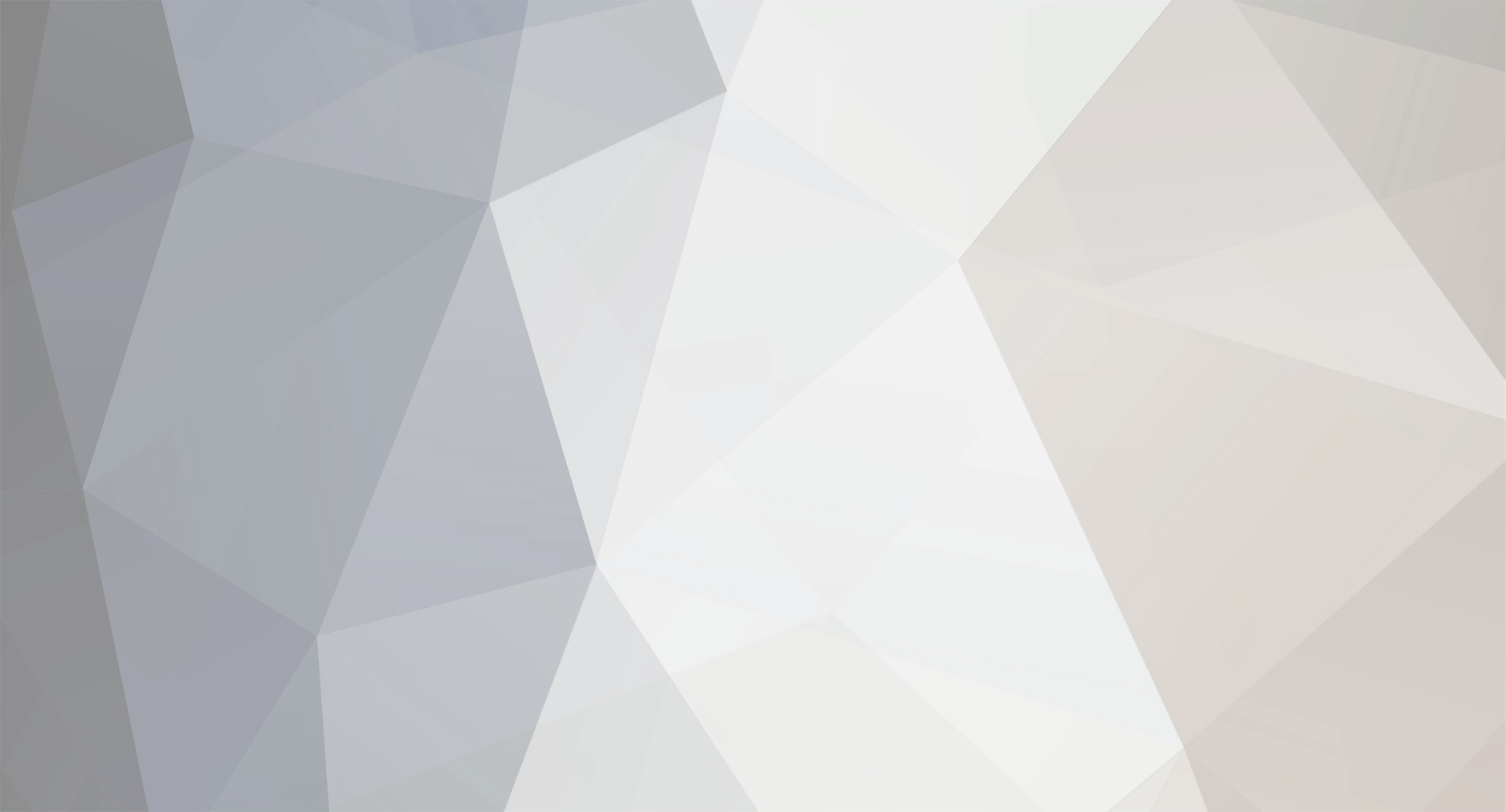
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.7.10] detect if specific player enters/leaves area.
coolAlias replied to denbukki's topic in Modder Support
You don't need any for loops, World#getEntitiesWithinAABB already searches within an area. int r = 25; // that's a pretty big range, btw, you may want to consider lowering it // I usually only search 1/2 the distance on the y axis as well, and sometimes only starting from the same yCoord // as the TE is at, unless you want players below it to also trigger it // following uses x/y/z instead of xCoord/yCoord/zCoord for brevity world.getEntitiesWithinAABB(EntityPlayer.class, AxisAlignedBB.getBoundingBox(x - r, y - r, z - r, x + r, y + r, z + r); -
Those are exactly the same... -.- @OP It sounds like your potion effect just wasn't active - applying it once per tick for one tick isn't nearly long enough. Do you want the player to take no damage ONLY while still wearing your armor? Check if the player is wearing the armor then, rather than using a potion effect, during the LivingAttackEvent.
-
[Solved][1.7.10]Rendering Nametag Above Custom Mob?
coolAlias replied to skullcrusher1005's topic in Modder Support
If it's your own custom mob class, you can set the name directly in your entity's constructor or in onSpawnWithEgg or pretty much anywhere else in your class - no need for a Forge Event. -
[1.7.10]How to check actual entity type with Check Spawn event
coolAlias replied to jabelar's topic in Modder Support
Casting to a type doesn't remove the true type. Try it: // let's try with an EntityPlayer since those are easy to come by, and we'll get it from a method parameter EntityLivingBase entity = (EntityLivingBase) player; if (entity instanceof EntityPlayer) { System.out.println("It's still a player!"); } Are you sure you are getting EntitySheep in the CheckSpawnEvent? As an aside, anytime you have an entity (such as in EntityConstructingEvent, though it is probably zero there), you automatically have its position: entity.posX, entity.posY, entity.posZ You could always use EntityJoinWorldEvent. -
LivingAttackEvent is called on both server and client side when the event.entity is a player; put some logging statements or printlns in there to see what exactly is going on and on which side it is being called, such as if the potion is active and if so on which side(s). At the very least, you want it to be active on the server side and cancel the server side event, as that is what will actually cause damage to the player. I don't think there is actually anything that happens as a result of processing on the client side, so you probably don't need to worry about that at all.
-
That should work - did you register your handler to the MinecraftForge event bus?
-
Basically: player.motionX = someValue; player.motionZ = someValue; It would help if you provided some context for what you are trying to do. When, exactly, do you need to change the player's motion?
-
[1.7.10] SimpleChannelHandlerWrapper exception (Client to Server only)
coolAlias replied to Belpois's topic in Modder Support
Show your Heartbeat class. -
Right-click on your project in Eclipse -> Build Path -> Configure Build Path -> Libraries tab -> click on forgeBin -> Edit -> navigate to the forgeSrc version which should be in the same folder; if not, open a command prompt and run 'gradlew setupDecompWorkspace'
-
You added forgeBin, you want forgeSrc.
-
[1.8][SOLVED] Problems with Server once again..
coolAlias replied to NovaViper's topic in Modder Support
Are you sure? Because I've done just that in some of my mods and they play on servers just fine. I understand that some things must be routed through the ClientProxy (the IMessageHandler getPlayer for example) because of the way classes load, but it seems such an inefficient solution. Imagine that you have 20 entities and each one has a spawnParticles method, and within each the implementation is radically different. Do you create a special 'spawnParticles' method for each one in your ClientProxy? Do you create one method, but have chained if/elses checking if (entity instanceof EntityOne) spawnParticlesOne ? That would just be absurd. Perhaps you could make an interface, ISpawnParticles, and cast your entity to that from the ClientProxy method which then calls the entity's class method, but it sounds like you are saying that would still crash since the actual implementation is within the entity class? What a convoluted solution to what should be a simple problem, in my opinion. Granted, things don't always work the way we'd like, but this particular design decision (if it was a decision at all or just plain necessity) boggles my mind. -
Hm, maybe there isn't a difference any more, but there used to be. Well, just listen to diesieben EDIT: Yeah, looks like the documentation is completely wrong, as event.getModLog just returns LogManager.getLogger with your mod id... Before FML switched to the Apache logger, though, I remember it being useful.
-
That's neat, but the main point of my post was about the benefits of getting the log from FMLPreInitializationEvent instead of using Apache's LogManager directly.
-
It can be useful wrapping the logger in another class, if only to save a few characters when using it: LogHelper.info("message") vs. MyMainMod.logger.info("Message"). Anyway, you can also get your Logger instance specifically for your mod from FMLPreInitializationEvent: @Mod.EventHandler public void preInit(FMLPreInitializationEvent event) { logger = event.getModLog(); } Similar to LogManager.getLogger("YourModId"), but it has the FML log as its parent, keeps formatting consistency in the console for all those log messages, and can be configured from the config/logging.properties file.
-
[1.8][SOLVED] Problems with Server once again..
coolAlias replied to NovaViper's topic in Modder Support
You shouldn't use FMLCommonHandler to get the effective side when you have a World object available - if (worldObj.isRemote) is a much cheaper operation. Whenever you have a method, class or field that operates on the client side only, you can only access it on the client side; i.e. any time you want to access it, nest your code in a side check: if (worldObj.isRemote) { // yay, you're on the client spawnParticles(); // or other client-only code } -
That's still not how events work... please read a tutorial.
-
[solved][1.7.10] LivingFallEvent get not catched
coolAlias replied to cad435's topic in Modder Support
Event handling methods need to be public; yours is private. Also, LivingFallEvent does not have a DamageSource 'source' member - that should be giving you an error. PlayerLoggedInEvent, btw, is on the FML bus, not the regular event bus, which is probably why it didn't work for you. -
I made a pretty generic render class - not perfect, but for probably 80-90% of the types of mobs people create, it will work. Example usage: RenderingRegistry.registerEntityRenderingHandler(EntityDarknut.class, new RenderGenericLiving( new ModelDarknut(), 0.5F, 1.5F, ModInfo.ID + ":textures/entity/darknut_standard.png")); Only thing missing is armor rendering - it renders held items and helmets, though all of that code will have to change drastically for 1.8. I don't have any entity classes that are simple enough for your project, except maybe the NPCs. Jabelar has some good tutorials on custom AI, animations, and probably some other stuff about entities that may help, especially since you have never coded an entity before.
-
[SOLVED][1.7.10] Detect if event.entity is mc.thePlayer?
coolAlias replied to Ernio's topic in Modder Support
Don't do that - you can send a packet from PlayerLoggedInEvent instead, or even EntityJoinWorldEvent, but not during EntityConstructingEvent. -
How do you know it is not running at all? Did you put some System.out.println statements inside the updateEntity method? If not, you should and see if they print to the console.
-
Did you register your TileEntity? Did you create a block that provides your tile entity?
-
[Forge 1.8] Crash When Trying to Create Armor
coolAlias replied to HassanS6000's topic in Modder Support
You should probably spend some time learning Java if that didn't make sense. This is a declaration: public static Item myItem; // now there is something named 'myItem', but it is NULL right now. This is an initialization: myItem = new ItemWhatever().setUnlocalizedName("myitem"); // now 'myItem' is a valid Item and no longer NULL 1. You never initialize your ArmorMaterial, all you did was declare it, so it is NULL. 2. renderIndex is used by vanilla, you can probably just pass in zero or whatever else you want. 3. Declare your Items where they are now, but do not initialize them. 4. Initialize your items inside of the method. P.S. Do not send private messages to people about your post. It is both annoying and unnecessary. People will respond if and when they have time / knowledge to do so, and pestering them is only going to make them less likely to help you. -
[Forge 1.8] Crash When Trying to Create Armor
coolAlias replied to HassanS6000's topic in Modder Support
If you bothered to look at the ItemArmor constructor, you would see: public ItemArmor(ItemArmor.ArmorMaterial material, int renderIndex, int armorType) So the 2nd parameter needs to be an integer render index, the same value for each piece of the set, but it doesn't matter because custom render classes probably won't use it at all. It's mostly for vanilla. But that's unrelated to your crash. Your crash is caused because you try to use your ArmorMaterial 'HaloArmor' (which should be 'haloArmor' - lowercase letter first) before it is initialized. Put your armor Item instantiation inside the FMLPreInitializationEvent method, after you have instantiated your armor material. -
[SOLVED][1.7.10] Detect if event.entity is mc.thePlayer?
coolAlias replied to Ernio's topic in Modder Support
What exactly are you trying to do that you would need such a comparison??? If you are on the client side (which, btw, it is much better to use entity.worldObj.isRemote instead of FMLCommonHandler method), Minecraft.getMinecraft().thePlayer is ALWAYS the player for the current client. -
[1.7.10][SOLVED]Detecting the way player is looking
coolAlias replied to Wyverndoes's topic in Modder Support
Don't use setBlockBounds directly - use the player's facing to determine the metadata for the block, like many vanilla blocks do (e.g. furnace), then override setBlockBoundsBasedOnState and use setBlockBounds in there based on the block's metadata. Look at some vanilla blocks and it should make sense.