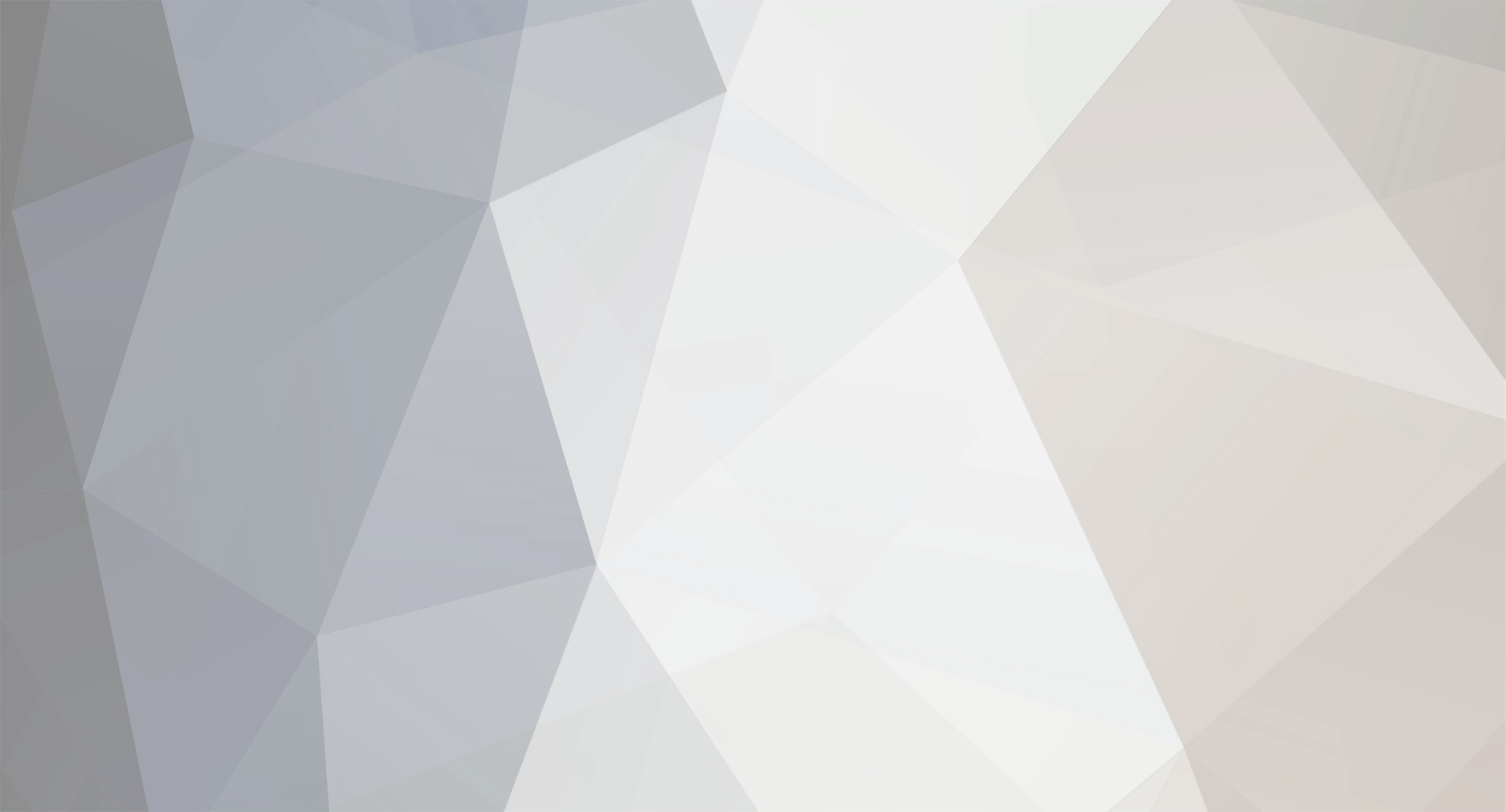
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
PlayerInteractEvent gets called twice, once on the client, once on the server. Just nest your chat messages in an if (world is or is not remote) statement. Done.
-
Have you not set up your network code yet? Try checking the wiki. That's the tutorial / code I used to get my network going in 1.7.2.
-
How to set area on fire when cooking apple in furnace
coolAlias replied to SkillCakey's topic in Modder Support
If you know where the furnace is, you can get the tile entity from the world. TileEntityFurnace implements ISidedInventory, so you have all the inventory methods available to you to find out what is in the furnace slot. TileEntity te = world.getBlockTileEntity(x, y, z); if (te instanceof TileEntityFurnace) { TileEntityFurnace furnace = (TileEntityFurnace) te; // now that you've got the furnace, you can go to town on the inventory } } -
I was under the impression that @Interface(iface="mods.external_mod.api.ISomeInterface", modid="modid", striprefs = true) would strip the interface during run-time if not found. Of course I understand it will throw that error if I don't include the api source, but the whole point is to not require it alongside my mod yet still be able to implement features in the case that the two mods are installed together. Previously I accomplished this by having a second class, MyClass2 extends MyClass1 implements IOtherModInterface, and the only thing it did was implement those interface methods. Then I would assign my items to MyClass2 if the other mod was loaded, otherwise assign them to MyClass1, but I was hoping to find some way around that. I'd appreciate more constructive comments in the future. Cheers.
-
That depends on how you set up your packets. If you set it up using a similar system of AbstractPackets, it's not that outdated at all. In fact, it's simply a matter of changing the streams to buffers and a few method names.
-
That's not odd at all. The UseHoeEvent is being called on both sides. ItemPickupEvent ONLY fires on the server. Ever. If you're ever curious whether an event or method of any kind is being called on both sides, place a println inside of it: System.out.println("Is client? " + worldObj.isRemote); Of course, getting the world object however you can, directly from the method parameters if you can or from an entity i.e. event.entity.worldObj. Run your mod and try to run that method, then check the console output. Try it with UseHoeEvent and I'll bet you whatever you want that it will print like this: Is client? true // client, yay particles Is client? false // server, boo, no particles Is client? true Is client? false I'm not 100% sure, but the UseHoeEvent may only fire for the player actually using the hoe, in which case your particles still will not be seen by other players unless you send a packet. Whether you care enough about those particular particles is of course up to you, but it's something to be aware of.
-
TGG is correct, that event only fires on the server, never on the client. You will need to create a packet to send to the client (or all clients within a certain distance if you want other players to see the particles as well) in order to spawn the particles. You can see an example particle packet of mine here. It's for 1.6.4, but it's simple to port over to 1.7.2. One of these days I'll get my 1.7.2 version up on git as well...
-
I'm using another mod as a referenced library / dependency, and it works fine when run in the development environment. However, after compiling, if I try to run Minecraft with only my mod loaded, the following MyClass throws a no class definition found error, as does the external mod interface class. It of course works fine when run with the other mod also installed. I'm not very familiar with the following annotations, but I was under the impression that it should strip the interface during run-time if the other mod is not found, but leave the rest of the class intact. This is the only class in which I reference this external interface. // The errors: Caused by: java.lang.NoClassDefFoundError: modid/package/MyClass Caused by: java.lang.ClassNotFoundException: mods.external_mod.api.package.IOtherModInterface @Interface(iface="mods.external_mod.api.ISomeInterface", modid="modid", striprefs = true) public class MyClass extends VanillaClass implements IOtherModInterface, IMyInterfaces { // my code // external mod API methods @Method(modid="external_modid") @Override public void someMethod() {} Thanks for whatever help you can give.
-
Try watching vswe's courses on interfaces. They aren't for 1.7.2 specifically, but almost all of the information is still valid and you will learn a lot that should help you.
-
Creating Dragon-proof blocks in Forge for 1.6.4 [Solved]
coolAlias replied to DragonGodGrapha's topic in Modder Support
I haven't tested this specifically with the Ender Dragon, but I'm pretty sure this is what you're looking for: // in your Block class, add this method: @Override public boolean canEntityDestroy(World world, int x, int y, int z, Entity entity) { return false; } -
Solved: Using missing texture, unable to load:
coolAlias replied to TheGreyGhost's topic in Modder Support
I believe the default location is the workspace directory, where build.gradle is located. Assets of course follow the format mentioned above, but resources that are not prefixed with "modid:" / (modid, ...) need to be specified in relation to the base working directory. -
[Solved] [1.7.2] Is there a ender pearl throw event?
coolAlias replied to MinecraftMart's topic in Modder Support
Chill guys, he's just a kid. Don't you remember what it was like when you first started coding? Everyone has to start somewhere. @OP: That being said, you would do yourself a huge favor by stopping what you're doing and spending some time learning Java or any other language. Try the New Boston for video tutorials, and / or spend some time browsing through the tutorials on Oracle's website. Regarding your problem, follow the steps I mentioned earlier: // get the held ItemStack (you may need to substitute 'player' with 'event.player' or whatever: ItemStack stack = player.getHeldItem(); // check if it's not null and the item contained therein is an ender pearl: if (stack != null && stack.getItem() == Items.ender_pearl) { // increment your counter or whatever else you want to do } This is very basic Java / IDE use, and as has been stated, this is not a Java help forum. Good luck. -
I'm not sure what exactly is wrong, but I think it has to do with you registering creative tabs for your ItemBlock, as well as setting texture names all over the place. Here is a class I use for my ItemBlocks with blocks that have metadata. Keep in mind that this is the entire class: public class ItemMetadataBlock extends ItemBlockWithMetadata { public ItemMetadataBlock(Block block) { super(block, block); } } Yes, just that. Here's how I register a block to use that class: // this is all for creating the block, since I set the tab and register several icons in the block class pedestal = new BlockPedestal().setBlockName("zss.pedestal"); GameRegistry.registerBlock(pedestal, ItemMetadataBlock.class, pedestal.getUnlocalizedName()); It uses the icons from the block class, so whatever texture you set / register for your block, that is what will render in the inventory. Likewise, whatever Creative Tab you set for your block in the block class is the one that will be used. Simple as those few lines. If your block doesn't use metadata, you could probably just use the vanilla ItemBlock class. ItemBlockWithMetadata doesn't have a single Block argument constructor, however, so it will crash the game, which is why I made that wrapper class above.
-
Those were the duplicate methods =.= Look: @Override public boolean canInsertItem(int i, ItemStack itemstack, int j) { // TODO Auto-generated method stub return false; } @Override public boolean canExtractItem(int i, ItemStack itemstack, int j) { // TODO Auto-generated method stub return false; } ALWAYS avoid func_21831_d named variables and methods UNLESS you are absolutely required to use them (methods only) when overriding a method from the parent class. And even then, you need to be careful, or you end up in a situation like this.
-
[Solved] [1.7.2] Is there a ender pearl throw event?
coolAlias replied to MinecraftMart's topic in Modder Support
event.entityPlayer gives you the player, right? What methods are there in EntityPlayer that give you the item the player is holding? There are at least 2, but the easiest one is getHeldItem(), which returns an ItemStack or null if the player is empty-handed. So the steps look like this: 1. Get the ItemStack the player is holding 2. Check if it's null 3. If it's not null, check that the item in the ItemStack is an Ender Pearl 4. Increment the counter for your player But as diesieben already pointed out, you are incrementing a single counter for all players, rather than a counter for each player. Perhaps you can tackle that another day after you have more Java under your belt, but if you're feeling adventurous, look into IExtendedEntityProperties. -
No, it's caused by this: Caused by: java.lang.ClassFormatError: Duplicate method name&signature in class file heitagetileentity/TileEntityTransmutation You need to double-check that none of your methods are duplicates; while you're at it, why don't you get rid of all those nasty func_32934_a names?
-
[SOLVED] Problem with items with subtypes
coolAlias replied to Bedrock_Miner's topic in Modder Support
Did you add "setHasSubtypes(true);" ? If you did, then post your code or we're just shooting in the dark here. -
[Solved] [1.7.2] Is there a ender pearl throw event?
coolAlias replied to MinecraftMart's topic in Modder Support
You have to import the class. Press "ctrl-shift-o" if you are using Eclipse, it will automatically import what you need. Or hover your mouse over "Action" and see that Eclipse gives you some ideas of how to fix what's wrong, one of which should be to import the class. -
[Solved] [1.7.2] Is there a ender pearl throw event?
coolAlias replied to MinecraftMart's topic in Modder Support
"if (p.action.RIGHT_CLICK_BLOCK != null)" ... <cough>, umm, that's not how enumerations are typically checked. I prefer to use a switch, but you can use if statements as well: if (event.action == Action.RIGHT_CLICK_AIR) { // do stuff } Look at the import for PlayerInteractEvent and you'll find out which event bus to register it with. import net.minecraftforge.event.entity.player.PlayerInteractEvent; -
In Eclipse, right-click your project, go to "build path -> configure build path" and then click on the "libraries" tab. From there, click "add external jars" and navigate to the folder where you placed CodeChickenCore or whatever library you are trying to use. If you also have the src file, you can then go to the "referenced libraries" heading in your package hierarchy (left-hand pane, usually), find the library you added, right-click it, go to "properties" and then select "external source location" and again navigate to the folder with the src code. That is of course optional, but I like to be able to see implementations for things that I'm using if need be.
-
[Solved] [1.7.2] Is there a ender pearl throw event?
coolAlias replied to MinecraftMart's topic in Modder Support
EnderTeleportEvent gets posted when the actual teleportation is about to occur, and EntityJoinWorldEvent is posted when the EntityEnderPearl is spawned, but if you want to intercept it just as it is about to be thrown, then the event mentioned by diesieben07 is your best bet. -
[1.6.4] Problems with ResourceLocation [coolAlias' tutorial]
coolAlias replied to Ablaze's topic in Modder Support
Yes, the launch configurations are the same. No, I'm not going to explain it, as Lex already did so much better than I could myself. Your problem isn't at all related to my tutorial, it's caused by your folder structure / workspace being set up incorrectly. -
[1.6.4] Problems with ResourceLocation [coolAlias' tutorial]
coolAlias replied to Ablaze's topic in Modder Support
Please watch LexManos' on setting up your workspace. It should look like: /modding/SomeMod/src/main/java | resources /modding/SomeOtherMod/src/main/java | resources There is no longer any reason to use a forge or eclipse folder as your workspace. Create a new workspace and import your project directly. -
[1.6.4] Problems with ResourceLocation [coolAlias' tutorial]
coolAlias replied to Ablaze's topic in Modder Support
The structure of your assets folder should not change from Python to Gradle, but the assets folder is now located in src/main/resources/ |__assets/modid/textures/ etc. ModInfo.ID, "knowledgebar.png" tells it to look in assets/modid/ for knowledgebar.png, so you should use ModInfo.ID, "textures/whateverfolderforGUIs/knowledgebar.png" The syntax for ResourceLocation is exactly the same as it always has been.