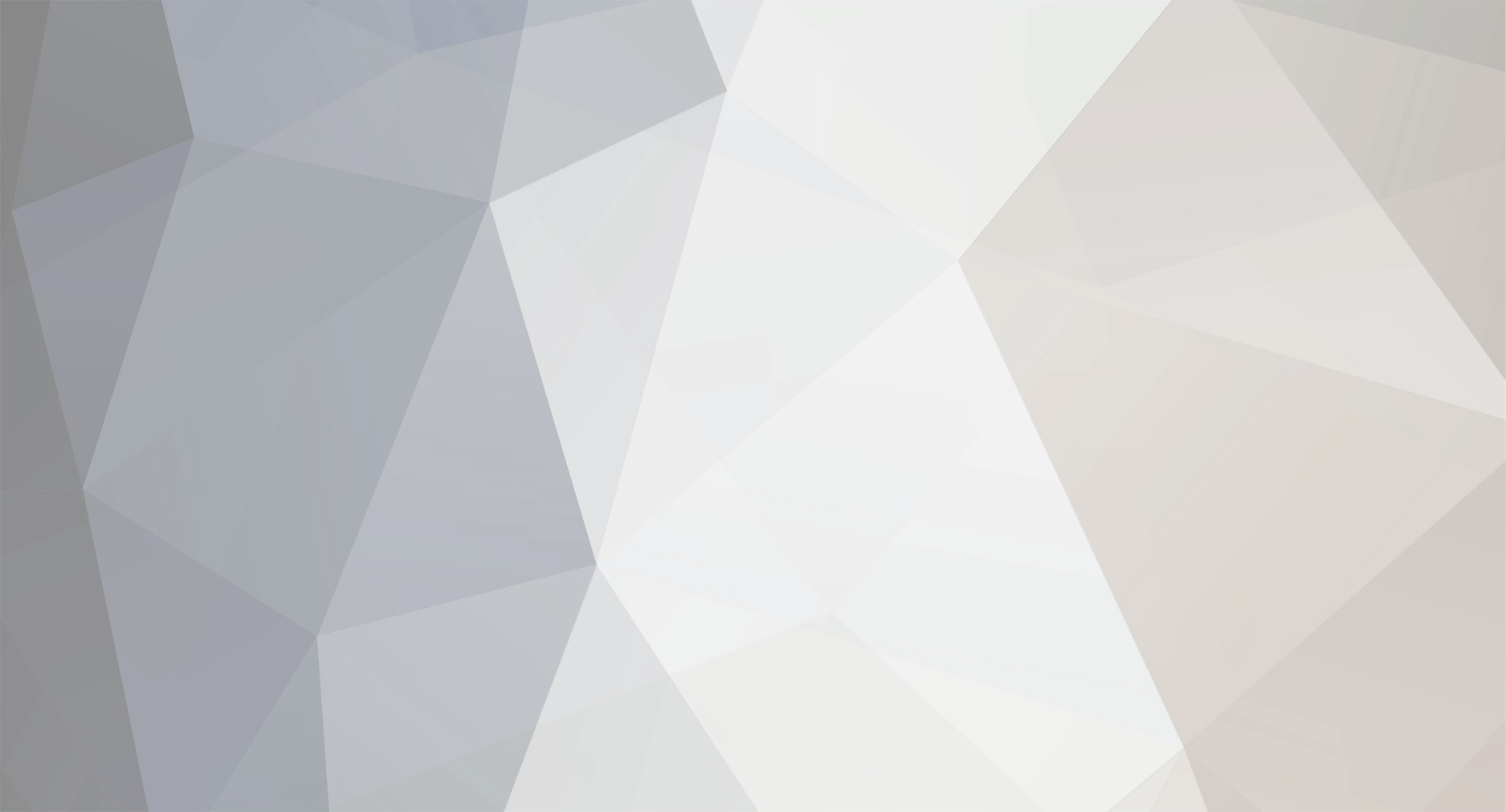
N247S
Forge Modder-
Posts
222 -
Joined
-
Last visited
Everything posted by N247S
-
I think a better way to do is, is by using no key registration at all. But check if the player is crouching when your EntityInteract event method is called. (event.entityPlayer.isCrouching(), ore something like that). Unless of course you really want to bind a specific key!
-
[1.8.8] Questions about API creation for a mod
N247S replied to HappyKiller1O1's topic in Modder Support
If people want to use your API as an optional option, they can use the @Optional annotation so forge knows not to crash when your API is missing. So no need to worry about that. -
Propably the easiest way is to clone/fork the github repository, and save it localy on your computer. The next step is creating a new project in eclipse with the folder of the cloned repository as main directory. When you got that setup, you only need to refer it as a dependency in eclipse and in the gradle file! Instead of saving a cloned repository, you can unpack the zipfile instead and do the same things as described above. Though updating the API is a bit less easier this way.
-
As OreCruncer said, you need to spawn the particles through: Minecraft.getMinecraft().effectRenderer.addEffect(new myParticle([param]));. On little side note, I would use FXLayer 3 instead of 1. For some reason MC is not capable of handling texture changes, unless you use the vanilla particleTextures file. Meaning that if you were about to spawn the particle, and its raining at the same time for example, all the rain particles would be rendered with the vanilla texture + your customParticleTexture.
-
[1.8-11.14.4.1563] How do I implement logging?
N247S replied to paradoxbomb's topic in Modder Support
This will do. Dont forget to replace the imports! Logger mylogger = LogManager.getLogger("myLoggerName"); -
There is a FluidRegistery wich should be self explaining enough to help you further. You need to create a new Fluid instance and register that through the FluidRegistery during PreInitialization. Take a look at the vanilla water and lava classes for an example.
-
Error when making the game go to the next shader
N247S replied to The_Fireplace's topic in Modder Support
This might be caused becuase your code is handled by a non opengl thread. Meaning that opengl will complain about that. And from the top of my head, messages/packets are handled in a side thread in MC 1.8. So maybe bringing it back to the mainThread would work? (Im not sure wich thread you need) -
Im not verry familar with tabula, though techne for sure (and I think tabula as wel) is only a program that creates models without specifiing what type of renderer it uses. So you can use it from a tileEntity to a mobEntity. How you should render the model? I can't tell you right now since Im not at my IDE, though it heavilly depends on the mc version you are modding for. Maybe take a look at the tutorials for examples on this.
-
I think you should be able to catch the mouse event before the methods inside the MouseHelper class are called. If I remember correctly those methods are directly called from the #doTick() method.(not at my IDE atm so not sure). So if there is a tick type event fired before the mouseInputs are handled, you can simply subscribe to that event and catch the mouse i put events from lwjgl directly(instead of blocking vanilla events), so that vanilla has no events to proces after the tick event has been fired.
-
If we are going to revive this thread anyway. Way would catching the input events per tick not work? (just like mc is doing). If you catch it before mc can, it means that mc will have no input events to handle. (Since they are removed after reading/catching).
-
It seems like a bug in the NEI codes. ConcurrentModification error means that a List is modified multiple times at the same moment(e.g. when the list is modified while its already getting with modifiied elsewhere). And from the stacktrace it seems like its happening inside NEI only. So I think you need to report this to NEI's creators instead. Maybe it is already fixed in a newer version, so if its possible, try to update as well.
-
[1.8] Using Resource Location Outside of Normal Minecraft Domain
N247S replied to 2piradians's topic in Modder Support
Isnt that just possible with a normal regular resourcepack? You can 'override' any texture inside the resourcefolder with a resourcepack as long as you use the ResourceLocation object for binding the texture. The only thing people need to do is holding on to the same datastructure you have used inside your resourcefolder. No need to do crazy things -
Im not sure, but the particle(EntityFX) class extends the Entity class right? Maybe you can use tbe onEntityJoin event, but than only on the client side. (No idea if its going to work!) Otherwise you could try to find how the particles are disabled through the vanilla configurations. (And Im sure you can find good examples in optifine's sourceCodes as wel)
-
[1.8, GL] Render a line between 2 BlockPos, with a colour gradient
N247S replied to CraftedCart's topic in Modder Support
I dont know much about opengl, though Im pretty sure you can apply colors to anything with a bunch of extra effects. I remeber seeing a ton of starter tutorials about how to use opengl, sampling a simple triangle in 3 different colors (each corner another color), with a nice blend effect. So why not give it a try and google for some opengl java tutorials? Im sure youll find what you are looking for! -
ConfigFiles can be world specific as well. I've been cooperating with someone who created IngameConfigManager. With this mod you have enough ways to create any kind of configuration type, without having to worry about ways to alter the configfiles.
-
There are some parts Im curious about. In the logs the Stitch event is memtioned, so do you have a onStitch event somewhere? Second have you changes/removed any textures? Otherwise I have no idea where to search for answers!
-
I would take a close look at the switch block. 1. Only the south direction will do anything 2. Are you sure those are the right metadata? Also have you tried Entity#setMotion instead of Entity#moveEntity?
-
Could you post what you've tried already? Its impossible to figure out a crash without codes or crashlog. So could you show us both?
-
I would higly recommend to look at the examples from MineCraft itself. Since there is a dozen of them. Also why do you need blockBreak particles? Aint there functions to spawn in 'vanilla' particles including blockbreak particles. Btw, on the internet there are a bunch of tutorials and examples on how to create a custom particle. All of them are usable since the concept hasn't changed in the past MC versions
-
So basically you want to convert a resourceLocation to a File object? I believe that there are methods to get the path of the resourceLocation meaning you can do something like this(dont know exact method, since Im not at my IDE) File vidFile = new File(resourceLocation.getPath());
-
Alright, sorry for the inconvienience (sorry the bad English). But with a litle google search I found a lot of easy ways to acomplish this. http://www.mkyong.com/java/how-do-convert-java-object-to-from-json-format-gson-api/ This is an simple example for creating a Json object and read it again. So Im not sure if its that big of a deal to create an whole API for it. Though if you realy want to... go ahead . Edit: I didnt use the right words in the first post, thoug what I meant is that every Entity/Item etc. Has its own instance of the NBT, meaning that you can simple get the instance and parse it. Backwards you may need to itterate through every NBT entry and set it the 'traditional' way.
-
I thought that NBT is an object, wich means you can simply parse the whole NBT object. No need for an API's in that case. Otherwise try to obtain the map/List with all the NBTentries. It can be parsed easilly as well. (With easy I mean that there is one method that will write everything to the Json/Gson object!)
-
If all the vertices are needed, than there is little optimization you can do, since most of it is already done by minecraft. (Using callLists from openGL). An superior optimization technic(wich is easy to handle with the 'newer' pc's) is VBO. I can't explain the details about it, though it should be easy to find with a litle google research. (And yea, this means that it will require a 'total' rewrite of the vanilla render codes. Im currently working on an api wich optimize rendering through the VBO technic, though it will take some time till Im able to make it public)
-
Since there is no easy support from minecraft to walk over water, I would suggest to either detect a jump/movement or use keybindings, and apply the correct speed, rotation, velocity, positions to the player. To make it 'smooth' you could use a flying mechanism (e.g. fly at the same level as water). Though think carefully about what should happen if a player hits for example a water fall (a non horizontal waterline)