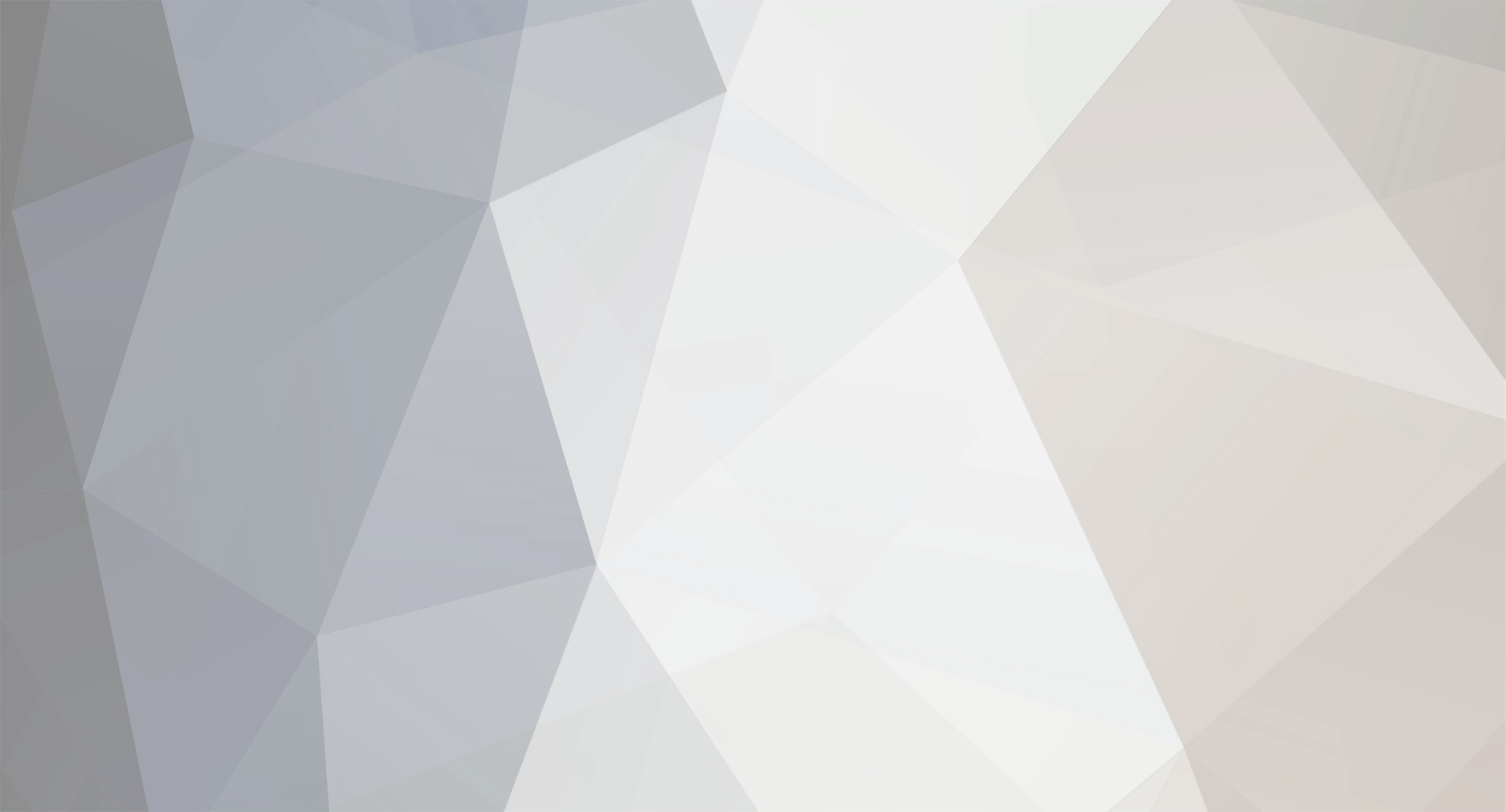
Lambda
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by Lambda
-
Oops, forgot to change that to the proper iterator.. Thanks, seems to work now.
-
Didn't know the PlayerTickEvent existed, will be using that and i've simplied all of my methods and its working now.. Thanks. However, my capability isnt reading from NBT, so it restores upon restart. Here is the updated capabilityData: public class CapabilityMagicData implements IManaData { protected int mana; protected int level; protected int corruption; protected int experience; protected int capacity; protected int xpIncrement; private int checkTick; protected int maxReceive; protected int maxExtract; private EntityLivingBase entity; private EntityPlayer entityPlayer; public CapabilityMagicData(int capacity) { this(capacity, capacity / 2, capacity, capacity); } public CapabilityMagicData(int capacity, int maxTransfer) { this(capacity, capacity / 2, maxTransfer, maxTransfer); } public CapabilityMagicData(int capacity, int xpIncrement, int maxReceive, int maxExtract) { this.capacity = capacity; this.xpIncrement = xpIncrement; this.maxReceive = maxReceive; this.maxExtract = maxExtract; } public EntityLivingBase getEntity() { return entity; } public EntityPlayer getPlayerEntity() { return entityPlayer; } public void setEntity(EntityLivingBase entity) { this.entity = entity; } public void setEntityPlayer(EntityPlayer entity) { this.entityPlayer = entity; } public void checkExp() { Minecraft mc = Minecraft.getMinecraft(); setEntityPlayer(mc.thePlayer); int currentExp = this.getExperienceStored(); int expToLevelup = this.xpIncrement * this.getLevelStored(); checkTick++; if (currentExp >= expToLevelup) { System.out.println("leval up"); this.setLevel(this.getLevelStored() + 1); this.setExperience(0); } PacketHandlerHelper.sendCapabilityPacket(getPlayerEntity(), true); } @Override public int receiveMana(int receive) { setManaStored(receive + this.getManaStored()); return this.getManaStored(); } @Override public int extractMana(int extract) { setManaStored(this.getMaxManaStored() - extract); return this.getManaStored(); } @Override public int receiveExperience(int receive) { setExperience(receive + this.getExperienceStored()); return this.getExperienceStored(); } @Override public int receiveCorruption(int receive) { setCorruption(receive + this.getCorruptionStored()); return this.getCorruptionStored(); } @Override public int extractCorruption(int extract) { setCorruption(this.getCorruptionStored() - extract); return this.getCorruptionStored(); } @Override public int getManaStored() { return mana; } @Override public int getLevelStored() { return level; } @Override public int getCorruptionStored() { return corruption; } @Override public int getExperienceStored() { return experience; } @Override public int getMaxManaStored() { return capacity; } @Override public boolean canExtract() { return this.maxExtract > 0; } @Override public boolean canReceive() { return this.maxReceive > 0; } public NBTBase writeData() { NBTTagCompound tag = new NBTTagCompound(); tag.setInteger("Mana", getManaStored()); tag.setInteger("Level", getLevelStored()); tag.setInteger("Corruption", getCorruptionStored()); tag.setInteger("Experience", getExperienceStored()); return tag; } public void readData(NBTBase nbt) { NBTTagCompound tag = (NBTTagCompound) nbt; this.setManaStored(tag.getInteger("Mana")); this.setLevel(tag.getInteger("Level")); this.setCorruption(tag.getInteger("Corruption")); this.setExperience(tag.getInteger("Experience")); } public void setManaStored(int manaToSet){ this.mana = manaToSet; } public void setCorruption(int corruption){ this.corruption = corruption; } public void setLevel(int level){ this.level = level; } public void setExperience(int experience){ this.experience = experience; } } the provider: public class CapabilityMagicProvider implements ICapabilityProvider, ICapabilitySerializable<NBTTagCompound> { public static final ResourceLocation KEY = new ResourceLocation(ModUtil.MOD_ID, "mana_atr"); private CapabilityMagicData INSTANCE = new CapabilityMagicData(5000); public CapabilityMagicProvider() {} public CapabilityMagicProvider(EntityLivingBase entity) { INSTANCE.setEntity(entity); if(entity instanceof EntityPlayerMP) INSTANCE.setEntityPlayer((EntityPlayerMP)entity); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { return capability == CapabilityMagic.MANA; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if (capability == CapabilityMagic.MANA) return (T) INSTANCE; return null; } @Override public NBTTagCompound serializeNBT() { return (NBTTagCompound) CapabilityMagic.MANA.writeNBT(INSTANCE, null); } @Override public void deserializeNBT(NBTTagCompound nbt) { CapabilityMagic.MANA.readNBT(INSTANCE, null, nbt); } } Thanks.
-
Now, it wont even add to the list.. updated code @Override public void updateEntity() { super.updateEntity(); System.out.println(crystalsAround.size()); Iterable<BlockPos> positions = BlockPos.getAllInBox(pos.add(-3,-3,-3), pos.add(3,3,3)); for(Iterator<BlockPos> i = positions.iterator(); i.hasNext(); ) { TileEntity te = worldObj.getTileEntity(i.next()); if (te instanceof TileEntityManaCrystal) { if (!crystalsAround.contains(te)) { crystalsAround.add(te); } } } validateTe(); } public void validateTe() { Iterator<TileEntity> it = crystalsAround.listIterator(); while (it.hasNext()) { TileEntity te = it.next(); if(worldObj.getTileEntity(te.getPos()) == null) { it.remove(); } } } }
-
public int receiveExperienceInternal(int receive) { receiveExperience(receive); int toReturn = receive+=experience; return toReturn; } @Override public int receiveExperience(int receive) { int currentExperience = this.getExperienceStored(); int experienceReceived = receive+=currentExperience; experience += currentExperience; return experienceReceived; } , is how, I temporarily add it here: @SubscribeEvent public static void LivingUpdateEvent(LivingEvent.LivingUpdateEvent event) { if(event.getEntity() instanceof EntityPlayer) { if(event.getEntity().isSneaking() && event.getEntity().hasCapability(CapabilityMagic.MANA, null)) { CapabilityMagicData cap = event.getEntity().getCapability(CapabilityMagic.MANA, null); cap.receiveExperienceInternal(200); } } }
-
This: public void validateTe() { Iterator<TileEntity> it = crystalsAround.listIterator(); while (it.hasNext()) { TileEntity te = it.next(); if(worldObj.getTileEntity(te.getPos()) == null) { crystalsAround.remove(te); } } } seems to crash with: ---- Minecraft Crash Report ---- // Surprise! Haha. Well, this is awkward. Time: 12/17/16 3:17 PM Description: Ticking block entity java.util.ConcurrentModificationException at java.util.ArrayList$Itr.checkForComodification(ArrayList.java:901) at java.util.ArrayList$Itr.next(ArrayList.java:851) at com.lambda.plentifulmisc.tile.magic.TileEntityCrystalSearch.validateTe(TileEntityCrystalSearch.java:45) at com.lambda.plentifulmisc.tile.magic.TileEntityCrystalSearch.updateEntity(TileEntityCrystalSearch.java:39) at com.lambda.plentifulmisc.tile.magic.TileEntityManaAbsorber.updateEntity(TileEntityManaAbsorber.java:16) at com.lambda.plentifulmisc.tile.TileEntityBase.update(TileEntityBase.java:188) at net.minecraft.world.World.updateEntities(World.java:1968) at net.minecraft.world.WorldServer.updateEntities(WorldServer.java:646) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:794) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:698) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:156) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:547) at java.lang.Thread.run(Thread.java:745)
-
Okay, my debugging is showing that everything is up to date: [15:08:23] [Client thread/INFO]: [sTDOUT]: TO Bytes DATA: {Experience:0,Mana:0,Corruption:0,Level:1} [15:08:23] [Netty Server IO #1/INFO]: [sTDOUT]: From Bytes DATA: {Experience:0,Mana:0,Corruption:0,Level:1} [15:08:23] [Netty Server IO #1/INFO]: [sTDOUT]: From Bytes DATA: {Experience:0,Mana:0,Corruption:0,Level:1} [15:08:23] [server thread/INFO]: [sTDOUT]: HANDLE DATA DATA: {Experience:0,Mana:0,Corruption:0,Level:1} [15:08:23] [server thread/INFO]: [sTDOUT]: Message Data: {Experience:0,Mana:0,Corruption:0,Level:1} I'm starting to think it has to do with how I'm adding the exp.
-
Thanks.. however, now the list doesnt remove it unless you destroy them in the same order you placed them, ex: placing block: 1,2,3 must be destroyed in: 1,2,3, for it to properly remove from the list. If its destroy in 3,2,1 it will remove all of them when 1 is destroyed. Here is the code that validates the te: public void validateTe() { for(int i = 0; i < crystalsAround.size(); i++) { TileEntity te = crystalsAround.get(i); if(worldObj.getTileEntity(te.getPos()) == null) { crystalsAround.remove(te); }else { return; } if(i > crystalsAround.size()) { return; } } } and here is the entire TE: public class TileEntityCrystalSearch extends TileEntityBase { public final List<TileEntity> crystalsAround = new ArrayList<TileEntity>(); public TileEntityCrystalSearch(String name) { super("crystalSearcher"); } @Override public void updateEntity() { super.updateEntity(); Iterable<BlockPos> positions = BlockPos.getAllInBox(pos.add(-3,-3,-3), pos.add(3,3,3)); for(Iterator<BlockPos> i = positions.iterator(); i.hasNext(); ) { TileEntity te = worldObj.getTileEntity(i.next()); if (te instanceof TileEntityManaCrystal) { if (!crystalsAround.contains(te)) { crystalsAround.add(te); } } } validateTe(); } public void validateTe() { for(int i = 0; i < crystalsAround.size(); i++) { TileEntity te = crystalsAround.get(i); if(worldObj.getTileEntity(te.getPos()) == null) { crystalsAround.remove(te); }else { return; } if(i > crystalsAround.size()) { return; } } } } Thanks.
-
Hey there, So I'm having an issue with trying to detect TEs in a box, using getAllInBox , how every, I'm having troubling interating though it.. Here is some pseudocode of what I want to happen: Iterable<BlockPos> positions = BlockPos.getAllInBox(pos.add(-3,-3,-3), pos.add(3,3,3)); for(BlockPos pos : positions.iterator().next()) { } However, BlockPos are not applicable. Thanks,
-
here, PacketHandlerHelper.sendCapabilityPacket(getPlayerEntity(), true); in the check function, which currently runs everytick
-
Hey there, so when trying to 'add experience' to my custom capability, it just.. doesn't add. Either my math is off, or my packets are not working correctly. So here is some code, I will highlight some parts so you don't have to go digging for it. And also, I'll post the github respective. Here some quick answers to some possible questions: - Registered - Yes. - Packets Registered - Yes So firstly, we have the 'CapabilityMagicData': public class CapabilityMagicData implements IManaData { protected int mana; protected int level; protected int corruption; protected int experience; protected int capacity; protected int xpIncrement; private int checkTick; protected int maxReceive; protected int maxExtract; private EntityLivingBase entity; private EntityPlayer entityPlayer; public CapabilityMagicData(int capacity) { this(capacity, capacity / 2, capacity, capacity); } public CapabilityMagicData(int capacity, int maxTransfer) { this(capacity, capacity / 2, maxTransfer, maxTransfer); } public CapabilityMagicData(int capacity, int xpIncrement, int maxReceive, int maxExtract) { this.capacity = capacity; this.xpIncrement = xpIncrement; this.maxReceive = maxReceive; this.maxExtract = maxExtract; } public EntityLivingBase getEntity() { return entity; } public EntityPlayer getPlayerEntity() { return entityPlayer; } public void setEntity(EntityLivingBase entity) { this.entity = entity; } public void setEntityPlayer(EntityPlayer entity) { this.entityPlayer = entity; } public void checkExp() { int currentExp = this.getExperienceStored(); int expToLevelup = this.xpIncrement * this.getLevelStored(); checkTick++; System.out.println("updating"); if(currentExp >= expToLevelup) { System.out.println("leval up"); this.setLevel(this.getLevelStored() + 1); this.setExperience(0); } if(checkTick >= 40) { System.out.println(this.getManaStored()); System.out.println(this.getCorruptionStored()); System.out.println(this.getLevelStored()); System.out.println(this.getExperienceStored()); checkTick=0; } PacketHandlerHelper.sendCapabilityPacket(getPlayerEntity(), true); } public int extractManaInternal(int maxExtract, boolean simulate){ int before = this.maxExtract; this.maxExtract = Integer.MAX_VALUE; int toReturn = this.extractMana(maxExtract, simulate); this.maxExtract = before; return toReturn; } public int receiveManaInternal(int maxReceive, boolean simulate){ int before = this.maxReceive; this.maxReceive = Integer.MAX_VALUE; int toReturn = this.receiveMana(maxReceive, simulate); this.maxReceive = before; return toReturn; } public int receiveExperienceInternal(int receive) { receiveExperience(receive); int toReturn = receive+=experience; return toReturn; } public int receiveCorruptionInternal(int receive) { int before = this.maxReceive; this.maxReceive = Integer.MAX_VALUE; int toReturn = this.receiveCorruption(receive); this.maxReceive = before; return toReturn; } public int extractCorruptionInternal(int extract) { int before = this.maxExtract; this.maxExtract = Integer.MAX_VALUE; int toReturn = this.extractCorruption(extract); this.maxExtract = before; return toReturn; } @Override public int receiveMana(int maxReceive, boolean simulate) { if (!canReceive()) return 0; int manaReceived = Math.min(capacity - mana, Math.min(this.maxReceive, maxReceive)); if (!simulate) mana += manaReceived; return manaReceived; } @Override public int extractMana(int maxExtract, boolean simulate) { if (!canExtract()) return 0; int manaExtracted = Math.min(mana, Math.min(this.maxExtract, maxExtract)); if (!simulate) mana -= manaExtracted; return manaExtracted; } @Override public int receiveExperience(int receive) { int currentExperience = this.getExperienceStored(); int experienceReceived = receive+=currentExperience; experience += currentExperience; return experienceReceived; } @Override public int receiveCorruption(int receive) { int currentCorruption = this.getCorruptionStored(); int corruptionReceived = receive+=currentCorruption; corruption += corruptionReceived; return corruptionReceived; } @Override public int extractCorruption(int extract) { int corruptionExtracted = Math.min(corruption, Math.min(this.maxExtract, extract)); corruption -= corruptionExtracted; return corruptionExtracted; } @Override public int getManaStored() { return mana; } @Override public int getLevelStored() { return level; } @Override public int getCorruptionStored() { return corruption; } @Override public int getExperienceStored() { return experience; } @Override public int getMaxManaStored() { return capacity; } @Override public boolean canExtract() { return this.maxExtract > 0; } @Override public boolean canReceive() { return this.maxReceive > 0; } public NBTBase writeData() { NBTTagCompound tag = new NBTTagCompound(); tag.setInteger("Mana", getManaStored()); tag.setInteger("Level", getLevelStored()); tag.setInteger("Corruption", getCorruptionStored()); tag.setInteger("Experience", getExperienceStored()); return tag; } public void readData(NBTBase nbt) { NBTTagCompound tag = (NBTTagCompound) nbt; tag.setInteger("Mana", this.getManaStored()); tag.setInteger("Level", this.getLevelStored()); tag.setInteger("Corruption", this.getCorruptionStored()); tag.setInteger("Experience", this.getExperienceStored()); } public void readFromNBT(NBTTagCompound compound){ this.setManaStored(compound.getInteger("Mana")); this.setLevel(compound.getInteger("Level")); this.setCorruption(compound.getInteger("Corruption")); this.setExperience(compound.getInteger("Experience")); } public void writeToNBT(NBTTagCompound compound){ compound.setInteger("Mana", this.getManaStored()); compound.setInteger("Level", this.getLevelStored()); compound.setInteger("Corruption", this.getCorruptionStored()); compound.setInteger("Experience", this.getExperienceStored()); } public void setManaStored(int manaToSet){ this.mana = manaToSet; } public void setCorruption(int corruption){ this.corruption = corruption; } public void setLevel(int level){ this.level = level; } public void setExperience(int experience){ this.experience = experience; } } Here are some highlight points at which I think the problem is happening: public int receiveExperienceInternal(int receive) { receiveExperience(receive); int toReturn = receive+=experience; return toReturn; } @Override public int receiveExperience(int receive) { int currentExperience = this.getExperienceStored(); int experienceReceived = receive+=currentExperience; experience += currentExperience; return experienceReceived; } public void checkExp() { int currentExp = this.getExperienceStored(); int expToLevelup = this.xpIncrement * this.getLevelStored(); checkTick++; System.out.println("updating"); if(currentExp >= expToLevelup) { System.out.println("leval up"); this.setLevel(this.getLevelStored() + 1); this.setExperience(0); } if(checkTick >= 40) { System.out.println(this.getManaStored()); System.out.println(this.getCorruptionStored()); System.out.println(this.getLevelStored()); System.out.println(this.getExperienceStored()); checkTick=0; } PacketHandlerHelper.sendCapabilityPacket(getPlayerEntity(), true); } Next, the PacketHandler, which as it names, handles all the packets: public final class PacketHandler{ public static final List<IDataHandler> DATA_HANDLERS = new ArrayList<IDataHandler>(); public static final IDataHandler PARTICLE_HANDLER = new IDataHandler(){ @Override @SideOnly(Side.CLIENT) public void handleData(NBTTagCompound compound){ AssetUtil.renderParticlesFromAToB(compound.getDouble("StartX"), compound.getDouble("StartY"), compound.getDouble("StartZ"), compound.getDouble("EndX"), compound.getDouble("EndY"), compound.getDouble("EndZ"), compound.getInteger("ParticleAmount"), compound.getFloat("ParticleSize"), new float[]{compound.getFloat("Color1"), compound.getFloat("Color2"), compound.getFloat("Color3")}, compound.getFloat("AgeMultiplier")); } }; public static final IDataHandler TILE_ENTITY_HANDLER = new IDataHandler(){ @Override @SideOnly(Side.CLIENT) public void handleData(NBTTagCompound compound){ World world = Minecraft.getMinecraft().theWorld; if(world != null){ TileEntity tile = world.getTileEntity(new BlockPos(compound.getInteger("X"), compound.getInteger("Y"), compound.getInteger("Z"))); if(tile instanceof TileEntityBase){ ((TileEntityBase)tile).readSyncableNBT(compound.getCompoundTag("Data"), TileEntityBase.NBTType.SYNC); } } } }; public static final IDataHandler GUI_BUTTON_TO_TILE_HANDLER = new IDataHandler(){ @Override public void handleData(NBTTagCompound compound){ World world = DimensionManager.getWorld(compound.getInteger("WorldID")); TileEntity tile = world.getTileEntity(new BlockPos(compound.getInteger("X"), compound.getInteger("Y"), compound.getInteger("Z"))); if(tile instanceof IButtonReactor){ IButtonReactor reactor = (IButtonReactor)tile; Entity entity = world.getEntityByID(compound.getInteger("PlayerID")); if(entity instanceof EntityPlayer){ reactor.onButtonPressed(compound.getInteger("ButtonID"), (EntityPlayer)entity); } } } }; public static final IDataHandler GUI_BUTTON_TO_CONTAINER_HANDLER = new IDataHandler(){ @Override public void handleData(NBTTagCompound compound){ World world = DimensionManager.getWorld(compound.getInteger("WorldID")); Entity entity = world.getEntityByID(compound.getInteger("PlayerID")); if(entity instanceof EntityPlayer){ Container container = ((EntityPlayer)entity).openContainer; if(container instanceof IButtonReactor){ ((IButtonReactor)container).onButtonPressed(compound.getInteger("ButtonID"), (EntityPlayer)entity); } } } }; public static final IDataHandler GUI_NUMBER_TO_TILE_HANDLER = new IDataHandler(){ @Override public void handleData(NBTTagCompound compound){ World world = DimensionManager.getWorld(compound.getInteger("WorldID")); TileEntity tile = world.getTileEntity(new BlockPos(compound.getInteger("X"), compound.getInteger("Y"), compound.getInteger("Z"))); if(tile instanceof INumberSender){ INumberSender reactor = (INumberSender)tile; reactor.onNumberReceived(compound.getInteger("Number"), compound.getInteger("NumberID"), (EntityPlayer)world.getEntityByID(compound.getInteger("PlayerID"))); } } }; public static final IDataHandler GUI_STRING_TO_TILE_HANDLER = new IDataHandler(){ @Override public void handleData(NBTTagCompound compound){ World world = DimensionManager.getWorld(compound.getInteger("WorldID")); TileEntity tile = world.getTileEntity(new BlockPos(compound.getInteger("X"), compound.getInteger("Y"), compound.getInteger("Z"))); if(tile instanceof IStringSender){ IStringSender reactor = (IStringSender)tile; reactor.onTextReceived(compound.getString("Text"), compound.getInteger("TextID"), (EntityPlayer)world.getEntityByID(compound.getInteger("PlayerID"))); } } }; public static final IDataHandler CHANGE_PLAYER_DATA_HANDLER = new IDataHandler(){ @Override public void handleData(NBTTagCompound compound){ NBTTagCompound data = compound.getCompoundTag("Data"); UUID id = compound.getUniqueId("UUID"); PlayerData.getDataFromPlayer(id).readFromNBT(data, false); if(compound.getBoolean("Log")){ ModUtil.LOGGER.info("Receiving (new or changed) Player Data for player with UUID "+id+"."); } } }; public static final IDataHandler MANA_CAPABILITY = new IDataHandler() { @Override public void handleData(NBTTagCompound compound) { Minecraft mc = Minecraft.getMinecraft(); if(mc.thePlayer.hasCapability(CapabilityMagic.MANA, null)) { CapabilityMagicData cap = mc.thePlayer.getCapability(CapabilityMagic.MANA, null); if(mc.thePlayer != null && mc.thePlayer.getCapability(CapabilityMagic.MANA, null) != null) { cap.readData(compound); } } } }; public static SimpleNetworkWrapper theNetwork; public static void init(){ theNetwork = NetworkRegistry.INSTANCE.newSimpleChannel(ModUtil.MOD_ID); theNetwork.registerMessage(PacketServerToClient.Handler.class, PacketServerToClient.class, 0, Side.CLIENT); theNetwork.registerMessage(PacketClientToServer.Handler.class, PacketClientToServer.class, 1, Side.SERVER); DATA_HANDLERS.add(PARTICLE_HANDLER); DATA_HANDLERS.add(TILE_ENTITY_HANDLER); DATA_HANDLERS.add(GUI_BUTTON_TO_TILE_HANDLER); DATA_HANDLERS.add(GUI_STRING_TO_TILE_HANDLER); DATA_HANDLERS.add(GUI_NUMBER_TO_TILE_HANDLER); DATA_HANDLERS.add(CHANGE_PLAYER_DATA_HANDLER); DATA_HANDLERS.add(GUI_BUTTON_TO_CONTAINER_HANDLER); DATA_HANDLERS.add(MANA_CAPABILITY); } } Here are the points that I've added with this Capability: public static final IDataHandler MANA_CAPABILITY = new IDataHandler() { @Override public void handleData(NBTTagCompound compound) { Minecraft mc = Minecraft.getMinecraft(); if(mc.thePlayer.hasCapability(CapabilityMagic.MANA, null)) { CapabilityMagicData cap = mc.thePlayer.getCapability(CapabilityMagic.MANA, null); if(mc.thePlayer != null && mc.thePlayer.getCapability(CapabilityMagic.MANA, null) != null) { cap.readData(compound); } } } }; DATA_HANDLERS.add(MANA_CAPABILITY); // Inside the init() function Here is the helper, which is so I can easily access the packets, and send them when needed: public static void sendCapabilityPacket(EntityPlayer player, boolean toClient) { NBTTagCompound compound = new NBTTagCompound(); if(player != null && player.hasCapability(CapabilityMagic.MANA, null)) { CapabilityMagicData cap = player.getCapability(CapabilityMagic.MANA, null); int tempMana = cap.getManaStored(); int tempCorruption = cap.getCorruptionStored(); int tempLevel = cap.getLevelStored(); int tempExperience = cap.getExperienceStored(); compound.setInteger("Mana", tempMana); compound.setInteger("Corruption", tempCorruption); compound.setInteger("Level", tempLevel); compound.setInteger("Experience", tempExperience); if(toClient) { if(player instanceof EntityPlayerMP) { PacketHandler.theNetwork.sendTo(new PacketServerToClient(compound, PacketHandler.MANA_CAPABILITY), (EntityPlayerMP)player); } } else { PacketHandler.theNetwork.sendToServer(new PacketClientToServer(compound, PacketHandler.MANA_CAPABILITY)); } } } Lastly, my PacketServerToClient: public class PacketServerToClient implements IMessage{ private NBTTagCompound data; private IDataHandler handler; public PacketServerToClient(){ } public PacketServerToClient(NBTTagCompound data, IDataHandler handler){ this.data = data; this.handler = handler; } @Override public void fromBytes(ByteBuf buf){ PacketBuffer buffer = new PacketBuffer(buf); try{ this.data = buffer.readNBTTagCompoundFromBuffer(); int handlerId = buffer.readInt(); if(handlerId >= 0 && handlerId < PacketHandler.DATA_HANDLERS.size()){ this.handler = PacketHandler.DATA_HANDLERS.get(handlerId); } } catch(Exception e){ ModUtil.LOGGER.error("Cannot receive a client packet!", e); } } @Override public void toBytes(ByteBuf buf){ PacketBuffer buffer = new PacketBuffer(buf); buffer.writeNBTTagCompoundToBuffer(this.data); buffer.writeInt(PacketHandler.DATA_HANDLERS.indexOf(this.handler)); } public static class Handler implements IMessageHandler<PacketServerToClient, IMessage> { @Override @SideOnly(Side.CLIENT) public IMessage onMessage(PacketServerToClient aMessage, MessageContext ctx){ final PacketServerToClient message = aMessage; Minecraft.getMinecraft().addScheduledTask(new Runnable(){ @Override public void run(){ if(message.data != null && message.handler != null){ message.handler.handleData(message.data); } } }); return null; } } } Also, the github: https://github.com/LambdaXV/PlentifulMisc/tree/master/src/main/java/com/lambda/plentifulmisc Thanks for your time.
-
Okay, this is a bit of a strange question... but would someone 'compile' me a list of tasks that progressively gets harder? I'll try to do them myself and check them off, then move on. ex: Basic block > a te that detects its environment > etc.
-
Hey there, So, I've been modding for 4 years, off and on... but I realized, I cant really do a lot.. my knowledge seems limited when it comes to Minecraft... I look at code from botaina or mods like this. I really, don't.. understand.. stand.. *A LOT*... So my questions is.. How would I learn these things..? Tessellations , TEs, Guis, etc.. Should I just spend a month studying, looking though the default classes, forge, other mods... etc? Maybe there is a better way? Thanks,
-
Edit: Using the UpdateEntity event, and it seems to add fine, maybe its something to do with my item?
-
Hey there, *Packets are being to used to sync everything* SO I have a capability that adds: level, corruption, and experience. Simple. However, when I'm using an Item to add to the capability, it displays for 1 frame, then resets, here are some pictures of whats happening: Okay, so I am syncing the client every time the value is changed and when the player connects.. So here is the capability data: public class CapabilityLevelData extends LevelHandler { private EntityLivingBase entity; public CapabilityLevelData(){ super(500); } public EntityLivingBase getEntity() { return entity; } public void setEntity(EntityLivingBase entity) { this.entity = entity; } public float addExperienceInternal(float experienceToAdd) { float toReturn = this.addExperience(experienceToAdd); sendPacket(); return toReturn; } public int addLevelsInternal(int levelsToAdd) { int toReturn = this.addLevels(levelsToAdd); sendPacket(); return toReturn; } public int removeLevelsInternal(int levelsToRemove) { int toReturn = this.removeLevels(levelsToRemove); sendPacket(); return toReturn; } public int addCorruptionInternal(int corruptionToAdd) { int toReturn = this.addCorruption(corruptionToAdd); sendPacket(); return toReturn; } public int removeCorruptionInternal(int corruptionToRemove) { int toReturn = this.removeCorruption(corruptionToRemove); sendPacket(); return toReturn; } //todo public void updateExpForLevelUp() { float currentXP = this.getExperience(); int currentLevel = this.getLevels(); int currentCorruption = this.getCorruption(); float currentXPToLevelUp = currentLevel * (levelUpIncrement + currentCorruption); if(currentXP >= currentXPToLevelUp) { this.addLevelsInternal(1); this.setExpereince(0); } if(currentLevel <= 0) { this.setLevel(1); } if(currentLevel >= 100) { this.setLevel(100); } } @Override public float addExperience(float experienceToAdd) { float currentExp = this.getExperience(); float returnedExp = currentExp+=experienceToAdd; this.setExpereince(returnedExp); return returnedExp; } @Override public int addLevels(int levelsToAdd) { int currentLevel = this.getLevels(); int returnedLevel = currentLevel+=levelsToAdd; this.setLevel(returnedLevel); sendPacket(); return returnedLevel; } @Override public int removeLevels(int levelsToRemove) { int currentLevel = this.getLevels(); int returnedLevel = currentLevel-+levelsToRemove; this.setLevel(returnedLevel); sendPacket(); return returnedLevel; } @Override public int addCorruption(int corruptionToAdd) { int currentCurruption = this.getCorruption(); int returnedCorruption = currentCurruption+=corruptionToAdd; this.setCorruption(returnedCorruption); sendPacket(); return returnedCorruption; } @Override public int removeCorruption(int corruptionToRemove) { int currentCurruption = this.getCorruption(); int returnedCorruption = currentCurruption-=corruptionToRemove; this.setCorruption(returnedCorruption); sendPacket(); return returnedCorruption; } public void readFromNBT(NBTTagCompound compound){ this.setLevel(compound.getInteger("Level")); this.setCorruption(compound.getInteger("Corruption")); this.setExpereince(compound.getFloat("Experience")); } public void writeToNBT(NBTTagCompound compound){ compound.setInteger("Level", this.getLevels()); compound.setInteger("Corruption", this.getCorruption()); compound.setFloat("Experience", this.getExperience()); } public NBTBase writeData() { NBTTagCompound tag = new NBTTagCompound(); tag.setInteger("Level", level); tag.setInteger("Corruption", levelCorruption); tag.setFloat("Experience", experience); return tag; } public void readData(NBTBase nbt) { NBTTagCompound tag = (NBTTagCompound) nbt; this.setLevel(tag.getInteger("Level")); this.setCorruption(tag.getInteger("Corruption")); this.setExpereince(tag.getFloat("Experience")); } public int getLevels() { return level; } public int getCorruption() { return levelCorruption; } public float getExperience() { return experience; } public int setLevel(int levelToSet) { return this.level = levelToSet; } public int setCorruption(int corruptionToSet) { return this.levelCorruption = corruptionToSet; } public float setExpereince(float experienceToSet) { return this.experience = experienceToSet; } public void sendPacket() { if(entity instanceof EntityPlayerMP && entity != null) { PlentifulMisc.network.sendTo(new LevelNetworkSyncClient(this.getLevels()), (EntityPlayerMP) entity); PlentifulMisc.network.sendTo(new CorruptionNetworkSyncClient(this.getCorruption()), (EntityPlayerMP) entity); PlentifulMisc.network.sendTo(new ExperienceNetworkSyncClient(this.getExperience()), (EntityPlayerMP) entity); } } } I'm thinking that its because I'm using 3 different networks for 3 different values, maybe I can store it into one? Last time I did that, I get an error... here is how I'm registering them: network.registerMessage(LevelClientNetworkHandler.class, LevelNetworkSyncClient.class, 1, Side.CLIENT); network.registerMessage(CorruptionClientNetworkHandler.class, CorruptionNetworkSyncClient.class, 2, Side.CLIENT); network.registerMessage(ExperienceClientNetworkHandler.class, ExperienceNetworkSyncClient.class, 3, Side.CLIENT); , then how I'm modifying the cap: public ActionResult<ItemStack> onItemRightClick(World worldIn, EntityPlayer playerIn, EnumHand itemStackIn) { if(!worldIn.isRemote) { if (playerIn.hasCapability(CapabilityLevel.LEVEL, null)) { CapabilityLevelData cap = playerIn.getCapability(CapabilityLevel.LEVEL, null); cap.addExperienceInternal(200); if(playerIn.isSneaking()) { cap.removeLevelsInternal(10); } return new ActionResult<>(EnumActionResult.SUCCESS, playerIn.getHeldItem(itemStackIn)); } } return new ActionResult(EnumActionResult.PASS, playerIn.getHeldItem(itemStackIn)); } } Thanks for your time,
-
hey there, So I've ran into an issue when trying to read/write to ints: io.netty.handler.codec.DecoderException: java.lang.IndexOutOfBoundsException: readerIndex(4) + length(4) exceeds writerIndex(4): SlicedByteBuf(ridx: 4, widx: 4, cap: 4/4, unwrapped: UnpooledHeapByteBuf(ridx: 0, widx: 5, cap: 256)) This leads me to believe that I have to use 2 different classes to handle the 2 ints? Here is the code, maybe there is a way? package com.lambda.plentifulmisc.network.magic.level; import io.netty.buffer.ByteBuf; import net.minecraftforge.fml.common.network.simpleimpl.IMessage; /** * Created by Blake on 12/14/2016. */ public class LevelNetworkSyncClient implements IMessage { public int level; public int corruption; public LevelNetworkSyncClient(){} public LevelNetworkSyncClient(int levelToSend, int corruptionToSend) { this.level = levelToSend; this.corruption = corruptionToSend; } @Override public void toBytes(ByteBuf buf) { buf.writeInt(level); buf.writeInt(corruption); } @Override public void fromBytes(ByteBuf buf) { level = buf.readInt(); corruption = buf.readInt(); } } Here is the stacktrace: [20:27:10] [Netty Local Client IO #0/ERROR]: SimpleChannelHandlerWrapper exception io.netty.handler.codec.DecoderException: java.lang.IndexOutOfBoundsException: readerIndex(4) + length(4) exceeds writerIndex(4): SlicedByteBuf(ridx: 4, widx: 4, cap: 4/4, unwrapped: UnpooledHeapByteBuf(ridx: 0, widx: 5, cap: 256)) at io.netty.handler.codec.MessageToMessageDecoder.channelRead(MessageToMessageDecoder.java:99) ~[MessageToMessageDecoder.class:4.0.23.Final] at io.netty.handler.codec.MessageToMessageCodec.channelRead(MessageToMessageCodec.java:111) ~[MessageToMessageCodec.class:4.0.23.Final] at io.netty.channel.AbstractChannelHandlerContext.invokeChannelRead(AbstractChannelHandlerContext.java:333) [AbstractChannelHandlerContext.class:4.0.23.Final] at io.netty.channel.AbstractChannelHandlerContext.fireChannelRead(AbstractChannelHandlerContext.java:319) [AbstractChannelHandlerContext.class:4.0.23.Final] at io.netty.channel.DefaultChannelPipeline.fireChannelRead(DefaultChannelPipeline.java:787) [DefaultChannelPipeline.class:4.0.23.Final] at io.netty.channel.embedded.EmbeddedChannel.writeInbound(EmbeddedChannel.java:169) [EmbeddedChannel.class:4.0.23.Final] at net.minecraftforge.fml.common.network.internal.FMLProxyPacket.processPacket(FMLProxyPacket.java:109) [FMLProxyPacket.class:?] at net.minecraft.network.NetworkManager.channelRead0(NetworkManager.java:156) [NetworkManager.class:?] at net.minecraft.network.NetworkManager.channelRead0(NetworkManager.java:51) [NetworkManager.class:?] at io.netty.channel.SimpleChannelInboundHandler.channelRead(SimpleChannelInboundHandler.java:105) [simpleChannelInboundHandler.class:4.0.23.Final] at io.netty.channel.AbstractChannelHandlerContext.invokeChannelRead(AbstractChannelHandlerContext.java:333) [AbstractChannelHandlerContext.class:4.0.23.Final] at io.netty.channel.AbstractChannelHandlerContext.fireChannelRead(AbstractChannelHandlerContext.java:319) [AbstractChannelHandlerContext.class:4.0.23.Final] at net.minecraftforge.fml.common.network.handshake.NetworkDispatcher.handleClientSideCustomPacket(NetworkDispatcher.java:412) [NetworkDispatcher.class:?] at net.minecraftforge.fml.common.network.handshake.NetworkDispatcher.channelRead0(NetworkDispatcher.java:278) [NetworkDispatcher.class:?] at net.minecraftforge.fml.common.network.handshake.NetworkDispatcher.channelRead0(NetworkDispatcher.java:73) [NetworkDispatcher.class:?] at io.netty.channel.SimpleChannelInboundHandler.channelRead(SimpleChannelInboundHandler.java:105) [simpleChannelInboundHandler.class:4.0.23.Final] at io.netty.channel.AbstractChannelHandlerContext.invokeChannelRead(AbstractChannelHandlerContext.java:333) [AbstractChannelHandlerContext.class:4.0.23.Final] at io.netty.channel.AbstractChannelHandlerContext.fireChannelRead(AbstractChannelHandlerContext.java:319) [AbstractChannelHandlerContext.class:4.0.23.Final] at io.netty.channel.DefaultChannelPipeline.fireChannelRead(DefaultChannelPipeline.java:787) [DefaultChannelPipeline.class:4.0.23.Final] at io.netty.channel.local.LocalChannel.finishPeerRead(LocalChannel.java:326) [LocalChannel.class:4.0.23.Final] at io.netty.channel.local.LocalChannel.access$400(LocalChannel.java:45) [LocalChannel.class:4.0.23.Final] at io.netty.channel.local.LocalChannel$5.run(LocalChannel.java:312) [LocalChannel$5.class:4.0.23.Final] at io.netty.channel.local.LocalEventLoop.run(LocalEventLoop.java:33) [LocalEventLoop.class:4.0.23.Final] at io.netty.util.concurrent.SingleThreadEventExecutor$2.run(SingleThreadEventExecutor.java:116) [singleThreadEventExecutor$2.class:4.0.23.Final] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_91] Thanks,
-
Go to the Item Class, more specifically Item#onItemRightClick Edit: To send a message, you could: Minecraft mc = Minecraft.getMinecraft(); mc.thePlayer.addChatMessage(new TextComponentTranslation("message.modid.item.desc")); (this will only be seen by player) or mc.thePlayer.sendChatMessage("x"); for all players to see it. when the item is right clicked. However, this needs to be translated via en_US.lang
-
Hey there, I'm having an issue with my bush that spawns in the world, it looks like a full cube, I want the effect that tall grass has when generated. I have tried what I did with the crops, which is set the default model to 'cross': { "parent": "block/cube_all", "defaults": { "model": "cross" }, "textures": { "all": "plentifulmisc:blocks/block_latex_plant" } } Thanks,
-
Nevermind, figured it out... Thanks.
-
Oh, where and how would I register it? Never used this system before.
-
Hey there, So I have this 'dust' Item here that uses IItemColor to get a color from a list so it a bit more flexible with other mods, and just easier / cleaner implementation. However, I have run into an issue: It wont display the colors. I have no clue what I'm doing wrong, so I'll go ahead and post here... So here is the item: public class ItemDust extends ItemBase implements IColorProvidingItem { public static final Dusts[] ALL_DUSTS = Dusts.values(); public ItemDust(String name){ super(name); this.setHasSubtypes(true); } @Override public int getMetadata(int damage){ return damage; } @Override public String getUnlocalizedName(ItemStack stack){ return stack.getItemDamage() >= ALL_DUSTS.length ? StringUtil.BUGGED_ITEM_NAME : this.getUnlocalizedName()+"_"+ALL_DUSTS[stack.getItemDamage()].name; } @Override public EnumRarity getRarity(ItemStack stack){ return stack.getItemDamage() >= ALL_DUSTS.length ? EnumRarity.COMMON : ALL_DUSTS[stack.getItemDamage()].rarity; } @Override @SideOnly(Side.CLIENT) public void getSubItems(Item item, CreativeTabs tab, NonNullList list){ for(int j = 0; j < ALL_DUSTS.length; j++){ list.add(new ItemStack(this, 1, j)); } } @Override protected void registerRendering(){ for(int i = 0; i < ALL_DUSTS.length; i++){ PlentifulMisc.proxy.addRenderRegister(new ItemStack(this, 1, i), this.getRegistryName(), "inventory"); } } @SideOnly(Side.CLIENT) @Override public IItemColor getColor(){ return new IItemColor(){ @Override public int getColorFromItemstack(ItemStack stack, int pass){ return stack.getItemDamage() >= ALL_DUSTS.length ? 0xFFFFFF : ALL_DUSTS[stack.getItemDamage()].color; } }; } } IColorProvidingItem (due to implementing the vanilla, the server cant load due to the class not being @SideOnly) public interface IColorProvidingItem{ @SideOnly(Side.CLIENT) IItemColor getColor(); } The Texture is White, so the color should be applied. Registration code is the same for most items. Thanks,
-
[1.11] SubItems stack onto each other when picked up.
Lambda replied to Lambda's topic in Modder Support
Yep, that seemed to fix it. Feel pretty dumb to forget that, Thanks! -
Actually, It seems that setting static values, doesnt scale with the screen. So maximizing =! minimizing.
-
Yeah, Just figured it out, the mc.displayHeight/Width, seemed to return null? So I set it to a static value and it displays now... Thanks regardless.
-
Ah, yeah I think so. However, it still wont render.
-
Hey there, So I have 4 types of 'gems', in the inventory, they cannot be stacked, however, when they are picked up or /give they look like the respective crystal, but they ended up stacking in the inventory. My explanation might be dull, so here are some captioned pictures of whats happening: Here is item class code: public class ItemMagicGems extends ItemBase { public static final Gems[] ALL_GEMS = Gems.values(); public Gems type; private final boolean isEmpowered; public ItemMagicGems(String name, boolean isEmpowered) { super(name); this.setFull3D(); this.isEmpowered = isEmpowered; } @Override public String getUnlocalizedName(ItemStack stack){ return stack.getItemDamage() >= ALL_GEMS.length ? StringUtil.BUGGED_ITEM_NAME : this.getUnlocalizedName()+"_"+ALL_GEMS[stack.getItemDamage()].name; } @Override public boolean hasEffect(ItemStack stack){ return this.isEmpowered; } @Override public EnumRarity getRarity(ItemStack stack){ return stack.getItemDamage() >= ALL_GEMS.length ? EnumRarity.COMMON : ALL_GEMS[stack.getItemDamage()].rarity; } @Override @SideOnly(Side.CLIENT) public void getSubItems(Item item, CreativeTabs tab, NonNullList list){ for(int j = 0; j < ALL_GEMS.length; j++){ list.add(new ItemStack(this, 1, j)); } } @Override protected void registerRendering(){ for(int i = 0; i < ALL_GEMS.length; i++){ String name = this.getRegistryName()+"_"+ALL_GEMS[i].name; PlentifulMisc.proxy.addRenderRegister(new ItemStack(this, 1, i), new ResourceLocation(name), "inventory"); } } } , here is how I'm registering them: item_gems = new ItemMagicGems("item_gem", false); Thanks