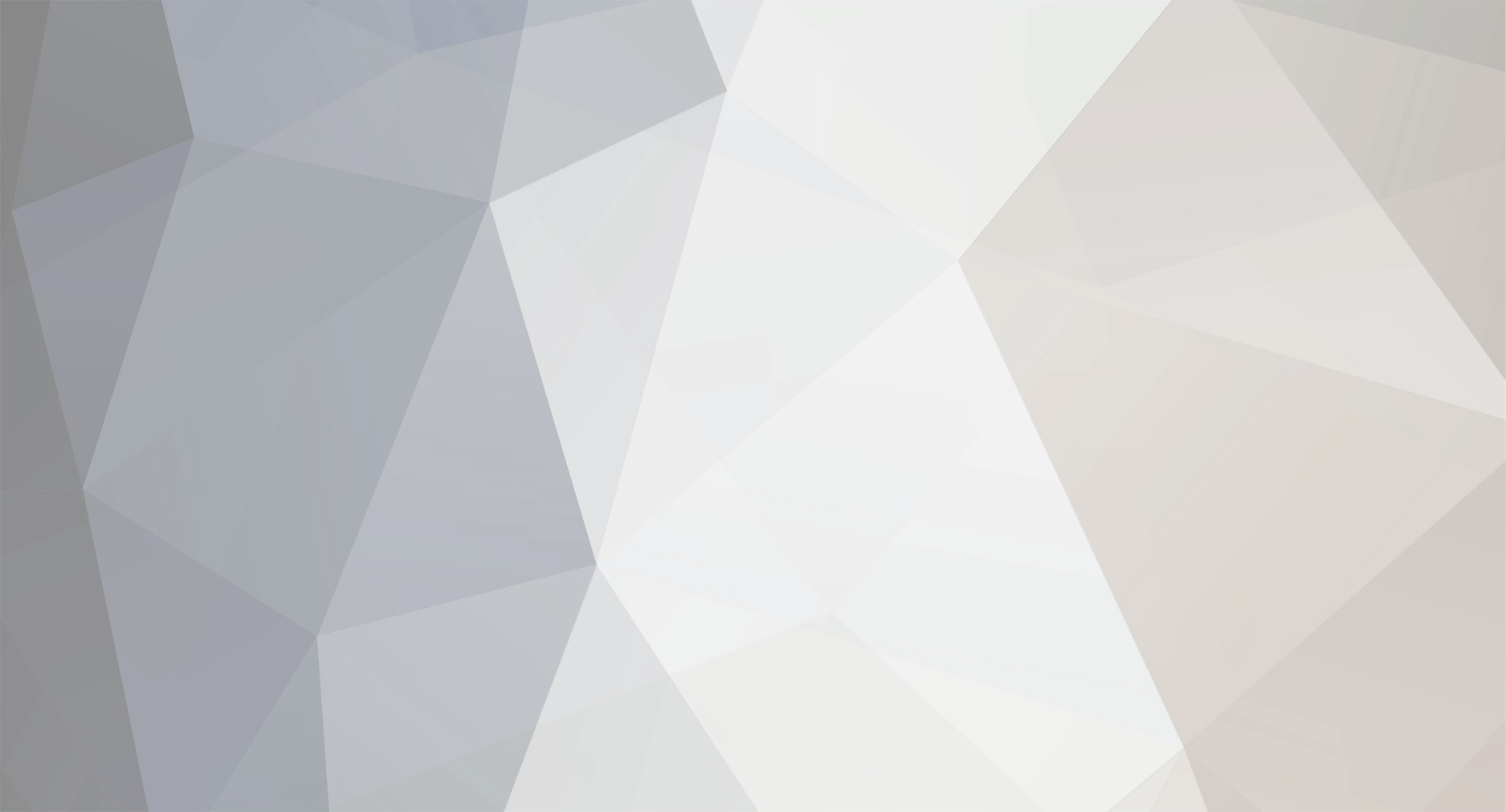
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
If you're really serious about learning OpenGL, check out GLProgramming. Kind of intense, but it will help you make sense of the vanilla rendering code (and then some!).
-
Indeed. Hm. @OP Please post the entire class again with the most recent code. Also, are you sure you registered your entity renderer to use this particular model class? A couple nitpicks: 1. ALWAYS put @Override above inherited methods; even though your method signature looks fine, better safe than sorry (plus it will help if / when you update to the next Minecraft version) 2. Please use standard Java naming conventions; variable names should start with lowercase 'entity' instead of 'Entity' - 'Entity.ticksExisted' looks like a static reference 3. You don't need to cast the entity parameter to access the #ticksExisted field, as it belongs to Entity not your class; unless you need to access fields or methods from your specific class, there is no reason to cast
-
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
It's not disabling the key, it is unsetting the key state so that, as far as the game is concerned, it is not pressed, even when it is. Obviously you would only unset the key state when the player is encumbered, otherwise you would prevent the player from sprinting ever, and that's not the goal. -
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
Well, keyboard input is all handled client-side only, so which do you think would be the better choice? -
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
You should most definitely NOT compare floating point values like that using equality... not to mention that there could be other attribute modifiers affecting the players speed besides yours. If you really want to stop the player from sprinting, the only reliable way to do so is to setSprinting(false) every tick and on the client side unset the sprint key: KeyBinding.setKeyBindState(mc.gameSettings.keyBindSprint.getKeyCode(), false); -
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
Sorry, looks like 1.8 moved the #getFovModifier to AbstractClientPlayer class. Take a look at it and all should become clear. See how they get the entire speed attribute value (including ALL modifiers)? Do the same, but then subtract your custom modifier value (if present) from it before doing the rest of the FOV calculation. -
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
It won't, but you can recalculate it manually based on the same formula as in EntityPlayerSP but removing your custom modifier from the equation, i.e. by subtracting it from the retrieved speed attribute total. -
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
Because you wouldn't be setting it, you would be adding a modifier. -
[SOLVED] [1.8] Something to use instead of DataWatcher
coolAlias replied to TheRedMezek's topic in Modder Support
Easy - #getDataWatcher() is public, after all, so you could add DataWatcher indexes at any point from anywhere, even though it is very unwise to do so. -
[1.8] How to stop the player from sprinting?
coolAlias replied to HappyKiller1O1's topic in Modder Support
You should consider adding AttributeModifiers to the player's speed instead of trying to manage it directly - it will play much better with vanilla / other mods and is also pretty easy. Take a look at the vanilla Sprinting modifier (in one of the Entity classes, maybe EntityLivingBase) and change the bonus to a penalty. -
[1.8] IndexOutOfBoundsException for #transferStackInSlot
coolAlias replied to HappyKiller1O1's topic in Modder Support
Draco is probably right - did you add the armor slots to your container? If so, your inventory size should be 40 and you can merge those slots; if not, you need to remove the ARMOR_START and ARMOR_END indexes and set INV_START to the inventory size. -
[1.8][SOLVED] setInventorySlotContents getting the wrong item
coolAlias replied to HappyKiller1O1's topic in Modder Support
In addition to what Draco said, why not use the 'slot' and 'stack' parameters from the method? Is 'SLOT_BACKPACK' the only slot that will affect weight? If so: if (slot == SLOT_BACKPACK) { // you already have the ItemStack in the slot, it is 'stack': if (stack == null || !(stack.getItem() instanceof ItemBackpack)) { // reset player's carry weight } else { // example only player.carryWeight += ((ItemBackpack) stack.getItem()).getCarryWeight(stack); } } Even better would be to create an interface such as ICarryWeight - then you could have any number of item types that provide carry weight modifiers. Even better than that would be to create a SharedMonsterAttribute for carry weight, then those same items could be in ANY armor or held-item slot (and possibly Baubles slots, haven't worked with it though) and they would affect your carry weight. -
[1.8] Checking if an item is in a custom slot
coolAlias replied to HappyKiller1O1's topic in Modder Support
Yes. Look at the vanilla inventories, e.g. InventoryPlayer, for an example. Always check vanilla - chances are, there is an example. -
[1.8] Checking if an item is in a custom slot
coolAlias replied to HappyKiller1O1's topic in Modder Support
Exactly. Don't do that. -
[1.8] Checking if an item is in a custom slot
coolAlias replied to HappyKiller1O1's topic in Modder Support
It looks like you are calling #setInventorySlotContents when loading the inventory from NBT - you should just set the stack directly: this.inventory[i] = stack; Otherwise, you are forcing some funky stuff to happen: the inventory is stored in the IEEP class for the player, which is loading itself from NBT and asks the inventory to read itself from NBT, too, but that tries to reference the IEEP class as well as do other things like #markDirty which should never happen until the inventory is actually finished creating itself. TL;DR - using #setter type methods when loading from NBT can actually cause bad things to happen -
[1.8] Block giving item back to player, among other things
coolAlias replied to CrazyBorg's topic in Modder Support
FYI, Blocks are singletons, so for YourBlocks.blockBarrel, there is only one instance of that class. This means any variables you try to store, such as 'this.hasWater', will fail utterly and miserably. If you want to have dynamic information about a Block that is unique to that Block, you need a TileEntity*. * Or use blockstates, i.e. metadata, which can store an integer from 0-15, which is enough to store 4 bit flags, but looks like you need at least 5. -
[1.8] Checking if an item is in a custom slot
coolAlias replied to HappyKiller1O1's topic in Modder Support
Override #setInventorySlotContents like I mentioned before, and in your IInventory implementation, store the instance of the player to which it belongs (or a reference to that player's IEEP), i.e. require an EntityPlayer be passed to the constructor and store that instance - then you can access the player any time directly from the inventory, so when the slot contents change, you can fetch the player's IEEP and adjust it. -
Items are singletons, ItemStacks are unique instances. NBT is stored in the ItemStack, so it is unique per instance.
-
[1.8] Checking if an item is in a custom slot
coolAlias replied to HappyKiller1O1's topic in Modder Support
That really depends: what is the point of knowing what item is in there? Are you trying to replicate the vanilla Item code where #onUpdate is called each tick for items in your inventory, or you just want to know if something is in the slot? For the first case, subscribe to PlayerTickEvent and cycle through your player's inventory each tick calling Item#onUpdate for any non-null stacks. For the second case, you could override setStackInSlot in your inventory class and have it notify the player each time something is added or removed, but again, what are you going to do with that? For most use cases, you will probably still need a tick handler to manage it, but it really depends. -
[1.8] Just wondering something about DataWatcher
coolAlias replied to HappyKiller1O1's topic in Modder Support
As diesieben mentioned, there are limited IDs available, so DataWatcher is not recommended for use in something like IEEP of any kind, especially one for players, as there are probably many other mods that unwisely use it in those cases and you will end up with game-breaking conflicts. I suggest you use custom packets to synchronize whatever variables you need available to you on the client; that's all DataWatcher does anyway, is send a packet with the data to the client. "Object" is the base Java class - everything extends from it, so it is the most general type that you could use for something, which is why you can pass int (auot-boxed to Integer), boolean (auto-boxed to Boolean), String, etc. all to the same method. Might want to brush up on those basics -
Entity teleports upwards whenever something collides with it
coolAlias replied to Asweez's topic in Modder Support
You need to call super for entityInit and the read/write NBT methods. Perhaps that will fix your issue, perhaps not, but it certainly is a problem not doing so. -
[SOLVED][1.8][FORGE] Ability to close a gui with a custom key?
coolAlias replied to HappyKiller1O1's topic in Modder Support
KeyInputEvent fires both when the key is pressed and when the key is released; Keyboard#getEventKeyState() will be true if it was pressed, and false if it was released, or you can use the ClientTickEvent as diesieben suggests. And yes, you can put the closing logic in your Gui's #keyTyped method if you want, or in the key handling section. I like to put it in my Gui so that the key doesn't close other screens (you can prevent that by checking the current screen's type, but it's easier to just put it in your Gui). -
[1.8.8] Structure generation generating in wrong Y coordenet
coolAlias replied to KingOfMiners's topic in Modder Support
Well your generateOtherlyworldDungen method uses: int startY = world.getActualHeight() - 1; Why don't you use World#getHeightValue(x, z)? That will give you the actual ground level for those coordinates.