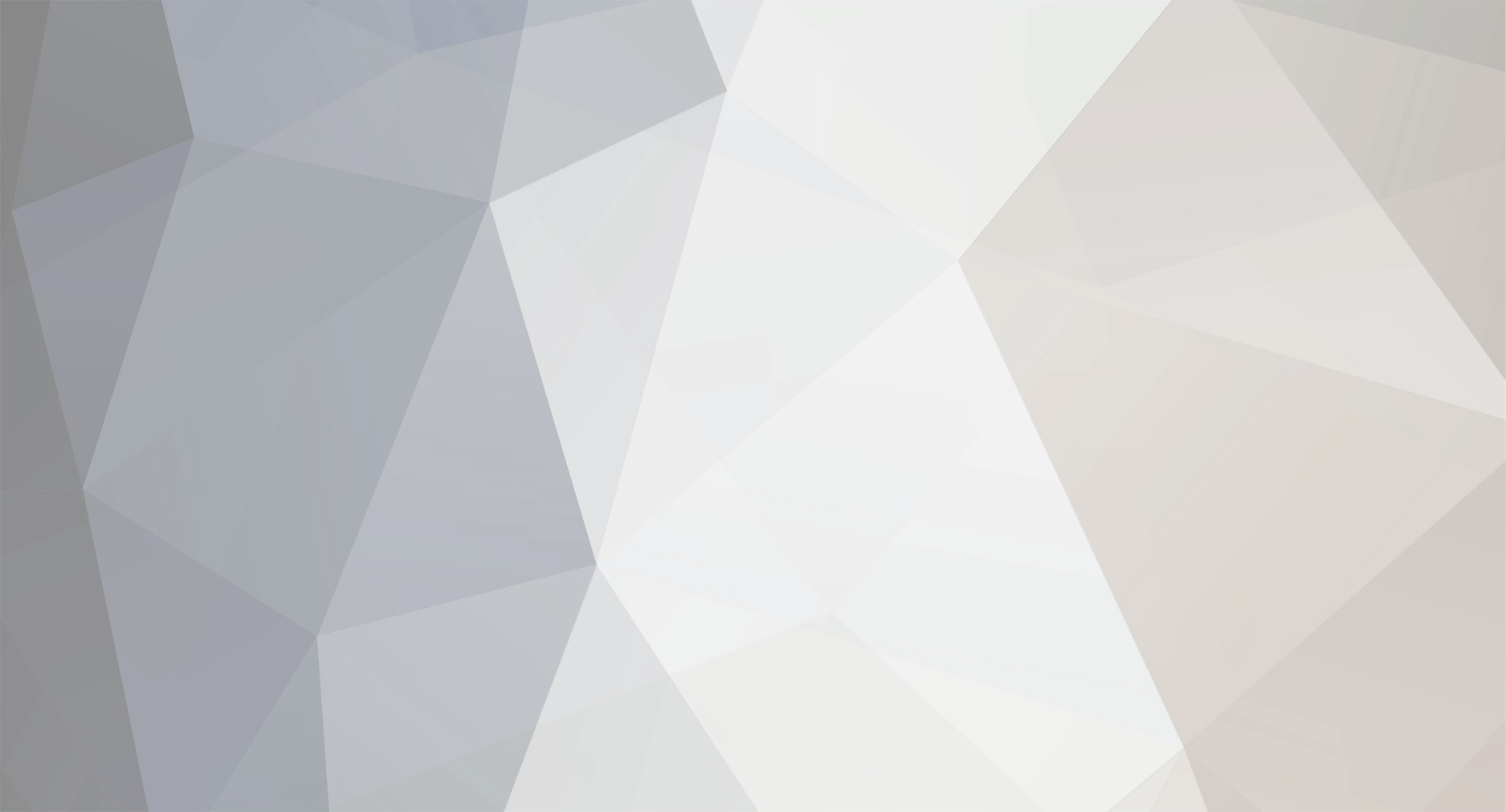
jabelar
Members-
Posts
3266 -
Joined
-
Last visited
-
Days Won
39
Everything posted by jabelar
-
Actually since they are daily snapshots you don't even have to check that list. I just always update it to the date of my build and it works (I guess in some timezones maybe you need to use the date for the day before the build).
-
Why do you think it is a RAM allocation problem? The error is a class def not found error. This is usually a problem with your classpath.
-
[Solved] [1.8] variants: #normal set to null
jabelar replied to LordMastodon's topic in Modder Support
So then you're already set. Just use JSON exports instead of special renderer. -
[Solved] [1.8] variants: #normal set to null
jabelar replied to LordMastodon's topic in Modder Support
Personally, with both the models and the JSONs, they are all just made up of cubes and ultimately are pretty easy to just code directly if you sketch out your idea on paper. It is annoying, but not difficult, and you can get pretty fast at it. Cut and paste, adjust the corners and UV mapping values and you're done. Certainly use a good editor if you find one, but don't be hung up on it either. -
Right. And item stacks have methods, including one called getItem().
-
As a really hacky solution that might work, what if you used the chunk populate event to start a timer, and instead of immediately building your structure, build it some ticks later. I'm not sure if a chunk can be guaranteed to complete generation in a certain number of ticks, but worst case it still might work. Actually, possibly better would be to both build your structure once during the generation and then again after some time. That should avoid making visual glitches most of the times.
-
Are you sure you registered it on the right bus? Can you post your registration code? Also, to eliminate the possibility that it is an issue with your chat handler, can you put in a console statement (with System.out.println) in the beginning of the event handling method to confirm that the event is firing?
-
[1.8] [Solved]Crash on planting custom crop
jabelar replied to Cleverpanda's topic in Modder Support
I think it depends on what you're debugging. If you've already narrowed down an error to very specific part of the code, the debugger can certainly be best way to get full view of what is going on. However in modding I find a lot of things I'm debugging are more general logic related to actual gameplay and it isn't easy to debug if it takes repeated user interaction or it takes multiple ticks for the problem to manifest itself. I find it is really good practice (at least for my own coding purposes) to put logger statements liberally through my code, basically indicating the entry to every key method and at every major decision point. I don't just do it after I notice a problem, rather I use the flow of logs to help confirm "healthy" operation of the code -- i.e. that everything happens in the order I expect. I think this especially important in modding because you often don't control when your methods are called, so you actually learn about the Minecraft functionality (like does a method only get called on one side, does a join event happen before or after a login even, etc.). Anyway, I personally find having real-time feedback to user interaction is key to validating and debugging a lot of problems. But yes, you should also be comfortable with a debugger and know when it is the sharper tool for quickly peeking into specific code areas. -
And...did you Google for UTF-8 in Eclipse? There are tons of hits, especially this one: https://stijndewitt.wordpress.com/2010/05/05/unicode-utf-8-in-eclipse-java/
-
[1.8] [Solved]Crash on planting custom crop
jabelar replied to Cleverpanda's topic in Modder Support
Well, the crash specifically says that it is a null pointer exception in line 61 of the ItemSeeds class. That line of code is: return this.crops.getDefaultState(); For that to return a null pointer exception, it would mean that the crops field is null. The crops field is assigned in the constructor, as the first parameter passed in. The constructor is: public ItemSeeds(Block crops, Block soil) { this.crops = crops; this.soilBlockID = soil; this.setCreativeTab(CreativeTabs.tabMaterials); } Your ItemKernels constructor is: public ItemKernels() { super(GameRegistry.findBlock(Corn.MODID, "corn"), Blocks.farmland); } So that means you're passing GameRegistry.findBlock(Corn.MODID, "corn"). For some reason that is failing and returning null. It does seem that you're registering it in the right order, but there must be something wrong with that. To confirm, can you add a console statement like this after the super call? System.out.println("The corn block is "+GameRegistry.findBlock(Corn.MODID, "corn").toString); I think that should print out what the findBlock is finding (or not finding). If it prints out null, or crashes, at that console print statement then it confirms that it is a problem with looking it up from the registry. -
Right, that's obvious, but how does the client/server work together in SMP and SP? Like in SP, is there a instance of the server and client running?, and if there is a instance of a server and client running, would there be any real difference than running a SMP server, to where the client/server aren't integrated into eachother? I'm just trying to think ahead incase I have to do something special for something to work on SMP if it works fine on SP. Yes, for the most part in SP you can think of it as a "separate" server and client both running in same JVM. That is the one gotcha though -- that it is in same JVM -- because when you get to the topic of sided proxies there are some classes that are only guaranteed to be loaded on one side or the other. In SMP there is different JVM running the server and client so if you don't handle the sided proxy properly it will fail, however such problems can be masked in JVM because each side might still be able to "see" the class loaded on the other side. Anyway, my point is you should test pretty regularly in SMP because SP can mask some issues. Hopefully you see what I mean. By the way, using Eclipse you can launch the server and client (or even multiple clients) simultaneously provided you have enough system memory in your computer. So you can test SMP on single computer. Some people get confused about the idea of sided proxy versus the World#isRemote check. World#isRemote is used in cases where the code may run on both sides and you need to distinguish (this is your case with the onUpdate() method in your question above). Side proxies are used when you need to access classes, methods or fields that are marked @SideOnly and therefore are only guaranteed to load on that side.
-
[1.7.10][semi-solved] Constructing a list of subItems on the server
jabelar replied to wesserboy's topic in Modder Support
Sounds like good progress. Yeah just create a scheme to write any NBT and you're good to go. The way I did it was to put a boolean for every item to indicate whether there was NBT and then if there was then write using ByteBufUtils. Seemed to work. -
On another thread I came up with a way to send information about all the possible item stacks back to the server (since only client generally knows all the available valid subtypes). This could be useful for example if you want to give random items to a player. Anyway, the approach is pretty simple. If the server hasn't asked before, it will send a message that requests the information to all clients. The clients then respond with a message containing the IDs, valid meta data values, and any client-side NBT (some mods like Tinker's Construct seem to distinguish subtypes with NBT instead of metadata). The messages seem to work well and are implemented pretty straight-forward using the simple network wrapper. For the server-side messages I use a scheduled task to avoid thread concurrent modification issues. Anyway, the scheme works except it can give the following error: [Chunk Batcher 0/WARN]: Needed to grow BufferBuilder buffer: Old size 524288 bytes, new size 2621440 bytes. To me that seems like some sort of memory leak. I am reading and writing to buffers, however I don't create any new buffers -- I just operate on the message payload buffers (PacketBuffer) passed into the fromBytes and toBytes methods. So I don't think I should need to release the buffers since I assume the networking stack does it elsewhere. Anyway, I don't know where to look next. Any pointers would be appreciated. Here is the full console log: And you can see my code here. From the console log it seems that the MessageSendItemStackRegistryToServer class seems to be the culprit. https://github.com/jabelar/JadensMod
-
Is this overlay for the block that the player is looking at? In that case I think maybe the DrawBlockHighlightEvent is better to use. You might actually be able to use the DrawBlockHighlightEvent to overlay other blocks in the local area as well.
-
You're missing the } symbol from the end of your JSON to close the variants code block. To learn to find these things yourself, first look a the error. It says that the GSON package (which processes JSON) has found an EOF (end of file) before it expected it at line 11 of your JSON (which is the end). JSON are a bit tricky because your IDE can't warn you about mistakes. But you can validate the format of your JSON with online validator tools like at: http://jsonlint.com/ When I put your JSON code into the validator, it highlights the last line and says: Parse error on line 27: ...t_7" } } ---------------------^ Expecting '}', ',', ']'" So it tells you your JSON isn't correct and that you need one of those symbols. (Note it says line 27 instead of 11 because the validator also re-formats your code nicely). It is a good idea to run all your JSON through a validator tool.
-
[1.7.10][semi-solved] Constructing a list of subItems on the server
jabelar replied to wesserboy's topic in Modder Support
Just use ByteBufUtils to write NBT and read NBT into your packet payload. I've gone ahead and think I have a full working implementation now to make the list, put it into a ByteBuf suitable to be a packet payload and then take that payload and convert it back to a list of ItemStacks, including both metadata and NBT. Here is my code for initializing a public field to contain an "ItemStack registry" and then creating and reading the ByteBuf packet payload. This must go in your client proxy since it calls some client side only methods: I put a lot of console statements to help trace the execution (this is really important in packet payloads because if you make any mismatch in the type or order of data in the payload it will screw up. The results show that it indeed creates a full list (I'm using 1.8 so you'll see multiple variants of stone and such) including a custom item ("sheep skin") that has NBT data. You can see that the final list finds the item with NBT and includes it in the list. First here is the ItemStack list on the sending side: Here you can see it recognizes my item has NBT and what the contents of the NBT are: Here is the contents of the packet payload: Here you can see that the receiving side recognizes my item with NBT and gets the correct NBT content: And finally here is the reconstructed ItemStack list, which you can see perfectly matches the one that was sent: Overall you can see that the code is really quite simple. The main thing is to come up with a mapping into the payload that can be decoded in the far end. The format I used was: a) the Item ID as an int b) the Item metadata as an int c) a boolean for whether or not there is NBT d) the NBT if any -
[1.7.10] isActive state on blocks to spawn particles
jabelar replied to IvanTune's topic in Modder Support
Do what diesieben07 said. But just also wanted to explain that you wouldn't want to use an isActive field in the Block class because that would affect all blocks of that type! You need to understand that there is only one Block class instance in the game, and that actual multitude of blocks in the world are actually just a map of that instance to a block location. Minecraft does allow for a little bit of difference using the block metadata, so potentially you use metadata to do what you want. But really a tile entity is intended to process any more complex information required per block location. Since you already have a tile entity, another approach would be to use the isBurning() method there as that would accurately reflect the state of each furnace separately. But anyway, since you are actually changing the block like vanilla furnace then you don't have to check any field in the block or tile entity -- the block type itself is enough to show that it is a burning furnace. Just wanted to explain some of the concepts related to blocks that have complex functionality. -
Well, you know that when playing Minecraft that using F5 can change the camera view. So start by looking for the code for the KeyBinding for F5.
-
[1.8] Why does armor rendering use ItemStack#isItemEnchanted ?
jabelar replied to coolAlias's topic in Modder Support
Hmmm. I guess that makes sense. What if there was code that used a tick event handler to replace the ItemStack in the player inventory with the custom extended ItemStack? The packets of course would not contain this information, but if both the client and server were replacing the ItemStack it might stay synced. -
[1.8] Why does armor rendering use ItemStack#isItemEnchanted ?
jabelar replied to coolAlias's topic in Modder Support
I have one weird idea that might work as a workaround. I think that the getSubTypes() method is called on all items when they are used on the client. This method provides a list of ItemStacks with quantity 1 for each subtype. I think this may be the source of ALL ItemStacks on the client, since it would make sense that you always need to check subtypes before rendering, creative tab, etc. Now, the problem method in ItemStack() is the isItemEnchanged(). So what if you made a custom class that extends ItemStack that overrides the isItemEnchanted() method to return false? Then in the getSubTypes() method for your custom Item, for the base item return the custom ItemStack and return regular ItemStacks for the rest? Just possibly might work with only a dozen lines of code. -
Why don't you look at how the camera gets changed when you switch to 3rd person view? For most cases where people ask "how can I do this", they should look at the Minecraft and Forge source to see how the closest vanilla method wordks and then see if you can adapt it.
-
The FMLProxyPacket has a constructor that takes the ByteBuf payload and a string for channel name. Before the SimpleNetworkWrapper was working, I actually made a packet system using FMLProxyPackets. I had a tutorial here: https://www.blogger.com/blogger.g?blogID=8871285205929815684#editor/target=page;pageID=4638645929701985216;onPublishedMenu=pages;onClosedMenu=pages;postNum=23;src=pagename That tutorial should show how to send and receive FMLProxyPackets. What are you trying to achieve? You realize that it might be a LOT of data to store...
-
[1.7.10][semi-solved] Constructing a list of subItems on the server
jabelar replied to wesserboy's topic in Modder Support
Okay, now that I look at it further you're right. I guess it is just a matter of extending the concept to check for NBT as well, and adding that to the buffer. I'll look at an implementation. -
[1.8] [RESOLVED] return blockPos of clicked spot- even if it is air
jabelar replied to DJD's topic in Modder Support
If you just always want the block immediately in front of the player, then you can just add the look vector to the player position. You might have to adjust a bit for eye height and such. The look vector is by definiton one block in length, so probably the right length, but you might want to multiply it a bit to make sure you get the block you want. -
You could just try to make it always move to the surface and it might work okay. You'd just check if the block at the position of the entity's feet is a fluid and if it is you'd move the entity up to point where it would be on top. I don't know how glitchy that would look, and as mentioned you might need to modify the AI and navigator to make it move in a sensible way while on the water. If that doesn't work you might, to make your entity to "walk" on water it might be easiest to actually make it technically a flying entity (extend EntityFlying like a Ghast) and prevent it from flying above the ground. That would be a fair bit of work, but probably more straight-forward than trying to prevent the normal sinking into water since that involves gravity and the navigator and things like that. You have a lot more control over the movement of an EntityFlying than an EntityMob or EntityCreature.