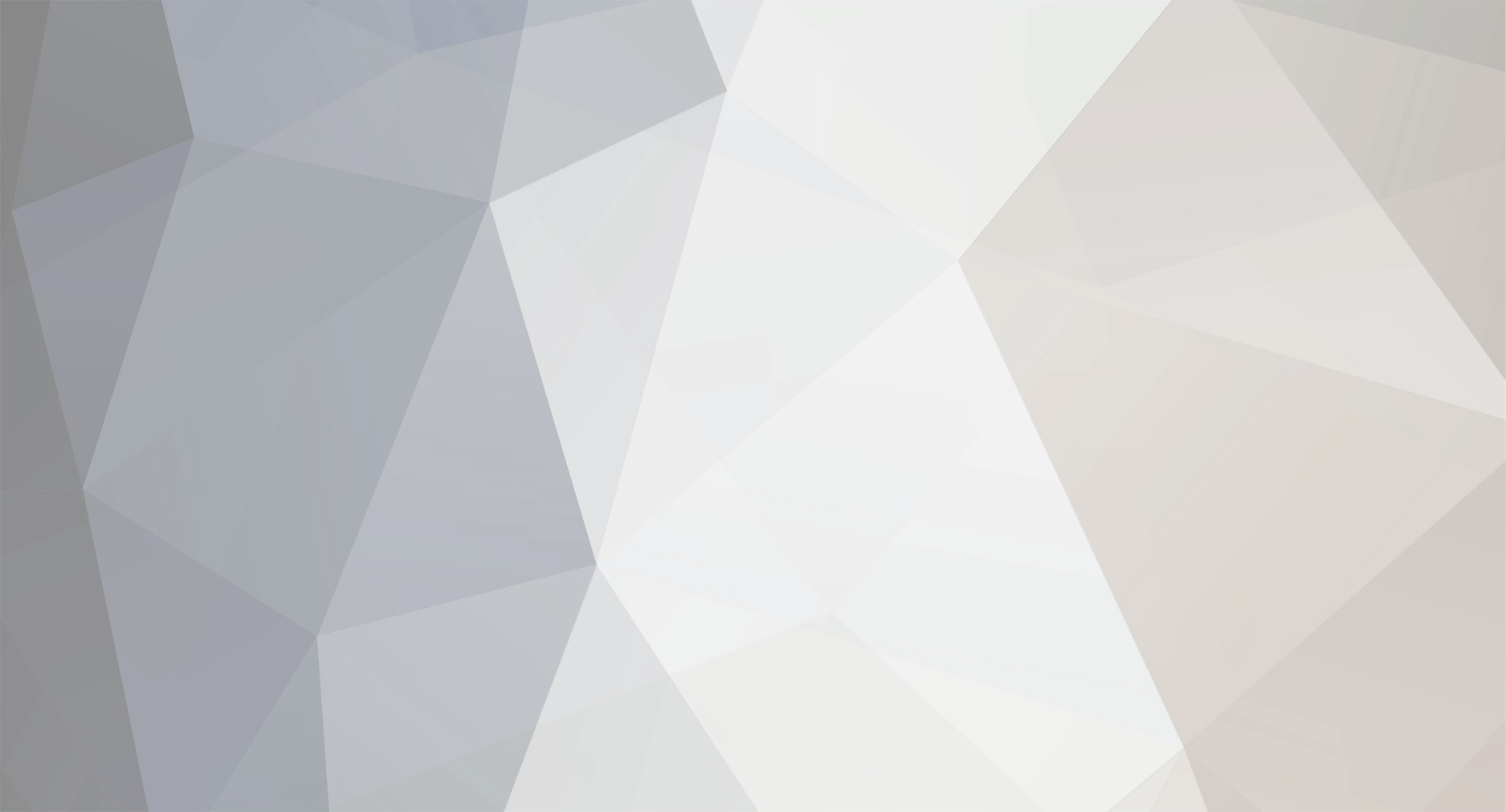
Notunknown
Members-
Posts
156 -
Joined
-
Last visited
Everything posted by Notunknown
-
Oh, I thought he wanted a block which contains an item. I guess that you probably would not need a TileEntitySpecialRenderer in either case. But it is easier than trying to do it with models.
-
You need a TileEntity and a TileEntitySpecialRenderer. The TileEntitySpecialRenderer renders the item inside the block, and the model renders the block itself.
-
As for rendering the item itself, I use this method: GlStateManager.pushMatrix(); GlStateManager.translate((float)x + 0.5F, (float)y + 0.5F, (float)z + 0.5F); Minecraft.getMinecraft().getRenderItem().renderItem(ITEM, TransformType.GROUND); GlStateManager.popMatrix(); Not quite sure if this is the best method for doing this, but it does work perfectly fine. Of course you will need to replace ITEM with an ItemStack.
-
I found a workaround, in the event that I cannot find a proper method: I used GuiScreenEvent#DrawScreenEvent and recreated GuiUtils#drawHoveringText , removing all the rendering code (this gets me the X and Y of the tooltip, as well as its size). Its a bit messy, but it works for my purposes.
-
That allows me to add to a tooltip (I am using that event) but it does not allow me to render additional information - such as what Thaumcraft does with its aspects.
-
Which event do I need to handle rendering with Tooltips? Its for something a little similar to Thaumcraft's Aspect display above the tooltip.
-
[UNSOLVED][1.7.10] Better Version of Is Tool Effective?
Notunknown replied to DanielDawn's topic in Modder Support
ItemPickaxe should have a method in it which works fine. After all, the pickaxe can harvest the stone just fine. -
[1.9] Getting the recipe of itemstacks that make up an item!
Notunknown replied to madcrazydrumma's topic in Modder Support
If its just your own item can't you just store the recipe somewhere? -
Some blocks do not respond correctly to getHarvestLevel - I find that it is mostly the various Stone based blocks, having no proper harvest tool.
-
[UNSOLVED][1.7.10] Better Version of Is Tool Effective?
Notunknown replied to DanielDawn's topic in Modder Support
I was not quite sure what you were asking. To be honest I am still not 100%, but do you want ItemPickaxe#canHarvestBlock(IBlockState) ? -
[UNSOLVED][1.7.10] Better Version of Is Tool Effective?
Notunknown replied to DanielDawn's topic in Modder Support
You are checking world.getBlock(x, y, z).isToolEffective("pickaxe", 3) , right? If so, then world.getBlock(x+1, y, z).isToolEffective("pickaxe", 3) is redundant, as you also check that world.getBlock(x, y, z) and world.getBlock(x+1, y, z) are the same block (and if the pickaxe is effective against the block it is effective against the block). -
Wait - I just found font/glyph_sizes.bin. But what does this do? I can only see one of them - does it need to contain information about all the fonts? How can I add to this file?
-
It IS defined - and is a valid ResourceLocation. There is no log because there is no error - the font just wont render. I think it is something around creating fonts which I don't understand - maybe fonts require a special png format?
-
Okay, this is really annoying me. Currently I have managed to do it, but it seems really inefficient. ModelLoaderRegistry.getModel(new ResourceLocation(model_location)).bake(new SimpleModelState(ImmutableMap.<IModelPart, TRSRTransformation>of()), DefaultVertexFormats.ITEM, ModelLoader.defaultTextureGetter()); I have gotten it to work for models which belong to pre-existing items with this, but when I try to load a model which does not belong to an actual item it returns the default model (the pink/black cube thing) Minecraft.getMinecraft().getRenderItem().getItemModelMesher().getModelManager().getModel(model_location); What am I missing? There must be a method that I have simply overlooked...
-
This is really annoying me. I am attempting to add a new "runes" based font to the game, but I am really struggling to get it to work. It only adds a-zA-Z, but no matter what I try it just fails to render anything. I am putting this at the top of my method (so I can change it easily) and, well, nothing renders (except for special characters, such as \20. But I didn't add those, so I am guessing Minecraft did.) This is me attempting to create the font: final FontRenderer runeFont = new FontRenderer(Minecraft.getMinecraft().gameSettings, FONT_TEXTURE_ASCII, Minecraft.getMinecraft().renderEngine, false);
-
You can change/disable that feature, but you probably shouldn't.
-
[1.8] how to change entity name with color
Notunknown replied to thvardhan's topic in Modder Support
I am quite sure that "" + ChatFormatting.BLACK + name should work. -
Sorry to bump this, but is there any info on this sort of thing? I have gotten it to (in theory) work, but additional input would be greatly appreciated!
-
Ok, so I think I have gotten the basics down. I just need some help with getting models from ModelResourceLocations, or preferably a String. I may also need help with getting custom data from a model's json.
-
ScreenGui#drawScreen draws all the buttons and labels. If you use those you would probably want to call it last, to prevent them from being behind background objects.
-
Okay, so I believe that I have gotten the basic system set up. Just a few questions: 1: How can I have a texture stitched into the texture atlas without an associated item or block to go with it (or does it automatically do this with everything in textures/items?) 2: How can I load a model without an associated item or block (for components to be used in composite models) (again, does it automatically do this with everything in the models folder?) 3: How can I load non-standard data from a model file (for use in composite models, things like relative translation and scales)? Edit: For (2), I basically want to turn a ModelResourceLocation into an IBakedModel. My attempts are failing... :'(
-
[1.9] Code-based item rendering for when held in hand
Notunknown replied to Notunknown's topic in Modder Support
Ah - well that is what I was asking - if it were possible to do rendering in GL for items for items which require freaky effects to pull off. -
What is wrong with using an NBTTagCompound either way, and just converting the keys to strings in a way which can be reverted?
-
Ok, so I am working on a mod which requires composite models (item models made from a set of sub-models joined together). Now, I am on track for getting a basic composite model which would fit my minimum requirements, but as I do not know enough at the moment about how models (and mostly quads) work, I am asking about some information regarding the power of models. Composite Models MUST have the following: *MUST be able to be formed from two or more models, the number and type of which are determiend by an input ItemStack. (This I believe I can do.) Composite Models SHOULD have the following: *SHOULD be able to define arbitrary rotations relative to "base" rotation, which is determined by the model determined by the input ItemStack. *SHOULD be able to define arbitrary translations relative to "base" translation, which is determined by the model determined by the input ItemStack. (This I believe I can do.) Composite Models MAY have the following: *MAY be able to define arbitrary scales relative to "base" scale, which is determined by the model determined by the input ItemStack. (This I believe I can do.) *MAY be able to define arbitrary rotations, translations and offsets relative to model relative rotation, translation and offset, which is determined by code determined by the input ItemStack. *MAY be able to specify a simple hierarchy within models, which is used to allow models to share with their parents relative rotation, translation and scale. For example, I might want to have a magical stone. The stone is made of three models, a core, a glyph and a ring. The core is the base model (determines the rotation, translation and scale relative to the hand), the glyph shares its rotation and translation with the core but has twice the scale (causing it to stick out) and the ring has the same translation and scale as the core but is rotated 90° to the core. The stone also gently floats above the players hand following a sine wave (giving it a floating effect), the core and glyph additionally slowly rotate clockwise at the same rate, and the ring rotates counter-clockwise at a faster rate (giving it a spinning effect). When right clicked the entire model would increase in scale, then decrease again, following a simple curve. So, before I waste time trying something which is impossible, which of those requirements are possible and which aren't?