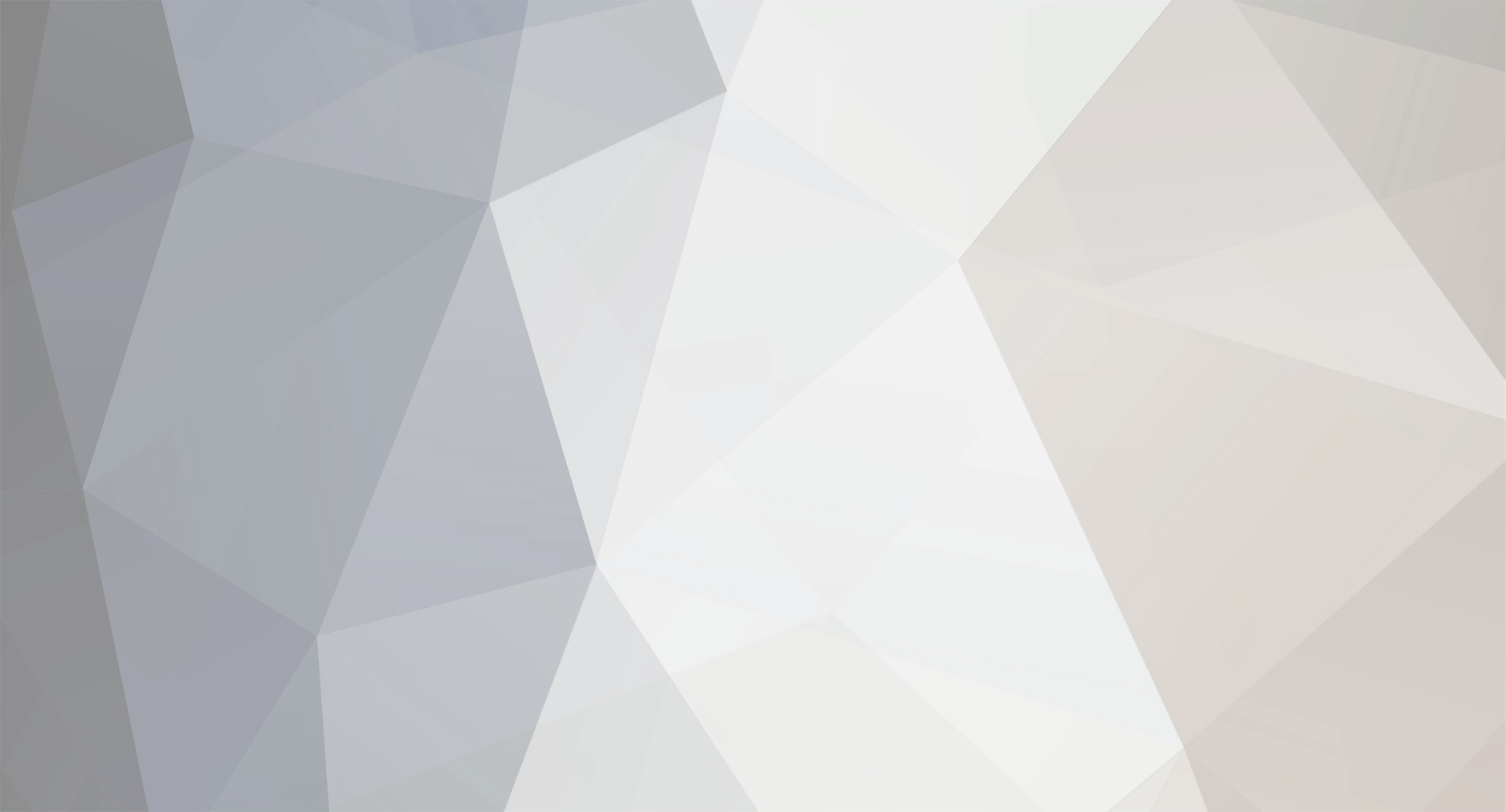
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
As PPD said, your render type needs to be cutout For example see here https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe03_block_variants/StartupClientOnly.java and some more background info here https://greyminecraftcoder.blogspot.com/2020/04/block-rendering-1144.html -TGG
-
[1.61.1] Missing addPropertyOverride function
TheGreyGhost replied to ViktorNguyen's topic in Modder Support
There's working tutorial example here, in case you are still having trouble https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe11_item_variants -TGG -
[1.14.4] Capability NBT for an ItemStack not loading
TheGreyGhost replied to InterdimensionalCat's topic in Modder Support
Howdy Your code looks right on a quick read through Try putting a breakpoint into both ItemStack:: private void forgeInit() { and ItemStack::deserializeNBT That should show you whether the capabilities are being constructed, serialised and deserialised properly. I have a working tutorial example here https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe32_inventory_item But I don't see any significant difference between yours and mine. -TGG -
[1.15.2] Drinking Particles and Sounds Never Finish (SOLVED)
TheGreyGhost replied to Warven22's topic in Modder Support
Howdy You might need to override all of the relevant methods, if the default doesn't match your item properties. For example // what animation to use when the player holds the "use" button @Override public UseAction getUseAction(ItemStack stack) { return UseAction.DRINK; } // how long the drinking will last for, in ticks (1 tick = 1/20 second) @Override public int getUseDuration(ItemStack stack) { final int TICKS_PER_SECOND = 20; final int DRINK_DURATION_SECONDS = 2; return DRINK_DURATION_SECONDS * TICKS_PER_SECOND; } // you could also consider implementing these two methods just to add breakpoints (or prints to the debug log) and see what is going on // called when the player starts holding right click; // --> start drinking the liquid (if the bottle isn't already empty) @Override public ActionResult<ItemStack> onItemRightClick(World worldIn, PlayerEntity playerIn, Hand hand) { ItemStack itemStackHeld = playerIn.getHeldItem(hand); EnumBottleFullness fullness = getFullness(itemStackHeld); if (fullness == EnumBottleFullness.EMPTY) return new ActionResult(ActionResultType.FAIL, itemStackHeld); playerIn.setActiveHand(hand); return new ActionResult(ActionResultType.PASS, itemStackHeld); } // called when the player has held down right button for the full item use duration // --> decrease the bottle fullness by one step @Override public ItemStack onItemUseFinish(ItemStack stack, World worldIn, LivingEntity entityLiving) { EnumBottleFullness fullness = getFullness(stack); fullness = fullness.decreaseFullnessByOneStep(); fullness.putIntoNBT(stack.getTag(), NBT_TAG_NAME_FULLNESS); return stack; } A breakpoint in LivingEntity::updateActiveHand might also shed some light on what's happening -TGG -
Making a bag item without a tile entity
TheGreyGhost replied to YaBoiChips's topic in Modder Support
Here's a working example of an Item which is also a container https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe32_inventory_item Cheers TGG -
[1.16.1] Trouble with displaying particles
TheGreyGhost replied to Tavi007's topic in Modder Support
Howdy I haven't played with 1.16 yet, but the best way to troubleshoot this is to set a breakpoint in your particle spawn, then trace into the vanilla code and see what it does after that. You might find that your spawn call is just ignored (because you're calling the wrong method), or that your particle type is not registered correctly, or the packet is never sent, or that when the packet is received it is ignored for some reason, or that your particle is actually rendering but is invisible or in a different space than you think. -TGG -
Howdy the block renderer uses the BlockPos to check the adjacent blocks to see if any are blocking out the faces eg if there is a full solid block to the EAST of your block, then the renderer doesn't draw the EAST face. You appear to use the same dummy BlockPos for both blocks, so if the EAST face is hidden for that blockpos (because there is a solid block to the EAST), then the EAST face is always hidden, regardless of where you have told the renderer to draw your block by translating the render. -TGG
-
Well my basic thought is something like public interface ICapabilitySerializable<T extends INBT> extends ICapabilityProvider, INBTSerializable<T> { default T serializeNBTforPacketToClient() {return null;} } CapabilityProvider:: protected final @Nullable CompoundNBT serializeCapsForPacketToClient() { final CapabilityDispatcher disp = getCapabilities(); if (disp != null) { return disp.serializeNBTforPacketToClient(); } return null; } IForgeItem:: default CompoundNBT getShareTag(ItemStack stack) { CompoundNBT cNBTbase = stack.getTag(); CompoundNBT cNBTcaps = this.serializeCapsForPacketToClient(); if (cNBTcaps != null && !cNBTcaps.isEmpty()) { cNBTbase.put("ForgeCaps", cNBTcaps); } } Seems simple enough. A lambda that the capability can use to inform the ItemStack (or Entity, World, etc) that it is dirty would also be useful.
-
hmm ok that would explain it. I'll do a bit more testing to figure out the best way to work around that (i.e. other than custom packets) for ItemStacks as well as Entity and the others. Do you think that the Forge overlords would support the addition of a "transmitToClient" flag for capabilities? Do you know who designed it / is maintaining it? It appears to be Lex. I'm more than happy to code it up and submit a pull request but I'm not so keen to waste a few days doing it only to have it rejected out of hand. -TGG
-
Creating a new type of ItemCameraTransforms/TransformType
TheGreyGhost replied to poopoodice's topic in Modder Support
Howdy Short answer is - you can use either one, your choice! handlePerspective is more flexible, but also more complicated. -TGG -
Hi Where is your StructurePiece create() method called from? For events and pretty much any other method you can attach your code to, there is nearly always an object available which has the relevant world in it. -TGG
-
[Solved] [1.15.2] Rendering fixed lines between tile entities
TheGreyGhost replied to profjb58's topic in Modder Support
Howdy I would suggest that you should use the TER to render your lines. Since your TileEntity stores the position of the next marker, just draw a line to the next position (relative to the position of the TE being drawn, of course). Very simple, very scaleable. renderworldlastevent should be a last resort I think. The only likely issue is that lines will disappear when the TE is unloaded (goes out of chunk range), which may become very noticeable if the markers are far apart from each other, i.e. you are close to one of the markers when the next marker goes off the edge of the chunk limit. You could probably work around this by drawing to both the previous and the next markers; if necessary keeping track of which lines have already been drawn during that frame so that you don't draw the same line twice. -TGG -
[1.14.4] Saving information for each player
TheGreyGhost replied to kaiser_'s topic in Modder Support
Howdy Here are some working examples of capabilities https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe65_capability and how to convert lists etc into NBT for permanent storage https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe20_tileentity_data/TileEntityData.java -TGG -
How to remove X-Ray Texture from custom model black?
TheGreyGhost replied to Help!'s topic in Modder Support
BTW: Some more information on VoxelShapes https://greyminecraftcoder.blogspot.com/2020/02/block-shapes-voxelshapes-1144.html -
Hi The names change a lot because they're not the official mojang names. Each version of minecraft has the names of all its methods, variables, classes scrambled (obfuscated). The Forge guys (well, MCP actually) decompile the minecraft code, and then the community assign names for the scrambled methods, variables, and classes. If the method signature hasn't changed, the name usually stays the same, but if it has changed then it relies upon someone in the community assigning a reasonable new name. Quite often the names are misleading. Sometimes the names change because different people have different opinions about what good names should look like. In my experience, the changing of the names is usually not a big problem- the thing which causes you many hours of frustrating rework is the refactoring of the game features and classes, because your code breaks in fundamental ways and large parts of it need to be rewritten almost from scratch. Some parts of Forge don't change much (the events, for example). But anything that is tightly integrated with vanilla is likely to change a lot. Cheers TGG
-
Howdy DieSieben Are you sure? I don't think that's right. I wrote a tutorial example which attaches a capability to an ItemStack, changing the rendering on the client based on the capability, and it worked fine. When I look at the Forge code it appears to send the caps nbt as well as the vanilla nbt. /** * Write the stack fields to a NBT object. Return the new NBT object. */ public CompoundNBT write(CompoundNBT nbt) { ResourceLocation resourcelocation = Registry.ITEM.getKey(this.getItem()); nbt.putString("id", resourcelocation == null ? "minecraft:air" : resourcelocation.toString()); nbt.putByte("Count", (byte)this.count); if (this.tag != null) { nbt.put("tag", this.tag.copy()); } CompoundNBT cnbt = this.serializeCaps(); if (cnbt != null && !cnbt.isEmpty()) { nbt.put("ForgeCaps", cnbt); } return nbt; } The one thing I did have to do was mark the ItemStack as dirty (after updating the capability) in order to force it to be resynched to the client: CompoundNBT nbt = itemStackBeingHeld.getOrCreateTag(); int dirtyCounter = nbt.getInt("dirtyCounter"); nbt.putInt("dirtyCounter", dirtyCounter + 1); itemStackBeingHeld.setTag(nbt); (dirtyCounter is an arbitrary nbt tag) -TGG
-
Hi The short answer is - Forge isn't really an API at all in the sense that you might expect. It does have some API-like aspects but if you're looking for documentation similar to the Guava library or the java util library, then you're not going to find it. Some of the Forge code is documented well, and some of it is not documented at all. Sometimes you can tell how the methods are intended to be used by reading the code, other times that is almost impossible to figure out. The best way I have found to learn it is to google for tutorials and to look at good existing mods. That can be difficult because there are a lot of not-very-good tutorials around. -TGG
-
Howdy You could consider implementing a custom BakedModel and overriding @Override public ItemCameraTransforms getItemCameraTransforms() { return parentModel.getItemCameraTransforms(); } There is an example of a custom BakedModel for items here, if you are interested https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe15_item_dynamic_item_model -TGG
- 1 reply
-
- 1
-
-
Hi I don't know the answer, but you can find out fairly easily yourself by putting a breakpoint into your LivingAttackEvent, getting another player to damage you, and then tracing back up the call stack to see why the damage is zero. -TGG
-
When your client joins to a multiplayer server, the FMLServerStartingEvent doesn't run on the client because it's not running a server. For some background info, see here http://greyminecraftcoder.blogspot.com/2020/02/the-client-server-division-1144.html Just to make sure - do you realise that the multiplayer server will need to be running your mod as well as the client that you're using to connect to it? You can create client-only mods but they are very limited in what they can do. -TGG
-
[1.15.2] Need help with an API provided by a mod
TheGreyGhost replied to Quizer9O8's topic in Modder Support
Howdy I haven't tried this since 1.12.2 but you might find useful clues in this mod, which uses several other mods as dependencies (see its build.gradle) https://github.com/Vazkii/Botania https://github.com/Vazkii/Botania/blob/master/build.gradle -TGG