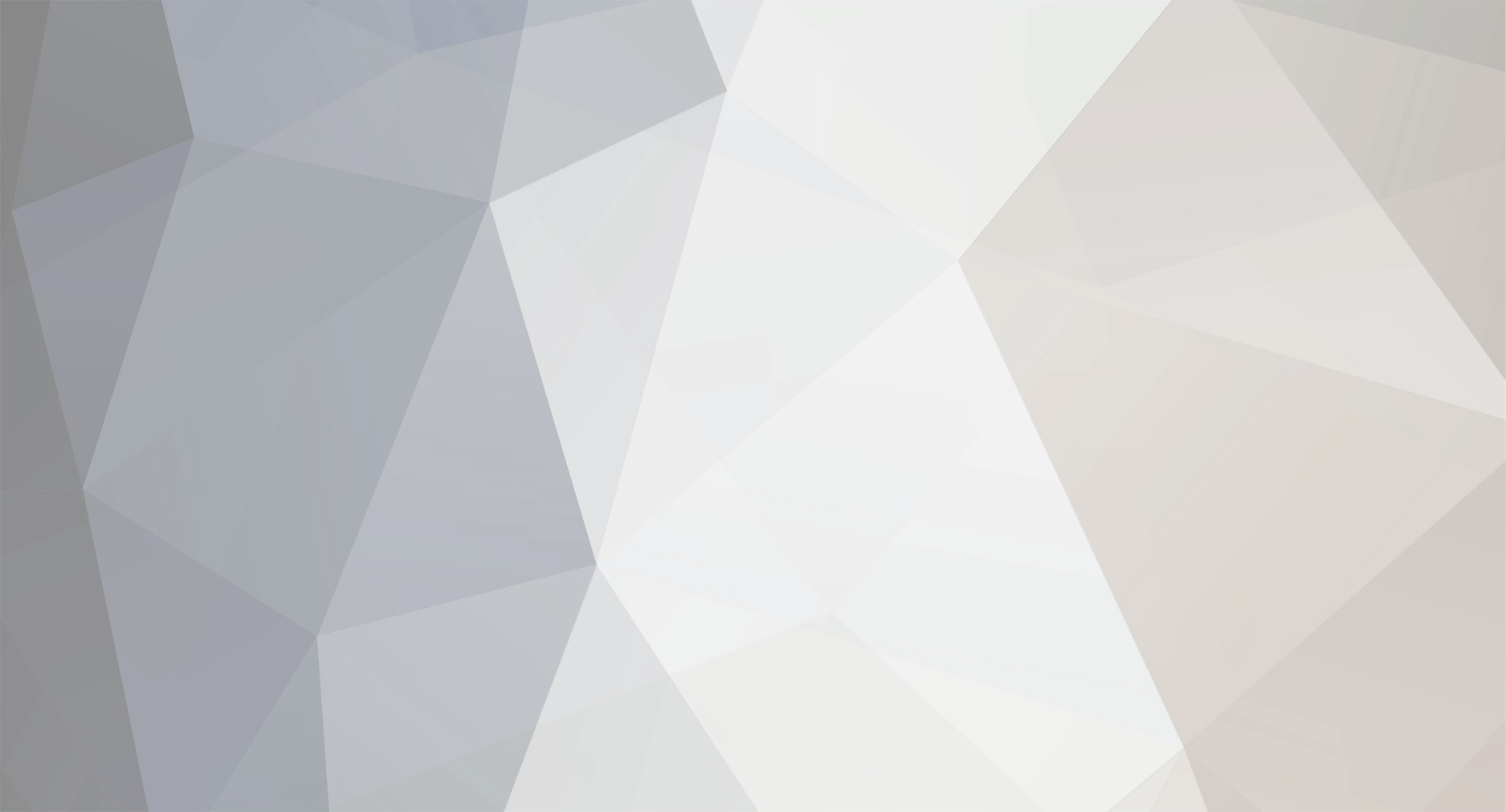
UberAffe
Forge Modder-
Posts
316 -
Joined
-
Last visited
Everything posted by UberAffe
-
You'll still need to figure out how to render it using OpenGL(maybe tesselator) but you will be tying it to the entity instead. This will be more help than me.
-
[1.8.9] Using capabilities to have a file that serializes data to NBT
UberAffe replied to GalianRyu's topic in Modder Support
All you need is a file to manage the alterations to the data and save/load that information using regular java io. I use this during preInit to get the file location for my extra information event.getModConfigurationDirectory().toString() +"\\LightDrafter\\Draftables.json" which is just a custom folder inside of the config colder. The biggest thing to remember is that the instance of this class will be different on server side and client side, so you will have to manage updates between them using IMessage and IMessageHandler, or make sure that you only create an instance when you are server side. -
You will have to figure out how to draw that using regular OpenGL (maybe a tesselator) and then hook into @SubscribeEvent(receiveCanceled=false) public void onEvent(RenderGameOverlayEvent.Pre event) { and call your draw method.
-
[Solved, m8]Modifying the functionality of torches
UberAffe replied to Toost's topic in Modder Support
pretty sure there is a block placed event, you can check what they type of block is and if the block is vanilla torch, cancel event and place your torch instead. You could probably even have your torch extend the vanilla torch and the only code you would need would be however you choose to handle lighting things on fire. By extending vanilla torch, without overriding anything it should automatically look like a vanilla torch and drop vanilla torches. -
I've tried that one, it gives the same value at night as it does during the day.
-
I haven't worked with biomes yet but you can take a look at how natura does it.
-
Thats what I can't figure out how to get. Lets say this is the folder structure: / mods/ LightDrafter.jar ... config/ LightDrafter/ Draftables.json How do I get the path/URL of Draftables.json
-
I am trying to save information to a file on server side. My save method is getting triggered by WorldEvent.Save I am getting this error when the world try's to save: 23:50:13] [server thread/ERROR] [FML]: Exception caught during firing event net.minecraftforge.event.world.WorldEvent$Save@7100ac37: java.lang.ExceptionInInitializerError at lidr.common.LiDrEvents.worldSave(LiDrEvents.java:32) ~[LiDrEvents.class:?] ... Caused by: java.lang.NullPointerException at lidr.common.DraftableReg.SaveDraftables(DraftableReg.java:57) and my WorldSave Method: @SubscribeEvent public void worldSave(WorldEvent.Save e){ if(!e.world.isRemote) DraftableReg.SaveDraftables(); // this is line 32 } DraftableReg is a java "static" class meaning every field and method is static, private instructor with another field that instantiates it. public final class DraftableReg { public static final DraftableReg draftableReg = new DraftableReg(); private static final URL LOCATION = draftableReg.getClass().getClassLoader().getResource("Draftables.json"); private DraftableReg(){ DraftableMap dMap = new DraftableMap(); dMap.AddPart(new TriTuple(0,0,0), new EdgePart(LuxinReg.RegisteredLuxin.get("Blue"), Purity.Base)); RegisterDraftable(CoreFactory.GenerateFrom("test", CoreType.Sword, dMap)); //Testing items } public static void SaveDraftables(){ try { GsonBuilder builder = new GsonBuilder(); builder.registerTypeHierarchyAdapter(IDraftable.class, new DraftableAdapter<DraftingItemCore>()); builder.enableComplexMapKeySerialization(); builder.setPrettyPrinting(); Gson gson = builder.create(); BufferedWriter out = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(LOCATION.getFile()), "UTF-8")); gson.toJson(knowledgeBase, out); // this is line 57 } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } So obviously my URL is faulty. How should I be choosing a location to save to?
-
None of the methods I have tried have given me the current light level at the block position, they seem to only be giving light levels for daytime. world.getLight(new BlockPos(player)) getLightFromNeighbors getLightBrightness how can I get the current light level?
-
You'll want a class that implements Runnable. The constructor should take whatever information you will be saving and it should take it as final to guarantee that nothing gets altered. From the run method that you override you will do any saving to file using regular java IO. Hook into whatever event you want to be the trigger and call (new Thread(new RunnableClass())).start(); You will have to figure out how you are going to prevent a race condition.
-
How to store nested information that isn't tied to an item or entity
UberAffe replied to UberAffe's topic in Modder Support
The example I gave is a little misleading with the names, I simplified everything down to the just the raw information I need to store. I have TypeCores(Melee, Mining, Ranged. etc) that extend Item and get registered during prInit() normally. I have a factory that knows how to generate an IBakedModel and relevant NBTTagCompound from this information and return that as an ItemStack for the appropriate TypeCore. I preform the generation after the item has been designed/or a design was altered and uses copies of that to create ItemStack instances. I am not doing the generation each time an ItemStack is created. This question is purely about how best to store the information and if there is an existing convention that people use, what is it? -
How to store nested information that isn't tied to an item or entity
UberAffe replied to UberAffe's topic in Modder Support
Restructured and Rephrased question so that it will make more sense and be easier to understand what I need. -
java.lang.NoSuchMethodError on Minecraft launch
UberAffe replied to Adityagupta's topic in Modder Support
Everything in your CommonProxy is the Server Side from a physical perspective (a dedicated server (logical server) will use CommonProxy). A physical client (always has logical client, in smp it also is the logical server) will use ClientProxy which includes server code. -
I recommend taking a look at this, it helped me understand how proxy is used in forge.
-
An implementation of IItem is used to generate an NBTTagCompound for an item, but the item only stores the generated information instead of all the information involved in the generation. I am doing it this way because the ModelMap will have a LOT of information and storing that information in every item is a massive waste of space. This is the class structure/ information that I need to store: Class Names were changed to simplify the example None of the implementation classes will have more significant information than what is given. public class ItemExample implements IItem{ private ModelMap map; private String name; } public class ModelMap { private HashMap<Coord, IPartType> map; } public class Coord { private int x,y,z; } public class PartExample implements IPartType { private String name; private Purity purity; } public Enum Purity{ } Is using a Json file a good way of going about this? Is there a convention for doing something like this that I am just unaware of?
-
Your welcome, and it is almost exactly what I have been going through. Let me know if you need help getting the client to update properly, that bit took a lot of trouble shooting for me.
-
your handler should be implementing IStorage<IBaseManaCapability>
-
Just went through an almost identical process I'll update this response with some specifics but for now you can take a look at my github. You'll want to look at DefaultDrafter and DrafterProvider. Update: IBaseManaCapability should be a regular interface(doesn't extend anything) PlayerManaCapability should implement IBaseManaCapability(this is the default implementation) For saving the players mana and loading it you would do something like this: public class PlayerManaCapability implements IBaseManaCapability{ //implement methods public static class ManaStorage implements IStorage<IBaseManaCapability>{ public static final ManaStorage manaStorage = new ManaStorage (); //implement methods of IStorage (read and write nbt) } } You can break ManaStorage into a different file like you have it but if you leave it as an inner class it lets you access the instance.privateVariables for read and write without extra method calls. register it like this: CapabilityManager.INSTANCE.register(IBaseManaCapability.class, ManaStorage .manaStorage , PlayerManaCapability .class); update 2: your factory should be a provider meaning something like this: public class ManaProvider implements ICapabilityProvider, INBTSerializable{ @CapabilityInject(IBaseManaCapability .class) public static Capability<IBaseManaCapability > MANAPLAYER = null; // implement methods } You will also want to hook into these two events @SubscribeEvent public void AttachCapability(AttachCapabilitiesEvent.Entity e) @SubscribeEvent public void onClonePlayer(PlayerEvent.Clone e)
-
Maybe he doesn't need to put in proxy but that call stack doesn't go down to common proxy, doesn't that mean that it is only registering the recipe on the physical client?
-
didn't notice this at first at com.drmdgg.marijuanacraft.CraftingManager.MarijuanaCraft(CraftingManager.java:16) at com.drmdgg.marijuanacraft.proxy.ClientProxy.preInit(ClientProxy.java:42) it looks like you are registering the recipe in your client proxy, you need to register it in common proxy
-
seriously /*code/errors here*/ [//code] maybe even all inside a spoiler tag for the long ones what do you have right now on this line CraftingManager.java:243
-
rendering is postInit() so in your client proxy you could have something like this: @Override public void postInit(){ registerRenderers(); } private void registerRenderers(){ MarijuanaBud.registerRenders(); Light.registerRenders(); MarijuanaPlant.registerRenders(); MarijuanaSeed.registerRenders(); Bong.registerRenders(); TrashBlock.registerRenders(); PlasticBottle.registerRenders(); BakedApple.registerRenders(); Shiv.registerRenders(); ToothBrush.registerRenders(); PlasticPen.registerRenders(); //it would be better still if you replace these calls with something like this Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(Bulb.bulb, 0, new ModelResourceLocation(Reference.MOD_ID + ":" + Bulb.bulb.getUnlocalizedName(), "inventory")); } you would use something like this in your common proxy for the creative tab: public void preInit(){ mCreativeTab.initializeTabs(); MarijuanaBud.MarijuanaCraft(); Light.MarijuanaCraft(); MarijuanaPlant.MarijuanaCraft(); MarijuanaSeed.MarijuanaCraft(); Bong.MarijuanaCraft(); TrashBlock.MarijuanaCraft(); PlasticBottle.MarijuanaCraft(); PlasticPen.MarijuanaCraft(); BakedApple.MarijuanaCraft(); Shiv.MarijuanaCraft(); ToothBrush.MarijuanaCraft(); // I would also suggest that you rework these so that you have something like this GameRegistry.registerBlock(Bulb.bulb, Bulb.bulb.getUnlocalizedName()); }
-
I just noticed that you are registering your creative tab after you try to use that tab to register your items. By convention you should have something like: public class ClientProxy extends CommonProxy { @Override public void preInit(){ super.preInit(); //register KeyBindings } //register renderers and other such things in the appropriate initialization method } public class CommonProxy { public void preInit(){ //register items/blocks } } @Mod(modid = Reference.MOD_ID, name = Reference.MOD_NAME, version = Reference.VERSION) public class MarijuanaCraft { @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.SERVER_PROXY_CLASS) public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event) { proxy.preInit(); }
-
For future reference put code inside of [//Code] tags, using one / instead of two I don't actually see a problem with what you have posted but you should move all your registry calls into your common proxy or client proxy, it will make it much easier to read/troubleshoot and maintain. And add the code for your proxies, we can't see when you call the registerRenders.