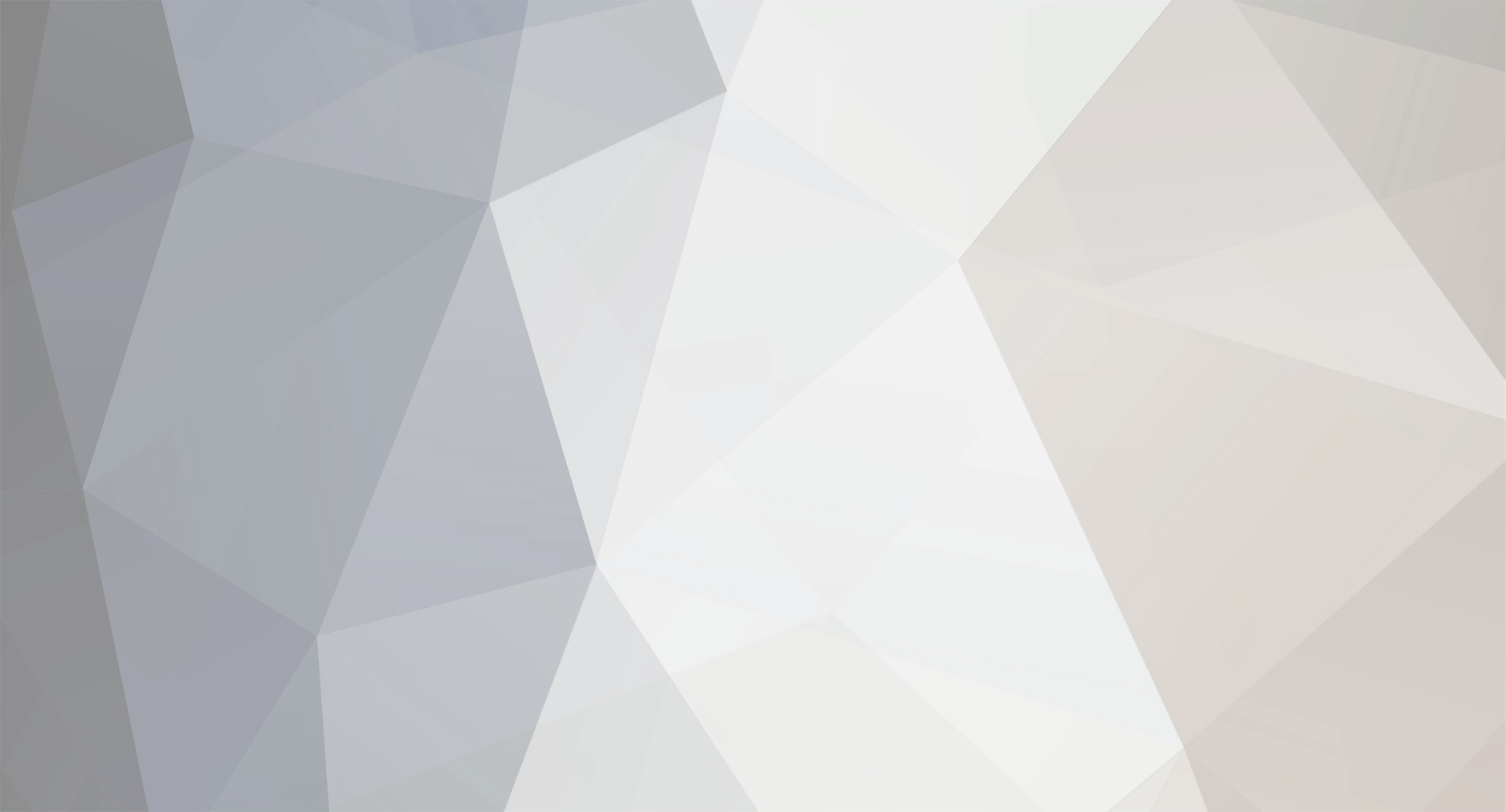
UberAffe
Forge Modder-
Posts
316 -
Joined
-
Last visited
Everything posted by UberAffe
-
java.lang.NoSuchMethodError on Minecraft launch
UberAffe replied to Adityagupta's topic in Modder Support
Proxy is used do separate registration code that is meant for the Physical client(logical client and logical server) and Physical server(logical server only) In your main mod file you use @SidedProxy(clientSide = Refs.CLIENTPROXY, serverSide = Refs.SERVERPROXY) public static CommonProxy proxy; CLIENTPROXY and SERVERPROXY are strings that would be equal to an import line. if an import would look like import modid.ClientProxy; @SidedProxy(clientSide = "modid.ClientProxy" your clientproxy class should extend common proxy. Your mod file will now have this: @SidedProxy(clientSide = Refs.CLIENTPROXY, serverSide = Refs.SERVERPROXY) public static CommonProxy proxy; @EventHandler public void preInit (FMLPreInitializationEvent event){ proxy.preInit(); } @EventHandler public void init (FMLInitializationEvent event){ proxy.init(); } @EventHandler public void postInit (FMLPostInitializationEvent event){ proxy.postInit(); } the majority of your registration will go in CommonProxy, and any client specific stuff (KeyBindings, GUI's, etc) will go in ClientProxy. -
Thanks, I'll take a look and see what I can find.
-
Items in my mod will designed by the player similar to tinkers but much more fine grained, to the point that a sword could be shaped like a bucket if that is how it was designed. I've got a pretty good idea of how to handle the properties of the item but frankly I suck at graphics. Is it feasible to render an item based on it's NBT and if so how would I attach the renderer to the item. This is what I had in mind for rendering an item: public class ItemRenderer { private static final int PARTDIM = 8; public void RenderItem(IDraftable draft){ IPartType[][][] parts = draft.GetPartArray(); int depth = draft.GetPartsDeep() * PARTDIM; int width = draft.GetPartsWide() * PARTDIM; int height = draft.GetPartsHigh() * PARTDIM; for(int i = 0; i < width; i++)//y position for(int j = 0; j < height; j++)//x position for(int k = 0; k < depth; k++)//z position parts[i][j][[k].GetRenderer().RenderPart(i,j,k);// render piece in location, pieces are partially transparent } } my items implement IDraftable and each IPartType implementation has an associated IPartRenderer implementation. Edit: I'm sure that the location won't be as simple as integers but is the underlying idea feasible?
-
This is the line that is throwing the error at least from what I can tell. CommonProxy.network.sendTo(new MessageIEEPChanged(prop.saveNBTData()), (EntityPlayerMP) player); I stepped through and checked player just before running this line and the player was instantiate and had all the expected information. I put a breakpoint in the runnable of MessageIEEPChanged and hit continue on the debugger and then the error happens. player is getting passed in and I make changes to the inventory and capability and those work. full method: full console trace:
-
Player capabilities not saving/loading[Solved]
UberAffe replied to UberAffe's topic in Modder Support
Thanks, I didn't see that in any of the examples I came across. Now I just need to convert my EntityJoinWorldEvent and I should be back up to where I was with IEEP. -
Capability not getting saved/loaded on world save/load. Using the same writeNBT and loadNBT methods for updating the client with changes works, so the methods themselves are writing to nbt and reading from it fine. public class DefaultDrafter implements IDrafter{ public static class DrafterStorage implements IStorage<IDrafter>{ public static final DrafterStorage drafterStorage = new DrafterStorage(); @Override public NBTBase writeNBT(Capability<IDrafter> capability, IDrafter instance, EnumFacing side) { NBTTagCompound nbtDrafterCapabilities = new NBTTagCompound(); NBTTagCompound nbtLuxinLevel = new NBTTagCompound(); NBTTagCompound nbtLuxinCap = new NBTTagCompound(); NBTTagCompound nbtLuxinAvailable = new NBTTagCompound(); NBTTagCompound nbtDraftables = new NBTTagCompound(); nbtDrafterCapabilities.setFloat("draft_cap", ((DefaultDrafter)instance).draftCap); nbtDrafterCapabilities.setFloat("draft_used", ((DefaultDrafter)instance).draftUsed); nbtDrafterCapabilities.setInteger("sick_ticks", ((DefaultDrafter)instance).sickTicks); nbtDrafterCapabilities.setString("drafting_selection", ((DefaultDrafter)instance).draftingSelection); for(String luxin : ((DefaultDrafter)instance).luxinLevel.keySet()) { nbtLuxinLevel.setFloat(luxin, ((DefaultDrafter)instance).luxinLevel.get(luxin)); nbtLuxinCap.setFloat(luxin, ((DefaultDrafter)instance).luxinCap.get(luxin)); nbtLuxinAvailable.setFloat(luxin, ((DefaultDrafter)instance).luxinAvailable.get(luxin)); } for(String draft : ((DefaultDrafter)instance).draftables.keySet()) nbtDraftables.setTag(draft, CoreFactory.saveNBT(((DefaultDrafter)instance).draftables.get(draft))); nbtDrafterCapabilities.setTag(CAP, nbtLuxinCap); nbtDrafterCapabilities.setTag(LEVEL, nbtLuxinLevel); nbtDrafterCapabilities.setTag(AVAILABLE, nbtLuxinAvailable); nbtDrafterCapabilities.setTag(DRAFTABLE, nbtDraftables); return nbtDrafterCapabilities; } @Override public void readNBT(Capability<IDrafter> capability, IDrafter instance, EnumFacing side, NBTBase nbt) { NBTTagCompound nbtExtendedProperties = (NBTTagCompound) nbt; NBTTagCompound nbtLuxinLevel = nbtExtendedProperties.getCompoundTag(LEVEL); NBTTagCompound nbtLuxinCap = nbtExtendedProperties.getCompoundTag(CAP); NBTTagCompound nbtLuxinAvailable = nbtExtendedProperties.getCompoundTag(AVAILABLE); NBTTagCompound nbtDraftables = nbtExtendedProperties.getCompoundTag(DRAFTABLE); ((DefaultDrafter)instance).draftCap = nbtExtendedProperties.getFloat("draft_cap"); ((DefaultDrafter)instance).draftUsed = nbtExtendedProperties.getFloat("draft_used"); ((DefaultDrafter)instance).sickTicks = nbtExtendedProperties.getInteger("sick_ticks"); ((DefaultDrafter)instance).draftingSelection = nbtExtendedProperties.getString("drafting_selection"); for(String luxin : nbtLuxinLevel.getKeySet()) { ((DefaultDrafter)instance).luxinLevel.put(luxin, nbtLuxinLevel.getFloat(luxin)); ((DefaultDrafter)instance).luxinCap.put(luxin, nbtLuxinCap.getFloat(luxin)); ((DefaultDrafter)instance).luxinAvailable.put(luxin, nbtLuxinAvailable.getFloat(luxin)); } for(String draft : ((DefaultDrafter)instance).draftables.keySet()) ((DefaultDrafter)instance).draftables.put(draft, CoreFactory.loadNBT((NBTTagCompound) nbtDraftables.getTag(draft))); } } } public class DraftingProvider implements ICapabilityProvider{ @CapabilityInject(IDrafter.class) public static Capability<IDrafter> CHROMATPLAYER = null; private IDrafter drafter = null; public DraftingProvider(){} public DraftingProvider(IDrafter drafter){ this.drafter = drafter; } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return CHROMATPLAYER != null && capability == CHROMATPLAYER; } @SuppressWarnings("unchecked") @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (CHROMATPLAYER != null && capability == CHROMATPLAYER) return (T)drafter; return null; } CommonProxy{ public void preInit(){ CapabilityManager.INSTANCE.register(IDrafter.class, DrafterStorage.drafterStorage, DefaultDrafter.class); } } and this in my EventHandler @SubscribeEvent public void AttachCapability(AttachCapabilitiesEvent.Entity e) { if(!e.getEntity().hasCapability(DraftingProvider.CHROMATPLAYER, null) && e.getEntity() instanceof EntityPlayer) e.addCapability(Refs.DRAFTER, new DraftingProvider(new DefaultDrafter())); } is there something I am missing? ------------------------ Solution: Implement INBTSerializable in your provider.
-
Draco18s: In IEEP I am giving the player mana and I want that mana to regen over time. I am assuming it is bad practice to just use an ITickable to perform the regen on the server and send an update packet to the client every tick. If possible I want to let the client perform its own regen for a short amount of time with occasional updates from the server. Choonster: Thanks, I'll take a look at that.
-
I have an IEEP stat that I want to regen per tick. What do I need to hook into to let the client do its own regen for x amount of ticks and request a sync on the x+1 tick?
-
Can you post your CommonProxy imports and init()
-
net.minecraft.server.MinecraftServer.registerTickable(Lnet/minecraft/util/ITickable;) that sounds like it is actually your playermana.get is the one having problems.
-
After a little debugging this is now updating the player on join, thanks for the help!
-
@SubscribeEvent public void entityJoinWorld(EntityJoinWorldEvent e) { ExtendedProperties data = null; if(e.entity instanceof EntityPlayerMP && LightDrafter.proxy.getWorld() != null) data = ExtendedProperties.get((EntityPlayer) e.entity); if (data != null) data.entitySpawned(); } CommonProxy public World getWorld() { return FMLCommonHandler.instance().getMinecraftServerInstance().worldServers[0]; } ClientProxy @Override public World getWorld() { return Minecraft.getMinecraft().theWorld; } In SMP this is catching only once and it is catching before the server has a world instance, which is stopping me from syncing player IEEP info. What can I do to trigger data.entitySpawned() when the server finishes loading?
-
Ok, so I stepped through things and I am getting this error java.util.concurrent.ExecutionException: java.lang.IllegalArgumentException: Empty string not allowed When I try to step over this -> nbtExtendedProperties.setString("drafting_selection", draftingSelection); for(String luxin : luxinLevel.keySet()) If I look at the contents of LuxinLevel before stepping over I see this {Blue=0.0, Green=0.0} Which is what I expect to be there. This is the declaration/ instantiation private HashMap<String, Float> luxinLevel = new HashMap<String, Float>(); any ideas as to why this is reporting an empty string? Edit: ------------------------------------ I'm dumb ... I was so fixated on the for loop that i didn't even notice that it was a string on the line before. I even included it in the code here ... had to instantiate that and once I did everything started working beautifully. Thanks for the help!
-
Looking at how the setBlockState method works it seems like you want to have a set of public IBlockState variables that you can use to set the block.
-
Simple Ways To Implement All Blocks List
UberAffe replied to Cloaking_Ocean's topic in Modder Support
what do you mean by minecraft block, are you asking for "Every Block" (Grass, Cobble, Piston, redstone, etc.) Are you trying to add similar blocks? trying to Reskin minecraft blocks? -
You will probably want to have logic to change blockbounds when you add bricks, and in your rendering class you will basically just section off the code that renders each brick behind if tests. I don't know if that is the recommended way of doing this but it will work.
-
I was hoping it was just some glaring thing I had missed. Oh well.
-
So I have been following this tutorial on IEEP. But my get seems to be returning something other than what I registered. I say this because all values that were set in the instructor are now set to 0. IEEP class Event Hooks Class that uses the get
-
Can I send the message from inside that runnable with: CommonProxy.network.sendTo(new MessageIEEPChanged(DraftingManager.PerformDrafting(player)), (EntityPlayerMP) player); or should I send the message from inside PerformDrafting?
-
Finally home again, and yes it is getting received. I guess that means there is something wrong with my save and load. Can you look over how I am passing the nbt information to make sure I didn't mess that up. Message code DraftingManager ExtendedProperties save and load are at the bottom.
-
I didn't specifically check but I registered it as side.client and changed ctx.serverhandler to minecraft.getminecraft
-
Here is a link to my code on github. Messagedrafting gets called by a keybinding and alters the players IEEP on the server. MessageIEEPchanged is getting called in messagedrafting to update the client. This setup is not updating the client. If I use the return of Messagedrafting the client freezes indefinitely. Any advice is appreciated. Solved: ----------------------- The solution is more of a trouble shooting list so if you have a similar problem take a look at the steps we took here.
-
I want to place an item into a players hotbar in the slot that they have selected, is this information I can get while server side or do I need to send the slot index from the client?
-
[1.8.9]How to tell when an item is removed from the players inventory?
UberAffe replied to Looke81's topic in Modder Support
I'm confused as to why you are going about things the way your are ... It sounds like you have a bauble that gives the user some buff as long as they have some other "fuel" item in their inventory. Can't you achieve what you want by using the baubles ontick event. Every x ticks look at the players inventory and if they have the item, give the buff for x ticks. If they don't have the item then the buff won't get refreshed. -
Please Check My Code for ERRORS regarding custom WorkBench[UNSOLVED]
UberAffe replied to avaikulis's topic in Modder Support
For mc, that is likely a private variable that was in the original class that you copied from but you didn't add. Should be Minecraft.getMinecraft() you can also get FontRendererObj from Minecraft.getMinecraft().fontRendererObj