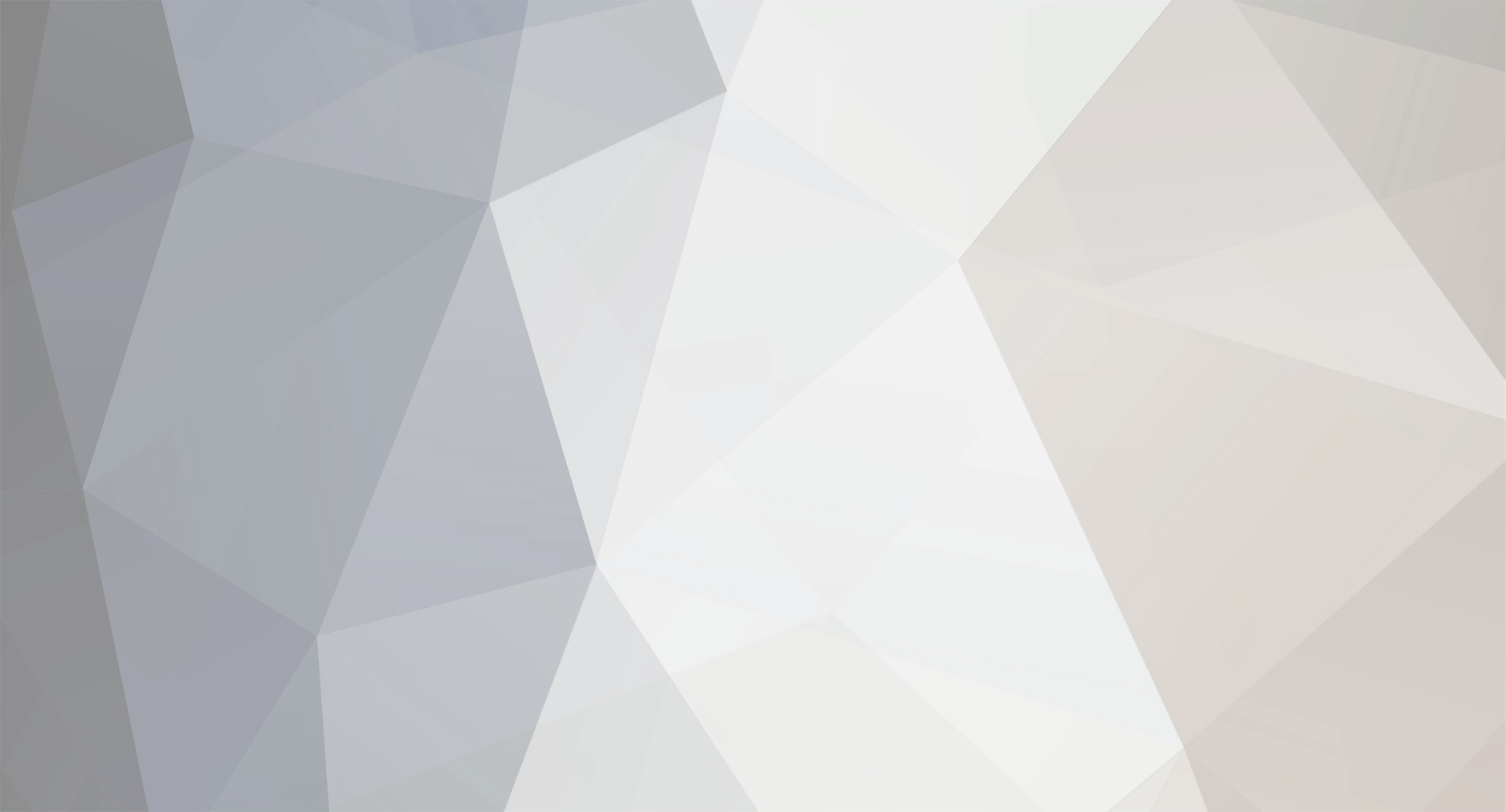
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Minecraft.getMinecraft().thePlayer.worldObj That always returns the CLIENT, what you want is the SERVER, since you sent the packet to the server, right? -
[SOLVED]Rendering Block (that has a custom model) In Inventory
coolAlias replied to TLHPoE's topic in Modder Support
Your code looks so innocuous... I don't really have any idea, but I wonder if it has to do with your render class both extending TileEntitySpecialRenderer AND implementing ISimpleBlockRenderingHandler. Have you tried using just one or the other? -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Do you see in your Container class that you store the TileEntity in "protected TileEntityReplacer tileEntity;" ? Do the exact same thing in your Gui, then when you need to send the packet you have the tile entity you need, so you can use te.xCoord, te.zCoord, etc. as parameters in your packet constructor, or pass it directly: public YourPacket(TileEntity te, int itemID) { this.x = te.xCoord; this.y = te.yCoord; this.z = te.zCoord; this.item = itemID; } Since you don't have a custom packet class though, you would just write those values directly to the output stream when creating your custom 250 payload packet. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
You pass the x/y/z coordinates to your GuiHandler when you open the Gui, don't you? Use those to get the TileEntity and pass it in to the Gui when you open it. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
public TileEntityReplacer tile = new TileEntityReplacer(); Why are you creating a new tile entity in your gui??? A tile entity is supposed to be attached to a block in the actual world, you can't just create one in the middle of a gui or anywhere else really and expect it to do anything. Where are you opening this gui from? Is it opened from a block? Does that block have a tile entity? You need to use that tile entity if so, by passing the tile entity in to your gui when you open it. If your gui is not opened from a block, then I don't know how you expect to be setting any tile entity's data from it, packet or not. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
You can use Packet250CustomPayload to do exactly the same thing, I was just providing an example of writing more than a single integer. Wherever you write the packet you will have to write all that data, and then read it all back in the same order when you receive the packet. Yes, you can use the regular tile entity / IInventory methods when you receive the packet. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
You can write as much information as you need in a packet. See here for an example using a tile entity and some other data. As for the items, no, don't rely on the ID that you think it's using, because those can be changed via config or other things. Just reference your item and get the ID that way, i.e. YourModItems.someItem.itemID, and sent that value in the packet. This way you will never be wrong. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Yeah no. You can't just set the inventory contents of a random tile inventory, you have to pass the coordinates of the TileEntity for which you want to set the contents, and get that exact TileEntity from the world. And is there any particular reason you're adding 4000 to the integer you send? That doesn't make any sense to me... why not just send the id of the item you want? Are you sure that 4000+whatever random number you are sending is really an item? -
[1.7.2] Localization Trouble with .name
coolAlias replied to Thecheatgamer1's topic in Modder Support
... setBlockName... if you had just typed your block and then period, you would have seen it in the method list that shows up. -
[1.7.2] Localization Trouble with .name
coolAlias replied to Thecheatgamer1's topic in Modder Support
Register your blocks using the block.getUnlocalizedName() rather than predefined names. It will prepend tile. and append .name automatically for you, and you just need to have that in your language file: Block block = new Block().setUnlocalizedName("block"); GameRegistry.registerBlock(block, block.getUnlocalizedName()); tile.block.name=Some Block -
[SOLVED]Rendering Block (that has a custom model) In Inventory
coolAlias replied to TLHPoE's topic in Modder Support
Oh yeah, that's pretty funky! Lots of rendering stuff seems trickier in 1.7.2. Anyway, it might have something to do with the final openGL calls you make, the one for setting the color when you render the tile entity, and another that enables GL_RESCALE_NORMAL in your render inventory block method. Try commenting out one or the other or both and see if that helps at all. If it doesn't, then the problem might be something going on in your super class. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
You make an ItemStack the exact same way regardless of what side you are on; it's just a matter of you sending the information you need to create the stack you want, which is going to be the same information you would have used to create the ItemStack on the client gui in the first place. If you are creating different stacks based on the button pressed, for example, just send the button id to the server and handle it there instead. Though you can send an entire ItemStack over the server quite easily by first writing it to NBT and then using CompressedStreamTools to send and receive the NBT tag compound. -
[SOLVED]Rendering Block (that has a custom model) In Inventory
coolAlias replied to TLHPoE's topic in Modder Support
You just have to play with the glTranslatef values until you get it in the right spot. // here, I typically just use x, y, and z GL11.glTranslatef((float) x, (float) y + 1.0F, (float) z + 1.0F); GL11.glScalef(1.0F, -1.0F, -1.0F); // and do my messing around here: GL11.glTranslatef(0.5F, 0.5F, 0.5F); Obviously, do whatever works for you. -
[1.7.2] How to render TileEntity in inventory?
coolAlias replied to Eternaldoom's topic in Modder Support
Alright, this is what I use to render a block with a custom model both in the world and in the inventory. It's probably not the most efficient way, because I use both a TESR and an ISBRH: GameRegistry.registerBlock(ceramicJar, ItemCeramicJar.class, ceramicJar.getUnlocalizedName()); ClientRegistry.bindTileEntitySpecialRenderer(TileEntityCeramicJar.class, new RenderTileEntityCeramicJar()); RenderingRegistry.registerBlockHandler(new RenderCeramicJar()); The TESR renders the model in the world, and the ISBRH just calls a dummy TESR to render the model in the inventory. You can see the code here. -
If you use an attribute modifier, you will have to be sure to remove it once the player is out of the water. A much simpler method is to just multiply the player's current motion by a factor: float f = whatever value you want, maybe 2.0F; player.motionX *= f; player.motionZ *= f; You can multiply the Y motion as well, but generally x and z are what you want.
-
[1.7.2] How to render TileEntity in inventory?
coolAlias replied to Eternaldoom's topic in Modder Support
Draco already gave you a link to his code where he does exactly what you are asking... can you at least take some time to look at it thoroughly? -
Also, there is no longer any LanguageRegistry, so I'm not sure what you're doing with "Items.addNames()". Are you registering your Items to the GameRegistry (before you add the recipes)? GameRegistry.registerItem(yourItem, yourItem.getUnlocalizedName().substring(5)); // you probably don't need substring(5) - mine is just left over from other things
-
at Lord_of_Block.armor.Armor.addArmorCrafting(Armor.java:34) ~[Armor.class:?] at Lord_of_Block.base.main.Init(main.java:34) ~[main.class:?] It shows exactly what I said above: your item is null when you try to add the recipe, because you haven't initialized it yet.
-
What's the error? Are your items finished initializing at this point? If not, that would be the cause of your crash.
-
That's what I thought as well, but you know, it was quite a long time ago when I set up that account, and to be honest I don't recall if I ever got it working back then either. I thought I had, but now I'm not so certain, as I recall it being quite difficult to set up. I realize that makes me look like an idiot, I mean come on, who can't set up an online account these days... but man, I've never encountered a service that is that freaking difficult to use before. I set up an account, log in and there seem to be all sorts of other account 'options', and later on I can't use the account anymore because it's not set up for whatever that damn on Demand crap is, and I sure as hell am not signing up for that because it sounds like a paid 'service'. I was definitely able to at least log in before, more than a year ago, but now I can't even do that. Anyway, I've wasted a lot of time messing around with the damn thing since its inception, and I've just had enough of it, in addition to my original point that I think it's absurd to require an account, especially one separate from the one we already use to play the game, just to report a bug. Sorry, but the whole situation has been grating on my nerves for some time, and it's been exceedingly frustrating to use (or not, since I haven't been successful since I first set up the account).
-
Not lazy, it's just one of those things that I can't stand for some illogical reason. I realize it's stupid, but it still irks me to no end that I have to create an account to post a bug report. I actually did create an account for the original Atlassian bug tracker, but somehow that's gotten all f-ed up and I can't use it anymore due to some onDemand gibberish, and I really don't feel like making another or wasting any more time than I already have on it, when they could just as easily link it to our Minecraft accounts. I don't see how they expect people to report bugs when they make it such a damn hassle to do so, but perhaps I'm the only one that feels that way.
-
[1.6.4] Digging particle fx AND destroy particle effects
coolAlias replied to cyberpwn's topic in Modder Support
The block breaking particles are called "tilecrack_" in 1.6.x, and you must append the id and metadata value: String particle = "tilecrack_" + event.block.blockID + "_" + worldObj.getBlockMetadata(event.x, event.y, event.z); Then just spawn that particle normally. You can look through vanilla code to find where those particles are spawned and copy how that's done, or write your own algorithm to get them spawning in the way that you want.