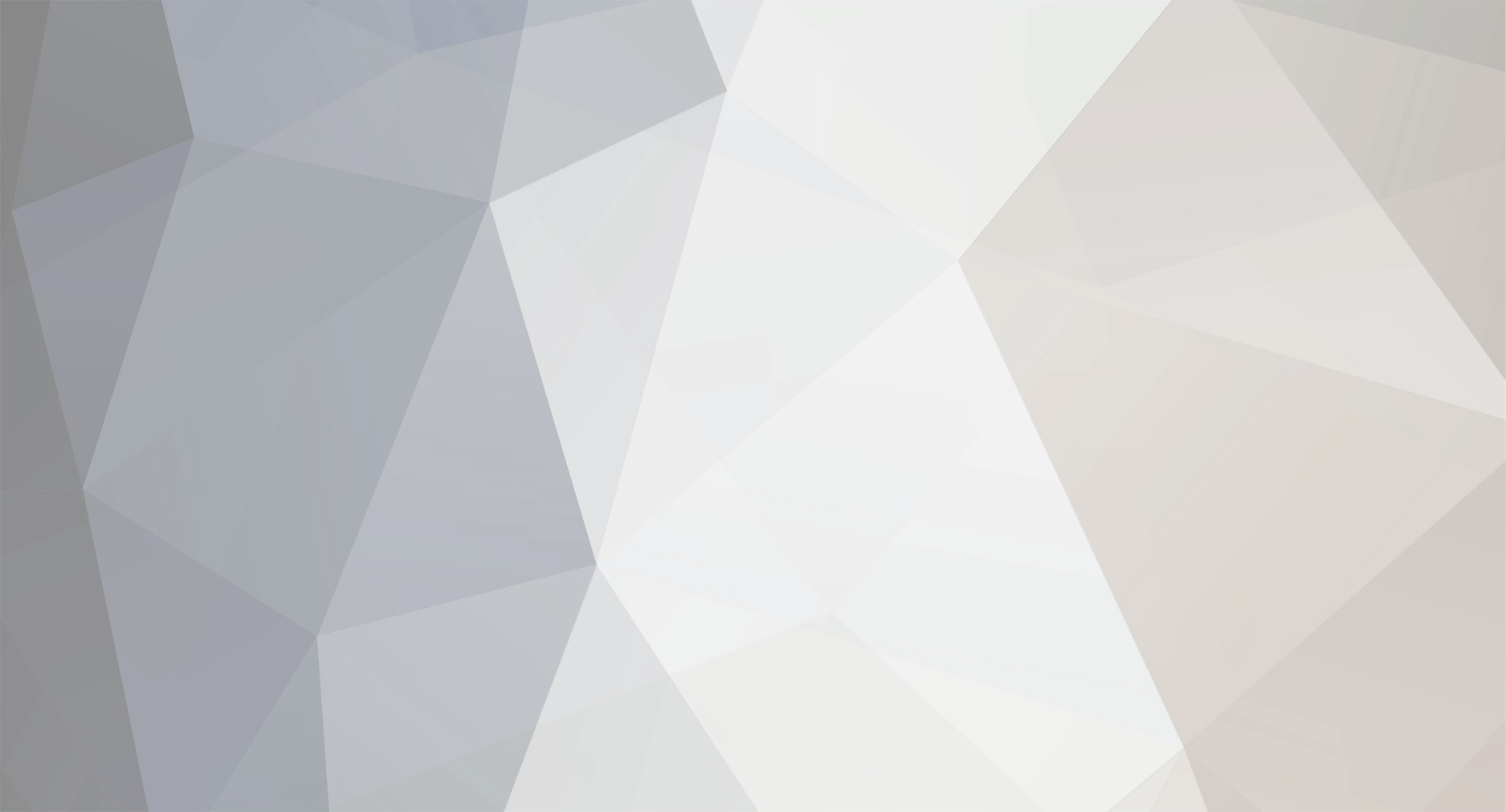
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
I've been trying for a while trying to figure out a way to get mobs to completely ignore the player that doesn't involve using capabilities.disableDamage or invisibility. LivingSetAttackTargetEvent doesn't cut it as that isn't posted each time a target is acquired in the updateEntityActionState method, so just as a test I searched for mobs near the player every tick and manually set their attack target to null - this just causes them to stutter-step towards the player very slowly, and eventually attack. Has anyone done anything like this before and if so, how did you accomplish it?
-
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
You need to do something like this: // notice you have a Player object in the parameters no matter what side you are on // that's your ticket to the world... public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { // packet received // make sure it's the packet you want // read in your data: item id, tile entity x, y, z TileEntity te = player.worldObj.getTileEntity(x, y, z); if (te instanceof YourTileEntity) { ((YourTileEntity) te).setInventorySlotContents(0, new ItemStack(itemID,1,0)); } } If you are still having problems after this, please post your packet handler code, and the code where you create and send the packet. -
[1.7.2] What ever happened to the ICraftingHandler?
coolAlias replied to Mitchellbrine's topic in Modder Support
Derp... sorry, the PlayerEvent is an FML event, my bad. You have to register it like this: FMLCommonHandler.instance().bus().register(new CraftingHandler()); EDIT: It's confusing, because there are multiple PlayerEvents, one for FML, and one for MinecraftForge, so you have to double-check the import you are using and subscribe based on that import. The FML PlayerEvents contain most of what used to be in IPlayerTracker, plus item crafting and smelting events. -
[1.7.2] What ever happened to the ICraftingHandler?
coolAlias replied to Mitchellbrine's topic in Modder Support
Post your code then, otherwise we're just guessing. Is your method public? If not, that's why, as the event bus can't access your method if it's not both public and void. -
[1.7.2] What ever happened to the ICraftingHandler?
coolAlias replied to Mitchellbrine's topic in Modder Support
You have to register the class containing the @SubscribeEvent method to the event bus; PlayerEvents happen on the MinecraftForge.EVENT_BUS: // typically somewhere in the main class, such as the load method: MinecraftForge.EVENT_BUS.register(new YourClassWithTheEvent()); -
Ah, sorry, I misunderstood your post. Yeah, what he said ^^
-
As long as you get the ID from the registry in the first place, it should be safe to store that for example in an NBT tag, since that's exactly what happens when you write an ItemStack to NBT anyway. The game will refuse to load or at least give you warning messages if any of the items / blocks have changed from the id to string mappings that get stored with the world save. Otherwise, how do you think Minecraft is storing the data itself? Certainly not as complex objects, and it wouldn't make any sense if the ids that were stored in your world save meant nothing the next time you load, as all the items and blocks in the game would be corrupt.
-
They are very unlikely to remove IDs; integers are just too handy of a storage method to get rid of entirely, though most users will never know they are there anymore. That was the point of moving to a registration system, but the game itself still tracks everything by ID, so you should be safe doing so as well.
-
Blocks and Items lists have been moved to the net/minecraft/init package and renamed to "Blocks" and "Items"; type "Items" followed by period "." and you'll get a list of all the items available.
-
This is calling a method: Tutorial.celluloseBlock() This is referencing a static object: Tutorial.celluloseBlock If you don't understand the difference between that, then please go here and learn more about Java. I understand you are frustrated, but on the other hand, please understand that creating a Block is probably one of the most basic things you can do in a mod. Most 1.7 tutorials are about updating from 1.6 to 1.7, so if you don't understand how to create a basic block, I recommend you start off by modding a little for 1.6 first, just to get the hang of it, and then port your code over to 1.7 later on when you are more comfortable with not only Java, but Minecraft code in general.
-
I may be wrong, but in 1.7 and up it shouldn't really be possible to get a null reference to a block anymore (unless you are working with world gen and have the possibility of not-yet loaded chunks), since air blocks are real blocks now. That being said, it doesn't hurt to check
-
[Solved] Why is this value desyncronized? [1.6.4]
coolAlias replied to larsgerrits's topic in Modder Support
You can pretty much literally just copy/paste the code from this tutorial (read the tutorial of course, so you know what's going on and where to register your packets and such). Then all you do is create your packet class and fill out the methods. Done. It's really simple once you get familiar with it. EDIT: Sorry, that tutorial is for 1.7.2 - didn't realize you were coding for 1.6.4 at the time. -
[Solved] Why is this value desyncronized? [1.6.4]
coolAlias replied to larsgerrits's topic in Modder Support
getDescriptionPacket is for updating the client tile entity with the data from the server, not the other way around (pretty sure about this - anyone correct me if I'm wrong). You're going to have to send a packet from your Gui when you click a button telling the server which button was clicked (or whatever data you want to send) and let the server process that information. -
Wow, no wonder it wasn't working for me, I was trying to add the @Optional directly...<facepalm> Thanks so much for explaining that. This will be extremely useful EDIT: Though this still doesn't prevent me from crashing when I try to run the client in Eclipse with BG2 as a referenced library... hmmm. One step at a time
-
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Right, you don't need all that code there unless you're trying to send it to different sides and you don't have anything more sophisticated set up to handle it for you. Instead of that super long bit with Minecraft.getMinecraft()..., why not just use "PacketDispatcher.sendPacketToServer(Packet packet);" ? It's much shorter when you don't have a player instance readily available. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
player.sendQueue.addToSendQueue(packet); PacketDispatcher.sendPacketToServer(Packet packet); Those are functionally equivalent, though I typically use the latter, for no particular reason. One may be more or less efficient than the other, I honestly have no idea, but they either one gets the job done. If you're using a custom method to send the packet, you need to pass in an EntityPlayer to your method parameters or you have no way of sending the packet -> EXCEPT, you are on the client, right? Remember I told you never to use Minecraft.getMinecraft()? Well, you CAN use it on the client, so if you have no other way of getting a player object to your custom method - really, if you can, just pass a player in - if you have NO other way, then you can use this ON THE CLIENT ONLY (excuse the excessive caps, but people seem to get confused about this): // this gives you the client side EntityPlayer object // (actually EntityClientPlayerMP, but that can be used just like EntityPlayer) Minecraft.getMinecraft().thePlayer Remember, you can only do that on the client side, and it's better to pass in the player to your method if you can from an existing player object. -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
Minecraft.getMinecraft() ALWAYS returns the CLIENT world. Never use that unless you know for a fact that you are on the client side, such as in a gui. I see now what you are asking, you're not sure what side you are on when you receive the packet, correct? That's determined by how you send the packet and parsed automatically by your packet handler, so if you send the packet to the server, you know it's received on the server. You mentioned you read the packet handling tutorial, but I think you ought to read it again more carefully; the section titled "Sending the Packet" explicitly covers how to send a packet to the client or server. Most packets are only used one-way, so you don't have to bother checking which side you are on, but if you are sending the same packet type to both sides at different times, then you can use the Player variable that is passed in to the packet handler to determine what side you are on, the same way you do everywhere else: if (player.worldObj.isRemote) { // you're on the client } else { // if (!player.worldObj.isRemote) <-- notice the "!" there // you're on the server } -
[1.6.4]Creating New ItemStack In Gui Slot.
coolAlias replied to TheMCJavaFre4k's topic in Modder Support
I'm not sure what you mean... use if (!world.isRemote) { print "we're on the server!" } ? or if (stack != null) { print "we made a stack!" } -
Thanks for that tutorial, Lex, it was extremely helpful. Quick question: are there specific naming conventions we should use for those of us who don't own a domain? The link seems to assume everyone owns a specific web domain, unless I'm totally off about the net./org./com.user.modid.
-
if(getStackInSlot(3).isItemEqual(new ItemStack(SuperNova.titaniumIngots))){ return Speed = 350; } If getStackInSlot(3) is ever empty and your hasUpgrade method gets called, that line will throw null because you are trying to access the isItemEqual method with a null object. Also, you don't need to create a new ItemStack just for this comparison, because the way you create it the stack has size 1, damage 0, and no NBT, which means you only need to compare the Item: ItemStack stack = getStackInSlot(3); // null check to avoid crashing, then compare the item if (stack != null && stack.getItem() == SuperNova.titaniumIngots) { return 350; }
-
I don't suppose you could provide a short example of how the annotation would look above a class? Whenever I try to add it, I get an error saying I need to fix my project setup because it can't find Optional, and even manually importing the cpw.mods.fml.common package doesn't resolve the issue. The java docs in the Optional class itself weren't enough for me to make sense of what the syntax ought to be. Sorry if this is considered basic Java, but it's not something I'm familiar with. Thanks for the help!
-
"Dependency" in this case does not mean that your mod requires the other mod, it's a field in the mcmod.info file that FML uses to determine load order, so if your mod is "dependent" i.e. capable of using stuff from another mod, then FML will be sure to load that mod first; this ensures that Loader.isModLoaded will work correctly when it comes your mod's turn to load. On a side note, the method I described works fine even for classes that implement / extend a class in another mod, so long as you do not use that class anywhere in your mod when the other mod is not loaded. For instance, I have a sword class for my Zelda swords, and I wanted them compatible with BattleGear2 dual-wielding, so this is what I did: // create a second class extending my swords: public class ItemZeldaSwordBG2 extends ItemZeldaSword implements IBattlegearWeapon { // super constructor plus BG2 interface implementation only } // then when I declare my items: if (Loader.isModLoaded("battlegear2")) { swordKokiri = new ItemZeldaSwordBG2(blah blah blah); } else { swordKokiri = new ItemZeldaSword(blah blah blah); } In this way my mod works fine with or without BattleGear2, but if BG2 is installed alongside, it will use the correct class that interfaces with BG2. It seems wasteful to have 2 classes for the same Item, but I couldn't figure out any way to add the interface and have the mod still work independently.
-
[1.6.4][SOLVED]Armor not giving player wearing it potion effects
coolAlias replied to The_Fireplace's topic in Modder Support
I'm pretty sure you've got the armor slots backwards; the vanilla java docs on this are very confusing, but this is what I've come up with after testing quite a bit: // this is what the java docs will tell you, but it's for rendering position only: Armor types as used in armor class: 0 helm, 1 chest, 2 legs, 3 boots // this is what is actually used for storing the positions in the player inventory: Armor types as used on player: 0 boots, 1 legs, 2 chest, 3 helm Use the second set of values with getCurrentArmor or add one for getCurrentItemOrArmor -
A simpler, but perhaps less elegant solution: // in your main mod class: public static boolean isOtherModLoaded; // in PreInit, initialize the field: isOtherModLoaded = Loader.isModLoaded("other_mod_id"); // then whenever you want to do something that relates to that other mod, // just check if it's loaded: if (isOtherModLoaded) { // do your stuff }
-
That depends on the TickEvent, of course: ServerTickEvent: obviously server only ClientTickEvent: again, obviously client only WorldTickEvent: both sides PlayerTickEvent: both sides RenderTickEvent: client only Don't just subscribe to "TickEvent", as that will listen to every single one of those tick types, just use the one that you need.