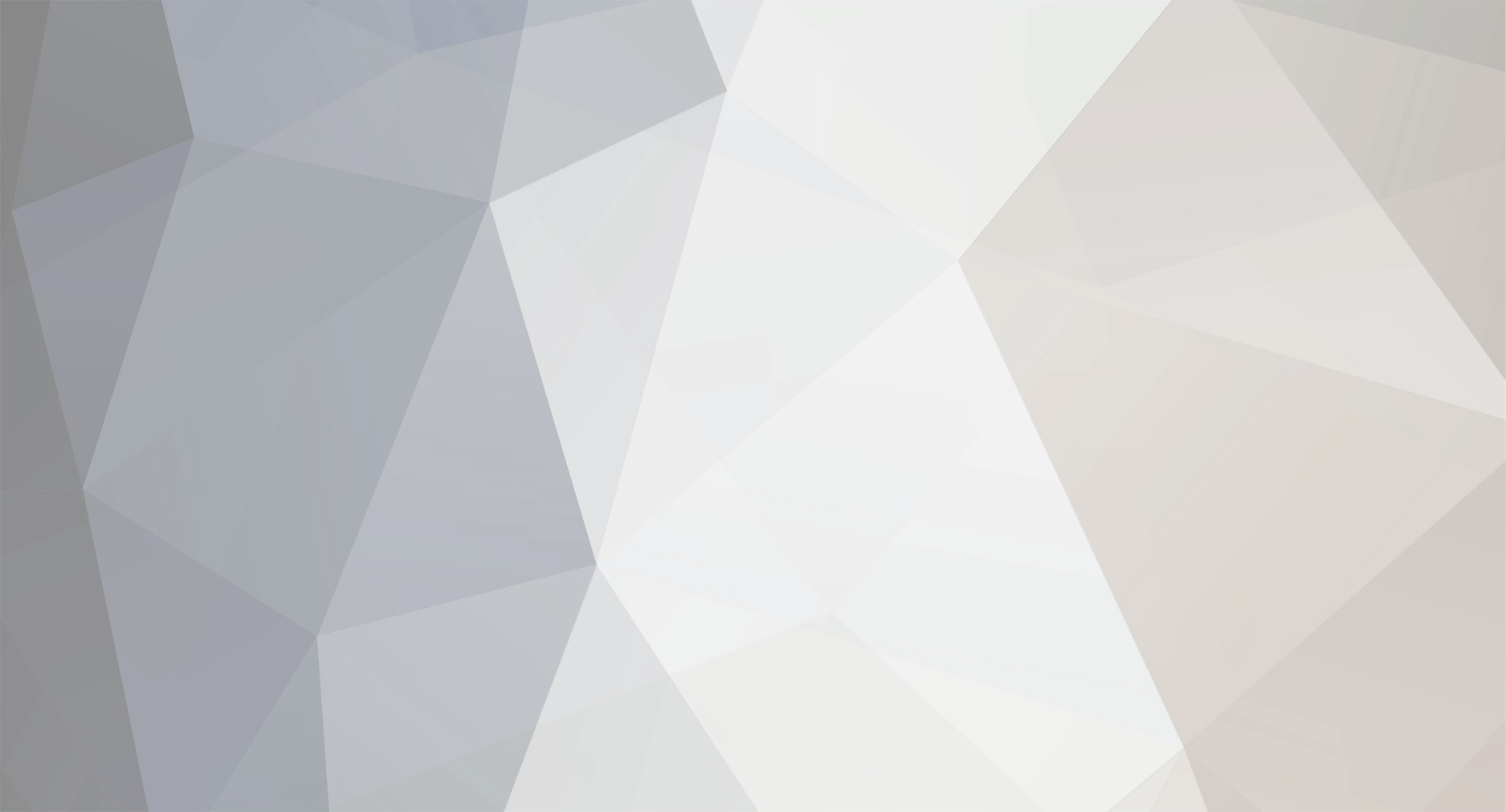
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
How to know what side a block was broke from?
coolAlias replied to American2050's topic in Modder Support
Item#getMovingObjectPositionFromPlayer still uses a ray trace, so if the block is not there, you will find the wrong block. However, you will probably be looking at the same face on the returned block as on the one you just broke, since that block is 'behind' it. Still, it won't be 100% accurate, just as my original solution is not 100% accurate - merely an approximation for when it's not application-critical. If you want 100% accuracy, I suggest you use events like I mentioned earlier. -
How to know what side a block was broke from?
coolAlias replied to American2050's topic in Modder Support
The only way to be completely 100% accurate is to use raytracing, but that is probably not an option because the block is already broken, so the best you can is get a rough approximation which is what that code does. In your case, you are looking down at the block (meaning you hit the top, which is why I suggested using #opposite [or don't use opposite if you are]). Try breaking a block not below your feet and you should get a cardinal direction. If it absolutely MUST be accurate, you are going to have to do some extra work BEFORE the block breaks. Subscribe to PlayerInteractEvent and listen for LEFT_CLICK_BLOCK; from here, figure out the face clicked and store it along with the block position; when the block is broken, check if its position is the last one the player clicked and fetch the side hit from there. -
[1.8.9] Inventory Persistence after Breaking Block [Solved]
coolAlias replied to lynchiem's topic in Modder Support
Write the TileEntity to a new NBT tag compound and then add that compound to the ItemStack that is dropped in #dropBlockAsItemWithChance. Then in #onBlockPlacedBy, you have the ItemStack used to place the block - fetch the NBT tag back and pass it to your TileEntity's #readFromNBT method. -
How to know what side a block was broke from?
coolAlias replied to American2050's topic in Modder Support
@Draco No, it is not immediately overwritten. Look at the code more carefully - it only assigns TOP or BOTTOM if the pitch angle is greater than 45 or less than 45, so that whole 90 degrees in between is given to the cardinal facings. Again, it is what vanilla uses somewhere for placing blocks, and I've used it in my own code with much success. If you want to go with a ray trace or something else, that's probably fine, too, provided the block is still in the world to be traced against. -
[1.8.9] Commands on a 1.8.9 forge mod
coolAlias replied to Elrol_Arrowsend's topic in Modder Support
Nope, hasn't changed one bit. Commands from 1.8 work perfectly fine in 1.8.9. -
How to know what side a block was broke from?
coolAlias replied to American2050's topic in Modder Support
How is that 'only considering the rotation pitch?' Look at the very first line: EnumFacing face = EnumFacing.fromAngle(entity.rotationYaw); -
I did something very similar, but used Reflection to auto-register all my variants, and custom resource locations. (Note that the following code was tested in 1.8, so may not work perfectly anymore - I'll be finding that out soon enough, I expect). Base item interface: Typical implementation of that interface: My model swapper interface: ModelBakeEvent: In my ClientProxy: I think that's about it. Makes the code really nice, imo, when a class is almost entirely self-descriptive; i.e. each class is responsible for itself, rather than expecting random lines of 'support' code all over the place. Here's an example of an Item actually using some of that stuff, and here's a Block. P.S. There are some who disagree with using getUnlocalizedName in the ways that I have above; if you're one of those, feel free to use something else in your own code
-
1563 build failed for task ':retromapReplacedMain'
coolAlias replied to coolAlias's topic in ForgeGradle
This also happens with the recommended 1.8.9 Forge build 1722, even after removing the offending class from the build path. Deleting that package entirely allows the project to build. Has the Forge team decided to explicitly disallow creating a class with a vanilla Minecraft package declaration, or is this a bug? I hope it's a bug, as it's really handy to access protected methods and fields and has worked great up until now. Yes, I know I can 'just use Reflection', but it's much more fun this way EDIT: Btw, the code still works fine while running in Eclipse - it just doesn't want to play nice at compile time. -
How to know what side a block was broke from?
coolAlias replied to American2050's topic in Modder Support
Unfortunately there isn't a direct way, at least none that I am aware of, but you can use the current rotation and pitch of the entity that broke the block to approximate which direction they broke it from: EnumFacing face = EnumFacing.fromAngle(entity.rotationYaw); if (entity.rotationPitch < -45.0F) { face = EnumFacing.UP; } else if (entity.rotationPitch > 45.0F) { face = EnumFacing.DOWN; } That's the same code (or essentially the same) that is used in the placement logic for directional blocks, so you probably need to use the opposite facing instead. -
The render code in your very first post did something... why did you take it all out? Diesieben only said to remove the redundant #doRender methods, not strip out the entire contents of the one method you kept.
-
[1.8.9] Switch contents between inventories on key press
coolAlias replied to LogicTechCorp's topic in Modder Support
Ah, so they are like different blades or something to that effect that the player carries around in a pouch and swaps at will? Or should the upgrades be more like player abilities that they gain when finding / using special items? E.g. after consuming some kind of power-up the player can then channel that power through their scythe? The reason I ask is because the implementation can be very different depending on exactly how you want it to play out. If they really are items that can be swapped in and out of the scythe on the fly, then sure, your idea of the implementation is fine, but if you only decided to use a pouch because you weren't sure how to implement your first idea (whatever that may have been), then I think we can do better. Not knocking on the pouch idea, it just doesn't strike me as very intuitive from an in-game lore point of view (possibly due to not having the full back-story). Anyway, regardless of how you decide to implement it, changing state (server-side) based on a key press (client-side) will require packets - do you have any experience with those? -
[1.8.9] Switch contents between inventories on key press
coolAlias replied to LogicTechCorp's topic in Modder Support
Right, I understood that's how you intend to implement it, but what exactly are you trying to do in terms of the game? I mean, why does the scythe move an item into the pouch? How are they related? For example, in my mod I have several projectile weapons that can each use different ammo which can be selected from the player's current inventory, but instead of storing that item in the projectile weapon, I just store the selected ammo type's index and retrieve it when needed. So, depending on what the relationship of the scythe and pouch is, you may not need to move things, is all I'm trying to say. -
[1.8.9] Switch contents between inventories on key press
coolAlias replied to LogicTechCorp's topic in Modder Support
I would opt for not moving anything at all - why not simply have the scythe hold an integer slot index for the pouch item? Or does the item have to exist in the scythe? Perhaps you could describe the concept in a little more detail? -
[1.8] Big solid door Block of 3x3 x1 size how ??
coolAlias replied to perromercenary00's topic in Modder Support
#getCollisionBoundingBox is the box used to detect collisions with entities. This is the part Fences set to 1.5F. #setBlockBoundsBasedOnState sets the block's 'hit box', i.e. the portion of the block that can be clicked, which is the part you see when hovering over the block with your mouse. For fences, this is still maxed out at 1.0F on the y-axis. -
[1.8] Big solid door Block of 3x3 x1 size how ??
coolAlias replied to perromercenary00's topic in Modder Support
You CAN make bounding boxes bigger than 1x1x1, but, in general, you should not. Fences make the collision bounding box taller than 1 block so that players and mobs cannot jump over them, but the fence, as a block, still restricts itself basically to the 1x1x1 cube, which is why you can place blocks on top of the fence and the top block is still only 1 meter rather than 1.5 meters from the ground. -
So to be clear, the crash happens using the vanilla crafting bench with the recipe you showed? If that's the case, try rolling back to an earlier version of Forge such as 1448 and testing if the crash still occurs there. Some Forge releases occasionally have issues such as this, and testing on a known stable version can at least rule out Forge as the culprit. Personally, I've had the best experience with the 1448 release for 1.7.10, which is why I recommend testing against that. If you still have issues on 1448, post your custom Item class, if there is one, and any other code that may relate to your issue.
-
[1.8] Big solid door Block of 3x3 x1 size how ??
coolAlias replied to perromercenary00's topic in Modder Support
You're already trying to do a hacky solution by making 'impassable air' - why insist on doing it both the hard and wrong way, when it is much easier to simply make real blocks at the positions you want to be real blocks? Sorry, but making a single Block bigger than 1x1x1, in the context of Minecraft, is a terrible idea. The entire Minecraft code is built around the idea that Blocks take up at most a 1x1x1 cube and that within that cube will only be 1 block - messing with that is just asking for trouble. You can make multi-block structures such as a 3x3 door, however, by placing and breaking all the blocks together, as I already mentioned. Vanilla doors and beds already do this - you just need to extend the concept to encompass a slightly larger scale. -
Just FYI, just as you don't need 'new Object[]{}' when adding crafting recipes, you also don't need 'new IProperty[]{}' when creating block states - it's just an artifact from Java that you see as a result of viewing decompiled code. @Override protected BlockState createBlockState() { return new BlockState(this, FACING, OPEN); // this works just fine }
-
[1.8] Big solid door Block of 3x3 x1 size how ??
coolAlias replied to perromercenary00's topic in Modder Support
You are going about it the wrong way. A Block in Minecraft can only be (or SHOULD only be) 1x1x1 maximum size, so if you want a larger structure, you need more blocks - that's why vanilla doors are actually TWO blocks, not one. In your case, you need to coordinate the placing and removal of 9 blocks. -
Hm? Didn't you mean to say "You should NOT set data on the client..." ?
-
That happens because you probably didn't do your renderer registration via your proxy, but did it in your main class or somewhere like that which is loaded on both sides; as a result, the server tries to load the renderer classes, which are all marked @SideOnly(Side.CLIENT), and cannot find them. Any such classes or methods should generally be called through your proxy.
-
I think you are missing a very critical word between 'should' and 'set'...