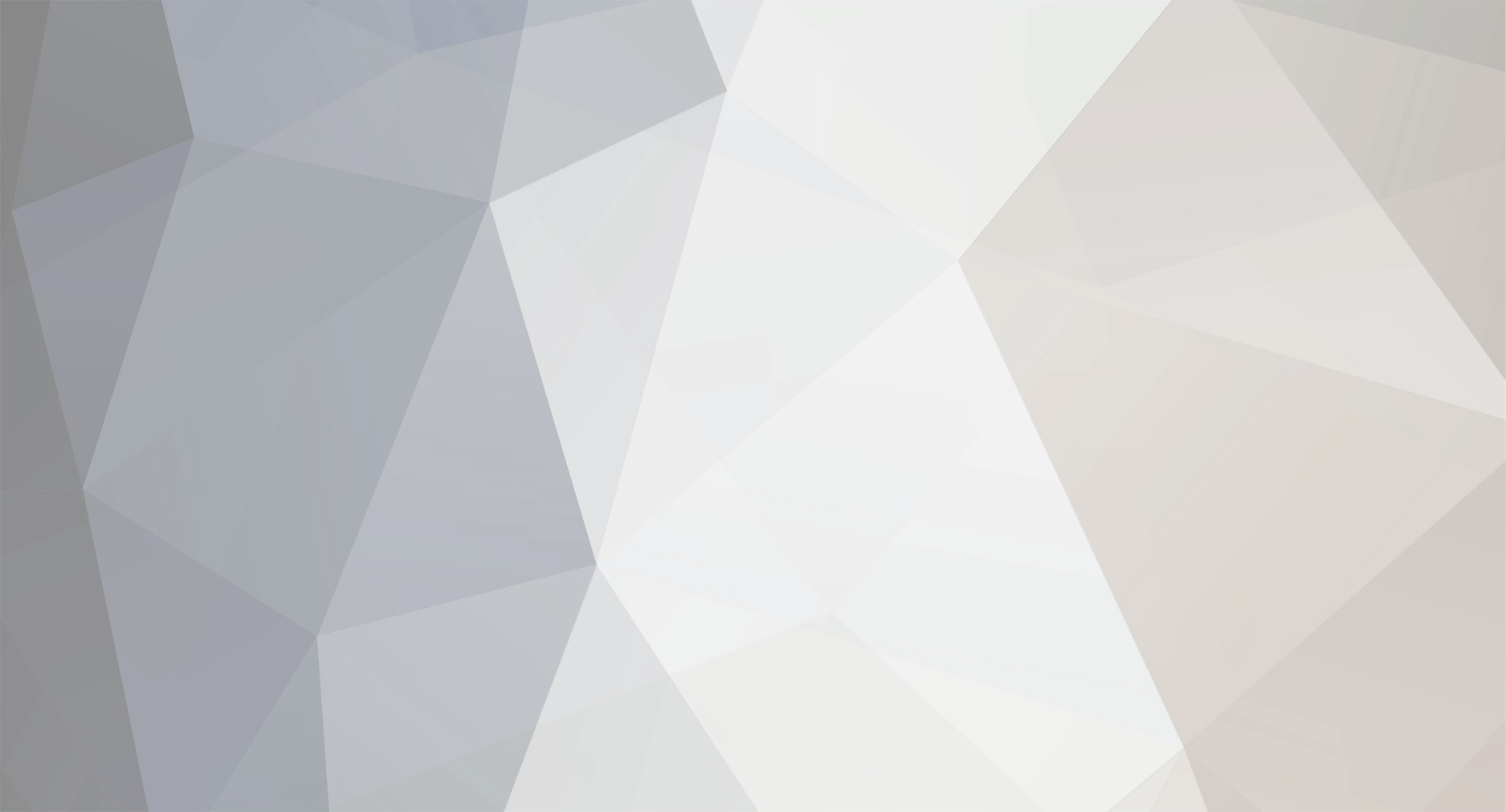
Raycoms
Members-
Posts
383 -
Joined
-
Last visited
Everything posted by Raycoms
-
This is still happening
-
Edited it a bit and it renders now correctly, but still. All the entities get stuck in it. @Override public AxisAlignedBB getCollisionBoundingBox(World worldIn, BlockPos pos, IBlockState state) { this.setBlockBoundsBasedOnState(worldIn, pos); this.maxY = 2D; return new AxisAlignedBB(pos.getX() - START_COLLISION, pos.getY() - BOTTOM_COLLISION, pos.getZ() - START_COLLISION, pos.getX() + END_COLLISION, pos.getY() + HEIGHT_COLLISION,pos.getZ() + END_COLLISION); }
-
When I do this he stops rendering the scarecrow and I am able to pass through it. (I still see the bounding box thoe) private static final double BOTTOM_COLLISION = 0.0; /** * Start of the collision box at x and z. */ private static final double START_COLLISION = 0.1; /** * End of the collision box. */ private static final double END_COLLISION = 0.9; /** * Height of the collision box. */ private static final double HEIGHT_COLLISION = 2.5; private static AxisAlignedBB boundingBox = new AxisAlignedBB(START_COLLISION, BOTTOM_COLLISION, START_COLLISION, END_COLLISION, HEIGHT_COLLISION, END_COLLISION);
-
Just did: Still not working. @Override public boolean isPassable(IBlockAccess worldIn, BlockPos pos) { return false; }
-
I added all this but entities still try to path over it. (Minecraft entities as well as custom entities) @Override public void setBlockBoundsBasedOnState(IBlockAccess worldIn, BlockPos pos) { setBlockBounds((float)START_COLLISION, (float)BOTTOM_COLLISION, (float)START_COLLISION, (float)END_COLLISION, (float)HEIGHT_COLLISION, (float)END_COLLISION); } @Override public AxisAlignedBB getCollisionBoundingBox(World worldIn, BlockPos pos, IBlockState state) { this.setBlockBoundsBasedOnState(worldIn, pos); this.maxY = 2D; return super.getCollisionBoundingBox(worldIn, pos, state); } @Override public boolean isFullCube() { return false; } and that is basically what walls etc do as well.
-
Our mod is pretty big and will need some time to update.
-
Even if this is 1.8.9 code and I have a custom GUI and an inventory in the block? (getTileEntity doesn't work). Also @Animefan8888 the cactus didn't help me a lot since it has various blocks.
-
I have a custom scarecrow which has a collission box which is two blocks high. But most entities think my block is just one high and get stuck in it. Is there a fix for this? package com.minecolonies.blocks; import com.minecolonies.MineColonies; import com.minecolonies.colony.Colony; import com.minecolonies.colony.ColonyManager; import com.minecolonies.creativetab.ModCreativeTabs; import com.minecolonies.inventory.InventoryField; import com.minecolonies.lib.Constants; import com.minecolonies.tileentities.ScarecrowTileEntity; import com.minecolonies.util.LanguageHandler; import net.minecraft.block.BlockContainer; import net.minecraft.block.ITileEntityProvider; import net.minecraft.block.material.Material; import net.minecraft.block.properties.PropertyDirection; import net.minecraft.block.state.BlockState; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.*; import net.minecraft.world.World; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; /** * The class handling the fieldBlocks, placement and activation. */ public class BlockHutField extends BlockContainer implements ITileEntityProvider { /** * Hardness of the block. */ private static final float HARDNESS = 10F; /** * Resistance of the block. */ private static final float RESISTANCE = 10F; /** * The position it faces. */ private static final PropertyDirection FACING = PropertyDirection.create("FACING", EnumFacing.Plane.HORIZONTAL); /** * Start of the collision box at y. */ private static final double BOTTOM_COLLISION = 0.0; /** * Start of the collision box at x and z. */ private static final double START_COLLISION = 0.1; /** * End of the collision box. */ private static final double END_COLLISION = 0.9; /** * Height of the collision box. */ private static final double HEIGHT_COLLISION = 2.5; /** * Constructor called on block placement. */ BlockHutField() { super(Material.wood); initBlock(); } /** * Getter of the description of the blockHut. * @return the name as a String. */ public String getName() { return "blockHutField"; } /** * Method called by constructor. * Sets basic details of the block. */ private void initBlock() { setRegistryName(getName()); setUnlocalizedName(Constants.MOD_ID.toLowerCase() + "." + getName()); setCreativeTab(ModCreativeTabs.MINECOLONIES); //Blast resistance for creepers etc. makes them explosion proof. setResistance(RESISTANCE); //Hardness of 10 takes a long time to mine to not loose progress. setHardness(HARDNESS); this.setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.NORTH)); GameRegistry.registerBlock(this); setBlockBounds((float)START_COLLISION, (float)BOTTOM_COLLISION, (float)START_COLLISION, (float)END_COLLISION, (float)HEIGHT_COLLISION, (float)END_COLLISION); } @Override public void onBlockPlacedBy(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase placer, ItemStack stack) { //Only work on server side. if(worldIn.isRemote) { return; } if(placer instanceof EntityPlayer) { Colony colony = ColonyManager.getColony(worldIn, pos); if (colony != null) { InventoryField inventoryField = new InventoryField(LanguageHandler.getString("com.minecolonies.gui.inventory.scarecrow"), true); ((ScarecrowTileEntity)worldIn.getTileEntity(pos)).setInventoryField(inventoryField); colony.addNewField(inventoryField, ((EntityPlayer) placer).inventory, pos, worldIn); } } } @Override public boolean isOpaqueCube() { return false; } @Override public int getRenderType() { return -1; } @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumFacing side, float hitX, float hitY, float hitZ) { //If the world is server, open the inventory of the field. if(!worldIn.isRemote) { Colony colony = ColonyManager.getColony(worldIn, pos); if(colony != null) { playerIn.openGui(MineColonies.instance, 0, worldIn, pos.getX(), pos.getY(), pos.getZ()); return true; } } return false; } @Override public void onBlockDestroyedByPlayer(final World worldIn, final BlockPos pos, final IBlockState state) { super.onBlockDestroyedByPlayer(worldIn, pos, state); //Only work on server side. if(worldIn.isRemote) { return; } Colony colony = ColonyManager.getColony(worldIn, pos); if (colony != null) { colony.removeField(pos); } } // ======================================================================= // ======================= Rendering & IBlockState ======================= // ======================================================================= @Override public IBlockState onBlockPlaced(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { EnumFacing enumFacing = (placer == null) ? EnumFacing.NORTH : EnumFacing.fromAngle(placer.rotationYaw); return this.getDefaultState().withProperty(FACING, enumFacing); } @Override @SideOnly(Side.CLIENT) public EnumWorldBlockLayer getBlockLayer() { return EnumWorldBlockLayer.SOLID; } @Override public IBlockState getStateFromMeta(int meta) { EnumFacing facing = EnumFacing.getFront(meta); if(facing.getAxis() == EnumFacing.Axis.Y) { facing = EnumFacing.NORTH; } return this.getDefaultState().withProperty(FACING, facing); } @Override public int getMetaFromState(IBlockState state) { return state.getValue(FACING).getIndex(); } @Override protected BlockState createBlockState() { return new BlockState(this, FACING); } @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new ScarecrowTileEntity(); } // ======================================================================= // ===================== END of Rendering & Meta-Data ==================== // ======================================================================= }
-
@SideOnly(Side.CLIENT) public class GuiField extends GuiContainer { /** * The resource location of the GUI background. */ private static final ResourceLocation TEXTURE = new ResourceLocation(Constants.MOD_ID, "textures/gui/scarecrow.png"); /** * Constructor of the GUI. * @param parInventoryPlayer the player inventory. * @param fieldInventory the field inventory. */ protected GuiField(InventoryPlayer parInventoryPlayer, IInventory fieldInventory, World world, BlockPos location) { super(new Field((InventoryField) fieldInventory, parInventoryPlayer, world, location)); } /** * Does draw the background of the GUI. * @param partialTicks the ticks delivered. * @param mouseX the mouseX position. * @param mouseY the mouseY position. */ @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); mc.getTextureManager().bindTexture(TEXTURE); int marginHorizontal = (width - xSize) / 2; int marginVertical = (height - ySize) / 2; drawTexturedModalRect(marginHorizontal, marginVertical, 0, 0, xSize, ySize); } }
-
Someone an idea?
-
Is there a way to detect if another day has started? A new day?
-
Already had removed the method completely out of the code, I only used it for debugging, since it only calls it's super method. But with or without final, with or without that method it doesn't work.
-
[16:23:15] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [16:26:01] [server thread/INFO]: Starting integrated minecraft server version 1.8.9 [16:26:01] [server thread/INFO]: Generating keypair [16:26:01] [server thread/INFO] [FML]: Injecting existing block and item data into this server instance [16:26:01] [server thread/INFO] [FML]: Applying holder lookups [16:26:01] [server thread/INFO] [FML]: Holder lookups applied [16:26:02] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [16:26:02] [server thread/INFO] [FML]: Loading dimension 0 (New World) (net.minecraft.server.integrated.IntegratedServer@24062a4b) [16:26:02] [server thread/INFO] [FML]: Loading dimension 1 (New World) (net.minecraft.server.integrated.IntegratedServer@24062a4b) [16:26:02] [server thread/INFO] [minecolonies]: Loaded 1 colonies [16:26:02] [server thread/INFO] [FML]: Loading dimension -1 (New World) (net.minecraft.server.integrated.IntegratedServer@24062a4b) [16:26:02] [server thread/INFO]: Preparing start region for level 0 [16:26:03] [server thread/INFO]: Preparing spawn area: 47% [16:26:04] [server thread/INFO] [minecolonies]: Starting AI job com.minecolonies.job.Lumberjack [16:26:04] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [16:26:04] [server thread/INFO] [minecolonies]: Starting AI job com.minecolonies.job.Builder [16:26:04] [server thread/INFO]: Changing view distance to 6, from 10 [16:26:06] [Netty Local Client IO #0/INFO] [FML]: Server protocol version 2 [16:26:06] [Netty Server IO #1/INFO] [FML]: Client protocol version 2 [16:26:06] [Netty Server IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],minecolonies@@VERSION@,[email protected] [16:26:06] [Netty Local Client IO #0/INFO] [FML]: [Netty Local Client IO #0] Client side modded connection established [16:26:06] [server thread/INFO] [FML]: [server thread] Server side modded connection established [16:26:06] [server thread/INFO]: Raycoms[local:E:fb690bcb] logged in with entity id 557 at (-180.58073028560275, 64.88756546798191, 268.0009106214668) [16:26:06] [server thread/INFO]: Raycoms joined the game [16:26:08] [pool-2-thread-1/WARN]: Couldn't look up profile properties for com.mojang.authlib.GameProfile@3ec8890[id=7a8d2f7a-e6ca-3651-b109-06fdcd7aa8cb,name=Raycoms,properties={},legacy=false] com.mojang.authlib.exceptions.AuthenticationException: The client has sent too many requests within a certain amount of time at com.mojang.authlib.yggdrasil.YggdrasilAuthenticationService.makeRequest(YggdrasilAuthenticationService.java:65) ~[YggdrasilAuthenticationService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillGameProfile(YggdrasilMinecraftSessionService.java:175) [YggdrasilMinecraftSessionService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:59) [YggdrasilMinecraftSessionService$1.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:56) [YggdrasilMinecraftSessionService$1.class:?] at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3524) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2317) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2280) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2195) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.get(LocalCache.java:3934) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:3938) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:4821) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:4827) [guava-17.0.jar:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillProfileProperties(YggdrasilMinecraftSessionService.java:165) [YggdrasilMinecraftSessionService.class:?] at net.minecraft.client.Minecraft.getProfileProperties(Minecraft.java:2951) [Minecraft.class:?] at net.minecraft.client.resources.SkinManager$3.run(SkinManager.java:130) [skinManager$3.class:?] at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:511) [?:1.8.0_102] at java.util.concurrent.FutureTask.run(FutureTask.java:266) [?:1.8.0_102] at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142) [?:1.8.0_102] at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617) [?:1.8.0_102] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_102] [16:26:33] [server thread/INFO] [minecolonies]: Colony 1 - new AbstractBuilding for class com.minecolonies.blocks.BlockHutBuilder at BlockPos{x=-193, y=67, z=254} [16:26:34] [server thread/INFO] [minecolonies]: Starting AI job com.minecolonies.job.Builder [16:26:34] [server thread/INFO] [minecolonies]: Colony 1 - removed AbstractBuilding BlockPos{x=-193, y=67, z=254} of type Builder [16:26:35] [Client thread/INFO]: [CHAT] Lumberjack James M. Robinson: I need axe! [16:26:35] [server thread/INFO] [minecolonies]: Colony 1 - new AbstractBuilding for class com.minecolonies.blocks.BlockHutBuilder at BlockPos{x=-194, y=66, z=253} [16:26:35] [server thread/INFO] [minecolonies]: Starting AI job com.minecolonies.job.Builder [16:26:37] [server thread/INFO] [minecolonies]: Colony 1 - removed AbstractBuilding BlockPos{x=-194, y=66, z=253} of type Builder [16:26:41] [server thread/INFO] [minecolonies]: Colony 1 - new AbstractBuilding for class com.minecolonies.blocks.BlockHutBuilder at BlockPos{x=-194, y=66, z=251} [16:26:41] [server thread/INFO] [minecolonies]: Starting AI job com.minecolonies.job.Builder [16:26:41] [server thread/INFO] [minecolonies]: Colony 1 - removed AbstractBuilding BlockPos{x=-194, y=66, z=251} of type Builder [16:27:22] [Client thread/INFO]: [CHAT] Finished building classic/Fisherman1 [16:27:22] [server thread/INFO] [minecolonies]: Starting AI job com.minecolonies.job.Fisherman [16:27:35] [Client thread/INFO]: [CHAT] Lumberjack James M. Robinson: I need axe! [16:27:40] [Client thread/INFO]: [CHAT] Fisherman Dorothy S. Johnson: I need Fishing Rod! [16:28:10] [Client thread/INFO]: [CHAT] Fisherman Dorothy S. Johnson: I need Fishing Rod! [16:29:11] [Client thread/INFO]: [CHAT] Fisherman Dorothy S. Johnson: I need Fishing Rod! Nothing interesting in the log.
-
The InventoryField extends InventoryCitizen which extends IInventory. I do not get any feedback at all when I try to drag out an object not even a log or exception. The GuiHandler. @SideOnly(Side.CLIENT) public class GuiHandler implements IGuiHandler { @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { BlockPos pos = new BlockPos(x,y,z); ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new Field(tileEntity.inventoryField, player.inventory, world, pos); } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { BlockPos pos = new BlockPos(x,y,z); ScarecrowTileEntity tileEntity = (ScarecrowTileEntity) world.getTileEntity(pos); return new GuiField(player.inventory, tileEntity.inventoryField, world, pos); } }
-
public ItemStack transferStackInSlot(EntityPlayer playerIn, int slotIndex) isn't called when I try to drag it out of the custom inventory, it gets called the other way around. public ItemStack slotClick(final int slotId, final int clickedButton, final int mode, final EntityPlayer playerIn) always gets called but I do not overwrite it.
-
[1.8.9] Correctly create an itemstack from a block.
Raycoms replied to Raycoms's topic in Modder Support
When I connect drops with silk touch it should work? Which method does it use (serverside) -
[1.8.9] Correctly create an itemstack from a block.
Raycoms replied to Raycoms's topic in Modder Support
So there is no way to get the item on the server since getPickBlock, like I said earlier, calls a client only method. -
[1.8.9] Correctly create an itemstack from a block.
Raycoms replied to Raycoms's topic in Modder Support
This also guarantees plantpots and doors? -
[1.8.9] Correctly create an itemstack from a block.
Raycoms replied to Raycoms's topic in Modder Support
I was able to use the "getItem(block) method inside the block class, the same method Block#getPickBlock uses. But when I tested my mod in smp it crashed since the getItem method is Clientside only. So how may I get the item correctly on the server (getPickBlock also will need that getItem method) ? -
I created my custom IInventory, Container, GUIContainer and GUIHandler. Without any problems I can drag an item inside the custom inventory slot and without any problems I can remove it double clicking it. (I overwrote transferStackInSlot) But somehow it isn't possible to remove the item in the slot dragging it. package com.minecolonies.colony; import com.minecolonies.inventory.InventoryField; import com.minecolonies.util.BlockPosUtil; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.InventoryPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.Slot; import net.minecraft.item.ItemSeeds; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.util.BlockPos; import org.jetbrains.annotations.NotNull; public class Field extends Container { /** * Tag to store the location. */ private static final String TAG_LOCATION = "location"; /** * Tag to store if the field has been taken. */ private static final String TAG_TAKEN = "taken"; /** * Tag to store the fields length. */ private static final String TAG_LENGTH = "length"; /** * Tag to store the fields width. */ private static final String TAG_WIDTH = "width"; /** * The max width/length of a field. */ private static final int MAX_RANGE = 10; /** * The fields location. */ private BlockPos location; /** * The colony of the field. */ private final Colony colony; /** * Has the field be taken by any worker? */ private boolean taken = false; /** * Checks if the field needsWork (Hoeig, Seedings, Farming etc) */ private boolean needsWork = false; /** * Has the field been planted? */ private boolean planted = false; /** * The set seed type for the field. */ private ItemSeeds seed; /** * The length of the field. */ private int length; /** * The width of the seed; */ private int width; /** * The inventorySlot of the field. */ private InventoryField inventory; /** * Private constructor to create field from NBT. * @param colony the colony the field belongs to. */ private Field(Colony colony) { this.colony = colony; } /** * Creates a new field object. * @param colony The colony the field is a part of. * @param location The location the field has been placed. * @param width The fields width. * @param length The fields length. */ public Field(Colony colony, BlockPos location, int width, int length, InventoryField inventory) { this.location = location; this.colony = colony; this.length = length; this.width = width; this.inventory = inventory; } public Field(InventoryField inventory, final InventoryPlayer playerInventory) { colony = this.getColony(); location = new BlockPos(0,1,2); this.inventory = inventory; addSlotToContainer(new Slot(inventory, 0, 80, 34)); //Ddd player inventory slots // Note: The slot numbers are within the player inventory and may be the same as the field inventory. int i; for (i = 0; i < 3; ++i) { for (int j = 0; j < 9; ++j) { addSlotToContainer(new Slot(playerInventory, j+i*9+9, 8+j*18, 84+i*18)); } } for (i = 0; i < 9; ++i) { addSlotToContainer(new Slot(playerInventory, i, 8 + i * 18, 142)); } } /** * Returns the {@link BlockPos} of the current object, also used as ID * * @return {@link BlockPos} of the current object */ public BlockPos getID() { return this.location; // Location doubles as ID } /** * Returns the colony of the field * * @return {@link com.minecolonies.colony.Colony} of the current object */ public Colony getColony() { return this.colony; } /** * Create and load a Field given it's saved NBTTagCompound * * @param colony The owning colony * @param compound The saved data * @return {@link com.minecolonies.colony.Field} created from the compound. */ public static Field createFromNBT(Colony colony, NBTTagCompound compound) { Field field = new Field(colony); field.readFromNBT(compound); return field; } /** * Save data to NBT compound. * Writes the {@link #location} value. * * @param compound {@link net.minecraft.nbt.NBTTagCompound} to write data to */ public void writeToNBT(NBTTagCompound compound) { BlockPosUtil.writeToNBT(compound, TAG_LOCATION, this.location); compound.setBoolean(TAG_TAKEN, taken); compound.setInteger(TAG_LENGTH, length); compound.setInteger(TAG_WIDTH, width); inventory.writeToNBT(compound); } /** * Save data to NBT compound. * Writes the {@link #location} value. * * @param compound {@link net.minecraft.nbt.NBTTagCompound} to write data to */ public void readFromNBT(NBTTagCompound compound) { location = BlockPosUtil.readFromNBT(compound, TAG_LOCATION); taken = compound.getBoolean(TAG_TAKEN); length = compound.getInteger(TAG_LENGTH); width = compound.getInteger(TAG_WIDTH); inventory = new InventoryField("Scarecrow", true); inventory.readFromNBT(compound); } /** * Has the field been taken? * @return true if the field is not free to use, false after releasing it. */ public boolean isTaken() { return this.taken; } /** * Sets the field taken. * @param taken is field free or not */ public void setTaken(boolean taken) { this.taken = taken; } /** * Checks if the field has been planted. * @return true if there are crops planted. */ public boolean isPlanted() { return this.planted; } /** * Sets if there are any crops planted. * @param planted true after planting, false after harvesting. */ public void setPlanted(boolean planted) { this.planted = planted; } /** * Checks if the field needs work (planting, hoeing) * @return true if so. */ public boolean needsWork() { return this.needsWork; } /** * Sets that the field needs work * @param needsWork true if work needed, false after completing the job. */ public void setNeedsWork(boolean needsWork) { this.needsWork = needsWork; } /** * Getter for the length. * @return the fields length. */ public int getLength() { return this.length; } /** * Getter for the width. * @return the fields with. */ public int getWidth() { return this.width; } /** * Getter for MAX_RANGE. * @return the max range. */ public int getMaxRange() { return MAX_RANGE; } /** * Return this citizens inventory. * * @return the inventory this citizen has. */ @NotNull public InventoryField getInventoryField() { return inventory; } @Override public boolean canInteractWith(final EntityPlayer playerIn) { return true; } @Override public ItemStack transferStackInSlot(EntityPlayer playerIn, int slotIndex) { if (slotIndex == 0) { playerIn.inventory.addItemStackToInventory(inventory.getStackInSlot(0)); inventory.setInventorySlotContents(0, null); } else if(inventory.getStackInSlot(0) == null) { int playerIndex = slotIndex < 28 ? slotIndex + 8 : slotIndex - 28; if(playerIn.inventory.getStackInSlot(playerIndex) != null) { inventory.setInventorySlotContents(0, playerIn.inventory.getStackInSlot(playerIndex).splitStack(1)); } } return null; } @Override public ItemStack slotClick(final int slotId, final int clickedButton, final int mode, final EntityPlayer playerIn) { return super.slotClick(slotId, clickedButton, mode, playerIn); } } package com.minecolonies.inventory; import com.minecolonies.colony.materials.MaterialSystem; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraftforge.common.util.Constants; /** * The custom chest of the field. */ public class InventoryField extends InventoryCitizen { /** * NBTTag to store the slot. */ private static final String TAG_SLOT = "slot"; /** * NBTTag to store the items. */ private static final String TAG_ITEMS = "items"; /** * NBTTag to store the custom name. */ private static final String TAG_CUSTOM_NAME = "name"; /** * NBTTag to store the inventory. */ private static final String TAG_INVENTORY = "inventory"; /** * Returned slot if no slat has been found. */ private static final int NO_SLOT = -1; /** * The inventory stack. */ private ItemStack[] stackResult = new ItemStack[1]; /** * The custom name of the inventory. */ private String customName = ""; /** * Creates the inventory of the citizen. * * @param title Title of the inventory. * @param localeEnabled Boolean whether the inventory has a custom name. */ public InventoryField(final String title, final boolean localeEnabled) { super(title, localeEnabled); customName = title; } @Override public int getSizeInventory() { return 1; } @Override public int getInventoryStackLimit() { return 1; } @Override public int getHotbarSize() { return 0; } public ItemStack getStackInSlot(int index) { return this.stackResult[0]; } @Override public boolean hasCustomName() { return true; } /** * Sets the given item stack to the specified slot in the inventory (can be crafting or armor sections). * @param index the slot to set the itemStack. * @param stack the itemStack to set. */ @Override public void setInventorySlotContents(int index, ItemStack stack) { this.stackResult[index] = stack; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) { stack.stackSize = this.getInventoryStackLimit(); } this.markDirty(); } /** * Get the name of this object. For citizens this returns their name. * @return the name of the inventory. */ @Override public String getName() { return this.hasCustomName() ? this.customName : "field.inventory"; } /** * Used to retrieve variables. * @param compound with the give tag. */ @Override public void readFromNBT(NBTTagCompound compound) { NBTTagList nbttaglist = compound.getTagList(TAG_ITEMS, Constants.NBT.TAG_COMPOUND); this.stackResult = new ItemStack[this.getSizeInventory()]; for (int i = 0; i < nbttaglist.tagCount(); ++i) { NBTTagCompound nbttagcompound = nbttaglist.getCompoundTagAt(i); int j = nbttagcompound.getByte(TAG_SLOT) & Byte.MAX_VALUE; if (j != NO_SLOT && j < this.stackResult.length) { this.stackResult[j] = ItemStack.loadItemStackFromNBT(nbttagcompound); } } if (compound.hasKey(TAG_CUSTOM_NAME, Constants.NBT.TAG_STRING)) { this.customName = compound.getString(TAG_CUSTOM_NAME); } } /** * Used to store variables. * @param compound with the given tag. */ @Override public void writeToNBT(NBTTagCompound compound) { NBTTagList nbttaglist = new NBTTagList(); for (int i = 0; i < this.stackResult.length; ++i) { if (this.stackResult[i] != null) { NBTTagCompound nbttagcompound = new NBTTagCompound(); nbttagcompound.setByte(TAG_SLOT, (byte) i); this.stackResult[i].writeToNBT(nbttagcompound); nbttaglist.appendTag(nbttagcompound); } } compound.setTag(TAG_ITEMS, nbttaglist); if (this.hasCustomName()) { compound.setString(TAG_CUSTOM_NAME, this.customName); } compound.setTag(TAG_INVENTORY, nbttaglist); } }
-
I thought there may be a way to do this without any custom gui and container. Since it is only 1 single inventory slot and I do not need anything besides from this =D But thank you for your help. I'll get into all that container and GUI stuff then =D
-
I open the inventory like this. InventoryField inventoryField = ColonyManager.getColony(colony.getID()).getField(pos).getInventoryField(); playerIn.displayGUIChest(inventoryField); And I put items in by drag and drop.
-
I don't use any container I think. InventoryCitizen inherits from IInventory.
-
I currently want to create a custom inventory for a tileEntity. My class inherits another inventory class which implements the rest of the methods But I don't get slots which are not multiples of 9. Is there a way to get that working without using the container class creating container, slots, inventory, GUI and all that overhead for only 1 slot inventory? (I want the player to put in 1 item, and I want to read the content of the slot and nothing more). /** * The custom chest of the field. */ public class InventoryField extends InventoryCitizen { private ItemStack[] stackResult = new ItemStack[1]; private String customName = ""; /** * Creates the inventory of the citizen. * * @param title Title of the inventory. * @param localeEnabled Boolean whether the inventory has a custom name. */ public InventoryField(final String title, final boolean localeEnabled) { super(title, localeEnabled); customName = title; } @Override public int getSizeInventory() { return 1; } @Override public int getInventoryStackLimit() { return 1; } @Override public int getHotbarSize() { return 0; } public ItemStack getStackInSlot(int index) { return this.stackResult[0]; } @Override public boolean hasCustomName() { return true; } /** * Sets the given item stack to the specified slot in the inventory (can be crafting or armor sections). * @param index the slot to set the itemStack. * @param stack the itemStack to set. */ @Override public void setInventorySlotContents(int index, ItemStack stack) { this.stackResult[index] = stack; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) { stack.stackSize = this.getInventoryStackLimit(); } this.markDirty(); } /** * Get the name of this object. For citizens this returns their name. * @return the name of the inventory. */ @Override public String getName() { return this.hasCustomName() ? this.customName : "field.inventory"; } }
-
I currently have a scarecrow model which I would like to Render as a block. The scarecrow is two blocks high. But it doesn't show it's texture and it also renders like into the ground and not into the air. http://i252.photobucket.com/albums/hh24/Raycoms/2016-08-19_08.32.55_zpsdytiqsog.png[/img] public class ModelScarecrowBoth extends ModelBase { //fields ModelRenderer Head; ModelRenderer Post; ModelRenderer LeftArmPeg; ModelRenderer RightArmPeg; ModelRenderer Torso; ModelRenderer RightLegPeg; ModelRenderer LeftLegPeg; ModelRenderer LeftArm; ModelRenderer RightArm; ModelRenderer RightLeg; ModelRenderer LeftLeg; public ModelScarecrowBoth() { textureWidth = 128; textureHeight = 64; Head = new ModelRenderer(this, 0, 0); Head.addBox(-8.2F, -35.6F, -4.2F, 8, 8, ; Head.setRotationPoint(7F, 24F, -1F); Head.setTextureSize(128, 64); Head.mirror = true; setRotation(Head, 0F, 0.1858931F, -0.1092638F); Post = new ModelRenderer(this, 0, 32); Post.addBox(-1F, -16F, -1F, 2, 16, 2); Post.setRotationPoint(0F, 24F, 0F); Post.setTextureSize(128, 64); Post.mirror = true; setRotation(Post, 0F, 0F, 0F); LeftArmPeg = new ModelRenderer(this, 9, 33); LeftArmPeg.addBox(23.5F, 1F, -1F, 2, 2, 2); LeftArmPeg.setRotationPoint(7F, 24F, 0F); LeftArmPeg.setTextureSize(128, 64); LeftArmPeg.mirror = true; setRotation(LeftArmPeg, 0F, 0F, -1.351339F); RightArmPeg = new ModelRenderer(this, 9, 33); RightArmPeg.addBox(-28F, 15.8F, -1F, 2, 2, 2); RightArmPeg.setRotationPoint(7F, 24F, 0F); RightArmPeg.setTextureSize(128, 64); RightArmPeg.mirror = true; setRotation(RightArmPeg, 0F, 0F, 1.351339F); Torso = new ModelRenderer(this, 16, 16); Torso.addBox(-10.3F, -27.6F, -2F, 8, 12, 4); Torso.setRotationPoint(7F, 24F, 0F); Torso.setTextureSize(128, 64); Torso.mirror = true; setRotation(Torso, 0F, 0F, -0.0349066F); RightLegPeg = new ModelRenderer(this, 9, 33); RightLegPeg.addBox(-11F, -4F, -1F, 2, 2, 2); RightLegPeg.setRotationPoint(7F, 24F, 0F); RightLegPeg.setTextureSize(128, 64); RightLegPeg.mirror = true; setRotation(RightLegPeg, 0F, 0F, 0.0872665F); LeftLegPeg = new ModelRenderer(this, 9, 33); LeftLegPeg.addBox(-4.5F, -5F, -1F, 2, 2, 2); LeftLegPeg.setRotationPoint(7F, 24F, 0F); LeftLegPeg.setTextureSize(128, 64); LeftLegPeg.mirror = true; setRotation(LeftLegPeg, 0F, 0F, -0.0872665F); LeftArm = new ModelRenderer(this, 40, 16); LeftArm.addBox(22.5F, -10F, -1.99F, 4, 12, 4); LeftArm.setRotationPoint(7F, 24F, 0F); LeftArm.setTextureSize(128, 64); LeftArm.mirror = true; setRotation(LeftArm, 0F, 0F, -1.351339F); RightArm = new ModelRenderer(this, 40, 16); RightArm.addBox(-29F, 4.8F, -1.99F, 4, 12, 4); RightArm.setRotationPoint(7F, 24F, 0F); RightArm.setTextureSize(128, 64); RightArm.mirror = true; setRotation(RightArm, 0F, 0F, 1.351339F); RightLeg = new ModelRenderer(this, 0, 16); RightLeg.addBox(-12F, -15F, -1.99F, 4, 12, 4); RightLeg.setRotationPoint(7F, 24F, 0F); RightLeg.setTextureSize(128, 64); RightLeg.mirror = true; setRotation(RightLeg, 0F, 0F, 0.0872665F); LeftLeg = new ModelRenderer(this, 0, 16); LeftLeg.addBox(-5.5F, -16F, -1.98F, 4, 12, 4); LeftLeg.setRotationPoint(7F, 24F, 0F); LeftLeg.setTextureSize(128, 64); LeftLeg.mirror = true; setRotation(LeftLeg, 0F, 0F, -0.0872665F); } public void render(float scaleFactor) { Head.render(scaleFactor); Post.render(scaleFactor); LeftArmPeg.render(scaleFactor); RightArmPeg.render(scaleFactor); Torso.render(scaleFactor); RightLegPeg.render(scaleFactor); LeftLegPeg.render(scaleFactor); LeftArm.render(scaleFactor); RightArm.render(scaleFactor); RightLeg.render(scaleFactor); LeftLeg.render(scaleFactor); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } } I also have a tileEntitySpecialRenderer @SideOnly(Side.CLIENT) public class TileEntityScarecrowRenderer extends TileEntitySpecialRenderer<ScarecrowTileEntity> { private final ModelScarecrowBoth model; public TileEntityScarecrowRenderer() { this.model = new ModelScarecrowBoth(); } @Override public void renderTileEntityAt(ScarecrowTileEntity te, double posX, double posY, double posZ, float partialTicks, int destroyStage) { GlStateManager.pushMatrix(); // store the transformation GlStateManager.translate(posX, posY, posZ); // set viewport to tile entity position to render it /* ============ Rendering Code goes here ============ */ EnumFacing facing = te.getWorld().getBlockState(te.getPos()).getValue(BlockHutField.FACING); this.bindTexture(this.getResourceLocation(te)); GlStateManager.rotate(180, te.getPos().getX(), te.getPos().getY(), te.getPos().getZ()); this.model.render(.0625f); /* ============ Rendering Code stops here =========== */ GlStateManager.popMatrix(); // restore the transformation, so other renderer's are not messed up } private ResourceLocation getResourceLocation(ScarecrowTileEntity tileentity) { String loc; if(tileentity.getType()) loc = "/resources/assets/minecolonies/textures/blocks/blockScarecrowPumpkin.png"; else loc = "/resources/assets/minecolonies/textures/blocks/blockScarecrowNormal.png"; return new ResourceLocation(Constants.MOD_ID + ":" + loc); } } I don't have any json files, are they necessary? How do I generate them?