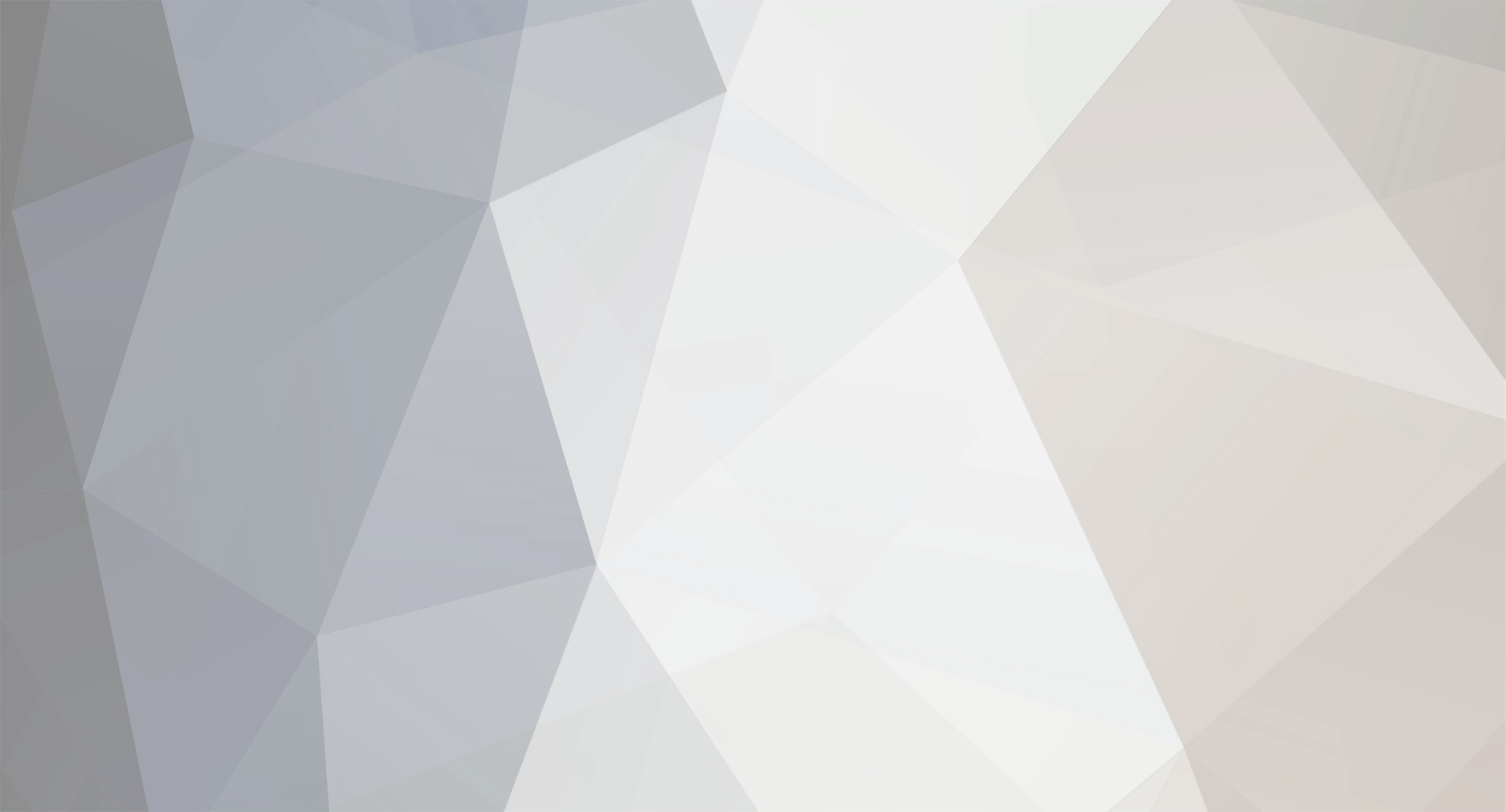
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
I use this method to write Strings as UTF: ByteBufUtils.writeUTF8String(buffer, String);
-
You guys might be interested in taking a look at my Structure Generation API; not exactly the same, but I faced a lot of similar issues such as placing torches, pistons, etc., which I solved by storing them separately and placing them after the rest of the blocks were finished. I was using static arrays, but it would work just the same if you build an array out of the blocks you were going to move, whether a two-dimensional array only for a line of blocks at a time (one array index for the blocks data: id and meta, at the very least), or you could fool around with 4-dimensional arrays like I did ;P
-
As far as the tick handler part of it is concerned, yeah, that's pretty much it. Why don't you try it and see if it works? Why you would name your variables var15 and such doesn't make much sense to me, but that's your call.
-
You can subscribe directly to the various TickEvents, rather than the generic TickEvent ServerTickEvent ClientTickEvent WorldTickEvent PlayerTickEvent RenderTickEvent Those are all the tick events available at this time, that I can find, so you can use: @SubscribeEvent public void onTick(ClientTickEvent event) { // codes }
-
Get the position of a block in the world when it is destroyed
coolAlias replied to Alix_The_Alicorn's topic in Modder Support
Can't you do whatever it is you need to do in the breakBlock method? That gives you both the position and the world object, and is only called when the block is destroyed. If it's about dropping items, just don't drop any items by default, and drop them yourself from within the breakBlock method. -
[SOLVED][1.7.2] Rendering icon in inventory turns completely grey
coolAlias replied to coolAlias's topic in Modder Support
It's really strange, the icons render perfectly fine if any of the preceding slots are highlighted with the mouse, whether or not a damaged stack is preceding, or if a non-damaged stack is preceding, but turns totally grey when a damaged stack is in a preceding slot with no slots or only empty slots in between and no highlighted slots. I've tried manually setting the color and zLevel as well as some other probably useless things, to no avail. Is this perhaps a bug with 1.7.2 or Forge version 1024, or am I doing something stupid here? -
Well you're creating a private Block just within that class, and you never register it to the game's registry... I suggest you follow some of Wuppy's tutorials on making a Block first, before you try to do world generation.
-
Why don't you update to the recommended version 1024?
-
No, it's exactly the same as before with the difference noted above. You just have to create your ArmorMaterial before you initialize your custom armor Item: public static final ArmorMaterial CUSTOM_MATERIAL= EnumHelper.addArmorMaterial("Custom", 5, new int[] {1,3,2,1}, 5); customArmor = new ItemCustomArmor(CUSTOM_MATERIAL, proxy.addArmor("custom"), 0).setUnlocalizedName("customArmor");
-
You need to use the setBlock method: world.setBlock(x, y, z, block, meta, flag); Exactly the same as World.setBlockId from 1.6, but instead of an ID you have a Block instance.
-
Try running gradlew --refresh-dependencies build If that doesn't fix it, then you are probably trying to access your SuperNova class / method on the server side when it is client-side only; at least, if it is client-side only.
-
[1.6.4] NPE which i can't solve myself [Solved]
coolAlias replied to larsgerrits's topic in Modder Support
What's on this line? at larsg310.mods.chemistrycraft.ChemistryCraft.preInit(ChemistryCraft.java:33) -
[1.6.4] NPE which i can't solve myself [Solved]
coolAlias replied to larsgerrits's topic in Modder Support
Line 145 points to the same line as before. Guess I was wrong about the cause, sorry, I don't know what it could be then... One random thing, you may want to put the untranslated name as the key and translate it on the fly, so if the user changes the language once they've started the game, they won't be locked in to the first language's names. -
This is an icon with numbers rendered over the top of it, which renders perfectly fine until there is a damaged ItemStack preceding its position in the inventory, at which point it turns completely grey. Never had this problem in 1.6.4, and after spending some time poking around in the updated rendering code and trying some things I'm no closer to a solution... Here's my IItemRenderer: @SideOnly(Side.CLIENT) public class RenderItemBombBag implements IItemRenderer { /** Defines the zLevel of rendering of item on GUI. */ public float zLevel; public RenderItemBombBag() {} @Override public boolean handleRenderType(ItemStack item, ItemRenderType type) { return type == ItemRenderType.INVENTORY; } @Override public boolean shouldUseRenderHelper(ItemRenderType type, ItemStack item, ItemRendererHelper helper) { return false; } @Override public void renderItem(ItemRenderType type, ItemStack item, Object... data) { if (type == ItemRenderType.INVENTORY) { Tessellator tessellator = Tessellator.instance; for (int i = 0; i < 3; ++i) { IIcon icon = item.getItem().getIcon(item, i); tessellator.startDrawingQuads(); tessellator.addVertexWithUV(0, 16, zLevel, icon.getMinU(), icon.getMaxV()); tessellator.addVertexWithUV(16, 16, zLevel, icon.getMaxU(), icon.getMaxV()); tessellator.addVertexWithUV(16, 0, zLevel, icon.getMaxU(), icon.getMinV()); tessellator.addVertexWithUV(0, 0, zLevel, icon.getMinU(), icon.getMinV()); tessellator.draw(); } } } }
-
[1.6.4] NPE which i can't solve myself [Solved]
coolAlias replied to larsgerrits's topic in Modder Support
No. When you are initializing an Object, what happens? The constructor is called. Look at your constructor. You are trying to access the Object 'this' before it is finished initializing its fields. Just try my suggestion. // just switch them around and see if it doesn't work private Atom(String name, String symbol, int index) { // here is where your fields get initialized: this.name = name; this.symbol = symbol; this.index = index; // here you are already trying to access 'this', which has not yet been initialized atomSymbol.put(name, symbol); atomIndex.put(name, index); atomList.put(name, this); } -
There are still IDs used internally, which you can access as follows for your Map: // getting the ID to put in your Map as a key: Item.getIdFromItem(stack.getItem()) // and getting an Item from an ID, if needed Item.getItemById(int) You can do the same thing with Blocks.
-
Oh, I didn't know that existed! Sweet
-
Don't use the item's damage field to store constantly changing data; use the ItemStack's NBT instead.
-
[1.6.4] NPE which i can't solve myself [Solved]
coolAlias replied to larsgerrits's topic in Modder Support
Well, you haven't finished constructing the Atom yet when you try to put it in the list: private Atom(String name, String symbol, int index) { // here you are already trying to access 'this', which has not yet been initialized atomSymbol.put(name, symbol); atomIndex.put(name, index); atomList.put(name, this); // here is where your fields get initialized: this.name = name; this.symbol = symbol; this.index = index; } Swap the order: initialize first, put in the list second. -
The types are defined in the NBTBase class: public static final String[] NBTTypes = new String[] {"END", "BYTE", "SHORT", "INT", "LONG", "FLOAT", "DOUBLE", "BYTE[]", "STRING", "LIST", "COMPOUND", "INT[]"}; Either count to the position of the type that you need, starting at zero (END is 0, BYTE is 1, etc.), or if you have an NBTBase of any type you can use nbtbase.getId() to return the correct id. @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); // here is use the compound.getId(), but I could just write '10' instead // since I know that I stored NBTTagCompounds in this particular NBTTagList NBTTagList items = compound.getTagList("Items", compound.getId()); // etc.
-
Well every update tick you are telling it to set the damage to 99, so what did you expect to happen?
-
Don't quote me on this, but I don't think Packet250CustomPayload is useful anymore, given the new layout of the network system. It basically requires that you create a custom packet class for each set of data you want to send, as the reading, writing, and processing of the packet is all done internally in the packet class itself now. PacketHandler classes with 20 different handling methods are a thing of the past
-
Yes, just copy and paste this into your TileEntity: @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); } If that's not working for you, did you update your IInventory code to use markDirty() now that onInventoryChanged() has been removed from that interface? You need to use it to ensure the inventory changes are recorded, as far as I can tell.
-
Are you sure it's not saving correctly, or are you trying to use these values on the client side? If you need the values client side, then you must override the getDescriptionPacket and onDataPacket methods: @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); }
-
Did you register your custom packet class? public void initialise() { this.channels = NetworkRegistry.INSTANCE.newChannel(ModInfo.CHANNEL, this); // add this line: registerPackets(); } // and make this method public void registerPackets() { registerPacket(YourCustomPacket1.class); registerPacket(YourCustomPacket2.class); // ... etc. for all your packet classes }