana to regenerate at the same speed that the players health does. I was just pointing out that the player only heals at all when the food bar is full. -
Yes, I could get that much done, I just don't get how it draws the full bar onto the empty one SO if that could be explained in the code you posted???
-
I have a Mana type system that I want to use, I can make the mana variable and such, I can make it decrease, but how do I make it increase? I mean, aside from manually making the mana increase, how do I make it regenerate like the players health does when the player is at full food? thanks for any help, Mew
-
I am wanting to make a bar like the vanilla experience bar but with my own values. I want the bar to have the two textures like the experience bar (the empty and full textures) and display the increments of "experience" shown when it gets to the right amount (what I mean is that the bar fills up with a little bit of the full texture being shown on the screen). I know this will involve a tick handler, but I am not entirely sure how to go about it... So any help will be appreciated.
-
Not without editing base classes I would assume
-
Can you send me your projectile code? or thrown entity code as you put it? I can't get mine to work... Also, does it actually render as visible? Whenever I try to make projectiles and such I only get an invisible entity
-
How to make your dimension generate without stone
Mew replied to Squawkers13's topic in Modder Support
Look through my Dimension code on the Spazzysmod github. -
That was the other one I was forgetting Its EntityRegistry.registerModEntity(EntityGrenade.class, "Grenade", 4, this, 350, 5, false); type thing instead of registerMob
-
This happened to me too... Apparently when registering an entity you have to do a double registry using EntityRegistry.registerUniqueModID or something as well as registerMob or something like that. I can't remember at this point in time
-
Your welcome. I can't believe that I didn't think of that earlier.... I hope nothing else botches on ya.
-
here is an example of my config file: # Configuration file #################### # block #################### block { I:"Minotaur Portal ID"=1102 I:"Taurite Block ID"=250 I:"Taurite Ore ID"=1101 } #################### # item #################### item { I:"Taurite Cloth ID"=1101 I:"Taurite ID"=1100 I:"Training Arrow ID"=1013 I:"Training Bow ID"=1012 I:"Training Cuirass ID"=1009 I:"Training Greaves ID"=1010 I:"Training Helm ID"=1005 I:"Training Helmet ID"=1008 I:"Training Pants ID"=1003 I:"Training Robe ID"=1006 I:"Training Sabatons ID"=1011 I:"Training Sandals ID"=1007 I:"Training Shoes ID"=1004 I:"Training Staff ID"=1014 I:"Training Sword ID"=1017 I:"Training Tunic ID"=1002 I:"Training Wand ID"=1015 I:"Training WarAxe ID"=1016 } and here is how I did some of the ID's: Item: bowTrainingID = config.get(Configuration.CATEGORY_ITEM, "Training Bow ID", 1012).getInt(); arrowTrainingID = config.get(Configuration.CATEGORY_ITEM, "Training Arrow ID", 1013).getInt(); Block: tauriteBlockID = config.get(Configuration.CATEGORY_BLOCK, "Taurite Block ID", 250).getInt(); oreTauriteID = config.get(Configuration.CATEGORY_BLOCK, "Taurite Ore ID", 1101).getInt(); portalMinoID = config.get(Configuration.CATEGORY_BLOCK, "Minotaur Portal ID", 1102).getInt();
-
Just use configInstance.get(); thats what I use. And it works
-
No, thats an actual block mate I am pretty sure that if he calls the variable "WhiteBlockID" and the little notation that goes next to the id in the config is "snow_block".
-
Your welcome!
-
Well, maybe. But all I know is that Forge only has biome removals. I haven't come across anything for structure generation
-
have fun with that. All I can say...