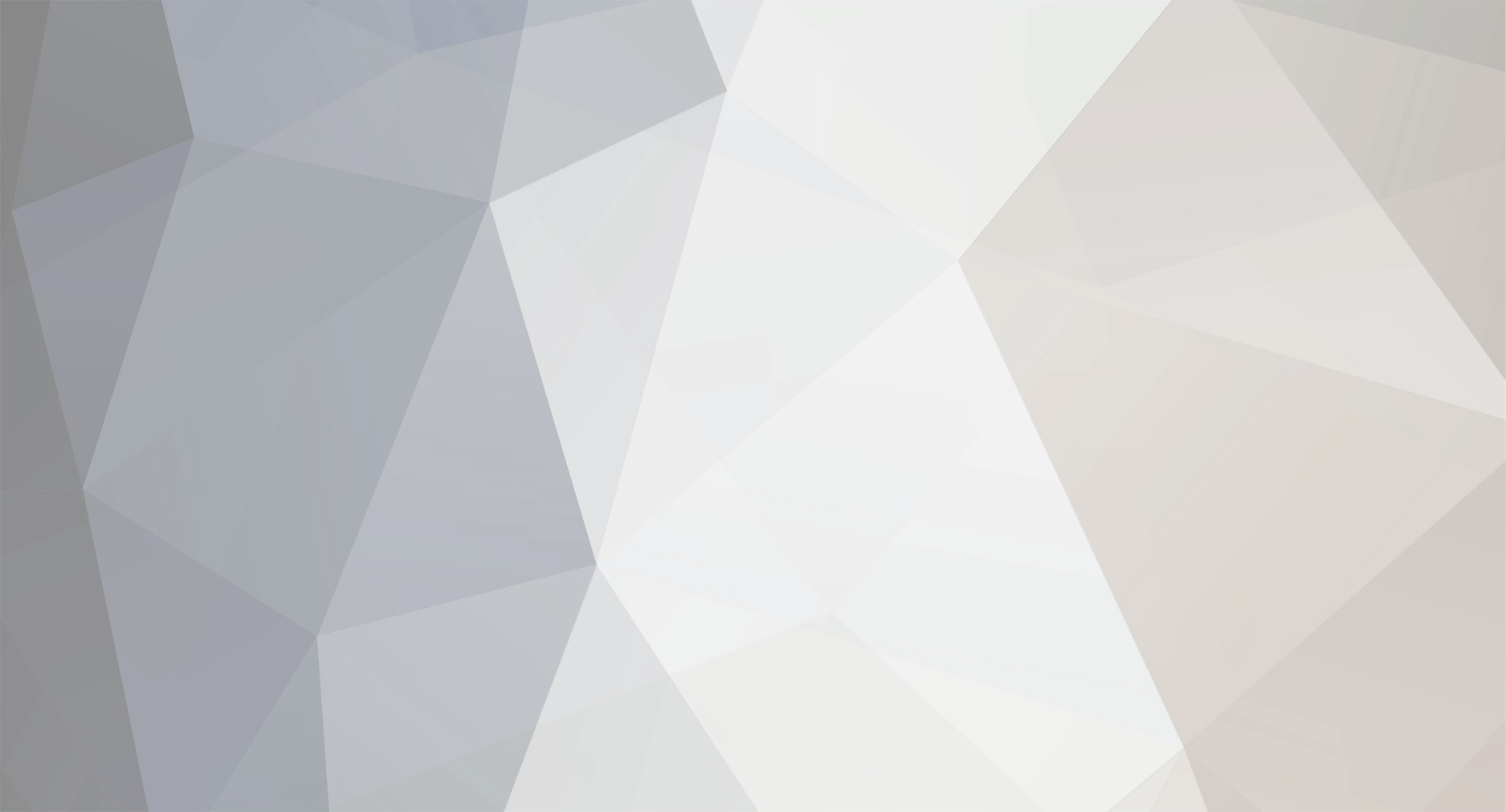
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
I need drawString by List<String> in guiscreen.
coolAlias replied to zlappedx3's topic in Modder Support
Just FYI, the font renderer has a FONT_HEIGHT field, so you don't need to use magic numbers when calculating the y-offset. -
The client handles anything that the player needs to see or interact with, but doesn't keep track of the actual game state - it merely tries to represent the game state. Anything that needs to be remembered or has a real effect in the game, such as health, needs to be handled on the server. The client only needs to know about it if you are going to display it.
-
[1.7.10] Custom EntityThrowable not rendering?
coolAlias replied to IceMetalPunk's topic in Modder Support
Hm, that's weird, then. Can you show your entity registration code? -
[1.8.9] Server Tick Counter Not Working
coolAlias replied to madcrazydrumma's topic in Modder Support
As mentioned, do some basic debugging: put 'System.out.println("Server tick called")' as the first line in your event listener to see if it is actually running, print out the tick counter to see what values it has, etc. Also, if you are going to reset the counter each time it reaches the final duration, use '>=' instead of the modulo '%' operator. There is no point in doing division if the counter simply loops from 1-max over and over again. -
The IDs are arbitrary and must be unique; if you use an already taken ID the conflict will result in a crash, so you must check all super classes to see what DataWatcher IDs they use. It is not recommended to use DataWatcher in things like IExtendedEntityProperties as it is impossible to know what DataWatcher IDs other mod entities use and/or add, but it's fine to use it in your own custom entity classes. As for the Objects that the DataWatcher contains, they can mean anything you want them to mean. An ItemStack, for example, may be the object held by the entity, or it may not be; it all depends on how that particular DW index is used in the code. It could be that it is a piece of armor, or a cape, or any number of other things. The only reason to use DataWatcher, btw, is when you have a value on the server (e.g. the held item) that you need to keep up-to-date on the client, usually because you want to use that value when rendering. If you don't need the value client side, don't use DataWatcher. Also, DataWatcher is just a convenience wrapper around a system that sends packets in the background, so you can easily provide the same functionality by sending a packet yourself and not using DataWatcher at all.
-
Personally, I would just ignore all of that and make your own, but here's how I understand it: - swingProgressInt, swingProgress, and #getSwingProgress are for when the player performs an action that triggers the 'attack' swing animation, having nothing to do with the cyclical swinging motion of the arms, and that attack animation does indeed take about 6 ticks from start to finish; the actual duration is determined by #getArmSwingAnimationEnd - limbSwing and limbSwingAmount, however, are used for the cyclical arm swinging motion, which increases in frequency (i.e. speed) based on the entity's current velocity. They are also used to calculate the values for the first two float parameters passed to #setLivingAnimations and #setRotationAngles, as can be seen in RenderLivingEntity#doRender. I believe limbSwingAmount represents the entity's actual current angle in radians, or a rough approximation thereof, but honestly I have no idea why limbSwing - entity.limbSwingAmount * (1.0F - partialTicks) capped at 1.0F is representative of a time unit, unless they mean 'time' as in progress toward the end of the animation. But even then, it's not the usual way of doing it. Anyway, I wouldn't worry too much about it - the 1st float is basically animation progress (or 'time') and the 2nd float is the target rotation angle (i.e. the 'max'), and you can do whatever you want with those 2 values in combination with all the other values at hand to animate however you like
-
[1.7.10] Custom EntityThrowable not rendering?
coolAlias replied to IceMetalPunk's topic in Modder Support
Make sure your Entity class has a constructor that takes a single World argument: public EntityPotionLifelock(World world) { super(world); } That is the constructor that gets called on the client side to construct the entity for rendering; if it is missing (which it is), then perhaps the client is failing to properly construct your entity and thus it is not able to render. That's just a guess, though, so let us know if that fixes the problem for you -
Well, only the first and last two parameters, in most cases, since the middle bunch are usually the same for similar types of entities. Just one less value to edit, which is always nice, and who doesn't like doing less work, no matter how trivial? Also: death to magic numbers!!! Huzzah. Except for 80, and 3, and the color values... can't win 'em all. EDIT: Oh yeah, and why do we even have to provide an id? Since everyone just increments anyway, and the registered integer ID is not really important for us to know, why not just save everyone the minute amount of trouble and auto-assign it in the background when an entity is registered? That's what I'd do, but I'm sure there are reasons. Oh the tedium lol. Btw, in case anyone can't tell, I don't care one way or the other that we need an ID when registering, or whether people increment a counter or assign manually, but I like to argue pointless things (as I believe some others here may as well)
-
No, ItemStack NBT data only syncs automatically if you set it server side, and only when it is accessed in an inventory using a Container class. NEVER set NBT data (or any other data) client side. Does your TileEntity-based GUI use a Container? If so, as diesieben said you should be able to manually call #detectAndSendChanges, but that's called automatically every update tick from EntityPlayerMP, so you shouldn't have to... I suspect there is probably something else going on in your code; do you have an online repository or other way to view your code? Also, I would not recommend using #setInventorySlotContents when reading your inventory from NBT. Usually this method implementation calls #markDirty() which may or may not iterate through the inventory contents. While you won't crash or anything like that, it's still much better, imo, to just set the inventory slot value directly with array access.
-
How can I figure out what the obfuscated fields are?
coolAlias replied to riderj's topic in Modder Support
Did you try searching? I know you want advice from people that have found good tutorials, but it really isn't hard to find them. The first 2 are the top results for 'learning OpenGL'; the 2nd and 3rd are both ones I had bookmarked and used previously. http://learnopengl.com/ http://www.glprogramming.com/red/index.html https://open.gl/introduction -
How can I figure out what the obfuscated fields are?
coolAlias replied to riderj's topic in Modder Support
The MCP mappings ARE the online resource containing all known 'translations' of the obfuscated names. You can try using a more recent mapping by changing the line in your build.gradle and re-setting up your workspace: mappings = "snapshot_20150617" // change this to today's date, for example, rather than over 6 months ago... Not all mappings are compatible with all versions of Forge / Minecraft, however, though if you are using the latest Forge, the latest mappings should always be usable. I'm not really sure what you mean by different methods of rendering mobs and items - it's all ultimately done using OpenGL, for which there are resources online via Google and resources within Minecraft via the source code. Really, though, the best thing you can do when playing with rendering is to run your mod in debug mode - then you can change most values while it is still running and immediately see the effects. This is priceless for tinkering with rendering code, when you might be changing values in increments of 0.001F or smaller to get them just right. Now imagine trying to do that having to quit and restart every single time. Of course you probably don't have to imagine, as you've probably dealt with that and already know how much it sucks. Debug mode = awesome. EDIT: To answer your question, though - if you want to figure out what a not-yet-clarified field or method does, you have to a. have decent Java skills so that b. you can make sense of what the code is actually doing, thus deciphering what that field or method is for. At that point, you could decide on an appropriate name for it and submit it via MCPBot so everyone can profit from your hard work -
[1.8] [SOLVED] Item added to inventory disappearing on world load
coolAlias replied to AstroEngiSci's topic in Modder Support
Lol, yeah, I'd probably try to reduce the indentation a few levels, for one public boolean onBlockActivated(World world, BlockPos pos, IBlockState state, EntityPlayer player, EnumFacing side, float hitX, float hitY, float hitZ) { TileEntity te = world.getTileEntity(pos); ItemStack stack = player.getHeldItem(); if (!worldIn.isRemote && te instanceof TeleporterTileEntity && stack != null && stack.getItem() == EnderScience.card) { // now we're cookin' with a whole lot less mess // do all your other stuff here } } But that's not really related to your problem... your problem sounds to me like you are managing the inventory from a client-side GUI and expecting it to persist, but the client is like a dream or a ghost - it is completely ephemeral. You need to make sure that any setting you do, be it adding items to inventories or changing NBT data, is done on the server. -
Colliding entity with block java.lang.ClassCastException
coolAlias replied to dwinget2008's topic in Modder Support
Well, the parameter if the method is of type Entity, yet you blindly cast to EntityLiving: } else{ ((EntityLiving)entity).attackEntityFrom(DamageSource.wither, 1.0F); } And, as you can see in the crash report, there are all sorts of other entities that might be colliding with the block: java.lang.ClassCastException: net.minecraft.client.particle.EntityBreakingFX cannot be cast to net.minecraft.entity.EntityLiving Always perform a type check (e.g. instanceof) before casting to a new type. -
ClientTickEvent / KeyInputEvent - check if keyboard.isKeyDown for the attack keybinding (I said the itemUse key before, but that's not the one for breaking blocks). Also be sure to check the mouse using MouseEvent: if (event.button - 100 == mc.gameSettings.keyBindAttack.getKeyCode()) { // mouse button is the attack key, do something } Once you know that the attack key was pressed AND that they are holding your item, you may want to check if they are hitting a block (use Minecraft.getMinecraft().objectMouseOver to easily check). Now that you have a boolean flag for that player, you are still checking each tick if they are holding the attack key down - as soon as it is released, unset the flag / remove the player. It would be much easier using the PlayerInteractEvent via the LEFT_CLICK_BLOCK action, but that, unfortunately, is only fired on the server side.
-
Unfortunately, that method is not used in this context. @OP I think your plan is probably fine. Part of the issue is that the check is happening client side, so you may be fine simply listening for the use-item key / mouse click, checking if it's your item, and adding that player's entityID to a Set. Then in your item's update code, check if the player's entity ID is in that set and if so, don't update the NBT. A Map could work, too, with playerID -> ItemStack and then you could update NBT using the map reference (make a copy of the ItemStack). One thing to watch out for with that, though, is when you copy the data back, if something else happened to change the real ItemStack's NBT in the meantime, those changes might be lost. I would recommend only copying the specific variables that you are tracking instead of replacing the whole NBT tag or ItemStack.
-
[1.8.8] How to make the player's hunger drain faster?
coolAlias replied to HappyKiller1O1's topic in Modder Support
Your tick event is handled in a class, is it not? And classes can have class members, can they not? So make your timer a class member, and simply update it in the tick counter. Or use player.ticksExisted, which is already a timer. -
[1.8.8] How to make the player's hunger drain faster?
coolAlias replied to HappyKiller1O1's topic in Modder Support
Well that depends: how do you want it to work? Either way you'll be doing math, and either way there should be a timer of some sort involved or else the player's hunger will go down pretty darn quick. Btw, why use LivingUpdateEvent when the only ones with hunger are players? This is what PlayerTickEvent is for. -
The reason is very likely what Lex was hinting at: I'm betting you are using your GUI to 'set' the NBT data in your ItemStack, which is not the correct way to do it. Send a packet to the server saying 'player pressed this GUI button' or whatever and let the server determine which coordinates to set in the ItemStack; ItemStack NBT is automatically synchronized from server to client (most other NBT is not, however), so whatever you set on the server will be visible on the client as well.
-
[1.8.8] How to make the player's hunger drain faster?
coolAlias replied to HappyKiller1O1's topic in Modder Support
Kind of. For my own purposes, adding exhaustion was sufficient, as once that reaches 0, hunger begins to deplete, and adding more exhaustion will deplete the hunger bar directly. So if you wanted a faster hunger bar drain, you could add 0.1F exhaustion (or however much) every so often and it would basically have the effect you want. -
Put a break point on CraftingManager line 236 and run your mod in Debugging mode - you'll be able to see exactly what is null. If that doesn't help you, post your code, including your entire pre-init and init routines and any item- or recipe-related methods that are called from them.
-
There is RenderLivingEvent.Pre/Post for this very purpose. Whatever flag(s) or other data you need will have to be sent to all tracking client players, however, so they know to perform the additional rendering.
-
Post your crash log, then.
-
[1.8]Tile Entity storage not synced server side?[SOLVED]
coolAlias replied to Atijaf's topic in Modder Support
That's a prime example of when you need to send data from the server (which is responsible for loading and maintaining the game state) to the client, not the other way around. Client side stuff like GUIs is only for displaying information, not setting it. -
Is there any particular reason you're trying to make it so complicated? 'new Object[] {}' is simply a wrapper automatically added by Java, so the following is perfectly acceptable: GameRegistry.addShapedRecipe(new ItemStack(Content.kingdom_key), "AAA", "ABA", "AAA", 'A', synth, 'B', Content.wooden_key); But yeah, as Choonster pointed out, pretty much the only reason you would be getting an NPE is if you try to register the recipes before all of your items are initialized.
-
[1.8.8] Questions about API creation for a mod
coolAlias replied to HappyKiller1O1's topic in Modder Support
Sorry, it's named "package-info.java"