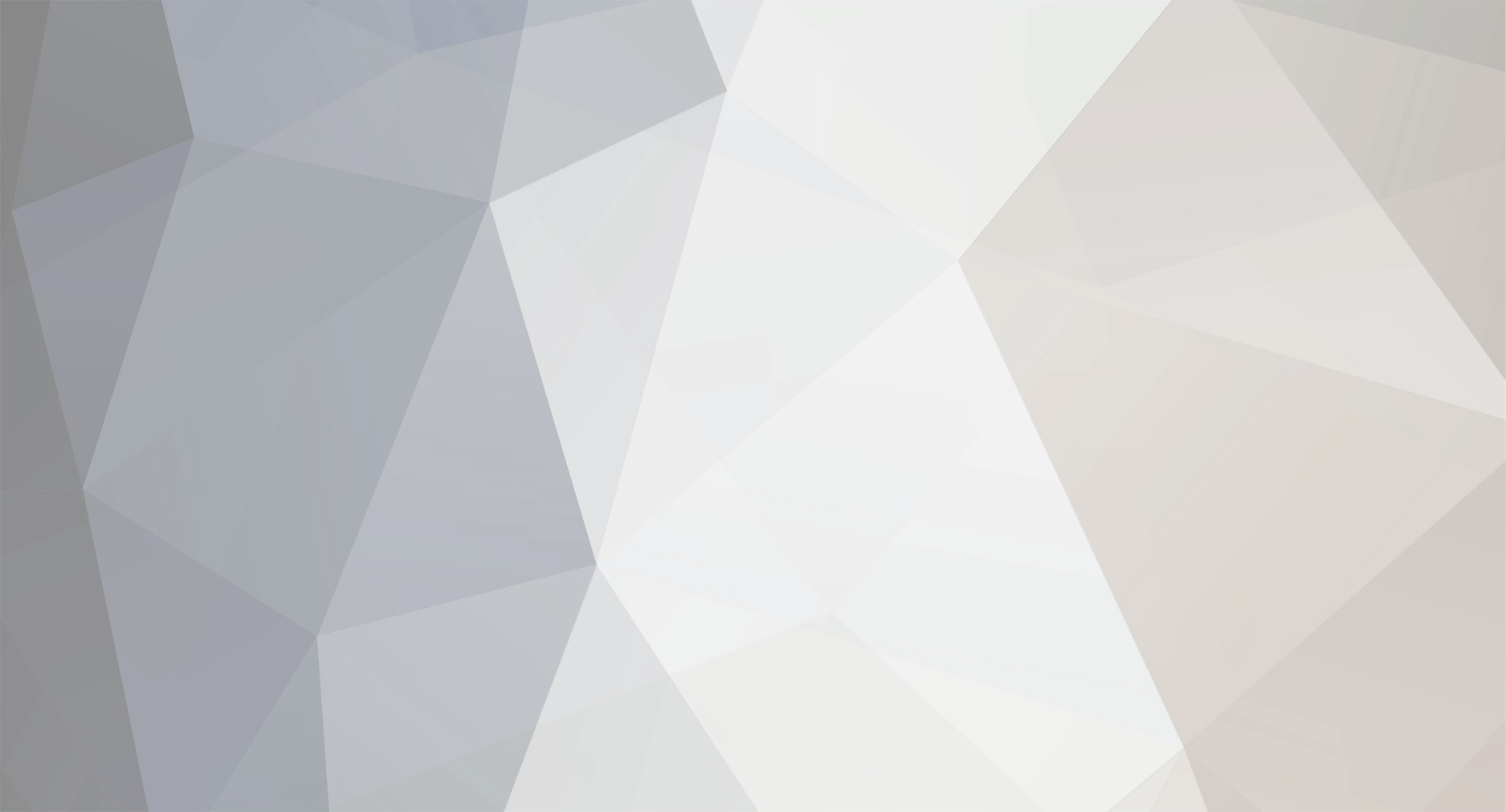
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
(1.15.1)[Solved] Baked Model is too dark
TheGreyGhost replied to PianoManu's topic in Modder Support
Howdy I don't see you put any lightmap information into your vertex, but I presume you are using the Block vertex format? In my previous digging through the rendering code, I sometime found that to be important. DefaultVertexFormats.BLOCK // IVertexBuilder::addQuad and FaceBakery; see also DefaultVertexFormats.BLOCK. // Summary: // faceData[i + 0] = Float.floatToRawIntBits(positionIn.getX()); // faceData[i + 1] = Float.floatToRawIntBits(positionIn.getY()); // faceData[i + 2] = Float.floatToRawIntBits(positionIn.getZ()); // faceData[i + 3] = shadeColor; // faceData[i + 4] = Float.floatToRawIntBits(textureU)); // faceData[i + 5] = Float.floatToRawIntBits(textureV)); // faceData[i + 6] = baked lighting (blocklight + skylight) // faceData[i + 7] = normal; You could consider using the face bakery to generate your quads, like this /** * Returns a quad for the given digit * @param digit the digit (0 -> 9) * @param isBlank if true: this digit should be blank (is a leading zero) * @param minX: the minimum [x,y,z] of the digit quad (from the viewer's point of view). units = model space i.e. 0->16 is 1 metre block * @param maxX: the maximum [x,y,z] of the digit quad (from the viewer's point of view). units = model space i.e. 0->16 is 1 metre block * @return */ private BakedQuad getQuadForDigit(int digit, boolean isBlank, Direction whichFace, double minX, double minY, double minZ, double maxX, double maxY, double maxZ) { // generate a BakedQuad for the given digit // we can do this manually by providing a list of vertex data, or we can use the FaceBakery::bakeQuads method // FaceBakery::bakeQuad is much simpler and suitable for pretty much any block-style rendering, so I've used that here // If you want to manually provide vertex data yourself, the format is an array of ints; look in // IVertexBuilder::addQuad and FaceBakery; see also DefaultVertexFormats.BLOCK. // Summary: // faceData[i + 0] = Float.floatToRawIntBits(positionIn.getX()); // faceData[i + 1] = Float.floatToRawIntBits(positionIn.getY()); // faceData[i + 2] = Float.floatToRawIntBits(positionIn.getZ()); // faceData[i + 3] = shadeColor; // faceData[i + 4] = Float.floatToRawIntBits(textureU)); // faceData[i + 5] = Float.floatToRawIntBits(textureV)); // faceData[i + 6] = baked lighting (blocklight + skylight) // faceData[i + 7] = normal; // When constructing a face manually in this way, the order of vertices is very important! // 1) must be added anti-clockwise (from the point of view of the person looking at the face). Otherwise the face // will point in the wrong direction and it may be invisible (backs of faces are usually culled for block rendering) // 2) ambient occlusion (a block lighting effect) assumes that the vertices are added in the order: // top left, then bottom left, then bottom right, then top right - for the east, west, north, south faces. // for the top face: NW, SW, SE, NE. for the bottom face: SW, NW, NE, SE // If your face has ambient occlusion enabled, and the order is wrong, then the shading will be messed up // FaceBakery: // Vanilla uses it to convert from the elements in a block model, i.e. // "elements": [ // { "from": [ 7, 0, 7 ], // "to": [ 9, 10, 9 ], // "shade": false, // "faces": { // "down": { "uv": [ 7, 13, 9, 15 ], "texture": "#torch" }, // "up": { "uv": [ 7, 6, 9, 8 ], "texture": "#torch" } // } // }, // see https://minecraft.gamepedia.com/Model#Block_models // In order to use the FaceBakery::bakeQuad method, we need to provide: // 1) A suitable cuboid 'from' and 'to', in model coordinate (eg the full 1 metre cube is from [0,0,0] to [16, 16, 16]) // 2) the corresponding [u,v] texture coordinates for the face: [minU,minV] first then [maxU,maxV], again in texels 0->16 // 3) the face we want to make the quad for (eg up, down, east, west, etc). Vector3f from = new Vector3f((float)minX, (float)minY, (float)minZ); Vector3f to = new Vector3f((float)maxX, (float)maxY, (float)maxZ); // texture UV order is important! i.e. [minU,minV] first then [maxU,maxV] float [] uvArray = getDigitUVs(digit, isBlank); final int ROTATION_NONE = 0; BlockFaceUV blockFaceUV = new BlockFaceUV(uvArray, ROTATION_NONE); final Direction NO_FACE_CULLING = null; final int TINT_INDEX_NONE = -1; // used for tintable blocks such as grass, which make a call to BlockColors to change their rendering colour. -1 for not tintable. final String DUMMY_TEXTURE_NAME = ""; // texture name is only needed for loading from json files; not needed here BlockPartFace blockPartFace = new BlockPartFace(NO_FACE_CULLING, TINT_INDEX_NONE, DUMMY_TEXTURE_NAME, blockFaceUV); // we have previously registered digitsTexture in StartupClientOnly::onTextureStitchEvent AtlasTexture blocksStitchedTextures = ModelLoader.instance().getSpriteMap().getAtlasTexture(AtlasTexture.LOCATION_BLOCKS_TEXTURE); TextureAtlasSprite digitsTextures = blocksStitchedTextures.getSprite(digitsTextureRL); final IModelTransform NO_TRANSFORMATION = IDENTITY; final BlockPartRotation DEFAULT_ROTATION = null; // rotate based on the face direction final boolean APPLY_SHADING = true; final ResourceLocation DUMMY_RL = new ResourceLocation("dummy_name"); // used for error message only BakedQuad bakedQuad = faceBakery.bakeQuad(from, to, blockPartFace, digitsTextures, whichFace, NO_TRANSFORMATION, DEFAULT_ROTATION, APPLY_SHADING, DUMMY_RL); return bakedQuad; } If that doesn't help, I'd suggest you add a breakpoint to ForgeBlockModelRenderer::renderModelSmooth (or much easier - ::renderModelFlat if you turn off ambient occlusion) and watch how your block's quads are rendered to the buffer. I've also had this problem arise previously with blocks which used the skylight+blocklight value for rendering but which had a calculated skylight+blocklight of 0 due to the block's settings (I forget which, sorry). The breakpoint I suggested above should show that, if it's the cause. -TGG -
Let sword in forge 1.15.2 render as sword not as item
TheGreyGhost replied to Joshi234's topic in Modder Support
Howdy Probably your item model doesn't have "builtin/entity" as the parent type. (see the "Item" section of https://minecraft.gamepedia.com/Model)? If that doesn't work, I'd suggest trying some breakpoints in strategic locations, eg: - inside your lambda for supplying the ItemStackTileEntityRenderer - inside the render method of vanilla ItemStackTileEntityRenderer - inside ItemRenderer::renderItem(ItemStack itemStackIn ....) However - based on your screenshots it doesn't look like you need an ISTER to render the sword. You can just use a vanilla icon (item/generated) and set the "display" parameters correctly in your item -i.e. the rotation, scaling, translation. If your icon looks similar to the sword icon, then try copying the vanilla sword item display parameters into yours. -TGG -
[1.15.2] "Using missing texture, unable to load" my texture
TheGreyGhost replied to WDNinja's topic in Modder Support
Howdy For what it's worth, here is an example of a mtl, obj, and json which work together correctly (all are in models\block) # Blender MTL File: 'mbe21_ter_gem.blend' # Material Count: 1 newmtl Material Ns 323.999994 Ka 1.000000 1.000000 1.000000 Kd 0.800000 0.800000 0.800000 Ks 0.500000 0.500000 0.500000 Ke 0.0 0.0 0.0 Ni 1.450000 d 1.000000 illum 2 map_Kd minecraftbyexample:model/mbe21_ter_gem # Blender v2.80 (sub 75) OBJ File: 'mbe21_ter_gem.blend' # www.blender.org mtllib mbe21_ter_gem.mtl o Gem v 0.000000 1.000000 0.000000 v -0.500000 0.000000 -0.500000 v 0.500000 0.000000 -0.500000 v 0.500000 -0.000000 0.500000 v -0.500000 -0.000000 0.500000 v 0.000000 -1.000000 -0.000000 vt 0.125000 0.000000 vt 0.000000 0.500000 vt 0.250000 0.500000 vt 0.375000 0.000000 vt 0.250000 0.500000 vt 0.500000 0.500000 vt 0.625000 0.000000 vt 0.500000 0.500000 vt 0.750000 0.500000 vt 0.875000 0.000000 vt 0.750000 0.500000 vt 1.000000 0.500000 vt 0.125000 1.000000 vt 0.000000 0.500000 vt 0.250000 0.500000 vt 0.375000 1.000000 vt 0.250000 0.500000 vt 0.500000 0.500000 vt 0.625000 1.000000 vt 0.500000 0.500000 vt 0.750000 0.500000 vt 0.875000 1.000000 vt 0.750000 0.500000 vt 1.000000 0.500000 vn 0.0000 0.4472 -0.8944 vn 0.8944 0.4472 0.0000 vn 0.0000 0.4472 0.8944 vn -0.8944 0.4472 0.0000 vn 0.0000 -0.4472 -0.8944 vn 0.8944 -0.4472 -0.0000 vn 0.0000 -0.4472 0.8944 vn -0.8944 -0.4472 -0.0000 usemtl Material s 1 f 1/1/1 3/2/1 2/3/1 f 1/4/2 4/5/2 3/6/2 f 1/7/3 5/8/3 4/9/3 f 1/10/4 2/11/4 5/12/4 f 6/13/5 2/14/5 3/15/5 f 6/16/6 3/17/6 4/18/6 f 6/19/7 4/20/7 5/21/7 f 6/22/8 5/23/8 2/24/8 { "loader": "forge:obj", "flip-v": true, "ambientToFullbright": false, "__comment": "flip-v may be required if your texture appears mirrored. See OBJloader::read() for other flags. currently the available options are", "__comment1": "detectCullableFaces (default true) = try to convert faces to Directional Quads (EAST, WEST, etc) instead of just general quads", "__comment2": "diffuseLighting (default false) = attempt to apply the direction-dependent lighting as per vanilla blocks. Currently does nothing.", "__comment3": "flipV (default false) = mirror the texture sheet top-->bottom (if your textures appear upside-down)", "__comment4": "ambientToFullbright (default true) = always render at maximum world illumination (combinedLight) regardless of the actual skylight or blocklight present", "__comment5": "materialLibraryOverrideLocation (default null) = use this path/filename for the material library file .mtl", "model" : "minecraftbyexample:models/block/mbe21_ter_gem.obj" } with a texture file mbe21_ter_gem.png in textures\model -
[1.15.2] Block Rendering based on TileEntity Inventory’s ItemStack
TheGreyGhost replied to Razzokk's topic in Modder Support
Hi A TileEntityRenderer is definitely the best way to go about this one. The renderer can ask the tileentity what is in its inventory, and alter the rendering based on that. You might find this tutorial project useful, it has a few working examples of TileEntityRenderers https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe21_tileentityrenderer -TGG -
How can I update my mod from an earlier version.
TheGreyGhost replied to Stanlyhalo's topic in Modder Support
These links are really useful for updating from 1.12.2 to 1.15.2 https://gist.github.com/williewillus/353c872bcf1a6ace9921189f6100d09a https://gist.github.com/williewillus/30d7e3f775fe93c503bddf054ef3f93e You might find this example project helpful too. https://github.com/TheGreyGhost/MinecraftByExample -TGG -
Sure, just spawn the particles along the line between the two points i.e. if your two points are [x1,y1,z1] and [x2,y2,z3], and you want them evenly spaced at 10% intervals, then spawn at [x1*0.9 + x2*0.1, y1*0.9 + y2 * 0.1, z1 * 0.9 + z2 * 0.1] and [x1*0.8 + x2*0.2, y1*0.8 + y2 * 0.2, z1 * 0.8 + z2 * 0.2] etc This tutorial project gives a working example of how to spawn custom particles; there are a few tricks about setting the initial velocity correctly and removing random variation from the initial position. https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe50_particle -TGG
-
Howdy Well that code doesn't look much like Java to me so I can't really help you with that. Except to suggest that ResourceLocation("minecraft") doesn't contain your registryName parameter. This working example of tags and recipes that use tags might be helpful, perhaps. https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe35_recipes -TGG
-
1) Short answer: yes you do. I find Excel useful for this (but that's because I use Excel a lot) 2) Not-quite-so-short answer - try out the vanilla DataGenerators to automatically generate all the recipes you need https://github.com/Vazkii/Botania/blob/master/src/main/java/vazkii/botania/data/DataGenerators.java and https://github.com/Vazkii/Botania/tree/master/src/generated 3) somewhat longer answer - Write your own custom SpecialRecipe code to generate the appropriate type of sword from a given set of inputs
-
FYI https://github.com/MinecraftForge/MinecraftForge/issues/6593
-
[1.15.2] Help rendering semi-transparent textures?
TheGreyGhost replied to SapphireSky's topic in Modder Support
Hmmm I wonder if that is sometime to do with ForgeIngameGUI? (It replaces IngameGUI) Possible causes I think: 1) alpha test is not enabled; or 2) alpha test threshold is not right (i.e. the "transparent" texels in your texture have an alpha value that is above the alpha test threshold and blending is off) I suggest you put a breakpoint into the start of renderGameOverlay and trace through step by step to see why alpha test is not enabled or the threshold is not right. -TGG -
Howdy This was a bug in an earlier version of Forge, they have fixed it in latest (at least I think so). If there's an error when loading a resource pack (i.e. your mod resources), it aborts the load but then tries again in an infinite loop. -TGG
-
Hi ... by digging into the Chunk generation code to understand how it works World generation is very complicated, I'd suggest starting at ServerChunkProvider as an entry point, but to be honest there are so many lambdas, futures and registers in there that I think it will be difficult to trace through. If you find a good technical reference somewhere, please let me know!! -TGG
- 1 reply
-
- 1
-
-
Hi Vanilla already has raytrace coded for you. No need to rewrite. Actually depending on the gameplay mechanics you want, you could just just use the player's look vec instead. i.e. if you are looking mostly down, your effect spreads out sideways even if you hit the side of the block instead of the top. The Direction class has methods to figure out which direction the player is facing based on the lookvec. -TGG
-
Howdy To be honest I'm really not sure what gameplay effect you're trying to achieve. Could you explain further? eg If player hits the block from the top or the bottom, then the blocks to the east, west, north, and south are also broken If player hits the block on the west face, then the blocks to the north, south, up, and down are also broken etc I don't understand what you mean "null case"? You mean that no direction is defined? I'm surprised by that if you're subscribing to LeftClickBlock. If it's not working, you might be able to figure out which face is being clicked by Raytracing where the player is looking. Try ToolCommons.rayTraceFromEntity. -TGG
-
Howdy THere's a problem with your block model (either the blockstate has the wrong path, or your fancyblock.json is in the wrong place) java.io.FileNotFoundException: mytutorial:models/block/fancyblock.json WHat's causing the crash though is this Caused by: java.lang.IllegalStateException: not enough data at net.minecraftforge.client.model.pipeline.UnpackedBakedQuad$Builder.build(UnpackedBakedQuad.java:175) ~[forge-1.14.4-28.2.16_mapped_stable_58-1.14.4-recomp.jar:?] at com.mekelaina.mytutorial.blocks.bakedmodels.FancyBakedModel.createQuad(FancyBakedModel.java:77) ~[main/:?] I think the error means that your block model is trying to construct quads using a different vertex format than expected. Some of the formats are longer (require more ints) than others. You might find some of the example code in this project useful, it also dynamically generates quads. https://github.com/TheGreyGhost/MinecraftByExample see mbe04 (altimeter), mbe15, mbe21 (the RenderQuads) -TGG
-
[1.15.2] Help with aggressive mobs (creating a swim boi)
TheGreyGhost replied to BadBichShaquonda's topic in Modder Support
Hi I haven't done it in 1.15.2 yet; in previous versions the AI was called from the entity's tick code, so all I needed to do was check if the key was being held down (CAPS LOCK I think from memory) and if so, just skip over the call to the AI code. You can stop the entity updating entirely by doing the same trick at the start of the entire tick() method eg MobEntity::updateEntityActionState looks like it might be where AI is controlled from, with updateAITasks() doing most of the work -TGG -
[1.15.2] Help with aggressive mobs (creating a swim boi)
TheGreyGhost replied to BadBichShaquonda's topic in Modder Support
Howdy In my experience, the Minecraft AI is difficult. You really need to invest the time to understand exactly what it's doing otherwise random stuff will just happen for no reason. I've found it very helpful to add logging code (print to console) into the AI code (even copying the vanilla classes out) to let you study what's going on without disrupting it using breakpoints. Likewise adding a switch in your code (eg triggered by pressing the control key) to 'freeze' the AI and stop it updating so you can breakpoint and inspect all the variables. It's also very helpful to strip the AI right back to one task and then slowly add them back (or add new) one at a time. I've also found it useful to add a spawn inhibitor which prevents all entities from spawning except your own entity, so there are no other AI tasks running except for your own (makes breakpoints much easier) eg like this https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/usefultools/debugging/DebugSpawnInhibitor.java -TGG -
Howdy You've got two choices i know of... 1) search for the source for the mod (many are open source .. often on github) 2) Download IntelliJ IDEA (it's free for hobbyist use) - it has an inbuilt decompiler called FernFlower which will automatically show you the decompiled code if it can't find the actual source. It's not as good as the original source of course, but is often good enough to identify what algorithms they're using for parts of interest. Personally I'd recommend you stick to tutorials at the start, it's hard enough to learn the intricacies of Minecraft and Forge with well-documented code and someone explaining it as you go, let alone trying to reverse engineer the logic from things which the author didn't write to be easy to understand. -TGG
-
Howdy You have a couple of options; if you just want to generate a flat "pizza box" model with your texture, you can use any of the methods in these example classes https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe04_block_dynamic_block_models/AltimeterBakedModel.java https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe21_tileentityrenderer/RenderQuads.java or BlockModel::bakeVanilla If you want to generate an item model with 'holes' in it, similar to vanillla, then look at ItemModelGenerator to see how vanilla does it or ItemLayerModel forge extension for further clues. Haven't done that myself.... -TGG
-
[1.14.4] missing id from the world Fixed minecraft:item id mismatch
TheGreyGhost replied to frakier's topic in Modder Support
When I get this type of error it's normally a sign that I've changed my code (eg added a new item) and re-used an old save. As a rule I always generate a new save after making significant code changes, I've been bitten a couple of times by weird behaviour that looks like a bug in my code but was actually just because I was re-using an old save. -TGG -
[1.15.2] Smoothly interpolating Power Generation
TheGreyGhost replied to Ahndrek Li'Cyri's topic in Modder Support
Howdy The basic algorithm for doing things like this is to add a different amount each tick. For example, if you wanted to add 30 power units over 20 ticks, you would add 2 units on the first tick 1 unit on the second tick 2 units on the third tick 1 unit on the fourth tick (etc) for 20 ticks in total. You need to track the fractional part yourself eg from your example INITIALISING: float ratePerTick = 2500.0/1600.0; float remainder = 0.0; EVERY TICK: remainder += ratePerTick; int powerToAddThisTick = (int)remainder; remainder -= powerToAddThisTick; addPower(powerToAddThisTick) -TGG -
I cant quite understand what is wrong with my code here.
TheGreyGhost replied to Hatsonboats58's topic in Modder Support
Looks like the hard way to me.... recipe json are very simple to write. -
[1.15.1] TER how to change texture with bindTexture
TheGreyGhost replied to MagicQuest's topic in Modder Support
Hi If you are rendering your own quads - you can specify it in the render buffer like this https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe21_tileentityrenderer/RenderQuads.java Is that what you mean? -TGG -
Detect Lightning Bolt impact with block
TheGreyGhost replied to dagobertdu94's topic in Modder Support
Howdy I think a Lightning bolt doesn't really behave like other entities do, it's more a placeholder and doesn't perform collision detection in the same way. I think the best you can probably do is to detect when it's spawned using (eg) EntityConstructing event, then look at its x,y,z to find the block that it is spawning at. -TGG