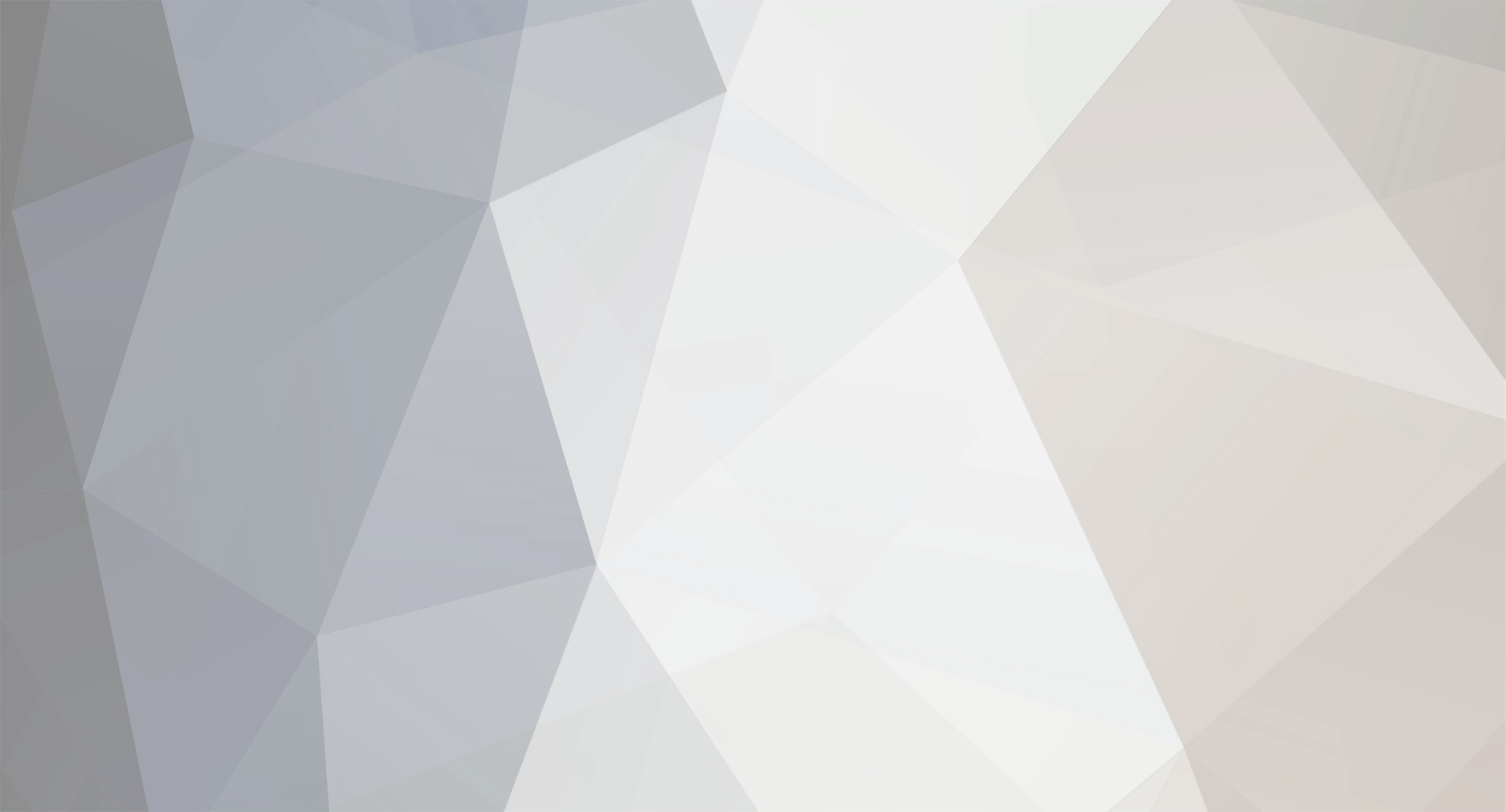
Sear_Heat
Members-
Posts
41 -
Joined
-
Last visited
Everything posted by Sear_Heat
-
Giving players potion effects when hit by a projectile
Sear_Heat replied to Sear_Heat's topic in Modder Support
Ok, but what is LivingHurtEvent? I can't seem to find a class or a (static) method by that name. -
What code should I add to a projectile to make it give a hit player a potion effect?
-
This should fix it. First, you should try changing your block file code to "public static BlockDarklandPortal darklandPortal = new BLockDarklandPortal()" I have previously had trouble with methods in parenthesis before itself. Then, for your code: public BlockDarklandPortal() { super("darklandPortal", Material.portal, false); this.setTickRandomly(true); setBlockName("darklandPortal"); setBlockTextureName( [YOURMODSCLASS].MODID + ":" +"textures/portals/darklandPortal.png"); setCreativeTab(CreativeTabs.tabBlock); GameRegistry.registerBlock(this, "darklandPortal"); } where [YOURMODSCLASS] would be replaced by whatever file contains your mod's mod ID (MODID). Note that I added .png to the end of your filename. Also, if you still have trouble but it is looking in the correct folder, make sure png is not capitalized (PNG). I have learned the hard way that that will break the texture.
-
I did. I actually solved the button problem by doing *gasp* nothing. I just tried gradlew building it again and it worked fine. Weird.
-
I think I see the problem. I did not use drawButton, only this.buttonList.add.
-
How are buttons in a GUI set up? Simple question, but I'm confused. Also, I have a deadline in 4 hours. [move][/move]
-
Ok. But... [move]THIS ARTICLE CAME VERY CLOSE TO BEING A HOT TOPIC![/move]
-
Bump. Sorry I'm bumping so much but I have to fix this by 2:00 Pacific time. That's 5 hours till deadline.
-
You have to be patient. For me it takes 5 minutes saying "Verifying Application Requirements..." before it actually starts up.
-
Wuppy's setup is a great setup, It makes everything a whole crapload easier. I've tried both ways. Also, I've heard the normal setup does not work for some people due to eclipse freaking out.
-
It fixed it... partially. The GUI will open and close now, but buttons don't do ANYTHING. So.. How do I set the texture of a button?, and How do I see if it is working?
-
This video helped me:
-
Looks like you need a common(server) proxy called CommonProxy in net.HogansCraft.mod.proxy. Here is some example code: package net.HogansCraft.mod.proxy; public class CommonProxy { }
-
If I were you, I wouldn't do that. Really. I strongly advise against it. However, if you must; There is editable code. This is how you get to it: In eclipse, look for the "build" folder. Navigate to "tmp", then to "recompSrc". Inside there, there is a huge repository of editable files. The Minecraft folders are located in recompSrc's "net", then "minecraft" folders. I don't know whether these are the actually running files when you start Minecraft in eclipse, or if it is the folders in forgeSrc in "Referenced Libraries" that contains uneditable code. Either way, the code in recompSrc is fully editable.
-
bump bump BUMP, this is very urgent! [move]SOMEBODY HELP ME PLEASE[/move]
-
Launch fireworks from player's location (and one other small question)
Sear_Heat replied to Zer0HD2's topic in Modder Support
Please paste the code that you used for leveling. I think I may have an idea. -
I have a bug when I open my GUI (or try to). IT DOESN'T WORK AND CRASHES THE GAME! Here is my relevant code= NetworkProxy: package com.sear.tutorial.proxy; import com.sear.tutorial.Tutorial; import com.sear.tutorial.gui.GuiHandler; import cpw.mods.fml.common.network.NetworkRegistry; public class ServerProxy { public void registerRenderThings(){ } public void registerNetworkStuff(){ NetworkRegistry.INSTANCE.registerGuiHandler(Tutorial.modInstance, new GuiHandler()); } } GuiHandler: package com.sear.tutorial.gui; import scala.Console; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.World; import com.sear.tutorial.Tutorial; import com.sear.tutorial.gui.containerMagicGui; import cpw.mods.fml.common.network.IGuiHandler; import cpw.mods.fml.common.network.NetworkRegistry; public class GuiHandler implements IGuiHandler{ public GuiHandler(){ NetworkRegistry.INSTANCE.registerGuiHandler(Tutorial.modInstance, this); Console.out().println("RUBIES: GuiHandler registered."); } @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID == 0){ return new containerMagicGui(player); } else{ return null; } } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID == 0){ return new magicGui(player); } else{ return null; } } } MagicGui: package com.sear.tutorial.gui; import org.lwjgl.opengl.GL11; import scala.Console; import com.sear.tutorial.Tutorial; import com.sear.tutorial.magic.spells; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiControls; import net.minecraft.client.gui.GuiScreen; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.ResourceLocation; public class magicGui extends GuiScreen{ ResourceLocation texture = new ResourceLocation(Tutorial.MODID, "textures/gui/magicGui.png"); public final int xSizeOfTexture= 200; public final int ySizeOfTexture= 500; public magicGui(EntityPlayer player){ } @Override public void drawScreen(int x, int y, float f){ drawDefaultBackground(); GL11.glColor4f(1F, 1F, 1F, 1F); Minecraft.getMinecraft().getTextureManager().getTexture(texture); int posX = (this.width - xSizeOfTexture) / 2; int posY = (this.height - ySizeOfTexture) / 2; drawTexturedModalRect(posX, posY, 0, 0, xSizeOfTexture, ySizeOfTexture); super.drawScreen(x, y, f); Console.out().println("RUBIES: MagicGUI screen drawn."); } @Override public boolean doesGuiPauseGame(){ return false; } public void initGui(){ int posX = (this.width - xSizeOfTexture) / 2; int posY = (this.height - ySizeOfTexture) / 2; this.buttonList.add(new GuiButton(1, posX, posY, 200, 20, "Fireball")); this.buttonList.add(new GuiButton(2, posX, posY + 20, 200, 20, "Ice Blast")); this.buttonList.add(new GuiButton(3, posX, posY + 20, 200, 20, "Weak Heal")); this.buttonList.add(new GuiButton(0, posX, posY + 20, 200, 20, "No Spell...")); } public void actionPerformed(GuiButton button){ switch(button.id){ case 0: spells.setActiveSpell(0); case 1: spells.setActiveSpell(1); case 2: spells.setActiveSpell(2); case 3: spells.setActiveSpell(3); default: Minecraft.getMinecraft().thePlayer.sendChatMessage("I am really good at breaking things!"); } Console.out().println("RUBIES: MagicGUI Switch completed."); } } ContainerMagicGui: package com.sear.tutorial.gui; import scala.Console; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.Container; public class containerMagicGui extends Container{ public containerMagicGui(EntityPlayer player){ Console.out().println("RUBIES: Dunno what this is... some server-side GUI mumbo-jumbo? But it's loaded, and that's the point."); } @Override public boolean canInteractWith(EntityPlayer var1) { return true; } } And the class that calls all this: package com.sear.tutorial.magic; import scala.Console; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import com.sear.tutorial.Tutorial; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class ItemWarlockSpellbook extends Item { public boolean hasEffect(ItemStack par1ItemStack){ return true; } public ItemWarlockSpellbook(){ super(); setUnlocalizedName("ItemWarlockSpellbook"); setTextureName(Tutorial.MODID + ":" + getUnlocalizedName().substring(5)); setCreativeTab(Tutorial.tabMagic); setMaxStackSize(1); } @Override public ItemStack onItemRightClick(ItemStack itemstack, World world, EntityPlayer player) { Console.out().println("RUBIES: GUI is attempting to open..."); player.openGui(Tutorial.modInstance, 0, world, (int)player.posX, (int)player.posY, (int)player.posZ); Console.out().println("RUBIES: GUI should have opened, check console."); return itemstack; } @Override @SideOnly(Side.CLIENT) public void registerIcons(IIconRegister icon) { this.itemIcon = icon.registerIcon(Tutorial.MODID + ":" + getUnlocalizedName().substring(5)); } } It has me stumped... Can anyone figure out why this is happening?
-
If you create a whole new item that works the same as a mob egg but is in a different creative tab, yes. The vanilla eggs will always be in Miscellaneous. This is what I would do for the new code for the egg item (This was written on the fly and may have errors):
-
Spawning Entity at player through keybind press
Sear_Heat replied to Sear_Heat's topic in Modder Support
just have one last question... what is the difference between the sendToServer and getPacketFrom methods? -
Spawning Entity at player through keybind press
Sear_Heat replied to Sear_Heat's topic in Modder Support
I was wondering, how do I do this implementing simpleimpl? I have the simpleimpl structure ready and don't know how to setup all the PacketPipelines and AbstractPackets. -
Spawning Entity at player through keybind press
Sear_Heat replied to Sear_Heat's topic in Modder Support
THANK YOU, this was a great explanation and exactly what I was looking for. -
I have created an abomination (custom entity model)
Sear_Heat replied to Zer0HD2's topic in Modder Support
I had a similar problem, but I solved it by removing some animations. Under setRotationAngles try removing different lines of code and see if it fixes the problem. -
Spawning Entity at player through keybind press
Sear_Heat replied to Sear_Heat's topic in Modder Support
How should I use the packets? I have the handler set up but because the lack of a tutorial I do not know how to communicate to the server to spawn an entity. -
Spawning Entity at player through keybind press
Sear_Heat replied to Sear_Heat's topic in Modder Support
I don't want to get too involved in this, just spawning an entity. It works fine with my own bow class where I did the same thing without needing any packets or server-side work.