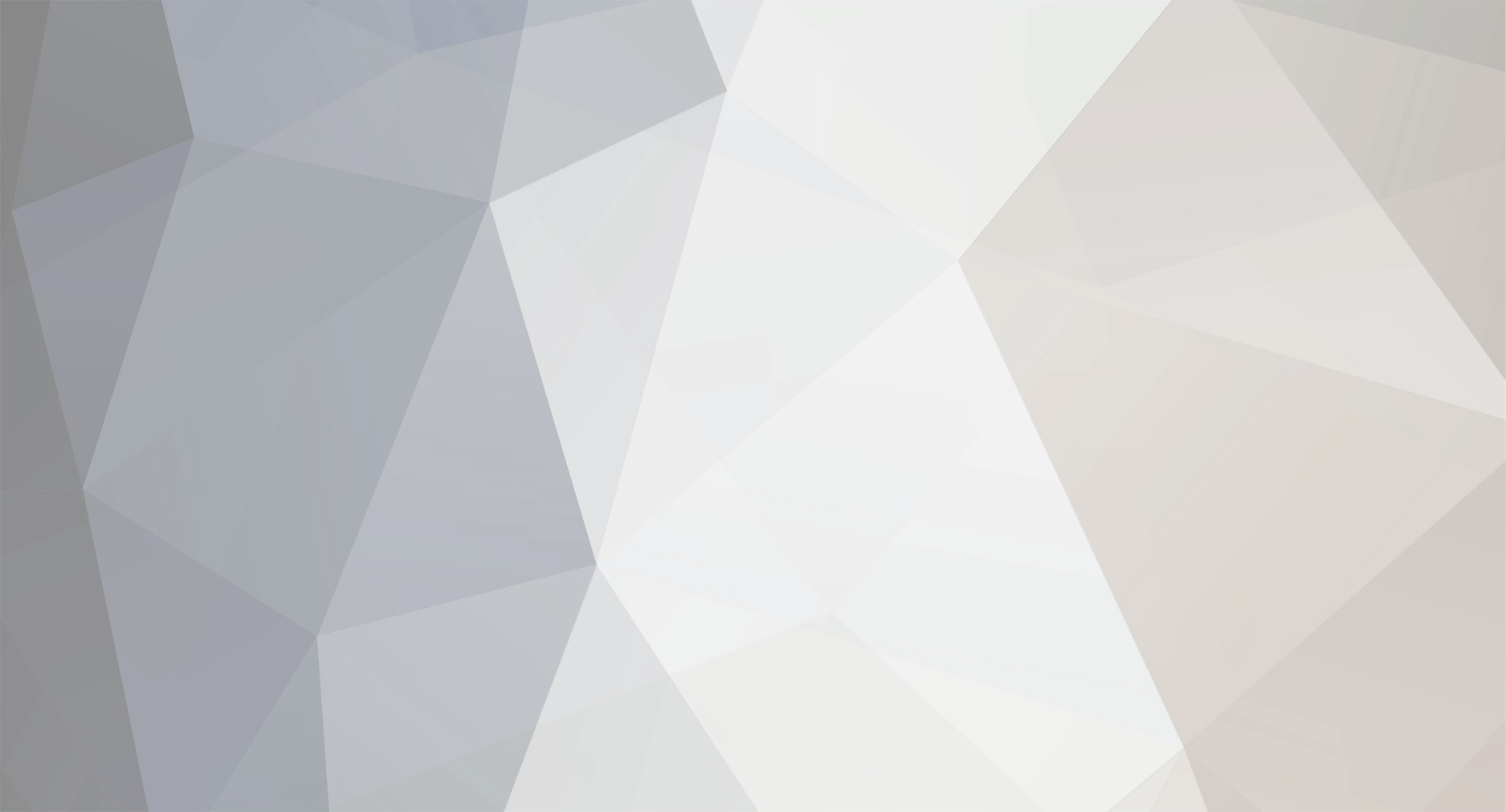
Raycoms
Members-
Posts
383 -
Joined
-
Last visited
Everything posted by Raycoms
-
We are printing several types of images into our GUI's using the following code. this.mc.getTextureManager().bindTexture(resourceLocation); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); //Draw drawModalRectWithCustomSizedTexture(x, y, imageOffsetX, imageOffsetY, imageWidth != 0 ? imageWidth : getWidth(), imageHeight != 0 ? imageHeight : getHeight(), mapWidth, mapHeight); This usually works very well. But if our resource is the icons.png from minecraft and we turn on f3 the images messes completely up and displays some white strange artifacts. This also happens if any text is written out ingame. Strangely this does not happen if we put the following line of code before the bingTexture. this.mc.getTextureManager().tick();
-
I have a problem with the synchronization between tileEntities. I am getting my tileEntity with world.getTileEntity with the server side representation of the world and I will set the custom name of the inventory of the tile entity. ScarecrowTileEntity scarecrow = (ScarecrowTileEntity) world.getTileEntity(field.getID()); scarecrow.getInventoryField().setCustomName("Myname"); On the other side I try to retrieve this in the CustomGUI @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { final BlockPos pos = new BlockPos(x,y,z); final ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new GuiField(player.inventory, tileEntity, world, pos); } Where the name hasn't been set correctly. I tried to mark the tileEntity dirty but that didn't help. Anyone any idea? /** * The scarecrow tile entity to store extra data. */ public class ScarecrowTileEntity extends TileEntityChest { /** * NBTTag to store the type. */ private static final String TAG_TYPE = "type"; /** * Random generator. */ private final Random random = new Random(); /** * The inventory connected with the scarecrow. */ private InventoryField inventoryField; /** * The type of the scarecrow. */ private ScareCrowType type; /** * Creates an instance of the tileEntity. */ public ScarecrowTileEntity() { super(); this.inventoryField = new InventoryField(LanguageHandler.format("com.minecolonies.gui.scarecrow.user", LanguageHandler.format("com.minecolonies.gui.scarecrow.user.noone"))); } @Override public void onLoad() { super.onLoad(); World world = getWorld(); if(world != null) { Colony colony = ColonyManager.getColony(world, pos); if (colony != null && colony.getField(pos) == null) { Entity entity = EntityUtils.getEntityFromUUID(world, colony.getPermissions().getOwner()); if (entity instanceof EntityPlayer) { colony.addNewField(this, ((EntityPlayer) entity).inventory, pos, world); } } } } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); type = ScareCrowType.values()[compound.getInteger(TAG_TYPE)]; getInventoryField().readFromNBT(compound); } @Override public void writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger(TAG_TYPE, this.getType().ordinal()); getInventoryField().writeToNBT(compound); } /** * Returns the type of the scarecrow (Important for the rendering). * * @return the enum type. */ public ScareCrowType getType() { if (this.type == null) { this.type = ScareCrowType.values()[this.random.nextInt(2)]; } return this.type; } /** * Set the inventory connected with the scarecrow. * * @param inventoryField the field to set it to */ public final void setInventoryField(final InventoryField inventoryField) { this.inventoryField = inventoryField; } /** * Get the inventory connected with the scarecrow. * * @return the inventory field of this scarecrow */ public InventoryField getInventoryField() { return inventoryField; } /** * Enum describing the different textures the scarecrow has. */ public enum ScareCrowType { PUMPKINHEAD, NORMAL } }
-
Thanks already What do these numbers mean? First probably x and y offset, but the third? this.fontRendererObj.drawString(tileEntity.getInventoryField().getDisplayName().getUnformattedText(), 8, 6, 4210752);
-
I have a custom GUI with a custom background and an inventory slot in the middle Is there a way to just draw a string out over my background somewhere? And if yes how do I do it? @SideOnly(Side.CLIENT) public class GuiField extends GuiContainer { /** * The resource location of the GUI background. */ private static final ResourceLocation TEXTURE = new ResourceLocation(Constants.MOD_ID, "textures/gui/scarecrow.png"); /** * Constructor of the GUI. * * @param parInventoryPlayer the player inventory. * @param tileEntity the tileEntity of the field, contains the inventory. * @param world the world the field is in. * @param location the location the field is at. */ protected GuiField(InventoryPlayer parInventoryPlayer, ScarecrowTileEntity tileEntity, World world, BlockPos location) { super(new Field(tileEntity, parInventoryPlayer, world, location)); } /** * Does draw the background of the GUI. * * @param partialTicks the ticks delivered. * @param mouseX the mouseX position. * @param mouseY the mouseY position. */ @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { //todo add field owned by x. GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(TEXTURE); final int marginHorizontal = (width - xSize) / 2; final int marginVertical = (height - ySize) / 2; drawTexturedModalRect(marginHorizontal, marginVertical, 0, 0, xSize, ySize); } }
-
Custom inventory field. package com.minecolonies.inventory; /** * The custom chest of the field. */ public class InventoryField extends InventoryCitizen { /** * NBTTag to store the slot. */ private static final String TAG_SLOT = "slot"; /** * NBTTag to store the items. */ private static final String TAG_ITEMS = "items"; /** * NBTTag to store the custom name. */ private static final String TAG_CUSTOM_NAME = "name"; /** * NBTTag to store the inventory. */ private static final String TAG_INVENTORY = "inventory"; /** * Returned slot if no slat has been found. */ private static final int NO_SLOT = -1; /** * The inventory stack. */ private ItemStack[] stackResult = new ItemStack[1]; /** * The custom name of the inventory. */ private String customName = ""; /** * Creates the inventory of the citizen. * * @param title Title of the inventory. * @param localeEnabled Boolean whether the inventory has a custom name. */ public InventoryField(final String title, final boolean localeEnabled) { super(title, localeEnabled); customName = title; } @Override public int getSizeInventory() { return 1; } @Override public int getInventoryStackLimit() { return 1; } @Override public int getHotbarSize() { return 0; } /** * Getter for the stack in the inventory. Since there is only one slot return always that one. * @param index the slot. * @return the itemStack in it. */ @Override public ItemStack getStackInSlot(int index) { return this.stackResult[0]; } @Override public boolean hasCustomName() { return true; } /** * Sets the given item stack to the specified slot in the inventory (can be crafting or armor sections). * @param index the slot to set the itemStack. * @param stack the itemStack to set. */ @Override public void setInventorySlotContents(int index, ItemStack stack) { this.stackResult[index] = stack; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) { stack.stackSize = this.getInventoryStackLimit(); } this.markDirty(); } /** * Get the name of this object. For citizens this returns their name. * @return the name of the inventory. */ @Override public String getName() { return this.hasCustomName() ? this.customName : "field.inventory"; } /** * Used to retrieve variables. * @param compound with the give tag. */ @Override public void readFromNBT(NBTTagCompound compound) { final NBTTagList nbttaglist = compound.getTagList(TAG_ITEMS, Constants.NBT.TAG_COMPOUND); this.stackResult = new ItemStack[this.getSizeInventory()]; for (int i = 0; i < nbttaglist.tagCount(); ++i) { final NBTTagCompound nbttagcompound = nbttaglist.getCompoundTagAt(i); final int j = nbttagcompound.getByte(TAG_SLOT) & Byte.MAX_VALUE; if (j != NO_SLOT && j < this.stackResult.length) { this.stackResult[j] = ItemStack.loadItemStackFromNBT(nbttagcompound); } } if (compound.hasKey(TAG_CUSTOM_NAME, Constants.NBT.TAG_STRING)) { this.customName = compound.getString(TAG_CUSTOM_NAME); } } /** * Used to store variables. * @param compound with the given tag. */ @Override public void writeToNBT(NBTTagCompound compound) { final NBTTagList nbttaglist = new NBTTagList(); for (int i = 0; i < this.stackResult.length; ++i) { if (this.stackResult[i] != null) { final NBTTagCompound nbttagcompound = new NBTTagCompound(); nbttagcompound.setByte(TAG_SLOT, (byte) i); this.stackResult[i].writeToNBT(nbttagcompound); nbttaglist.appendTag(nbttagcompound); } } compound.setTag(TAG_ITEMS, nbttaglist); if (this.hasCustomName()) { compound.setString(TAG_CUSTOM_NAME, this.customName); } compound.setTag(TAG_INVENTORY, nbttaglist); } } Inherits from inventory citizen package com.minecolonies.inventory; import com.minecolonies.colony.materials.MaterialStore; import com.minecolonies.colony.materials.MaterialSystem; import com.minecolonies.colony.permissions.Permissions; import com.minecolonies.entity.EntityCitizen; import net.minecraft.crash.CrashReport; import net.minecraft.crash.CrashReportCategory; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.IInventory; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.util.ChatComponentText; import net.minecraft.util.ChatComponentTranslation; import net.minecraft.util.IChatComponent; import net.minecraft.util.ReportedException; import net.minecraftforge.common.util.Constants; /** * Basic inventory for the citizens. */ public class InventoryCitizen implements IInventory { /** * Number of slots in the inventory. */ private static final int INVENTORY_SIZE = 27; /** * Max size of the stacks. */ private static final int MAX_STACK_SIZE = 64; /** * The inventory content. */ private ItemStack[] stacks = new ItemStack[iNVENTORY_SIZE]; /** * The inventories custom name. In our case the citizens name. */ private String customName; /** * The held item. */ private int heldItem; /** * The material store object. */ private MaterialStore materialStore; /** * NBT tag to store and retrieve the inventory. */ private static final String TAG_INVENTORY = "Inventory"; /** * NBT tag to store and retrieve the custom name. */ private static final String TAG_CUSTOM_NAME = "CustomName"; /** * NBT tag to store and retrieve the custom name. */ private static final String TAG_ITEMS = "Items"; /** * NBT tag to store and retrieve the custom name. */ private static final String TAG_SLOT= "Slot"; /** * Updated after the inventory has been changed */ private boolean inventoryChanged = false; /** * The returned slot if a slot hasn't been found. */ private static final int NO_SLOT = -1; /** * The citizen which owns the inventory. */ private EntityCitizen citizen; /** * Creates the inventory of the citizen. * * @param title Title of the inventory. * @param localeEnabled Boolean whether the inventory has a custom name. * @param citizen Citizen owner of the inventory. */ public InventoryCitizen(String title, boolean localeEnabled, EntityCitizen citizen) { this.citizen = citizen; if(localeEnabled) { customName = title; } } /** * Creates the inventory of the citizen. * * @param title Title of the inventory. * @param localeEnabled Boolean whether the inventory has a custom name. */ public InventoryCitizen(String title, boolean localeEnabled) { if(localeEnabled) { customName = title; } } private int getInventorySlotContainItem(Item itemIn) { for (int i = 0; i < this.stacks.length; ++i) { if (this.stacks[i] != null && this.stacks[i].getItem() == itemIn) { return i; } } return NO_SLOT; } /** * stores an itemstack in the users inventory */ private int storeItemStack(ItemStack itemStackIn) { for (int i = 0; i < this.stacks.length; ++i) { if (this.stacks[i] != null && this.stacks[i].getItem() == itemStackIn.getItem() && this.stacks[i].isStackable() && this.stacks[i].stackSize < this.stacks[i].getMaxStackSize() && this.stacks[i].stackSize < this.getInventoryStackLimit() && (!this.stacks[i].getHasSubtypes() || this.stacks[i].getMetadata() == itemStackIn.getMetadata()) && ItemStack.areItemStackTagsEqual(this.stacks[i], itemStackIn)) { return i; } } return NO_SLOT; } /** * Returns the first item stack that is empty. * * @return the id of the first empty slot. */ public int getFirstEmptyStack() { for (int i = 0; i < this.stacks.length; ++i) { if (this.stacks[i] == null) { return i; } } return NO_SLOT; } /** * Get the size of the citizens hotbar inventory * @return the size. */ public int getHotbarSize() { return 0; } /** * Contains the size of the inventory. * @return the size. */ @Override public int getSizeInventory() { return INVENTORY_SIZE; } /** * Gets the stack of a certain slot. * @param index the slot. * @return the ItemStack. */ @Override public ItemStack getStackInSlot(int index) { return this.stacks[index]; } /** * Removes up to a specified number of items from an inventory slot and returns them in a new stack. * @param index the slot of the itemStack. * @param count the amount of items to reduce. * @return the resulting stack. */ @Override public ItemStack decrStackSize(int index, int count) { if (this.stacks[index] != null) { if (this.stacks[index].stackSize <= count) { ItemStack itemstack1 = this.stacks[index]; this.stacks[index] = null; this.markDirty(); if(index == heldItem) { if(citizen != null) { citizen.removeHeldItem(); } heldItem = 0; } return itemstack1; } else { ItemStack itemstack = this.stacks[index].splitStack(count); if (this.stacks[index].stackSize == 0) { this.stacks[index] = null; } this.markDirty(); return itemstack; } } else { return null; } } /** * Removes a stack from the given slot and returns it. * @param index the slot of the stack. * @return the removed itemStack. */ @Override public ItemStack removeStackFromSlot(int index) { if (this.stacks[index] != null) { ItemStack itemstack = this.stacks[index]; this.stacks[index] = null; return itemstack; } else { return null; } } /** * Sets the given item stack to the specified slot in the inventory (can be crafting or armor sections). * @param index the slot to set the itemStack. * @param stack the itemStack to set. */ @Override public void setInventorySlotContents(int index, ItemStack stack) { if(index == heldItem && stack == null) { if(citizen != null) { citizen.removeHeldItem(); } heldItem = 0; } this.stacks[index] = stack; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) { stack.stackSize = this.getInventoryStackLimit(); } this.markDirty(); } /** * Get the name of this object. For citizens this returns their name. * @return the name of the inventory. */ @Override public String getName() { return this.hasCustomName() ? this.customName : "citizen.inventory"; } /** * Sets the name of the inventory. * @param customName the string to use to set the name. */ public void setCustomName(String customName) { this.customName = customName; } /** * Checks if the inventory is named. * @return true if the inventory has a custom name. */ @Override public boolean hasCustomName() { return this.customName != null; } /** * Get the formatted ChatComponent that will be used for the sender's username in chat */ @Override public IChatComponent getDisplayName() { return this.hasCustomName() ? new ChatComponentText(this.getName()) : new ChatComponentTranslation(this.getName()); } /** * Contains the maximum stack size for a inventory slot. Seems to always be 64, possibly will be extended. * @return the stack size. */ @Override public int getInventoryStackLimit() { return MAX_STACK_SIZE; } /** * For tile entities, ensures the chunk containing the tile entity is saved to disk later - the game won't think it * hasn't changed and skip it. */ @Override public void markDirty() { this.inventoryChanged = true; } /** * Checks if the inventory has been changed and then resets the boolean. * @return true if it changed. */ public boolean hasInventoryChanged() { if(inventoryChanged) { inventoryChanged = false; return true; } return false; } /** * Do not give this method the name canInteractWith because it clashes with Container * @param player the player acessing the inventory. * @return if the player is allowed to access. */ @Override public boolean isUseableByPlayer(EntityPlayer player) { return this.citizen.getColony().getPermissions().hasPermission(player, Permissions.Action.ACCESS_HUTS); } /** * Called when inventory is opened by a player. * @param player the player who opened the inventory. */ @Override public void openInventory(EntityPlayer player) { /* * This may be filled in order to specify some custom handling. */ } /** * Called after the inventory has been closed by a player. * @param player the player who opened the inventory. */ @Override public void closeInventory(EntityPlayer player) { /* * This may be filled in order to specify some custom handling. */ } /** * Returns true if automation is allowed to insert the given stack (ignoring stack size) into the given slot. * @param index the accessing slot. * @param stack the stack trying to enter. * @return if the stack may be inserted. */ @Override public boolean isItemValidForSlot(int index, ItemStack stack) { return true; } /** * This may be used in order to return values of different GUI areas like the ones in the beacon. * @param id the id of the field. * @return the value of the field. */ @Override public int getField(int id) { return 0; } /** * This may be used to set GUI areas with a certain id and value. * @param id some id. * @param value some value. */ @Override public void setField(int id, int value) { /* * We currently need no fields. */ } /** * Returns the number of fields. * @return the amount. */ @Override public int getFieldCount() { return 0; } /** * Completely clears the inventory. */ @Override public void clear() { for (int i = 0; i < this.stacks.length; ++i) { this.stacks[i] = null; } } /** * Set item to be held by citizen * * @param slot Slot index with item to be held by citizen */ public void setHeldItem(int slot) { this.heldItem = slot; } /** * Returns the item that is currently being held by citizen * * @return {@link ItemStack} currently being held by citizen */ public ItemStack getHeldItem() { return getStackInSlot(heldItem); } /** * This function stores as many items of an ItemStack as possible in a matching slot and returns the quantity of * left over items. */ private int storePartialItemStack(ItemStack itemStackIn) { int i = itemStackIn.stackSize; int j = this.storeItemStack(itemStackIn); if (j < 0) { j = this.getFirstEmptyStack(); } if (j < 0) { return i; } else { if (this.stacks[j] == null) { // Forge: Replace Item clone above to preserve item capabilities when picking the item up. this.stacks[j] = itemStackIn.copy(); this.stacks[j].stackSize = 0; } int k = i; if (i > this.stacks[j].getMaxStackSize() - this.stacks[j].stackSize) { k = this.stacks[j].getMaxStackSize() - this.stacks[j].stackSize; } if (k > this.getInventoryStackLimit() - this.stacks[j].stackSize) { k = this.getInventoryStackLimit() - this.stacks[j].stackSize; } if (k == 0) { return i; } else { i = i - k; this.stacks[j].stackSize += k; return i; } } } /** * Removes one item of specified Item from inventory (if it is in a stack, the stack size will reduce with 1) * @param itemIn the item to consume. * @return true if succeed. */ public boolean consumeInventoryItem(Item itemIn) { int i = this.getInventorySlotContainItem(itemIn); if (i < 0) { return false; } else { --this.stacks[i].stackSize; if (this.stacks[i].stackSize <= 0) { this.stacks[i] = null; } return true; } } /** * Adds the item stack to the inventory, returns false if it is impossible. * @param itemStackIn the stack to add * @return true if succeeded. */ public boolean addItemStackToInventory(final ItemStack itemStackIn) { if (itemStackIn != null && itemStackIn.stackSize != 0 && itemStackIn.getItem() != null) { try { if (itemStackIn.isItemDamaged()) { int j = this.getFirstEmptyStack(); if (j != NO_SLOT) { this.stacks[j] = ItemStack.copyItemStack(itemStackIn); itemStackIn.stackSize = 0; return true; } else { return false; } } else { int i; while (true) { i = itemStackIn.stackSize; itemStackIn.stackSize = this.storePartialItemStack(itemStackIn); if (itemStackIn.stackSize <= 0 || itemStackIn.stackSize >= i) { break; } } return itemStackIn.stackSize < i; } } catch (RuntimeException exp) { CrashReport crashreport = CrashReport.makeCrashReport(exp, "Adding item to inventory"); CrashReportCategory crashreportcategory = crashreport.makeCategory("Item being added"); crashreportcategory.addCrashSection("Item ID", Item.getIdFromItem(itemStackIn.getItem())); crashreportcategory.addCrashSection("Item data", itemStackIn.getMetadata()); crashreportcategory.addCrashSectionCallable("Item name", itemStackIn::getDisplayName); throw new ReportedException(crashreport); } } else { return false; } } /** * Checks if a specified Item is inside the inventory * @param itemIn the item to check for. * @return if itemIn in inventory. */ public boolean hasItem(Item itemIn) { return getInventorySlotContainItem(itemIn) != NO_SLOT; } /** * Gets slot that hold item that is being held by citizen. * {@link #getHeldItem()}. * * @return Slot index of held item */ public int getHeldItemSlot() { return heldItem; } /** * Checks if a certain slot is empty. * @param index the slot. * @return true if empty. */ public boolean isSlotEmpty(int index) { return getStackInSlot(index) == null; } //-----------------------------Material Handling-------------------------------- public void createMaterialStore(MaterialSystem system) { if (materialStore == null) { materialStore = new MaterialStore(MaterialStore.Type.INVENTORY, system); } } public MaterialStore getMaterialStore() { return materialStore; } //todo missing now /* @Override public ItemStack getStackInSlotOnClosing(int index) { ItemStack removed = super.getStackInSlotOnClosing(index); removeStackFromMaterialStore(removed); return removed; }*/ private void addStackToMaterialStore(ItemStack stack) { if (stack == null) { return; } if (MaterialSystem.isEnabled) { materialStore.addMaterial(stack.getItem(), stack.stackSize); } } private void removeStackFromMaterialStore(ItemStack stack) { if (stack == null) { return; } if (MaterialSystem.isEnabled) { materialStore.removeMaterial(stack.getItem(), stack.stackSize); } } /** * Used to retrieve variables. * @param compound with the give tag. */ public void readFromNBT(NBTTagCompound compound) { NBTTagList nbttaglist = compound.getTagList(TAG_ITEMS, Constants.NBT.TAG_COMPOUND); this.stacks = new ItemStack[this.getSizeInventory()]; for (int i = 0; i < nbttaglist.tagCount(); ++i) { NBTTagCompound nbttagcompound = nbttaglist.getCompoundTagAt(i); int j = nbttagcompound.getByte(TAG_SLOT) & Byte.MAX_VALUE; if (j != NO_SLOT && j < this.stacks.length) { this.stacks[j] = ItemStack.loadItemStackFromNBT(nbttagcompound); } } if (compound.hasKey(TAG_CUSTOM_NAME, Constants.NBT.TAG_STRING)) { this.customName = compound.getString(TAG_CUSTOM_NAME); } } /** * Used to store variables. * @param compound with the given tag. */ public void writeToNBT(NBTTagCompound compound) { NBTTagList nbttaglist = new NBTTagList(); for (int i = 0; i < this.stacks.length; ++i) { if (this.stacks[i] != null) { NBTTagCompound nbttagcompound = new NBTTagCompound(); nbttagcompound.setByte(TAG_SLOT, (byte) i); this.stacks[i].writeToNBT(nbttagcompound); nbttaglist.appendTag(nbttagcompound); } } compound.setTag(TAG_ITEMS, nbttaglist); if (this.hasCustomName()) { compound.setString(TAG_CUSTOM_NAME, this.customName); } if (MaterialSystem.isEnabled) { materialStore.writeToNBT(compound); } compound.setTag(TAG_INVENTORY, nbttaglist); } } TileEntity package com.minecolonies.tileentities; /** * The scarecrow tile entity to store extra data. */ public class ScarecrowTileEntity extends TileEntityChest { /** * NBTTag to store the type. */ private static final String TAG_TYPE = "type"; /** * Random generator. */ private final Random random = new Random(); /** * The inventory connected with the scarecrow. */ private InventoryField inventoryField; /** * The type of the scarecrow. */ private ScareCrowType type; /** * Creates an instance of the tileEntity. */ public ScarecrowTileEntity() { super(); this.inventoryField = new InventoryField(LanguageHandler.getString("com.minecolonies.gui.inventory.scarecrow"), true); } @Override public void onLoad() { super.onLoad(); World world = getWorld(); if(world != null) { Colony colony = ColonyManager.getColony(world, pos); if (colony != null && colony.getField(pos) == null) { Entity entity = EntityUtils.getEntityFromUUID(world, colony.getPermissions().getOwner()); if (entity instanceof EntityPlayer) { colony.addNewField(this, ((EntityPlayer) entity).inventory, pos, world); } } } } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); type = ScareCrowType.values()[compound.getInteger(TAG_TYPE)]; getInventoryField().readFromNBT(compound); } @Override public void writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger(TAG_TYPE, this.getType().ordinal()); getInventoryField().writeToNBT(compound); } /** * Returns the type of the scarecrow (Important for the rendering). * * @return the enum type. */ public ScareCrowType getType() { if (this.type == null) { this.type = ScareCrowType.values()[this.random.nextInt(2)]; } return this.type; } /** * Set the inventory connected with the scarecrow. * * @param inventoryField the field to set it to */ public final void setInventoryField(final InventoryField inventoryField) { this.inventoryField = inventoryField; } /** * Get the inventory connected with the scarecrow. * * @return the inventory field of this scarecrow */ public InventoryField getInventoryField() { return inventoryField; } /** * Enum describing the different textures the scarecrow has. */ public enum ScareCrowType { PUMPKINHEAD, NORMAL } }
-
I can drag stuff around in the players inventory perfectly well I also can drag stuff from the players inventory to the custom inventory but not the other way around.
-
Yeah I have a pretty big AI accessing my Container class that's why I need these getters and setters for. I also thought about the overriding of the transferStackInSlot() but dragging doesn't call this method(I debugged tested it) Only the slotClicked methods are called on dragging but I have no idea how I could use that to enable dragging normally the original onSlotClicked should handle the drag event. The transferStackInSlot() is only for shift click in and out. (That's why shift-clicking it works great)
-
I think the problem might be somewhere in the container class but I have no idea why, anyone has any suggestion?
-
In the Block. /** * The class handling the fieldBlocks, placement and activation. */ public class BlockHutField extends BlockContainer { /** * Hardness of the block. */ private static final float HARDNESS = 10F; /** * Resistance of the block. */ private static final float RESISTANCE = 10F; /** * The position it faces. */ public static final PropertyDirection FACING = PropertyDirection.create("FACING", Plane.HORIZONTAL); /** * Start of the collision box at y. */ private static final double BOTTOM_COLLISION = 0.0; /** * Start of the collision box at x and z. */ private static final double START_COLLISION = 0.1; /** * End of the collision box. */ private static final double END_COLLISION = 0.9; /** * Height of the collision box. */ private static final double HEIGHT_COLLISION = 2.5; /** * Registry name for this block. */ private static final String REGISTRY_NAME = "blockHutField"; /** * Constructor called on block placement. */ BlockHutField() { super(Material.wood); initBlock(); } /** * Method called by constructor. * Sets basic details of the block. */ private void initBlock() { setRegistryName(REGISTRY_NAME); setUnlocalizedName(Constants.MOD_ID.toLowerCase() + "." + "blockHutField"); setCreativeTab(ModCreativeTabs.MINECOLONIES); //Blast resistance for creepers etc. makes them explosion proof. setResistance(RESISTANCE); //Hardness of 10 takes a long time to mine to not loose progress. setHardness(HARDNESS); this.setDefaultState(this.blockState.getBaseState().withProperty(FACING, NORTH)); GameRegistry.registerBlock(this); setBlockBounds((float) START_COLLISION, (float) BOTTOM_COLLISION, (float) START_COLLISION, (float) END_COLLISION, (float) HEIGHT_COLLISION, (float) END_COLLISION); } @Override public int getRenderType() { return -1; } @Override public IBlockState getStateFromMeta(int meta) { EnumFacing facing; switch (getFront(meta)) { case WEST: facing = WEST; break; case EAST: facing = EAST; break; case NORTH: facing = NORTH; break; default: facing = SOUTH; } return this.getDefaultState().withProperty(FACING, facing); } @Override public int getMetaFromState(IBlockState state) { return state.getValue(FACING).getIndex(); } @Override public boolean isFullCube() { return false; } @Override public boolean isPassable(IBlockAccess worldIn, BlockPos pos) { return false; } @Override public boolean isOpaqueCube() { return false; } @Override @SideOnly(Side.CLIENT) public EnumWorldBlockLayer getBlockLayer() { return EnumWorldBlockLayer.SOLID; } @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumFacing side, float hitX, float hitY, float hitZ) { //If the world is server, open the inventory of the field. if (!worldIn.isRemote) { final Colony colony = ColonyManager.getColony(worldIn, pos); if (colony != null) { playerIn.openGui(MineColonies.instance, 0, worldIn, pos.getX(), pos.getY(), pos.getZ()); return true; } } return false; } // ======================================================================= // ======================= Rendering & IBlockState ======================= // ======================================================================= @Override public IBlockState onBlockPlaced(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { final EnumFacing enumFacing = (placer == null) ? NORTH : fromAngle(placer.rotationYaw); return this.getDefaultState().withProperty(FACING, enumFacing); } @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { //Only work on server side. if (worldIn.isRemote) { return; } if (placer instanceof EntityPlayer) { final Colony colony = ColonyManager.getColony(worldIn, pos); if (colony != null) { final InventoryField inventoryField = new InventoryField(LanguageHandler.getString("com.minecolonies.gui.inventory.scarecrow"), true); ((ScarecrowTileEntity) worldIn.getTileEntity(pos)).setInventoryField(inventoryField); colony.addNewField((ScarecrowTileEntity) worldIn.getTileEntity(pos), ((EntityPlayer) placer).inventory, pos, worldIn); } } } @Override protected BlockState createBlockState() { return new BlockState(this, FACING); } @Override public boolean hasTileEntity(final IBlockState state) { return true; } @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new ScarecrowTileEntity(); } // ======================================================================= // ===================== END of Rendering & Meta-Data ==================== // ======================================================================= }
-
TileEntitySpecialRenderer does not face the correct direction.
Raycoms replied to Raycoms's topic in Modder Support
I use GlStateManager.rotate(180, 0.3, 0.0 2.845); This fixed variables let my scarecrow face north being in the correct position. What do I have to change to have him show into any EnumFacing? -
Container class: /** * Handles the field class. */ public class Field extends Container { /** * The size of a normal inventory. */ private static final int MAX_INVENTORY_INDEX = 28; /** * The size of the the inventory hotbar. */ private static final int INVENTORY_BAR_SIZE = 8; /** * X-Offset of the inventory slot in the GUI of the scarecrow. */ private static final int X_OFFSET = 80; /** * Y-Offset of the inventory slot in the GUI of the scarecrow. */ private static final int Y_OFFSET = 34; /** * Tag to store the location. */ private static final String TAG_LOCATION = "location"; /** * Tag to store if the field has been taken. */ private static final String TAG_TAKEN = "taken"; /** * Tag to store the fields positive length. */ private static final String TAG_LENGTH_PLUS = "length+"; /** * Tag to store the fields positive width. */ private static final String TAG_WIDTH_PLUS = "width+"; /** * Tag to store the fields negative length. */ private static final String TAG_LENGTH_MINUS = "length-"; /** * Tag to store the fields negative width. */ private static final String TAG_WIDTH_MINUS = "width-"; /** * Tag to store the fields stage. */ private static final String TAG_STAGE = "stage"; /** * Amount of rows in the player inventory. */ private static final int PLAYER_INVENTORY_ROWS = 3; /** * Amount of columns in the player inventory. */ private static final int PLAYER_INVENTORY_COLUMNS = 9; /** * Initial x-offset of the inventory slot. */ private static final int PLAYER_INVENTORY_INITIAL_X_OFFSET = 8; /** * Initial y-offset of the inventory slot. */ private static final int PLAYER_INVENTORY_INITIAL_Y_OFFSET = 84; /** * Each offset of the inventory slots. */ private static final int PLAYER_INVENTORY_OFFSET_EACH = 18; /** * Initial y-offset of the inventory slots in the hotbar. */ private static final int PLAYER_INVENTORY_HOTBAR_OFFSET = 142; /** * The max width/length of a field. */ private static final int MAX_RANGE = 5; /** * The colony of the field. */ private final Colony colony; /** * The fields location. */ private BlockPos location; /** * Has the field be taken by any worker? */ private boolean taken = false; /** * Checks if the field needsWork (Hoeig, Seedings, Farming etc). */ private boolean needsWork = false; /** * Has the field been planted? */ private FieldStage fieldStage = FieldStage.EMPTY; /** * The length to plus x of the field. */ private int lengthPlusX; /** * The width to plus z of the seed. */ private int widthPlusZ; /** * The length to minus xof the field. */ private int lengthMinusX; /** * The width to minus z of the seed. */ private int widthMinusZ; /** * The inventorySlot of the field. */ private InventoryField inventory; /** * Name of the citizen claiming the field. */ private String owner = ""; /** * Private constructor to create field from NBT. * * @param colony the colony the field belongs to. */ private Field(Colony colony) { super(); this.colony = colony; } /** * Creates an instance of our field container, this may be serve to open the GUI. * * @param scarecrowTileEntity the tileEntity of the field containing the inventory. * @param playerInventory the player inventory. * @param world the world. * @param location the position of the field. */ public Field(ScarecrowTileEntity scarecrowTileEntity, InventoryPlayer playerInventory, World world, BlockPos location) { super(); this.colony = ColonyManager.getColony(world, location); this.location = location; this.inventory = scarecrowTileEntity.getInventoryField(); addSlotToContainer(new Slot(inventory, 0, X_OFFSET, Y_OFFSET)); //Ddd player inventory slots // Note: The slot numbers are within the player inventory and may be the same as the field inventory. int i; for (i = 0; i < PLAYER_INVENTORY_ROWS; i++) { for (int j = 0; j < PLAYER_INVENTORY_COLUMNS; j++) { addSlotToContainer(new Slot( playerInventory, j + i * PLAYER_INVENTORY_COLUMNS + PLAYER_INVENTORY_COLUMNS, PLAYER_INVENTORY_INITIAL_X_OFFSET + j * PLAYER_INVENTORY_OFFSET_EACH, PLAYER_INVENTORY_INITIAL_Y_OFFSET + i * PLAYER_INVENTORY_OFFSET_EACH )); } } for (i = 0; i < PLAYER_INVENTORY_COLUMNS; i++) { addSlotToContainer(new Slot( playerInventory, i, PLAYER_INVENTORY_INITIAL_X_OFFSET + i * PLAYER_INVENTORY_OFFSET_EACH, PLAYER_INVENTORY_HOTBAR_OFFSET )); } calculateSize(world, location.down()); } /** * Create and load a Field given it's saved NBTTagCompound. * * @param colony The owning colony. * @param compound The saved data. * @return {@link Field} created from the compound. */ public static Field createFromNBT(Colony colony, NBTTagCompound compound) { final Field field = new Field(colony); field.readFromNBT(compound); return field; } /** * Save data to NBT compound. * Writes the {@link #location} value. * * @param compound {@link net.minecraft.nbt.NBTTagCompound} to write data to. */ public void readFromNBT(NBTTagCompound compound) { location = BlockPosUtil.readFromNBT(compound, TAG_LOCATION); taken = compound.getBoolean(TAG_TAKEN); fieldStage = FieldStage.values()[compound.getInteger(TAG_STAGE)]; lengthPlusX = compound.getInteger(TAG_LENGTH_PLUS); widthPlusZ = compound.getInteger(TAG_WIDTH_PLUS); lengthMinusX = compound.getInteger(TAG_LENGTH_MINUS); widthMinusZ = compound.getInteger(TAG_WIDTH_MINUS); inventory = new InventoryField("Scarecrow", true); inventory.readFromNBT(compound); } /** * Getter for MAX_RANGE. * * @return the max range. */ private static int getMaxRange() { return MAX_RANGE; } @Override public ItemStack transferStackInSlot(EntityPlayer playerIn, int slotIndex) { if (slotIndex == 0) { playerIn.inventory.addItemStackToInventory(inventory.getStackInSlot(0)); inventory.setInventorySlotContents(0, null); } else if (inventory.getStackInSlot(0) == null) { final int playerIndex = slotIndex < MAX_INVENTORY_INDEX ? (slotIndex + INVENTORY_BAR_SIZE) : (slotIndex - MAX_INVENTORY_INDEX); if (playerIn.inventory.getStackInSlot(playerIndex) != null) { final ItemStack stack = playerIn.inventory.getStackInSlot(playerIndex).splitStack(1); inventory.setInventorySlotContents(0, stack); if (playerIn.inventory.getStackInSlot(playerIndex).stackSize == 0) { playerIn.inventory.removeStackFromSlot(playerIndex); } } } return null; } @Override public boolean canInteractWith(EntityPlayer playerIn) { return getColony().getPermissions().hasPermission(playerIn, Permissions.Action.ACCESS_HUTS); } /** * Returns the colony of the field. * * @return {@link com.minecolonies.colony.Colony} of the current object. */ public Colony getColony() { return this.colony; } /** * Calculates recursively the length of the field until a certain point. * * This mutates the field! * * @param position the start position. * @param world the world the field is in. */ public final void calculateSize(World world, BlockPos position) { //Calculate in all 4 directions this.lengthPlusX = searchNextBlock(0, position.east(), EnumFacing.EAST, world); this.lengthMinusX = searchNextBlock(0, position.west(), EnumFacing.WEST, world); this.widthPlusZ = searchNextBlock(0, position.south(), EnumFacing.SOUTH, world); this.widthMinusZ = searchNextBlock(0, position.north(), EnumFacing.NORTH, world); } /** * Calculates the field size into a specific direction. * * @param blocksChecked how many blocks have been checked. * @param position the start position. * @param direction the direction to search. * @param world the world object. * @return the distance. */ private int searchNextBlock(int blocksChecked, BlockPos position, EnumFacing direction, World world) { if (blocksChecked == getMaxRange() || isNoPartOfField(world, position)) { return blocksChecked; } return searchNextBlock(blocksChecked + 1, position.offset(direction), direction, world); } /** * Checks if a certain position is part of the field. Complies with the definition of field block. * * @param world the world object. * @param position the position. * @return true if it is. */ public boolean isNoPartOfField(World world, BlockPos position) { return world.isAirBlock(position) || world.getBlockState(position.up()).getBlock().getMaterial().isSolid(); } /** * Returns the {@link BlockPos} of the current object, also used as ID. * * @return {@link BlockPos} of the current object. */ public BlockPos getID() { // Location doubles as ID return this.location; } /** * Save data to NBT compound. * Writes the {@link #location} value. * * @param compound {@link net.minecraft.nbt.NBTTagCompound} to write data to. */ public void writeToNBT(NBTTagCompound compound) { BlockPosUtil.writeToNBT(compound, TAG_LOCATION, this.location); compound.setBoolean(TAG_TAKEN, taken); compound.setInteger(TAG_STAGE, fieldStage.ordinal()); compound.setInteger(TAG_LENGTH_PLUS, lengthPlusX); compound.setInteger(TAG_WIDTH_PLUS, widthPlusZ); compound.setInteger(TAG_LENGTH_MINUS, lengthMinusX); compound.setInteger(TAG_WIDTH_MINUS, widthMinusZ); inventory.writeToNBT(compound); } /** * Has the field been taken? * * @return true if the field is not free to use, false after releasing it. */ public boolean isTaken() { return this.taken; } /** * Sets the field taken. * * @param taken is field free or not */ public void setTaken(boolean taken) { this.taken = taken; } /** * Checks if the field has been planted. * * @return true if there are crops planted. */ public FieldStage getFieldStage() { return this.fieldStage; } /** * Sets if there are any crops planted. * * @param fieldStage true after planting, false after harvesting. */ public void setFieldStage(FieldStage fieldStage) { this.fieldStage = fieldStage; } /** * Checks if the field needs work (planting, hoeing). * * @return true if so. */ public boolean needsWork() { return this.needsWork; } /** * Sets that the field needs work. * * @param needsWork true if work needed, false after completing the job. */ public void setNeedsWork(boolean needsWork) { this.needsWork = needsWork; } /** * Getter of the seed of the field. * * @return the ItemSeed */ public Item getSeed() { if (inventory.getStackInSlot(0) != null && inventory.getStackInSlot(0).getItem() instanceof IPlantable) { return inventory.getStackInSlot(0).getItem(); } return null; } /** * Getter of the length in plus x direction. * * @return field length. */ public int getLengthPlusX() { return lengthPlusX; } /** * Getter of the with in plus z direction. * * @return field width. */ public int getWidthPlusZ() { return widthPlusZ; } /** * Getter of the length in minus x direction. * * @return field length. */ public int getLengthMinusX() { return lengthMinusX; } /** * Getter of the with in minus z direction. * * @return field width. */ public int getWidthMinusZ() { return widthMinusZ; } /** * Location getter. * * @return the location of the scarecrow of the field. */ public BlockPos getLocation() { return this.location; } /** * Return this citizens inventory. * * @return the inventory this citizen has. */ @NotNull public InventoryField getInventoryField() { return inventory; } /** * Sets the inventory of the field. * * @param inventory the inventory to set. */ public void setInventoryField(InventoryField inventory) { this.inventory = inventory; } /** * Sets the owner of the field. * @param owner the name of the citizen. */ public void setOwner(final String owner) { this.owner = owner; } /** * Getter of the owner of the field. * @return the string description of the citizen. */ public String getOwner() { return owner; } /** * Describes the stage the field is in. * Like if it has been hoed, planted or is empty. */ public enum FieldStage { EMPTY, HOED, PLANTED } } GuiField: /** * Class which creates the GUI of our field inventory. */ @SideOnly(Side.CLIENT) public class GuiField extends GuiContainer { /** * The resource location of the GUI background. */ private static final ResourceLocation TEXTURE = new ResourceLocation(Constants.MOD_ID, "textures/gui/scarecrow.png"); /** * Constructor of the GUI. * * @param parInventoryPlayer the player inventory. * @param tileEntity the tileEntity of the field, contains the inventory. * @param world the world the field is in. * @param location the location the field is at. */ protected GuiField(InventoryPlayer parInventoryPlayer, ScarecrowTileEntity tileEntity, World world, BlockPos location) { super(new Field(tileEntity, parInventoryPlayer, world, location)); } /** * Does draw the background of the GUI. * * @param partialTicks the ticks delivered. * @param mouseX the mouseX position. * @param mouseY the mouseY position. */ @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(TEXTURE); final int marginHorizontal = (width - xSize) / 2; final int marginVertical = (height - ySize) / 2; drawTexturedModalRect(marginHorizontal, marginVertical, 0, 0, xSize, ySize); } } Gui Handler: /** * Class which handles the GUI inventory. */ public class GuiHandler implements IGuiHandler { @Override public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { final BlockPos pos = new BlockPos(x,y,z); final ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new Field(tileEntity, player.inventory, world, pos); } @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { final BlockPos pos = new BlockPos(x,y,z); final ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new GuiField(player.inventory, tileEntity, world, pos); } }
-
Still unsolved.
-
TileEntitySpecialRenderer does not face the correct direction.
Raycoms replied to Raycoms's topic in Modder Support
I stored the rotation in the BlockState of my custom block and I am able to retrieve it in the tileEntitySpecialRenderer. But the State is an EnumFacing and I don't actually know how I should rotate it. -
TileEntitySpecialRenderer does not face the correct direction.
Raycoms replied to Raycoms's topic in Modder Support
Let's assume I didn't model that and that also I have no idea of modelling and the guy that models that kind of stuff is not available. Or just assume I plan on animating it later on. How can I let it face the correct direction for now? -
TileEntitySpecialRenderer does not face the correct direction.
Raycoms replied to Raycoms's topic in Modder Support
I unfortunately only have a .java model of the scarecrow how should I do it then? -
TileEntitySpecialRenderer does not face the correct direction.
Raycoms replied to Raycoms's topic in Modder Support
But he doesn't use a tileEntityRenderer, I already set the blockStates correct I now have to render it accordingly. -
I have a tileEntity which should face the way the player places it. currently I used some constants so it faces north at least. Without the constants if I fill in x and z as posX and posZ the block will follow the player. Without the rotation my block seems upside down. I set all the variables in the block so I am able to get the facing from the block state. But I don't know how to set the renderer up. @SideOnly(Side.CLIENT) public class TileEntityScarecrowRenderer extends TileEntitySpecialRenderer<ScarecrowTileEntity> { /** * The model of the scarecrow. */ private final ModelScarecrowBoth model; /** * Offset to the block middle. */ private static final double BLOCK_MIDDLE = 0.5; /** * Y-Offset in order to have the scarecrow over ground. */ private static final double YOFFSET = 1.5; /** * Which size the scarecrow should have ingame. */ private static final double SIZERATIO = .0625; /** * Rotate the model some degrees. */ private static final int ROTATION = 180; /** * Rotate it on the following x offset. */ private static final float XROTATIONOFFSET = 0.311F; /** * Rotate it on the following y offset. */ private static final float YROTATIONOFFSET = 0.0F; /** * Rotate it on the following z offset. */ private static final float ZROTATIONOFFSET = 2.845F; /** * The public constructor for the renderer. */ public TileEntityScarecrowRenderer() { super(); this.model = new ModelScarecrowBoth(); } @Override public void renderTileEntityAt(ScarecrowTileEntity te, double posX, double posY, double posZ, float partialTicks, int destroyStage) { //Store the transformation GlStateManager.pushMatrix(); //Set viewport to tile entity position to render it GlStateManager.translate(posX+BLOCK_MIDDLE, posY+YOFFSET, posZ+BLOCK_MIDDLE); this.bindTexture(getResourceLocation(te)); GlStateManager.rotate(ROTATION, XROTATIONOFFSET, YROTATIONOFFSET, ZROTATIONOFFSET); this.model.render((float) SIZERATIO); /* ============ Rendering Code stops here =========== */ //Restore the transformation, so other renderer's are not messed up. GlStateManager.popMatrix(); } /** * Returns the ResourceLocation of the scarecrow texture. * @param tileEntity the tileEntity of the scarecrow. * @return the location. */ private static ResourceLocation getResourceLocation(ScarecrowTileEntity tileEntity) { String loc; if(tileEntity.getType() == ScarecrowTileEntity.ScareCrowType.PUMPKINHEAD) { loc = "textures/blocks/blockScarecrowPumpkin.png"; } else { loc = "textures/blocks/blockScarecrowNormal.png"; } return new ResourceLocation(Constants.MOD_ID + ":" + loc); }
-
Anyone any idea? The GuiHandler calls the Field constructor and calls the GuiField constructor which again calls the Field constructor and therefore client and server side are called and instantiated correctly.
-
The onSlotClicked methods as well as the transferItemStack Method always get called by server and client, so I do not understand why there may be a problem.
-
Sorry, but I still do not understand what I should do with that tileEntity. public Field(ScarecrowTileEntity scarecrowTileEntity, InventoryPlayer playerInventory, World world, BlockPos location) { super(); this.colony = ColonyManager.getColony(world, location); this.location = location; this.inventory = scarecrowTileEntity.getInventoryField(); addSlotToContainer(new Slot(inventory, 0, X_OFFSET, Y_OFFSET)); //Ddd player inventory slots // Note: The slot numbers are within the player inventory and may be the same as the field inventory. int i; for (i = 0; i < PLAYER_INVENTORY_ROWS; i++) { for (int j = 0; j < PLAYER_INVENTORY_COLUMNS; j++) { addSlotToContainer(new Slot( playerInventory, j + i * PLAYER_INVENTORY_COLUMNS + PLAYER_INVENTORY_COLUMNS, PLAYER_INVENTORY_INITIAL_X_OFFSET + j * PLAYER_INVENTORY_OFFSET_EACH, PLAYER_INVENTORY_INITIAL_Y_OFFSET + i * PLAYER_INVENTORY_OFFSET_EACH )); } } for (i = 0; i < PLAYER_INVENTORY_COLUMNS; i++) { addSlotToContainer(new Slot( playerInventory, i, PLAYER_INVENTORY_INITIAL_X_OFFSET + i * PLAYER_INVENTORY_OFFSET_EACH, PLAYER_INVENTORY_HOTBAR_OFFSET )); } calculateSize(world, location.down()); }
-
How can I get the playerInventory from the tileEntity? This in the GUIHandler does not call both constructors of the field? Also I am able to transfer the items between the slots inside the inventory and back again, just not by drag and drop. @Override public Object getServerGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { final BlockPos pos = new BlockPos(x,y,z); final ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new Field(tileEntity.getInventoryField(), player.inventory, world, pos); } @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { final BlockPos pos = new BlockPos(x,y,z); final ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new GuiField(player.inventory, tileEntity.getInventoryField(), world, pos); }
-
The first constructor I only call in order to recreate the Field object vom NBT. If you have any idea how I could fix it, I'd try =P Our code is also on GitHub if you'd like to test it out. https://github.com/Minecolonies/minecolonies
-
Anyone has an idea, tried everything =/
-
I have a special TileEntity but when I try to get the world and position object in it's constructor they both are empty. Is it impossible to get the world and position in the constructor or is there some way? /** * The scarecrow tile entity to store extra data. */ public class ScarecrowTileEntity extends TileEntityChest { /** * NBTTag to store the type. */ private static final String TAG_TYPE = "type"; /** * Random generator. */ private final Random random = new Random(); /** * The inventory connected with the scarecrow. */ private InventoryField inventoryField; /** * The type of the scarecrow. */ private ScareCrowType type; /** * Creates an instance of the tileEntity. */ public ScarecrowTileEntity() { super(); World world = getWorld(); this.inventoryField = new InventoryField(LanguageHandler.getString("com.minecolonies.gui.inventory.scarecrow"), true); if(world != null) { Colony colony = ColonyManager.getColony(world, pos); if (colony != null && colony.getField(pos) == null) { Entity entity = EntityUtils.getEntityFromUUID(world, colony.getPermissions().getOwner()); if (entity instanceof EntityPlayer) { colony.addNewField(inventoryField, ((EntityPlayer) entity).inventory, pos, world); } } } } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); type = ScareCrowType.values()[compound.getInteger(TAG_TYPE)]; getInventoryField().readFromNBT(compound); } @Override public void writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger(TAG_TYPE, this.getType().ordinal()); getInventoryField().writeToNBT(compound); } /** * Returns the type of the scarecrow (Important for the rendering). * * @return the enum type. */ public ScareCrowType getType() { if (this.type == null) { this.type = ScareCrowType.values()[this.random.nextInt(1)]; } return this.type; } /** * Set the inventory connected with the scarecrow. * * @param inventoryField the field to set it to */ public final void setInventoryField(final InventoryField inventoryField) { this.inventoryField = inventoryField; } /** * Get the inventory connected with the scarecrow. * * @return the inventory field of this scarecrow */ public InventoryField getInventoryField() { return inventoryField; } /** * Enum describing the different textures the scarecrow has. */ public enum ScareCrowType { PUMPKINHEAD, NORMAL } } Creation of tileEntity: @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new ScarecrowTileEntity(); }
-
Exactly, when I try to move the object out of the custom inventory by clicking on it and then trying to move it with clicked mouse out of it, nothing happens.