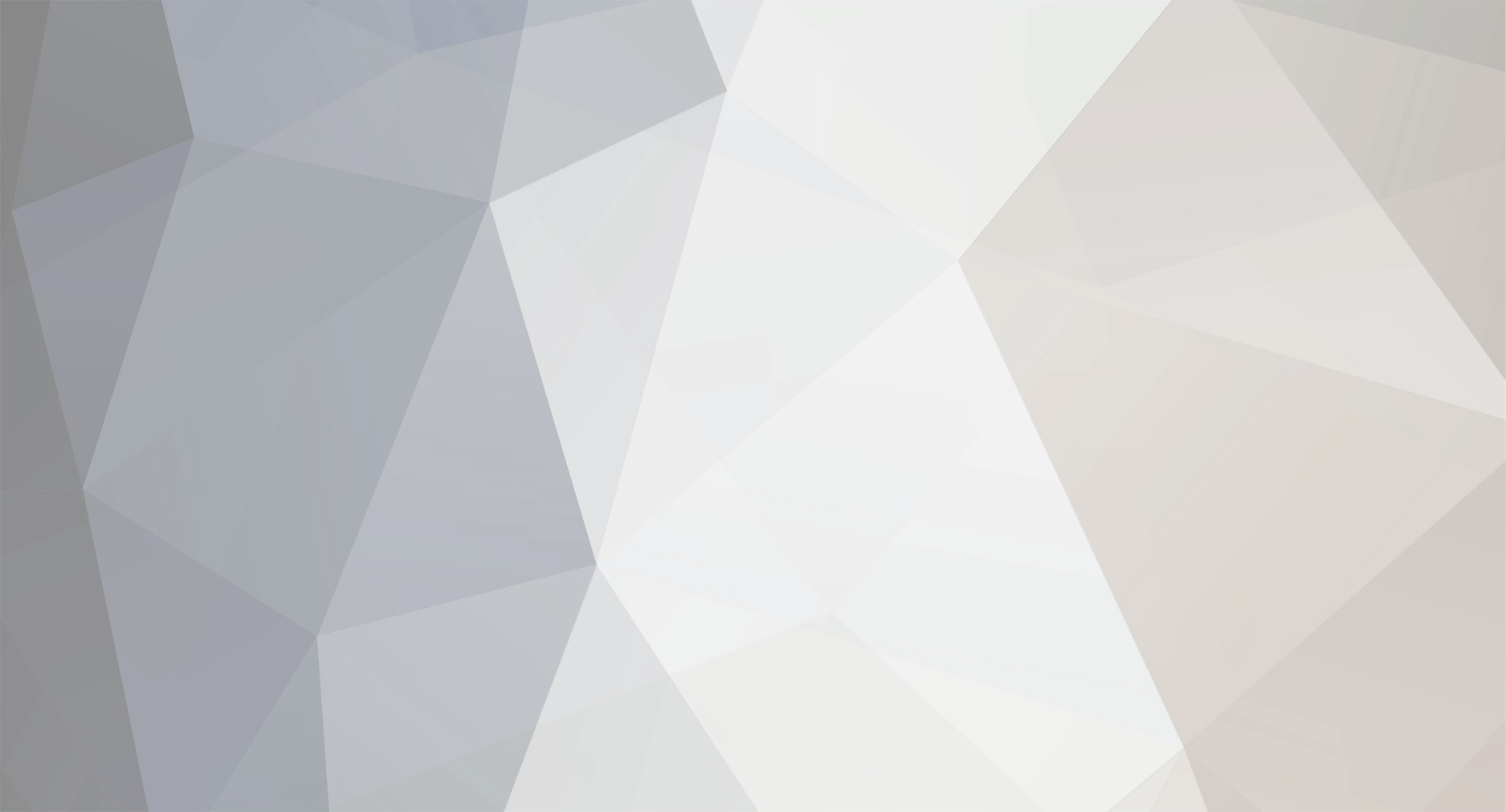
Godis_apan
Members-
Posts
109 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Godis_apan
-
[1.7.2] Player Overlay like Creeper when Powered
Godis_apan replied to Eastonium's topic in Modder Support
net.minecraft.client.renderer.entity.RenderCreeper, that should be all you need to know -
[SOLVED] [1.7.2] Rendering bow in 3rd person
Godis_apan replied to JimiIT92's topic in Modder Support
Do you want it to be rendered as a normal minecraft bow, if so remove all IItemRendering code and in your item constructor add this.setFull3d(true); or add: @SideOnly(Side.CLIENT) @Override public boolean isFull3D() { return true; } -
[1.7.2] Dimensional teleporting, without the portals
Godis_apan replied to whiskeyfur's topic in Modder Support
Problem, sry don't know how to fix, maybe check i WorldProvider for something useful. -
package something; import net.minecraft.client.gui.Gui; import net.minecraftforge.client.event.RenderGameOverlayEvent; import net.minecraftforge.client.event.RenderGameOverlayEvent.ElementType; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.eventhandler.EventPriority; import cpw.mods.fml.common.eventhandler.SubscribeEvent; public class MyClass extends Gui { @SubscribeEvent(priority = EventPriority.NORMAL) public void hej(RenderGameOverlayEvent event) { if (event.isCancelable() || event.type != ElementType.EXPERIENCE) { return; } drawString(FMLClientHandler.instance().getClient().fontRenderer, "heeeejjjjj", 40, 40, 0xffffff); } } Mainmod: MinecraftForge.EVENT_BUS.register(new MyClass());
-
[1.7.2] Dimensional teleporting, without the portals
Godis_apan replied to whiskeyfur's topic in Modder Support
entity.travelToDimension(dimensionID); (-1 for nether) although this spawn a portal in the nether. -
[1.6.4>>1.7.2]Major problems updating mod from 1.6.4 to 1.7.2
Godis_apan replied to chimera27's topic in Modder Support
FMLClientHandler.instance().getClient().displayGuiScreen(new GuiChat()); Does this help? -
[1.6.4>>1.7.2]Major problems updating mod from 1.6.4 to 1.7.2
Godis_apan replied to chimera27's topic in Modder Support
How was it before when you used the rendertickevent in 1.7.2, was it black as well, or just nothing. Does it work if you try to keep the rendertickevent with MinecraftForge.event_bus? Otherwise try to scale and translate stuff in the overlay and see how it's working. -
[1.6.4>>1.7.2]Major problems updating mod from 1.6.4 to 1.7.2
Godis_apan replied to chimera27's topic in Modder Support
Ohh and btw I don't think you should use FMLCommonHandler to register. Use MinecraftForge.EVENT_BUS instead -
[1.7.2] Relogging causes TileEntity (Block) to disappear
Godis_apan replied to tattyseal's topic in Modder Support
Is there an error in log when you enter the world? -
[1.6.4>>1.7.2]Major problems updating mod from 1.6.4 to 1.7.2
Godis_apan replied to chimera27's topic in Modder Support
First of all, you don't init your Items outside of the preInit, you just don't. It should look like: public static Item item; public void preInit(PreInitializationEvent event) { item = new Item().randomshit(); GameRegistry.registerItem(item, "sdjhflszhfl") } Can u please post your Item classes cuz I need to see the class. For your HUD use the RenderOverlayEvent instead of the ticking one. As for the KeyBinding I don't know, I've heard that that isn't complete, but I could be wrong. -
Hey, first your entity must be extending EntityLiving, then in the constuctor put setCustomNameTag("myYoutuber");
-
GameRegistry.addRecipe(new ItemStack(myProduct, 2, 0), "x", 'x', new ItemStack(Items.coal, 1, 1));
-
Yep that is an unregistred tileentity! You need to add this in your common proxy: GameRegistry.registerTileEntity(MyTileEntity.class, "MyTileEntityName"); I recommend in your proxy have a method called registerTileEntities(). ClientProxy: @Override public static void registerTileEntities() { super.registerTileEntities(); ClientRegistry.bindTileEntitySpecialRenderer(TileEntityToilet.class, new TileEntitySpecialRendererToilet()); } CommonProxy: public static void registerTileEntities() { GameRegistry.registerTileEntity(TileEntityToilet.class, "Toilet"); } And in your mod class in preInit add this: proxy.registerTileEntities();
-
First have you registered you tileentity? If you have add this to the tileentity code: @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); }
-
[1.7.2][Not needed now]Making an uncollideable block
Godis_apan replied to Naiten's topic in Modder Support
Can the player go through it? Describe exactly how you want it to work. -
My bad, looked into it and made it work, here you go: public Item getItemDropped(int damage, Random random, int fortune) { return myItem; } public int quantityDropped(Random random) { return 1; } public int quantityDroppedWithBonus(int fortune, Random random) { if (fortune > 0) { int j = random.nextInt(fortune + 2) - 1; if (j < 0) { j = 0; } return quantityDropped(random) * (j + 1); } else { return quantityDropped(random); } }
-
Ohh and a important thing is the order the GL11 codes comes in, so here is the order: GL11.glPushMatrix(); GL11.glTranslatef(); GL11.glScalef(); GL11.glRotatef(); Here your model goes!!! GL11.glPopMatrix();
-
GL11.glRotatef(); The params it takes are: GL11.glRotatef(float angle, float x, float y, float z); So if you want to rotate it on the y axis 90 degress the code should be: GL11.glRotatef(90F, 0F, 1.0F, 0F); The most simple way is to play around with it 'til it works!
-
[1.7.2][Not needed now]Making an uncollideable block
Godis_apan replied to Naiten's topic in Modder Support
What do you want? Can you go through the block? Is that the problem or is it that it still displays the message even though the collision box is null? -
http://www.minecraftforge.net/forum/index.php/topic,18439.0.html Might be some help. In the renderItem do: FMLClientHandler.getClient().getMinecraft().renderEngine/textureManager.bindTexture(resourceloc); //or something like that GL11.glPushMatrix(); GL11.glTranslatef(); GL11.glScalef(); GL11.glRotatef(); itemModel.render(size); //0.0625 GL11.glPopMatrix(); Hope that helps
-
As I've been a modder since a long time ago I know perfectly well what configs are, but why are you putting your entity codes within the config.load and save? It shouldn't be there except if you were to add config stuff there!
-
Ok, then try: return 1 + random.nextInt(fortune); if that doesn't work the do: return 1 + random.nextInt(fortune - 1)
-
Ok I would use a custom item renderer. Make a new class and make it implement the IItemRenderer. Example: package godis_apan.thedecorationpack.medieval.client.render.item; import godis_apan.thedecorationpack.medieval.client.model.ModelOrnateTable; import godis_apan.thedecorationpack.medieval.client.model.ModelTable; import godis_apan.thedecorationpack.medieval.lib.Reference; import godis_apan.thedecorationpack.medieval.lib.Textures; import godis_apan.thedecorationpack.medieval.tileentity.TileEntityTable; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraftforge.client.IItemRenderer; import org.lwjgl.opengl.GL11; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; @SideOnly(Side.CLIENT) public class RenderItem implements IItemRenderer { public RenderItemTable() { } @Override public boolean handleRenderType(ItemStack stack, ItemRenderType renderType) { return true; } @Override public boolean shouldUseRenderHelper(ItemRenderType renderType, ItemStack stack, ItemRendererHelper renderHelper) { return true; } @Override public void renderItem(ItemRenderType renderType, ItemStack stack, Object... data) { } } Thats the basic class. Now in the renderItem() method you want to check the item render type. If it's in the inventory, first/third person or rendered as an entity item on the ground. if (renderType == renderType.EQUIPPED) { GL11.glPushMatrix(); GL11.glScalef(1.5F, 1.5F, 1,5F); GL11.glPopMatrix(); } The you scale it up using the glScalef(). If you scale it alot you will have to translate the item in a correct position (GL11.glTranlatef(x, y, z)). Lastly you want to register the item renderer using: MinecraftForgeClient.registerItemRenderer(myItem, new myRenderer()); I'm suggesting you do that in the client proxy.
-
You could use world.scheduleBlockUpdate(), use the onNeighborChanged if you want to update the block while the neighbor blocks are updated (added, removed). If you want a constant tick, I suggest using a tileentity and inside the TileEntity class, add the updateEntity() method. That updates once every tick and is used to make furnaces and other machine update their progress bars and cooking.