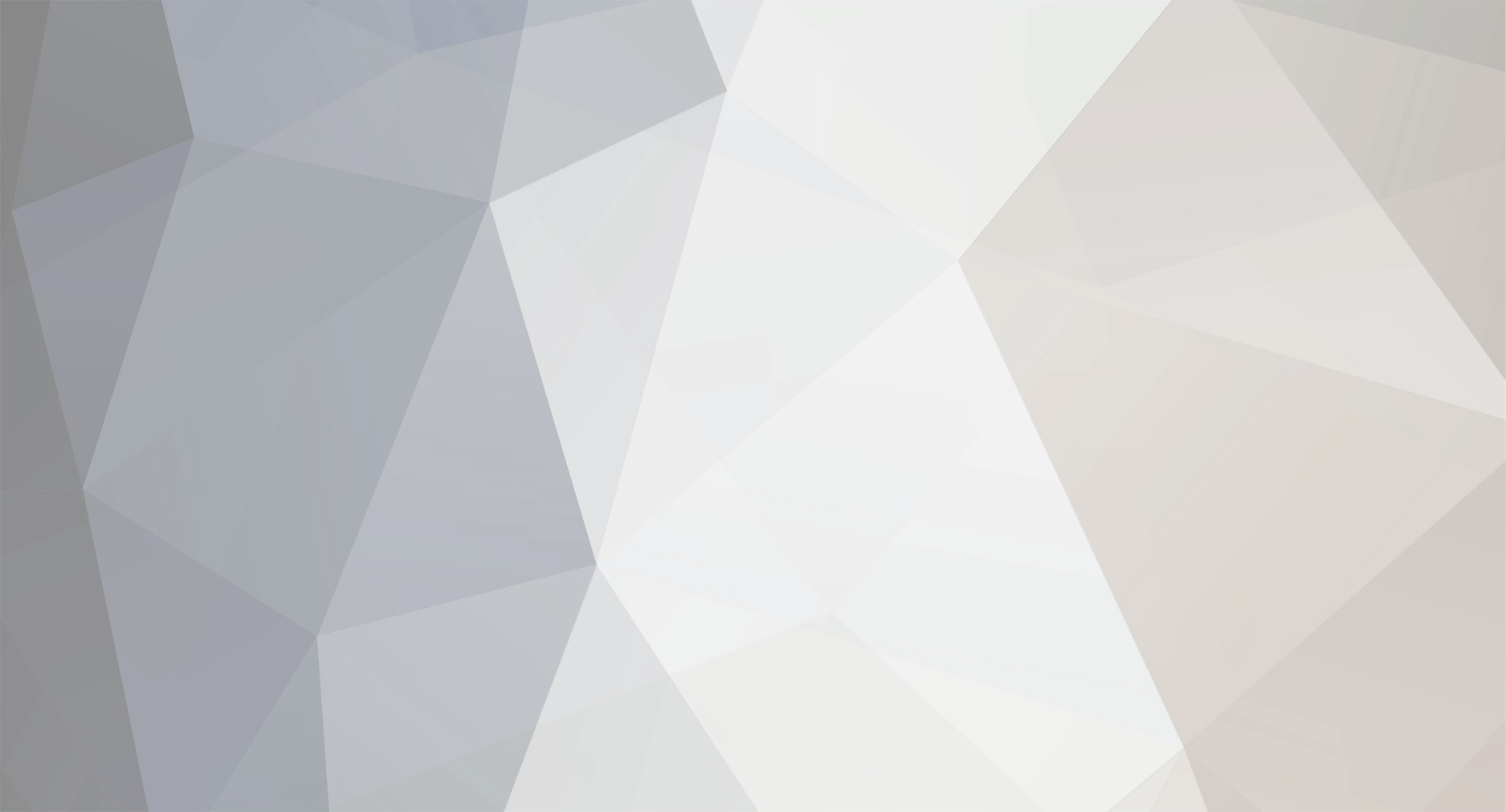
Raycoms
Members-
Posts
383 -
Joined
-
Last visited
Everything posted by Raycoms
-
Tessellator tessellator = Tessellator.getInstance(); WorldRenderer renderer = Tessellator.getInstance().getWorldRenderer(); renderer.begin(GL11.GL_QUADS, DefaultVertexFormats.BLOCK); // The default vertex format depends on your use case, experiment. For every vertex: worldrenderer.pos(x,y,z) // If you have u,v you have to add some additional stuff tessellator.draw()
-
1.7.10 Making my custom dimension a void world
Raycoms replied to Elrol_Arrowsend's topic in Modder Support
You probably could extend the worldChunkManagerHell class and overwrite the given methods. -
[1.8] [1.7] Rendering glitches in some areas [Unsolved]
Raycoms replied to Raycoms's topic in Modder Support
Through further debugging I found out that the problem seems to be that in the ForgeBlockModelRenderer class in the following method the quads always empty is and the render method, therefore, mostly returns false. It seems that for some blocks the method returns true but if(!quads.isEmpty() && (!checkSides || block.shouldSideBeRendered(world, pos.offset(side), side))) returns false and that is why these mods are rendered 2 dimensionally. It seems like block.shouldSideBeRendered returns a bad result and that's why sometimes a few sides are rendered and some other times no side at all. But, it is strange that the floor blocks are rendered 2 dimensionally but the others not. The stairs, torches and fence posts are rendered correctly because they have a EnumFacing. public static boolean render(VertexLighterFlat lighter, IBlockAccess world, IBakedModel model, Block block, BlockPos pos, WorldRenderer wr, boolean checkSides) { lighter.setWorld(world); lighter.setBlock(block); lighter.setBlockPos(pos); boolean empty = true; List quads = model.getGeneralQuads(); if(!quads.isEmpty()) { lighter.updateBlockInfo(); empty = false; Iterator var9 = quads.iterator(); while(var9.hasNext()) { BakedQuad quad = (BakedQuad)var9.next(); quad.pipe(lighter); } } EnumFacing[] var15 = EnumFacing.values(); int var16 = var15.length; for(int var11 = 0; var11 < var16; ++var11) { EnumFacing side = var15[var11]; quads = model.getFaceQuads(side); if(!quads.isEmpty() && (!checkSides || block.shouldSideBeRendered(world, pos.offset(side), side))) { if(empty) { lighter.updateBlockInfo(); } empty = false; Iterator var13 = quads.iterator(); while(var13.hasNext()) { BakedQuad quad1 = (BakedQuad)var13.next(); quad1.pipe(lighter); } } } return !empty; } It seems like these rendering issues come from a point where there is checked if the block on the side is opaque to decide if the block should be rendered and in some strange way, this is sometimes true when it should be false. But I have no Idea why this depends on the position and mostly happens in snow biomes. -
[1.8] [1.7] Rendering glitches in some areas [Unsolved]
Raycoms replied to Raycoms's topic in Modder Support
This is only reproducible in some random places but easily in any biome with ice/snow. Outside of this particular places, the rendering works perfectly well and even inside the snow biomes, in about 20-50% of the area the rendering works well. -
It seems to me that this method sets your block Translucent and not solid. @Override public BlockRenderLayer getBlockLayer() { return BlockRenderLayer.TRANSLUCENT; }
-
I ran into some pretty interesting rendering glitches in 1.7.10 as well as 1.8.9 in some areas, mostly in snowy biomes. I am at two different points in the same biome. At point 1 everything renders great. I click with my tool on the ground and the whole building renders perfectly. At point 2 everything renders very bad. I click with my tool on the ground and the blocks do not render at all or do render only 2 dimensional. The interesting thing. If I render the building from point 1 at point 2 it will work and also if I render it from point 2 to point 1 it won't. It seems that the position where the command is executed influences the correct or incorrect rendering and not the place where the blocks are actually rendered. Function: public void renderBlocks(int renderPass, int minX, int minY, int minZ, int maxX, int maxY, int maxZ) { IBlockAccess mcWorld = this.minecraft.theWorld; BlockRendererDispatcher renderBlocks = this.settings.renderBlocks; int x, y, z, wx, wy, wz; int sides; Block block, mcBlock; Vector3f zero = new Vector3f(); Vector3f size = new Vector3f(); int ambientOcclusion = this.minecraft.gameSettings.ambientOcclusion; this.minecraft.gameSettings.ambientOcclusion = 0; Tessellator tessellator = Tessellator.getInstance(); WorldRenderer renderer = Tessellator.getInstance().getWorldRenderer(); renderer.begin(GL11.GL_QUADS, DefaultVertexFormats.BLOCK); for (y = minY; y < maxY; y++) { for (z = minZ; z < maxZ; z++) { for (x = minX; x < maxX; x++) { try { block = this.schematic.getBlock(x, y, z); wx = (int) this.settings.offset.x + x; wy = (int) this.settings.offset.y + y; wz = (int) this.settings.offset.z + z; BlockPos pos = new BlockPos(x, y, z); BlockPos wPos = new BlockPos(wx, wy, wz); mcBlock = mcWorld.getBlockState(wPos).getBlock(); sides = 0; if (block != null) { if (y-1 > minY && block.shouldSideBeRendered(this.schematic, pos.down(), EnumFacing.DOWN)) { sides |= RenderHelper.QUAD_DOWN; } if (y+1 < maxY && block.shouldSideBeRendered(this.schematic, pos.up(), EnumFacing.UP)) { sides |= RenderHelper.QUAD_UP; } if (z-1 > minZ && block.shouldSideBeRendered(this.schematic, pos.north(), EnumFacing.NORTH)) { sides |= RenderHelper.QUAD_NORTH; } if (z+1 < maxZ && block.shouldSideBeRendered(this.schematic, pos.south(), EnumFacing.SOUTH)) { sides |= RenderHelper.QUAD_SOUTH; } if (x-1 > minX && block.shouldSideBeRendered(this.schematic, pos.west(), EnumFacing.WEST)) { sides |= RenderHelper.QUAD_WEST; } if (x+1 < maxX && block.shouldSideBeRendered(this.schematic, pos.east(), EnumFacing.EAST)) { sides |= RenderHelper.QUAD_EAST; } } boolean isAirBlock = mcWorld.isAirBlock(wPos); if (!isAirBlock && mcBlock != Blocks.snow_layer) { if (Config.highlight && renderPass == 2) { if (block == Blocks.air && Config.highlightAir) { zero.set(x, y, z); size.set(x + 1, y + 1, z + 1); if (Config.drawQuads) { RenderHelper.drawCuboidSurface(zero, size, RenderHelper.QUAD_ALL, 0.75f, 0.0f, 0.75f, 0.25f); } if (Config.drawLines) { RenderHelper.drawCuboidOutline(zero, size, RenderHelper.LINE_ALL, 0.75f, 0.0f, 0.75f, 0.25f); } } else if (block != mcBlock) { zero.set(x, y, z); size.set(x + 1, y + 1, z + 1); if (Config.drawQuads) { RenderHelper.drawCuboidSurface(zero, size, sides, 1.0f, 0.0f, 0.0f, 0.25f); } if (Config.drawLines) { RenderHelper.drawCuboidOutline(zero, size, sides, 1.0f, 0.0f, 0.0f, 0.25f); } } else if (this.schematic.getBlockState(pos) != mcWorld.getBlockState(wPos)) { zero.set(x, y, z); size.set(x + 1, y + 1, z + 1); if (Config.drawQuads) { RenderHelper.drawCuboidSurface(zero, size, sides, 0.75f, 0.35f, 0.0f, 0.25f); } if (Config.drawLines) { RenderHelper.drawCuboidOutline(zero, size, sides, 0.75f, 0.35f, 0.0f, 0.25f); } } } } else if (block != Blocks.air) { if (Config.highlight && renderPass == 2) { zero.set(x, y, z); size.set(x + 1, y + 1, z + 1); if (Config.drawQuads) { RenderHelper.drawCuboidSurface(zero, size, sides, 0.0f, 0.75f, 1.0f, 0.25f); } if (Config.drawLines) { RenderHelper.drawCuboidOutline(zero, size, sides, 0.0f, 0.75f, 1.0f, 0.25f); } } if (block != null && block.getBlockLayer().ordinal() == renderPass && block != Blocks.wooden_pressure_plate) { renderBlocks.renderBlock(this.schematic.getBlockState(new BlockPos(x,y,z)), new BlockPos(x,y,z), mcWorld, renderer); } } } catch (Exception e) { Log.logger.error("Failed to render block!", e); } } } } tessellator.draw(); this.minecraft.gameSettings.ambientOcclusion = ambientOcclusion; } Whole code: https://bitbucket.org/cltnschlosser/minecolonies/src/af6bc4ceddc5c7f6e7a60e3dde9f0c95424f9b57/src/main/java/com/schematica/client/renderer/RendererSchematicChunk.java?at=master&fileviewer=file-view-default This code is executed from a "build-tool" which has a GUI with buttons to execute the rendering. Here it is rendered correctly (When rendering from the top green block) https://www.dropbox.com/s/2jgh2yt5lashghj/2016-04-21_20.40.47.png?dl=0 Here it is rendered incorrectly (When rendering from the top red block) https://www.dropbox.com/s/ye9scw4t7rfphdd/2016-04-21_20.41.10.png?dl=0 Here is it rendered correctly(When rendering from the top green block) https://www.dropbox.com/s/wmfaqdlqbur5igo/2016-04-21_20.41.24.png?dl=0 and here again rendered incorrectly(Rendered from the top red block) https://www.dropbox.com/s/rmri3stuib9x5os/2016-04-21_20.41.40.png?dl=0
-
Solved it. It seems that I have to execute mc.renderEngine.bindTexture(TEXTURE); before drawing any string. If any minecraft text is displayed(F3, chat, etc) this will automatically set the minecraft textures and therefore it will display the Strings conforming this texture. The problem with this is, if you want to draw any images, this may destroy the images.
-
No, I do not call these events manually. After InitGui() has finished. These forge events are automatically called. Window.onOpen Instantiates the text.
-
This init gui is called by entityInteraction (No magic in this code) public class Screen extends GuiScreen { @Override public void drawScreen(int mx, int my, float f) { if (window.hasLightbox()) { super.drawDefaultBackground(); } scale = new ScaledResolution(mc).getScaleFactor(); GL11.glPushMatrix(); GL11.glTranslatef(x, y, 0); window.draw(mx - x, my - y); GL11.glPopMatrix(); } @Override public void initGui() { x = (width - window.getWidth()) / 2; y = (height - window.getHeight()) / 2; Keyboard.enableRepeatEvents(true); window.onOpened(); } Which calls the onOpened in the Window. Which eventually calls MinecraftForge.EVENT_BUS.post(new net.minecraftforge.client.event.GuiScreenEvent.InitGuiEvent.Post(this, this.buttonList)); and then eventually public void showGuiScreen(Object clientGuiElement) { GuiScreen gui = (GuiScreen)clientGuiElement; this.client.displayGuiScreen(gui); } which contains our custom gui. and therefor, eventually the drawSelf from our custom guy (which I already posted) is called...
-
Ah understood. The previously mentioned View extends the minecraft Gui. and we draw the stuff like you mentioned: "Opening a custom GuiScreen (stuff like guis like GuiContainer, MainMenu, Options, etc.)"
-
I bound an entity object to a "custom window" object. This windows object is called when I right click my entity. /** * Called when the gui is opened by an player. */ @Override public void onOpened() { findPaneOfTypeByID(WINDOW_ID_NAME, Label.class).setLabel(citizen.getName()); createHealthBar(); createXpBar(); createSkillContent(); } This fills various different Label.class with text (Some of them work correctly, some not) This window extends a view with the method drawSelf. This draw methods calls all the drawSelf methods of its childs (Labels, Images etc) @Override protected void drawSelf(int mx, int my) { // Translate the drawing origin to our x,y GL11.glPushMatrix(); GL11.glTranslatef((float)x + padding, (float)y + padding, 0); // Translate Mouse into the View mx -= x + padding; my -= y + padding; for (Pane child : children) { if (childIsVisible(child)) { child.draw(mx, my); } } GL11.glPopMatrix(); } from there the drawSelf of the label is called. But all my images, icons and buttons works 100% correctly. Only text is sometimes displayed correctly, sometimes not.
-
The Gui text is rendered correctly if whatever text enters the console. May be some code, may be some chat. The text renders exactly in the console text color.
-
I am rendering on "rightclick block or entity" This is the function: @Override public void drawSelf(int mx, int my) { int color = isPointInPane(mx, my) ? hoverColor : textColor; int offsetX = 0; int offsetY = 0; if (textAlignment.rightAligned) { offsetX = (getWidth() - getStringWidth()); } else if (textAlignment.horizontalCentered) { offsetX = (getWidth() - getStringWidth()) / 2; } if (textAlignment.bottomAligned) { offsetY = (getHeight() - getTextHeight()); } else if (textAlignment.verticalCentered) { offsetY = (getHeight() - getTextHeight()) / 2; } GL11.glPushMatrix(); GL11.glTranslated(getX() + offsetX, getY() + offsetY, 0); GL11.glScalef(scale, scale, scale); mc.fontRendererObj.drawString(label, 0, 0, color, shadow); GL11.glPopMatrix(); }
-
In 1.7.10 the text in my mods GUI's always has been rendered correctly. After porting to 1.8.9 my custom labels only sometimes render it correctly. mc.fontRendererObj.drawString(label, 0, 0, color, shadow); My label extends a custom pane which extends the minecraft gui. Sometimes of the text is displayed correctly the other part looks pretty strange. The strangest thing is, that when I execute console commands (/time set 0... /weather clear) all the labels which previously bugged are now displayed how they should be.
-
May that change in 1.8.9? Since we're updating to 1.8.9 atm.
-
The Method is called by an EntityAi class.
-
Setting these "motion" variables didn't resolve anything. Using "moveEntity" with + 0.001 did despawn the entity.
-
Moving like actual let them walk or also: this.setPositionAndUpdate(posX+InsignificantAmount, posY, posZ);
-
Adding: this.setPositionAndUpdate(posX, posY, posZ); Still doesn't affect that the entity turns into his correct rotation.
-
I am setting it directly in the entity (So client I guess). I used the faceEntity method and changed it to faceBlock. After the execution of the code the rotation variables are correctly set ( Fishinghook is thrown in the correct direction). But as said the entity will still face the previous direction until an event occurs. public void faceBlock(ChunkCoordinates block) { double xDifference = block.posX - this.posX; double zDifference = block.posZ - this.posZ; double yDifference = block.posY - (this.posY + (double) this.getEyeHeight() - 0.5); double squareDifference = Math.sqrt(xDifference * xDifference + zDifference * zDifference); double intendedRotationYaw = (Math.atan2(zDifference, xDifference) * 180.0D / Math.PI) - 90.0; double intendedRotationPitch = (-(Math.atan2(yDifference, squareDifference) * 180.0D / Math.PI)); this.rotationPitch = (float) this.updateRotation(this.rotationPitch, intendedRotationPitch, 30); this.rotationYaw = (float) this.updateRotation(this.rotationYaw, intendedRotationYaw, 30); } private double updateRotation(double currentRotation, double intendedRotation, double maxIncrement) { double wrappedAngle = MathHelper.wrapAngleTo180_double(intendedRotation - currentRotation); if (wrappedAngle > maxIncrement) { wrappedAngle = maxIncrement; } if (wrappedAngle < -maxIncrement) { wrappedAngle = -maxIncrement; } return currentRotation + wrappedAngle; }
-
I have some problems with the entity rotations. When I set the rotationYaw and Pitch parameters the entity only rotates after a time or after the entity has been hit or "touched". Is there a way to accelerate this process?