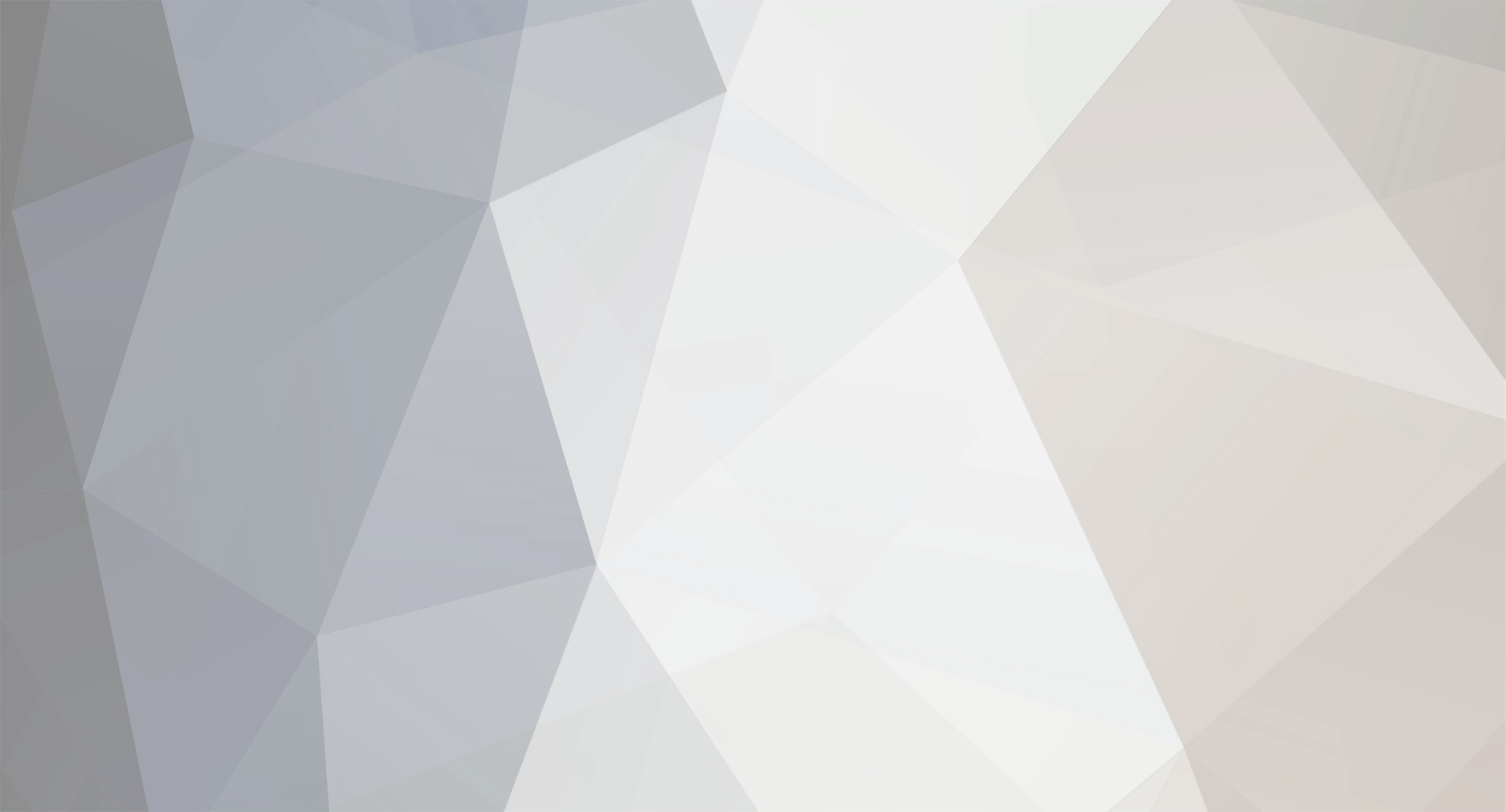
EverythingGames
Forge Modder-
Posts
388 -
Joined
-
Last visited
Everything posted by EverythingGames
-
[1.8] Texture an item WITHOUT needing a model
EverythingGames replied to benloz10's topic in Modder Support
You are wanting a multi-textured block. This is all handled in your block model .JSON. Check out MinecraftByExample by TheGreyGhost on GitHub, showing you how to use different textures based on different sides. Just rename the .JSON to your needing (name, modid, texture paths). Here's the link: https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/resources/assets/minecraftbyexample/models/block/mbe01_block_simple_model.json -
[1.7.10] Register Items with 'for' loop
EverythingGames replied to jackmano's topic in Modder Support
For my mod Armed, I use a Map to cache a string key and an item type value - string being both the key and unlocalized name for the item. I add new elements to the map in a static block (the Map is a constant) and have methods that iterate through the Map, using a lambda iterator ( Java 8 ), registering the item, setting the unlocalized name with the Map's key, and finally initializing its render. (Registering called on Common, rendering called on Client). That is just how I like to do it - it's very fast when adding new instances / items by having it automatically setup. There are many other ways you can do this, this is a more efficient way. EDIT: Your looking to do something like this with your code, considering your using an array. You should not use exactly this code, I wrote this quick just to give you an example - reason is because it gets an item from the array based on a given integer index - you're better off grabbing your item instances from an actual static instance. private static final Item[] ITEMINSTANCES = { new Item().setUnlocalizedName("test"), new Item().setUnlocalizedName("test2") }; public static final Item getItem(int index) { return ITEMINSTANCES[index]; } public static final void registerItemInstances() { for(Item item : ITEMINSTANCES) { GameRegistry.registerItem(item, item.getUnlocalizedName().substring(5)); } } -
Temporarily overlay texture on existing blocks.
EverythingGames replied to mossyblog's topic in Modder Support
I would use ISmartItemModel to alternate models with NBT, but there should be an easier way - just a suggestion. -
[1.8] Texture an item WITHOUT needing a model
EverythingGames replied to benloz10's topic in Modder Support
You can only copy 4 things on a model in JSON - parent model, texture, elements, and transform. Copying "built-in/generated" is not making a model look like the model you copied it from - built-in / generated tells Minecraft to bake your model with the given texture by providing the needed baked quads (elements) for you with added width so that your item is not a plane like element with a texture. The reason I say copy the iron ingot model is because it uses built-in / generated, but mainly because he needs the transforms there (unless he wants his own). All you would need to do is rename the texture and provide your modid (path to your texture). Usually, all vanilla built-in / generated models use the same transforms , excluding tools / weapons, etc. -
Try enabling / disabling GlStateManager.enableAlpha() in RenderPlayerEvent.Pre. You need to have transparency on the player skin, though. Otherwise you'll either have to set the skin yourself in NetworkPlayeInfo - scheduled on a Thread Pool (manually causing the Thread to sleep for around 10 milliseconds) to add a scheduled task to Minecraft (to set the skin with reflection after the NetworkPlayerInfo instance is created - all this is executed on the EntityJoinWorldEvent). Your second choice would be to somehow enable the transparency on te player skin. I would try messing around with GL first before falling back on skin overriding implementation.
-
[1.7.10] ranged mob fire rate
EverythingGames replied to BoonieQuafter-CrAfTeR's topic in Modder Support
Have you looked at EntitySkeleton class? Show what you have tried. -
[1.8] Texture an item WITHOUT needing a model
EverythingGames replied to benloz10's topic in Modder Support
Correct. When Minecraft bakes your built-in / generated model, it automatically forms to your texture and adds width to the model so that it isn't just a plane. For creating 3D item models, I strongly recommend MrCrayfish's Model creator, and also TheGreyGhost's item in-game transformer - that allows you to position any model in-game with an easy user interface. Those two tools allow you to use the new rendering system and make 3D items (even complex models) just as you did in Techne in prev-1.7.10. .JSON Model Creator www.mrcrayfish.com -
[1.8] Texture an item WITHOUT needing a model
EverythingGames replied to benloz10's topic in Modder Support
Your misunderstanding 1.8 models. Every single item uses a model, even the ones with just textures. Look at the iron ingot model JSON - it uses the built-in / generated model (all models using the texture as the model should use this). Copy the iron ingot JSON and rename everything. Register it to the ItemModelMesher in FMLInitializationEvent on the client to your item. Provide the given texture listed in the JSON. You can watch tutorials on this, but it should be very straight forward. -
That is an interesting thought, I actually thought of doing that earlier but then decided to go to the forums for input. Right now I am just rendering all of the Biped parts and Biped Ware parts and binding the player skin to it. The reason that I needed to do this was because I wrote a few classes that changes the slim or thick arms, the player skin, while being able to cache skins. It wasn't really hard - you just have to schedule the reflection business on the Main Thread so that the NetworkPlayerInfo instance is created before the skin change, using a Thread Pool (just info in case someone wants to know why and how). Anyway, consider it solved unless anyone has any other suggestions, though Ernio's suggestion was probably how it should be approached.
-
Cool down not working afte4 changing
EverythingGames replied to Mightydanp's topic in Modder Support
This is merely an example - it is an efficient cooldown in which NBT is not being stressed every tick. The world total time does the counting for you. This WILL break if you use getWorldTime() instead of using getTotalWorldTime(). Reason for that - if you are using getWorldTime() and then set the world time to day / night / custom time, it WILL break and return a cooldown that is either extensively long, or no cooldown at all. Just something to remember. if(itemstack.hasTagCompound() { final Long time = itemstack.getTagCompound().getLong("Cooldown"); if(time < THE TOTAL WORLD TIME) { DO WHAT YOU NEED HERE THEN SET THE COOLDOWN itemstack.setTagCompound("Cooldown", THE TOTAL WORLD TIME + YOUR COOLDOWN TIME IN TICKS); } } else { itemstack.setTagCompound(new NBTTagCompound()); } -
I realize how to render the player in a GUI (i.e the GuiInventory - survival inventory). That is simple, yet I want to be able to render the player in the Main Menu, or more simply put, in a GUI in which the player instance isn't created / is null. Maybe I should create a new Player Model and then try and render it? This would be straight forward if it wasn't due to the fact that rendering the ModelPlayer takes an Entity / EntityLivingBase parameter. If anyone has any suggestions I would surely appreciate it.
-
Ernio is correct. There are too many hooks, coremods should not be a thing. I mean with events, I completely replaced the player's skin, the player model, the Main Menu, the InGame GUI - I was even able to combine Java models with IBakedModel types - control rendering for Entity Items, Item Frames, etc. Tell us what you really need that makes a coremod useful 99% of the time.
-
Show your ModelBakeEvent. The code from your first post didn't seem like it would do anything. Also, post your current Wrapper class implementing ISmartBlockModel.
-
Cool down not working afte4 changing
EverythingGames replied to Mightydanp's topic in Modder Support
If your having those problems then you do not need to be worrying about NBT xp. -
Your wrapper implementation and ModelBakeEvent is wrong. Do not bake the IBakedModel yourself. Set a final IBakedModel field in your ModelPipe class and initialize it in the constructor. Then in the ModelBakeEvent (remove the SideOnly, that is not needed at all), create your ModelResourceLocation field pointing to its location - after that use event.modelManager.getModel(MODELRESOURCELOCATION) - that will return an IBakedModel from your item's ModelResourceLocation that you provided. Use that IBakedModel when creating your ModelPipe instance. Modify the ModelPipe wrapper class to your needs, and don't forget to register your event on the Forge Event Bus. Hope that gives you a general idea, if you still need help I can provide examples when I'm not on mobile.
-
(UNSOLVED) [1.7.10/1.8] Help replacing the in-game HUD?
EverythingGames replied to Ms_Raven's topic in Modder Support
This isn't hard at all just remember: 1.) You should cancel this event in Pre 2) Cancelling "ElementType.ALL" will disable every HUD rendered element Take a look at this example: public void onEvent(RenderGameOverlayEvent.Pre event) { switch(event.type) { case HOTBAR : event.setCanceled(event.isCancelable); break; case ANOTHERELEMENT : yourRenderMethod(); event.setCanceled(event.isCancelable); break; default : break; } } EDIT: Depending on your rendering you should probably render your new widgets / icons in current / post. You can also decide when to render it based on when other elements render, shown below: public void onEvent(RenderGameOverlayEvent event) { if(event.type.equals(ElementType.EXPERIENCE) { yourRenderMethodOnExperienceBar(); } } -
thanks! if I create a new function but call that function through a isRemote check is that function only run on server side then? No. In a way, this is what makes @SideOnly different from isRemote(). You can use isRemote() to run a part of the method on a specified side, or choose to only run it on one side (yet the method exists on both sides, unlike @SideOnly). This code has a block that is only called on the server. The method is called on both sides, but the block checks with side to execute on. public void example(World world) { System.out.println("This code is ran on both the client and the server (if the method is called that way I.E called on common)"); if(!world.isRemote) { System.out.println("This code is ran only on the server."); } } You shouldn't really need to do this - it will make your method fully server side but the method will exist on both sides public void example(World world) { if(world.isRemote) { return; } System.out.println("You will only see this code displayed through the server in the console, yet the method exists on both sides." }
-
[Need really help] Change displayed GUI
EverythingGames replied to JimiIT92's topic in Modder Support
By all means my above post was an example of code taken from my mod Armed - I had to give an EntityPlayer instance on the client for my GUI. Use your own constructor. -
[SOLVED] [1.8] Where Are The Armor Slots Rendered
EverythingGames replied to EverythingGames's topic in Modder Support
I got it working, thanks. Though I did have to register dummy items with my slot textures because the armor slots actually render the item textures, rather than excepting a regular ResourceLocation. -
As the title says, I want to know where the armor slots are rendered (the armor slots used for equipping armor). I can't seem to find it for the life of me - I've looked in GuiInventory, GuiCreativeInventory, GuiContainer, etc. and I still haven't found it. Reason being the armor outlines it draws indicating the different pieces of armor for each slot - I need to be able to call a GL function (colorf) on it / change the texture of it - oddly enough I can't find the texture for the armor slot overlays in the Minecraft assets folder. If anyone has any information on the armor slots and / or the overlay drawn over it to indicate the armor types, please let me know - thanks.
-
[1.8] B3D Model Matierial Color Not Displayed In-Game
EverythingGames replied to EverythingGames's topic in Modder Support
Of course they support materials - that's what makes up a mesh and it's different features. What I meant to say was "colored materials." Guess I'll just have to use textures. -
[1.8] B3D model EntityItem transformations
EverythingGames replied to Wehavecookies56's topic in Modder Support
That's probably because your rendering class is never being called. It's called when your custom entity item is spawned, and you haven't done that yet. Your entity class and rendering class looks good, you just need to subscribe to the EntityJoinWorldEvent and spawn your custom entity item there. EDIT: Not sure if the custom entity item is assigned to your item by overriding item methods like you did above. If you don't get that working, I would try the suggestion above. -
[SOLVED] [1.8] GUI Rendering GL Alpha Problem
EverythingGames replied to EverythingGames's topic in Modder Support
I fixed the problem. Solution - push the matrix, enable blend, bind the texture and draw the textured rect., disable blend, then finally pop the matrix. TIP - You can simply modify the GUI's by extending them and overriding their methods (then set the GUI field in the GUIOpenEvent to your GUI) rather than copying the whole GUI class and calling displayGuiScreen() like shown above, unless you need access to private methods / fields and don't want to use reflection; -
[Need really help] Change displayed GUI
EverythingGames replied to JimiIT92's topic in Modder Support
Replace the crafting inventory with your own. Your own should have all the extra slots handled. @SubscribeEvent public void onEvent(GuiOpenEvent event) { if(event.gui instanceof TheInventoryYouWantToReplace) { event.gui = new YourNewInventory(Minecraft.getMinecraft().thePlayer); } } -
[SOLVED] [1.8] GUI Rendering GL Alpha Problem
EverythingGames replied to EverythingGames's topic in Modder Support
That didn't do a thing. Again, I think its located where the armor slots are rendered, but I can't find any trace of where they are.