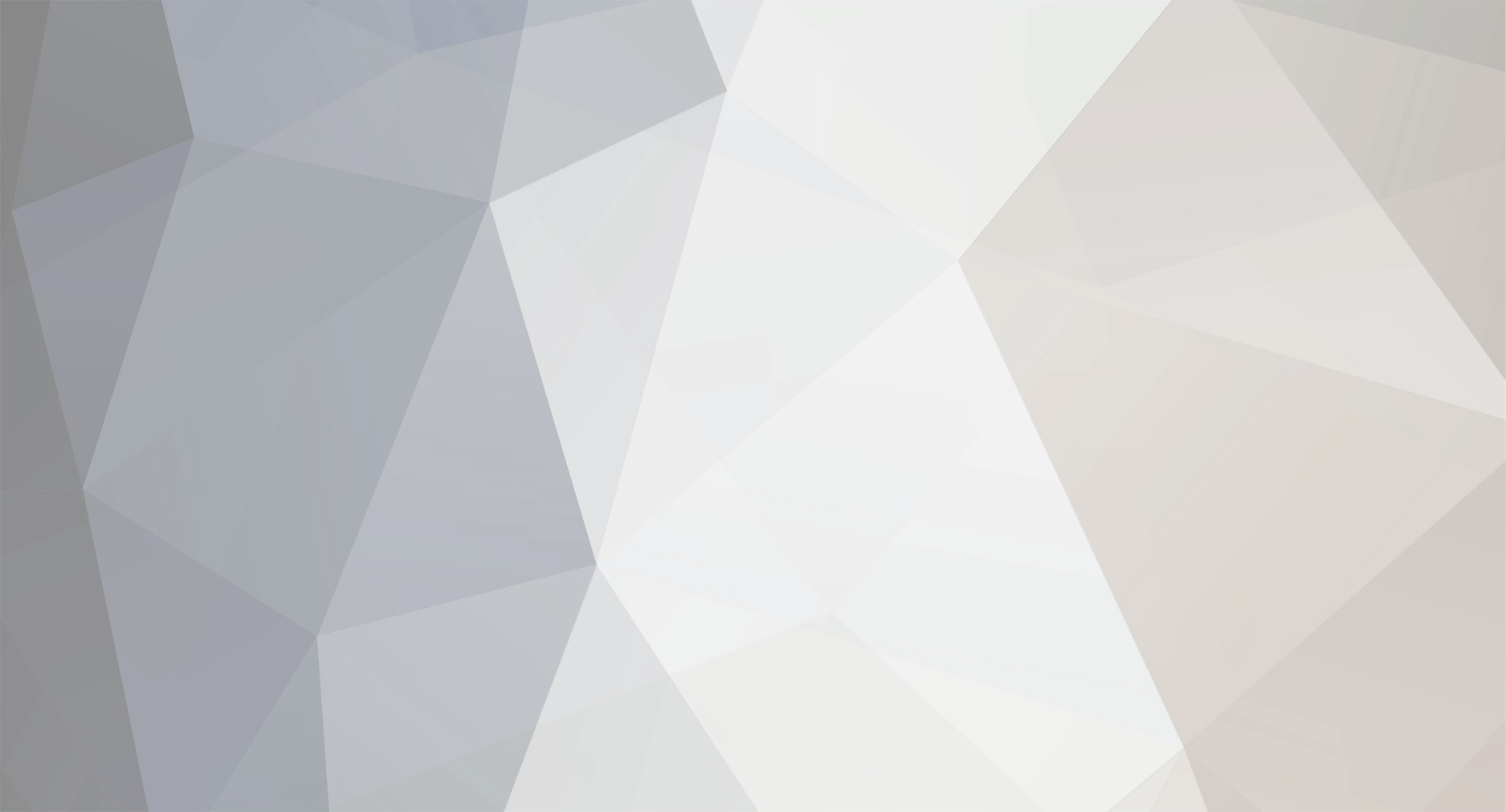
Lambda
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by Lambda
-
Okay, so how bout defining my output, current I feel like this isnt 'correct', maybe it is, but I dont think so. public void addDualRecipes(ItemStack input, ItemStack inputSecond, ItemStack output) { dualList.add(new InscriberWrapper(input, inputSecond, output)); } adds the recipes to the list public void addDualRecipe(Item inputFirst, Item inputSecond, Item output) { this.addDualRecipes(new ItemStack(inputFirst, 1), new ItemStack(inputSecond, 1), new ItemStack(output, 1)); } adds recipes to the recipes list. here is what the recipe looks like: this.addDualRecipe(Items.APPLE, Items.ARROW, Items.IRON_AXE); in my eyes, APPLE + ARROW = IRON_AXE however, I dont feel like this will work for some reason. Anyways, so what your saying with my getResult is that ill return the output? I just want it to be the output. So would I just do: return recipe.item3; //which is the output item and change the function to an ItemStack instead of a InscriberWrapper: public ItemStack getResult(ItemStack input, ItemStack inputSecond) { for(InscriberWrapper recipe : dualList) { if(ItemUtil.areItemsEqual(recipe.item1, input, true) && ItemUtil.areItemsEqual(recipe.item2, inputSecond, true)) { return recipe.item3; } } return null; }
-
Okay, would I have to do anything different to this function: public InscriberWrapper getResult(ItemStack input, ItemStack inputSecond) { for(InscriberWrapper recipe : dualList) { if(ItemUtil.areItemsEqual(recipe.item1, input, true) && ItemUtil.areItemsEqual(recipe.item2, inputSecond, true)) { return recipe; } } return null; }
-
So how would I even get the output?
-
Yes Darco is right, I only need a 2 stack obj. Anyways, with these functions: public void addDualRecipes(Item inputFirst, Item inputSecond) { this.addDualRecipes(new ItemStack(inputFirst, 1), new ItemStack(inputSecond, 1)); } public void addDualRecipes(ItemStack input, ItemStack inputSecond) { dualList.add(new InscriberWrapper(input, inputSecond)); } public InscriberWrapper getResult(ItemStack input, ItemStack inputSecond) { for(InscriberWrapper recipe : dualList) { if(ItemUtil.areItemsEqual(recipe.item1, input, true) && ItemUtil.areItemsEqual(recipe.item2, inputSecond, true)) { return recipe; } } return null; } where would I calculate the output?
-
public void addDualRecipes(ItemStack input, ItemStack inputSecond, ItemStack output) { dualList.add(new InscriberWrapper(input, inputSecond, output)); } Ah! So that should work now! Is everything else 'ok', because all I have to do now is integrate it into my TE. Note: Should I remove the output ItemStack?
-
public void addDualRecipes(InscriberWrapper input, InscriberWrapper inputSecond) { dualList.add(input); dualList.add(inputSecond); } So this then :? and Choonster: (Quotes got screwed up) Yeah, I updated them.. saw that after I posted it and forgot to edit and Yes, I'm using an ArrayList to store my ItemStacks now. So I would not need the HashMap key correct? here is the entire class for reference:
-
however, dualList.add(//adding an itemstack or ....
-
Okay futher progress: getResult has been finished I belive: public InscriberWrapper getResult(ItemStack input, ItemStack inputSecond) { for(InscriberWrapper recipe : dualList) { if(ItemUtil.areItemsEqual(recipe.item1, stack, true) && ItemUtil.areItemsEqual(recipe.item2, inputSecond, true)) { return recipe; } } return null; } however, addDualListRecipes is confusing me a bit, how would I 'put' ItemStacks into the ArrayList public void addDualRecipes(ItemStack input, ItemStack inputSecond, ItemStack output) { }
-
Okay I belive these are correct now.. So would I just iterate like I do with regular lists, and get the result? Or is there something else?
-
here is the hash my IDE created. @Override public int hashCode() { int result = item1 != null ? item1.hashCode() : 0; result = 31 * result + (item2 != null ? item2.hashCode() : 0); result = 31 * result + (item3 != null ? item3.hashCode() : 0); return result; } Aswell, here is the updated equals: return ItemUtil.areItemsEqual(other.item1, this.item2, true) && ItemUtil.areItemsEqual(other.item2, this.item2, true) && ItemUtil.areItemsEqual(other.item1, this.item3, true); areItemsEqual: public static boolean areItemsEqual(ItemStack stack1, ItemStack stack2, boolean checkWildcard){ return StackUtil.isValid(stack1) && StackUtil.isValid(stack2) && (stack1.isItemEqual(stack2) || (checkWildcard && stack1.getItem() == stack2.getItem() && (stack1.getItemDamage() == UtilBase.WILDCARD || stack2.getItemDamage() == UtilBase.WILDCARD))); }
-
player.addVelocity(x, y, z) or player.setVelocity
-
Oh, well thats exactly what I dont want... So is this how'd I do it: other.item1.getItem() == this.item1.getItem();
-
Yeah I somewhat knew that when writing, so I'm guessing just compare the metadatas? or is there a more effient way.
-
Your wording is a bit hard to understand, So I'm guessing this is happening: So it renders when one of the Checks are true, but on reconnected they disappear until an update? I think you have to update the TE somehow.
-
KeyBindings can be referred to here: If there is any issues with this, then post them here. This tutorial is a bit outdated but should still hold the same concept
-
Okay, how bout the hashcode? or would I not need that due to using an ArrayList instead? return other.item1 == this.item1 && (other.item1.getMetadata() == -1 || this.item1.getMetadata() == -1 || other.item1.getMetadata() == this.item1.getMetadata()) && other.item2 == this.item2 &&(other.item2.getMetadata() == -1 || this.item2.getMetadata() == -1 || other.item2.getMetadata() == this.item2.getMetadata()) && other.item3 == this.item3 &&(other.item3.getMetadata() == -1 || this.item3.getMetadata() == -1 || other.item3.getMetadata() == this.item3.getMetadata()); This is what equals returns now.
-
Probably would need to create a key event and add to the players velocity when the event is triggered and use: this.extractEnergyInternal(item, amount, false); (if your using CoFH api) to extract energy when pressed.
-
How would I handle the hashcode + equals functions? This should work right?: private List<InscriberWrapper> dualList = new ArrayList<InscriberWrapper>();
-
Okay, I'm having 'good' progress so far: I now have the wrapper class that will hopefully will handle the ItemStacks. package com.lambda.plentifulmisc.recipe; import net.minecraft.item.ItemStack; /** * Created by Blake on 11/29/2016. */ public class InscriberWrapper { public final ItemStack item; public InscriberWrapper(ItemStack first, ItemStack i) { item = i; } @Override public int hashCode() { return 37*item.getItem().hashCode() + item.getMetadata(); } @Override public boolean equals(Object o) { if(o instanceof InscriberWrapper) { InscriberWrapper other = (InscriberWrapper)o; return other.item == this.item && (other.item.getMetadata() == -1 || this.item.getMetadata() == -1 || other.item.getMetadata() == this.item.getMetadata()); } return false; } } Also, I have some progress in the recipes, but here is where I'm stuck, comparing the Items: public void getResult(ItemStack stack) { for(Map.Entry<List<InscriberWrapper>, ItemStack> entry : this.dualList.entrySet()) { if(ItemUtil.areItemsEqual(stack, entry.getKey()) { // Here, I know why, but I dont know how'd I do this } } } ItemUtil#areItemsEqual method is a simple comparison using the item &metadata. Here is the Hash: private Map<List<InscriberWrapper>, ItemStack> dualList = new HashMap();
-
Hello, So I recently started rewrite the Dual Input frunace from previous post. My issue is however, is creating a map that can store all 3 ItemStacks.. I know I have 2 options: - A Wrapper - Itteration However, I dont really know how to create a Wrapper and apply it to the class, and I dont know how to iterate through the ItemStacks. Any tips?
-
Hm, I'm not really able to lauch the game w/ breakpoints, it took 2 hours to load, and cant even click play..
-
Okay it half-works, Upon reboot, it seems to lose the connection data, if you just reload the world it doesnt.
-
Just got into Intellj, would you know how to add breakpoints, google search shows ALT+INS but that isnt working
-
Where would I start? I probably should do some kind of rewrite anyways, it seems a bit messy
-
public static boolean areItemsEqual(ItemStack stack1, ItemStack stack2, boolean checkWildcard){ return StackUtil.isValid(stack1) && StackUtil.isValid(stack2) && (stack1.isItemEqual(stack2) || (checkWildcard && stack1.getItem() == stack2.getItem() && (stack1.getItemDamage() == UtilBase.WILDCARD || stack2.getItemDamage() == UtilBase.WILDCARD))); }