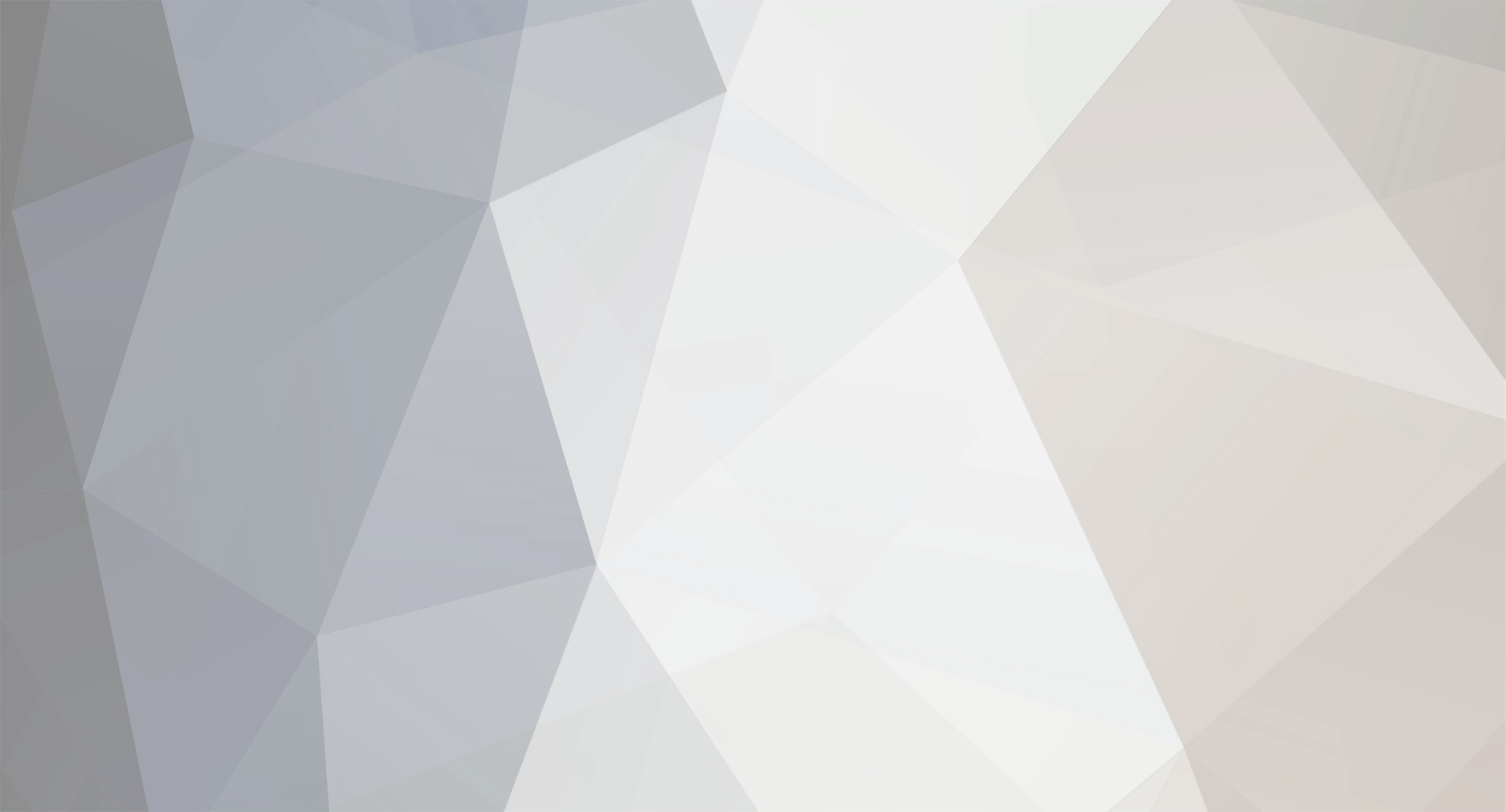
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
It's not final in Forge 965 for 1.6.4, but you're updating to 1.7.2 aren't you? I wonder why they made it final... being able to change the amount of damage seemed to me to be the main point of using LivingHurtEvent.
-
The TileEntity is created automatically when the block is placed. There is no 'getEntityData' for TileEntity, but you can get the TE from the world and set class fields accordingly, which will then be saved/loaded if you wrote your TE correctly. You're on the right track: par3World.setBlock(par4, par5 + 1, par6, this.blockType); --par1ItemStack.stackSize; TileEntity te = par3World.getBlockTileEntity(par4, par5+1, par6); // you've got the tile entity, now just make sure it's yours and do whatever you want with it: if (te instanceof YourTileEntityClass) { YourTileEntityClass myTile = (YourTileEntityClass) te; // now you can use your tile entity class methods: myTile.setSomeField(someValue); // now some field = some value, which will save and load with the tile entity } return true;
-
event.ammount += 2.0F; // adds one heart of damage Or you could give the player an AttributeModifier for attackDamage if you want to permanently (or even temporarily, but that's more of a hassle) allow the player to inflict more damage.
-
Are you sure the crash is caused by trying to open the gui and not by anything going on in your DataHelper class? Try putting println's between each of your lines and see which ones print before the game crashes; that will at least let you know if it truly is the gui that's causing the crash. EntityPlayer p = (EntityPlayer) event.entity; System.out.println("DataHelper pre-load"); DataHelper.load(event.world); System.out.println("DataHelper post-load"); if(DataHelper.getRace(p.getDisplayName()) == -1) { System.out.println("Opening race gui"); p.openGui(RPG.instance, 1, p.worldObj, 0, 0, 0); } Something like that. If it is, you may want to use the player's position when opening the gui and see if that makes any difference: p.openGui(RPG.instance, 1, p.worldObj, p.posX, p.posY, p.posZ);
-
[1.6.4] Generating after vanilla structures?
coolAlias replied to coolAlias's topic in Modder Support
Using PopulateChunkEvent.Populate event, posted to TERRAIN_GEN_BUS, and generating only if the event type == EventType.ICE partially works. ICE generates after both LAVA and DUNGEON, which were both responsible for some of the structures breaking; however, this still doesn't fire after Mineshafts, Temples, etc. generate, so those are still able to break the structures, and not all other EventTypes are reliably posted for every chunk. Is there not a way using the generation Events available to generate after Mineshafts and Temples? -
Yet another method is to use a language file to store your descriptions and make sure each item has the same number of lines of lore: // just to note, this is in one of my classes that has 5+ different items using it, each with different lore @Override @SideOnly(Side.CLIENT) public void addInformation(ItemStack stack, EntityPlayer player, List list, boolean isHeld) { list.add(EnumChatFormatting.ITALIC + StatCollector.translateToLocal("tooltip.zss." + getUnlocalizedName().substring(9) + ".desc.0")); } I use substring(9) because I prefix my items with 'zss.' + 'item.'; anyway, so my language file (named 'en_US' and located in 'assets/lang') would look like this: tooltip.zss.some_item.desc.0=Some item's lore tooltip.zss.some_other_item.desc.0=Some other item's lore Obviously you can add as many fields as you want, desc.1, desc.2, etc. and just add them all in the tooltip. Mew's suggestion is easier, but if you plan on adding language support, then you'll need to do this anyway. I'm sure someone with better Java than me could figure out a way to support each item containing different numbers of lore lines as well, but I just standardized all my items.
-
[1.6.4] How do I add armor piercing to my weapon?
coolAlias replied to zhangh's topic in Modder Support
The way I did it was to create a new static DamageSource class: public static class DamageSourceArmorBreak extends EntityDamageSource { public DamageSourceArmorBreak(String name, Entity entity) { super(name, entity); setDamageBypassesArmor(); } } Then I listen for the LivingHurtEvent, check if the source of the damage is a player using, in this case, a special skill (substitute your weapon), and then damage the entity with my new damage source instead. DirtyEntityAccessor.damageEntity((EntityLivingBase) event.entity, DamageUtils.causeArmorBreakDamage(player), event.ammount); event.ammount = 0.0F; // set to 0 and/or cancel the event so entity not damaged twice DirtyEntityAccessor is just a class that I placed in the net.minecraft.entity folder that allows me to call the protected 'damageEntity' method: public class DirtyEntityAccessor { public static void damageEntity(EntityLivingBase target, DamageSource source, float amount) { target.damageEntity(source, amount); } } Thanks again to diesieben07 for his help with that particular solution. -
Firstly, if it's working when you play normally but not when you restart, then you need to make sure that your entity is reading and writing all the proper data from / to NBT. Secondly, since you are changing things from within a GUI, you need to send packets informing the server of each change so the correct data can be stored.
-
If you're using an Entity, you can always just use entity.ticksExisted for an easy, built-in tick counter. That's what I usually do.
-
[1.6.4] Generating after vanilla structures?
coolAlias replied to coolAlias's topic in Modder Support
This is the code I'm using with PopulateChunkEvent.Post, but the vanilla structures still seem to generate after this event fires because they will generate through my structures. Here is an image of what I mean when I say the vanilla structures are cutting through mine - those sandstone blocks are part of a desert temple, and that chest is mine that should have been inside a hollow stone cube. @ForgeSubscribe public void postPopulate(PopulateChunkEvent.Post event) { // I tried printing a simple message here when using PopulateChunkEvent.Populate to no avail // but the Post event performs as expected switch(event.world.provider.dimensionId) { case -1: // the Nether netherRoomGen.generate(event.chunkProvider, event.world, event.rand, event.chunkX, event.chunkZ); netherBossGen.generate(event.chunkProvider, event.world, event.rand, event.chunkX, event.chunkZ); break; case 0: // the Overworld secretRoomGen.generate(event.chunkProvider, event.world, event.rand, event.chunkX, event.chunkZ); bossRoomGen.generate(event.chunkProvider, event.world, event.rand, event.chunkX, event.chunkZ); break; default: break; } } -
I didn't mean to be rude about it, but you've got a lot of wonky stuff going on in your code. For instance, when you get the TileEntityChest, it is already an IInventory, meaning you should have access right away; why are you doing all this other weird stuff getting the block and then getting the block's inventory, when you already have it? If you're absolutely 100% sure that the IInventory you want is at zCoord+1, that's fine, but it seems off to me. This code is in a TileEntity already, right? But you don't want this TileEntity's inventory, you want a different one? Ok. Still: TileEntity te = worldObj.getBlockTileEntity(xCoord, yCoord, zCoord + 1); // going along with you here if (te instanceof IInventory) { // now you've got an inventory IInventory inv = (IInventory) te; for (int i = 0; i < inv.getSizeInventory(); ++i) { // etc. } } Should be as simple as that. If it's not working, try putting println's all over the place, if you haven't already, to make sure it's getting called and that the variables are what you think they are.
-
Well then can you please post your real code, because it's impossible to tell what's going on as it is in your post. Why zCoord + 1? Where are these coordinates from? What method is this code being run in? The information you posted is not enough.
-
For one, you are using different coordinates to retrieve the tile entity than you do when you retrieve the inventory if it's a chest. Where are you getting xCoord/yCoord/zCoord from, and where are you getting xChest/yChest/zChest coordinates from, and why are you using two different coordinate sets?
-
[1.6.4] [SOLVED] KeyBinding multiplayer problem
coolAlias replied to Jacky2611's topic in Modder Support
Use PacketDispatcher.sendPacketToServer(whatever Packet you made goes here); -
[1.6.4] [SOLVED] KeyBinding multiplayer problem
coolAlias replied to Jacky2611's topic in Modder Support
You DO have the player, that's what they've been trying to tell you: EntityPlayer player = Minecraft.getMinecraft().thePlayer; Minecraft.getMinecraft() returns the client instance of Minecraft, which always has a reference to the client side player and world objects. But that's a moot point: when sending packets to the server, you don't need an instance of the player because the server already knows from whom it came... -
Tile Entites, GUIs and player-entered information
coolAlias replied to Flenix's topic in Modder Support
Climbing the Interface Ladder covers this kind of stuff pretty well, if you can spare the time to watch the whole thing. -
Why don't you try it and see? coolboy4531's post broke it down pretty well, so you should notice that the y position is the height at which your ore will spawn. You are using rand.nextInt(40), which returns a value between 0 and 39, meaning your ore will be in those layers of the world, and those layers only. Look deeper if you're not finding it.
-
Help with making a block that generates in the sky
coolAlias replied to MINERGUY67880's topic in Modder Support
First you will need to understand basic Java, then you will immediately understand why you are getting errors with par1World, etc. Second, you can't just add any method you want and expect it to run automatically. Sure, there is a method named updateTick in Block, but you don't have any of the arguments. Look in the Block class and you will see: @Override public void updateTick(World world, int x, int y, int z, Random rand) {} That's the correct method signature, and if you deviate at all from that your method is no longer a Block class method, meaning you'd have to call it yourself. Now that you have the correct method signature, you just have to make your block tick randomly or schedule ticks for it. Look at some ticking vanilla blocks to see how they do it. -
Originally I was using the typical IWorldGenerator to generate some structures into the world, but I noticed that vanilla Dungeons, Desert Temples, and the like would generate afterwards, sometimes breaking up my custom structures. I tried using PopulateChunkEvent.Post, but that had exactly the same results as IWorldGenerator: my structures generated fine, but vanilla structures would generate over them occasionally. I saw the Populate event called for different types such as DUNGEON, but there didn't seem to be a way to use this event after the structures have generated. Using DecorateBiomeEvent.Post gave me a runtime exception "java.lang.RuntimeException: Already decorating!!" tracing back to me structure gen code (which generated fine in the other attempts). Not sure what's going on here or if it's even important to my issue. Anyway, my question is: How do I run my structure gen code after vanilla structures generate? Thanks for any suggestions.
-
Just saying that it's not working isn't going to get you anywhere, because we cannot divine what is in your code that's causing it to fail. No code = no help. Simple as that.
-
You also need to make sure your texture has an alpha layer. If you use GIMP, it usually adds one automatically when you create a new file or, if it didn't, you can add one. Once you have an alpha layer, in the 'Layers, Channels, Paths,...' pane (shows up on the right-hand side for me), just above the 'Lock' box there is a slider that says 'Opacity'. Sliding it to the left will make your texture more transparent, which you can then export/save as a .png file. From there you can do what TGG said and use the openGL BLEND methods to render your transparent texture in a custom IItemRenderer. Just be sure to disable blend when you're done.
-
Excellent point. I was just showing that it's possible. Anyway, as Draco said you should just use reflection API or some other method of changing the private xpValue field. If you don't want to do it that way, then your best bet would be what I showed, but use a custom entity that extends xp orb instead so you avoid the nasty recursion when the entity joins the world (unless you are familiar with ASM, then you can do as diesieben pointed out and make a core mod).
-
One way would be to intercept EntityXPOrb when they join the world and spawn a new xp orb in its place: @ForgeSubscribe public void onEntityJoin(EntityJoinWorldEvent event) { if (event.entity instanceof EntityXPOrb) { // first spawn a new xp orb entity with amount of xp you want event.entity.setDead(); // kill the old xp orb } } You don't even have to make a new class, just use the vanilla xp orb's constructor: public EntityXPOrb(World world, double x, double y, double z, int xpAmount); You can see from that how easy this should be to adjust the amount. It doesn't looked like it is capped at any max value other than the limits of Short values.
-
There are lots of things that can be streamlined; most of what I did I did that way on purpose to make it more clear as to what's going on. It's a tutorial, after all, not end-product polished code. You can do it however you want