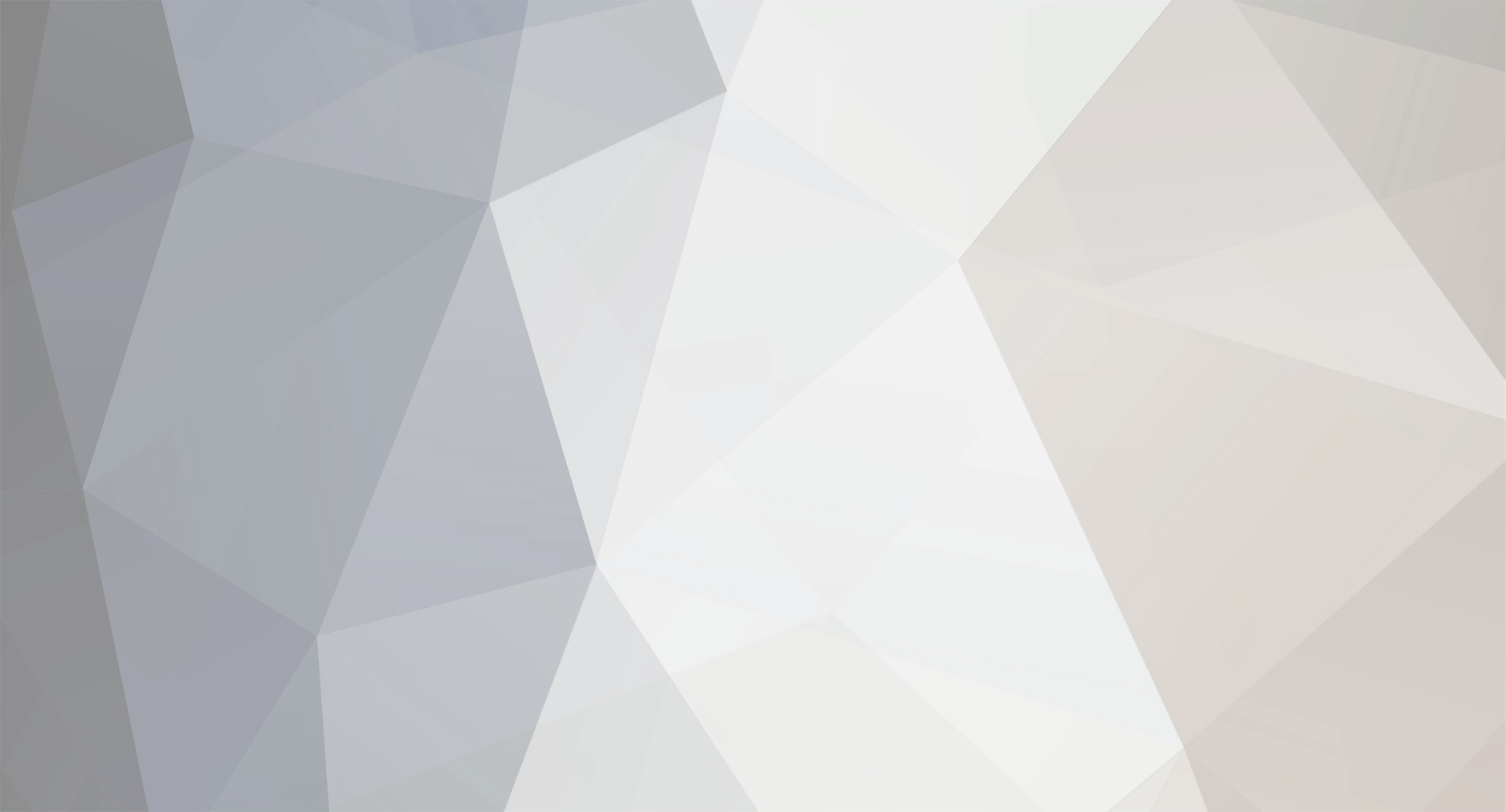
UberAffe
Forge Modder-
Posts
316 -
Joined
-
Last visited
Everything posted by UberAffe
-
I am also trying to do stuff on left click, here is what I have: @SideOnly(Side.CLIENT) @SubscribeEvent public void LeftClick(ClientTickEvent event) { //LogHelper.info("At least I get called"); if(Minecraft.getMinecraft().gameSettings.keyBindAttack.isPressed()) { LogHelper.info("Attacking"); } } When the first LogHelper is uncommented it spams the log, so I know this method is getting called, but the second LogHelper never gets called. Any idea what I have wrong with this if statement?
-
Well this is going to be tiresome to switch up ... oh well, Thanks for the help!
-
Override the onItemUse method.
-
So what I am getting out of that is that I need to make a separate class that will only get accessed by (logical) client side code.
-
I am trying to use one class for handling all the available NBT instances of an item along with the models that will get generated by each NBT instance (dynamic items with dynamic models). This is the code I have so far: public final class DraftableReg { private static HashMap<String, IDraftable> knowledgeBase = new HashMap<String, IDraftable>(); @SideOnly(Side.CLIENT) //this is line 24 private static HashMap<String, DraftableBakedModel> models = new HashMap<String, DraftableBakedModel>(); public static void RegisterDraftable(IDraftable draftable, World world){ if(world.isRemote){ LogHelper.info(draftable.GetName() + " registered by Player"); models.put(draftable.GetName(), new DraftableBakedModel(draftable)); ;//send draft to server for registry } else { LogHelper.info("Registering Draftable :" + draftable.GetName()); knowledgeBase.put(draftable.GetName(), draftable); } } } This is fine when I am running in an Integrated server but it fails when I try to launch a dedicated server. This is the chrash: java.lang.NoSuchFieldError: models at main.java.lidr.common.DraftableReg.<clinit>(DraftableReg.java:24) ~[DraftableReg.class:?] at main.java.lidr.common.LiDrEvents.ServerStartup(LiDrEvents.java:40) ~[LiDrEvents.class:?] at net.minecraftforge.fml.common.eventhandler.ASMEventHandler_8_LiDrEvents_ServerStartup_Load.invoke(.dynamic) ~[?:?] at net.minecraftforge.fml.common.eventhandler.ASMEventHandler.invoke(ASMEventHandler.java:49) ~[ASMEventHandler.class:?] at net.minecraftforge.fml.common.eventhandler.EventBus.post(EventBus.java:140) ~[EventBus.class:?] at net.minecraft.server.MinecraftServer.loadAllWorlds(MinecraftServer.java:312) ~[MinecraftServer.class:?] at net.minecraft.server.dedicated.DedicatedServer.startServer(DedicatedServer.java:257) ~[DedicatedServer.class:?] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:508) [MinecraftServer.class:?] at java.lang.Thread.run(Unknown Source) [?:1.8.0_45] is there a reasonable way for me to do this in one class or will I have to make a clientside only class that handles models?
-
Both in a sense. You should have something like this: public class ModClass{ @SidedProxy(...) CommonProxy proxy; @EventHandler public void init (FMLInitializationEvent event){ proxy.init(event); } } public class ClientProxy extends CommonProxy{ public void init(FMLInitializationEvent event) { super.init(); //client side things } } public class CommonProxy{ public void init(FMLInitializationEvent event) { entReg.registerEntity(); } }
-
Like I said this thread was getting very off topic. I was trying to register a test item when the world loads and I noticed that it was registering the item 3 times in the event I was using. It is not a problem for this particular case but I am curious what events are available that are only triggered once during the startup process and are after postInit.
-
why are you registering it twice?
-
I am using this: public class DraftableSmartItemModel implements ISmartItemModel{ @Override public IFlexibleBakedModel handleItemState(ItemStack stack) { IFlexibleBakedModel model = null; if(stack.getTagCompound().hasKey(Refs.DRAFTABLE) && DraftableReg.Exists(stack.getTagCompound().getString(Refs.DRAFTABLE))) model = DraftableReg.getModel(stack.getTagCompound().getString(Refs.DRAFTABLE)); return model; } } I generate the model for each item once, when they get added to my registry public class DraftableReg { public static void RegisterDraftable(IDraftable draftable){ models.put(draftable.GetName(), new DraftableBakedModel(draftable)); LogHelper.info("Registering Draftable :" + draftable.GetName()); knowledgeBase.put(draftable.GetName(), draftable); } } public class DraftableBakedModel implements IFlexibleBakedModel{ private List<BakedQuad> quads = new ArrayList<BakedQuad>(); public DraftableBakedModel(IDraftable draft){ DraftableMap parts = draft.GetPartArray(); for(int xPos = 0; xPos < 16; xPos++){ for(int yPos = 0; yPos < 16; yPos++){ for(int zPos = 0; zPos < 16; zPos++){ if(parts.GetPart(xPos + "," + yPos + "," + zPos) != null) quads.addAll(getQuadFrom(parts.GetPart(xPos + "," + yPos + "," + zPos), xPos, yPos, zPos)); } } } } private List<BakedQuad> getQuadFrom(IPartType getPart, int x, int y, int z) { List<BakedQuad> list = new ArrayList<BakedQuad>(); BakedQuad quad; //North quad = new BakedQuad(applyOffset(getPart.GetVertices(EnumFacing.NORTH),x,y,z), 0, EnumFacing.NORTH); list.add(quad); //Up quad = new BakedQuad(getPart.GetVertices(EnumFacing.UP), z, EnumFacing.UP); list.add(quad); //East quad = new BakedQuad(getPart.GetVertices(EnumFacing.EAST), z, EnumFacing.EAST); list.add(quad); //South quad = new BakedQuad(getPart.GetVertices(EnumFacing.SOUTH), z, EnumFacing.SOUTH); list.add(quad); //Down quad = new BakedQuad(getPart.GetVertices(EnumFacing.DOWN), z, EnumFacing.DOWN); list.add(quad); //West quad = new BakedQuad(getPart.GetVertices(EnumFacing.WEST), z, EnumFacing.WEST); list.add(quad); return list; } private int[] applyOffset(int[] vertices, int x, int y, int z) { for(int i = 0; i < 7; i++)//7 elements per vertex { for(int j = 1; j < 5; j++)// 4 vertices { switch(i){ case 0: vertices[i*j] = vertices[i*j] + (x*Refs.OFFSET);//xpos break; case 1: vertices[i*j] = vertices[i*j] + (y*Refs.OFFSET);//ypos break; case 2: vertices[i*j] = vertices[i*j] + (z*Refs.OFFSET);//zpos break; default: break; } } } return vertices; } } I'm not completely done with it but this is most of the code relevant to my model generation.
-
[1.9] Crash when getting EntityPlayer from PlayerTickEvent
UberAffe replied to Ringosham's topic in Modder Support
The tick event is client and server side so this line should cause a problem on the server side in every minecraft version Minecraft.getMinecraft().thePlayer.addChatMessage(new TextComponentString("Text 2")); Logs would be helpful. -
There is really no event that only happens once??? To be clear I mean once in the sense that it would only get called when a server is starting up, I do not mean once per world. I'm pretty sure it just seems like I'm doing this the wrong way because this thread is a bunch of half explanations. But I would like to find out sooner rather than later if I really am doing something wrong. So can you clarify what part you think I am doing wrong and I will give a full explanation of how I am doing that part to see if I actually am wrong.
-
Well this topic has gotten quite off course. I'm just looking for events that I can use that only occur once when a world is starting up.
-
When I register the nbt items (if it is client side) I generate a bakedModel from the nbt information and that can't happen on server side because the class bakedModel doesn't exist serverside. New items can be designed and added to the registry during run-time. Which means that the items available are determined by the server during run-time not during initialization.
-
I do need the world object because this also generates a bakedModel if it is on the client side and the registry is global but the selection is per user. I already have capabilities set up for the user specific things. I just don't have the system in place for designing the items yet, so in order to test other things I need to prime the registry with an item.
-
It isn't really a problem for this purpose but for future reference I am trying to figure out what events I can use for initializing different things when a world starts up. In this particular case the NBT information is getting stored in a custom registry that can be added to at any point during the game (I want to add a test item when the world starts). Each user has a selected item that will get created and given to them on a hotkey press.
-
The IDraftable that you can see me adding to a hashmap is a structure that I use to create an NBT based item (think tinkers but many more parts) I already have all the communication lines for giving an item to a player and syncing the nbt items between server and client. I am just trying to prime the world with a test item so I can test my save/load/item creation/item rendering methods.
-
This is what I see in my log: [18:29:39] [server thread/INFO] [FML/]: Loading dimension 0 (New World) (net.minecraft.server.integrated.IntegratedServer@17eb9432) [18:29:39] [server thread/INFO] [FML/]: Loading dimension 1 (New World) (net.minecraft.server.integrated.IntegratedServer@17eb9432) [18:29:39] [server thread/INFO] [lidr/]: Registering Draftable :test [18:29:39] [server thread/INFO] [FML/]: Loading dimension -1 (New World) (net.minecraft.server.integrated.IntegratedServer@17eb9432) [18:29:39] [server thread/INFO] [lidr/]: Registering Draftable :test [18:29:39] [server thread/INFO] [lidr/]: Registering Draftable :test This is my event handler: @SubscribeEvent public void worldLoad(WorldEvent.Load e){ if(!e.world.isRemote) { DraftableMap dMap = new DraftableMap(); dMap.AddPart("0,0,0", new EdgePart(LuxinReg.RegisteredLuxin.get("Blue"), Purity.Base)); DraftableReg.RegisterDraftable(CoreFactory.GenerateFrom("test", CoreType.Sword, dMap)); //Testing items } } and this is my registerDraftable method public static void RegisterDraftable(IDraftable draftable){ LogHelper.info("Registering Draftable :" + draftable.GetName()); knowledgeBase.put(draftable.GetName(), draftable); } This is happening when I run through eclipse and play in an smp world, any ideas? So I'm dumb, this event is obviously getting triggered for each dimension. Revised question, I am looking for an event that happens once when you launch an smp world or when a server is starting its world so I can check if the world is remote. What event should I be using here?
-
Thanks, seems like that worked.
-
I used streams and lambda expression in a few places to make a few methods easier to implement. I wasn't able to run my project so I did the setup and eclipse again just to refresh my workspace but that didn't fix it. I tried building and the build failed with this: warning: [options] bootstrap class path not set in conjunction with -source 1.6 {folderlocation}.java:80: error: lambda expressions are not supported in -source 1.6 (use -source 8 or higher to enable lambda expressions) I know if I require java 8 or higher than people will need to have that to play with this mod but will making this change cause compatibility issues with other mods? If it won't cause problems where would I tell it to compile with -source 8?
-
also, where ever you are going to have your loop you need to do a null check on .getStackInSlot(slot) before you call .getItem().
-
pretty sure that is what the method isGui3D is used for, override it in your item to return true;
-
As a side not, you should send the packet any time they press the key and check for the item serverside. It's not common but the inventory sometimes get's desynced.
-
you need to register you keybindings in your clientproxy preinit, it seems like you are trying to register them serverside which would cause a crash. if you aren't registering stuff in your proxies (which I recommend doing, it helps me keep things separated) then you can do if(proxy instanceof ClientProxy)
-
How are you trying to install it? Is this server being hosted/ what kind of access do you have to the files? Is this for an official modpack or a custom/3rdparty pack?
-
you are probably getting a null pointer exception because you are doing an assignment inside of a function call. Move your assignment statement in front of the register call and pass in the variable.