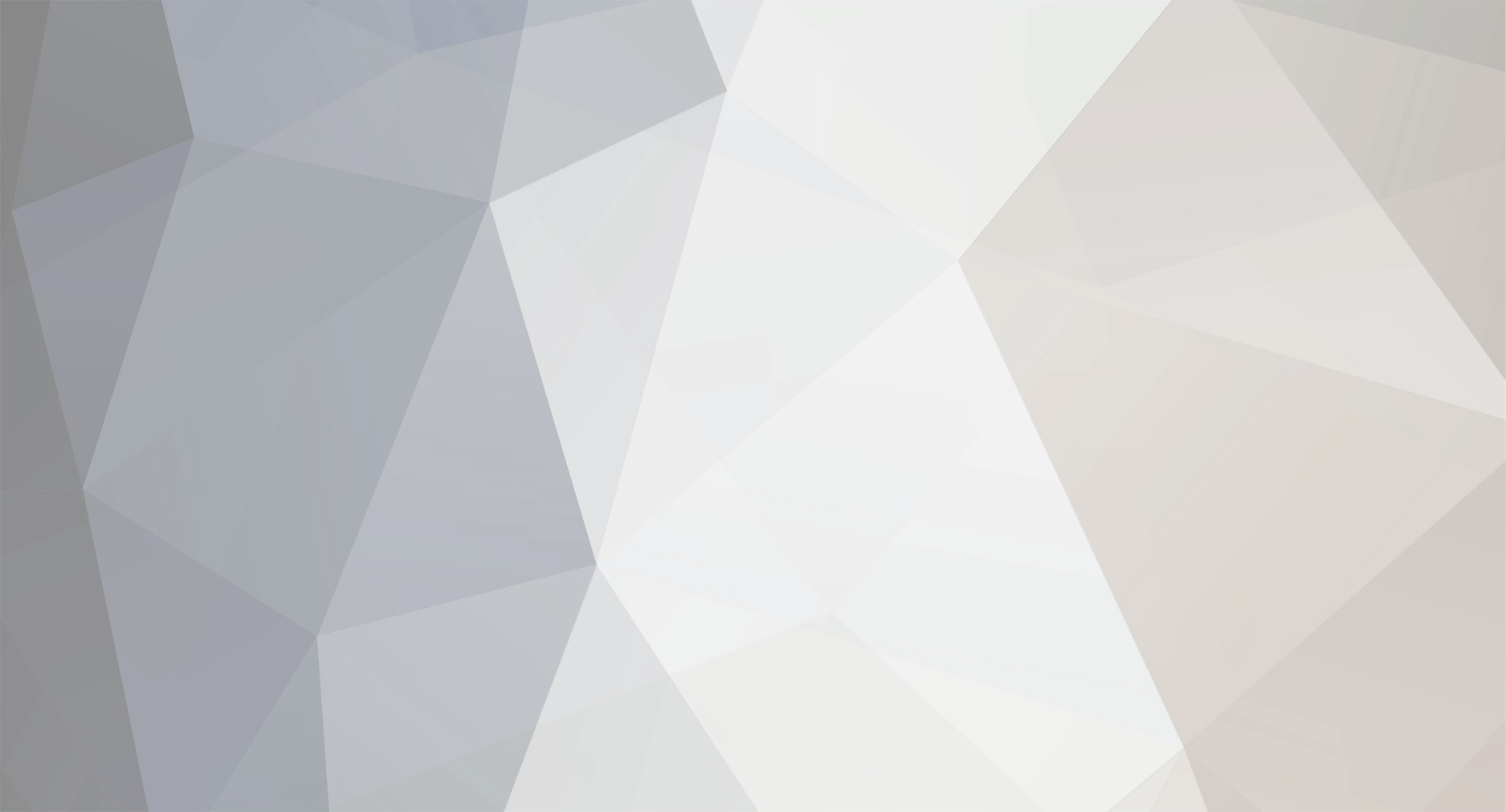
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
In your 'update players around me' packet, you need to send the original player's entityId so that the other players, when they receive the packet, can update the information for THAT player, otherwise you are just setting their own data to whatever the other player's is. So, in your packet: public YourPacket(EntityPlayer playerWhoChanged) { this.entityId = playerWhoChanged.getEntityId(); this.isMorphed = YourIEEP.get(playerWhoChanged).isMorphed; } // obviously taking liberties with the method parameters here public process(Message msg, EntityPlayer player) { EntityPlayer playerWhoChanged = player.worldObj.getEntityById(msg.entityId); YourIEEP.get(player).setOtherPlayerInfo(playerWhoChanged, msg.isMorphed); } Each player needs to know the status of the other player, and you cannot do that unless you have the information for that other player when updating the current one. Does that make sense?
-
[1.7.10] TileEntity Mimicing Changes on All Instances
coolAlias replied to Izzy Axel's topic in Modder Support
Yes (he is referring to 'light'), but local variables do not work as expected due to all Block and Item instances being singletons, i.e. effectively every local variable is static, meaning it has the same value across all of your Block classes. Another thing, you should NEVER call updateEntity or any other update method, or any write to NBT methods, manually - these are all called at the appropriate times by Minecraft, and calling them manually not only makes things messy, it is apt to introduce buggy or other unwanted behavior (as well as just being less performant). -
do you mean something like public static final void sendToPlayers(IMessage message, Set<EntityPlayer> player) { PacketOverlord.dispatcher.sendTo(message, (EntityPlayerMP) player); } A Set is a type of Collection, so you would need a for each syntax: public static final void sendToPlayers(IMessage message, Set<EntityPlayer> players) { for(EntityPlayer player : players) { PacketOverlord.dispatcher.sendTo(message, (EntityPlayerMP) player); } } EDIT in reply to your question about the player not knowing the values of other players: you should be able to send the IEEP values for each player to each other when they begin tracking each other. That's what we've been discussing so far. I haven't personally used PlayerEvent.StartTracking before, so I cannot confirm if it works 100% as advertised, but it should fire each time a player starts to track an entity, not constantly while tracking an entity. At least that's how I would assume it works. If that's not how it works, another option would be as you suggested: keep a list / map of each player with their associated model/state values that is updated any time any player changes their status, and keep that list updated on each client so they are aware of every other player's state.
-
No, you don't need it - what he was doing there is transforming his custom packet class into a vanilla packet and sending it through the vanilla network (via EntityTracker's method). Pretty slick, but not needed if you don't already have it (I could swear there was some built in code somewhere that was able to do the above for your packets, but I'm not finding it quickly...). What you can do instead is make a method to send to a collection of players, then send to the set of players returned by EntityTracker: Set<EntityPlayer> players = ((WorldServer) player.worldObj).getEntityTracker().getTrackingPlayers(entityBeingTracked)); PacketDispatcher.sendToPlayers(new YourPacket(), players);
-
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
You shouldn't use global entity ID - ONLY use registerModEntity, especially if your entity does not need a spawn egg. Are you sure that the ItemStack NBT tag has the appropriate data when creating your entity? Print that out, too. This is basic debugging, and something you should become more familiar with if you wish to solve problems on your own. -
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
Did you register your entity using EntityRegistry#registerModEntity? If so, then did you set the entity's element before spawning it? If you put a println in the write method, what is the value of element there vs. the value when you read it back from the buffer? -
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
IEntityAdditionalSpawnData is very simple: it lets you add extra data to the spawn packet (on the server) that gets sent to the client, and then read your extra data on the client. You just have to implement it, then make sure that whatever data you need is set BEFORE you spawn the entity in the world; usually onSpawnWithEgg is a good place to do that, or, since it's a magic spell, just call setElement(int) before you spawn the entity. -
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
Basically like this: public YourClass extends Render { public void doRender(Entity entity, double x, double y, double z, float yaw, float partialTick) { // probably call GL pushMatrix here bindTexture(yourTextureResourceLocation); // you'll probably want some rotate and / or translates here to get everything looking right Tessellator tessellator = Tessellator.instance; tessellator.startDrawingQuads(); tessellator.setNormal(0.0F, 1.0F, 0.0F); // then add all the vertices - you'll need to change the numbers to match your texture tessellator.addVertexWithUV(-0.5D, -0.25D, 0.0D, 0, 1); tessellator.addVertexWithUV(0.5D, -0.25D, 0.0D, 1, 1); tessellator.addVertexWithUV(0.5D, 0.75D, 0.0D, 1, 0); tessellator.addVertexWithUV(-0.5D, 0.75D, 0.0D, 0, 0); tessellator.draw(); // pop the matrix if you pushed } } You'll probably want to search on Google for information about Tessellator and addVertexWithUV to better understand what's going on. -
While I don't know the answer to all of those questions, one thing is for certain - you need to store the current model ID in the player's SERVER-side IEEP, then have that send out a packet to all other players (on the client) letting them know what that player's current model is. The trouble is that players don't have any way to know what another player's client-side status is, thus the need to relay that information to the server first, then back to all client players. As for the models overlapping and that kind of thing, I'm not really sure, but you may want to try keeping a list of players and their current model IDs on each client (synchronized from the server, of course, whenever one of their IEEPs changes) - this way when your client rendering code is called, it can find the correct model for the player being rendered.
-
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
Did you put 'break;' in each of your switch cases as diesieben pointed out? If they are missing, each case simply falls through to the next and you end up returning the snowball icon. As an alternative to break, you could simply return the icon directly in each case, effectively breaking out of the switch that way. Re: #1, you don't need to make a hidden item to render a texture, you can bind your ResourceLocation and then draw it using the Tessellator. -
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
See my post just above yours - the client does not know the value of 'element' unless you tell it, so at the moment all of your entities have element '0'. -
[1.7.10] Questions about rendering thrown entities
coolAlias replied to CactusCoffee's topic in Modder Support
For #2, you need to make sure that the entity 'type' is known by the client, typically by implementing IEntityAdditionalSpawnData (which is perfect for fields like 'type' that probably won't change once the entity is spawned) or by using DataWatcher / custom packets to sync the client. -
Alternatively, if you are not comfortable with iterators / you just want one thing to drop, an easier solution is to clear the drops list completely and then add your stack to it. event.drops.clear(); event.drops.add(new ItemStack(yourItem)); Done. However, if you don't know how to use iterators, you could use this as an excuse to learn about them, because they are very useful.
-
[1.7.10] [UNSOLVED] Ticking memory exception/NullPointerException...
coolAlias replied to Ms_Raven's topic in Modder Support
It would help you a lot to learn some basic debugging skills: 1. When you crash, read the log 2. At the lines the log indicates, put break points or println's to determine the values of variables 3. Run your code again and observe the variable values At that point you have likely at least discovered the culprit, and can then figure out why it is behaving unexpectedly. In your crash log, for example, there is a NULL pointer exception during your gui's updateScreen method - looking in there, you only have a few possible culprits: 'this' when accessing any class field (e.g. 'this.width'), which seems unlikely to be null, and 'amount1'-4 when trying to call a class method 'updateCursorCounter'. Hm. amount1-4 are not initialized when the class is created, relying on initGui, so perhaps the updateScreen is getting called once before the gui has initialized. In that case, you could simply check if each one is null before calling a class method, or you could initialize them inline with their declarations, or however else you want to solve it. -
Without searching through your entire code base for unnamed classes, the issue seems to simply be that damage / speed attributes are not automatically sent to the client, whereas health and others are. Are you using attribute modifiers for damage and speed also? If you are not using attribute modifiers for the talent bonuses, then that is your solution. If you are, then the solution is either to send a packet to update the client whenever a talent changes or, if the code happens to run on both sides, just apply the talent on both sides. You'll probably still want to send a packet at some point to validate that the value is correct on the client. Show me your 'talent' code that affects damage and speed, as well as where you apply them.
-
applyEntityAttributes is called when the entity is first constructed (i.e. before NBT is read), so your entity does not yet know if it is a child or evolved or anything else. You will need to update the attributes somewhere else, such as readFromNBT, onSpawnWithEgg, or better, when you set 'hasEvolved' or any other field that influences them. The shared monster attributes are all saved, so if you set them once (such as from setEvolved() or whatever), they will retain the values you set when you reload the game.
-
I don't know, but one way to find out would be to print to your logger during the world tick event - you will see how many server vs. client ticks appear while generating your structure, and then you will know if the client is continuing along on its dandy way whilst the server is entangled in your structure gen's for loops.
-
Typically you see that kind of error when you do not correctly register your entity via EntityRegistry#registerModEntity - can you show that code as well? So, ItemCoalGun is a Block with a TileEntityCoalGun... can your names get any more confusing? I don't understand your logic here - you can render a 3D model with an Item just fine, so why switch to a block, let alone one with a TileEntity? I highly advise against this kind of 'solution' - much better to actually learn how to properly handle rendering.
-
For items, you cannot add a timer directly to the Item class due to the fact there is only 1 item instance of each item you declare, so you need to store any mutable information in the ItemStack NBT tag. For a timer, you have two options: actually use a ticking timer that needs updating each tick, OR set one time value that is X ticks in the future, and check for when the world time reaches it. The second is nice because it avoids the NBT update - when the ItemStack NBT updates, it usually causes a rendering 'glitch'. So: 1. Override Item#onItemRightClick a. Add an NBTTagCompound to the ItemStack given if it doesn't already have one b. Set a Long value (that's what Minecraft time uses) in the tag with world.getWorldTime() + X, where X is number of ticks (20 ticks = 1 second) 2. Override Item#onUpdate a. Check if the ItemStack in question has an NBTTagCompound and the correct tag (and the value is > 0) b. If so, check if the current world time is >= the value stored in the tag c. If so, do whatever you wanted to do, and either remove the time tag or set it back to zero
-
using extended player properties in command
coolAlias replied to jamiemac262's topic in Modder Support
@EventHandler is only for the FML events involved in the mod loading process - all other events use @SubscribeEvent. Next time something doesn't seem to be working, put a println statement inside and watch your console - if it doesn't print, you then have more knowledge of what the problem might be. E.g. @EventHandler public void onEntityConstructing(EntityConstructing event) { System.out.println("Entity is constructing."); // Doesn't print, so you know the problem has to do with your event } Also, for code, either use [ code ]code here[ / code ] tags (without spaces) or a service like pastebin - it makes it far more readable. -
using extended player properties in command
coolAlias replied to jamiemac262's topic in Modder Support
If your IEEP class is returning null, chances are you did not register it correctly during EntityConstructing event, or you did not register your event handler class. Show your event handling class and registration. -
[1.8] MinecraftByExample sample code project
coolAlias replied to TheGreyGhost's topic in Modder Support
Don't know if you're looking for anything to do with armor, and it's for 1.7.10, but perhaps you can adapt this to your needs. -
Skills like seeing what fields a class has - super super basic. Skills like not using non-existent or unrelated objects (Draco already pointed it out - where'd that itemstack come from? why is it stored in some random place? how can you possibly expect it to relate to the event?). Sure you may have made some interesting mods, but that doesn't mean you have sufficient Java skills, especially considering that you think that your current project is 'complicated' - I don't wish to offend you, but it is very simple, and your lack of skill is what is making it difficult for you. Basic Java skills. Learn them. You may think you already know them, but 'basic Java' is way more than knowing the difference between int and String.