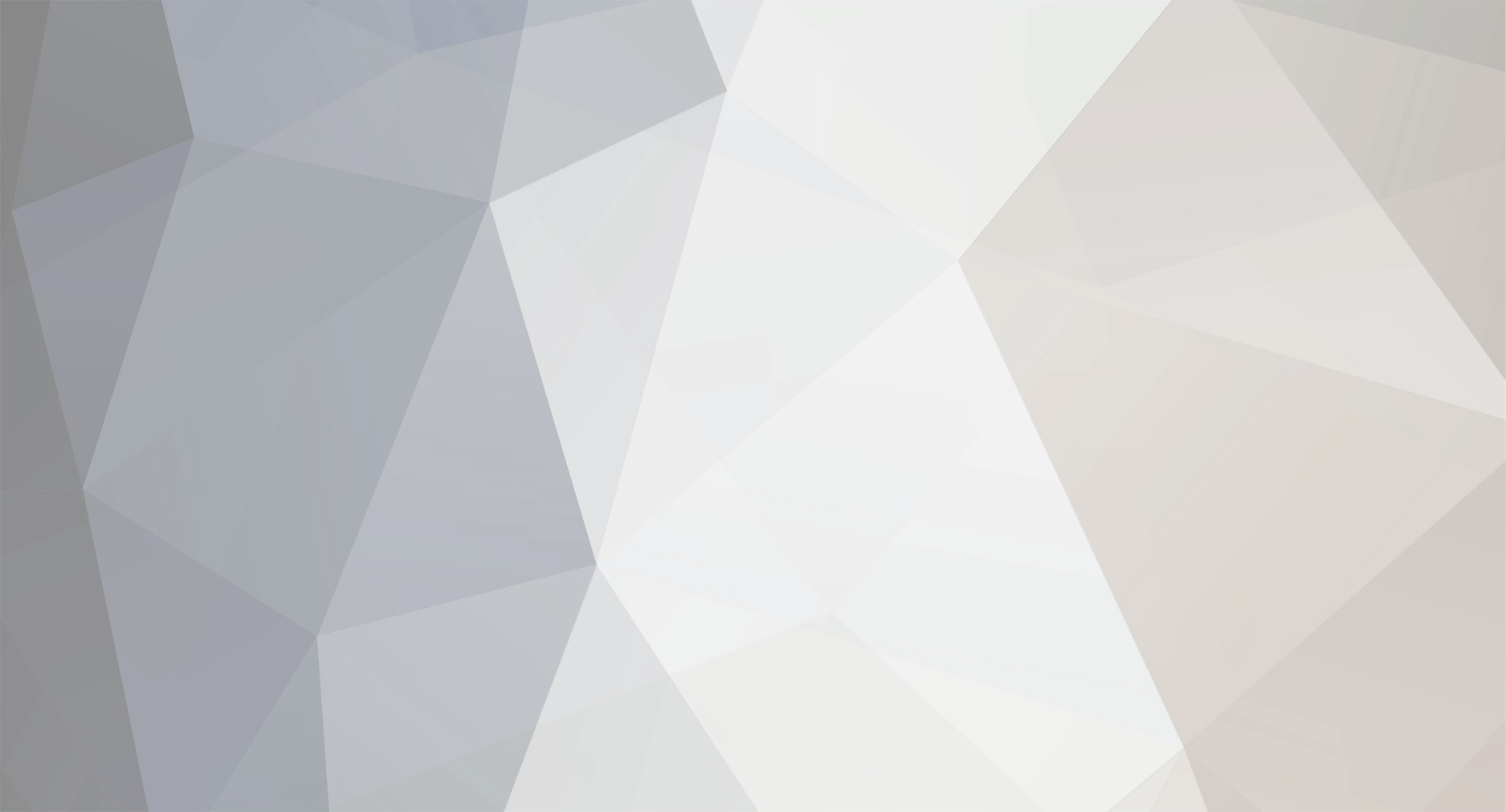
Raycoms
Members-
Posts
383 -
Joined
-
Last visited
Everything posted by Raycoms
-
Inventory change listener doesn't recognize stack increase.
Raycoms replied to Raycoms's topic in Modder Support
Our custom inventory class: https://bitbucket.org/cltnschlosser/minecolonies/src/a302f11cfe70c8e12f51dcbfde3ebc878cf77f62/src/main/java/com/minecolonies/inventory/InventoryCitizen.java?at=master&fileviewer=file-view-default -
Inventory change listener doesn't recognize stack increase.
Raycoms posted a topic in Modder Support
We have a custom Entity public class EntityCitizen extends EntityAgeable implements IInvBasic, INpc Its inventory works great. We may put one item in it or remove it. But we cannot increase it's stack size. The entity won't recognize that change and still assumes the initial stack size. @Override public void onInventoryChanged(InventoryBasic inventoryBasic) { setCurrentItemOrArmor(0, inventory.getHeldItem()); } Is called everytime something changes but the inventoryBasic object doesn't contain the changes in the case of a stack increase. How do I get my entity recognizing the changes correctly? Full source: https://bitbucket.org/cltnschlosser/minecolonies/src/a302f11cfe70c8e12f51dcbfde3ebc878cf77f62/src/main/java/com/minecolonies/entity/EntityCitizen.java?at=master&fileviewer=file-view-default -
We have a wide selection of language files for our mod. en_US en_UK en_CA but isn't there a way to reassign automatically? Like if someone chooses en_UK and we do not have the file he will choose en_US?
-
The solution: /** * Returns a fenceBlock if available * @param pos the position to be searched * @return fenceBlock or null */ private BlockFenceGate getBlockFence(BlockPos pos) { Block block = this.theEntity.worldObj.getBlockState(pos).getBlock(); if(!(block instanceof BlockFenceGate && block.getMaterial() == Material.wood)) { block = this.theEntity.worldObj.getBlockState(this.theEntity.getPosition()).getBlock(); gatePosition = this.theEntity.getPosition(); } return (block instanceof BlockFenceGate && block.getMaterial() == Material.wood ? (BlockFenceGate) block : null); }
-
And the winner is.... private BlockFenceGate getBlockFence(BlockPos pos) { Block block = this.theEntity.worldObj.getBlockState(pos.up()).getBlock(); return (block instanceof BlockFenceGate && block.getMaterial() == Material.wood ? (BlockFenceGate) block : null); } Edit it's not about the hovering. The problem is that the entity already stands inside the gate position and therefore tries to path one more to the front. But at this point there is no gate anymore so the above check returns null.
-
It seems that something wipes it but even that doesn't explain why it works perfectly for doors and not for the fences.
-
Thank you it is working a bit better now. But still. He only passes sometimes. PathNavigateGround pathnavigateground = (PathNavigateGround) this.theEntity.getNavigator(); PathEntity pathentity = pathnavigateground.getPath(); if (pathentity != null && !pathentity.isFinished() && pathnavigateground.getEnterDoors()) { Is stopping the execution mostly because pathentity is almost everytime null.
-
Update, strangely it seems that sometimes he is able to open and close the fence gates when they are on his head level. But he is not able to do it on a lower level.
-
Thanks, now the EntityAIGateInteraction is being called. But strangely he still will not open the fence gate. He stuck at shouldExecute because the pathEntity is null which is strange. I marked the fenceGate as a passable block and the pathfinder clearly is trying to path the NPC through it. It works perfectly for doors but doesn't for fenceGates... /** * Checks if the Interaction should be executed * @return true or false depending on the conditions */ public boolean shouldExecute() { if (!this.theEntity.isCollidedHorizontally) { return false; } else { PathNavigateGround pathnavigateground = (PathNavigateGround) this.theEntity.getNavigator(); PathEntity pathentity = pathnavigateground.getPath(); if (pathentity != null && !pathentity.isFinished() && pathnavigateground.getEnterDoors()) { for (int i = 0; i < Math.min(pathentity.getCurrentPathIndex() + 2, pathentity.getCurrentPathLength()); ++i) { PathPoint pathpoint = pathentity.getPathPointFromIndex(i); this.gatePosition = new BlockPos(pathpoint.xCoord, pathpoint.yCoord + 1, pathpoint.zCoord); if (this.theEntity.getDistanceSq((double) this.gatePosition.getX(), this.theEntity.posY, (double) this.gatePosition.getZ()) <= 2.25D) { this.gateBlock = this.getBlockFence(this.gatePosition); if (this.gateBlock != null) { return true; } } } this.gatePosition = (new BlockPos(this.theEntity)).up(); this.gateBlock = this.getBlockFence(this.gatePosition); return this.gateBlock != null; } else { return false; } } }
-
The AI classes I posted were never called, I put some breakpoints there never entered.
-
So I need an additional code to detect fence gates? (In the Pathfinder he detects the fence gate and paths through it but the NPC gets stuck on the gate). But I don't know where it should open it.
-
The classes: EntityAIGateInteract package com.minecolonies.entity.ai; import net.minecraft.block.Block; import net.minecraft.block.BlockFenceGate; import net.minecraft.block.material.Material; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.ai.EntityAIBase; import net.minecraft.pathfinding.PathEntity; import net.minecraft.pathfinding.PathNavigateGround; import net.minecraft.pathfinding.PathPoint; import net.minecraft.util.BlockPos; public class EntityAIGateInteract extends EntityAIBase { /** * Our citizen */ protected EntityLiving theEntity; /** * The gate position */ protected BlockPos gatePosition; /** * The gate block */ protected BlockFenceGate gateBlock; /** * Check if the interaction with the fenceGate stopped already. */ private boolean hasStoppedFenceInteraction; /** * The entities x and z position */ private float entityPositionX; private float entityPositionZ; /** * Constructor called to register the AI class with an entity * @param entityIn the registering entity */ public EntityAIGateInteract(EntityLiving entityIn) { this.gatePosition = BlockPos.ORIGIN; this.theEntity = entityIn; if (!(entityIn.getNavigator() instanceof PathNavigateGround)) { throw new IllegalArgumentException("Unsupported mob type for DoorInteractGoal"); } } /** * Checks if the Interaction should be executed * @return true or false depending on the conditions */ public boolean shouldExecute() { if (!this.theEntity.isCollidedHorizontally) { return false; } else { PathNavigateGround pathnavigateground = (PathNavigateGround) this.theEntity.getNavigator(); PathEntity pathentity = pathnavigateground.getPath(); if (pathentity != null && !pathentity.isFinished() && pathnavigateground.getEnterDoors()) { for (int i = 0; i < Math.min(pathentity.getCurrentPathIndex() + 2, pathentity.getCurrentPathLength()); ++i) { PathPoint pathpoint = pathentity.getPathPointFromIndex(i); this.gatePosition = new BlockPos(pathpoint.xCoord, pathpoint.yCoord + 1, pathpoint.zCoord); if (this.theEntity.getDistanceSq((double) this.gatePosition.getX(), this.theEntity.posY, (double) this.gatePosition.getZ()) <= 2.25D) { this.gateBlock = this.getBlockFence(this.gatePosition); if (this.gateBlock != null) { return true; } } } this.gatePosition = (new BlockPos(this.theEntity)).up(); this.gateBlock = this.getBlockFence(this.gatePosition); return this.gateBlock != null; } else { return false; } } } /** * Checks if the execution is still ongoing * @return true or false */ public boolean continueExecuting() { return !this.hasStoppedFenceInteraction; } /** * Starts the execution */ public void startExecuting() { this.hasStoppedFenceInteraction = false; this.entityPositionX = (float) ((double) ((float) this.gatePosition.getX() + 0.5F) - this.theEntity.posX); this.entityPositionZ = (float) ((double) ((float) this.gatePosition.getZ() + 0.5F) - this.theEntity.posZ); } /** * Updates the task */ public void updateTask() { float f = (float) ((double) ((float) this.gatePosition.getX() + 0.5F) - this.theEntity.posX); float f1 = (float) ((double) ((float) this.gatePosition.getZ() + 0.5F) - this.theEntity.posZ); float f2 = this.entityPositionX * f + this.entityPositionZ * f1; if (f2 < 0.0F) { this.hasStoppedFenceInteraction = true; } } /** * Returns a fenceBlock if available * @param pos the position to be searched * @return fenceBlock or null */ private BlockFenceGate getBlockFence(BlockPos pos) { Block block = this.theEntity.worldObj.getBlockState(pos).getBlock(); return block instanceof BlockFenceGate && block.getMaterial() == Material.wood ? (BlockFenceGate) block : null; } } EntityAIOpenFenceGate package com.minecolonies.entity.ai; import net.minecraft.block.BlockFenceGate; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.player.EntityPlayer; public class EntityAIOpenFenceGate extends EntityAIGateInteract { /** * Checks if the gate should be closed */ private boolean closeDoor; /** * Ticks until the gate should be closed */ private int closeDoorTemporisation; /** * Constructor called to register the AI class with an entity * @param entityLivingIn the registering entity * @param shouldClose should the entity close the gate? */ public EntityAIOpenFenceGate(EntityLiving entityLivingIn, boolean shouldClose) { super(entityLivingIn); this.theEntity = entityLivingIn; this.closeDoor = shouldClose; } /** * Should the AI continue to execute? * @return true or false */ public boolean continueExecuting() { return this.closeDoor && this.closeDoorTemporisation > 0 && super.continueExecuting(); } /** * Start the execution */ public void startExecuting() { this.closeDoorTemporisation = 20; toggleDoor(true); } /** * Reset the action */ public void resetTask() { if (this.closeDoor) { toggleDoor(false); } } /** * Toggles the door(Opens or closes) * @param open if open or close */ private void toggleDoor(boolean open) { IBlockState iblockstate = this.theEntity.worldObj.getBlockState(this.gatePosition); if (iblockstate.getBlock() == this.gateBlock) { if ((iblockstate.getValue(BlockFenceGate.OPEN)) != open) { this.theEntity.worldObj.setBlockState(this.gatePosition, iblockstate.withProperty(BlockFenceGate.OPEN, open), 2); this.theEntity.worldObj.playAuxSFXAtEntity((EntityPlayer) null, open ? 1003 : 1006, this.gatePosition, 0); } } } /** * Updates the task. */ public void updateTask() { --this.closeDoorTemporisation; super.updateTask(); } } and how I add them: private void initTasks() { this.tasks.addTask(0, new EntityAISwimming(this)); this.tasks.addTask(1, new EntityAICitizenAvoidEntity(this, EntityMob.class, 8.0F, 0.6D, 1.6D)); this.tasks.addTask(2, new EntityAIGoHome(this)); this.tasks.addTask(3, new EntityAISleep(this)); this.tasks.addTask(4, new EntityAIOpenDoor(this, true)); this.tasks.addTask(4, new EntityAIOpenFenceGate(this, true)); this.tasks.addTask(5, new EntityAIWatchClosest2(this, EntityPlayer.class, 3.0F, 1.0F)); this.tasks.addTask(6, new EntityAIWatchClosest2(this, EntityCitizen.class, 5.0F, 0.02F)); this.tasks.addTask(7, new EntityAICitizenWander(this, 0.6D)); this.tasks.addTask(8, new EntityAIWatchClosest(this, EntityLiving.class, 6.0F)); onJobChanged(getColonyJob()); }
-
I created both these AIClasses and changed them for fence gates but they are never being called.
-
@diesieben07 That means there is no easy way (Like with doors) to get my npcs to open them. I will have to detect a fenceGate at my next node (Of the pathfinder) and then manually open and close it?
-
Sorry I don't get it. I know where canOpenDoors is set but I want "canOpenFenceGates" to work...
-
Is there a way to get custom npcs automatically go through fence gates like doors? It is easy for doors where I only have to set canOpenDoors to true but there seems no easy way for fence gates. I copied the openDoorAI classes from minecraft and simulated them for fence gates, my pathfinder also pathes my entities through the gates, but they still won't open the fence gates? Is there some special trick?
-
Is there a way to detect if a block exists in the current version? I have a block in code only which has been saved. I now want to see if this block still does exist. Examples: We had in 1.7.10 the block shrub which now in 1.8.9 turned "dead_bush". Or maybe the slimeblock from 1.8.9 which I shouldn't place in 1.7.10. I want to detect if these blocks do exist in the current minecraft version with the current mods.
-
Previously we were using these SRG names "func_29484b". But since we've updated to the 1.8.9-Mappings we had to change it to these "obfuscated notch names".
-
Using the deobfuscates names lead to a error in the dev environment that's why I changed it. (One of our devs installed the 1.8.9-Mappings)
-
We have the following code. This code works great in our dev environment (Using Intellij) But the moment we leave the dev environment this doesn't work anymore. try (DataOutputStream dataOutputStream = new DataOutputStream(new GZIPOutputStream(new FileOutputStream(file)))) { Method method = ReflectionHelper.findMethod(NBTTagCompound.class, null, new String[] { "writeEntry", "a" }, String.class, NBTBase.class, DataOutput.class); method.invoke(null, "Schematic", tagCompound, dataOutputStream); } Executing it on technic leads to: [b#347] net.minecraftforge.fml.relauncher.ReflectionHelper$UnableToFindMethodException: java.lang.NoSuchMethodException: net.minecraft.nbt.NBTTagCompound.a(java.lang.String, net.minecraft.nbt.NBTBase, java.io.DataOutput) [b#347] at net.minecraftforge.fml.relauncher.ReflectionHelper.findMethod(ReflectionHelper.java:183) ~[modpack.jar:?] [b#347] at com.schematica.world.schematic.SchematicFormat.writeToFile(SchematicFormat.java:92) [schematicFormat.class:?] [b#347] at com.minecolonies.util.Schematic.saveSchematic(Schematic.java:264) [schematic.class:?] [b#347] at com.minecolonies.items.ItemScanTool.func_180614_a(ItemScanTool.java:58) [itemScanTool.class:?] [b#347] at net.minecraft.item.ItemStack.func_179546_a(ItemStack.java:135) [zx.class:?] [b#347] at net.minecraft.client.multiplayer.PlayerControllerMP.func_178890_a(PlayerControllerMP.java:403) [bda.class:?] [b#347] at net.minecraft.client.Minecraft.func_147121_ag(Minecraft.java:1491) [ave.class:?] [b#347] at net.minecraft.client.Minecraft.func_71407_l(Minecraft.java:2036) [ave.class:?] [b#347] at net.minecraft.client.Minecraft.func_71411_J(Minecraft.java:1024) [ave.class:?] [b#347] at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:349) [ave.class:?] [b#347] at net.minecraft.client.main.Main.main(SourceFile:124) [Main.class:?] [b#347] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] [b#347] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_91] [b#347] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_91] [b#347] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_91] [b#347] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] [b#347] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] [b#347] Caused by: java.lang.NoSuchMethodException: net.minecraft.nbt.NBTTagCompound.a(java.lang.String, net.minecraft.nbt.NBTBase, java.io.DataOutput) [b#347] at java.lang.Class.getDeclaredMethod(Unknown Source) ~[?:1.8.0_91] [b#347] at net.minecraftforge.fml.relauncher.ReflectionHelper.findMethod(ReflectionHelper.java:174) ~[modpack.jar:?] [b#347] ... 16 more
-
[1.8.9] Correctly create an itemstack from a block.
Raycoms replied to Raycoms's topic in Modder Support
Thank you. What is "MovingObjectPosition" for. What do I enter in this field? -
[1.8.9] Correctly create an itemstack from a block.
Raycoms replied to Raycoms's topic in Modder Support
and If I don't have the worldBlock? If I only have the IBlockState? -
Before the 1.8.9 update we simply created new ItemStacks by: new ItemStack(world.getBlock(x,y,z) now when I try new ItemStack(world.getBlockState(x,y,z).getBlock() the ItemStacks do not contain the necessary metadata (all slabs are always stone slabs etc) and ItemStack stack = new ItemStack(metadata.getBlock(), 1); stack.setItemDamage(block.getMetaFromState(metadata)); leads to crashes for BlockDoor and probably other blocks as well.
-
[1.8] [1.7] Rendering glitches in some areas [Unsolved]
Raycoms replied to Raycoms's topic in Modder Support
Exactly these schematics we're rendering. (With his permission of course) -
[1.8] [1.7] Rendering glitches in some areas [Unsolved]
Raycoms replied to Raycoms's topic in Modder Support
I am still running into this problem, today it happened in an island biome.