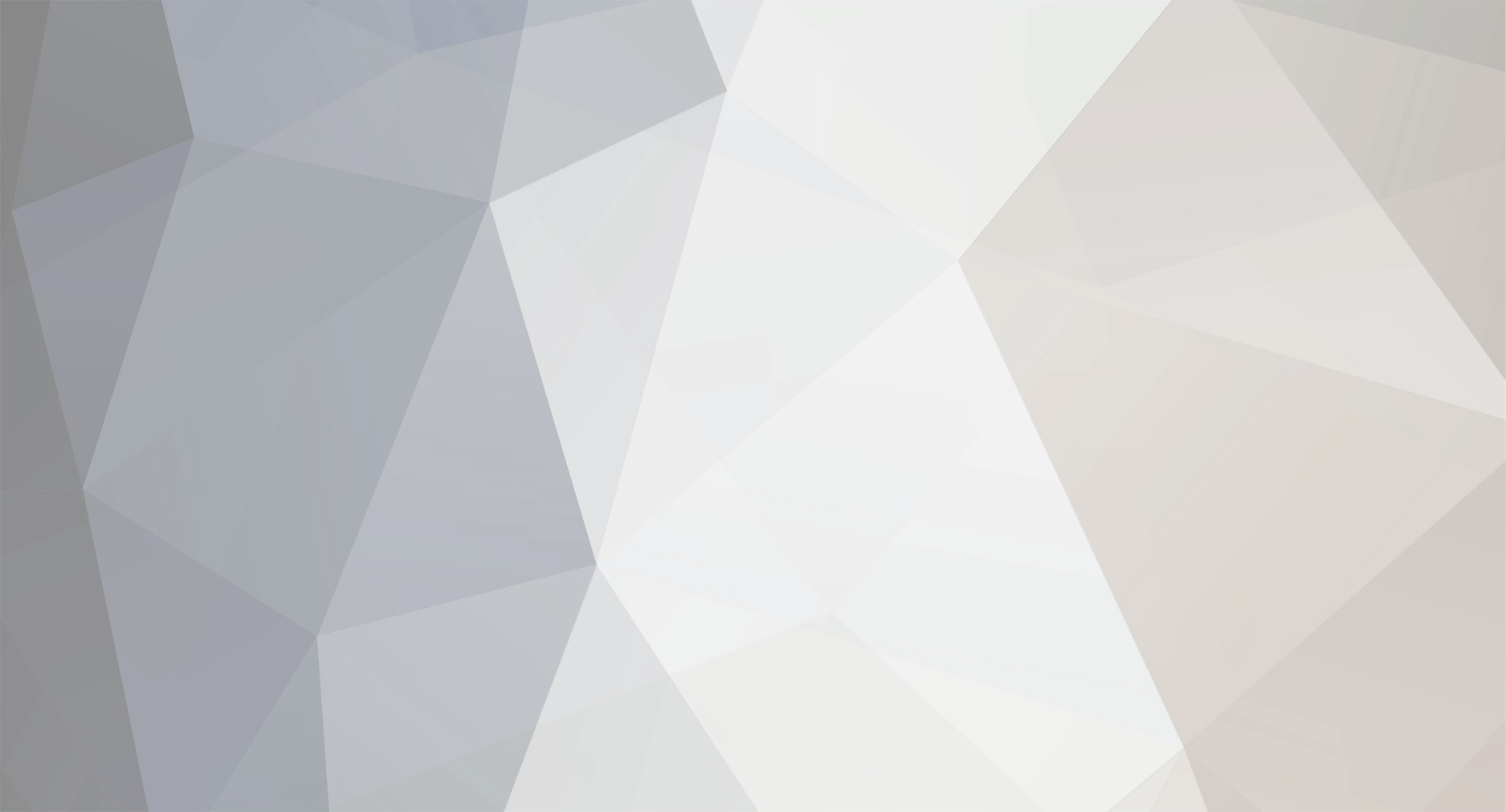
UberAffe
Forge Modder-
Posts
316 -
Joined
-
Last visited
Everything posted by UberAffe
-
instead of the line where you have this.lastTile = toConnect you would use this: NBTTagCompound nbt = (stack.hasTagCompound()) ? stack.getTagCompound() : new NBTTagCompound(); nbt.setIntegerArray("lastTile",new int[]{toConnectTile.xCoord, toConnectTile.yCoord, toConnectTile.zCoord}; stack.setTagCompound(nbt); when you need to get the last tile entity: int[] position = stack.getTagCompound().getIntegerArray("lastTile"); TileEntity lastTE = MinecraftServer.getServer().getWorld().getTileEntityAt(position[0], position[1], position[2]); I might have some of the method names wrong because I don't have an IDE right now, but you should be doing something like this, but you will want to add in some more checks to make sure you only use applicable TE's.
-
Multiple collisionboxes in an entity?
UberAffe replied to ItsAMysteriousYT's topic in Modder Support
I don't know that you would even be able to make a single entity for a plane, generally a collision/ bounding box can not be larger than a 1x1x1 cube, your best bet would be to have the one model for your TE that would probably only actually be the nose of the plane or something, then there would be a bunch of invisible boxes of different sizes/shapes the make up the collision space for the rest of the plane. -
Hook into the playerinteract event and check for player leftclick on block as the action and that the block is your block and set the result to DENY. This is a good reference to keep on hand: http://www.minecraftforge.net/wiki/Event_Reference
-
[SOLVED][1.7.10] - Player max health AttributeModifier
UberAffe replied to Agravaine's topic in Modder Support
Take a look here: https://gist.github.com/Choonster/b435956191898d34c7f8 I'm not very familiar with using attributes but it looks like you are applying the change wrong. -
Not positive if this would fix your problem but using either the onplayertick event or the onplayerxpgained event(thats probably not the name). Those should give you access to the player and somewhere, either in its own public class or as additional nbt info on the player you could store the pets it has, then you would have access to both the player and the pet and you could do what you need with them.
-
I was not planning on making a separate gui and this effect is meant to be possible to use on anything that extends ItemArmor. Well, that will get the job done, it just seems like unnecessary processing. I know the if checks take practically 0 runtime but it feels like such a waste to check them every tick when I only need it when they switch what they are wearing.
-
First I want to say that I did search for other topics and there were some similar ones but none have given me a solution. I am adding information to the nbt of any item that extends ItemArmor and is used with my TE. This integer tag represents a tier that I am adding to the item which will increases the wearers reach based on the tier Because this is not specific to my armor I cannot use the onArmorTick method. The closest thing I have found is to use the player event for onOpenContainer but that is not going to be a very clean or reliable way of applying this effect. I'd rather not mess with enchantments because as far as I can tell they have the most unfriendly setup for adding new effects I have ever seen, besides the fact that they are not going to merge well with how I want to set up my tiering(practically infinite, actually log base 2 of max int). Hopefully someone here knows a good place that I can use to hook into otherwise I guess I'll have to bite the bullet and figure out enchantments.
-
[1.8] Best way to send packets to specified amount of people
UberAffe replied to Jedispencer21's topic in Modder Support
Store your array of players that share a waypoint serverside and when any of them change the waypoint it sends the update to everyone on the list that isn't that player. -
[SOLVED]1.7.10 crash on player specific login (with logs!)
UberAffe replied to UberAffe's topic in Support & Bug Reports
Found it, sort of. Removing Teleplates and its dependency xcore fixed the problem, it is a new mod and seems to be causing a problem with its player tick event. Reporting this to mod author. Thanks for taking a look at this anyways LexManos! -
[SOLVED]1.7.10 crash on player specific login (with logs!)
UberAffe replied to UberAffe's topic in Support & Bug Reports
I got a fresh log server started player, player tried to connect server stopped Full fml-server-latest: https://raw.githubusercontent.com/UberAffe/excessivly-large-files/master/technexx-full-fml-log.log 10,500 lines is way to much for this, a surprisingly simple number. -
I have a custom modpack that a few people have started playing on. One of the players got kicked with an internal server error while going down a ladder and a villager walked beneath them. Things I tried: having them restart their client, restarting both server and client, editing that players .dat file to put them to known safe coordinates This is what happens on the server when they try to log in: the last line is what happens when I shut down the server, not when they get kicked. Their client doesn't actually crash but this is their fml latest log: All the parts that I omitted don't list any errors and they can load in single player fine I expect that it is just the last few lines that will be important. Edit: let the confusion continue ... I removed the players uuid.dat file along with all the other player specific files in that folder and they still get the same issue when trying to connect. Other players can connect and play just fine, we have no items that are linked to players either. Edit2: Began happening to another player when they tried opening a vanilla furnace gui, didn't happen with crafting table gui though.
-
I think you might have a misunderstand of what the client does. Is the held item the only way in/out of that dimension? What is the purpose of this dimension?(is it somewhere to put like a personal chest room or power generators? If you have those chunks be permanently loaded once assigned to a player, I can not think of a use case where you would need to send information specifically to the user. However if you do NEED to, you can use regular packet handling and send your information to the player, the player is a parameter. this tutorial should help if you don't know how to send packets yet: http://www.minecraftforge.net/forum/index.php/topic,20135.0.html
-
I am not positive that I understand exactly how withinAABB works but you have way more for loops than necessary in your code. you only need the one loop to go through the entities. create a max and min x,y,z variable to outline your zone and when you loop through the entities check if that entities coordinates are between the max/min's if so damage them.
-
[1.8] Best way to send packets to specified amount of people
UberAffe replied to Jedispencer21's topic in Modder Support
have a list of all players that are receiving the waypoint and for(string pName : list) if(!pName.equals(editingplayer.name) sendmessage(msg, world.getplayer(pname); can't be copy pasted but you can use that structure to updated everyone you need to. -
Seems like you are trying use "new" on something that you aren't supposed to.
-
the "world" you are talking about would be a dimension on the server. if you are only giving 4 chunks to your "world" you could just make a single dimension and have that dimension generate a bunch of seperate 4chunk areas and each user gets a way to teleport to a specific one of those areas.
-
just do a check on the message string(mymessage.text if you set it up like the tutorial) inside your onMessage method: if(mymessage.text.equals("player invis")) dostuff();
-
What do you mean when you say you want them to be in multiple worlds? Like what does the player see? how do the interact with these worlds?
-
You would want to look at how the movement control for horses are coded, at least for where the hooks are. Also might want to look at the EntityPlayer class. I imagine that it is something along the lines of: player tries to move; player checks if it is riding something; if so, call some method of the entity being ridden; you would then override that method in your vehicle entity to do what you want. But I haven't actually looked at the code for this, that is just my guess/ suggestion for where to look.
-
Are you trying to have every player start in their own dimension or something?
-
ctx has the player, I think it is ctx.getPlayer() also you don't need ctx to get the world, you can use something like this: MinecraftServer.server.world (its a global static) also take a look at this: http://www.minecraftforge.net/forum/index.php/topic,20135.0.html and this: http://www.minecraftforge.net/forum/index.php/topic,33112.msg173479.html#msg173479 should help you understand the buffer stuff.
-
For those who don't read the original post in the link, the confusion with trying to send the player uuid in the message comes from me. I wasn't aware that you could get the player that sent the message from the context. @BlackHyp3r you don't need to send the uuid in the message, in your onmessage override you can get the player from the MessageContext variable.
-
[1.7.10]hotswapping armor sets to gain multiple effects
UberAffe replied to UberAffe's topic in Modder Support
Well I guess theres nothing more to it but to get coding. I'll come back when I figure out if this works or not. -
[1.7.10]hotswapping armor sets to gain multiple effects
UberAffe replied to UberAffe's topic in Modder Support
I was planning on doing more than just fire the event, I was planning to duplicate? the event. As the Player being hit If I was attacked by an arrow and had the full mod set on I would then get hit by that arrow while wearing each of the armor sets and my health would get set back to the version that was the most beneficial. So it's like that one attack got split and attacked an identical player with each of the armor sets and the one that faired the best is the one that the original player gets set to. Actually I just realized that I probably need to make a full copy of the player, not just their health, otherwise they might get sent flying pretty far backwards or accidentally be murdered and suddenly get health back. -
[1.7.10]hotswapping armor sets to gain multiple effects
UberAffe replied to UberAffe's topic in Modder Support
@delpi I can find tutorials just fine, I was asking because the best tutorials don't always make it to the top and you might know of a better one. @Failender Unfortunately I think I agree that this might simply be too much going on in too short a time and cause horrid tick lag. I updated the original posted to better clarify my idea(i had forgotten to put a part)