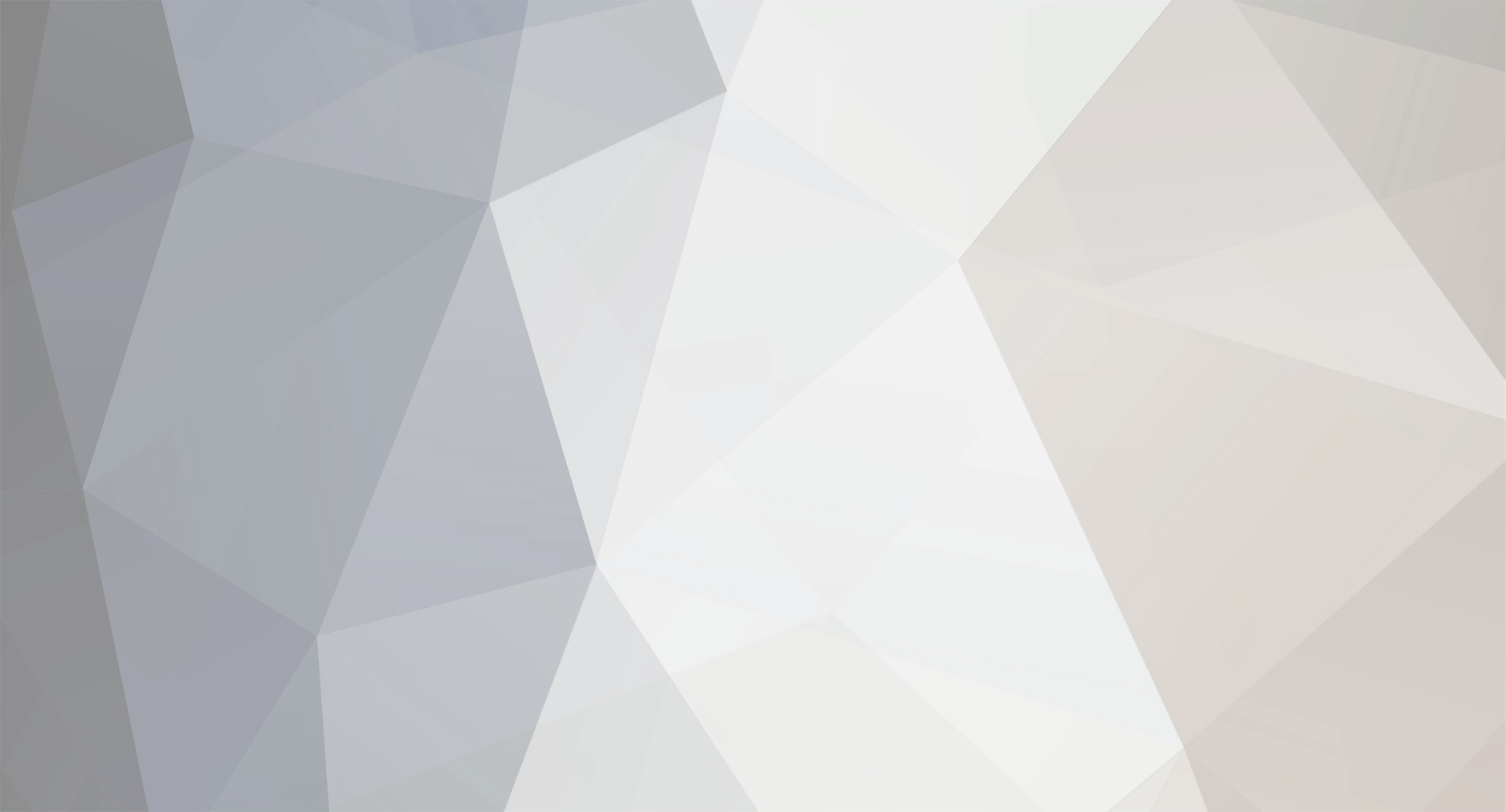
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.8]Annormal status , How to stun a mob ??
coolAlias replied to perromercenary00's topic in Modder Support
A couple options: 1. Subscribe to LivingUpdateEvent and cancel it for the mob in question. Pros - mob will not move or attack; Cons - it cancels the mob's update tick, meaning lots of stuff that's supposed to happen doesn't 2. As above, but instead of canceling it, set the mob's position to its previous position, then also subscribe to LivingAttackEvent and cancel it for the mob in question. Pros - mob does not attack and mostly doesn't move, mob still gets its update tick; Cons - more work, mob still moves a little bit, for players you will also want to cancel mouse and keyboard input using the KeyInputEvent and MouseEvent. -
Even if you had the correct code for targeting, your arrow would not work because you extend EntityArrow - the EntityArrow's onUpdate method and yours are conflicting. A workaround is to override onUpdate and make sure to NOT call super.onUpdate; instead, call super.onEntityUpdate - this will bypass the EntityArrow update code (meaning you will need to duplicate it in your class, changing it where you need) and go straight to Entity#onUpdate, which you still need to be called. For homing, the basic trig looks about like this: double dx = target.posX - this.posX; double dy = target.boundingBox.minY + (double)(target.height) - this.posY; double dz = target.posZ - this.posZ; setThrowableHeading(dx, dy, dz, 3.0F, 1.0F); Of course, it can get more complicated if you want the arrow to have a pretty arc instead of aiming directly at the target every tick.
-
That's strange - when I was working on my structure gen code in 1.6.4, I could easily generate 10-20,000 blocks with tons of metadata blocks (many of them rotated based on the structure), complicated tile entities (chests filled with loot), and loads of other stuff, and it never took more than 3-5 seconds. Note that this was not during world-gen either; structures were generated on right clicking with an item. I haven't played much with that kind of structure gen in 1.7.x and up, but I can't imagine it would have slowed down that much. Have you tried simply generating the structure all in one go, rather than using a tick event to do it? Perhaps forcing it to break up like that is making it worse?
-
It's much faster to make a list of blocks that need to be placed after the structure is complete during the first loop (storing x/y/z as well), then iterate through that for the second pass. Beats going through the full loop again by a long-shot. I'd do the same for the 3rd pass, though I've never found a need for a third.
-
I think worrying about number of blocks generating is premature optimization, and it all depends on the algorithm(s) you use to do the generation. If you just call setBlock(someBlock) 50,000 times or so, it won't take very long at all, but if you have a complex algorithm to figure out how to place each block, then placing those same blocks will take exponentially longer. I'm not sure what the exact ramifications of the 'ticks taking too long' message, but it happens to me all the time ever since 1.7.x.
-
[1.7.10] Player hitbox and hit mechanics - how to change stuff
coolAlias replied to Thornack's topic in Modder Support
The only major change I would make is to use the KeyInputEvent and/or MouseEvent to process directly when the player presses the attack key (left clicks), rather than adding yet another thing to process every single tick. I would only use the tick event if you are trying to wait until the player's arm swing has reached a certain point, but I'm pretty sure vanilla doesn't bother to do that, and in your example code you process right when the player first swings anyway. Another point is you can write an int directly with ByteBuf#writeInt; I don't know if you're trying to save a byte or two in your packet or whatever, but for a tutorial simpler is usually better - unless you want to explain to folks what the arguments are and why you chose '4' I would also encourage you (and everyone else) to name variables something relevant, rather than var2, var9, etc., especially in a tutorial where you'll have lots of people that already have a harder time understanding code trying to figure out what you're doing. You'll probably want to add comments within the code to help people out, too, and maybe break the code up into finer sections which are easier to explain rather than entire blocks of code - otherwise all you've made is copy-pasta for hungry folks who don't know how to cook, and they'll eat it without even checking the ingredients. -
[1.7.10] Player hitbox and hit mechanics - how to change stuff
coolAlias replied to Thornack's topic in Modder Support
Yep, that's what I was saying, but I couldn't recall exactly where it took place. The packet HAS to VERIFY that the action is valid on the server, thus the distance check when processing the vanilla attack packet. You will need to do the same in yours, or you could use ASM to modify the vanilla packet to include a hook for determining the player's reach distance, perhaps via your Item interface. Easier to make your own packet As for FMLClientHandler's getClient() method, I don't see how that is any different from Minecraft.getMinecraft() except for it takes longer to type. It will still crash you on a dedicated server, and shouldn't be used on the integrated server either, although the code will function if you do it on the latter. The integrated server can technically run @SideOnly(Side.CLIENT) code because it is part of the 'client' environment, i.e. what used to be the single player .jar. This is why diesieben and other people always say not to use that annotation and try to stress that it has absolutely nothing to do with logical side - it is very misleadingly named, or rather its name overlaps the terminology used to describe other things. EDIT: Looked through BWM's code and I'm not sure how they managed to get the reach to work - I can see that they use the vanilla PlayerControllerMP method which calls the vanilla packet, and this makes me suspect that perhaps their reach system doesn't really work the way they think it does. Or they may have patched it with ASM, or I just haven't found their code yet. -
[1.7.10] Player hitbox and hit mechanics - how to change stuff
coolAlias replied to Thornack's topic in Modder Support
You need to use PlayerControllerMP#attackEntity - this is what vanilla code does on the client when you left click, and it sends an attack packet. EntityRenderer and objectMouseOver are all also client-side only, which is why I brought that up earlier - you are running single player with the integrated server, which has access to client only code because it is (was) part of the client jar. Just because it works in single player 'on the server' does not mean it really works like you would expect - your code will most certainly crash on the dedicated server. When you call entity#setDead, you are on the client, so you 'kill' the visible version of the entity for you, but the server and any other players will still see it just fine. Anyway, yes, allowing the target to be determined on the client side (which is what vanilla does) could open up to hacking, but only if you write your server-side packet handling poorly; you should be validating on the server that the player can actually reach (like vanilla does) and calculating damage and everything else independently of the client - all the client does is say 'hey, the player is trying to attack entity X'. PlayerControllerMP#attackEntity is called on the client and triggers an attack on the server via a vanilla packet, and that is what I have used in much of my code because I am not messing with reach; to change the reach, you will probably need to send your own attack packet, but I highly suggest looking at the vanilla code first - you may be able to use it as is, and if not, it at least gives you a solid template for what you need to have in your own version of the attack packet. -
[1.7.10] Player hitbox and hit mechanics - how to change stuff
coolAlias replied to Thornack's topic in Modder Support
Why are you using FMLClientHandler.instance().getClient() instead of just Minecraft.getMinecraft() ? They both return the client-side only Minecraft class (the docs say 'server instance', but that makes no sense when the class is client-only), and your code will crash on the dedicated server (unless there is some cpw magic going on that let's his code do what no one else's can). There's nothing wrong, imo, with calculating the distance on the client side and calling PlayerControllerMP#attackEntity - this causes a packet to be sent and the attack will process on the server perfectly fine (though I haven't tested it with altered reach). Alternatively, you can check out the Item#getMovingObjectPositionFromPlayer code which works on either side, alter it a little for your reach distance, and use that to find the object hit and go from there. -
I was just pointing out that any exposed part of a library/mod is an API and that the way to use an API, whether from a mod or a library, is identical. It would of course be silly to call a mod with some exposed methods a library, even though that would be semantically correct
-
@Abastro What's wrong with using an anonymous class? @OP For getting the player name, you'd have to look at what type of Object is stored in the 'playerInfoList'. Without looking, my guess is it would be an EntityPlayer of some type, in which case you can use any of the EntityPlayer methods to find their usernames: for (int i = 0; i < this.list.size(); ++i) { ((EntityPlayer) this.list.get(i)).getDisplayName(); } Yes you can display it over a background by drawing the background and then drawing the foreground, or you can make the scrolling frame part of the background and just draw the text and scrollbar - look at vanilla and/or modded GUI classes, watch the VSWE interface videos again, etc. and you will be able to figure it out. Here is an example of a GUI with a scrollbar.
-
An API and a library are one and the same, the API being the public interface to the library. Semantics aside, they are used in exactly the same fashion: developers use a deobfuscated jar and everyone else uses the normal jar.
-
People will need to have the compiled .jar in order to run any mods using your library, unless your plan is to have each modder compile your code and distribute it with their mod (which would be a version nightmare, among other things). So yes, you do still need the normal .jar.
-
[1.8]Split a mod in multiplex packages.jar
coolAlias replied to perromercenary00's topic in Modder Support
Just a random FYI about your tutorial comments: the 'Mod Settings' gui in Forge was never intended to be functional - it was added by cpw purely as an example for modders to see what was possible with the config API. Many of the features (parent/child menus etc) need to be implemented by the modder, but Forge will correctly interpret them. You may want to check out GotoLink's Battlegear2 config code - it's not really 'replacing' the gui menu so much as adding yours to the list of mods with config guis using IModGuiFactory. -
Bow damages player when shooting on survival
coolAlias replied to 2FastAssassin's topic in Modder Support
Like an arrow with a different texture? Then all you need is to create your class 'public class EntityCustomArrow extends EntityArrow' and register a custom render class for it, and spawn your custom arrow entity from your custom bow. You shouldn't need to copy any of the EntityArrow code into your custom class - just let the super class take care of everything except for colliding with the player (otherwise you will get regular arrows back). -
Bow damages player when shooting on survival
coolAlias replied to 2FastAssassin's topic in Modder Support
Then find a better tutorial; better yet, start by learning Java. Also, your first and second arrow are exactly the same, and it doesn't look like they are doing anything that the vanilla arrow doesn't already do. What are you trying to accomplish? -
Bow damages player when shooting on survival
coolAlias replied to 2FastAssassin's topic in Modder Support
Never heard of pastebin or at least code and spoiler tags? That wall of text is atrocious to read. Why do you copy the entire EntityArrow class into your class that extends EntityArrow? Do you not realize how object inheritance works? That's almost guaranteed to be the reason your code is not working as you expect. -
Bow damages player when shooting on survival
coolAlias replied to 2FastAssassin's topic in Modder Support
If you look at any of the vanilla projectile classes, they (almost?) all have a bit of code that looks something like: if (this.ticksExisted > 5 || entityHit != this.getThrower()) { // impact entity hit, deal damage, etc. That gives the projectile 5 ticks before it is able to hit the entity that shot / threw it; without it, the projectile detects the player / shooter as intersecting its hit box right when it spawns and thus attacks the player instead of going anywhere. -
Saving a Reference to an Entity (Save/Load Persistence)
coolAlias replied to Draco18s's topic in Modder Support
TE and cart will be in the same chunk. Specifically 1 block away from each other. In that case you can simply store the UUID, and if the world#getEntityById returns null, get a list of entities in a bounding box enclosing that area and iterate through them until you find one with a matching UUID - there's your minecart. -
Saving a Reference to an Entity (Save/Load Persistence)
coolAlias replied to Draco18s's topic in Modder Support
Also keep in mind that your entity reference will always return null if the chunk that it is in is not currently loaded. I've gotten around that by also storing the last known chunk coordinates, then force loading those chunks for the search when necessary. As diesieben pointed out, though, it might not be a bad idea to store the coordinates anyway for the sake of narrowing your search. For my part, I just use the UUID when the entityId is no longer valid, and use the entityId once the entity is found. See here. -
What's the most efficient way to use event handlers?
coolAlias replied to Bedrock_Miner's topic in Modder Support
I doubt that having more or fewer classes really makes a difference as far as efficiency. If your mod is running slowly, profile your code and see where the bottleneck is, then optimize that, but don't prematurely sacrifice clean organization of your project for what you imagine may be more efficient code. In my case, I keep logical groups of events together in the same class, e.g. all my combat-related events go in CombatEventHandler class, whereas my world gen events go in GenEventHandler, etc. Some of these are registered to multiple buses, but most just one. For GuiOverlays, I make one class per overlay, treating it like any other GUI even though it uses an event. Keeping client events separate is also nice, as then you can have a class member for Minecraft.getMinecraft and other such things that would crash your game otherwise. Everyone has their personal preferences, and you should just choose one that makes sense for you and your project. -
[1.7.10] Getting Entity Data based off of look vector doesnt work
coolAlias replied to Thornack's topic in Modder Support
I have some code which isn't exactly what you need, but you could use it until you figure out a better solution (which you could find by researching / duplicating the code Minecraft uses on the client to determine the objectMouseOver). Anyway, it's a method that searches for the nearest entity to the player within a certain distance and with a maximum deviation from line-of-sight; if you set the deviation to be small, it will effectively get the objectMouseOver. Still, I would look into EntityRenderer#getMouseOver, or you could even just send the current objectMouseOver's entityId (or null) in a packet from the client, then handle your effect on the server when you receive that packet. That would save you from duplicating a bunch of code at the cost of delaying your action by the amount of time it takes to send a packet (which is usually negligible).