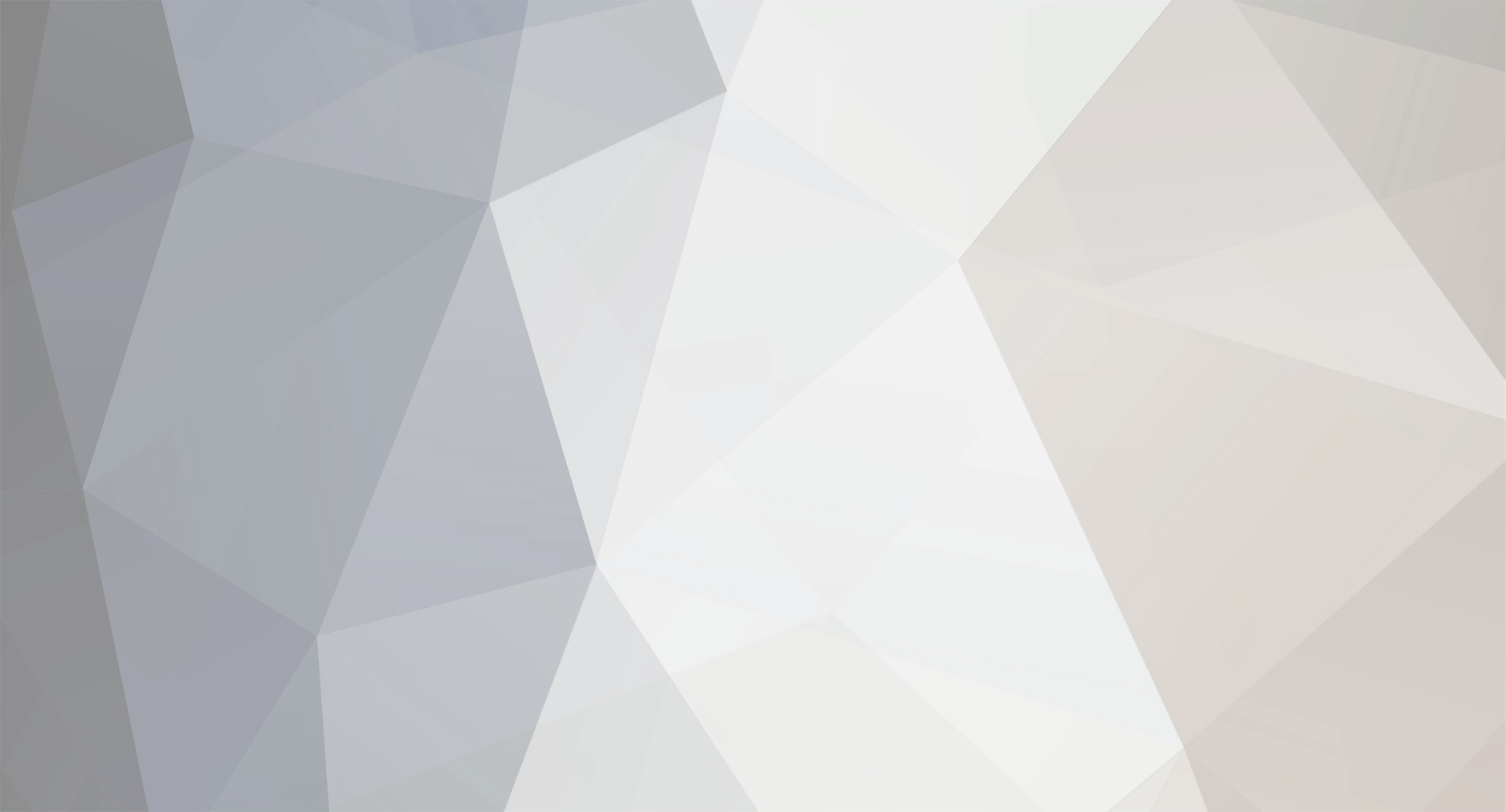
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi In IntelliJ, the minecraft source can still be found by opening the ForgeSrc library. I'm sure it's the same in Eclipse. Likewise, the "Find class x" shortcut (ctrl-N IntelliJ, ctrl-shift-T in Eclipse) still finds library classes. -TGG
-
No OpenGL context found in the current thread
TheGreyGhost replied to charsmud's topic in Modder Support
Hi If all you really want to do is copy/backup some files, you can force the server to stop saving to disk for a short time. See the save-off command. I think your current approach of relaunching the server thread from within the server thread is asking for trouble. Perhaps you could describe in more detail what you're trying to achieve by moving files around? -TGG -
Hi These links have some background info that might help you understand what's going on. http://greyminecraftcoder.blogspot.com.au/2013/08/rendering-items.html and http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html and http://greyminecraftcoder.blogspot.com.au/2013/09/custom-item-rendering-using.html -TGG
-
Hi For some clues look in TileEntityBeacon.updateState() --> Block.isBeaconBase() has been thoughtfully added by Forge folks exactly for this purpose, i.e. override it for your own block(s). -TGG
-
Hi >How does minecraft do it (leaf blocks)? Not sure what you mean? Leaf blocks don't overwrite existing blocks, only empty space. If you just want your autobuild to overwrite air only, it's easy. Get the block using world.getBlock, check block.isAirBlock(x,y,z), and only overwrite if it's air. If you want your autobuild to overwrite (eg) naturally generated stone, but not stone placed by a player, then that is possible but very complicated (and almost certainly not worth the trouble) -TGG
-
Hi Hmm yeah there are a few things wrong in that code So what you really want to do is rotate your stair before you place it, not after. Some thoughts- - the rotation of the stairs is determined by the metadata - the initial rotation of the stairs when you place them is determined in onBlockPlacedBy - see the setBlockMetadataWithNotify - your Horizontal stair probably doesn't need to override any of the stair methods except onBlockPlacedBy - storing the "rotation to be used when I place the block" in a static field_rotated is a bad idea because it won't work on multiplayer - your keypresses are on the client, but the block placement is on the server which has a different copy of HorizontalStair (ie might be on a different computer). Unfortunately you will probably need to use packets to overcome this problem - i.e. the client needs to tell the server how many times the rotate has been pressed. This link might help understand metadata, if you don't already http://greyminecraftcoder.blogspot.com.au/2013/07/blocks.html This link will help with user input and how it is sent to the server. It's for 1.6.4 but hasn't changed much. http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.html It's possible there's an easier way than packets for this, but if so I'm not sure what it is. If you can intercept the "Place a block" packet sent from the client to the server (Packet15Place in 1.6.4) you can change the direction parameter there; if you trace through the vanilla code you should be able to track that down (but it will take a fair bit of effort I think ) -TGG
-
[1.7.2] Texture name from NBT. Edit: Updating texture.
TheGreyGhost replied to Ernio's topic in Modder Support
Hi vanilla automatically synchronises ItemStack NBT information between the client and the server. The server keeps the master copy (saves to disk in the save game files), the client keeps a copy in RAM. This is true regardless of whether you're running SP or MP, because SP runs its own internal server (IntegratedServer). So the idea would be: generate the image on the server, store it in the itemstack NBT (it gets saved to disk on the server) and the server will automatically send it to the client for you, where the client can render it. You don't need to save it to disk if you take the information from the NBT and create a texture from it directly (as in SimpleTexture) -TGG -
Having a little trouble with the first person rendering
TheGreyGhost replied to Minothor's topic in Modder Support
Hi The effect of GL11.glTranslate depends on how the caller has set things up. Sometimes (eg with GUI) the x, y are the left/right and up/down on the screen, with z being the depth. Sometimes, they are aligned with the east/west, up/down, north/south in the world. Sometimes they're aligned with an object - for example for items held in the player's hand, or the map, or similar. In case you're not up to speed with OpenGL yet, this book is very useful for coming to grips with OpenGL (although it's a bit hard to follow in places) http://www.glprogramming.com/red/ The part on transformations is here http://www.glprogramming.com/red/chapter03.html I have to admit I often struggle to get the transformation right if it's not something simple. I usually wind up with trial and error of a combination of rotate and translate to get a mental picture of how the axes are sitting relative to what I want, and then figuring out what the correct rotate and translate should be. -TGG -
Hi Some info on the tessellator here http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html Alpha blending in minecraft is usually done using GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); Your image will need to have alpha channels, or optionally you can set its alpha using eg GL11.glColor4f(red, green, blue, alpha); -TGG
-
No OpenGL context found in the current thread
TheGreyGhost replied to charsmud's topic in Modder Support
Hi You're calling client side code (including OpenGL) from the server side, which is an extremely bad idea because it will cause random crashes. You must make sure that your server-side code doesn't run any client-side code. Perhaps you could explain what exactly you want to run on the server side; certainly not the GUI? -TGG -
Hi Yes it is possible. Personally I would use an ISimpleBlockRenderingHandler (a google on that keyword should get you plenty of tutorials) and render twice, first the underlying block then the tranparent. However apparently it is also possible to do it using just vanilla pass 0 and pass 1, I've never done that personally. Some more info here: http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-transparent-blocks.html -TGG
-
Could be... show your code? -TGG
-
[1.7.2] Texture name from NBT. Edit: Updating texture.
TheGreyGhost replied to Ernio's topic in Modder Support
Hi If your textures truly are unique for every item, why not just store the texture itself in the NBT and avoid the temporary file problem? a 16x16 pixel 32 bit texture is only 1K which is nothing. See SimpleTexture.java for ideas on how to convert a BufferedImage stream to a minecraft texture you can bind and use for a custom IIconRenderer. -TGG -
Hi try these methods under Block:: -TGG /** * Rotate the block. For vanilla blocks this rotates around the axis passed in (generally, it should be the "face" that was hit). * Note: for mod blocks, this is up to the block and modder to decide. It is not mandated that it be a rotation around the * face, but could be a rotation to orient *to* that face, or a visiting of possible rotations. * The method should return true if the rotation was successful though. * * @param worldObj The world * @param x X position * @param y Y position * @param z Z position * @param axis The axis to rotate around * @return True if the rotation was successful, False if the rotation failed, or is not possible */ public boolean rotateBlock(World worldObj, int x, int y, int z, ForgeDirection axis) { return RotationHelper.rotateVanillaBlock(this, worldObj, x, y, z, axis); } /** * Get the rotations that can apply to the block at the specified coordinates. Null means no rotations are possible. * Note, this is up to the block to decide. It may not be accurate or representative. * @param worldObj The world * @param x X position * @param y Y position * @param z Z position * @return An array of valid axes to rotate around, or null for none or unknown */ public ForgeDirection[] getValidRotations(World worldObj, int x, int y, int z) { return RotationHelper.getValidVanillaBlockRotations(this); }
-
Having a little trouble with the first person rendering
TheGreyGhost replied to Minothor's topic in Modder Support
No worries FYI partialTick is only there to help make your animations smoother. Minecraft ticks 20 times per second but can render many more frames per second than that. Let's say your renderer is called 80 times per second. First call: (tick = 0) , partialTick = 0.0 Second call: (tick = 0) , partialTick = 0.25 Third call: (tick = 0) , partialTick = 0.50 Fourth call: (tick = 0) , partialTick = 0.75 Fifth call: (tick = 1) , partialTick = 0.0 etc. So if you don't have it, just choose a number and stick with it. The animations / moving hands / objects etc (if any) will be a bit jerkier but that's all. -TGG -
Hi If you know OpenGL already, try ISimpleBlockRenderingHandler, or alternatively a Pass 1 render. Some more information here http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html especially http://greyminecraftcoder.blogspot.com.au/2013/07/block-rendering.html and http://greyminecraftcoder.blogspot.com/2013/07/rendering-transparent-blocks.html -TGG
-
Having a little trouble with the first person rendering
TheGreyGhost replied to Minothor's topic in Modder Support
Hi Unless I've misunderstood what you're trying to do, you will need to call renderItemInFirstPerson from your IItemRenderer.renderItem method, because nothing else in vanilla or forge will call it directly. eg @Override public void renderItem(ItemRenderType type, ItemStack item, Object... data) { // TODO Auto-generated method stub renderItemInFirstPerson(0.0F); } -TGG -
How to disable rendering for some sides from specified blocks
TheGreyGhost replied to rafradek's topic in Modder Support
Hi There are lots of ways to render blocks without modifying RenderBlock. Block.getBlockTexture, ISimpleBlockRenderingHandler, TileEntitySpecialRenderer. This link might help. See the Block Rendering sections in particular. http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html A quick google on those keywords should also bring up plenty of tutorials. Why do you want to store the "dont render this side" information in separate arrays, rather than in metadata or in TileEntity? -TGG -
Having a little trouble with the first person rendering
TheGreyGhost replied to Minothor's topic in Modder Support
Hi I'm not sure I understand how your code is supposed to work. How are you expecting renderItemInFirstPerson to be called? This link on Rendering Items might help, see the sections under Item Rendering and especially the 'Using IItemRenderer' http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html -TGG -
Custom Rendered Block Not Saving Direction
TheGreyGhost replied to Mottbro's topic in Modder Support
Hi Check out TileEntityNote for a simple vanilla example. The missing super.writeToNBT(par1NBTTagCompound); and super.readFromNBT(par1NBTTagCompound); is probably the problem I imagine. -TGG -
How to make a block light up the area around it?
TheGreyGhost replied to Toastrackenigma's topic in Modder Support
Hi I don't think there is any way to make a block brighter than 1.0F unless you modify the vanilla lighting code which is a very big task. Some background info on lighting here in case you are interested. http://greyminecraftcoder.blogspot.com.au/2013/08/lighting.html -TGG -
Hi This link has a bit more info on Proxy that might help further. http://greyminecraftcoder.blogspot.com/2013/11/how-forge-starts-up-your-code.html -TGG
-
[SOLVED] Trying to make IMessageHandler more generic
TheGreyGhost replied to coolAlias's topic in Modder Support
Life gets in the way, huh how inconvenient... I've into the problem before that a Server-Side class which refers to Client Side classes cause a runtime error before a single line of code gets executed. I don't know much about Java runtime linking but it seems that the first use of the Server-side class makes the jvm look for all the other classes referred to by the Server-Side class. Those classes don't exist so it gives a runtime error. Doesn't make any difference that the client-side classes are unreachable when the Server-side methods are called. Could be I've misinterpreted the cause of the errors I was getting, but in the end I fixed it by moving all references to client-side classes into the proxy class. It was quite painful actually. -TGG -
Monitor packet sending to avoid overburdening network?
TheGreyGhost posted a topic in Modder Support
Hi guys Has anyone found a good way to monitor the amount of network traffic to avoid overburdening the network with packets? I'm writing a mod which needs to send a lot of packets in the background. If I send too many at once, it overloads the network and slows down everything for other players. But if I go too conservative it takes an overly long time to send all the packets. What I'm really looking for is a way to monitor how much network traffic there is and throttle my packet sending accordingly. I have a couple of ideas from looking through the code (still 1.6.4), but nothing great. Anyone found a successful method for this before? -TGG