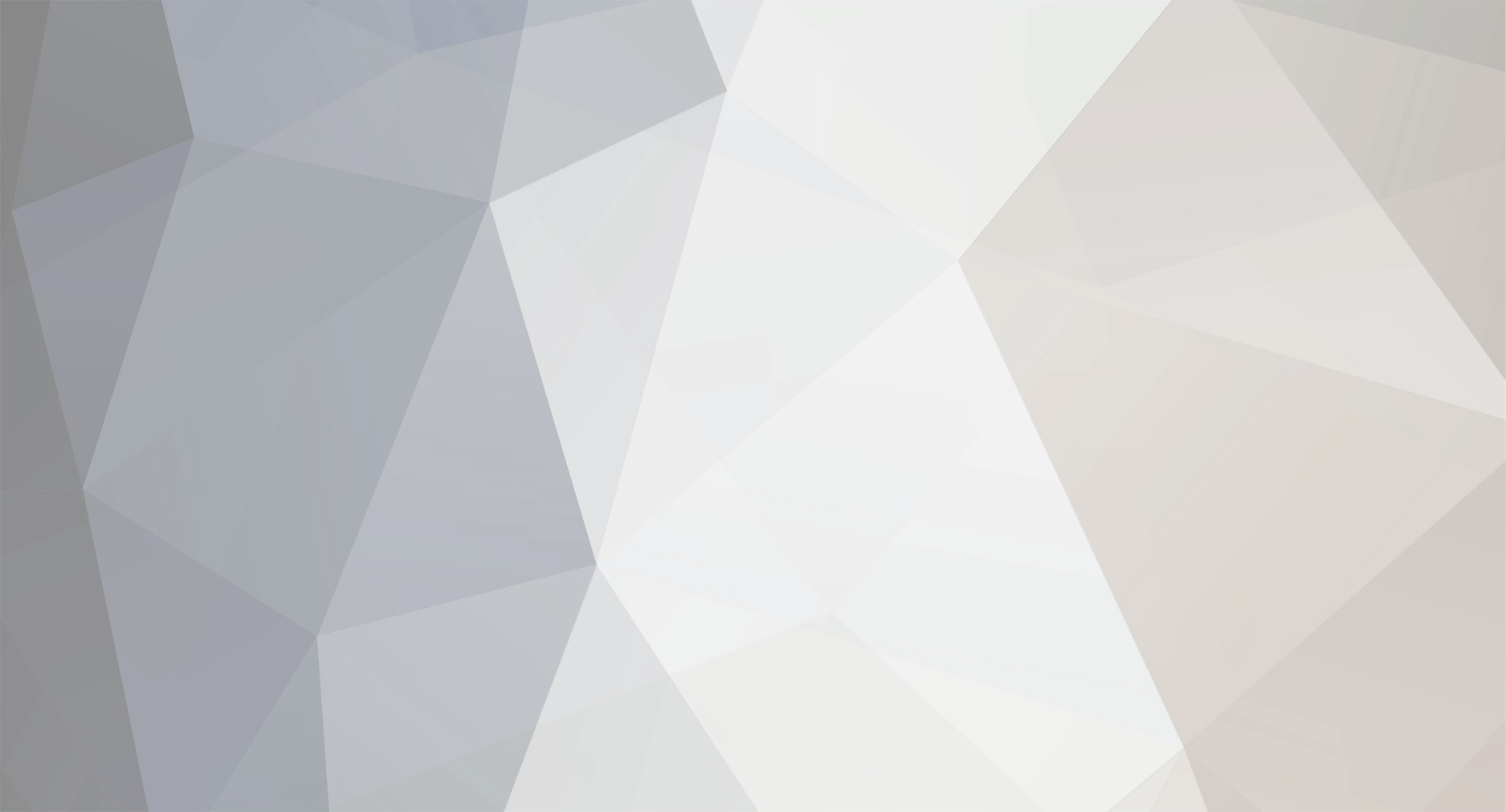
Mew
Members-
Posts
567 -
Joined
-
Last visited
Everything posted by Mew
-
You NEED a new for loop everytime. You also NEED the switch statement. Just in case I read that wrong, sorry.
-
because then I have them there when I need them
-
If a new minecraft version comes out, you should GENERALLY move to it. But if it wrecks your code too much, then stay on the previous version.
-
Here is how I do generation: https://github.com/ModderPenguin/JC-s-Dragons-Mod---add-ons/blob/master/source/motoolsnstuff/worldgen/WorldGeneratorDragonBros.java And I realised that you had the right implementation, but I got it confused with another one
-
have you tried following a tutorial? This way seems weird for me...
-
[Solved + example Code][1.5.2][Forge 8.7.705] Custom Bow in Third Person View
Mew replied to keynah's topic in Modder Support
Give me a few minutes... Ill have an example for you... -
[Solved + example Code][1.5.2][Forge 8.7.705] Custom Bow in Third Person View
Mew replied to keynah's topic in Modder Support
yes it did. I would suggest looking into the actual ItemBow class and then copying it all to your bow class, and then trying to figure out how it does the textures. Best way to learn is to be pointed in the right direction -
No. YourConfigVariable.getBlock()* is for any ole' block. YourConfigVariable.getTerrainBlock()* makes sure that the block ID isunder 255. So any time you want to make a block that will be a biome block, use .getTerrainBlock(). any other block use .getBlock. Notes: * You do know that YourConfigVariable is the variable you assign to Configuration? I'm sure you do, but I am just checking
-
[Solved + example Code][1.5.2][Forge 8.7.705] Custom Bow in Third Person View
Mew replied to keynah's topic in Modder Support
Say what? will not work register as a normal bow? And also, what I got from this was that you WANTED to have the player appear to shoot himself? -
Making a Energy Framework... Hmm. I figured out what my next project is going to be! Don't be too expectant on me though, you should try to build one yourself. After all, knowledge is gained through asking questions, and experience is gained through using knowledge, but also, experience is gained by doing, and knowledge is gained from experience. Point of that is, there are many ways to learn. You just have to use them. Have fun exploring and creating!
-
Use config files. It creates a ton more compatibility with other mods. It allows the user to change the ID's that are used for items and such. Basically I highly recommend using config files. And even though the code may SAY that it is under 255, doesn't mean it is. Its kinda weird
-
Do you use a config file? With that you can make sure that block ID's are under 255. using YourConfigVariable.getTerrainBlock(Parameters...)
-
Simple. The ID for your block is not below 255.
-
Here is the link https://github.com/tuyapin/InvertedWorld That link is not there anymore
-
Reading string from PlayerInformation (extends IExtendedEntityProperties)
Mew replied to Mew's topic in Modder Support
I can't quite figure it out... I will try again a bit later. but any pointers in the right direction would be helpful. -
Reading string from PlayerInformation (extends IExtendedEntityProperties)
Mew replied to Mew's topic in Modder Support
So I would need to make a packet that first, got the data needed, then second, sent the data to the client, and thirdly, take the received data to a String variable inside the item class, and lastly, do what I was doing before? -
When I read the string via my connection handler class inside the playerLoggedIn() method (connection handler class extends IConnectionHandler), it reads the string as it should be. But when I try to read the string from any of my items, addInformation() methods, the string returns as either null, or "". So either A) how do I fix this, or, B) what am I doing wrong? Code for PlayerInformation: package rpg.playerinfo; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.world.World; import net.minecraftforge.common.IExtendedEntityProperties; public final class PlayerInformation implements IExtendedEntityProperties { public static enum CountableKarmaEvent { PIGMEN_ATTACK(1), CREATE_SNOWGOLEM(2), CREATE_IRONGOLEM(3); private final int maxCount; private CountableKarmaEvent(int maxCount) { this.maxCount = maxCount; } public int getMaxCount() { return maxCount; } } public static final String IDENTIFIER = "minepg_playerinfo"; public static final int MAX_KARMA_VALUE = 99999999; public static PlayerInformation forPlayer(Entity player) { return (PlayerInformation) player.getExtendedProperties(IDENTIFIER); } // called by the ASM hook in EntityPlayer.clonePlayer public static void handlePlayerClone(EntityPlayer source, EntityPlayer target) { target.registerExtendedProperties(IDENTIFIER, source.getExtendedProperties(IDENTIFIER)); } public boolean dirty = true; private float karma = 0; public byte[] eventAmounts = new byte[PlayerInformation.CountableKarmaEvent .values().length]; private String playersClass; private int danris = 0; private final EntityPlayer player; public PlayerInformation(EntityPlayer player) { this.player = player; } public int getCurrency() { return danris; } public byte getEventAmount(CountableKarmaEvent event) { return eventAmounts[event.ordinal()]; } public float getKarma() { return karma; } public String getPlayersClass() { return playersClass; } public boolean increaseEventAmount( PlayerInformation.CountableKarmaEvent event) { return setEventAmount(event, eventAmounts[event.ordinal()] + 1); } @Override public void init(Entity entity, World world) { } @Override public void loadNBTData(NBTTagCompound playerNbt) { NBTTagCompound nbt = playerNbt.getCompoundTag(IDENTIFIER); playersClass = nbt.getString("playersClass"); System.out .println("Debug message. If this has been printed to the console then NBTData has been read."); danris = nbt.getInteger("danris"); karma = nbt.getFloat("karma"); NBTTagList eventList = nbt.getTagList("events"); for (int i = 0; i < eventList.tagCount(); i++) { NBTTagCompound evtInfo = (NBTTagCompound) eventList.tagAt(i); byte eventId = evtInfo.getByte("id"); if (eventId >= 0 && eventId < eventAmounts.length) { eventAmounts[eventId] = evtInfo.getByte("value"); } } } public float modifyKarma(float modifier) { player.worldObj.playSoundAtEntity(player, "minepg.karma" + (modifier < 0 ? "down" : "up"), 1, 1); return setKarma(karma + modifier); } public float modifyKarmaWithMax(float modifier, float max) { if (karma < max) { modifyKarma(modifier); } return karma; } public float modifyKarmaWithMin(float modifier, float min) { if (karma > min) { modifyKarma(modifier); } return karma; } @Override public void saveNBTData(NBTTagCompound compound) { NBTTagCompound nbt = new NBTTagCompound(); nbt.setString("playersClass", playersClass); nbt.setInteger("danris", danris); nbt.setFloat("karma", karma); NBTTagList eventList = new NBTTagList(); for (int i = 0; i < eventAmounts.length; i++) { NBTTagCompound evtInfo = new NBTTagCompound(); evtInfo.setByte("id", (byte) i); evtInfo.setByte("value", eventAmounts[i]); eventList.appendTag(evtInfo); } nbt.setTag("events", eventList); compound.setCompoundTag(IDENTIFIER, nbt); } public int setCurrency(int danris) { if (this.danris != danris) { this.danris = danris; setDirty(); } if (this.danris > 999999) { this.danris = 999999; setDirty(); } return this.danris; } /** * marks that this needs to be resend to the client */ public void setDirty() { dirty = true; } public boolean setEventAmount(CountableKarmaEvent event, int amount) { if (amount < event.getMaxCount() && eventAmounts[event.ordinal()] != amount) { eventAmounts[event.ordinal()] = (byte) amount; setDirty(); return true; } else return false; } public float setKarma(float karma) { if (this.karma != karma) { this.karma = karma; if (this.karma > MAX_KARMA_VALUE) { this.karma = MAX_KARMA_VALUE; } if (this.karma < -MAX_KARMA_VALUE) { this.karma = -MAX_KARMA_VALUE; } setDirty(); } return this.karma; } public String setPlayersClass(String playersClass) { if (this.playersClass != playersClass) { this.playersClass = playersClass; setDirty(); } return this.playersClass; } } Code for ConnectionHandler: package rpg.comm; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.network.INetworkManager; import net.minecraft.network.NetLoginHandler; import net.minecraft.network.packet.NetHandler; import net.minecraft.network.packet.Packet1Login; import net.minecraft.server.MinecraftServer; import rpg.RPG; import rpg.enums.EnumGui; import rpg.network.packet.PacketUpdatePlayersClassName; import rpg.playerinfo.PlayerInformation; import rpg.sounds.SoundLoader; import cpw.mods.fml.common.network.IConnectionHandler; import cpw.mods.fml.common.network.Player; public class ConnectionHandler implements IConnectionHandler { @Override public void clientLoggedIn(NetHandler clientHandler, INetworkManager manager, Packet1Login login) { } @Override public void connectionClosed(INetworkManager manager) { } @Override public void connectionOpened(NetHandler netClientHandler, MinecraftServer server, INetworkManager manager) { } @Override public void connectionOpened(NetHandler netClientHandler, String server, int port, INetworkManager manager) { } @Override public String connectionReceived(NetLoginHandler netHandler, INetworkManager manager) { return null; } @Override public void playerLoggedIn(Player player, NetHandler netHandler, INetworkManager manager) { PlayerInformation playerInfo = PlayerInformation .forPlayer((EntityPlayerMP) player); if (playerInfo.getPlayersClass().equals("")) { ((EntityPlayerMP) player).openGui(RPG.instance, EnumGui.LoreStartingPage.getIndex(), ((EntityPlayerMP) player).worldObj, 0, 0, 0); } else { ((EntityPlayerMP) player) .sendChatToPlayer("<Mysterious Voice> Welcome back master " + playerInfo.getPlayersClass()); new PacketUpdatePlayersClassName().sendToServer(); } if (SoundLoader.didSoundsLoad == true) { ((EntityPlayerMP) player) .sendChatToPlayer("[MinePG Sound Loader] Loaded Sounds Successfully"); } else if (SoundLoader.didSoundsLoad == false) { ((EntityPlayerMP) player) .sendChatToPlayer("[MinePG Sound Loader] Failed to load one or more sounds"); } } } Code for an item's addInformation() method: @Override @SuppressWarnings({ "rawtypes", "unchecked" }) public void addInformation(ItemStack par1ItemStack, EntityPlayer player, List par3List, boolean par4) { PlayerInformation playerInfo = PlayerInformation.forPlayer(player); // Checks the players class and colored item name // accordingly if (playerInfo.getPlayersClass() == "Warrior" && player.experienceLevel >= 1) { par3List.add("Class: §AWarrior"); par3List.add("Level: §A1"); } else if (playerInfo.getPlayersClass() == "Warrior" && player.experienceLevel != 1) { par3List.add("Class: §AWarrior"); par3List.add("Level: §41"); } else if (playerInfo.getPlayersClass() != "Warrior" && player.experienceLevel == 1) { par3List.add("Class: §4Warrior"); par3List.add("Level: §A1"); } else { par3List.add("Class: §4Warrior"); par3List.add("Level: §41"); } } [sIDE NOTE] As the code for the addInformation() method is above, it returns as "", but if I use the proper .equals("String here"), it returns as null.
-
Ahh. Thank you good sir. Jolly good show wot?
-
Do you want the block to drop the spawner item or the item to turn into the spawner item or the spawner item just be added into your inventory? This question is quite diverse
-
So, what are good uses for core mods?
-
I FIXED IT!!!! ;D ;D But now I have the problem of some other things not saving... Like the level of the player. The players level gets set DOWN one level on world reloaded. What am I doing wrong? same code as before except I changed compound.setCompoundTag(IDENTIFIER, player.getEntityData()); to: compound.setCompoundTag(IDENTIFIER, nbt); Nothing has changed except that. So what am I doing wrong to have it stuff up the players level?
-
So how would I fix this? I know you say to understand, but I only understand by someone explaining it to me. Then I can understand. Sorry for being annoying if I seem that way. I am just a bit thick at times