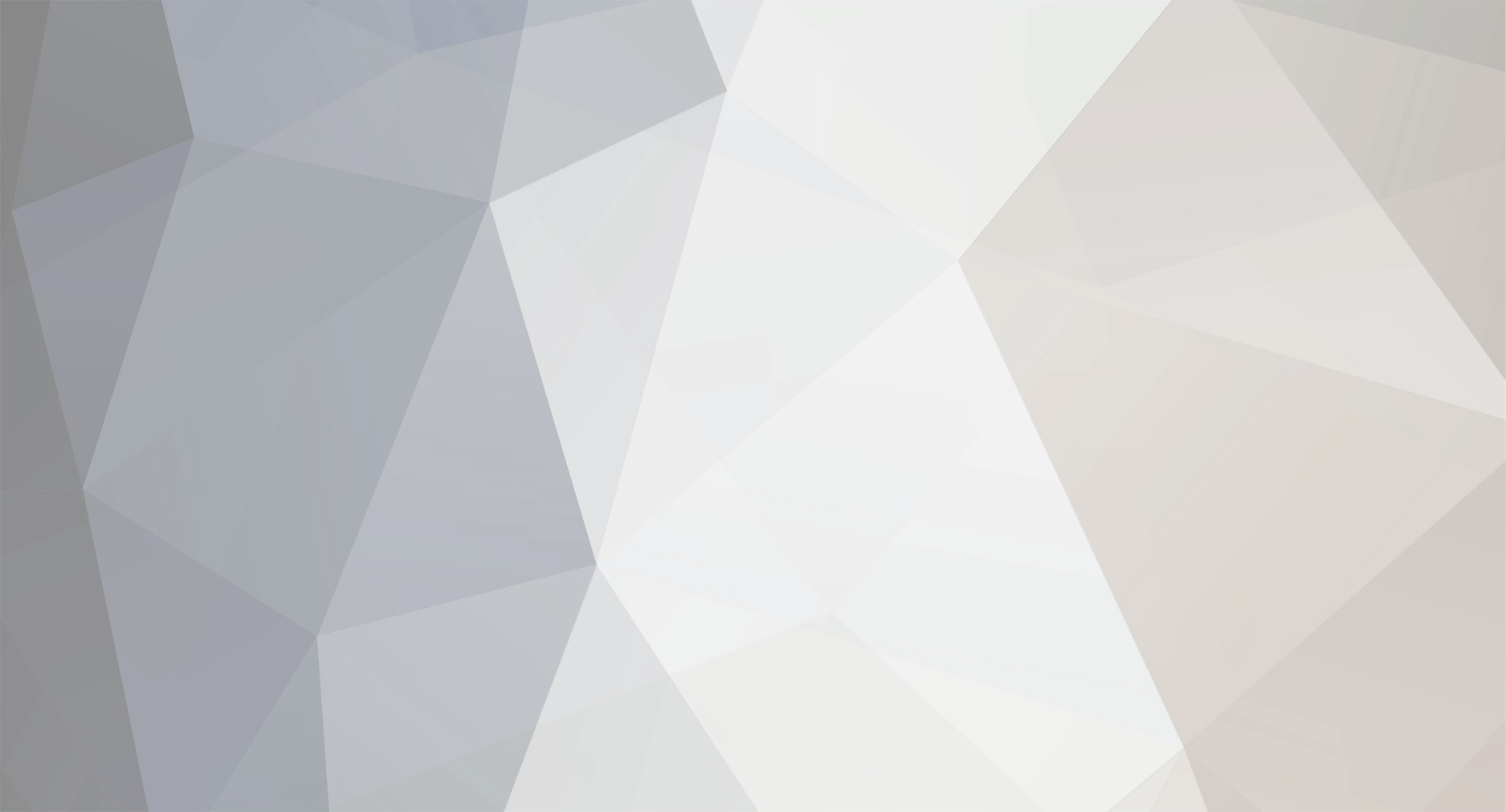
Notunknown
Members-
Posts
156 -
Joined
-
Last visited
Everything posted by Notunknown
-
Check if their sunlight is below a certain threshold (I would say about 10). However, being inside would trigger it. You may have to do other checks, such as Y-level (if you can, base it off sea level) and checking their surroundings (you would only need to do this once per second at most).
-
PlayerEvent.Clone was not working on the client thread, and EntityJoinWorldEvent was not working for sending from the server thread, for some reason.
-
Whenever I respawn I would lose all the capability data, unless there is an event I am missing.
-
This is why I need to stop using literals as unique ids... As it turns out I had registered both my messages under the same id. I am now using a counter and incrementing.
-
I am having another issue: I am having the client send a request to the server, and the server is supposed to respond with a response message. However, it then proceeds to crash. For some reason, the request message is being re-processed (I think) on the incorrect side (I think). I have no idea what is happening. package com.phantasma.phantasma.network; import io.netty.buffer.ByteBuf; import net.minecraftforge.fml.common.FMLCommonHandler; import net.minecraftforge.fml.common.network.simpleimpl.IMessage; public class RequestMessage implements IMessage { public RequestType type; public RequestMessage() { } public RequestMessage(RequestType type) { this.type = type; } @Override public void fromBytes(ByteBuf buf) { int ordinal = buf.readInt(); //java.lang.IndexOutOfBoundsException: readerIndex(0) + length(4) exceeds writerIndex(2): SlicedByteBuf(ridx: 0, widx: 2, cap: 2/2, unwrapped: UnpooledHeapByteBuf(ridx: 0, widx: 3, cap: 256)) this.type = RequestType.values()[ordinal]; } @Override public void toBytes(ByteBuf buf) { int ordinal = this.type.ordinal(); buf.writeInt(ordinal); } public static enum RequestType { RESEARCH } } package com.phantasma.phantasma.network; import com.phantasma.phantasma.Phantasma; import com.phantasma.phantasma.network.RequestMessage.RequestType; import net.minecraft.entity.player.EntityPlayer; import net.minecraftforge.fml.common.network.simpleimpl.IMessage; import net.minecraftforge.fml.common.network.simpleimpl.IMessageHandler; import net.minecraftforge.fml.common.network.simpleimpl.MessageContext; import net.minecraftforge.fml.relauncher.Side; public class RequestMessageHandler implements IMessageHandler<RequestMessage, IMessage> { @Override public IMessage onMessage(RequestMessage message, MessageContext ctx) { if (ctx.side == Side.SERVER) { EntityPlayer player = Phantasma.proxy.getEntityPlayer(ctx); switch(message.type) { case RESEARCH: { return new ResearchMessage(player.getCapability(Phantasma.RESEARCH_CAP, null).serialize()); } } } return null; } }
-
Thanks! I was going to ask about this once I had fixed the bugs, but its good to know in advance.
-
I think I may need to do this in reverse, use EntityJoinWorldEvent on the client to request that the data be sent. I should be able to do that, if I make it serializable.
-
The sending of the message is complicated. The code which initiates the sending of the message is some test code in an EntityJoinWorldEvent, which ensures that the logical side is SERVER. The part which actually sends the code is: NETWORK_WRAPPER.sendTo(new ResearchMessage(research, state), (EntityPlayerMP)player);
-
As it turns out it was succeeding because of a bad if-statement. Same scenario. public class ResearchMessageHandler implements IMessageHandler<ResearchMessage, IMessage> { @Override public IMessage onMessage(final ResearchMessage message, MessageContext ctx) { Minecraft.getMinecraft().addScheduledTask(new Runnable() { @Override public void run() { EntityPlayer player = Minecraft.getMinecraft().thePlayer; if (player.hasCapability(CommonProxy.RESEARCH_CAP, null)) { IPlayerResearchCapability cap = player.getCapability(CommonProxy.RESEARCH_CAP, null); cap.setResearchState(null, message.research, message.state); } } }); return null; } }
-
Never mind - my eyes started working again. Got it, thanks!
-
When the packet arrives the player has not yet respawned - resulting in a null pointer exception.
-
I am having trouble syncing Capabilities. So in PlayerEvent.Clone I set a field in a capability. This field change in a capability causes it to send data to the client (if logically on the server) using my packets. However, I must be doing something wrong, because it isn't changing. Here is my message handler (it is intended solely for the logical client): public class ResearchMessageHandler implements IMessageHandler<ResearchMessage, IMessage> { @Override public IMessage onMessage(ResearchMessage message, MessageContext ctx) { EntityPlayer player = Minecraft.getMinecraft().thePlayer; if (player.hasCapability(CommonProxy.RESEARCH_CAP, null)) //This is true { IPlayerResearchCapability cap = player.getCapability(CommonProxy.RESEARCH_CAP, null); cap.setResearchStateNoCheck(null, message.research, message.state); //This works successfully. } return null; //Here it still works. But after here the changes mysteriously vanish. } }
-
Yeah, I just remembered about the docs. One thing: Is my capability supposed to extend something? That was not made all that clear.
-
Will I need to take any additional actions to sync client and server data, or do capabilities automatically do that?
-
I am creating a Thaumcraft-style documentation book for my mod, where players research entries before being granted access. However, I am not certain on how to proceed. Obviously I need to store the data on the server and download it to the client when required. Should I be using capabilities, or something else entirely?
-
Its a good thing that Java has a way to negate the purpose of private
-
[1.9] [UNSOLVED] Let entities be thrown into my block
Notunknown replied to Bektor's topic in Modder Support
Vectors are why I want operator overloading in Java. -
Why has nobody mentioned Item#addInformation?
-
[1.8.9] Multiple item textures in one file (image)
Notunknown replied to robmart's topic in Modder Support
Because I have a lot of items that change texture depending on the metadata. I figured it would be a lot cleaner to have it this way then to have 17 different image files. You can put all the textures relating to an object in their own folder (I do this for complex models) -
[1.8.9] Multiple item textures in one file (image)
Notunknown replied to robmart's topic in Modder Support
Why can you not split it into two 16x16 files? -
dispenser noteblock bed brick_block mob_spawner oak_stairs chest crafting_table furnace lit_furnace standing_sign oak_door spruce_door birch_door jungle_door acacia_door dark_oak_door ladder stone_stairs wall_sign iron_door oak_fence birch_fence jungle_fence dark_oak_fence acacia_fence trapdoor stonebrick brown_mushroom_block red_mushroom_block iron_bars oak_fence_gate spruce_fence_gate birch_fence_gate jungle_fence_gate dark_oak_fence_gate acacia_fence_gate brick_stairs stone_brick_stairs nether_brick nether_brick_fence nether_brick_stairs enchanting_table brewing_stand cauldron end_stone double_wooden_slab wooden_slab sandstone_stairs ender_chest spruce_stairs birch_stairs jungle_stairs cobblestone_wall anvil trapped_chest light_weighted_pressure_plate heavy_weighted_pressure_plate daylight_detector daylight_detector_inverted redstone_block hopper quartz_block quartz_stairs dropper stained_hardened_clay iron_trapdoor hardened_clay coal_block acacia_stairs dark_oak_stairs prismarine sea_lantern standing_banner wall_banner red_sandstone_stairs double_stone_slab2 stone_slab2 chorus_plant chorus_flower purpur_block purpur_pillar purpur_stairs purpur_double_slab purpur_slab end_bricks
-
Oh, right. I was going to use that but an error occured because CreativeTabs is abstract, didn't notice and changed the constructor. I must have forgotten to change it back when I realised it was abstract.
-
The 1.8 tutorials should work in 1.9 But here, I use this: TAB = new CreativeTabs(MOD_ID + ":" + TAB_NAME) { @Override public Item getTabIconItem() { return TAB_ICON; } }; in FMLPreInitializationEvent, before initializing anything else.
-
I was under the assumption that items would automatically get models added for them (like blocks seem to do) unless ModelLoader.setCustomModelResourceLocation is called. Was I wrong about this and all items require ModelLoader.setCustomModelResourceLocation to be called on them in order to work, or have I done something wrong somewhere? It is just that I have a lot of items and blocks which need ItemBlocks, and adding them all will be a pain.
-
This is also true for Crafting Benches, and probably other wooden blocks. This is especially serious as you cannot extend ItemAxe, and thus cannot use Block#getHarvestLevel to check if your custom axe can mine a block without risking mod incompatibility.