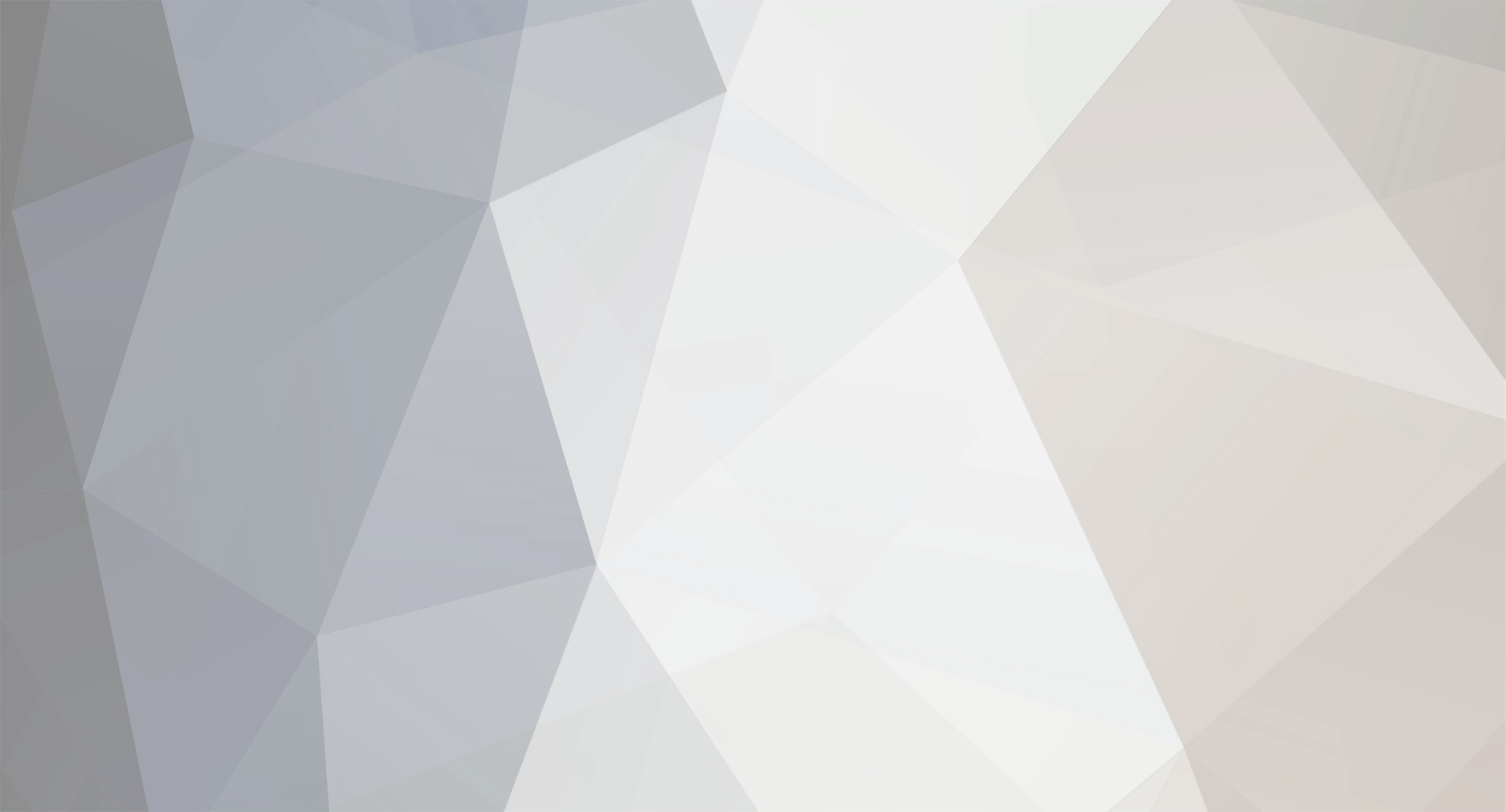
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
If you are coding your own mob, you can use attackTime as a built-in timer. It decrements automatically each update tick (from EntityLivingBase), so when the mob attacks, set this.attackTime = 40; and check if (attackTime == 0) before you allow the mob to attack.
-
[1.7.2]Unreported exception thrown in DataWatcher
coolAlias replied to DuskFall's topic in Modder Support
Yes, show us your packet class. Depending on the maximum size of your integers, you could store them as bytes and write it directly to the stream using buffer.writeBytes(byte[]), or you could convert your integers to a byte array instead. What about your packet class isn't working? -
[1.7.2]Unreported exception thrown in DataWatcher
coolAlias replied to DuskFall's topic in Modder Support
Yes, this tutorial was very handy in getting me going: it provides everything you need including the entire framework for handling packets, all you have to do is add your own packets and then use the provided structure to send them. Everything is handled directly in the packets. You can see some examples of it in action here. -
See my answer here for an explanation.
-
The Gui Overlay tutorial from the wiki is still highly useful, regardless of Minecraft version, though a few minor things (names) have changed since it was written. For IExtendedEntityProperties, either check the Forge wiki tutorial on that subject (can't seem to find it...diesieben's linked it a couple times, I'll change this when I find it) or check mine here. Either one should be plenty to get you started.
-
I haven't downloaded your source (please use Pastebin, an online repository, or some other way to let us view your source, as I doubt most will be willing to download), but here are a few suggestions: 1. If your entity is a mob, are you on peaceful? They will automatically despawn on peaceful, making them "disappear" 2. If you are not using a spawn egg, make sure you are spawning your mob on the server side only, like so: if (!world.isRemote) { world.spawnEntityInWorld(yourEntity); } If neither of those work, please post your code so we can see it, starting with your custom Entity class and the method you use to spawn it.
-
[1.6.4] [Solved] How to check if an entity is moving?
coolAlias replied to SackCastellon's topic in Modder Support
That's right, though motionY can be a bit odd sometimes, so you can also check entity.onGround if you really care about the vertical motion. If you need the entity's velocity, you can use the Pythagorean formula Math.sqrt(x * x + y * y + z * z) to get the velocity, including or leaving out y depending on your needs. -
[1.7.2] NBTTagCompound.getTagList() not working
coolAlias replied to chbachman's topic in Modder Support
Yes, you are, unless you are storing a list of NBTTagLists, you should not be using Constants.NBT.TAG_LIST. Use whatever type of NBTBase is stored in your list, for example when storing a list of NBTTagCompound such as found in inventories: public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); NBTTagList items = compound.getTagList("Items", Constants.NBT.TAG_COMPOUND); for (int i = 0; i < items.tagCount(); ++i) { NBTTagCompound item = (NBTTagCompound) items.getCompoundTagAt(i); // etc. } } -
This line in your packet is unnecessary; tile entities store the data between world saves and save automatically when the world does. You should never need to call writeToNBT for a tile entity, and certainly not with a new tag compound (unless you are sending it with getDescriptionPacket). ((TileEntityComputer) te).writeToNBT(new NBTTagCompound()); Anyway, do the debugging statements in your packet class all print the values you expect them to? When you pass in your TileEntity to the gui, are you sure it is the correct one? (world.getTileEntity(x, y, z)) When you open your gui, are you passing the correct x/y/z coordinates, i.e. using the Block / TileEntity coordinates and not the player's?
-
You're going to want to write your own gen code rather than using WorldGenMinable, especially since WorldGenMinable is not very optimized for use with large veins. Not only that, but keep in mind that Minecraft's generation code is handled chunk by chunk, and a chunk is only 16x16 blocks in area. If you try to create a vein of 1024 using WorldGenMinable, your gen code will find itself trying to access coordinates in a chunk that is not yet loaded. When that happens, the new chunk will be forced to load, calling your ore generation for that chunk as well, which then forces yet more chunks to load, and so on until your game crashes because it's caught in an infinite loop.
-
onArmorTickUpdate was renamed to onArmorTick. Seriously people, use the @Override annotation. It's one simple line for a whole lot of error protection. Also, the ticking armor is only called when you are wearing the item, and it's called for that item, so you don't need to getCurrentArmor or null check unless you are checking slots other than the one ticking. You can even do this: @Override public void onArmorTick(World world, EntityPlayer player, ItemStack stack) { if (this == armorMod.Flippers) { // do stuff } } That's the beauty of non-static class methods - they can only be called with an actual object of that class, so you are always safe from null and can use "this", because the Item in question is the one calling the method.
-
tag.setString(ConsoleLogLine1, "ConsoleLogLine1"); tag.setString(ConsoleLogLine2, "ConsoleLogLine2"); tag.setString(ConsoleLogLine3, "ConsoleLogLine3"); tag.setString(ConsoleLogLine4, "ConsoleLogLine4"); tag.setString(ConsoleLogLine5, "ConsoleLogLine5"); } Looks to me like you've got that backwards... NBT set methods take the key first, and the value second.
-
[1.6.4] RenderItemIntoGUI - Blocks appear dark
coolAlias replied to Kremnari's topic in Modder Support
You're just going to have to dig around in the vanilla code until you find what's causing it. I had a similar issue which you can see here. Hope that helps! -
[1.7.2] Make player "bounce" when they touch block?
coolAlias replied to Eternaldoom's topic in Modder Support
^^ Exactly. This is why you should ALWAYS put in the @Override annotation when using vanilla methods, especially if you are not familiar with the code. It will error-check for you and let you know when you are using the incorrect method signature. Also, if you want your player to bounce only when jumping on the trampoline, you might want to consider using the Block.onFallenUpon method instead. -
[1.6.4] [Solved] How to check if an entity is moving?
coolAlias replied to SackCastellon's topic in Modder Support
Use entity.motionX, motionY, and motionZ. -
GotoLink already pointed out your problem - you say you are "doing that", but if you are reading and writing from NBT properly, on the server side, the gui will have all of the Strings that you saved the next time you open it. The fact that it doesn't mean there is something wrong either with the way you are "writing" strings to the NBT from your gui, with your packet class, or with the tile entity you are using to store the NBT, if any. Without seeing the code, there is no way for us to pinpoint your error, so if you'd like help, post your Gui, Packet, and TileEntity.
-
(unsolved)(1.7.2) Manually Creating Mob Models
coolAlias replied to Sanocon's topic in Modder Support
Oh, TGG, thank you, but I stole that code from the Squid I actually have no idea what I'm doing when it comes to models / rendering, so you may be better off doing what I did and poking around in the vanilla code, as those do just about everything you could possibly want to do. Hm, now that I think about it, the new Octorok model in the code was submitted to me by a user, created in Techne, but I cleaned it up quite a bit since the java output from Techne can be horrendous. Originally I used the squid, though, and that's what I used as a comparison whilst cleaning up the code. I have my mod updated to 1.7.2 as well, just haven't gotten around to putting it on Github yet. Whenever I do, you're more than welcome to browse, if you think it will help you more than the vanilla code that is -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
You're welcome, and dear god no. Comparing with the == operator against a new ItemStack will always return false, because you are comparing identity as in the memory location, but how can a reference to an old location be identical to a new location? Get the stack like you were, using ItemStack stack = ((YourInventory) te).getStackInSlot(i), then either compare the items in it OR compare to a new ItemStack using the stack1.isItemEqual(stack2): ItemStack stack2 = new ItemStack(Item.whateverYouWantToCheckFor); 1. if (stack1.getItem() == stack2.getItem()) // you'll want to null check for stack1 if it is capable of being null 2. if (stack1.isItemEqual(stack2)) // again, null check 3. if (ItemStack.areItemStacksEqual(stack1, stack2)) // null check is inherent in the method Of course, in case number 1, you really don't need to create a new itemstack at all, just compare the items directly: if (stack != null && stack.getItem() == Item.whateverYouWant) -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Nope, don't think so, but that means the item IS getting set, but the container is not updating because you aren't using the Container to set the contents, you are doing it manually. This means you must manually tell the client about it as well - try overriding the getDescriptionPacket method in tile entity and you may get lucky; if not, you'll have to send a packet back to the client yourself (you can use the exact same packet) to have it update the contents on the client side, or (don't kill me diesieben!) you could 'set' the contents directly from the gui when you send the packet, as the contents will update automatically anyway the next time you open it. That's not ideal, though, as if other players are using your container, they will never know about the contents you set, so if you care about other players seeing what you just put in your tile entity, you'll have to use PacketDispatcher.sendToAllAround with a range of about 64 (squared, so 8 not squared) to make sure anyone else that might be using your inventory are made aware of the changes. That's sent from the server, of course, to the player clients. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
That's all looking like it should as well. What happens if you increase the inventory stack max size from 1 to 64? Vanilla's slotClick method is a bit broken when it comes to stack sizes of 1. Here's a rewrite of that method that I wrote for my own use to stop the inventory from having weird ghost stacks and such behavior: That goes in the Container class. Who knows, maybe that's your issue? -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Alright, that looks fine, too. If you put a println in your setInventorySlotContents method and print the stack, I'm sure you'll see it's correct. What happens if you press your button, close the inventory, and open it back up again? Is there an apple inside? Whether or not there is, it sounds like your problem is going to be in the Container class - are you using a Container? You may want to change the following to return true, even though you are not using it directly: @Override public boolean isItemValidForSlot(int i, ItemStack itemstack) { // TODO Auto-generated method stub return false; } -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Hm, well that looks okay actually. In your packet handler, you should remove the "public int posX, posY, posZ" fields and just use local integers in the method itself, as you don't really need them as class fields and certainly not public; it would be really bad if while you were handling the packet somewhere else in your code changed the values. Anyway, it looks like your packets should work, so the problem is likely to be in your TileEntity class. Mind posting that? -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
What about the code where you send the packet? Not just the part where you write the packet the entire code, because when I see stuff like "TileEntityRecordingUnit te = new TileEntityRecordingUnit();" in your packet handler, it really makes me wonder. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
This is super basic Java - you have to pass the parameter yourself. Look at your method: // you are passing the packet "par1", why not pass the player too? this.handlePacketData(par1); // here's your method, just add ", EntityPlayer player" inside it private void handlePacketData(Packet250CustomPayload par1) // now those two lines look like this // (after renaming your parameters to meaningful names, which you should always do) handlePacketData(packet, (EntityPlayer) player); private void handlePacketData(Packet250CustomPayload packet, EntityPlayer player) { // voila, you now have access to the server side player and therefore world objects TileEntity te = player.worldObj.getBlockTileEntity(x, y, z); // etc. } Please take some time, head over to Oracle's website, the New Boston, or wherever, and brush up on / learn more about Java. You will save yourself a ton of time and frustration that way.