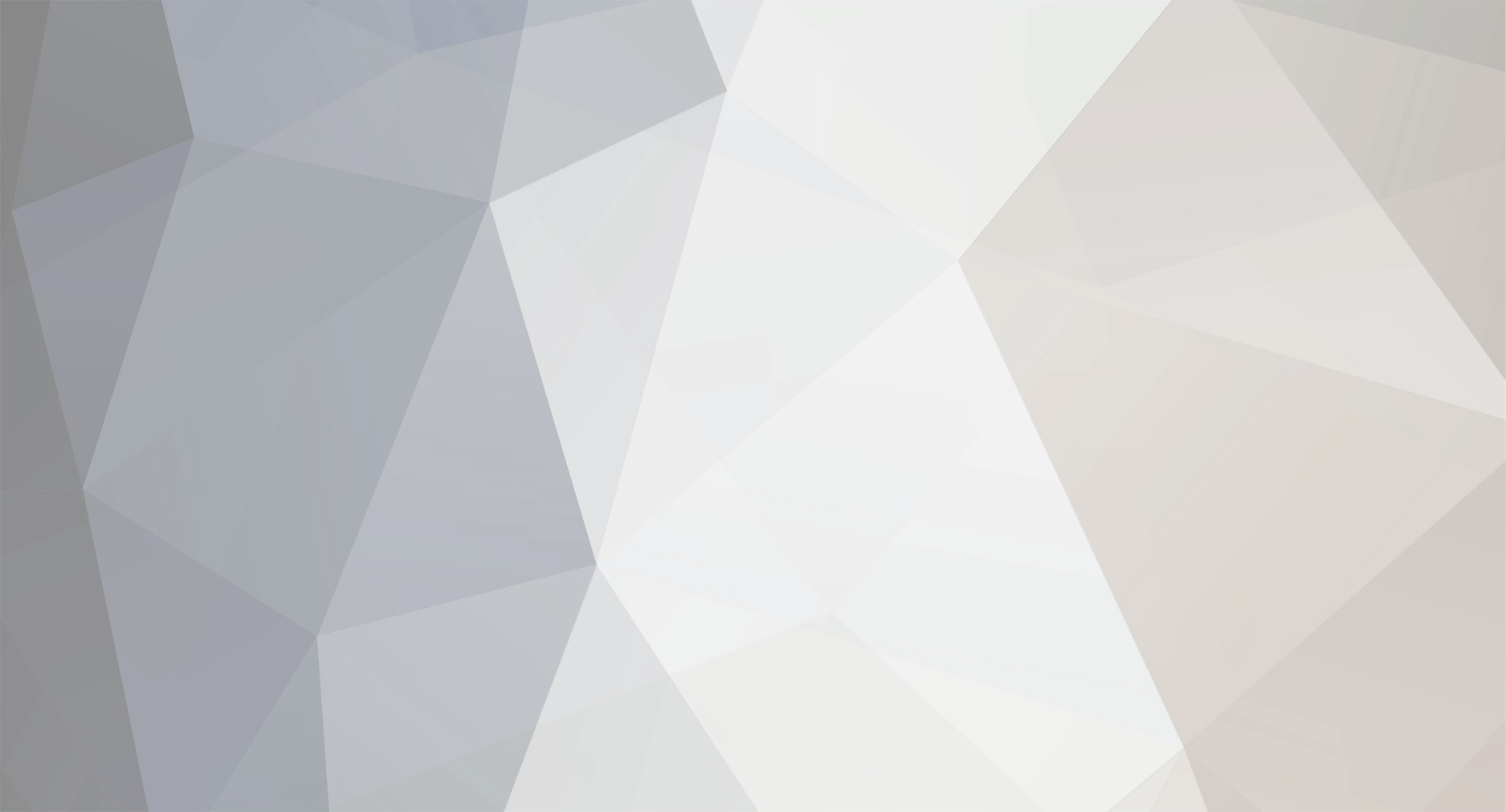
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Proper way to render multiblock. 1.15.2
TheGreyGhost replied to AntonBespoiasov's topic in Modder Support
Howdy Based on your description, I'd recommend using standard Blocks and Blockstates for all the slaves and TileEntities for the masters. Your Multimap cache idea sounds ok but you will need to pay careful attention to Chunk loading, i.e. you will need to synchronise your multimap to the currently loaded chunks somehow. That is more difficult than it sounds because they are multithreaded. -TGG -
1.15.2 Rendering particle fullbright
TheGreyGhost replied to xX_deadbush_Xx's topic in Modder Support
Howdy Easiest way is just to return full brightness from your particle's method. Particle rendering has changed quite a bit from earlier versions of vanilla and you no longer need to do custom rendering for most particles. eg // can be used to change the skylight+blocklight brightness of the rendered Particle. @Override protected int getBrightnessForRender(float partialTick) { final int BLOCK_LIGHT = 15; // maximum brightness final int SKY_LIGHT = 15; // maximum brightness final int FULL_BRIGHTNESS_VALUE = LightTexture.packLight(BLOCK_LIGHT, SKY_LIGHT); return FULL_BRIGHTNESS_VALUE; // if you want the brightness to be the local illumination (from block light and sky light) you can just use // the Particle.getBrightnessForRender() base method, which contains: // BlockPos blockPos = new BlockPos(this.posX, this.posY, this.posZ); // return this.world.isBlockLoaded(blockPos) ? WorldRenderer.getCombinedLight(this.world, blockPos) : 0; } For a working example, see here https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe50_particle -TGG -
Proper way to render multiblock. 1.15.2
TheGreyGhost replied to AntonBespoiasov's topic in Modder Support
Hi There are some example implementations here for IBakedModel and custom IModelData to pass information to a block renderer. https://github.com/TheGreyGhost/MinecraftByExample (mbe03, mbe04, mbe05) My first thought: make the master block a tileentity and the slave blocks normal blocks. If your slaves don't have too many different states, then store them as a blockstate. Use the master tileentity to coordinate the slaves and keep them up-to-date. If at all possible, avoid having the slaves try to talk to the master because they may be in different chunks which may be loaded at different times (i.e. the master may not be loaded). If you describe your multiblock structure in more detail (i.e. in gameplay terms - from the point of view of someone playing the game, not from the point of view of what you think the code should look like) then we could make some more concrete suggestions... Cheers TGG -
[1.15.2] Render TERs from RenderWorldLastEvent
TheGreyGhost replied to nanorover59's topic in Modder Support
Howdy What you're trying to do is quite difficult. Are your TileEntities actually in a world at all? Blocks (or rather blockstates) can be rendered independently of a World if you're careful but a TileEntityRenderer nearly always needs to speak to its corresponding TileEntity for specific information. You could simulate this by creating your own world (perhaps in another dimension that the player can never visit) that you then use for rendering in this world. You may even be able to use the vanilla rendering code to render a tiny version of it to a dynamic texture, which you then draw into this world. It will probably be messy to get it right but it should work. You'll probably need to spend a fair bit of time understanding the vanilla rendering code. It's not difficult but there are lots of details to understand and the chunk render caching can be quite difficult to follow / troubleshoot. -TGG -
Hi It really depends on what effect you're trying to achieve. If you just want a block shape, consider using BlockBench and exporting as an entity model or block model, and rendering that. Or alternatively, using Blender and exporting as an obj (wavefront model). (Examples in this project if you need them: https://github.com/TheGreyGhost/MinecraftByExample) Adding vertices manually is usually the hard way of doing it. Unless you're aiming for a specific effect (eg like the Beacon) I'd consider using a standard method. -TGG
-
[1.15.2] Custom RenderType does not render
TheGreyGhost replied to Alex Sim's topic in Modder Support
Hi Nothing easy springs to mind; if you only have a few of your blocks in the world at any one time then a TileEntityRenderer will do it. Alternatively you could render them in RenderWorldLastEvent with your own caching + chunk-culling logic but that may have side effects with translucent textures. The first thing I would personally try would be to replace WorldRenderer with a derived class (will require reflection)- at the cost of being pretty fragile and perhaps making your mod incompatible with others. Other folks have used Reflection and asm to locate and overwrite the byte code to insert a call to a new method at the desired location - that's getting very arcane and perhaps not worth the trouble. There might be other ways to do it if you have other mods installed (perhaps Optifine or similar provides an alternative mechanism for what you want to do) -TGG -
Hi A vertex follows a particular format (see DefaultVertexFormats and RenderType) depending on which render buffer you are writing to eg private static final RenderType SOLID = makeType("solid", DefaultVertexFormats.BLOCK, 7, 2097152, true, false, RenderType.State.getBuilder().shadeModel(SHADE_ENABLED).lightmap(LIGHTMAP_ENABLED).texture(BLOCK_SHEET_MIPPED).build(true)); public static final VertexFormat BLOCK = new VertexFormat(ImmutableList.<VertexFormatElement>builder().add(POSITION_3F).add(COLOR_4UB).add(TEX_2F).add(TEX_2SB).add(NORMAL_3B).add(PADDING_1B).build()); versus public static final RenderType.Type LINES = makeType("lines", DefaultVertexFormats.POSITION_COLOR, 1, 256, RenderType.State.getBuilder().line(new RenderState.LineState(OptionalDouble.empty())).layer(PROJECTION_LAYERING).transparency public static final VertexFormat POSITION_COLOR = new VertexFormat(ImmutableList.<VertexFormatElement>builder().add(POSITION_3F).add(COLOR_4UB).build()); When you write a vertex to SOLID, you need to supply position (3 floats), colour (4 bytes), texture (2 floats), light map (2 shorts), normals (3 bytes), plus 1 byte padding. But for LINES you only supply position (3 floats) and colour (4 bytes). So for example- if the code you're using to render to the buffer (during your TER rendering) assumes that the buffer is LINES, but the buffer is actually SOLID, then your code will only write (3 floats + 4 bytes) for each vertex, which is not enough to fill all the elements of the vertex. -TGG
-
[1.15.2] Custom RenderType does not render
TheGreyGhost replied to Alex Sim's topic in Modder Support
Howdy I'm not sure I understand what you're trying to do. If you make a custom render type for your block, you need to render those blocks yourselves manually. Minecraft renders blocks as follows: 1) Creates a render buffer based on SOLID. Look for all blocks which have SOLID render layer; call their rendering methods to write to the SOLID render buffer 2) Create a render buffer based on CUTOUT.Look for all blocks which have CUTOUT render layer; call their rendering methods to write to the CUTOUT render buffer 3) CUTOUTMIPPED 4) TRANSLUCENT Just because you've given your block a custom RenderType, doesn't mean that vanilla will create a suitable render buffer for it. If your render type doesn't match the four vanilla block render types, it won't get drawn at all. If it does match a vanilla render type (because you have done something tricky with equals()) then it will be rendered with the vanilla render type, not your custom render type. -TGG -
Howdy don't use capital letters in your namespace -TGG
-
[1.14.4] Custom elytra layer not rendering transparency
TheGreyGhost replied to ReconCubed's topic in Modder Support
no worries you're welcome, was an interesting debugging exercise for me -
Hi I think it's better to split your obj file into 8 separate files. If you really want to load just a small part of one obj file, have a look at OBJLoader and OBJmodel to see how it parses the file (g and o in particular); you can copy it to your own loader class and tweak it if you want to. TGG
-
[1.14.4] Custom elytra layer not rendering transparency
TheGreyGhost replied to ReconCubed's topic in Modder Support
Well after a bit of refactoring I figured out the problem It's more obvious when I replaced the magic numbers with the proper OpenGL constants TextureUtil.prepareImage(this.getGlTextureId(), overlaidElytra.getWidth(), overlaidElytra.getHeight()); GlStateManager.pixelTransfer(GL11.GL_ALPHA_BIAS, Float.MAX_VALUE); // Sets alpha of final image to max.... delete this line and it works fine. overlaidElytra.uploadTextureSub(0, 0, 0, false); GlStateManager.pixelTransfer(GL11.GL_ALPHA_BIAS, 0.0F); -TGG PS the refactored code FYI package me.reconcubed.communityupdate.client.render; import com.mojang.blaze3d.platform.GlStateManager; import com.mojang.blaze3d.platform.TextureUtil; import java.io.IOException; import java.util.List; import net.minecraft.client.renderer.texture.NativeImage; import net.minecraft.client.renderer.texture.Texture; import net.minecraft.item.DyeColor; import net.minecraft.resources.IResource; import net.minecraft.resources.IResourceManager; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraftforge.api.distmarker.Dist; import net.minecraftforge.api.distmarker.OnlyIn; import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.Logger; import org.lwjgl.opengl.GL11; import org.lwjgl.opengl.GL12; import org.lwjgl.opengl.GL14; @OnlyIn(Dist.CLIENT) public class LayeredColorMaskTextureCustom extends Texture { private static final Logger LOGGER = LogManager.getLogger(); private final ResourceLocation textureLocation; private final List<String> listTextures; private final List<DyeColor> listDyeColors; public LayeredColorMaskTextureCustom(ResourceLocation textureLocationIn, List<String> p_i46101_2_, List<DyeColor> p_i46101_3_) { this.textureLocation = textureLocationIn; this.listTextures = p_i46101_2_; this.listDyeColors = p_i46101_3_; } public void loadTexture(IResourceManager manager) throws IOException { try ( IResource iresource = manager.getResource(this.textureLocation); NativeImage baseElytra = NativeImage.read(iresource.getInputStream()); NativeImage overlaidElytra = new NativeImage(baseElytra.getWidth(), baseElytra.getHeight(), false); ) { overlaidElytra.copyImageData(baseElytra); for (int i = 0; i < 17 && i < this.listTextures.size() && i < this.listDyeColors.size(); ++i) { String bannerTextureRL = this.listTextures.get(i); if (bannerTextureRL != null) { try ( NativeImage bannerLayer = net.minecraftforge.client.MinecraftForgeClient.getImageLayer(new ResourceLocation(bannerTextureRL), manager); ) { int bannerLayerColour = this.listDyeColors.get(i).getSwappedColorValue(); if (bannerLayer.getWidth() == overlaidElytra.getWidth() && bannerLayer.getHeight() == overlaidElytra.getHeight()) { for (int height = 0; height < bannerLayer.getHeight(); ++height) { for (int width = 0; width < bannerLayer.getWidth(); ++width) { int alphaBanner = bannerLayer.getPixelRGBA(width, height) & 0xff; // extract the red channel, could have used green or blue also. int alphaElytra = baseElytra.getPixelLuminanceOrAlpha(width, height) & 0xff; // algorithm is: // if elytra pixel is transparent, do nothing // otherwise: // the banner blend layer is a greyscale which is converted to a transparency: // blend the banner's colour into elytra pixel using the banner blend transparency if (alphaElytra != 0 && alphaBanner != 0) { int elytraPixelRGBA = baseElytra.getPixelRGBA(width, height); int multipliedColorRGB = MathHelper.multiplyColor(elytraPixelRGBA, bannerLayerColour) & 0xFFFFFF; int multipliedColorRGBA = multipliedColorRGB | (alphaBanner << 24); overlaidElytra.blendPixel(width, height, multipliedColorRGBA); } } } } } } } TextureUtil.prepareImage(this.getGlTextureId(), overlaidElytra.getWidth(), overlaidElytra.getHeight()); GlStateManager.pixelTransfer(GL11.GL_ALPHA_BIAS, 0.0F); overlaidElytra.uploadTextureSub(0, 0, 0, false); } catch (IOException ioexception) { LOGGER.error("Couldn't load layered color mask image", (Throwable) ioexception); } } } -
Rendering block and layering it with a texture. 1.15.2
TheGreyGhost replied to AntonBespoiasov's topic in Modder Support
Hi You might get some clues from this project - it uses a 'camouflage cube' which copies the model + textures from adjacent blocks. https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe04_block_dynamic_block_models -TGG -
Howdy A couple of things - it looks like you have multiple faces one on top of the other which is leading to 'z-fighting', https://en.wikipedia.org/wiki/Z-fighting - the 'recommended' version of forge has a bug where the vertex order of faces in obj files doesn't match what vanilla expects for ambient occlusion and leads to strange lighting. Try latest instead of recommended. -TGG
-
Howdy You could look at the source for the Dynamic Lights mod and update it yourself to 1.15.2. Lighting hasn't changed much between the two, although from memory the mod does poke around fairly deeply in the vanilla code so you might need to bend your brain around it for a while. -TGG
-
Hi I'm not sure I understand your question but here's an example of using a .obj model in minecraft https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe21_tileentityrenderer -TGG
-
[1.15.2] Problems rendering a block
TheGreyGhost replied to xX_deadbush_Xx's topic in Modder Support
Hi BTW your translucent texture should only have the up face, and none of the others on that element. If it renders translucent in item but not block, it's almost certainly a renderlayer problem. Are you sure the setRenderLayer is being executed and the RITUAL_STONE is correct? What happens if you swap the order of translucent and solid? It shouldn't matter, but it might show up a subtle problem in your json. You could consider simplifying your model to two simple elements then putting a breakpoint into MultiLayerModel.Loader to see whether your json file is being parsed as you expect You could try putting a breakpoint into isSolidOrTranslucent and seeing if it's called when you place your block into the world; alternatively in ChunkRenderDispatcher::compile with a conditional breakpoint, or in MultiLayerModel::getQuads to see whether the quads are being rendered (and you just can't see them). Painful trial and error was the only way that worked for me unfortunately -TGG -
[1.15.2] Problems rendering a block
TheGreyGhost replied to xX_deadbush_Xx's topic in Modder Support
Try this: // does the Glass Lantern render in the given layer (RenderType) - used as Predicate<RenderType> lambda for setRenderLayer public static boolean isGlassLanternValidLayer(RenderType layerToCheck) { return layerToCheck == RenderType.getCutout() || layerToCheck == RenderType.getTranslucent(); } -TGG -
[1.15.2] Strange behavior with non cube blocks.
TheGreyGhost replied to ken2020's topic in Modder Support
Howdy What's happening is that minecraft thinks the bottom face of your block occupies the entire bottom face of the cube, so it doesn't need to draw the blocks underneath your block. You need to either 1) set the VoxelShape for your block correctly; For example see here: https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe02_block_partial or alternatively 2) give your block the notSolid() property -TGG- 1 reply
-
- 1
-
-
[1.15.2] Problems rendering a block
TheGreyGhost replied to xX_deadbush_Xx's topic in Modder Support
Howdy Yeah it could be related to that. It is possible to render one quad translucent and the rest solid using a forge multilayer block. You could look at this working example here: https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe05_block_advanced_models -TGG -
[1.15.2] Item Property Override with both texture and model
TheGreyGhost replied to VirtCraft's topic in Modder Support
Howdy You could create a custom model that creates a model on the fly (or combines existing model) based on the itemstack properties. There is an example of how this works for blocks; it can be adapted to items fairly easily https://github.com/TheGreyGhost/MinecraftByExample (see mbe04). You could also consider using an ItemStackTileEntityRenderer (see Item.Properties.setISTER) -TGG -
1.15.2 Mod Loads over and over again after Finishing Forge
TheGreyGhost replied to Link__95's topic in Modder Support
Hi Due to a bug in Forge, when there is an error loading client resources, it cycles infinitely Examples of cases where this happens: A mod resource failed loading, such as a particle texture list A registry entry is registered twice, causing an exception java.util.concurrent.CompletionException: java.lang.RuntimeException: One of more entry values did not copy to the correct id. Check log for details! An uncaught exception shows up when a mod is loading a custom resource https://github.com/MinecraftForge/MinecraftForge/issues/6593 -TGG -
[1.15.2] OutOfBounds Exception When Opening Container
TheGreyGhost replied to Paperclippy's topic in Modder Support
Howdy This is a working example of containers with a lot of explanation in it, you might find it helpful https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe30_inventory_basic -TGG -
[Solved][1.15.2]Custom command always return error
TheGreyGhost replied to kyazuki's topic in Modder Support
Howdy The syntax of commands is tricky to get right. This working example might help explain it https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe45_commands -TGG -
[1.14.4] Custom elytra layer not rendering transparency
TheGreyGhost replied to ReconCubed's topic in Modder Support
Hmm ok If you put your code into a github repository I'll download it in the next few days and have a look, if you like. Cheers TGG